diff --git a/src/miniprogram-4/.DS_Store b/src/miniprogram-4/.DS_Store
new file mode 100644
index 0000000..17eb537
Binary files /dev/null and b/src/miniprogram-4/.DS_Store differ
diff --git a/src/miniprogram-4/.eslintrc.js b/src/miniprogram-4/.eslintrc.js
new file mode 100644
index 0000000..115cc02
--- /dev/null
+++ b/src/miniprogram-4/.eslintrc.js
@@ -0,0 +1,31 @@
+/*
+ * Eslint config file
+ * Documentation: https://eslint.org/docs/user-guide/configuring/
+ * Install the Eslint extension before using this feature.
+ */
+module.exports = {
+ env: {
+ es6: true,
+ browser: true,
+ node: true,
+ },
+ ecmaFeatures: {
+ modules: true,
+ },
+ parserOptions: {
+ ecmaVersion: 2018,
+ sourceType: 'module',
+ },
+ globals: {
+ wx: true,
+ App: true,
+ Page: true,
+ getCurrentPages: true,
+ getApp: true,
+ Component: true,
+ requirePlugin: true,
+ requireMiniProgram: true,
+ },
+ // extends: 'eslint:recommended',
+ rules: {},
+}
diff --git a/src/miniprogram-4/Components/test1/test1.js b/src/miniprogram-4/Components/test1/test1.js
new file mode 100644
index 0000000..5a7ef5a
--- /dev/null
+++ b/src/miniprogram-4/Components/test1/test1.js
@@ -0,0 +1,79 @@
+// Components/test1/test1.js
+Component({
+
+ /**
+ * 组件的属性列表
+ */
+ properties: {
+ // 1、简化方式
+ // max: Number
+ // 2、完整方式
+ max: {
+ type: Number,
+ // 默认值
+ value: 10
+ },
+ num: {
+ type: Number,
+ value: 0
+ }
+ },
+
+ /**
+ * 组件的初始数据
+ */
+ data: {
+ count: 0,
+ n1: 1,
+ n2: 2,
+ sum: 3
+ },
+ options: {
+ //纯数据字段
+ pureDataPattern: /^_/,
+ //支持多个插槽
+ multipleSlots: true
+ },
+ /**
+ * 组件的方法列表
+ */
+ methods: {
+ pre() {
+ this.setData({
+ count: this.data.count + 1
+ })
+ if(this.data.count == this.properties.max) {
+ wx.showToast({
+ title: '请求过于频繁',
+ icon: 'error',
+ duration: 2000
+ })
+ this.setData({
+ count: 0
+ })
+ }
+ else {
+ wx.showLoading({
+ title: '预测中',
+ duration: 2000
+ })
+ }
+ },
+ // addN1() {
+ // this.setData({
+ // n1: this.data.n1 + 1
+ // })
+ // },
+ // addN2() {
+ // this.setData({
+ // n2: this.data.n2 + 1
+ // })
+ // }
+ },
+ // 数据监听
+ // observers: {
+ // 'n1, n2': function(n1, n2) {
+ // this.setData({sum: n1 + n2})
+ // }
+ // }
+})
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test1/test1.json b/src/miniprogram-4/Components/test1/test1.json
new file mode 100644
index 0000000..e8cfaaf
--- /dev/null
+++ b/src/miniprogram-4/Components/test1/test1.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test1/test1.wxml b/src/miniprogram-4/Components/test1/test1.wxml
new file mode 100644
index 0000000..5f4123a
--- /dev/null
+++ b/src/miniprogram-4/Components/test1/test1.wxml
@@ -0,0 +1,12 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/Components/test1/test1.wxss b/src/miniprogram-4/Components/test1/test1.wxss
new file mode 100644
index 0000000..94208e6
--- /dev/null
+++ b/src/miniprogram-4/Components/test1/test1.wxss
@@ -0,0 +1,8 @@
+/* Components/test1/test1.wxss */
+.pre {
+ box-shadow: 0px 0px 5px 1px rgba(0, 0, 0, 0.3);
+}
+.plus {
+ display: flex;
+ /* justify-content: space-evenly; */
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test2/test2.js b/src/miniprogram-4/Components/test2/test2.js
new file mode 100644
index 0000000..5c7ede6
--- /dev/null
+++ b/src/miniprogram-4/Components/test2/test2.js
@@ -0,0 +1,24 @@
+// Components/test2/test2.js
+Component({
+
+ /**
+ * 组件的属性列表
+ */
+ properties: {
+
+ },
+
+ /**
+ * 组件的初始数据
+ */
+ data: {
+
+ },
+
+ /**
+ * 组件的方法列表
+ */
+ methods: {
+
+ }
+})
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test2/test2.json b/src/miniprogram-4/Components/test2/test2.json
new file mode 100644
index 0000000..e8cfaaf
--- /dev/null
+++ b/src/miniprogram-4/Components/test2/test2.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test2/test2.wxml b/src/miniprogram-4/Components/test2/test2.wxml
new file mode 100644
index 0000000..4bf16ee
--- /dev/null
+++ b/src/miniprogram-4/Components/test2/test2.wxml
@@ -0,0 +1,2 @@
+
+Components/test2/test2.wxml
\ No newline at end of file
diff --git a/src/miniprogram-4/Components/test2/test2.wxss b/src/miniprogram-4/Components/test2/test2.wxss
new file mode 100644
index 0000000..7c85d08
--- /dev/null
+++ b/src/miniprogram-4/Components/test2/test2.wxss
@@ -0,0 +1 @@
+/* Components/test2/test2.wxss */
\ No newline at end of file
diff --git a/src/miniprogram-4/README.md b/src/miniprogram-4/README.md
new file mode 100644
index 0000000..fe80a36
--- /dev/null
+++ b/src/miniprogram-4/README.md
@@ -0,0 +1,2 @@
+# 111
+it's a test demo
diff --git a/src/miniprogram-4/app.js b/src/miniprogram-4/app.js
new file mode 100644
index 0000000..7e2bcea
--- /dev/null
+++ b/src/miniprogram-4/app.js
@@ -0,0 +1,29 @@
+// app.js
+
+
+wx.navigateTo({
+ url: '/pages/login/login',
+})
+
+App({
+ onLaunch() {
+ // 展示本地存储能力
+ const logs = wx.getStorageSync('logs') || []
+ logs.unshift(Date.now())
+ wx.setStorageSync('logs', logs)
+
+ // 登录
+ wx.login({
+ success: res => {
+ // 发送 res.code 到后台换取 openId, sessionKey, unionId
+ }
+ })
+ },
+ globalData: {
+ userInfo: {
+ avatarUrl: '',
+ nickName: 'hhh',
+ psw: '123456'
+ }
+ }
+})
diff --git a/src/miniprogram-4/app.json b/src/miniprogram-4/app.json
new file mode 100644
index 0000000..0de4d05
--- /dev/null
+++ b/src/miniprogram-4/app.json
@@ -0,0 +1,75 @@
+{
+ "usingComponents": {
+ "test2": "/Components/test2/test2",
+ "van-tabbar": "@vant/weapp/tabbar/index",
+ "van-tabbar-item": "@vant/weapp/tabbar-item/index"
+ },
+ "pages": [
+ "pages/index/index",
+ "pages/login/login",
+ "pages/list/list",
+ "pages/info/info",
+ "pages/prediction/prediction",
+ "pages/logs/logs",
+ "pages/map/map",
+ "pages/navigation_bus/navigation",
+ "pages/navigation_ride/navigation",
+ "pages/navigation_walk/navigation",
+ "pages/my/my",
+ "pages/register/register",
+ "pages/pswChange/pswChange",
+ "pages/innerLogin/innerLogin",
+ "pages/result/result",
+ "pages/predict/predict"
+ ],
+ "requiredPrivateInfos": [
+ "choosePoi"
+ ],
+ "window": {
+ "navigationBarTextStyle": "black",
+ "navigationBarTitleText": "预测通",
+ "navigationBarBackgroundColor": "#00b26a",
+ "backgroundColor": "#efefef",
+ "onReachBottomDistance": 50
+ },
+ "plugins": {
+ "routePlan": {
+ "version": "1.0.19",
+ "provider": "wx50b5593e81dd937a"
+ }
+ },
+ "permission": {
+ "scope.userLocation": {
+ "desc": "你的位置信息将用于小程序定位"
+ }
+ },
+ "tabBar": {
+ "custom": true,
+ "list": [
+ {
+ "pagePath": "pages/index/index",
+ "text": "首页",
+ "iconPath": "/image/tabBar/zhuye.png",
+ "selectedIconPath": "/image/tabBar/home-active.png"
+ },
+ {
+ "pagePath": "pages/list/list",
+ "text": "计划",
+ "iconPath": "/image/tabBar/list.png",
+ "selectedIconPath": "/image/tabBar/list-active-icon.png"
+ },
+ {
+ "pagePath": "pages/my/my",
+ "text": "我的",
+ "iconPath": "/image/tabBar/login_User.png",
+ "selectedIconPath": "/image/tabBar/my-active.png"
+ }
+ ],
+ "borderStyle": "black",
+ "backgroundColor": "#ffffff"
+ },
+ "style": "v2",
+ "componentFramework": "glass-easel",
+ "sitemapLocation": "sitemap.json",
+ "lazyCodeLoading": "requiredComponents"
+}
diff --git a/src/miniprogram-4/app.wxss b/src/miniprogram-4/app.wxss
new file mode 100644
index 0000000..9e1dba7
--- /dev/null
+++ b/src/miniprogram-4/app.wxss
@@ -0,0 +1,18 @@
+/**app.wxss**/
+view {
+ box-sizing: border-box;
+}
+.container {
+ height: 100%;
+ display: flex;
+ flex-direction: column;
+ align-items: center;
+ justify-content: space-between;
+ padding: 200rpx 0;
+ box-sizing: border-box;
+}
+
+/* 仅类选择器具有对组件的隔离 */
+.red-text {
+ color: red;
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/commom/common.wxss b/src/miniprogram-4/commom/common.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/custom-tab-bar/index.js b/src/miniprogram-4/custom-tab-bar/index.js
new file mode 100644
index 0000000..6733e89
--- /dev/null
+++ b/src/miniprogram-4/custom-tab-bar/index.js
@@ -0,0 +1,80 @@
+// custom-tab-bar/index.js
+import { storeBindingsBehavior } from 'mobx-miniprogram-bindings'
+import { store } from '../store/store'
+
+Component({
+
+ options: {
+ styleIsolation: 'shared'
+ },
+ behaviors: [storeBindingsBehavior],
+ storeBindings: {
+ store,
+ fields: {
+ num: 'num',
+ active: 'activeTabBar'
+ },
+ actions: {
+ updateActive: 'updateActiveTabBar',
+ updateNum: 'updateNum'
+ },
+ },
+ observers: {
+ 'num': function(val) {
+ // console.log(val)
+ this.setData({
+ 'list[1].info': val
+ })
+ }
+ },
+ /**
+ * 组件的属性列表
+ */
+ properties: {
+
+ },
+
+ /**
+ * 组件的初始数据
+ */
+ data: {
+ icon: {
+ normal: 'https://img.yzcdn.cn/vant/user-inactive.png',
+ active: 'https://img.yzcdn.cn/vant/user-active.png',
+ },
+ "list": [
+ {
+ "pagePath": "/pages/index/index",
+ "text": "首页",
+ "iconPath": "/image/tabBar/zhuye.png",
+ "selectedIconPath": "/image/tabBar/home-active.png"
+ },
+ {
+ "pagePath": "/pages/list/list",
+ "text": "计划",
+ "iconPath": "/image/tabBar/list.png",
+ "selectedIconPath": "/image/tabBar/list-active-icon.png",
+ info: 3
+ },
+ {
+ "pagePath": "/pages/my/my",
+ "text": "我的",
+ "iconPath": "/image/tabBar/login_User.png",
+ "selectedIconPath": "/image/tabBar/my-active.png"
+ }
+ ],
+ },
+
+ /**
+ * 组件的方法列表
+ */
+ methods: {
+ onChange(event) {
+ // this.setData({ active: event.detail });
+ this.updateActive(event.detail)
+ wx.switchTab({
+ url: this.data.list[event.detail].pagePath,
+ })
+ }
+ }
+})
\ No newline at end of file
diff --git a/src/miniprogram-4/custom-tab-bar/index.json b/src/miniprogram-4/custom-tab-bar/index.json
new file mode 100644
index 0000000..e8cfaaf
--- /dev/null
+++ b/src/miniprogram-4/custom-tab-bar/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/custom-tab-bar/index.wxml b/src/miniprogram-4/custom-tab-bar/index.wxml
new file mode 100644
index 0000000..79f9390
--- /dev/null
+++ b/src/miniprogram-4/custom-tab-bar/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+ {{item.text}}
+
+
diff --git a/src/miniprogram-4/custom-tab-bar/index.wxss b/src/miniprogram-4/custom-tab-bar/index.wxss
new file mode 100644
index 0000000..850314d
--- /dev/null
+++ b/src/miniprogram-4/custom-tab-bar/index.wxss
@@ -0,0 +1,4 @@
+/* custom-tab-bar/index.wxss */
+.van-tabbar-item{
+ --tabbar-item-margin-bottom: 0;
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/image/background.jpg b/src/miniprogram-4/image/background.jpg
new file mode 100644
index 0000000..36ebbe6
Binary files /dev/null and b/src/miniprogram-4/image/background.jpg differ
diff --git a/src/miniprogram-4/image/delete.png b/src/miniprogram-4/image/delete.png
new file mode 100644
index 0000000..bfda6cd
Binary files /dev/null and b/src/miniprogram-4/image/delete.png differ
diff --git a/src/miniprogram-4/image/lock.png b/src/miniprogram-4/image/lock.png
new file mode 100644
index 0000000..f6d0e63
Binary files /dev/null and b/src/miniprogram-4/image/lock.png differ
diff --git a/src/miniprogram-4/image/phone1.png b/src/miniprogram-4/image/phone1.png
new file mode 100644
index 0000000..041977c
Binary files /dev/null and b/src/miniprogram-4/image/phone1.png differ
diff --git a/src/miniprogram-4/image/picA.jpg b/src/miniprogram-4/image/picA.jpg
new file mode 100644
index 0000000..d8a717f
Binary files /dev/null and b/src/miniprogram-4/image/picA.jpg differ
diff --git a/src/miniprogram-4/image/picB.jpg b/src/miniprogram-4/image/picB.jpg
new file mode 100644
index 0000000..149209a
Binary files /dev/null and b/src/miniprogram-4/image/picB.jpg differ
diff --git a/src/miniprogram-4/image/tabBar/home-active.png b/src/miniprogram-4/image/tabBar/home-active.png
new file mode 100644
index 0000000..3d30722
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/home-active.png differ
diff --git a/src/miniprogram-4/image/tabBar/list-active-icon.png b/src/miniprogram-4/image/tabBar/list-active-icon.png
new file mode 100644
index 0000000..501f793
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/list-active-icon.png differ
diff --git a/src/miniprogram-4/image/tabBar/list.png b/src/miniprogram-4/image/tabBar/list.png
new file mode 100644
index 0000000..187513a
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/list.png differ
diff --git a/src/miniprogram-4/image/tabBar/login_User.png b/src/miniprogram-4/image/tabBar/login_User.png
new file mode 100644
index 0000000..2c4e019
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/login_User.png differ
diff --git a/src/miniprogram-4/image/tabBar/my-active.png b/src/miniprogram-4/image/tabBar/my-active.png
new file mode 100644
index 0000000..3d2f558
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/my-active.png differ
diff --git a/src/miniprogram-4/image/tabBar/zhuye.png b/src/miniprogram-4/image/tabBar/zhuye.png
new file mode 100644
index 0000000..0ec0a98
Binary files /dev/null and b/src/miniprogram-4/image/tabBar/zhuye.png differ
diff --git a/src/miniprogram-4/image/user.png b/src/miniprogram-4/image/user.png
new file mode 100644
index 0000000..bae877a
Binary files /dev/null and b/src/miniprogram-4/image/user.png differ
diff --git a/src/miniprogram-4/image/user2.png b/src/miniprogram-4/image/user2.png
new file mode 100644
index 0000000..3dd58de
Binary files /dev/null and b/src/miniprogram-4/image/user2.png differ
diff --git a/src/miniprogram-4/image/xiaochengxu-shanchu.png b/src/miniprogram-4/image/xiaochengxu-shanchu.png
new file mode 100644
index 0000000..8011d04
Binary files /dev/null and b/src/miniprogram-4/image/xiaochengxu-shanchu.png differ
diff --git a/src/miniprogram-4/miniprogram-4 b/src/miniprogram-4/miniprogram-4
deleted file mode 160000
index c6e5f32..0000000
--- a/src/miniprogram-4/miniprogram-4
+++ /dev/null
@@ -1 +0,0 @@
-Subproject commit c6e5f32ea2539d738f798733919e8328e27a5656
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.js
new file mode 100644
index 0000000..8403b68
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.js
@@ -0,0 +1,78 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+(0, component_1.VantComponent)({
+ classes: ['list-class'],
+ mixins: [button_1.button],
+ props: {
+ show: Boolean,
+ title: String,
+ cancelText: String,
+ description: String,
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ actions: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickAction: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onSelect: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var _a = this.data, actions = _a.actions, closeOnClickAction = _a.closeOnClickAction, canIUseGetUserProfile = _a.canIUseGetUserProfile;
+ var item = actions[index];
+ if (item) {
+ this.$emit('select', item);
+ if (closeOnClickAction) {
+ this.onClose();
+ }
+ if (item.openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: item.getUserProfileDesc || ' ',
+ complete: function (userProfile) {
+ _this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ }
+ },
+ onCancel: function () {
+ this.$emit('cancel');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ this.onClose();
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.json
new file mode 100644
index 0000000..19bf989
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-popup": "../popup/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxml
new file mode 100644
index 0000000..6311e33
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
+
+
+
+ {{ cancelText }}
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxss
new file mode 100644
index 0000000..eedd361
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/action-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-action-sheet{color:var(--action-sheet-item-text-color,#323233);max-height:var(--action-sheet-max-height,90%)!important}.van-action-sheet__cancel,.van-action-sheet__item{background-color:var(--action-sheet-item-background,#fff);font-size:var(--action-sheet-item-font-size,16px);line-height:var(--action-sheet-item-line-height,22px);padding:14px 16px;text-align:center}.van-action-sheet__cancel--hover,.van-action-sheet__item--hover{background-color:#f2f3f5}.van-action-sheet__cancel:after,.van-action-sheet__item:after{border-width:0}.van-action-sheet__cancel{color:var(--action-sheet-cancel-text-color,#646566)}.van-action-sheet__gap{background-color:var(--action-sheet-cancel-padding-color,#f7f8fa);display:block;height:var(--action-sheet-cancel-padding-top,8px)}.van-action-sheet__item--disabled{color:var(--action-sheet-item-disabled-text-color,#c8c9cc)}.van-action-sheet__item--disabled.van-action-sheet__item--hover{background-color:var(--action-sheet-item-background,#fff)}.van-action-sheet__subname{color:var(--action-sheet-subname-color,#969799);font-size:var(--action-sheet-subname-font-size,12px);line-height:var(--action-sheet-subname-line-height,20px);margin-top:var(--padding-xs,8px)}.van-action-sheet__header{font-size:var(--action-sheet-header-font-size,16px);font-weight:var(--font-weight-bold,500);line-height:var(--action-sheet-header-height,48px);text-align:center}.van-action-sheet__description{color:var(--action-sheet-description-color,#969799);font-size:var(--action-sheet-description-font-size,14px);line-height:var(--action-sheet-description-line-height,20px);padding:20px var(--padding-md,16px);text-align:center}.van-action-sheet__close{color:var(--action-sheet-close-icon-color,#c8c9cc);font-size:var(--action-sheet-close-icon-size,22px)!important;line-height:inherit!important;padding:var(--action-sheet-close-icon-padding,0 16px);position:absolute!important;right:0;top:0}.van-action-sheet__loading{display:flex!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.js
new file mode 100644
index 0000000..73de66d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.js
@@ -0,0 +1,235 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var shared_1 = require("../picker/shared");
+var utils_1 = require("../common/utils");
+var EMPTY_CODE = '000000';
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { showToolbar: {
+ type: Boolean,
+ value: true,
+ }, value: {
+ type: String,
+ observer: function (value) {
+ this.code = value;
+ this.setValues();
+ },
+ }, areaList: {
+ type: Object,
+ value: {},
+ observer: 'setValues',
+ }, columnsNum: {
+ type: null,
+ value: 3,
+ }, columnsPlaceholder: {
+ type: Array,
+ observer: function (val) {
+ this.setData({
+ typeToColumnsPlaceholder: {
+ province: val[0] || '',
+ city: val[1] || '',
+ county: val[2] || '',
+ },
+ });
+ },
+ } }),
+ data: {
+ columns: [{ values: [] }, { values: [] }, { values: [] }],
+ typeToColumnsPlaceholder: {},
+ },
+ mounted: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.setValues();
+ });
+ },
+ methods: {
+ getPicker: function () {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-area__picker');
+ }
+ return this.picker;
+ },
+ onCancel: function (event) {
+ this.emit('cancel', event.detail);
+ },
+ onConfirm: function (event) {
+ var index = event.detail.index;
+ var value = event.detail.value;
+ value = this.parseValues(value);
+ this.emit('confirm', { value: value, index: index });
+ },
+ emit: function (type, detail) {
+ detail.values = detail.value;
+ delete detail.value;
+ this.$emit(type, detail);
+ },
+ parseValues: function (values) {
+ var columnsPlaceholder = this.data.columnsPlaceholder;
+ return values.map(function (value, index) {
+ if (value &&
+ (!value.code || value.name === columnsPlaceholder[index])) {
+ return __assign(__assign({}, value), { code: '', name: '' });
+ }
+ return value;
+ });
+ },
+ onChange: function (event) {
+ var _this = this;
+ var _a;
+ var _b = event.detail, index = _b.index, picker = _b.picker, value = _b.value;
+ this.code = value[index].code;
+ (_a = this.setValues()) === null || _a === void 0 ? void 0 : _a.then(function () {
+ _this.$emit('change', {
+ picker: picker,
+ values: _this.parseValues(picker.getValues()),
+ index: index,
+ });
+ });
+ },
+ getConfig: function (type) {
+ var areaList = this.data.areaList;
+ return (areaList && areaList["".concat(type, "_list")]) || {};
+ },
+ getList: function (type, code) {
+ if (type !== 'province' && !code) {
+ return [];
+ }
+ var typeToColumnsPlaceholder = this.data.typeToColumnsPlaceholder;
+ var list = this.getConfig(type);
+ var result = Object.keys(list).map(function (code) { return ({
+ code: code,
+ name: list[code],
+ }); });
+ if (code != null) {
+ // oversea code
+ if (code[0] === '9' && type === 'city') {
+ code = '9';
+ }
+ result = result.filter(function (item) { return item.code.indexOf(code) === 0; });
+ }
+ if (typeToColumnsPlaceholder[type] && result.length) {
+ // set columns placeholder
+ var codeFill = type === 'province'
+ ? ''
+ : type === 'city'
+ ? EMPTY_CODE.slice(2, 4)
+ : EMPTY_CODE.slice(4, 6);
+ result.unshift({
+ code: "".concat(code).concat(codeFill),
+ name: typeToColumnsPlaceholder[type],
+ });
+ }
+ return result;
+ },
+ getIndex: function (type, code) {
+ var compareNum = type === 'province' ? 2 : type === 'city' ? 4 : 6;
+ var list = this.getList(type, code.slice(0, compareNum - 2));
+ // oversea code
+ if (code[0] === '9' && type === 'province') {
+ compareNum = 1;
+ }
+ code = code.slice(0, compareNum);
+ for (var i = 0; i < list.length; i++) {
+ if (list[i].code.slice(0, compareNum) === code) {
+ return i;
+ }
+ }
+ return 0;
+ },
+ setValues: function () {
+ var picker = this.getPicker();
+ if (!picker) {
+ return;
+ }
+ var code = this.code || this.getDefaultCode();
+ var provinceList = this.getList('province');
+ var cityList = this.getList('city', code.slice(0, 2));
+ var stack = [];
+ var indexes = [];
+ var columnsNum = this.data.columnsNum;
+ if (columnsNum >= 1) {
+ stack.push(picker.setColumnValues(0, provinceList, false));
+ indexes.push(this.getIndex('province', code));
+ }
+ if (columnsNum >= 2) {
+ stack.push(picker.setColumnValues(1, cityList, false));
+ indexes.push(this.getIndex('city', code));
+ if (cityList.length && code.slice(2, 4) === '00') {
+ code = cityList[0].code;
+ }
+ }
+ if (columnsNum === 3) {
+ stack.push(picker.setColumnValues(2, this.getList('county', code.slice(0, 4)), false));
+ indexes.push(this.getIndex('county', code));
+ }
+ return Promise.all(stack)
+ .catch(function () { })
+ .then(function () { return picker.setIndexes(indexes); })
+ .catch(function () { });
+ },
+ getDefaultCode: function () {
+ var columnsPlaceholder = this.data.columnsPlaceholder;
+ if (columnsPlaceholder.length) {
+ return EMPTY_CODE;
+ }
+ var countyCodes = Object.keys(this.getConfig('county'));
+ if (countyCodes[0]) {
+ return countyCodes[0];
+ }
+ var cityCodes = Object.keys(this.getConfig('city'));
+ if (cityCodes[0]) {
+ return cityCodes[0];
+ }
+ return '';
+ },
+ getValues: function () {
+ var picker = this.getPicker();
+ if (!picker) {
+ return [];
+ }
+ return this.parseValues(picker.getValues().filter(function (value) { return !!value; }));
+ },
+ getDetail: function () {
+ var values = this.getValues();
+ var area = {
+ code: '',
+ country: '',
+ province: '',
+ city: '',
+ county: '',
+ };
+ if (!values.length) {
+ return area;
+ }
+ var names = values.map(function (item) { return item.name; });
+ area.code = values[values.length - 1].code;
+ if (area.code[0] === '9') {
+ area.country = names[1] || '';
+ area.province = names[2] || '';
+ }
+ else {
+ area.province = names[0] || '';
+ area.city = names[1] || '';
+ area.county = names[2] || '';
+ }
+ return area;
+ },
+ reset: function (code) {
+ this.code = code || '';
+ return this.setValues();
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxml
new file mode 100644
index 0000000..3a437b7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxml
@@ -0,0 +1,20 @@
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxs
new file mode 100644
index 0000000..07723c1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxs
@@ -0,0 +1,8 @@
+/* eslint-disable */
+function displayColumns(columns, columnsNum) {
+ return columns.slice(0, +columnsNum);
+}
+
+module.exports = {
+ displayColumns: displayColumns,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/area/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.js
new file mode 100644
index 0000000..984135c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.js
@@ -0,0 +1,67 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var version_1 = require("../common/version");
+var mixins = [button_1.button];
+if ((0, version_1.canIUseFormFieldButton)()) {
+ mixins.push('wx://form-field-button');
+}
+(0, component_1.VantComponent)({
+ mixins: mixins,
+ classes: ['hover-class', 'loading-class'],
+ data: {
+ baseStyle: '',
+ },
+ props: {
+ formType: String,
+ icon: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ plain: Boolean,
+ block: Boolean,
+ round: Boolean,
+ square: Boolean,
+ loading: Boolean,
+ hairline: Boolean,
+ disabled: Boolean,
+ loadingText: String,
+ customStyle: String,
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ type: {
+ type: String,
+ value: 'default',
+ },
+ dataset: null,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loadingSize: {
+ type: String,
+ value: '20px',
+ },
+ color: String,
+ },
+ methods: {
+ onClick: function (event) {
+ var _this = this;
+ this.$emit('click', event);
+ var _a = this.data, canIUseGetUserProfile = _a.canIUseGetUserProfile, openType = _a.openType, getUserProfileDesc = _a.getUserProfileDesc, lang = _a.lang;
+ if (openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: getUserProfileDesc || ' ',
+ lang: lang || 'en',
+ complete: function (userProfile) {
+ _this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxml
new file mode 100644
index 0000000..e7f60f1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxs
new file mode 100644
index 0000000..8b649fe
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ if (!data.color) {
+ return data.customStyle;
+ }
+
+ var properties = {
+ color: data.plain ? data.color : '#fff',
+ background: data.plain ? null : data.color,
+ };
+
+ // hide border when color is linear-gradient
+ if (data.color.indexOf('gradient') !== -1) {
+ properties.border = 0;
+ } else {
+ properties['border-color'] = data.color;
+ }
+
+ return style([properties, data.customStyle]);
+}
+
+function loadingColor(data) {
+ if (data.plain) {
+ return data.color ? data.color : '#c9c9c9';
+ }
+
+ if (data.type === 'default') {
+ return '#c9c9c9';
+ }
+
+ return '#fff';
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxss
new file mode 100644
index 0000000..bd8bb5a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-button{-webkit-text-size-adjust:100%;align-items:center;-webkit-appearance:none;border-radius:var(--button-border-radius,2px);box-sizing:border-box;display:inline-flex;font-size:var(--button-default-font-size,16px);height:var(--button-default-height,44px);justify-content:center;line-height:var(--button-line-height,20px);padding:0;position:relative;text-align:center;transition:opacity .2s;vertical-align:middle}.van-button:before{background-color:#000;border:inherit;border-color:#000;border-radius:inherit;content:" ";height:100%;left:50%;opacity:0;position:absolute;top:50%;transform:translate(-50%,-50%);width:100%}.van-button:after{border-width:0}.van-button--active:before{opacity:.15}.van-button--unclickable:after{display:none}.van-button--default{background:var(--button-default-background-color,#fff);border:var(--button-border-width,1px) solid var(--button-default-border-color,#ebedf0);color:var(--button-default-color,#323233)}.van-button--primary{background:var(--button-primary-background-color,#07c160);border:var(--button-border-width,1px) solid var(--button-primary-border-color,#07c160);color:var(--button-primary-color,#fff)}.van-button--info{background:var(--button-info-background-color,#1989fa);border:var(--button-border-width,1px) solid var(--button-info-border-color,#1989fa);color:var(--button-info-color,#fff)}.van-button--danger{background:var(--button-danger-background-color,#ee0a24);border:var(--button-border-width,1px) solid var(--button-danger-border-color,#ee0a24);color:var(--button-danger-color,#fff)}.van-button--warning{background:var(--button-warning-background-color,#ff976a);border:var(--button-border-width,1px) solid var(--button-warning-border-color,#ff976a);color:var(--button-warning-color,#fff)}.van-button--plain{background:var(--button-plain-background-color,#fff)}.van-button--plain.van-button--primary{color:var(--button-primary-background-color,#07c160)}.van-button--plain.van-button--info{color:var(--button-info-background-color,#1989fa)}.van-button--plain.van-button--danger{color:var(--button-danger-background-color,#ee0a24)}.van-button--plain.van-button--warning{color:var(--button-warning-background-color,#ff976a)}.van-button--large{height:var(--button-large-height,50px);width:100%}.van-button--normal{font-size:var(--button-normal-font-size,14px);padding:0 15px}.van-button--small{font-size:var(--button-small-font-size,12px);height:var(--button-small-height,30px);min-width:var(--button-small-min-width,60px);padding:0 var(--padding-xs,8px)}.van-button--mini{display:inline-block;font-size:var(--button-mini-font-size,10px);height:var(--button-mini-height,22px);min-width:var(--button-mini-min-width,50px)}.van-button--mini+.van-button--mini{margin-left:5px}.van-button--block{display:flex;width:100%}.van-button--round{border-radius:var(--button-round-border-radius,999px)}.van-button--square{border-radius:0}.van-button--disabled{opacity:var(--button-disabled-opacity,.5)}.van-button__text{display:inline}.van-button__icon+.van-button__text:not(:empty),.van-button__loading-text{margin-left:4px}.van-button__icon{line-height:inherit!important;min-width:1em;vertical-align:top}.van-button--hairline{border-width:0;padding-top:1px}.van-button--hairline:after{border-color:inherit;border-radius:calc(var(--button-border-radius, 2px)*2);border-width:1px}.van-button--hairline.van-button--round:after{border-radius:var(--button-round-border-radius,999px)}.van-button--hairline.van-button--square:after{border-radius:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/calendar.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/calendar.wxml
new file mode 100644
index 0000000..2ddb048
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/calendar.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.js
new file mode 100644
index 0000000..544b3a4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.js
@@ -0,0 +1,45 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../../../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ subtitle: String,
+ showTitle: Boolean,
+ showSubtitle: Boolean,
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'initWeekDay',
+ },
+ },
+ data: {
+ weekdays: [],
+ },
+ created: function () {
+ this.initWeekDay();
+ },
+ methods: {
+ initWeekDay: function () {
+ var defaultWeeks = ['日', '一', '二', '三', '四', '五', '六'];
+ var firstDayOfWeek = this.data.firstDayOfWeek || 0;
+ this.setData({
+ weekdays: __spreadArray(__spreadArray([], defaultWeeks.slice(firstDayOfWeek, 7), true), defaultWeeks.slice(0, firstDayOfWeek), true),
+ });
+ },
+ onClickSubtitle: function (event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxml
new file mode 100644
index 0000000..7e56c83
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxss
new file mode 100644
index 0000000..272537e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/header/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar__header{box-shadow:var(--calendar-header-box-shadow,0 2px 10px hsla(220,1%,50%,.16));flex-shrink:0}.van-calendar__header-subtitle,.van-calendar__header-title{font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__header-title+.van-calendar__header-title,.van-calendar__header-title:empty{display:none}.van-calendar__header-title:empty+.van-calendar__header-title{display:block!important}.van-calendar__weekdays{display:flex}.van-calendar__weekday{flex:1;font-size:var(--calendar-weekdays-font-size,12px);line-height:var(--calendar-weekdays-height,30px);text-align:center}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.d.ts
new file mode 100644
index 0000000..3ccf85a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.d.ts
@@ -0,0 +1,6 @@
+export interface Day {
+ date: Date;
+ type: string;
+ text: number;
+ bottomInfo?: string;
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.js
new file mode 100644
index 0000000..4d137f5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.js
@@ -0,0 +1,158 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../../../common/component");
+var utils_1 = require("../../utils");
+(0, component_1.VantComponent)({
+ props: {
+ date: {
+ type: null,
+ observer: 'setDays',
+ },
+ type: {
+ type: String,
+ observer: 'setDays',
+ },
+ color: String,
+ minDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ maxDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ showMark: Boolean,
+ rowHeight: null,
+ formatter: {
+ type: null,
+ observer: 'setDays',
+ },
+ currentDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'setDays',
+ },
+ allowSameDay: Boolean,
+ showSubtitle: Boolean,
+ showMonthTitle: Boolean,
+ },
+ data: {
+ visible: true,
+ days: [],
+ },
+ methods: {
+ onClick: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var item = this.data.days[index];
+ if (item.type !== 'disabled') {
+ this.$emit('click', item);
+ }
+ },
+ setDays: function () {
+ var days = [];
+ var startDate = new Date(this.data.date);
+ var year = startDate.getFullYear();
+ var month = startDate.getMonth();
+ var totalDay = (0, utils_1.getMonthEndDay)(startDate.getFullYear(), startDate.getMonth() + 1);
+ for (var day = 1; day <= totalDay; day++) {
+ var date = new Date(year, month, day);
+ var type = this.getDayType(date);
+ var config = {
+ date: date,
+ type: type,
+ text: day,
+ bottomInfo: this.getBottomInfo(type),
+ };
+ if (this.data.formatter) {
+ config = this.data.formatter(config);
+ }
+ days.push(config);
+ }
+ this.setData({ days: days });
+ },
+ getMultipleDayType: function (day) {
+ var currentDate = this.data.currentDate;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ var isSelected = function (date) {
+ return currentDate.some(function (item) { return (0, utils_1.compareDay)(item, date) === 0; });
+ };
+ if (isSelected(day)) {
+ var prevDay = (0, utils_1.getPrevDay)(day);
+ var nextDay = (0, utils_1.getNextDay)(day);
+ var prevSelected = isSelected(prevDay);
+ var nextSelected = isSelected(nextDay);
+ if (prevSelected && nextSelected) {
+ return 'multiple-middle';
+ }
+ if (prevSelected) {
+ return 'end';
+ }
+ return nextSelected ? 'start' : 'multiple-selected';
+ }
+ return '';
+ },
+ getRangeDayType: function (day) {
+ var _a = this.data, currentDate = _a.currentDate, allowSameDay = _a.allowSameDay;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ var startDay = currentDate[0], endDay = currentDate[1];
+ if (!startDay) {
+ return '';
+ }
+ var compareToStart = (0, utils_1.compareDay)(day, startDay);
+ if (!endDay) {
+ return compareToStart === 0 ? 'start' : '';
+ }
+ var compareToEnd = (0, utils_1.compareDay)(day, endDay);
+ if (compareToStart === 0 && compareToEnd === 0 && allowSameDay) {
+ return 'start-end';
+ }
+ if (compareToStart === 0) {
+ return 'start';
+ }
+ if (compareToEnd === 0) {
+ return 'end';
+ }
+ if (compareToStart > 0 && compareToEnd < 0) {
+ return 'middle';
+ }
+ return '';
+ },
+ getDayType: function (day) {
+ var _a = this.data, type = _a.type, minDate = _a.minDate, maxDate = _a.maxDate, currentDate = _a.currentDate;
+ if ((0, utils_1.compareDay)(day, minDate) < 0 || (0, utils_1.compareDay)(day, maxDate) > 0) {
+ return 'disabled';
+ }
+ if (type === 'single') {
+ return (0, utils_1.compareDay)(day, currentDate) === 0 ? 'selected' : '';
+ }
+ if (type === 'multiple') {
+ return this.getMultipleDayType(day);
+ }
+ /* istanbul ignore else */
+ if (type === 'range') {
+ return this.getRangeDayType(day);
+ }
+ return '';
+ },
+ getBottomInfo: function (type) {
+ if (this.data.type === 'range') {
+ if (type === 'start') {
+ return '开始';
+ }
+ if (type === 'end') {
+ return '结束';
+ }
+ if (type === 'start-end') {
+ return '开始/结束';
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxml
new file mode 100644
index 0000000..0c73b2f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxml
@@ -0,0 +1,39 @@
+
+
+
+
+
+ {{ computed.formatMonthTitle(date) }}
+
+
+
+
+ {{ computed.getMark(date) }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxs
new file mode 100644
index 0000000..55e45a5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxs
@@ -0,0 +1,71 @@
+/* eslint-disable */
+var utils = require('../../utils.wxs');
+
+function getMark(date) {
+ return getDate(date).getMonth() + 1;
+}
+
+var ROW_HEIGHT = 64;
+
+function getDayStyle(type, index, date, rowHeight, color, firstDayOfWeek) {
+ var style = [];
+ var current = getDate(date).getDay() || 7;
+ var offset = current < firstDayOfWeek ? (7 - firstDayOfWeek + current) :
+ current === 7 && firstDayOfWeek === 0 ? 0 :
+ (current - firstDayOfWeek);
+
+ if (index === 0) {
+ style.push(['margin-left', (100 * offset) / 7 + '%']);
+ }
+
+ if (rowHeight !== ROW_HEIGHT) {
+ style.push(['height', rowHeight + 'px']);
+ }
+
+ if (color) {
+ if (
+ type === 'start' ||
+ type === 'end' ||
+ type === 'start-end' ||
+ type === 'multiple-selected' ||
+ type === 'multiple-middle'
+ ) {
+ style.push(['background', color]);
+ } else if (type === 'middle') {
+ style.push(['color', color]);
+ }
+ }
+
+ return style
+ .map(function(item) {
+ return item.join(':');
+ })
+ .join(';');
+}
+
+function formatMonthTitle(date) {
+ date = getDate(date);
+ return date.getFullYear() + '年' + (date.getMonth() + 1) + '月';
+}
+
+function getMonthStyle(visible, date, rowHeight) {
+ if (!visible) {
+ date = getDate(date);
+
+ var totalDay = utils.getMonthEndDay(
+ date.getFullYear(),
+ date.getMonth() + 1
+ );
+ var offset = getDate(date).getDay();
+ var padding = Math.ceil((totalDay + offset) / 7) * rowHeight;
+
+ return 'padding-bottom:' + padding + 'px';
+ }
+}
+
+module.exports = {
+ getMark: getMark,
+ getDayStyle: getDayStyle,
+ formatMonthTitle: formatMonthTitle,
+ getMonthStyle: getMonthStyle
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxss
new file mode 100644
index 0000000..9aee73d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/components/month/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:100%}.van-calendar__month-title{font-size:var(--calendar-month-title-font-size,14px);font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__days{display:flex;flex-wrap:wrap;position:relative;-webkit-user-select:none;user-select:none}.van-calendar__month-mark{color:var(--calendar-month-mark-color,rgba(242,243,245,.8));font-size:var(--calendar-month-mark-font-size,160px);left:50%;pointer-events:none;position:absolute;top:50%;transform:translate(-50%,-50%);z-index:0}.van-calendar__day,.van-calendar__selected-day{align-items:center;display:flex;justify-content:center;text-align:center}.van-calendar__day{font-size:var(--calendar-day-font-size,16px);height:var(--calendar-day-height,64px);position:relative;width:14.285%}.van-calendar__day--end,.van-calendar__day--multiple-middle,.van-calendar__day--multiple-selected,.van-calendar__day--start,.van-calendar__day--start-end{background-color:var(--calendar-range-edge-background-color,#ee0a24);color:var(--calendar-range-edge-color,#fff)}.van-calendar__day--start{border-radius:4px 0 0 4px}.van-calendar__day--end{border-radius:0 4px 4px 0}.van-calendar__day--multiple-selected,.van-calendar__day--start-end{border-radius:4px}.van-calendar__day--middle{color:var(--calendar-range-middle-color,#ee0a24)}.van-calendar__day--middle:after{background-color:currentColor;bottom:0;content:"";left:0;opacity:var(--calendar-range-middle-background-opacity,.1);position:absolute;right:0;top:0}.van-calendar__day--disabled{color:var(--calendar-day-disabled-color,#c8c9cc);cursor:default}.van-calendar__bottom-info,.van-calendar__top-info{font-size:var(--calendar-info-font-size,10px);left:0;line-height:var(--calendar-info-line-height,14px);position:absolute;right:0}@media (max-width:350px){.van-calendar__bottom-info,.van-calendar__top-info{font-size:9px}}.van-calendar__top-info{top:6px}.van-calendar__bottom-info{bottom:6px}.van-calendar__selected-day{background-color:var(--calendar-selected-day-background-color,#ee0a24);border-radius:4px;color:var(--calendar-selected-day-color,#fff);height:var(--calendar-selected-day-size,54px);width:var(--calendar-selected-day-size,54px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.js
new file mode 100644
index 0000000..7a7324d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.js
@@ -0,0 +1,383 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+var __importDefault = (this && this.__importDefault) || function (mod) {
+ return (mod && mod.__esModule) ? mod : { "default": mod };
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+var toast_1 = __importDefault(require("../toast/toast"));
+var utils_2 = require("../common/utils");
+var initialMinDate = (0, utils_1.getToday)().getTime();
+var initialMaxDate = (function () {
+ var now = (0, utils_1.getToday)();
+ return new Date(now.getFullYear(), now.getMonth() + 6, now.getDate()).getTime();
+})();
+var getTime = function (date) {
+ return date instanceof Date ? date.getTime() : date;
+};
+(0, component_1.VantComponent)({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ color: String,
+ show: {
+ type: Boolean,
+ observer: function (val) {
+ if (val) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ },
+ formatter: null,
+ confirmText: {
+ type: String,
+ value: '确定',
+ },
+ confirmDisabledText: {
+ type: String,
+ value: '确定',
+ },
+ rangePrompt: String,
+ showRangePrompt: {
+ type: Boolean,
+ value: true,
+ },
+ defaultDate: {
+ type: null,
+ value: (0, utils_1.getToday)().getTime(),
+ observer: function (val) {
+ this.setData({ currentDate: val });
+ this.scrollIntoView();
+ },
+ },
+ allowSameDay: Boolean,
+ type: {
+ type: String,
+ value: 'single',
+ observer: 'reset',
+ },
+ minDate: {
+ type: Number,
+ value: initialMinDate,
+ },
+ maxDate: {
+ type: Number,
+ value: initialMaxDate,
+ },
+ position: {
+ type: String,
+ value: 'bottom',
+ },
+ rowHeight: {
+ type: null,
+ value: utils_1.ROW_HEIGHT,
+ },
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ poppable: {
+ type: Boolean,
+ value: true,
+ },
+ showMark: {
+ type: Boolean,
+ value: true,
+ },
+ showTitle: {
+ type: Boolean,
+ value: true,
+ },
+ showConfirm: {
+ type: Boolean,
+ value: true,
+ },
+ showSubtitle: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ maxRange: {
+ type: null,
+ value: null,
+ },
+ minRange: {
+ type: Number,
+ value: 1,
+ },
+ firstDayOfWeek: {
+ type: Number,
+ value: 0,
+ },
+ readonly: Boolean,
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ subtitle: '',
+ currentDate: null,
+ scrollIntoView: '',
+ },
+ watch: {
+ minDate: function () {
+ this.initRect();
+ },
+ maxDate: function () {
+ this.initRect();
+ },
+ },
+ created: function () {
+ this.setData({
+ currentDate: this.getInitialDate(this.data.defaultDate),
+ });
+ },
+ mounted: function () {
+ if (this.data.show || !this.data.poppable) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ methods: {
+ reset: function () {
+ this.setData({ currentDate: this.getInitialDate(this.data.defaultDate) });
+ this.scrollIntoView();
+ },
+ initRect: function () {
+ var _this = this;
+ if (this.contentObserver != null) {
+ this.contentObserver.disconnect();
+ }
+ var contentObserver = this.createIntersectionObserver({
+ thresholds: [0, 0.1, 0.9, 1],
+ observeAll: true,
+ });
+ this.contentObserver = contentObserver;
+ contentObserver.relativeTo('.van-calendar__body');
+ contentObserver.observe('.month', function (res) {
+ if (res.boundingClientRect.top <= res.relativeRect.top) {
+ // @ts-ignore
+ _this.setData({ subtitle: (0, utils_1.formatMonthTitle)(res.dataset.date) });
+ }
+ });
+ },
+ limitDateRange: function (date, minDate, maxDate) {
+ if (minDate === void 0) { minDate = null; }
+ if (maxDate === void 0) { maxDate = null; }
+ minDate = minDate || this.data.minDate;
+ maxDate = maxDate || this.data.maxDate;
+ if ((0, utils_1.compareDay)(date, minDate) === -1) {
+ return minDate;
+ }
+ if ((0, utils_1.compareDay)(date, maxDate) === 1) {
+ return maxDate;
+ }
+ return date;
+ },
+ getInitialDate: function (defaultDate) {
+ var _this = this;
+ if (defaultDate === void 0) { defaultDate = null; }
+ var _a = this.data, type = _a.type, minDate = _a.minDate, maxDate = _a.maxDate, allowSameDay = _a.allowSameDay;
+ if (!defaultDate)
+ return [];
+ var now = (0, utils_1.getToday)().getTime();
+ if (type === 'range') {
+ if (!Array.isArray(defaultDate)) {
+ defaultDate = [];
+ }
+ var _b = defaultDate || [], startDay = _b[0], endDay = _b[1];
+ var startDate = getTime(startDay || now);
+ var start = this.limitDateRange(startDate, minDate, allowSameDay ? startDate : (0, utils_1.getPrevDay)(new Date(maxDate)).getTime());
+ var date = getTime(endDay || now);
+ var end = this.limitDateRange(date, allowSameDay ? date : (0, utils_1.getNextDay)(new Date(minDate)).getTime());
+ return [start, end];
+ }
+ if (type === 'multiple') {
+ if (Array.isArray(defaultDate)) {
+ return defaultDate.map(function (date) { return _this.limitDateRange(date); });
+ }
+ return [this.limitDateRange(now)];
+ }
+ if (!defaultDate || Array.isArray(defaultDate)) {
+ defaultDate = now;
+ }
+ return this.limitDateRange(defaultDate);
+ },
+ scrollIntoView: function () {
+ var _this = this;
+ (0, utils_2.requestAnimationFrame)(function () {
+ var _a = _this.data, currentDate = _a.currentDate, type = _a.type, show = _a.show, poppable = _a.poppable, minDate = _a.minDate, maxDate = _a.maxDate;
+ if (!currentDate)
+ return;
+ // @ts-ignore
+ var targetDate = type === 'single' ? currentDate : currentDate[0];
+ var displayed = show || !poppable;
+ if (!targetDate || !displayed) {
+ return;
+ }
+ var months = (0, utils_1.getMonths)(minDate, maxDate);
+ months.some(function (month, index) {
+ if ((0, utils_1.compareMonth)(month, targetDate) === 0) {
+ _this.setData({ scrollIntoView: "month".concat(index) });
+ return true;
+ }
+ return false;
+ });
+ });
+ },
+ onOpen: function () {
+ this.$emit('open');
+ },
+ onOpened: function () {
+ this.$emit('opened');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClosed: function () {
+ this.$emit('closed');
+ },
+ onClickDay: function (event) {
+ if (this.data.readonly) {
+ return;
+ }
+ var date = event.detail.date;
+ var _a = this.data, type = _a.type, currentDate = _a.currentDate, allowSameDay = _a.allowSameDay;
+ if (type === 'range') {
+ // @ts-ignore
+ var startDay_1 = currentDate[0], endDay = currentDate[1];
+ if (startDay_1 && !endDay) {
+ var compareToStart = (0, utils_1.compareDay)(date, startDay_1);
+ if (compareToStart === 1) {
+ var days_1 = this.selectComponent('.month').data.days;
+ days_1.some(function (day, index) {
+ var isDisabled = day.type === 'disabled' &&
+ getTime(startDay_1) < getTime(day.date) &&
+ getTime(day.date) < getTime(date);
+ if (isDisabled) {
+ (date = days_1[index - 1].date);
+ }
+ return isDisabled;
+ });
+ this.select([startDay_1, date], true);
+ }
+ else if (compareToStart === -1) {
+ this.select([date, null]);
+ }
+ else if (allowSameDay) {
+ this.select([date, date], true);
+ }
+ }
+ else {
+ this.select([date, null]);
+ }
+ }
+ else if (type === 'multiple') {
+ var selectedIndex_1;
+ // @ts-ignore
+ var selected = currentDate.some(function (dateItem, index) {
+ var equal = (0, utils_1.compareDay)(dateItem, date) === 0;
+ if (equal) {
+ selectedIndex_1 = index;
+ }
+ return equal;
+ });
+ if (selected) {
+ // @ts-ignore
+ var cancelDate = currentDate.splice(selectedIndex_1, 1);
+ this.setData({ currentDate: currentDate });
+ this.unselect(cancelDate);
+ }
+ else {
+ // @ts-ignore
+ this.select(__spreadArray(__spreadArray([], currentDate, true), [date], false));
+ }
+ }
+ else {
+ this.select(date, true);
+ }
+ },
+ unselect: function (dateArray) {
+ var date = dateArray[0];
+ if (date) {
+ this.$emit('unselect', (0, utils_1.copyDates)(date));
+ }
+ },
+ select: function (date, complete) {
+ if (complete && this.data.type === 'range') {
+ var valid = this.checkRange(date);
+ if (!valid) {
+ // auto selected to max range if showConfirm
+ if (this.data.showConfirm) {
+ this.emit([
+ date[0],
+ (0, utils_1.getDayByOffset)(date[0], this.data.maxRange - 1),
+ ]);
+ }
+ else {
+ this.emit(date);
+ }
+ return;
+ }
+ }
+ this.emit(date);
+ if (complete && !this.data.showConfirm) {
+ this.onConfirm();
+ }
+ },
+ emit: function (date) {
+ this.setData({
+ currentDate: Array.isArray(date) ? date.map(getTime) : getTime(date),
+ });
+ this.$emit('select', (0, utils_1.copyDates)(date));
+ },
+ checkRange: function (date) {
+ var _a = this.data, maxRange = _a.maxRange, rangePrompt = _a.rangePrompt, showRangePrompt = _a.showRangePrompt;
+ if (maxRange && (0, utils_1.calcDateNum)(date) > maxRange) {
+ if (showRangePrompt) {
+ (0, toast_1.default)({
+ context: this,
+ message: rangePrompt || "\u9009\u62E9\u5929\u6570\u4E0D\u80FD\u8D85\u8FC7 ".concat(maxRange, " \u5929"),
+ });
+ }
+ this.$emit('over-range');
+ return false;
+ }
+ return true;
+ },
+ onConfirm: function () {
+ var _this = this;
+ if (this.data.type === 'range' &&
+ !this.checkRange(this.data.currentDate)) {
+ return;
+ }
+ wx.nextTick(function () {
+ // @ts-ignore
+ _this.$emit('confirm', (0, utils_1.copyDates)(_this.data.currentDate));
+ });
+ },
+ onClickSubtitle: function (event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.json
new file mode 100644
index 0000000..397d5ae
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.json
@@ -0,0 +1,10 @@
+{
+ "component": true,
+ "usingComponents": {
+ "header": "./components/header/index",
+ "month": "./components/month/index",
+ "van-button": "../button/index",
+ "van-popup": "../popup/index",
+ "van-toast": "../toast/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxml
new file mode 100644
index 0000000..9d0fc6b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxs
new file mode 100644
index 0000000..0a56646
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxs
@@ -0,0 +1,37 @@
+/* eslint-disable */
+var utils = require('./utils.wxs');
+
+function getMonths(minDate, maxDate) {
+ var months = [];
+ var cursor = getDate(minDate);
+
+ cursor.setDate(1);
+
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (utils.compareMonth(cursor, getDate(maxDate)) !== 1);
+
+ return months;
+}
+
+function getButtonDisabled(type, currentDate, minRange) {
+ if (currentDate == null) {
+ return true;
+ }
+
+ if (type === 'range') {
+ return !currentDate[0] || !currentDate[1];
+ }
+
+ if (type === 'multiple') {
+ return currentDate.length < minRange;
+ }
+
+ return !currentDate;
+}
+
+module.exports = {
+ getMonths: getMonths,
+ getButtonDisabled: getButtonDisabled
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxss
new file mode 100644
index 0000000..a1f1cf0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:var(--calendar-height,100%)}.van-calendar__close-icon{top:11px}.van-calendar__popup--bottom,.van-calendar__popup--top{height:var(--calendar-popup-height,90%)}.van-calendar__popup--left,.van-calendar__popup--right{height:100%}.van-calendar__body{-webkit-overflow-scrolling:touch;flex:1;overflow:auto}.van-calendar__footer{flex-shrink:0;padding:0 var(--padding-md,16px)}.van-calendar__footer--safe-area-inset-bottom{padding-bottom:env(safe-area-inset-bottom)}.van-calendar__footer+.van-calendar__footer,.van-calendar__footer:empty{display:none}.van-calendar__footer:empty+.van-calendar__footer{display:block!important}.van-calendar__confirm{height:var(--calendar-confirm-button-height,36px)!important;line-height:var(--calendar-confirm-button-line-height,34px)!important;margin:var(--calendar-confirm-button-margin,7px 0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.d.ts
new file mode 100644
index 0000000..889e6e7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.d.ts
@@ -0,0 +1,12 @@
+export declare const ROW_HEIGHT = 64;
+export declare function formatMonthTitle(date: Date): string;
+export declare function compareMonth(date1: Date | number, date2: Date | number): 0 | 1 | -1;
+export declare function compareDay(day1: Date | number, day2: Date | number): 0 | 1 | -1;
+export declare function getDayByOffset(date: Date, offset: number): Date;
+export declare function getPrevDay(date: Date): Date;
+export declare function getNextDay(date: Date): Date;
+export declare function getToday(): Date;
+export declare function calcDateNum(date: [Date, Date]): number;
+export declare function copyDates(dates: Date | Date[]): Date | Date[];
+export declare function getMonthEndDay(year: number, month: number): number;
+export declare function getMonths(minDate: number, maxDate: number): number[];
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.js
new file mode 100644
index 0000000..c9e5df7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.js
@@ -0,0 +1,97 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.getMonths = exports.getMonthEndDay = exports.copyDates = exports.calcDateNum = exports.getToday = exports.getNextDay = exports.getPrevDay = exports.getDayByOffset = exports.compareDay = exports.compareMonth = exports.formatMonthTitle = exports.ROW_HEIGHT = void 0;
+exports.ROW_HEIGHT = 64;
+function formatMonthTitle(date) {
+ if (!(date instanceof Date)) {
+ date = new Date(date);
+ }
+ return "".concat(date.getFullYear(), "\u5E74").concat(date.getMonth() + 1, "\u6708");
+}
+exports.formatMonthTitle = formatMonthTitle;
+function compareMonth(date1, date2) {
+ if (!(date1 instanceof Date)) {
+ date1 = new Date(date1);
+ }
+ if (!(date2 instanceof Date)) {
+ date2 = new Date(date2);
+ }
+ var year1 = date1.getFullYear();
+ var year2 = date2.getFullYear();
+ var month1 = date1.getMonth();
+ var month2 = date2.getMonth();
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+ return year1 > year2 ? 1 : -1;
+}
+exports.compareMonth = compareMonth;
+function compareDay(day1, day2) {
+ if (!(day1 instanceof Date)) {
+ day1 = new Date(day1);
+ }
+ if (!(day2 instanceof Date)) {
+ day2 = new Date(day2);
+ }
+ var compareMonthResult = compareMonth(day1, day2);
+ if (compareMonthResult === 0) {
+ var date1 = day1.getDate();
+ var date2 = day2.getDate();
+ return date1 === date2 ? 0 : date1 > date2 ? 1 : -1;
+ }
+ return compareMonthResult;
+}
+exports.compareDay = compareDay;
+function getDayByOffset(date, offset) {
+ date = new Date(date);
+ date.setDate(date.getDate() + offset);
+ return date;
+}
+exports.getDayByOffset = getDayByOffset;
+function getPrevDay(date) {
+ return getDayByOffset(date, -1);
+}
+exports.getPrevDay = getPrevDay;
+function getNextDay(date) {
+ return getDayByOffset(date, 1);
+}
+exports.getNextDay = getNextDay;
+function getToday() {
+ var today = new Date();
+ today.setHours(0, 0, 0, 0);
+ return today;
+}
+exports.getToday = getToday;
+function calcDateNum(date) {
+ var day1 = new Date(date[0]).getTime();
+ var day2 = new Date(date[1]).getTime();
+ return (day2 - day1) / (1000 * 60 * 60 * 24) + 1;
+}
+exports.calcDateNum = calcDateNum;
+function copyDates(dates) {
+ if (Array.isArray(dates)) {
+ return dates.map(function (date) {
+ if (date === null) {
+ return date;
+ }
+ return new Date(date);
+ });
+ }
+ return new Date(dates);
+}
+exports.copyDates = copyDates;
+function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+exports.getMonthEndDay = getMonthEndDay;
+function getMonths(minDate, maxDate) {
+ var months = [];
+ var cursor = new Date(minDate);
+ cursor.setDate(1);
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (compareMonth(cursor, maxDate) !== 1);
+ return months;
+}
+exports.getMonths = getMonths;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.wxs
new file mode 100644
index 0000000..e57f6b3
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/calendar/utils.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+function getMonthEndDay(year, month) {
+ return 32 - getDate(year, month - 1, 32).getDate();
+}
+
+function compareMonth(date1, date2) {
+ date1 = getDate(date1);
+ date2 = getDate(date2);
+
+ var year1 = date1.getFullYear();
+ var year2 = date2.getFullYear();
+ var month1 = date1.getMonth();
+ var month2 = date2.getMonth();
+
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+
+ return year1 > year2 ? 1 : -1;
+}
+
+module.exports = {
+ getMonthEndDay: getMonthEndDay,
+ compareMonth: compareMonth
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.js
new file mode 100644
index 0000000..2815655
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.js
@@ -0,0 +1,51 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var link_1 = require("../mixins/link");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'num-class',
+ 'desc-class',
+ 'thumb-class',
+ 'title-class',
+ 'price-class',
+ 'origin-price-class',
+ ],
+ mixins: [link_1.link],
+ props: {
+ tag: String,
+ num: String,
+ desc: String,
+ thumb: String,
+ title: String,
+ price: {
+ type: String,
+ observer: 'updatePrice',
+ },
+ centered: Boolean,
+ lazyLoad: Boolean,
+ thumbLink: String,
+ originPrice: String,
+ thumbMode: {
+ type: String,
+ value: 'aspectFit',
+ },
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ },
+ methods: {
+ updatePrice: function () {
+ var price = this.data.price;
+ var priceArr = price.toString().split('.');
+ this.setData({
+ integerStr: priceArr[0],
+ decimalStr: priceArr[1] ? ".".concat(priceArr[1]) : '',
+ });
+ },
+ onClickThumb: function () {
+ this.jumpLink('thumbLink');
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.json
new file mode 100644
index 0000000..e917407
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-tag": "../tag/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxml
new file mode 100644
index 0000000..62173e4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxss
new file mode 100644
index 0000000..0f4d7c5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/card/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-card{background-color:var(--card-background-color,#fafafa);box-sizing:border-box;color:var(--card-text-color,#323233);font-size:var(--card-font-size,12px);padding:var(--card-padding,8px 16px);position:relative}.van-card__header{display:flex}.van-card__header--center{align-items:center;justify-content:center}.van-card__thumb{flex:none;height:var(--card-thumb-size,88px);margin-right:var(--padding-xs,8px);position:relative;width:var(--card-thumb-size,88px)}.van-card__thumb:empty{display:none}.van-card__img{border-radius:8px;height:100%;width:100%}.van-card__content{display:flex;flex:1;flex-direction:column;justify-content:space-between;min-height:var(--card-thumb-size,88px);min-width:0;position:relative}.van-card__content--center{justify-content:center}.van-card__desc,.van-card__title{word-wrap:break-word}.van-card__title{font-weight:700;line-height:var(--card-title-line-height,16px)}.van-card__desc{color:var(--card-desc-color,#646566);line-height:var(--card-desc-line-height,20px)}.van-card__bottom{line-height:20px}.van-card__price{color:var(--card-price-color,#ee0a24);display:inline-block;font-size:var(--card-price-font-size,12px);font-weight:700}.van-card__price-integer{font-size:var(--card-price-integer-font-size,16px)}.van-card__price-decimal,.van-card__price-integer{font-family:var(--card-price-font-family,Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif)}.van-card__origin-price{color:var(--card-origin-price-color,#646566);display:inline-block;font-size:var(--card-origin-price-font-size,10px);margin-left:5px;text-decoration:line-through}.van-card__num{float:right}.van-card__tag{left:0;position:absolute!important;top:2px}.van-card__footer{flex:none;text-align:right;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.js
new file mode 100644
index 0000000..1b45831
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.js
@@ -0,0 +1,223 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var FieldName;
+(function (FieldName) {
+ FieldName["TEXT"] = "text";
+ FieldName["VALUE"] = "value";
+ FieldName["CHILDREN"] = "children";
+})(FieldName || (FieldName = {}));
+var defaultFieldNames = {
+ text: FieldName.TEXT,
+ value: FieldName.VALUE,
+ children: FieldName.CHILDREN,
+};
+(0, component_1.VantComponent)({
+ props: {
+ title: String,
+ value: {
+ type: String,
+ },
+ placeholder: {
+ type: String,
+ value: '请选择',
+ },
+ activeColor: {
+ type: String,
+ value: '#1989fa',
+ },
+ options: {
+ type: Array,
+ value: [],
+ },
+ swipeable: {
+ type: Boolean,
+ value: false,
+ },
+ closeable: {
+ type: Boolean,
+ value: true,
+ },
+ showHeader: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ fieldNames: {
+ type: Object,
+ value: defaultFieldNames,
+ observer: 'updateFieldNames',
+ },
+ useTitleSlot: Boolean,
+ },
+ data: {
+ tabs: [],
+ activeTab: 0,
+ textKey: FieldName.TEXT,
+ valueKey: FieldName.VALUE,
+ childrenKey: FieldName.CHILDREN,
+ innerValue: '',
+ },
+ watch: {
+ options: function () {
+ this.updateTabs();
+ },
+ value: function (newVal) {
+ this.updateValue(newVal);
+ },
+ },
+ created: function () {
+ this.updateTabs();
+ },
+ methods: {
+ updateValue: function (val) {
+ var _this = this;
+ if (val !== undefined) {
+ var values = this.data.tabs.map(function (tab) { return tab.selected && tab.selected[_this.data.valueKey]; });
+ if (values.indexOf(val) > -1) {
+ return;
+ }
+ }
+ this.innerValue = val;
+ this.updateTabs();
+ },
+ updateFieldNames: function () {
+ var _a = this.data.fieldNames || defaultFieldNames, _b = _a.text, text = _b === void 0 ? 'text' : _b, _c = _a.value, value = _c === void 0 ? 'value' : _c, _d = _a.children, children = _d === void 0 ? 'children' : _d;
+ this.setData({
+ textKey: text,
+ valueKey: value,
+ childrenKey: children,
+ });
+ },
+ getSelectedOptionsByValue: function (options, value) {
+ for (var i = 0; i < options.length; i++) {
+ var option = options[i];
+ if (option[this.data.valueKey] === value) {
+ return [option];
+ }
+ if (option[this.data.childrenKey]) {
+ var selectedOptions = this.getSelectedOptionsByValue(option[this.data.childrenKey], value);
+ if (selectedOptions) {
+ return __spreadArray([option], selectedOptions, true);
+ }
+ }
+ }
+ },
+ updateTabs: function () {
+ var _this = this;
+ var options = this.data.options;
+ var innerValue = this.innerValue;
+ if (!options.length) {
+ return;
+ }
+ if (innerValue !== undefined) {
+ var selectedOptions = this.getSelectedOptionsByValue(options, innerValue);
+ if (selectedOptions) {
+ var optionsCursor_1 = options;
+ var tabs_1 = selectedOptions.map(function (option) {
+ var tab = {
+ options: optionsCursor_1,
+ selected: option,
+ };
+ var next = optionsCursor_1.find(function (item) { return item[_this.data.valueKey] === option[_this.data.valueKey]; });
+ if (next) {
+ optionsCursor_1 = next[_this.data.childrenKey];
+ }
+ return tab;
+ });
+ if (optionsCursor_1) {
+ tabs_1.push({
+ options: optionsCursor_1,
+ selected: null,
+ });
+ }
+ this.setData({
+ tabs: tabs_1,
+ });
+ wx.nextTick(function () {
+ _this.setData({
+ activeTab: tabs_1.length - 1,
+ });
+ });
+ return;
+ }
+ }
+ this.setData({
+ tabs: [
+ {
+ options: options,
+ selected: null,
+ },
+ ],
+ });
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClickTab: function (e) {
+ var _a = e.detail, tabIndex = _a.index, title = _a.title;
+ this.$emit('click-tab', { title: title, tabIndex: tabIndex });
+ this.setData({
+ activeTab: tabIndex,
+ });
+ },
+ // 选中
+ onSelect: function (e) {
+ var _this = this;
+ var _a = e.currentTarget.dataset, option = _a.option, tabIndex = _a.tabIndex;
+ if (option && option.disabled) {
+ return;
+ }
+ var _b = this.data, valueKey = _b.valueKey, childrenKey = _b.childrenKey;
+ var tabs = this.data.tabs;
+ tabs[tabIndex].selected = option;
+ if (tabs.length > tabIndex + 1) {
+ tabs = tabs.slice(0, tabIndex + 1);
+ }
+ if (option[childrenKey]) {
+ var nextTab = {
+ options: option[childrenKey],
+ selected: null,
+ };
+ if (tabs[tabIndex + 1]) {
+ tabs[tabIndex + 1] = nextTab;
+ }
+ else {
+ tabs.push(nextTab);
+ }
+ wx.nextTick(function () {
+ _this.setData({
+ activeTab: tabIndex + 1,
+ });
+ });
+ }
+ this.setData({
+ tabs: tabs,
+ });
+ var selectedOptions = tabs.map(function (tab) { return tab.selected; }).filter(Boolean);
+ var value = option[valueKey];
+ var params = {
+ value: value,
+ tabIndex: tabIndex,
+ selectedOptions: selectedOptions,
+ };
+ this.innerValue = value;
+ this.$emit('change', params);
+ if (!option[childrenKey]) {
+ this.$emit('finish', params);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.json
new file mode 100644
index 0000000..d0f75eb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-tab": "../tab/index",
+ "van-tabs": "../tabs/index"
+ }
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxml
new file mode 100644
index 0000000..9417234
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ option[textKey] }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxs
new file mode 100644
index 0000000..b1aab58
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxs
@@ -0,0 +1,24 @@
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function isSelected(tab, valueKey, option) {
+ return tab.selected && tab.selected[valueKey] === option[valueKey]
+}
+
+function optionClass(tab, valueKey, option) {
+ return utils.bem('cascader__option', { selected: isSelected(tab, valueKey, option), disabled: option.disabled })
+}
+
+function optionStyle(data) {
+ var color = data.option.color || (isSelected(data.tab, data.valueKey, data.option) ? data.activeColor : undefined);
+ return style({
+ color
+ });
+}
+
+
+module.exports = {
+ isSelected: isSelected,
+ optionClass: optionClass,
+ optionStyle: optionStyle,
+};
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxss
new file mode 100644
index 0000000..7062486
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cascader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cascader__header{align-items:center;display:flex;height:48px;justify-content:space-between;padding:0 16px}.van-cascader__title{font-size:16px;font-weight:600;line-height:20px}.van-cascader__close-icon{color:#c8c9cc;font-size:22px;height:22px}.van-cascader__tabs-wrap{height:48px!important;padding:0 8px}.van-cascader__tab{color:#323233!important;flex:none!important;font-weight:600!important;padding:0 8px!important}.van-cascader__tab--unselected{color:#969799!important;font-weight:400!important}.van-cascader__option{align-items:center;cursor:pointer;display:flex;font-size:14px;justify-content:space-between;line-height:20px;padding:10px 16px}.van-cascader__option:active{background-color:#f2f3f5}.van-cascader__option--selected{color:#1989fa;font-weight:600}.van-cascader__option--disabled{color:#c8c9cc;cursor:not-allowed}.van-cascader__option--disabled:active{background-color:initial}.van-cascader__options{-webkit-overflow-scrolling:touch;box-sizing:border-box;height:384px;overflow-y:auto;padding-top:6px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.js
new file mode 100644
index 0000000..34a93a6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.js
@@ -0,0 +1,13 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ title: String,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ inset: Boolean,
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxml
new file mode 100644
index 0000000..311e064
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxml
@@ -0,0 +1,11 @@
+
+
+
+ {{ title }}
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxss
new file mode 100644
index 0000000..08b252f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell-group--inset{border-radius:var(--cell-group-inset-border-radius,8px);margin:var(--cell-group-inset-padding,0 16px);overflow:hidden}.van-cell-group__title{color:var(--cell-group-title-color,#969799);font-size:var(--cell-group-title-font-size,14px);line-height:var(--cell-group-title-line-height,16px);padding:var(--cell-group-title-padding,16px 16px 8px)}.van-cell-group__title--inset{padding:var(--cell-group-inset-title-padding,16px 16px 8px 32px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.js
new file mode 100644
index 0000000..80f3039
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.js
@@ -0,0 +1,40 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var link_1 = require("../mixins/link");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'title-class',
+ 'label-class',
+ 'value-class',
+ 'right-icon-class',
+ 'hover-class',
+ ],
+ mixins: [link_1.link],
+ props: {
+ title: null,
+ value: null,
+ icon: String,
+ size: String,
+ label: String,
+ center: Boolean,
+ isLink: Boolean,
+ required: Boolean,
+ clickable: Boolean,
+ titleWidth: String,
+ customStyle: String,
+ arrowDirection: String,
+ useLabelSlot: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ titleStyle: String,
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxml
new file mode 100644
index 0000000..8387c3c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+ {{ title }}
+
+
+
+
+ {{ label }}
+
+
+
+
+ {{ value }}
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxs
new file mode 100644
index 0000000..e3500c4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function titleStyle(data) {
+ return style([
+ {
+ 'max-width': addUnit(data.titleWidth),
+ 'min-width': addUnit(data.titleWidth),
+ },
+ data.titleStyle,
+ ]);
+}
+
+module.exports = {
+ titleStyle: titleStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxss
new file mode 100644
index 0000000..1802f8e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell{background-color:var(--cell-background-color,#fff);box-sizing:border-box;color:var(--cell-text-color,#323233);display:flex;font-size:var(--cell-font-size,14px);line-height:var(--cell-line-height,24px);padding:var(--cell-vertical-padding,10px) var(--cell-horizontal-padding,16px);position:relative;width:100%}.van-cell:after{border-bottom:1px solid #ebedf0;bottom:0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:16px;transform:scaleY(.5);transform-origin:center}.van-cell--borderless:after{display:none}.van-cell-group{background-color:var(--cell-background-color,#fff)}.van-cell__label{color:var(--cell-label-color,#969799);font-size:var(--cell-label-font-size,12px);line-height:var(--cell-label-line-height,18px);margin-top:var(--cell-label-margin-top,3px)}.van-cell__value{color:var(--cell-value-color,#969799);overflow:hidden;text-align:right;vertical-align:middle}.van-cell__title,.van-cell__value{flex:1}.van-cell__title:empty,.van-cell__value:empty{display:none}.van-cell__left-icon-wrap,.van-cell__right-icon-wrap{align-items:center;display:flex;font-size:var(--cell-icon-size,16px);height:var(--cell-line-height,24px)}.van-cell__left-icon-wrap{margin-right:var(--padding-base,4px)}.van-cell__right-icon-wrap{color:var(--cell-right-icon-color,#969799);margin-left:var(--padding-base,4px)}.van-cell__left-icon{vertical-align:middle}.van-cell__left-icon,.van-cell__right-icon{line-height:var(--cell-line-height,24px)}.van-cell--clickable.van-cell--hover{background-color:var(--cell-active-color,#f2f3f5)}.van-cell--required{overflow:visible}.van-cell--required:before{color:var(--cell-required-color,#ee0a24);content:"*";font-size:var(--cell-font-size,14px);left:var(--padding-xs,8px);position:absolute}.van-cell--center{align-items:center}.van-cell--large{padding-bottom:var(--cell-large-vertical-padding,12px);padding-top:var(--cell-large-vertical-padding,12px)}.van-cell--large .van-cell__title{font-size:var(--cell-large-title-font-size,16px)}.van-cell--large .van-cell__value{font-size:var(--cell-large-value-font-size,16px)}.van-cell--large .van-cell__label{font-size:var(--cell-large-label-font-size,14px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.js
new file mode 100644
index 0000000..80c93a1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.js
@@ -0,0 +1,39 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useChildren)('checkbox', function (target) {
+ this.updateChild(target);
+ }),
+ props: {
+ max: Number,
+ value: {
+ type: Array,
+ observer: 'updateChildren',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ value: 'vertical',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ var _this = this;
+ this.children.forEach(function (child) { return _this.updateChild(child); });
+ },
+ updateChild: function (child) {
+ var _a = this.data, value = _a.value, disabled = _a.disabled, direction = _a.direction;
+ child.setData({
+ value: value.indexOf(child.data.name) !== -1,
+ parentDisabled: disabled,
+ direction: direction,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxml
new file mode 100644
index 0000000..638bf9d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxss
new file mode 100644
index 0000000..c5666d7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.js
new file mode 100644
index 0000000..6247365
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.js
@@ -0,0 +1,79 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+function emit(target, value) {
+ target.$emit('input', value);
+ target.$emit('change', value);
+}
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useParent)('checkbox-group'),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ value: Boolean,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ parentDisabled: false,
+ direction: 'vertical',
+ },
+ methods: {
+ emitChange: function (value) {
+ if (this.parent) {
+ this.setParentValue(this.parent, value);
+ }
+ else {
+ emit(this, value);
+ }
+ },
+ toggle: function () {
+ var _a = this.data, parentDisabled = _a.parentDisabled, disabled = _a.disabled, value = _a.value;
+ if (!disabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ onClickLabel: function () {
+ var _a = this.data, labelDisabled = _a.labelDisabled, parentDisabled = _a.parentDisabled, disabled = _a.disabled, value = _a.value;
+ if (!disabled && !labelDisabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ setParentValue: function (parent, value) {
+ var parentValue = parent.data.value.slice();
+ var name = this.data.name;
+ var max = parent.data.max;
+ if (value) {
+ if (max && parentValue.length >= max) {
+ return;
+ }
+ if (parentValue.indexOf(name) === -1) {
+ parentValue.push(name);
+ emit(parent, parentValue);
+ }
+ }
+ else {
+ var index = parentValue.indexOf(name);
+ if (index !== -1) {
+ parentValue.splice(index, 1);
+ emit(parent, parentValue);
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxml
new file mode 100644
index 0000000..39a7bb0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxml
@@ -0,0 +1,31 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxs
new file mode 100644
index 0000000..eb9c772
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxs
@@ -0,0 +1,20 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(checkedColor, value, disabled, parentDisabled, iconSize) {
+ var styles = {
+ 'font-size': addUnit(iconSize),
+ };
+
+ if (checkedColor && value && !disabled && !parentDisabled) {
+ styles['border-color'] = checkedColor;
+ styles['background-color'] = checkedColor;
+ }
+
+ return style(styles);
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxss
new file mode 100644
index 0000000..da2272a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/checkbox/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-checkbox--horizontal{margin-right:12px}.van-checkbox__icon-wrap,.van-checkbox__label{line-height:var(--checkbox-size,20px)}.van-checkbox__icon-wrap{flex:none}.van-checkbox__icon{align-items:center;border:1px solid var(--checkbox-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--checkbox-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--checkbox-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-checkbox__icon--round{border-radius:100%}.van-checkbox__icon--checked{background-color:var(--checkbox-checked-icon-color,#1989fa);border-color:var(--checkbox-checked-icon-color,#1989fa);color:#fff}.van-checkbox__icon--disabled{background-color:var(--checkbox-disabled-background-color,#ebedf0);border-color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__icon--disabled.van-checkbox__icon--checked{color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__label{word-wrap:break-word;color:var(--checkbox-label-color,#323233);padding-left:var(--checkbox-label-margin,10px)}.van-checkbox__label--left{float:left;margin:0 var(--checkbox-label-margin,10px) 0 0}.van-checkbox__label--disabled{color:var(--checkbox-disabled-label-color,#c8c9cc)}.van-checkbox__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.d.ts
new file mode 100644
index 0000000..8a0b71e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.d.ts
@@ -0,0 +1,4 @@
+///
+type CanvasContext = WechatMiniprogram.CanvasContext;
+export declare function adaptor(ctx: CanvasContext & Record): CanvasContext;
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.js
new file mode 100644
index 0000000..d81df74
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/canvas.js
@@ -0,0 +1,47 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.adaptor = void 0;
+function adaptor(ctx) {
+ // @ts-ignore
+ return Object.assign(ctx, {
+ setStrokeStyle: function (val) {
+ ctx.strokeStyle = val;
+ },
+ setLineWidth: function (val) {
+ ctx.lineWidth = val;
+ },
+ setLineCap: function (val) {
+ ctx.lineCap = val;
+ },
+ setFillStyle: function (val) {
+ ctx.fillStyle = val;
+ },
+ setFontSize: function (val) {
+ ctx.font = String(val);
+ },
+ setGlobalAlpha: function (val) {
+ ctx.globalAlpha = val;
+ },
+ setLineJoin: function (val) {
+ ctx.lineJoin = val;
+ },
+ setTextAlign: function (val) {
+ ctx.textAlign = val;
+ },
+ setMiterLimit: function (val) {
+ ctx.miterLimit = val;
+ },
+ setShadow: function (offsetX, offsetY, blur, color) {
+ ctx.shadowOffsetX = offsetX;
+ ctx.shadowOffsetY = offsetY;
+ ctx.shadowBlur = blur;
+ ctx.shadowColor = color;
+ },
+ setTextBaseline: function (val) {
+ ctx.textBaseline = val;
+ },
+ createCircularGradient: function () { },
+ draw: function () { },
+ });
+}
+exports.adaptor = adaptor;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.js
new file mode 100644
index 0000000..e131e4b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.js
@@ -0,0 +1,207 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var version_1 = require("../common/version");
+var canvas_1 = require("./canvas");
+function format(rate) {
+ return Math.min(Math.max(rate, 0), 100);
+}
+var PERIMETER = 2 * Math.PI;
+var BEGIN_ANGLE = -Math.PI / 2;
+var STEP = 1;
+(0, component_1.VantComponent)({
+ props: {
+ text: String,
+ lineCap: {
+ type: String,
+ value: 'round',
+ },
+ value: {
+ type: Number,
+ value: 0,
+ observer: 'reRender',
+ },
+ speed: {
+ type: Number,
+ value: 50,
+ },
+ size: {
+ type: Number,
+ value: 100,
+ observer: function () {
+ this.drawCircle(this.currentValue);
+ },
+ },
+ fill: String,
+ layerColor: {
+ type: String,
+ value: color_1.WHITE,
+ },
+ color: {
+ type: null,
+ value: color_1.BLUE,
+ observer: function () {
+ var _this = this;
+ this.setHoverColor().then(function () {
+ _this.drawCircle(_this.currentValue);
+ });
+ },
+ },
+ type: {
+ type: String,
+ value: '',
+ },
+ strokeWidth: {
+ type: Number,
+ value: 4,
+ },
+ clockwise: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ hoverColor: color_1.BLUE,
+ },
+ methods: {
+ getContext: function () {
+ var _this = this;
+ var _a = this.data, type = _a.type, size = _a.size;
+ if (type === '' || !(0, version_1.canIUseCanvas2d)()) {
+ var ctx = wx.createCanvasContext('van-circle', this);
+ return Promise.resolve(ctx);
+ }
+ var dpr = (0, utils_1.getSystemInfoSync)().pixelRatio;
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(_this)
+ .select('#van-circle')
+ .node()
+ .exec(function (res) {
+ var canvas = res[0].node;
+ var ctx = canvas.getContext(type);
+ if (!_this.inited) {
+ _this.inited = true;
+ canvas.width = size * dpr;
+ canvas.height = size * dpr;
+ ctx.scale(dpr, dpr);
+ }
+ resolve((0, canvas_1.adaptor)(ctx));
+ });
+ });
+ },
+ setHoverColor: function () {
+ var _this = this;
+ var _a = this.data, color = _a.color, size = _a.size;
+ if ((0, validator_1.isObj)(color)) {
+ return this.getContext().then(function (context) {
+ if (!context)
+ return;
+ var LinearColor = context.createLinearGradient(size, 0, 0, 0);
+ Object.keys(color)
+ .sort(function (a, b) { return parseFloat(a) - parseFloat(b); })
+ .map(function (key) {
+ return LinearColor.addColorStop(parseFloat(key) / 100, color[key]);
+ });
+ _this.hoverColor = LinearColor;
+ });
+ }
+ this.hoverColor = color;
+ return Promise.resolve();
+ },
+ presetCanvas: function (context, strokeStyle, beginAngle, endAngle, fill) {
+ var _a = this.data, strokeWidth = _a.strokeWidth, lineCap = _a.lineCap, clockwise = _a.clockwise, size = _a.size;
+ var position = size / 2;
+ var radius = position - strokeWidth / 2;
+ context.setStrokeStyle(strokeStyle);
+ context.setLineWidth(strokeWidth);
+ context.setLineCap(lineCap);
+ context.beginPath();
+ context.arc(position, position, radius, beginAngle, endAngle, !clockwise);
+ context.stroke();
+ if (fill) {
+ context.setFillStyle(fill);
+ context.fill();
+ }
+ },
+ renderLayerCircle: function (context) {
+ var _a = this.data, layerColor = _a.layerColor, fill = _a.fill;
+ this.presetCanvas(context, layerColor, 0, PERIMETER, fill);
+ },
+ renderHoverCircle: function (context, formatValue) {
+ var clockwise = this.data.clockwise;
+ // 结束角度
+ var progress = PERIMETER * (formatValue / 100);
+ var endAngle = clockwise
+ ? BEGIN_ANGLE + progress
+ : 3 * Math.PI - (BEGIN_ANGLE + progress);
+ this.presetCanvas(context, this.hoverColor, BEGIN_ANGLE, endAngle);
+ },
+ drawCircle: function (currentValue) {
+ var _this = this;
+ var size = this.data.size;
+ this.getContext().then(function (context) {
+ if (!context)
+ return;
+ context.clearRect(0, 0, size, size);
+ _this.renderLayerCircle(context);
+ var formatValue = format(currentValue);
+ if (formatValue !== 0) {
+ _this.renderHoverCircle(context, formatValue);
+ }
+ context.draw();
+ });
+ },
+ reRender: function () {
+ var _this = this;
+ // tofector 动画暂时没有想到好的解决方案
+ var _a = this.data, value = _a.value, speed = _a.speed;
+ if (speed <= 0 || speed > 1000) {
+ this.drawCircle(value);
+ return;
+ }
+ this.clearMockInterval();
+ this.currentValue = this.currentValue || 0;
+ var run = function () {
+ _this.interval = setTimeout(function () {
+ if (_this.currentValue !== value) {
+ if (Math.abs(_this.currentValue - value) < STEP) {
+ _this.currentValue = value;
+ }
+ else if (_this.currentValue < value) {
+ _this.currentValue += STEP;
+ }
+ else {
+ _this.currentValue -= STEP;
+ }
+ _this.drawCircle(_this.currentValue);
+ run();
+ }
+ else {
+ _this.clearMockInterval();
+ }
+ }, 1000 / speed);
+ };
+ run();
+ },
+ clearMockInterval: function () {
+ if (this.interval) {
+ clearTimeout(this.interval);
+ this.interval = null;
+ }
+ },
+ },
+ mounted: function () {
+ var _this = this;
+ this.currentValue = this.data.value;
+ this.setHoverColor().then(function () {
+ _this.drawCircle(_this.currentValue);
+ });
+ },
+ destroyed: function () {
+ this.clearMockInterval();
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxml
new file mode 100644
index 0000000..52bc59f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxss
new file mode 100644
index 0000000..2200751
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/circle/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-circle{display:inline-block;position:relative;text-align:center}.van-circle__text{color:var(--circle-text-color,#323233);left:0;position:absolute;top:50%;transform:translateY(-50%);width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.js
new file mode 100644
index 0000000..63c56eb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('row'),
+ props: {
+ span: Number,
+ offset: Number,
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxml
new file mode 100644
index 0000000..975348b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxs
new file mode 100644
index 0000000..507c1cb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'padding-right': addUnit(data.gutter / 2),
+ 'padding-left': addUnit(data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxss
new file mode 100644
index 0000000..2fa265e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/col/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-col{box-sizing:border-box;float:left}.van-col--1{width:4.16666667%}.van-col--offset-1{margin-left:4.16666667%}.van-col--2{width:8.33333333%}.van-col--offset-2{margin-left:8.33333333%}.van-col--3{width:12.5%}.van-col--offset-3{margin-left:12.5%}.van-col--4{width:16.66666667%}.van-col--offset-4{margin-left:16.66666667%}.van-col--5{width:20.83333333%}.van-col--offset-5{margin-left:20.83333333%}.van-col--6{width:25%}.van-col--offset-6{margin-left:25%}.van-col--7{width:29.16666667%}.van-col--offset-7{margin-left:29.16666667%}.van-col--8{width:33.33333333%}.van-col--offset-8{margin-left:33.33333333%}.van-col--9{width:37.5%}.van-col--offset-9{margin-left:37.5%}.van-col--10{width:41.66666667%}.van-col--offset-10{margin-left:41.66666667%}.van-col--11{width:45.83333333%}.van-col--offset-11{margin-left:45.83333333%}.van-col--12{width:50%}.van-col--offset-12{margin-left:50%}.van-col--13{width:54.16666667%}.van-col--offset-13{margin-left:54.16666667%}.van-col--14{width:58.33333333%}.van-col--offset-14{margin-left:58.33333333%}.van-col--15{width:62.5%}.van-col--offset-15{margin-left:62.5%}.van-col--16{width:66.66666667%}.van-col--offset-16{margin-left:66.66666667%}.van-col--17{width:70.83333333%}.van-col--offset-17{margin-left:70.83333333%}.van-col--18{width:75%}.van-col--offset-18{margin-left:75%}.van-col--19{width:79.16666667%}.van-col--offset-19{margin-left:79.16666667%}.van-col--20{width:83.33333333%}.van-col--offset-20{margin-left:83.33333333%}.van-col--21{width:87.5%}.van-col--offset-21{margin-left:87.5%}.van-col--22{width:91.66666667%}.van-col--offset-22{margin-left:91.66666667%}.van-col--23{width:95.83333333%}.van-col--offset-23{margin-left:95.83333333%}.van-col--24{width:100%}.van-col--offset-24{margin-left:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.d.ts
new file mode 100644
index 0000000..32157b6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.d.ts
@@ -0,0 +1,2 @@
+///
+export declare function setContentAnimate(context: WechatMiniprogram.Component.TrivialInstance, expanded: boolean, mounted: boolean): void;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.js
new file mode 100644
index 0000000..5734087
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/animate.js
@@ -0,0 +1,43 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.setContentAnimate = void 0;
+var utils_1 = require("../common/utils");
+function useAnimation(context, expanded, mounted, height) {
+ var animation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'ease-in-out',
+ });
+ if (expanded) {
+ if (height === 0) {
+ animation.height('auto').top(1).step();
+ }
+ else {
+ animation
+ .height(height)
+ .top(1)
+ .step({
+ duration: mounted ? 300 : 1,
+ })
+ .height('auto')
+ .step();
+ }
+ context.setData({
+ animation: animation.export(),
+ });
+ return;
+ }
+ animation.height(height).top(0).step({ duration: 1 }).height(0).step({
+ duration: 300,
+ });
+ context.setData({
+ animation: animation.export(),
+ });
+}
+function setContentAnimate(context, expanded, mounted) {
+ (0, utils_1.getRect)(context, '.van-collapse-item__content')
+ .then(function (rect) { return rect.height; })
+ .then(function (height) {
+ useAnimation(context, expanded, mounted, height);
+ });
+}
+exports.setContentAnimate = setContentAnimate;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.js
new file mode 100644
index 0000000..982490e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.js
@@ -0,0 +1,62 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var animate_1 = require("./animate");
+(0, component_1.VantComponent)({
+ classes: ['title-class', 'content-class'],
+ relation: (0, relation_1.useParent)('collapse'),
+ props: {
+ size: String,
+ name: null,
+ title: null,
+ value: null,
+ icon: String,
+ label: String,
+ disabled: Boolean,
+ clickable: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ isLink: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ expanded: false,
+ },
+ mounted: function () {
+ this.updateExpanded();
+ this.mounted = true;
+ },
+ methods: {
+ updateExpanded: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent.data, value = _a.value, accordion = _a.accordion;
+ var _b = this.parent.children, children = _b === void 0 ? [] : _b;
+ var name = this.data.name;
+ var index = children.indexOf(this);
+ var currentName = name == null ? index : name;
+ var expanded = accordion
+ ? value === currentName
+ : (value || []).some(function (name) { return name === currentName; });
+ if (expanded !== this.data.expanded) {
+ (0, animate_1.setContentAnimate)(this, expanded, this.mounted);
+ }
+ this.setData({ index: index, expanded: expanded });
+ },
+ onClick: function () {
+ if (this.data.disabled) {
+ return;
+ }
+ var _a = this.data, name = _a.name, expanded = _a.expanded;
+ var index = this.parent.children.indexOf(this);
+ var currentName = name == null ? index : name;
+ this.parent.switch(currentName, !expanded);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxml
new file mode 100644
index 0000000..f11d0d4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxml
@@ -0,0 +1,45 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxss
new file mode 100644
index 0000000..4a65b5a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-collapse-item__title .van-cell__right-icon{transform:rotate(90deg);transition:transform var(--collapse-item-transition-duration,.3s)}.van-collapse-item__title--expanded .van-cell__right-icon{transform:rotate(-90deg)}.van-collapse-item__title--disabled .van-cell,.van-collapse-item__title--disabled .van-cell__right-icon{color:var(--collapse-item-title-disabled-color,#c8c9cc)!important}.van-collapse-item__title--disabled .van-cell--hover{background-color:#fff!important}.van-collapse-item__wrapper{overflow:hidden}.van-collapse-item__content{background-color:var(--collapse-item-content-background-color,#fff);color:var(--collapse-item-content-text-color,#969799);font-size:var(--collapse-item-content-font-size,13px);line-height:var(--collapse-item-content-line-height,1.5);padding:var(--collapse-item-content-padding,15px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.js
new file mode 100644
index 0000000..943d542
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.js
@@ -0,0 +1,48 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('collapse-item'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateExpanded',
+ },
+ accordion: {
+ type: Boolean,
+ observer: 'updateExpanded',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updateExpanded: function () {
+ this.children.forEach(function (child) {
+ child.updateExpanded();
+ });
+ },
+ switch: function (name, expanded) {
+ var _a = this.data, accordion = _a.accordion, value = _a.value;
+ var changeItem = name;
+ if (!accordion) {
+ name = expanded
+ ? (value || []).concat(name)
+ : (value || []).filter(function (activeName) { return activeName !== name; });
+ }
+ else {
+ name = expanded ? name : '';
+ }
+ if (expanded) {
+ this.$emit('open', changeItem);
+ }
+ else {
+ this.$emit('close', changeItem);
+ }
+ this.$emit('change', name);
+ this.$emit('input', name);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxml
new file mode 100644
index 0000000..fd4e171
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxml
@@ -0,0 +1,3 @@
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/collapse/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.d.ts
new file mode 100644
index 0000000..386f307
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.d.ts
@@ -0,0 +1,7 @@
+export declare const RED = "#ee0a24";
+export declare const BLUE = "#1989fa";
+export declare const WHITE = "#fff";
+export declare const GREEN = "#07c160";
+export declare const ORANGE = "#ff976a";
+export declare const GRAY = "#323233";
+export declare const GRAY_DARK = "#969799";
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.js
new file mode 100644
index 0000000..008a45a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/color.js
@@ -0,0 +1,10 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.GRAY_DARK = exports.GRAY = exports.ORANGE = exports.GREEN = exports.WHITE = exports.BLUE = exports.RED = void 0;
+exports.RED = '#ee0a24';
+exports.BLUE = '#1989fa';
+exports.WHITE = '#fff';
+exports.GREEN = '#07c160';
+exports.ORANGE = '#ff976a';
+exports.GRAY = '#323233';
+exports.GRAY_DARK = '#969799';
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.d.ts
new file mode 100644
index 0000000..1d0fd27
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.d.ts
@@ -0,0 +1,4 @@
+///
+import { VantComponentOptions } from 'definitions/index';
+declare function VantComponent(vantOptions: VantComponentOptions): void;
+export { VantComponent };
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.js
new file mode 100644
index 0000000..66da00e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/component.js
@@ -0,0 +1,49 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.VantComponent = void 0;
+var basic_1 = require("../mixins/basic");
+function mapKeys(source, target, map) {
+ Object.keys(map).forEach(function (key) {
+ if (source[key]) {
+ target[map[key]] = source[key];
+ }
+ });
+}
+function VantComponent(vantOptions) {
+ var options = {};
+ mapKeys(vantOptions, options, {
+ data: 'data',
+ props: 'properties',
+ watch: 'observers',
+ mixins: 'behaviors',
+ methods: 'methods',
+ beforeCreate: 'created',
+ created: 'attached',
+ mounted: 'ready',
+ destroyed: 'detached',
+ classes: 'externalClasses',
+ });
+ // add default externalClasses
+ options.externalClasses = options.externalClasses || [];
+ options.externalClasses.push('custom-class');
+ // add default behaviors
+ options.behaviors = options.behaviors || [];
+ options.behaviors.push(basic_1.basic);
+ // add relations
+ var relation = vantOptions.relation;
+ if (relation) {
+ options.relations = relation.relations;
+ options.behaviors.push(relation.mixin);
+ }
+ // map field to form-field behavior
+ if (vantOptions.field) {
+ options.behaviors.push('wx://form-field');
+ }
+ // add default options
+ options.options = {
+ multipleSlots: true,
+ addGlobalClass: true,
+ };
+ Component(options);
+}
+exports.VantComponent = VantComponent;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/index.wxss
new file mode 100644
index 0000000..a73bb7a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/index.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}.van-clearfix:after{clear:both;content:"";display:table}.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.d.ts
new file mode 100644
index 0000000..10193fa
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.d.ts
@@ -0,0 +1,15 @@
+///
+type TrivialInstance = WechatMiniprogram.Component.TrivialInstance;
+export declare function useParent(name: string, onEffect?: (this: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export declare function useChildren(name: string, onEffect?: (this: TrivialInstance, target: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.js
new file mode 100644
index 0000000..008256c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/relation.js
@@ -0,0 +1,65 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.useChildren = exports.useParent = void 0;
+function useParent(name, onEffect) {
+ var _a;
+ var path = "../".concat(name, "/index");
+ return {
+ relations: (_a = {},
+ _a[path] = {
+ type: 'ancestor',
+ linked: function () {
+ onEffect && onEffect.call(this);
+ },
+ linkChanged: function () {
+ onEffect && onEffect.call(this);
+ },
+ unlinked: function () {
+ onEffect && onEffect.call(this);
+ },
+ },
+ _a),
+ mixin: Behavior({
+ created: function () {
+ var _this = this;
+ Object.defineProperty(this, 'parent', {
+ get: function () { return _this.getRelationNodes(path)[0]; },
+ });
+ Object.defineProperty(this, 'index', {
+ // @ts-ignore
+ get: function () { var _a, _b; return (_b = (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.children) === null || _b === void 0 ? void 0 : _b.indexOf(_this); },
+ });
+ },
+ }),
+ };
+}
+exports.useParent = useParent;
+function useChildren(name, onEffect) {
+ var _a;
+ var path = "../".concat(name, "/index");
+ return {
+ relations: (_a = {},
+ _a[path] = {
+ type: 'descendant',
+ linked: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ linkChanged: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ unlinked: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ },
+ _a),
+ mixin: Behavior({
+ created: function () {
+ var _this = this;
+ Object.defineProperty(this, 'children', {
+ get: function () { return _this.getRelationNodes(path) || []; },
+ });
+ },
+ }),
+ };
+}
+exports.useChildren = useChildren;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/clearfix.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/clearfix.wxss
new file mode 100644
index 0000000..442246f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/clearfix.wxss
@@ -0,0 +1 @@
+.van-clearfix:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/ellipsis.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/ellipsis.wxss
new file mode 100644
index 0000000..ee701df
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/ellipsis.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/hairline.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/hairline.wxss
new file mode 100644
index 0000000..f7c6260
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/hairline.wxss
@@ -0,0 +1 @@
+.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/clearfix.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/clearfix.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/ellipsis.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/ellipsis.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/hairline.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/mixins/hairline.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/var.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/style/var.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.d.ts
new file mode 100644
index 0000000..a77d8c6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.d.ts
@@ -0,0 +1,21 @@
+///
+///
+///
+///
+///
+export { isDef } from './validator';
+export { getSystemInfoSync } from './version';
+export declare function range(num: number, min: number, max: number): number;
+export declare function nextTick(cb: (...args: any[]) => void): void;
+export declare function addUnit(value?: string | number): string | undefined;
+export declare function requestAnimationFrame(cb: () => void): NodeJS.Timeout;
+export declare function pickExclude(obj: unknown, keys: string[]): {};
+export declare function getRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function getAllRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function groupSetData(context: WechatMiniprogram.Component.TrivialInstance, cb: () => void): void;
+export declare function toPromise(promiseLike: Promise | unknown): Promise;
+export declare function addNumber(num1: any, num2: any): number;
+export declare const clamp: (num: any, min: any, max: any) => number;
+export declare function getCurrentPage(): T & WechatMiniprogram.OptionalInterface & WechatMiniprogram.Page.InstanceProperties & WechatMiniprogram.Page.InstanceMethods & WechatMiniprogram.Page.Data & WechatMiniprogram.IAnyObject;
+export declare const isPC: boolean;
+export declare const isWxWork: boolean;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.js
new file mode 100644
index 0000000..1727628
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/utils.js
@@ -0,0 +1,109 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isWxWork = exports.isPC = exports.getCurrentPage = exports.clamp = exports.addNumber = exports.toPromise = exports.groupSetData = exports.getAllRect = exports.getRect = exports.pickExclude = exports.requestAnimationFrame = exports.addUnit = exports.nextTick = exports.range = exports.getSystemInfoSync = exports.isDef = void 0;
+var validator_1 = require("./validator");
+var version_1 = require("./version");
+var validator_2 = require("./validator");
+Object.defineProperty(exports, "isDef", { enumerable: true, get: function () { return validator_2.isDef; } });
+var version_2 = require("./version");
+Object.defineProperty(exports, "getSystemInfoSync", { enumerable: true, get: function () { return version_2.getSystemInfoSync; } });
+function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+exports.range = range;
+function nextTick(cb) {
+ if ((0, version_1.canIUseNextTick)()) {
+ wx.nextTick(cb);
+ }
+ else {
+ setTimeout(function () {
+ cb();
+ }, 1000 / 30);
+ }
+}
+exports.nextTick = nextTick;
+function addUnit(value) {
+ if (!(0, validator_1.isDef)(value)) {
+ return undefined;
+ }
+ value = String(value);
+ return (0, validator_1.isNumber)(value) ? "".concat(value, "px") : value;
+}
+exports.addUnit = addUnit;
+function requestAnimationFrame(cb) {
+ return setTimeout(function () {
+ cb();
+ }, 1000 / 30);
+}
+exports.requestAnimationFrame = requestAnimationFrame;
+function pickExclude(obj, keys) {
+ if (!(0, validator_1.isPlainObject)(obj)) {
+ return {};
+ }
+ return Object.keys(obj).reduce(function (prev, key) {
+ if (!keys.includes(key)) {
+ prev[key] = obj[key];
+ }
+ return prev;
+ }, {});
+}
+exports.pickExclude = pickExclude;
+function getRect(context, selector) {
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(context)
+ .select(selector)
+ .boundingClientRect()
+ .exec(function (rect) {
+ if (rect === void 0) { rect = []; }
+ return resolve(rect[0]);
+ });
+ });
+}
+exports.getRect = getRect;
+function getAllRect(context, selector) {
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(context)
+ .selectAll(selector)
+ .boundingClientRect()
+ .exec(function (rect) {
+ if (rect === void 0) { rect = []; }
+ return resolve(rect[0]);
+ });
+ });
+}
+exports.getAllRect = getAllRect;
+function groupSetData(context, cb) {
+ if ((0, version_1.canIUseGroupSetData)()) {
+ context.groupSetData(cb);
+ }
+ else {
+ cb();
+ }
+}
+exports.groupSetData = groupSetData;
+function toPromise(promiseLike) {
+ if ((0, validator_1.isPromise)(promiseLike)) {
+ return promiseLike;
+ }
+ return Promise.resolve(promiseLike);
+}
+exports.toPromise = toPromise;
+// 浮点数精度处理
+function addNumber(num1, num2) {
+ var cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+exports.addNumber = addNumber;
+// 限制value在[min, max]之间
+var clamp = function (num, min, max) { return Math.min(Math.max(num, min), max); };
+exports.clamp = clamp;
+function getCurrentPage() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+exports.getCurrentPage = getCurrentPage;
+exports.isPC = ['mac', 'windows'].includes((0, version_1.getSystemInfoSync)().platform);
+// 是否企业微信
+exports.isWxWork = (0, version_1.getSystemInfoSync)().environment === 'wxwork';
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.d.ts
new file mode 100644
index 0000000..152894a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.d.ts
@@ -0,0 +1,9 @@
+export declare function isFunction(val: unknown): val is Function;
+export declare function isPlainObject(val: unknown): val is Record;
+export declare function isPromise(val: unknown): val is Promise;
+export declare function isDef(value: unknown): boolean;
+export declare function isObj(x: unknown): x is Record;
+export declare function isNumber(value: string): boolean;
+export declare function isBoolean(value: unknown): value is boolean;
+export declare function isImageUrl(url: string): boolean;
+export declare function isVideoUrl(url: string): boolean;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.js
new file mode 100644
index 0000000..169e796
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/validator.js
@@ -0,0 +1,43 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isVideoUrl = exports.isImageUrl = exports.isBoolean = exports.isNumber = exports.isObj = exports.isDef = exports.isPromise = exports.isPlainObject = exports.isFunction = void 0;
+// eslint-disable-next-line @typescript-eslint/ban-types
+function isFunction(val) {
+ return typeof val === 'function';
+}
+exports.isFunction = isFunction;
+function isPlainObject(val) {
+ return val !== null && typeof val === 'object' && !Array.isArray(val);
+}
+exports.isPlainObject = isPlainObject;
+function isPromise(val) {
+ return isPlainObject(val) && isFunction(val.then) && isFunction(val.catch);
+}
+exports.isPromise = isPromise;
+function isDef(value) {
+ return value !== undefined && value !== null;
+}
+exports.isDef = isDef;
+function isObj(x) {
+ var type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+exports.isObj = isObj;
+function isNumber(value) {
+ return /^\d+(\.\d+)?$/.test(value);
+}
+exports.isNumber = isNumber;
+function isBoolean(value) {
+ return typeof value === 'boolean';
+}
+exports.isBoolean = isBoolean;
+var IMAGE_REGEXP = /\.(jpeg|jpg|gif|png|svg|webp|jfif|bmp|dpg)/i;
+var VIDEO_REGEXP = /\.(mp4|mpg|mpeg|dat|asf|avi|rm|rmvb|mov|wmv|flv|mkv)/i;
+function isImageUrl(url) {
+ return IMAGE_REGEXP.test(url);
+}
+exports.isImageUrl = isImageUrl;
+function isVideoUrl(url) {
+ return VIDEO_REGEXP.test(url);
+}
+exports.isVideoUrl = isVideoUrl;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.d.ts
new file mode 100644
index 0000000..3393221
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.d.ts
@@ -0,0 +1,15 @@
+///
+interface WxWorkSystemInfo extends WechatMiniprogram.SystemInfo {
+ environment?: 'wxwork';
+}
+interface SystemInfo extends WxWorkSystemInfo, WechatMiniprogram.SystemInfo {
+}
+export declare function getSystemInfoSync(): SystemInfo;
+export declare function canIUseModel(): boolean;
+export declare function canIUseFormFieldButton(): boolean;
+export declare function canIUseAnimate(): boolean;
+export declare function canIUseGroupSetData(): boolean;
+export declare function canIUseNextTick(): boolean;
+export declare function canIUseCanvas2d(): boolean;
+export declare function canIUseGetUserProfile(): boolean;
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.js
new file mode 100644
index 0000000..5937008
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/common/version.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.canIUseGetUserProfile = exports.canIUseCanvas2d = exports.canIUseNextTick = exports.canIUseGroupSetData = exports.canIUseAnimate = exports.canIUseFormFieldButton = exports.canIUseModel = exports.getSystemInfoSync = void 0;
+var systemInfo;
+function getSystemInfoSync() {
+ if (systemInfo == null) {
+ systemInfo = wx.getSystemInfoSync();
+ }
+ return systemInfo;
+}
+exports.getSystemInfoSync = getSystemInfoSync;
+function compareVersion(v1, v2) {
+ v1 = v1.split('.');
+ v2 = v2.split('.');
+ var len = Math.max(v1.length, v2.length);
+ while (v1.length < len) {
+ v1.push('0');
+ }
+ while (v2.length < len) {
+ v2.push('0');
+ }
+ for (var i = 0; i < len; i++) {
+ var num1 = parseInt(v1[i], 10);
+ var num2 = parseInt(v2[i], 10);
+ if (num1 > num2) {
+ return 1;
+ }
+ if (num1 < num2) {
+ return -1;
+ }
+ }
+ return 0;
+}
+function gte(version) {
+ var system = getSystemInfoSync();
+ return compareVersion(system.SDKVersion, version) >= 0;
+}
+function canIUseModel() {
+ return gte('2.9.3');
+}
+exports.canIUseModel = canIUseModel;
+function canIUseFormFieldButton() {
+ return gte('2.10.3');
+}
+exports.canIUseFormFieldButton = canIUseFormFieldButton;
+function canIUseAnimate() {
+ return gte('2.9.0');
+}
+exports.canIUseAnimate = canIUseAnimate;
+function canIUseGroupSetData() {
+ return gte('2.4.0');
+}
+exports.canIUseGroupSetData = canIUseGroupSetData;
+function canIUseNextTick() {
+ try {
+ return wx.canIUse('nextTick');
+ }
+ catch (e) {
+ return gte('2.7.1');
+ }
+}
+exports.canIUseNextTick = canIUseNextTick;
+function canIUseCanvas2d() {
+ return gte('2.9.0');
+}
+exports.canIUseCanvas2d = canIUseCanvas2d;
+function canIUseGetUserProfile() {
+ return !!wx.getUserProfile;
+}
+exports.canIUseGetUserProfile = canIUseGetUserProfile;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.js
new file mode 100644
index 0000000..21fb1c4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ themeVars: {
+ type: Object,
+ value: {},
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxml
new file mode 100644
index 0000000..3cfb461
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxs
new file mode 100644
index 0000000..7ca0203
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/config-provider/index.wxs
@@ -0,0 +1,29 @@
+/* eslint-disable */
+var object = require('../wxs/object.wxs');
+var style = require('../wxs/style.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+ .replace(getRegExp("^-"), '');
+
+ return newWord;
+}
+
+function mapThemeVarsToCSSVars(themeVars) {
+ var cssVars = {};
+ object.keys(themeVars).forEach(function (key) {
+ var cssVarsKey = '--' + kebabCase(key);
+ cssVars[cssVarsKey] = themeVars[key];
+ });
+
+ return style(cssVars);
+}
+
+module.exports = {
+ kebabCase: kebabCase,
+ mapThemeVarsToCSSVars: mapThemeVarsToCSSVars,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.js
new file mode 100644
index 0000000..afc780b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.js
@@ -0,0 +1,104 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+function simpleTick(fn) {
+ return setTimeout(fn, 30);
+}
+(0, component_1.VantComponent)({
+ props: {
+ useSlot: Boolean,
+ millisecond: Boolean,
+ time: {
+ type: Number,
+ observer: 'reset',
+ },
+ format: {
+ type: String,
+ value: 'HH:mm:ss',
+ },
+ autoStart: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ timeData: (0, utils_1.parseTimeData)(0),
+ formattedTime: '0',
+ },
+ destroyed: function () {
+ clearTimeout(this.tid);
+ this.tid = null;
+ },
+ methods: {
+ // 开始
+ start: function () {
+ if (this.counting) {
+ return;
+ }
+ this.counting = true;
+ this.endTime = Date.now() + this.remain;
+ this.tick();
+ },
+ // 暂停
+ pause: function () {
+ this.counting = false;
+ clearTimeout(this.tid);
+ },
+ // 重置
+ reset: function () {
+ this.pause();
+ this.remain = this.data.time;
+ this.setRemain(this.remain);
+ if (this.data.autoStart) {
+ this.start();
+ }
+ },
+ tick: function () {
+ if (this.data.millisecond) {
+ this.microTick();
+ }
+ else {
+ this.macroTick();
+ }
+ },
+ microTick: function () {
+ var _this = this;
+ this.tid = simpleTick(function () {
+ _this.setRemain(_this.getRemain());
+ if (_this.remain !== 0) {
+ _this.microTick();
+ }
+ });
+ },
+ macroTick: function () {
+ var _this = this;
+ this.tid = simpleTick(function () {
+ var remain = _this.getRemain();
+ if (!(0, utils_1.isSameSecond)(remain, _this.remain) || remain === 0) {
+ _this.setRemain(remain);
+ }
+ if (_this.remain !== 0) {
+ _this.macroTick();
+ }
+ });
+ },
+ getRemain: function () {
+ return Math.max(this.endTime - Date.now(), 0);
+ },
+ setRemain: function (remain) {
+ this.remain = remain;
+ var timeData = (0, utils_1.parseTimeData)(remain);
+ if (this.data.useSlot) {
+ this.$emit('change', timeData);
+ }
+ this.setData({
+ formattedTime: (0, utils_1.parseFormat)(this.data.format, timeData),
+ });
+ if (remain === 0) {
+ this.pause();
+ this.$emit('finish');
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxml
new file mode 100644
index 0000000..e206e16
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxml
@@ -0,0 +1,4 @@
+
+
+ {{ formattedTime }}
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxss
new file mode 100644
index 0000000..8b957f7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-count-down{color:var(--count-down-text-color,#323233);font-size:var(--count-down-font-size,14px);line-height:var(--count-down-line-height,20px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.d.ts
new file mode 100644
index 0000000..876a6c1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.d.ts
@@ -0,0 +1,10 @@
+export type TimeData = {
+ days: number;
+ hours: number;
+ minutes: number;
+ seconds: number;
+ milliseconds: number;
+};
+export declare function parseTimeData(time: number): TimeData;
+export declare function parseFormat(format: string, timeData: TimeData): string;
+export declare function isSameSecond(time1: number, time2: number): boolean;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.js
new file mode 100644
index 0000000..a7cfa5f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/count-down/utils.js
@@ -0,0 +1,64 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isSameSecond = exports.parseFormat = exports.parseTimeData = void 0;
+function padZero(num, targetLength) {
+ if (targetLength === void 0) { targetLength = 2; }
+ var str = num + '';
+ while (str.length < targetLength) {
+ str = '0' + str;
+ }
+ return str;
+}
+var SECOND = 1000;
+var MINUTE = 60 * SECOND;
+var HOUR = 60 * MINUTE;
+var DAY = 24 * HOUR;
+function parseTimeData(time) {
+ var days = Math.floor(time / DAY);
+ var hours = Math.floor((time % DAY) / HOUR);
+ var minutes = Math.floor((time % HOUR) / MINUTE);
+ var seconds = Math.floor((time % MINUTE) / SECOND);
+ var milliseconds = Math.floor(time % SECOND);
+ return {
+ days: days,
+ hours: hours,
+ minutes: minutes,
+ seconds: seconds,
+ milliseconds: milliseconds,
+ };
+}
+exports.parseTimeData = parseTimeData;
+function parseFormat(format, timeData) {
+ var days = timeData.days;
+ var hours = timeData.hours, minutes = timeData.minutes, seconds = timeData.seconds, milliseconds = timeData.milliseconds;
+ if (format.indexOf('DD') === -1) {
+ hours += days * 24;
+ }
+ else {
+ format = format.replace('DD', padZero(days));
+ }
+ if (format.indexOf('HH') === -1) {
+ minutes += hours * 60;
+ }
+ else {
+ format = format.replace('HH', padZero(hours));
+ }
+ if (format.indexOf('mm') === -1) {
+ seconds += minutes * 60;
+ }
+ else {
+ format = format.replace('mm', padZero(minutes));
+ }
+ if (format.indexOf('ss') === -1) {
+ milliseconds += seconds * 1000;
+ }
+ else {
+ format = format.replace('ss', padZero(seconds));
+ }
+ return format.replace('SSS', padZero(milliseconds, 3));
+}
+exports.parseFormat = parseFormat;
+function isSameSecond(time1, time2) {
+ return Math.floor(time1 / 1000) === Math.floor(time2 / 1000);
+}
+exports.isSameSecond = isSameSecond;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.js
new file mode 100644
index 0000000..e30afef
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.js
@@ -0,0 +1,329 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var shared_1 = require("../picker/shared");
+var currentYear = new Date().getFullYear();
+function isValidDate(date) {
+ return (0, validator_1.isDef)(date) && !isNaN(new Date(date).getTime());
+}
+function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+function padZero(val) {
+ return "00".concat(val).slice(-2);
+}
+function times(n, iteratee) {
+ var index = -1;
+ var result = Array(n < 0 ? 0 : n);
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+function getTrueValue(formattedValue) {
+ if (formattedValue === undefined) {
+ formattedValue = '1';
+ }
+ while (isNaN(parseInt(formattedValue, 10))) {
+ formattedValue = formattedValue.slice(1);
+ }
+ return parseInt(formattedValue, 10);
+}
+function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+var defaultFormatter = function (type, value) { return value; };
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { value: {
+ type: null,
+ observer: 'updateValue',
+ }, filter: null, type: {
+ type: String,
+ value: 'datetime',
+ observer: 'updateValue',
+ }, showToolbar: {
+ type: Boolean,
+ value: true,
+ }, formatter: {
+ type: null,
+ value: defaultFormatter,
+ }, minDate: {
+ type: Number,
+ value: new Date(currentYear - 10, 0, 1).getTime(),
+ observer: 'updateValue',
+ }, maxDate: {
+ type: Number,
+ value: new Date(currentYear + 10, 11, 31).getTime(),
+ observer: 'updateValue',
+ }, minHour: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxHour: {
+ type: Number,
+ value: 23,
+ observer: 'updateValue',
+ }, minMinute: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxMinute: {
+ type: Number,
+ value: 59,
+ observer: 'updateValue',
+ } }),
+ data: {
+ innerValue: Date.now(),
+ columns: [],
+ },
+ methods: {
+ updateValue: function () {
+ var _this = this;
+ var data = this.data;
+ var val = this.correctValue(data.value);
+ var isEqual = val === data.innerValue;
+ this.updateColumnValue(val).then(function () {
+ if (!isEqual) {
+ _this.$emit('input', val);
+ }
+ });
+ },
+ getPicker: function () {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-datetime-picker');
+ var picker_1 = this.picker;
+ var setColumnValues_1 = picker_1.setColumnValues;
+ picker_1.setColumnValues = function () {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ return setColumnValues_1.apply(picker_1, __spreadArray(__spreadArray([], args, true), [false], false));
+ };
+ }
+ return this.picker;
+ },
+ updateColumns: function () {
+ var _a = this.data.formatter, formatter = _a === void 0 ? defaultFormatter : _a;
+ var results = this.getOriginColumns().map(function (column) { return ({
+ values: column.values.map(function (value) { return formatter(column.type, value); }),
+ }); });
+ return this.set({ columns: results });
+ },
+ getOriginColumns: function () {
+ var filter = this.data.filter;
+ var results = this.getRanges().map(function (_a) {
+ var type = _a.type, range = _a.range;
+ var values = times(range[1] - range[0] + 1, function (index) {
+ var value = range[0] + index;
+ return type === 'year' ? "".concat(value) : padZero(value);
+ });
+ if (filter) {
+ values = filter(type, values);
+ }
+ return { type: type, values: values };
+ });
+ return results;
+ },
+ getRanges: function () {
+ var data = this.data;
+ if (data.type === 'time') {
+ return [
+ {
+ type: 'hour',
+ range: [data.minHour, data.maxHour],
+ },
+ {
+ type: 'minute',
+ range: [data.minMinute, data.maxMinute],
+ },
+ ];
+ }
+ var _a = this.getBoundary('max', data.innerValue), maxYear = _a.maxYear, maxDate = _a.maxDate, maxMonth = _a.maxMonth, maxHour = _a.maxHour, maxMinute = _a.maxMinute;
+ var _b = this.getBoundary('min', data.innerValue), minYear = _b.minYear, minDate = _b.minDate, minMonth = _b.minMonth, minHour = _b.minHour, minMinute = _b.minMinute;
+ var result = [
+ {
+ type: 'year',
+ range: [minYear, maxYear],
+ },
+ {
+ type: 'month',
+ range: [minMonth, maxMonth],
+ },
+ {
+ type: 'day',
+ range: [minDate, maxDate],
+ },
+ {
+ type: 'hour',
+ range: [minHour, maxHour],
+ },
+ {
+ type: 'minute',
+ range: [minMinute, maxMinute],
+ },
+ ];
+ if (data.type === 'date')
+ result.splice(3, 2);
+ if (data.type === 'year-month')
+ result.splice(2, 3);
+ return result;
+ },
+ correctValue: function (value) {
+ var data = this.data;
+ // validate value
+ var isDateType = data.type !== 'time';
+ if (isDateType && !isValidDate(value)) {
+ value = data.minDate;
+ }
+ else if (!isDateType && !value) {
+ var minHour = data.minHour;
+ value = "".concat(padZero(minHour), ":00");
+ }
+ // time type
+ if (!isDateType) {
+ var _a = value.split(':'), hour = _a[0], minute = _a[1];
+ hour = padZero(range(hour, data.minHour, data.maxHour));
+ minute = padZero(range(minute, data.minMinute, data.maxMinute));
+ return "".concat(hour, ":").concat(minute);
+ }
+ // date type
+ value = Math.max(value, data.minDate);
+ value = Math.min(value, data.maxDate);
+ return value;
+ },
+ getBoundary: function (type, innerValue) {
+ var _a;
+ var value = new Date(innerValue);
+ var boundary = new Date(this.data["".concat(type, "Date")]);
+ var year = boundary.getFullYear();
+ var month = 1;
+ var date = 1;
+ var hour = 0;
+ var minute = 0;
+ if (type === 'max') {
+ month = 12;
+ date = getMonthEndDay(value.getFullYear(), value.getMonth() + 1);
+ hour = 23;
+ minute = 59;
+ }
+ if (value.getFullYear() === year) {
+ month = boundary.getMonth() + 1;
+ if (value.getMonth() + 1 === month) {
+ date = boundary.getDate();
+ if (value.getDate() === date) {
+ hour = boundary.getHours();
+ if (value.getHours() === hour) {
+ minute = boundary.getMinutes();
+ }
+ }
+ }
+ }
+ return _a = {},
+ _a["".concat(type, "Year")] = year,
+ _a["".concat(type, "Month")] = month,
+ _a["".concat(type, "Date")] = date,
+ _a["".concat(type, "Hour")] = hour,
+ _a["".concat(type, "Minute")] = minute,
+ _a;
+ },
+ onCancel: function () {
+ this.$emit('cancel');
+ },
+ onConfirm: function () {
+ this.$emit('confirm', this.data.innerValue);
+ },
+ onChange: function () {
+ var _this = this;
+ var data = this.data;
+ var value;
+ var picker = this.getPicker();
+ var originColumns = this.getOriginColumns();
+ if (data.type === 'time') {
+ var indexes = picker.getIndexes();
+ value = "".concat(+originColumns[0].values[indexes[0]], ":").concat(+originColumns[1]
+ .values[indexes[1]]);
+ }
+ else {
+ var indexes = picker.getIndexes();
+ var values = indexes.map(function (value, index) { return originColumns[index].values[value]; });
+ var year = getTrueValue(values[0]);
+ var month = getTrueValue(values[1]);
+ var maxDate = getMonthEndDay(year, month);
+ var date = getTrueValue(values[2]);
+ if (data.type === 'year-month') {
+ date = 1;
+ }
+ date = date > maxDate ? maxDate : date;
+ var hour = 0;
+ var minute = 0;
+ if (data.type === 'datetime') {
+ hour = getTrueValue(values[3]);
+ minute = getTrueValue(values[4]);
+ }
+ value = new Date(year, month - 1, date, hour, minute);
+ }
+ value = this.correctValue(value);
+ this.updateColumnValue(value).then(function () {
+ _this.$emit('input', value);
+ _this.$emit('change', picker);
+ });
+ },
+ updateColumnValue: function (value) {
+ var _this = this;
+ var values = [];
+ var type = this.data.type;
+ var formatter = this.data.formatter || defaultFormatter;
+ var picker = this.getPicker();
+ if (type === 'time') {
+ var pair = value.split(':');
+ values = [formatter('hour', pair[0]), formatter('minute', pair[1])];
+ }
+ else {
+ var date = new Date(value);
+ values = [
+ formatter('year', "".concat(date.getFullYear())),
+ formatter('month', padZero(date.getMonth() + 1)),
+ ];
+ if (type === 'date') {
+ values.push(formatter('day', padZero(date.getDate())));
+ }
+ if (type === 'datetime') {
+ values.push(formatter('day', padZero(date.getDate())), formatter('hour', padZero(date.getHours())), formatter('minute', padZero(date.getMinutes())));
+ }
+ }
+ return this.set({ innerValue: value })
+ .then(function () { return _this.updateColumns(); })
+ .then(function () { return picker.setValues(values); });
+ },
+ },
+ created: function () {
+ var _this = this;
+ var innerValue = this.correctValue(this.data.value);
+ this.updateColumnValue(innerValue).then(function () {
+ _this.$emit('input', innerValue);
+ });
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxml
new file mode 100644
index 0000000..ade2202
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/datetime-picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.d.ts
new file mode 100644
index 0000000..d0554f6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.d.ts
@@ -0,0 +1,28 @@
+///
+interface VantComponentInstance {
+ parent: WechatMiniprogram.Component.TrivialInstance;
+ children: WechatMiniprogram.Component.TrivialInstance[];
+ index: number;
+ $emit: (name: string, detail?: unknown, options?: WechatMiniprogram.Component.TriggerEventOption) => void;
+}
+export type VantComponentOptions = {
+ data?: Data;
+ field?: boolean;
+ classes?: string[];
+ mixins?: string[];
+ props?: Props;
+ relation?: {
+ relations: Record;
+ mixin: string;
+ };
+ watch?: Record any>;
+ methods?: Methods;
+ beforeCreate?: () => void;
+ created?: () => void;
+ mounted?: () => void;
+ destroyed?: () => void;
+} & ThisType, Props, Methods> & Record>;
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/definitions/index.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.d.ts
new file mode 100644
index 0000000..db2da5f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.d.ts
@@ -0,0 +1,55 @@
+///
+///
+export type Action = 'confirm' | 'cancel' | 'overlay';
+type DialogContext = WechatMiniprogram.Page.TrivialInstance | WechatMiniprogram.Component.TrivialInstance;
+interface DialogOptions {
+ lang?: string;
+ show?: boolean;
+ title?: string;
+ width?: string | number | null;
+ zIndex?: number;
+ theme?: string;
+ context?: (() => DialogContext) | DialogContext;
+ message?: string;
+ overlay?: boolean;
+ selector?: string;
+ ariaLabel?: string;
+ /**
+ * @deprecated use custom-class instead
+ */
+ className?: string;
+ customStyle?: string;
+ transition?: string;
+ /**
+ * @deprecated use beforeClose instead
+ */
+ asyncClose?: boolean;
+ beforeClose?: null | ((action: Action) => Promise | void);
+ businessId?: number;
+ sessionFrom?: string;
+ overlayStyle?: string;
+ appParameter?: string;
+ messageAlign?: string;
+ sendMessageImg?: string;
+ showMessageCard?: boolean;
+ sendMessagePath?: string;
+ sendMessageTitle?: string;
+ confirmButtonText?: string;
+ cancelButtonText?: string;
+ showConfirmButton?: boolean;
+ showCancelButton?: boolean;
+ closeOnClickOverlay?: boolean;
+ confirmButtonOpenType?: string;
+}
+declare const Dialog: {
+ (options: DialogOptions): Promise;
+ alert(options: DialogOptions): Promise;
+ confirm(options: DialogOptions): Promise;
+ close(): void;
+ stopLoading(): void;
+ currentOptions: DialogOptions;
+ defaultOptions: DialogOptions;
+ setDefaultOptions(options: DialogOptions): void;
+ resetDefaultOptions(): void;
+};
+export default Dialog;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.js
new file mode 100644
index 0000000..400f4f1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/dialog.js
@@ -0,0 +1,92 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var queue = [];
+var defaultOptions = {
+ show: false,
+ title: '',
+ width: null,
+ theme: 'default',
+ message: '',
+ zIndex: 100,
+ overlay: true,
+ selector: '#van-dialog',
+ className: '',
+ asyncClose: false,
+ beforeClose: null,
+ transition: 'scale',
+ customStyle: '',
+ messageAlign: '',
+ overlayStyle: '',
+ confirmButtonText: '确认',
+ cancelButtonText: '取消',
+ showConfirmButton: true,
+ showCancelButton: false,
+ closeOnClickOverlay: false,
+ confirmButtonOpenType: '',
+};
+var currentOptions = __assign({}, defaultOptions);
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+var Dialog = function (options) {
+ options = __assign(__assign({}, currentOptions), options);
+ return new Promise(function (resolve, reject) {
+ var context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ var dialog = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (dialog) {
+ dialog.setData(__assign({ callback: function (action, instance) {
+ action === 'confirm' ? resolve(instance) : reject(instance);
+ } }, options));
+ wx.nextTick(function () {
+ dialog.setData({ show: true });
+ });
+ queue.push(dialog);
+ }
+ else {
+ console.warn('未找到 van-dialog 节点,请确认 selector 及 context 是否正确');
+ }
+ });
+};
+Dialog.alert = function (options) { return Dialog(options); };
+Dialog.confirm = function (options) {
+ return Dialog(__assign({ showCancelButton: true }, options));
+};
+Dialog.close = function () {
+ queue.forEach(function (dialog) {
+ dialog.close();
+ });
+ queue = [];
+};
+Dialog.stopLoading = function () {
+ queue.forEach(function (dialog) {
+ dialog.stopLoading();
+ });
+};
+Dialog.currentOptions = currentOptions;
+Dialog.defaultOptions = defaultOptions;
+Dialog.setDefaultOptions = function (options) {
+ currentOptions = __assign(__assign({}, currentOptions), options);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions();
+exports.default = Dialog;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.js
new file mode 100644
index 0000000..b0acfa0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.js
@@ -0,0 +1,135 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ mixins: [button_1.button],
+ classes: ['cancle-button-class', 'confirm-button-class'],
+ props: {
+ show: {
+ type: Boolean,
+ observer: function (show) {
+ !show && this.stopLoading();
+ },
+ },
+ title: String,
+ message: String,
+ theme: {
+ type: String,
+ value: 'default',
+ },
+ confirmButtonId: String,
+ className: String,
+ customStyle: String,
+ asyncClose: Boolean,
+ messageAlign: String,
+ beforeClose: null,
+ overlayStyle: String,
+ useSlot: Boolean,
+ useTitleSlot: Boolean,
+ useConfirmButtonSlot: Boolean,
+ useCancelButtonSlot: Boolean,
+ showCancelButton: Boolean,
+ closeOnClickOverlay: Boolean,
+ confirmButtonOpenType: String,
+ width: null,
+ zIndex: {
+ type: Number,
+ value: 2000,
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonColor: {
+ type: String,
+ value: color_1.RED,
+ },
+ cancelButtonColor: {
+ type: String,
+ value: color_1.GRAY,
+ },
+ showConfirmButton: {
+ type: Boolean,
+ value: true,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ transition: {
+ type: String,
+ value: 'scale',
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ callback: (function () { }),
+ },
+ methods: {
+ onConfirm: function () {
+ this.handleAction('confirm');
+ },
+ onCancel: function () {
+ this.handleAction('cancel');
+ },
+ onClickOverlay: function () {
+ this.close('overlay');
+ },
+ close: function (action) {
+ var _this = this;
+ this.setData({ show: false });
+ wx.nextTick(function () {
+ _this.$emit('close', action);
+ var callback = _this.data.callback;
+ if (callback) {
+ callback(action, _this);
+ }
+ });
+ },
+ stopLoading: function () {
+ this.setData({
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ });
+ },
+ handleAction: function (action) {
+ var _a;
+ var _this = this;
+ this.$emit(action, { dialog: this });
+ var _b = this.data, asyncClose = _b.asyncClose, beforeClose = _b.beforeClose;
+ if (!asyncClose && !beforeClose) {
+ this.close(action);
+ return;
+ }
+ this.setData((_a = {},
+ _a["loading.".concat(action)] = true,
+ _a));
+ if (beforeClose) {
+ (0, utils_1.toPromise)(beforeClose(action)).then(function (value) {
+ if (value) {
+ _this.close(action);
+ }
+ else {
+ _this.stopLoading();
+ }
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.json
new file mode 100644
index 0000000..43417fc
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-button": "../button/index",
+ "van-goods-action": "../goods-action/index",
+ "van-goods-action-button": "../goods-action-button/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxml
new file mode 100644
index 0000000..a1d8e3c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxml
@@ -0,0 +1,125 @@
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+ {{ cancelButtonText }}
+
+
+ {{ confirmButtonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxss
new file mode 100644
index 0000000..507a789
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dialog/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dialog{background-color:var(--dialog-background-color,#fff);border-radius:var(--dialog-border-radius,16px);font-size:var(--dialog-font-size,16px);overflow:hidden;top:45%!important;width:var(--dialog-width,320px)}@media (max-width:321px){.van-dialog{width:var(--dialog-small-screen-width,90%)}}.van-dialog__header{font-weight:var(--dialog-header-font-weight,500);line-height:var(--dialog-header-line-height,24px);padding-top:var(--dialog-header-padding-top,24px);text-align:center}.van-dialog__header--isolated{padding:var(--dialog-header-isolated-padding,24px 0)}.van-dialog__message{-webkit-overflow-scrolling:touch;font-size:var(--dialog-message-font-size,14px);line-height:var(--dialog-message-line-height,20px);max-height:var(--dialog-message-max-height,60vh);overflow-y:auto;padding:var(--dialog-message-padding,24px);text-align:center}.van-dialog__message-text{word-wrap:break-word}.van-dialog__message--hasTitle{color:var(--dialog-has-title-message-text-color,#646566);padding-top:var(--dialog-has-title-message-padding-top,8px)}.van-dialog__message--round-button{color:#323233;padding-bottom:16px}.van-dialog__message--left{text-align:left}.van-dialog__message--right{text-align:right}.van-dialog__message--justify{text-align:justify}.van-dialog__footer{display:flex}.van-dialog__footer--round-button{padding:8px 24px 16px!important;position:relative!important}.van-dialog__button{flex:1}.van-dialog__cancel,.van-dialog__confirm{border:0!important}.van-dialog-bounce-enter{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-dialog-bounce-leave-active{opacity:0;transform:translate3d(-50%,-50%,0) scale(.9)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.js
new file mode 100644
index 0000000..5c63844
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.js
@@ -0,0 +1,14 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ dashed: Boolean,
+ hairline: Boolean,
+ contentPosition: String,
+ fontSize: String,
+ borderColor: String,
+ textColor: String,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxml
new file mode 100644
index 0000000..f6a5a45
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxs
new file mode 100644
index 0000000..215b14f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ 'border-color': data.borderColor,
+ color: data.textColor,
+ 'font-size': addUnit(data.fontSize),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxss
new file mode 100644
index 0000000..e91dc44
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/divider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-divider{align-items:center;border:0 solid var(--divider-border-color,#ebedf0);color:var(--divider-text-color,#969799);display:flex;font-size:var(--divider-font-size,14px);line-height:var(--divider-line-height,24px);margin:var(--divider-margin,16px 0)}.van-divider:after,.van-divider:before{border-color:inherit;border-style:inherit;border-width:1px 0 0;box-sizing:border-box;display:block;flex:1;height:1px}.van-divider:before{content:""}.van-divider--hairline:after,.van-divider--hairline:before{transform:scaleY(.5)}.van-divider--dashed{border-style:dashed}.van-divider--center:before,.van-divider--left:before,.van-divider--right:before{margin-right:var(--divider-content-padding,16px)}.van-divider--center:after,.van-divider--left:after,.van-divider--right:after{content:"";margin-left:var(--divider-content-padding,16px)}.van-divider--left:before{max-width:var(--divider-content-left-width,10%)}.van-divider--right:after{max-width:var(--divider-content-right-width,10%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.js
new file mode 100644
index 0000000..826c26a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.js
@@ -0,0 +1,136 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['item-title-class'],
+ field: true,
+ relation: (0, relation_1.useParent)('dropdown-menu', function () {
+ this.updateDataFromParent();
+ }),
+ props: {
+ value: {
+ type: null,
+ observer: 'rerender',
+ },
+ title: {
+ type: String,
+ observer: 'rerender',
+ },
+ disabled: Boolean,
+ titleClass: {
+ type: String,
+ observer: 'rerender',
+ },
+ options: {
+ type: Array,
+ value: [],
+ observer: 'rerender',
+ },
+ popupStyle: String,
+ useBeforeToggle: {
+ type: Boolean,
+ value: false,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ transition: true,
+ showPopup: false,
+ showWrapper: false,
+ displayTitle: '',
+ safeAreaTabBar: false,
+ },
+ methods: {
+ rerender: function () {
+ var _this = this;
+ wx.nextTick(function () {
+ var _a;
+ (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.updateItemListData();
+ });
+ },
+ updateDataFromParent: function () {
+ if (this.parent) {
+ var _a = this.parent.data, overlay = _a.overlay, duration = _a.duration, activeColor = _a.activeColor, closeOnClickOverlay = _a.closeOnClickOverlay, direction = _a.direction, safeAreaTabBar = _a.safeAreaTabBar;
+ this.setData({
+ overlay: overlay,
+ duration: duration,
+ activeColor: activeColor,
+ closeOnClickOverlay: closeOnClickOverlay,
+ direction: direction,
+ safeAreaTabBar: safeAreaTabBar,
+ });
+ }
+ },
+ onOpen: function () {
+ this.$emit('open');
+ },
+ onOpened: function () {
+ this.$emit('opened');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClosed: function () {
+ this.$emit('closed');
+ this.setData({ showWrapper: false });
+ },
+ onOptionTap: function (event) {
+ var option = event.currentTarget.dataset.option;
+ var value = option.value;
+ var shouldEmitChange = this.data.value !== value;
+ this.setData({ showPopup: false, value: value });
+ this.$emit('close');
+ this.rerender();
+ if (shouldEmitChange) {
+ this.$emit('change', value);
+ }
+ },
+ toggle: function (show, options) {
+ var _this = this;
+ if (options === void 0) { options = {}; }
+ var showPopup = this.data.showPopup;
+ if (typeof show !== 'boolean') {
+ show = !showPopup;
+ }
+ if (show === showPopup) {
+ return;
+ }
+ this.onBeforeToggle(show).then(function (status) {
+ var _a;
+ if (!status) {
+ return;
+ }
+ _this.setData({
+ transition: !options.immediate,
+ showPopup: show,
+ });
+ if (show) {
+ (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.getChildWrapperStyle().then(function (wrapperStyle) {
+ _this.setData({ wrapperStyle: wrapperStyle, showWrapper: true });
+ _this.rerender();
+ });
+ }
+ else {
+ _this.rerender();
+ }
+ });
+ },
+ onBeforeToggle: function (status) {
+ var _this = this;
+ var useBeforeToggle = this.data.useBeforeToggle;
+ if (!useBeforeToggle) {
+ return Promise.resolve(true);
+ }
+ return new Promise(function (resolve) {
+ _this.$emit('before-toggle', {
+ status: status,
+ callback: function (value) { return resolve(value); },
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.json
new file mode 100644
index 0000000..88d5409
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxml
new file mode 100644
index 0000000..63904f4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxml
@@ -0,0 +1,50 @@
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxss
new file mode 100644
index 0000000..80505e9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-item{left:0;overflow:hidden;position:fixed;right:0}.van-dropdown-item__option{text-align:left}.van-dropdown-item__option--active .van-dropdown-item__icon,.van-dropdown-item__option--active .van-dropdown-item__title{color:var(--dropdown-menu-option-active-color,#ee0a24)}.van-dropdown-item--up{top:0}.van-dropdown-item--down{bottom:0}.van-dropdown-item__icon{display:block;line-height:inherit}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.d.ts
new file mode 100644
index 0000000..774eb4c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.d.ts
@@ -0,0 +1,5 @@
+export interface Option {
+ text: string;
+ value: string | number;
+ icon: string;
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-item/shared.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.js
new file mode 100644
index 0000000..aed2921
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.js
@@ -0,0 +1,122 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+var ARRAY = [];
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['title-class'],
+ relation: (0, relation_1.useChildren)('dropdown-item', function () {
+ this.updateItemListData();
+ }),
+ props: {
+ activeColor: {
+ type: String,
+ observer: 'updateChildrenData',
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ zIndex: {
+ type: Number,
+ value: 10,
+ },
+ duration: {
+ type: Number,
+ value: 200,
+ observer: 'updateChildrenData',
+ },
+ direction: {
+ type: String,
+ value: 'down',
+ observer: 'updateChildrenData',
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ closeOnClickOutside: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ itemListData: [],
+ },
+ beforeCreate: function () {
+ var windowHeight = (0, utils_1.getSystemInfoSync)().windowHeight;
+ this.windowHeight = windowHeight;
+ ARRAY.push(this);
+ },
+ destroyed: function () {
+ var _this = this;
+ ARRAY = ARRAY.filter(function (item) { return item !== _this; });
+ },
+ methods: {
+ updateItemListData: function () {
+ this.setData({
+ itemListData: this.children.map(function (child) { return child.data; }),
+ });
+ },
+ updateChildrenData: function () {
+ this.children.forEach(function (child) {
+ child.updateDataFromParent();
+ });
+ },
+ toggleItem: function (active) {
+ this.children.forEach(function (item, index) {
+ var showPopup = item.data.showPopup;
+ if (index === active) {
+ item.toggle();
+ }
+ else if (showPopup) {
+ item.toggle(false, { immediate: true });
+ }
+ });
+ },
+ close: function () {
+ this.children.forEach(function (child) {
+ child.toggle(false, { immediate: true });
+ });
+ },
+ getChildWrapperStyle: function () {
+ var _this = this;
+ var _a = this.data, zIndex = _a.zIndex, direction = _a.direction;
+ return (0, utils_1.getRect)(this, '.van-dropdown-menu').then(function (rect) {
+ var _a = rect.top, top = _a === void 0 ? 0 : _a, _b = rect.bottom, bottom = _b === void 0 ? 0 : _b;
+ var offset = direction === 'down' ? bottom : _this.windowHeight - top;
+ var wrapperStyle = "z-index: ".concat(zIndex, ";");
+ if (direction === 'down') {
+ wrapperStyle += "top: ".concat((0, utils_1.addUnit)(offset), ";");
+ }
+ else {
+ wrapperStyle += "bottom: ".concat((0, utils_1.addUnit)(offset), ";");
+ }
+ return wrapperStyle;
+ });
+ },
+ onTitleTap: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var child = this.children[index];
+ if (!child.data.disabled) {
+ ARRAY.forEach(function (menuItem) {
+ if (menuItem &&
+ menuItem.data.closeOnClickOutside &&
+ menuItem !== _this) {
+ menuItem.close();
+ }
+ });
+ this.toggleItem(index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxml
new file mode 100644
index 0000000..ec165a9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxs
new file mode 100644
index 0000000..6538854
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxs
@@ -0,0 +1,16 @@
+/* eslint-disable */
+function displayTitle(item) {
+ if (item.title) {
+ return item.title;
+ }
+
+ var match = item.options.filter(function(option) {
+ return option.value === item.value;
+ });
+ var displayTitle = match.length ? match[0].text : '';
+ return displayTitle;
+}
+
+module.exports = {
+ displayTitle: displayTitle
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxss
new file mode 100644
index 0000000..dba000e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/dropdown-menu/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-menu{background-color:var(--dropdown-menu-background-color,#fff);box-shadow:var(--dropdown-menu-box-shadow,0 2px 12px hsla(210,1%,40%,.12));display:flex;height:var(--dropdown-menu-height,50px);-webkit-user-select:none;user-select:none}.van-dropdown-menu__item{align-items:center;display:flex;flex:1;justify-content:center;min-width:0}.van-dropdown-menu__item:active{opacity:.7}.van-dropdown-menu__item--disabled:active{opacity:1}.van-dropdown-menu__item--disabled .van-dropdown-menu__title{color:var(--dropdown-menu-title-disabled-text-color,#969799)}.van-dropdown-menu__title{box-sizing:border-box;color:var(--dropdown-menu-title-text-color,#323233);font-size:var(--dropdown-menu-title-font-size,15px);line-height:var(--dropdown-menu-title-line-height,18px);max-width:100%;padding:var(--dropdown-menu-title-padding,0 24px 0 8px);position:relative}.van-dropdown-menu__title:after{border-color:transparent transparent currentcolor currentcolor;border-style:solid;border-width:3px;content:"";margin-top:-5px;opacity:.8;position:absolute;right:11px;top:50%;transform:rotate(-45deg)}.van-dropdown-menu__title--active{color:var(--dropdown-menu-title-active-text-color,#ee0a24)}.van-dropdown-menu__title--down:after{margin-top:-1px;transform:rotate(135deg)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.js
new file mode 100644
index 0000000..755e638
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.js
@@ -0,0 +1,12 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ description: String,
+ image: {
+ type: String,
+ value: 'default',
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxml
new file mode 100644
index 0000000..9c7b719
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxs
new file mode 100644
index 0000000..cf92ece
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var PRESETS = ['error', 'search', 'default', 'network'];
+
+function imageUrl(image) {
+ if (PRESETS.indexOf(image) !== -1) {
+ return 'https://img.yzcdn.cn/vant/empty-image-' + image + '.png';
+ }
+
+ return image;
+}
+
+module.exports = {
+ imageUrl: imageUrl,
+};
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxss
new file mode 100644
index 0000000..0fb74fe
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/empty/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-empty{align-items:center;box-sizing:border-box;display:flex;flex-direction:column;justify-content:center;padding:32px 0}.van-empty__image{height:160px;width:160px}.van-empty__image:empty{display:none}.van-empty__image__img{height:100%;width:100%}.van-empty__image:not(:empty)+.van-empty__image{display:none}.van-empty__description{color:#969799;font-size:14px;line-height:20px;margin-top:16px;padding:0 60px}.van-empty__description:empty,.van-empty__description:not(:empty)+.van-empty__description{display:none}.van-empty__bottom{margin-top:24px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.js
new file mode 100644
index 0000000..c20d266
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.js
@@ -0,0 +1,137 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var props_1 = require("./props");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['input-class', 'right-icon-class', 'label-class'],
+ props: __assign(__assign(__assign(__assign({}, props_1.commonProps), props_1.inputProps), props_1.textareaProps), { size: String, icon: String, label: String, error: Boolean, center: Boolean, isLink: Boolean, leftIcon: String, rightIcon: String, autosize: null, required: Boolean, iconClass: String, clickable: Boolean, inputAlign: String, customStyle: String, errorMessage: String, arrowDirection: String, showWordLimit: Boolean, errorMessageAlign: String, readonly: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearable: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearTrigger: {
+ type: String,
+ value: 'focus',
+ }, border: {
+ type: Boolean,
+ value: true,
+ }, titleWidth: {
+ type: String,
+ value: '6.2em',
+ }, clearIcon: {
+ type: String,
+ value: 'clear',
+ }, extraEventParams: {
+ type: Boolean,
+ value: false,
+ } }),
+ data: {
+ focused: false,
+ innerValue: '',
+ showClear: false,
+ },
+ created: function () {
+ this.value = this.data.value;
+ this.setData({ innerValue: this.value });
+ },
+ methods: {
+ formatValue: function (value) {
+ var maxlength = this.data.maxlength;
+ if (maxlength !== -1 && value.length > maxlength) {
+ return value.slice(0, maxlength);
+ }
+ return value;
+ },
+ onInput: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ var formatValue = this.formatValue(value);
+ this.value = formatValue;
+ this.setShowClear();
+ return this.emitChange(__assign(__assign({}, event.detail), { value: formatValue }));
+ },
+ onFocus: function (event) {
+ this.focused = true;
+ this.setShowClear();
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ this.focused = false;
+ this.setShowClear();
+ this.$emit('blur', event.detail);
+ },
+ onClickIcon: function () {
+ this.$emit('click-icon');
+ },
+ onClickInput: function (event) {
+ this.$emit('click-input', event.detail);
+ },
+ onClear: function () {
+ var _this = this;
+ this.setData({ innerValue: '' });
+ this.value = '';
+ this.setShowClear();
+ (0, utils_1.nextTick)(function () {
+ _this.emitChange({ value: '' });
+ _this.$emit('clear', '');
+ });
+ },
+ onConfirm: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ this.value = value;
+ this.setShowClear();
+ this.$emit('confirm', value);
+ },
+ setValue: function (value) {
+ this.value = value;
+ this.setShowClear();
+ if (value === '') {
+ this.setData({ innerValue: '' });
+ }
+ this.emitChange({ value: value });
+ },
+ onLineChange: function (event) {
+ this.$emit('linechange', event.detail);
+ },
+ onKeyboardHeightChange: function (event) {
+ this.$emit('keyboardheightchange', event.detail);
+ },
+ emitChange: function (detail) {
+ var extraEventParams = this.data.extraEventParams;
+ this.setData({ value: detail.value });
+ var result;
+ var data = extraEventParams
+ ? __assign(__assign({}, detail), { callback: function (data) {
+ result = data;
+ } }) : detail.value;
+ this.$emit('input', data);
+ this.$emit('change', data);
+ return result;
+ },
+ setShowClear: function () {
+ var _a = this.data, clearable = _a.clearable, readonly = _a.readonly, clearTrigger = _a.clearTrigger;
+ var _b = this, focused = _b.focused, value = _b.value;
+ var showClear = false;
+ if (clearable && !readonly) {
+ var hasValue = !!value;
+ var trigger = clearTrigger === 'always' || (clearTrigger === 'focus' && focused);
+ showClear = hasValue && trigger;
+ }
+ this.setData({ showClear: showClear });
+ },
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.json
new file mode 100644
index 0000000..5906c50
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxml
new file mode 100644
index 0000000..6018993
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxs
new file mode 100644
index 0000000..78575b9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function inputStyle(autosize) {
+ if (autosize && autosize.constructor === 'Object') {
+ return style({
+ 'min-height': addUnit(autosize.minHeight),
+ 'max-height': addUnit(autosize.maxHeight),
+ });
+ }
+
+ return '';
+}
+
+module.exports = {
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxss
new file mode 100644
index 0000000..5f7d306
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-field{--cell-icon-size:var(--field-icon-size,16px)}.van-field__label{color:var(--field-label-color,#646566)}.van-field__label--disabled{color:var(--field-disabled-text-color,#c8c9cc)}.van-field__body{align-items:center;display:flex}.van-field__body--textarea{box-sizing:border-box;line-height:1.2em;min-height:var(--cell-line-height,24px);padding:3.6px 0}.van-field__control:empty+.van-field__control{display:block}.van-field__control{background-color:initial;border:0;box-sizing:border-box;color:var(--field-input-text-color,#323233);display:none;height:var(--cell-line-height,24px);line-height:inherit;margin:0;min-height:var(--cell-line-height,24px);padding:0;position:relative;resize:none;text-align:left;width:100%}.van-field__control:empty{display:none}.van-field__control--textarea{height:var(--field-text-area-min-height,18px);min-height:var(--field-text-area-min-height,18px)}.van-field__control--error{color:var(--field-input-error-text-color,#ee0a24)}.van-field__control--disabled{background-color:initial;color:var(--field-input-disabled-text-color,#c8c9cc);opacity:1}.van-field__control--center{text-align:center}.van-field__control--right{text-align:right}.van-field__control--custom{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__placeholder{color:var(--field-placeholder-text-color,#c8c9cc);left:0;pointer-events:none;position:absolute;right:0;top:0}.van-field__placeholder--error{color:var(--field-error-message-color,#ee0a24)}.van-field__icon-root{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__clear-root,.van-field__icon-container{line-height:inherit;margin-right:calc(var(--padding-xs, 8px)*-1);padding:0 var(--padding-xs,8px);vertical-align:middle}.van-field__button,.van-field__clear-root,.van-field__icon-container{flex-shrink:0}.van-field__clear-root{color:var(--field-clear-icon-color,#c8c9cc);font-size:var(--field-clear-icon-size,16px)}.van-field__icon-container{color:var(--field-icon-container-color,#969799);font-size:var(--field-icon-size,16px)}.van-field__icon-container:empty{display:none}.van-field__button{padding-left:var(--padding-xs,8px)}.van-field__button:empty{display:none}.van-field__error-message{color:var(--field-error-message-color,#ee0a24);display:block;font-size:var(--field-error-message-text-font-size,12px);text-align:left}.van-field__error-message--center{text-align:center}.van-field__error-message--right{text-align:right}.van-field__word-limit{color:var(--field-word-limit-color,#646566);font-size:var(--field-word-limit-font-size,12px);line-height:var(--field-word-limit-line-height,16px);margin-top:var(--padding-base,4px);text-align:right}.van-field__word-num{display:inline}.van-field__word-num--full{color:var(--field-word-num-full-color,#ee0a24)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/input.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/input.wxml
new file mode 100644
index 0000000..c10f4c4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/input.wxml
@@ -0,0 +1,30 @@
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.d.ts
new file mode 100644
index 0000000..5cd130a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.d.ts
@@ -0,0 +1,4 @@
+///
+export declare const commonProps: WechatMiniprogram.Component.PropertyOption;
+export declare const inputProps: WechatMiniprogram.Component.PropertyOption;
+export declare const textareaProps: WechatMiniprogram.Component.PropertyOption;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.js
new file mode 100644
index 0000000..3cb8dca
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/props.js
@@ -0,0 +1,67 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.textareaProps = exports.inputProps = exports.commonProps = void 0;
+exports.commonProps = {
+ value: {
+ type: String,
+ observer: function (value) {
+ if (value !== this.value) {
+ this.setData({ innerValue: value });
+ this.value = value;
+ }
+ },
+ },
+ placeholder: String,
+ placeholderStyle: String,
+ placeholderClass: String,
+ disabled: Boolean,
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 50,
+ },
+ autoFocus: Boolean,
+ focus: Boolean,
+ cursor: {
+ type: Number,
+ value: -1,
+ },
+ selectionStart: {
+ type: Number,
+ value: -1,
+ },
+ selectionEnd: {
+ type: Number,
+ value: -1,
+ },
+ adjustPosition: {
+ type: Boolean,
+ value: true,
+ },
+ holdKeyboard: Boolean,
+};
+exports.inputProps = {
+ type: {
+ type: String,
+ value: 'text',
+ },
+ password: Boolean,
+ confirmType: String,
+ confirmHold: Boolean,
+ alwaysEmbed: Boolean,
+};
+exports.textareaProps = {
+ autoHeight: Boolean,
+ fixed: Boolean,
+ showConfirmBar: {
+ type: Boolean,
+ value: true,
+ },
+ disableDefaultPadding: {
+ type: Boolean,
+ value: true,
+ },
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/textarea.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/textarea.wxml
new file mode 100644
index 0000000..945d03e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/textarea.wxml
@@ -0,0 +1,32 @@
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.d.ts
new file mode 100644
index 0000000..357ccbe
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.d.ts
@@ -0,0 +1,8 @@
+export interface InputDetails {
+ /** 输入框内容 */
+ value: string;
+ /** 光标位置 */
+ cursor?: number;
+ /** keyCode 为键值 (目前工具还不支持返回keyCode参数) `2.1.0` 起支持 */
+ keyCode?: number;
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/field/types.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.js
new file mode 100644
index 0000000..8179e89
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.js
@@ -0,0 +1,46 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var button_1 = require("../mixins/button");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ mixins: [link_1.link, button_1.button],
+ relation: (0, relation_1.useParent)('goods-action'),
+ props: {
+ text: String,
+ color: String,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loading: Boolean,
+ disabled: Boolean,
+ plain: Boolean,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ customStyle: {
+ type: String,
+ value: '',
+ },
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ updateStyle: function () {
+ if (this.parent == null) {
+ return;
+ }
+ var index = this.index;
+ var _a = this.parent.children, children = _a === void 0 ? [] : _a;
+ this.setData({
+ isFirst: index === 0,
+ isLast: index === children.length - 1,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.json
new file mode 100644
index 0000000..b567686
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxml
new file mode 100644
index 0000000..4530ab8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxml
@@ -0,0 +1,35 @@
+
+
+ {{ text }}
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxss
new file mode 100644
index 0000000..759a1d9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-goods-action-button{--button-warning-background-color:var(--goods-action-button-warning-color,linear-gradient(to right,#ffd01e,#ff8917));--button-danger-background-color:var(--goods-action-button-danger-color,linear-gradient(to right,#ff6034,#ee0a24));--button-default-height:var(--goods-action-button-height,40px);--button-line-height:var(--goods-action-button-line-height,20px);--button-plain-background-color:var(--goods-action-button-plain-color,#fff);--button-border-width:0;display:block}.van-goods-action-button--first{--button-border-radius:999px 0 0 var(--goods-action-button-border-radius,999px);margin-left:5px}.van-goods-action-button--last{--button-border-radius:0 999px var(--goods-action-button-border-radius,999px) 0;margin-right:5px}.van-goods-action-button--first.van-goods-action-button--last{--button-border-radius:var(--goods-action-button-border-radius,999px)}.van-goods-action-button--plain{--button-border-width:1px}.van-goods-action-button__inner{font-weight:var(--font-weight-bold,500)!important;width:100%}@media (max-width:321px){.van-goods-action-button{font-size:13px}}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.js
new file mode 100644
index 0000000..828e1f5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.js
@@ -0,0 +1,29 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ classes: ['icon-class', 'text-class', 'info-class'],
+ mixins: [link_1.link, button_1.button],
+ props: {
+ text: String,
+ dot: Boolean,
+ info: String,
+ icon: String,
+ size: String,
+ color: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ disabled: Boolean,
+ loading: Boolean,
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.json
new file mode 100644
index 0000000..93bfe8a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxml
new file mode 100644
index 0000000..30c1a8c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxss
new file mode 100644
index 0000000..6e4758d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action-icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action-icon{border:none!important;color:var(--goods-action-icon-text-color,#646566)!important;display:flex!important;flex-direction:column;font-size:var(--goods-action-icon-font-size,10px)!important;height:var(--goods-action-icon-height,50px)!important;justify-content:center!important;line-height:1!important;min-width:var(--goods-action-icon-width,48px)}.van-goods-action-icon__icon{color:var(--goods-action-icon-color,#323233);display:flex;font-size:var(--goods-action-icon-size,18px);margin:0 auto 5px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.js
new file mode 100644
index 0000000..e49bcbc
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.js
@@ -0,0 +1,17 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('goods-action-button', function () {
+ this.children.forEach(function (item) {
+ item.updateStyle();
+ });
+ }),
+ props: {
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxml
new file mode 100644
index 0000000..569450c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxss
new file mode 100644
index 0000000..7793e77
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/goods-action/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action{align-items:center;background-color:var(--goods-action-background-color,#fff);bottom:0;box-sizing:initial;display:flex;height:var(--goods-action-height,50px);left:0;position:fixed;right:0}.van-goods-action--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.js
new file mode 100644
index 0000000..a7d47a2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.js
@@ -0,0 +1,54 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('grid'),
+ classes: ['content-class', 'icon-class', 'text-class'],
+ mixins: [link_1.link],
+ props: {
+ icon: String,
+ iconColor: String,
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ dot: Boolean,
+ info: null,
+ badge: null,
+ text: String,
+ useSlot: Boolean,
+ },
+ data: {
+ viewStyle: '',
+ },
+ mounted: function () {
+ this.updateStyle();
+ },
+ methods: {
+ updateStyle: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent, data = _a.data, children = _a.children;
+ var columnNum = data.columnNum, border = data.border, square = data.square, gutter = data.gutter, clickable = data.clickable, center = data.center, direction = data.direction, reverse = data.reverse, iconSize = data.iconSize;
+ this.setData({
+ center: center,
+ border: border,
+ square: square,
+ gutter: gutter,
+ clickable: clickable,
+ direction: direction,
+ reverse: reverse,
+ iconSize: iconSize,
+ index: children.indexOf(this),
+ columnNum: columnNum,
+ });
+ },
+ onClick: function () {
+ this.$emit('click');
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxml
new file mode 100644
index 0000000..e95087d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxs
new file mode 100644
index 0000000..2cfe37d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapperStyle(data) {
+ var width = 100 / data.columnNum + '%';
+
+ return style({
+ width: width,
+ 'padding-top': data.square ? width : null,
+ 'padding-right': addUnit(data.gutter),
+ 'margin-top':
+ data.index >= data.columnNum && !data.square
+ ? addUnit(data.gutter)
+ : null,
+ });
+}
+
+function contentStyle(data) {
+ return data.square
+ ? style({
+ right: addUnit(data.gutter),
+ bottom: addUnit(data.gutter),
+ height: 'auto',
+ })
+ : '';
+}
+
+module.exports = {
+ wrapperStyle: wrapperStyle,
+ contentStyle: contentStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxss
new file mode 100644
index 0000000..acaea84
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid-item{box-sizing:border-box;float:left;position:relative}.van-grid-item--square{height:0}.van-grid-item__content{background-color:var(--grid-item-content-background-color,#fff);box-sizing:border-box;display:flex;flex-direction:column;height:100%;padding:var(--grid-item-content-padding,16px 8px)}.van-grid-item__content:after{border-width:0 1px 1px 0;z-index:1}.van-grid-item__content--surround:after{border-width:1px}.van-grid-item__content--center{align-items:center;justify-content:center}.van-grid-item__content--square{left:0;position:absolute;right:0;top:0}.van-grid-item__content--horizontal{flex-direction:row}.van-grid-item__content--horizontal .van-grid-item__text{margin:0 0 0 8px}.van-grid-item__content--reverse{flex-direction:column-reverse}.van-grid-item__content--reverse .van-grid-item__text{margin:0 0 8px}.van-grid-item__content--horizontal.van-grid-item__content--reverse{flex-direction:row-reverse}.van-grid-item__content--horizontal.van-grid-item__content--reverse .van-grid-item__text{margin:0 8px 0 0}.van-grid-item__content--clickable:active{background-color:var(--grid-item-content-active-color,#f2f3f5)}.van-grid-item__icon{align-items:center;display:flex;font-size:var(--grid-item-icon-size,26px);height:var(--grid-item-icon-size,26px)}.van-grid-item__text{word-wrap:break-word;color:var(--grid-item-text-color,#646566);font-size:var(--grid-item-text-font-size,12px)}.van-grid-item__icon+.van-grid-item__text{margin-top:8px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.js
new file mode 100644
index 0000000..28d14f4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.js
@@ -0,0 +1,57 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('grid-item'),
+ props: {
+ square: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ gutter: {
+ type: null,
+ value: 0,
+ observer: 'updateChildren',
+ },
+ clickable: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ columnNum: {
+ type: Number,
+ value: 4,
+ observer: 'updateChildren',
+ },
+ center: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ iconSize: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ reverse: {
+ type: Boolean,
+ value: false,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ this.children.forEach(function (child) {
+ child.updateStyle();
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxml
new file mode 100644
index 0000000..2e4118f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxs
new file mode 100644
index 0000000..cd3b1bd
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'padding-left': addUnit(data.gutter),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxss
new file mode 100644
index 0000000..e347440
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/grid/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid{box-sizing:border-box;overflow:hidden;position:relative}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.js
new file mode 100644
index 0000000..6758092
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.js
@@ -0,0 +1,23 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['info-class'],
+ props: {
+ dot: Boolean,
+ info: null,
+ size: null,
+ color: String,
+ customStyle: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ name: String,
+ },
+ methods: {
+ onClick: function () {
+ this.$emit('click');
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxml
new file mode 100644
index 0000000..91b47f9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxs
new file mode 100644
index 0000000..a906f76
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxs
@@ -0,0 +1,43 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isImage(name) {
+ return name.indexOf('/') !== -1;
+}
+
+function rootClass(data) {
+ var classes = ['custom-class'];
+
+ if (data.classPrefix !== 'van-icon') {
+ classes.push('van-icon--custom')
+ }
+
+ if (data.classPrefix != null) {
+ classes.push(data.classPrefix);
+ }
+
+ if (isImage(data.name)) {
+ classes.push('van-icon--image');
+ } else if (data.classPrefix != null) {
+ classes.push(data.classPrefix + '-' + data.name);
+ }
+
+ return classes.join(' ');
+}
+
+function rootStyle(data) {
+ return style([
+ {
+ color: data.color,
+ 'font-size': addUnit(data.size),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ isImage: isImage,
+ rootClass: rootClass,
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxss
new file mode 100644
index 0000000..feb3d7e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-icon{text-rendering:auto;-webkit-font-smoothing:antialiased;font:normal normal normal 14px/1 vant-icon;font:normal normal normal 14px/1 var(--van-icon-font-family,"vant-icon");font-size:inherit;position:relative}.van-icon,.van-icon:before{display:inline-block}.van-icon-contact:before{content:"\e753"}.van-icon-notes:before{content:"\e63c"}.van-icon-records:before{content:"\e63d"}.van-icon-cash-back-record:before{content:"\e63e"}.van-icon-newspaper:before{content:"\e63f"}.van-icon-discount:before{content:"\e640"}.van-icon-completed:before{content:"\e641"}.van-icon-user:before{content:"\e642"}.van-icon-description:before{content:"\e643"}.van-icon-list-switch:before{content:"\e6ad"}.van-icon-list-switching:before{content:"\e65a"}.van-icon-link-o:before{content:"\e751"}.van-icon-miniprogram-o:before{content:"\e752"}.van-icon-qq:before{content:"\e74e"}.van-icon-wechat-moments:before{content:"\e74f"}.van-icon-weibo:before{content:"\e750"}.van-icon-cash-o:before{content:"\e74d"}.van-icon-guide-o:before{content:"\e74c"}.van-icon-invitation:before{content:"\e6d6"}.van-icon-shield-o:before{content:"\e74b"}.van-icon-exchange:before{content:"\e6af"}.van-icon-eye:before{content:"\e6b0"}.van-icon-enlarge:before{content:"\e6b1"}.van-icon-expand-o:before{content:"\e6b2"}.van-icon-eye-o:before{content:"\e6b3"}.van-icon-expand:before{content:"\e6b4"}.van-icon-filter-o:before{content:"\e6b5"}.van-icon-fire:before{content:"\e6b6"}.van-icon-fail:before{content:"\e6b7"}.van-icon-failure:before{content:"\e6b8"}.van-icon-fire-o:before{content:"\e6b9"}.van-icon-flag-o:before{content:"\e6ba"}.van-icon-font:before{content:"\e6bb"}.van-icon-font-o:before{content:"\e6bc"}.van-icon-gem-o:before{content:"\e6bd"}.van-icon-flower-o:before{content:"\e6be"}.van-icon-gem:before{content:"\e6bf"}.van-icon-gift-card:before{content:"\e6c0"}.van-icon-friends:before{content:"\e6c1"}.van-icon-friends-o:before{content:"\e6c2"}.van-icon-gold-coin:before{content:"\e6c3"}.van-icon-gold-coin-o:before{content:"\e6c4"}.van-icon-good-job-o:before{content:"\e6c5"}.van-icon-gift:before{content:"\e6c6"}.van-icon-gift-o:before{content:"\e6c7"}.van-icon-gift-card-o:before{content:"\e6c8"}.van-icon-good-job:before{content:"\e6c9"}.van-icon-home-o:before{content:"\e6ca"}.van-icon-goods-collect:before{content:"\e6cb"}.van-icon-graphic:before{content:"\e6cc"}.van-icon-goods-collect-o:before{content:"\e6cd"}.van-icon-hot-o:before{content:"\e6ce"}.van-icon-info:before{content:"\e6cf"}.van-icon-hotel-o:before{content:"\e6d0"}.van-icon-info-o:before{content:"\e6d1"}.van-icon-hot-sale-o:before{content:"\e6d2"}.van-icon-hot:before{content:"\e6d3"}.van-icon-like:before{content:"\e6d4"}.van-icon-idcard:before{content:"\e6d5"}.van-icon-like-o:before{content:"\e6d7"}.van-icon-hot-sale:before{content:"\e6d8"}.van-icon-location-o:before{content:"\e6d9"}.van-icon-location:before{content:"\e6da"}.van-icon-label:before{content:"\e6db"}.van-icon-lock:before{content:"\e6dc"}.van-icon-label-o:before{content:"\e6dd"}.van-icon-map-marked:before{content:"\e6de"}.van-icon-logistics:before{content:"\e6df"}.van-icon-manager:before{content:"\e6e0"}.van-icon-more:before{content:"\e6e1"}.van-icon-live:before{content:"\e6e2"}.van-icon-manager-o:before{content:"\e6e3"}.van-icon-medal:before{content:"\e6e4"}.van-icon-more-o:before{content:"\e6e5"}.van-icon-music-o:before{content:"\e6e6"}.van-icon-music:before{content:"\e6e7"}.van-icon-new-arrival-o:before{content:"\e6e8"}.van-icon-medal-o:before{content:"\e6e9"}.van-icon-new-o:before{content:"\e6ea"}.van-icon-free-postage:before{content:"\e6eb"}.van-icon-newspaper-o:before{content:"\e6ec"}.van-icon-new-arrival:before{content:"\e6ed"}.van-icon-minus:before{content:"\e6ee"}.van-icon-orders-o:before{content:"\e6ef"}.van-icon-new:before{content:"\e6f0"}.van-icon-paid:before{content:"\e6f1"}.van-icon-notes-o:before{content:"\e6f2"}.van-icon-other-pay:before{content:"\e6f3"}.van-icon-pause-circle:before{content:"\e6f4"}.van-icon-pause:before{content:"\e6f5"}.van-icon-pause-circle-o:before{content:"\e6f6"}.van-icon-peer-pay:before{content:"\e6f7"}.van-icon-pending-payment:before{content:"\e6f8"}.van-icon-passed:before{content:"\e6f9"}.van-icon-plus:before{content:"\e6fa"}.van-icon-phone-circle-o:before{content:"\e6fb"}.van-icon-phone-o:before{content:"\e6fc"}.van-icon-printer:before{content:"\e6fd"}.van-icon-photo-fail:before{content:"\e6fe"}.van-icon-phone:before{content:"\e6ff"}.van-icon-photo-o:before{content:"\e700"}.van-icon-play-circle:before{content:"\e701"}.van-icon-play:before{content:"\e702"}.van-icon-phone-circle:before{content:"\e703"}.van-icon-point-gift-o:before{content:"\e704"}.van-icon-point-gift:before{content:"\e705"}.van-icon-play-circle-o:before{content:"\e706"}.van-icon-shrink:before{content:"\e707"}.van-icon-photo:before{content:"\e708"}.van-icon-qr:before{content:"\e709"}.van-icon-qr-invalid:before{content:"\e70a"}.van-icon-question-o:before{content:"\e70b"}.van-icon-revoke:before{content:"\e70c"}.van-icon-replay:before{content:"\e70d"}.van-icon-service:before{content:"\e70e"}.van-icon-question:before{content:"\e70f"}.van-icon-search:before{content:"\e710"}.van-icon-refund-o:before{content:"\e711"}.van-icon-service-o:before{content:"\e712"}.van-icon-scan:before{content:"\e713"}.van-icon-share:before{content:"\e714"}.van-icon-send-gift-o:before{content:"\e715"}.van-icon-share-o:before{content:"\e716"}.van-icon-setting:before{content:"\e717"}.van-icon-points:before{content:"\e718"}.van-icon-photograph:before{content:"\e719"}.van-icon-shop:before{content:"\e71a"}.van-icon-shop-o:before{content:"\e71b"}.van-icon-shop-collect-o:before{content:"\e71c"}.van-icon-shop-collect:before{content:"\e71d"}.van-icon-smile:before{content:"\e71e"}.van-icon-shopping-cart-o:before{content:"\e71f"}.van-icon-sign:before{content:"\e720"}.van-icon-sort:before{content:"\e721"}.van-icon-star-o:before{content:"\e722"}.van-icon-smile-comment-o:before{content:"\e723"}.van-icon-stop:before{content:"\e724"}.van-icon-stop-circle-o:before{content:"\e725"}.van-icon-smile-o:before{content:"\e726"}.van-icon-star:before{content:"\e727"}.van-icon-success:before{content:"\e728"}.van-icon-stop-circle:before{content:"\e729"}.van-icon-records-o:before{content:"\e72a"}.van-icon-shopping-cart:before{content:"\e72b"}.van-icon-tosend:before{content:"\e72c"}.van-icon-todo-list:before{content:"\e72d"}.van-icon-thumb-circle-o:before{content:"\e72e"}.van-icon-thumb-circle:before{content:"\e72f"}.van-icon-umbrella-circle:before{content:"\e730"}.van-icon-underway:before{content:"\e731"}.van-icon-upgrade:before{content:"\e732"}.van-icon-todo-list-o:before{content:"\e733"}.van-icon-tv-o:before{content:"\e734"}.van-icon-underway-o:before{content:"\e735"}.van-icon-user-o:before{content:"\e736"}.van-icon-vip-card-o:before{content:"\e737"}.van-icon-vip-card:before{content:"\e738"}.van-icon-send-gift:before{content:"\e739"}.van-icon-wap-home:before{content:"\e73a"}.van-icon-wap-nav:before{content:"\e73b"}.van-icon-volume-o:before{content:"\e73c"}.van-icon-video:before{content:"\e73d"}.van-icon-wap-home-o:before{content:"\e73e"}.van-icon-volume:before{content:"\e73f"}.van-icon-warning:before{content:"\e740"}.van-icon-weapp-nav:before{content:"\e741"}.van-icon-wechat-pay:before{content:"\e742"}.van-icon-warning-o:before{content:"\e743"}.van-icon-wechat:before{content:"\e744"}.van-icon-setting-o:before{content:"\e745"}.van-icon-youzan-shield:before{content:"\e746"}.van-icon-warn-o:before{content:"\e747"}.van-icon-smile-comment:before{content:"\e748"}.van-icon-user-circle-o:before{content:"\e749"}.van-icon-video-o:before{content:"\e74a"}.van-icon-add-square:before{content:"\e65c"}.van-icon-add:before{content:"\e65d"}.van-icon-arrow-down:before{content:"\e65e"}.van-icon-arrow-up:before{content:"\e65f"}.van-icon-arrow:before{content:"\e660"}.van-icon-after-sale:before{content:"\e661"}.van-icon-add-o:before{content:"\e662"}.van-icon-alipay:before{content:"\e663"}.van-icon-ascending:before{content:"\e664"}.van-icon-apps-o:before{content:"\e665"}.van-icon-aim:before{content:"\e666"}.van-icon-award:before{content:"\e667"}.van-icon-arrow-left:before{content:"\e668"}.van-icon-award-o:before{content:"\e669"}.van-icon-audio:before{content:"\e66a"}.van-icon-bag-o:before{content:"\e66b"}.van-icon-balance-list:before{content:"\e66c"}.van-icon-back-top:before{content:"\e66d"}.van-icon-bag:before{content:"\e66e"}.van-icon-balance-pay:before{content:"\e66f"}.van-icon-balance-o:before{content:"\e670"}.van-icon-bar-chart-o:before{content:"\e671"}.van-icon-bars:before{content:"\e672"}.van-icon-balance-list-o:before{content:"\e673"}.van-icon-birthday-cake-o:before{content:"\e674"}.van-icon-bookmark:before{content:"\e675"}.van-icon-bill:before{content:"\e676"}.van-icon-bell:before{content:"\e677"}.van-icon-browsing-history-o:before{content:"\e678"}.van-icon-browsing-history:before{content:"\e679"}.van-icon-bookmark-o:before{content:"\e67a"}.van-icon-bulb-o:before{content:"\e67b"}.van-icon-bullhorn-o:before{content:"\e67c"}.van-icon-bill-o:before{content:"\e67d"}.van-icon-calendar-o:before{content:"\e67e"}.van-icon-brush-o:before{content:"\e67f"}.van-icon-card:before{content:"\e680"}.van-icon-cart-o:before{content:"\e681"}.van-icon-cart-circle:before{content:"\e682"}.van-icon-cart-circle-o:before{content:"\e683"}.van-icon-cart:before{content:"\e684"}.van-icon-cash-on-deliver:before{content:"\e685"}.van-icon-cash-back-record-o:before{content:"\e686"}.van-icon-cashier-o:before{content:"\e687"}.van-icon-chart-trending-o:before{content:"\e688"}.van-icon-certificate:before{content:"\e689"}.van-icon-chat:before{content:"\e68a"}.van-icon-clear:before{content:"\e68b"}.van-icon-chat-o:before{content:"\e68c"}.van-icon-checked:before{content:"\e68d"}.van-icon-clock:before{content:"\e68e"}.van-icon-clock-o:before{content:"\e68f"}.van-icon-close:before{content:"\e690"}.van-icon-closed-eye:before{content:"\e691"}.van-icon-circle:before{content:"\e692"}.van-icon-cluster-o:before{content:"\e693"}.van-icon-column:before{content:"\e694"}.van-icon-comment-circle-o:before{content:"\e695"}.van-icon-cluster:before{content:"\e696"}.van-icon-comment:before{content:"\e697"}.van-icon-comment-o:before{content:"\e698"}.van-icon-comment-circle:before{content:"\e699"}.van-icon-completed-o:before{content:"\e69a"}.van-icon-credit-pay:before{content:"\e69b"}.van-icon-coupon:before{content:"\e69c"}.van-icon-debit-pay:before{content:"\e69d"}.van-icon-coupon-o:before{content:"\e69e"}.van-icon-contact-o:before{content:"\e69f"}.van-icon-descending:before{content:"\e6a0"}.van-icon-desktop-o:before{content:"\e6a1"}.van-icon-diamond-o:before{content:"\e6a2"}.van-icon-description-o:before{content:"\e6a3"}.van-icon-delete:before{content:"\e6a4"}.van-icon-diamond:before{content:"\e6a5"}.van-icon-delete-o:before{content:"\e6a6"}.van-icon-cross:before{content:"\e6a7"}.van-icon-edit:before{content:"\e6a8"}.van-icon-ellipsis:before{content:"\e6a9"}.van-icon-down:before{content:"\e6aa"}.van-icon-discount-o:before{content:"\e6ab"}.van-icon-ecard-pay:before{content:"\e6ac"}.van-icon-envelop-o:before{content:"\e6ae"}@font-face{font-display:auto;font-family:vant-icon;font-style:normal;font-weight:400;src:url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff2?t=1694918397022) format("woff2"),url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff?t=1694918397022) format("woff")}:host{align-items:center;display:inline-flex;justify-content:center}.van-icon--custom{position:relative}.van-icon--image{height:1em;width:1em}.van-icon__image{height:100%;width:100%}.van-icon__info{z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.js
new file mode 100644
index 0000000..40f6812
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.js
@@ -0,0 +1,66 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+(0, component_1.VantComponent)({
+ mixins: [button_1.button],
+ classes: ['custom-class', 'loading-class', 'error-class', 'image-class'],
+ props: {
+ src: {
+ type: String,
+ observer: function () {
+ this.setData({
+ error: false,
+ loading: true,
+ });
+ },
+ },
+ round: Boolean,
+ width: null,
+ height: null,
+ radius: null,
+ lazyLoad: Boolean,
+ useErrorSlot: Boolean,
+ useLoadingSlot: Boolean,
+ showMenuByLongpress: Boolean,
+ fit: {
+ type: String,
+ value: 'fill',
+ },
+ webp: {
+ type: Boolean,
+ value: false,
+ },
+ showError: {
+ type: Boolean,
+ value: true,
+ },
+ showLoading: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ error: false,
+ loading: true,
+ viewStyle: '',
+ },
+ methods: {
+ onLoad: function (event) {
+ this.setData({
+ loading: false,
+ });
+ this.$emit('load', event.detail);
+ },
+ onError: function (event) {
+ this.setData({
+ loading: false,
+ error: true,
+ });
+ this.$emit('error', event.detail);
+ },
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxml
new file mode 100644
index 0000000..a6f573c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxs
new file mode 100644
index 0000000..cec14b8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ width: addUnit(data.width),
+ height: addUnit(data.height),
+ 'border-radius': addUnit(data.radius),
+ },
+ data.radius ? 'overflow: hidden' : null,
+ ]);
+}
+
+var FIT_MODE_MAP = {
+ none: 'center',
+ fill: 'scaleToFill',
+ cover: 'aspectFill',
+ contain: 'aspectFit',
+ widthFix: 'widthFix',
+ heightFix: 'heightFix',
+};
+
+function mode(fit) {
+ return FIT_MODE_MAP[fit];
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ mode: mode,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxss
new file mode 100644
index 0000000..a9c6ebb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/image/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-image{display:inline-block;position:relative}.van-image--round{border-radius:50%;overflow:hidden}.van-image--round .van-image__img{border-radius:inherit}.van-image__error,.van-image__img,.van-image__loading{display:block;height:100%;width:100%}.van-image__error,.van-image__loading{align-items:center;background-color:var(--image-placeholder-background-color,#f7f8fa);color:var(--image-placeholder-text-color,#969799);display:flex;flex-direction:column;font-size:var(--image-placeholder-font-size,14px);justify-content:center;left:0;position:absolute;top:0}.van-image__loading-icon{color:var(--image-loading-icon-color,#dcdee0);font-size:var(--image-loading-icon-size,32px)!important}.van-image__error-icon{color:var(--image-error-icon-color,#dcdee0);font-size:var(--image-error-icon-size,32px)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.js
new file mode 100644
index 0000000..9a361a9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.js
@@ -0,0 +1,28 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('index-bar'),
+ props: {
+ useSlot: Boolean,
+ index: null,
+ },
+ data: {
+ active: false,
+ wrapperStyle: '',
+ anchorStyle: '',
+ },
+ methods: {
+ scrollIntoView: function (scrollTop) {
+ var _this = this;
+ (0, utils_1.getRect)(this, '.van-index-anchor-wrapper').then(function (rect) {
+ wx.pageScrollTo({
+ duration: 0,
+ scrollTop: scrollTop + rect.top - _this.parent.data.stickyOffsetTop,
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxml
new file mode 100644
index 0000000..49affa7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxml
@@ -0,0 +1,14 @@
+
+
+
+
+ {{ index }}
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxss
new file mode 100644
index 0000000..4b91560
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-anchor/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-anchor{background-color:var(--index-anchor-background-color,transparent);color:var(--index-anchor-text-color,#323233);font-size:var(--index-anchor-font-size,14px);font-weight:var(--index-anchor-font-weight,500);line-height:var(--index-anchor-line-height,32px);padding:var(--index-anchor-padding,0 16px)}.van-index-anchor--active{background-color:var(--index-anchor-active-background-color,#fff);color:var(--index-anchor-active-text-color,#07c160);left:0;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.js
new file mode 100644
index 0000000..afc5412
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.js
@@ -0,0 +1,243 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+var page_scroll_1 = require("../mixins/page-scroll");
+var indexList = function () {
+ var indexList = [];
+ var charCodeOfA = 'A'.charCodeAt(0);
+ for (var i = 0; i < 26; i++) {
+ indexList.push(String.fromCharCode(charCodeOfA + i));
+ }
+ return indexList;
+};
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('index-anchor', function () {
+ this.updateData();
+ }),
+ props: {
+ sticky: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ highlightColor: {
+ type: String,
+ value: color_1.GREEN,
+ },
+ stickyOffsetTop: {
+ type: Number,
+ value: 0,
+ },
+ indexList: {
+ type: Array,
+ value: indexList(),
+ },
+ },
+ mixins: [
+ (0, page_scroll_1.pageScrollMixin)(function (event) {
+ this.scrollTop = (event === null || event === void 0 ? void 0 : event.scrollTop) || 0;
+ this.onScroll();
+ }),
+ ],
+ data: {
+ activeAnchorIndex: null,
+ showSidebar: false,
+ },
+ created: function () {
+ this.scrollTop = 0;
+ },
+ methods: {
+ updateData: function () {
+ var _this = this;
+ wx.nextTick(function () {
+ if (_this.timer != null) {
+ clearTimeout(_this.timer);
+ }
+ _this.timer = setTimeout(function () {
+ _this.setData({
+ showSidebar: !!_this.children.length,
+ });
+ _this.setRect().then(function () {
+ _this.onScroll();
+ });
+ }, 0);
+ });
+ },
+ setRect: function () {
+ return Promise.all([
+ this.setAnchorsRect(),
+ this.setListRect(),
+ this.setSiderbarRect(),
+ ]);
+ },
+ setAnchorsRect: function () {
+ var _this = this;
+ return Promise.all(this.children.map(function (anchor) {
+ return (0, utils_1.getRect)(anchor, '.van-index-anchor-wrapper').then(function (rect) {
+ Object.assign(anchor, {
+ height: rect.height,
+ top: rect.top + _this.scrollTop,
+ });
+ });
+ }));
+ },
+ setListRect: function () {
+ var _this = this;
+ return (0, utils_1.getRect)(this, '.van-index-bar').then(function (rect) {
+ if (!(0, utils_1.isDef)(rect)) {
+ return;
+ }
+ Object.assign(_this, {
+ height: rect.height,
+ top: rect.top + _this.scrollTop,
+ });
+ });
+ },
+ setSiderbarRect: function () {
+ var _this = this;
+ return (0, utils_1.getRect)(this, '.van-index-bar__sidebar').then(function (res) {
+ if (!(0, utils_1.isDef)(res)) {
+ return;
+ }
+ _this.sidebar = {
+ height: res.height,
+ top: res.top,
+ };
+ });
+ },
+ setDiffData: function (_a) {
+ var target = _a.target, data = _a.data;
+ var diffData = {};
+ Object.keys(data).forEach(function (key) {
+ if (target.data[key] !== data[key]) {
+ diffData[key] = data[key];
+ }
+ });
+ if (Object.keys(diffData).length) {
+ target.setData(diffData);
+ }
+ },
+ getAnchorRect: function (anchor) {
+ return (0, utils_1.getRect)(anchor, '.van-index-anchor-wrapper').then(function (rect) { return ({
+ height: rect.height,
+ top: rect.top,
+ }); });
+ },
+ getActiveAnchorIndex: function () {
+ var _a = this, children = _a.children, scrollTop = _a.scrollTop;
+ var _b = this.data, sticky = _b.sticky, stickyOffsetTop = _b.stickyOffsetTop;
+ for (var i = this.children.length - 1; i >= 0; i--) {
+ var preAnchorHeight = i > 0 ? children[i - 1].height : 0;
+ var reachTop = sticky ? preAnchorHeight + stickyOffsetTop : 0;
+ if (reachTop + scrollTop >= children[i].top) {
+ return i;
+ }
+ }
+ return -1;
+ },
+ onScroll: function () {
+ var _this = this;
+ var _a = this, _b = _a.children, children = _b === void 0 ? [] : _b, scrollTop = _a.scrollTop;
+ if (!children.length) {
+ return;
+ }
+ var _c = this.data, sticky = _c.sticky, stickyOffsetTop = _c.stickyOffsetTop, zIndex = _c.zIndex, highlightColor = _c.highlightColor;
+ var active = this.getActiveAnchorIndex();
+ this.setDiffData({
+ target: this,
+ data: {
+ activeAnchorIndex: active,
+ },
+ });
+ if (sticky) {
+ var isActiveAnchorSticky_1 = false;
+ if (active !== -1) {
+ isActiveAnchorSticky_1 =
+ children[active].top <= stickyOffsetTop + scrollTop;
+ }
+ children.forEach(function (item, index) {
+ if (index === active) {
+ var wrapperStyle = '';
+ var anchorStyle = "\n color: ".concat(highlightColor, ";\n ");
+ if (isActiveAnchorSticky_1) {
+ wrapperStyle = "\n height: ".concat(children[index].height, "px;\n ");
+ anchorStyle = "\n position: fixed;\n top: ".concat(stickyOffsetTop, "px;\n z-index: ").concat(zIndex, ";\n color: ").concat(highlightColor, ";\n ");
+ }
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle: anchorStyle,
+ wrapperStyle: wrapperStyle,
+ },
+ });
+ }
+ else if (index === active - 1) {
+ var currentAnchor = children[index];
+ var currentOffsetTop = currentAnchor.top;
+ var targetOffsetTop = index === children.length - 1
+ ? _this.top
+ : children[index + 1].top;
+ var parentOffsetHeight = targetOffsetTop - currentOffsetTop;
+ var translateY = parentOffsetHeight - currentAnchor.height;
+ var anchorStyle = "\n position: relative;\n transform: translate3d(0, ".concat(translateY, "px, 0);\n z-index: ").concat(zIndex, ";\n color: ").concat(highlightColor, ";\n ");
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle: anchorStyle,
+ },
+ });
+ }
+ else {
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: false,
+ anchorStyle: '',
+ wrapperStyle: '',
+ },
+ });
+ }
+ });
+ }
+ },
+ onClick: function (event) {
+ this.scrollToAnchor(event.target.dataset.index);
+ },
+ onTouchMove: function (event) {
+ var sidebarLength = this.children.length;
+ var touch = event.touches[0];
+ var itemHeight = this.sidebar.height / sidebarLength;
+ var index = Math.floor((touch.clientY - this.sidebar.top) / itemHeight);
+ if (index < 0) {
+ index = 0;
+ }
+ else if (index > sidebarLength - 1) {
+ index = sidebarLength - 1;
+ }
+ this.scrollToAnchor(index);
+ },
+ onTouchStop: function () {
+ this.scrollToAnchorIndex = null;
+ },
+ scrollToAnchor: function (index) {
+ var _this = this;
+ if (typeof index !== 'number' || this.scrollToAnchorIndex === index) {
+ return;
+ }
+ this.scrollToAnchorIndex = index;
+ var anchor = this.children.find(function (item) { return item.data.index === _this.data.indexList[index]; });
+ if (anchor) {
+ anchor.scrollIntoView(this.scrollTop);
+ this.$emit('select', anchor.data.index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxml
new file mode 100644
index 0000000..19a59cf
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxss
new file mode 100644
index 0000000..8568801
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/index-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-bar{position:relative}.van-index-bar__sidebar{display:flex;flex-direction:column;position:fixed;right:0;text-align:center;top:50%;transform:translateY(-50%);-webkit-user-select:none;user-select:none}.van-index-bar__index{font-size:var(--index-bar-index-font-size,10px);font-weight:500;line-height:var(--index-bar-index-line-height,14px);padding:0 var(--padding-base,4px) 0 var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.js
new file mode 100644
index 0000000..e61af73
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.js
@@ -0,0 +1,10 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ dot: Boolean,
+ info: null,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxml
new file mode 100644
index 0000000..b39b524
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxml
@@ -0,0 +1,7 @@
+
+
+{{ dot ? '' : info }}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxss
new file mode 100644
index 0000000..375ed5a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/info/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-info{align-items:center;background-color:var(--info-background-color,#ee0a24);border:var(--info-border-width,1px) solid #fff;border-radius:var(--info-size,16px);box-sizing:border-box;color:var(--info-color,#fff);display:inline-flex;font-family:var(--info-font-family,-apple-system-font,Helvetica Neue,Arial,sans-serif);font-size:var(--info-font-size,12px);font-weight:var(--info-font-weight,500);height:var(--info-size,16px);justify-content:center;min-width:var(--info-size,16px);padding:var(--info-padding,0 3px);position:absolute;right:0;top:0;transform:translate(50%,-50%);transform-origin:100%;white-space:nowrap}.van-info--dot{background-color:var(--info-dot-color,#ee0a24);border-radius:100%;height:var(--info-dot-size,8px);min-width:0;width:var(--info-dot-size,8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.js
new file mode 100644
index 0000000..be9c0ef
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.js
@@ -0,0 +1,18 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ color: String,
+ vertical: Boolean,
+ type: {
+ type: String,
+ value: 'circular',
+ },
+ size: String,
+ textSize: String,
+ },
+ data: {
+ array12: Array.from({ length: 12 }),
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxml
new file mode 100644
index 0000000..7d4a539
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxs
new file mode 100644
index 0000000..02a0b80
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function spinnerStyle(data) {
+ return style({
+ color: data.color,
+ width: addUnit(data.size),
+ height: addUnit(data.size),
+ });
+}
+
+function textStyle(data) {
+ return style({
+ 'font-size': addUnit(data.textSize),
+ });
+}
+
+module.exports = {
+ spinnerStyle: spinnerStyle,
+ textStyle: textStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxss
new file mode 100644
index 0000000..fc84e84
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/loading/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{font-size:0;line-height:1}.van-loading{align-items:center;color:var(--loading-spinner-color,#c8c9cc);display:inline-flex;justify-content:center}.van-loading__spinner{animation:van-rotate var(--loading-spinner-animation-duration,.8s) linear infinite;box-sizing:border-box;height:var(--loading-spinner-size,30px);max-height:100%;max-width:100%;position:relative;width:var(--loading-spinner-size,30px)}.van-loading__spinner--spinner{animation-timing-function:steps(12)}.van-loading__spinner--circular{border:1px solid transparent;border-radius:100%;border-top-color:initial}.van-loading__text{color:var(--loading-text-color,#969799);font-size:var(--loading-text-font-size,14px);line-height:var(--loading-text-line-height,20px);margin-left:var(--padding-xs,8px)}.van-loading__text:empty{display:none}.van-loading--vertical{flex-direction:column}.van-loading--vertical .van-loading__text{margin:var(--padding-xs,8px) 0 0}.van-loading__dot{height:100%;left:0;position:absolute;top:0;width:100%}.van-loading__dot:before{background-color:currentColor;border-radius:40%;content:" ";display:block;height:25%;margin:0 auto;width:2px}.van-loading__dot:first-of-type{opacity:1;transform:rotate(30deg)}.van-loading__dot:nth-of-type(2){opacity:.9375;transform:rotate(60deg)}.van-loading__dot:nth-of-type(3){opacity:.875;transform:rotate(90deg)}.van-loading__dot:nth-of-type(4){opacity:.8125;transform:rotate(120deg)}.van-loading__dot:nth-of-type(5){opacity:.75;transform:rotate(150deg)}.van-loading__dot:nth-of-type(6){opacity:.6875;transform:rotate(180deg)}.van-loading__dot:nth-of-type(7){opacity:.625;transform:rotate(210deg)}.van-loading__dot:nth-of-type(8){opacity:.5625;transform:rotate(240deg)}.van-loading__dot:nth-of-type(9){opacity:.5;transform:rotate(270deg)}.van-loading__dot:nth-of-type(10){opacity:.4375;transform:rotate(300deg)}.van-loading__dot:nth-of-type(11){opacity:.375;transform:rotate(330deg)}.van-loading__dot:nth-of-type(12){opacity:.3125;transform:rotate(1turn)}@keyframes van-rotate{0%{transform:rotate(0deg)}to{transform:rotate(1turn)}}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.d.ts
new file mode 100644
index 0000000..b273369
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.d.ts
@@ -0,0 +1 @@
+export declare const basic: string;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.js
new file mode 100644
index 0000000..4373ad4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/basic.js
@@ -0,0 +1,14 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.basic = void 0;
+exports.basic = Behavior({
+ methods: {
+ $emit: function (name, detail, options) {
+ this.triggerEvent(name, detail, options);
+ },
+ set: function (data) {
+ this.setData(data);
+ return new Promise(function (resolve) { return wx.nextTick(resolve); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.d.ts
new file mode 100644
index 0000000..b51db87
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.d.ts
@@ -0,0 +1 @@
+export declare const button: string;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.js
new file mode 100644
index 0000000..8c1c499
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/button.js
@@ -0,0 +1,54 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.button = void 0;
+var version_1 = require("../common/version");
+exports.button = Behavior({
+ externalClasses: ['hover-class'],
+ properties: {
+ id: String,
+ buttonId: String,
+ lang: String,
+ businessId: Number,
+ sessionFrom: String,
+ sendMessageTitle: String,
+ sendMessagePath: String,
+ sendMessageImg: String,
+ showMessageCard: Boolean,
+ appParameter: String,
+ ariaLabel: String,
+ openType: String,
+ getUserProfileDesc: String,
+ },
+ data: {
+ canIUseGetUserProfile: (0, version_1.canIUseGetUserProfile)(),
+ },
+ methods: {
+ onGetUserInfo: function (event) {
+ this.triggerEvent('getuserinfo', event.detail);
+ },
+ onContact: function (event) {
+ this.triggerEvent('contact', event.detail);
+ },
+ onGetPhoneNumber: function (event) {
+ this.triggerEvent('getphonenumber', event.detail);
+ },
+ onGetRealTimePhoneNumber: function (event) {
+ this.triggerEvent('getrealtimephonenumber', event.detail);
+ },
+ onError: function (event) {
+ this.triggerEvent('error', event.detail);
+ },
+ onLaunchApp: function (event) {
+ this.triggerEvent('launchapp', event.detail);
+ },
+ onOpenSetting: function (event) {
+ this.triggerEvent('opensetting', event.detail);
+ },
+ onAgreePrivacyAuthorization: function (event) {
+ this.triggerEvent('agreeprivacyauthorization', event.detail);
+ },
+ onChooseAvatar: function (event) {
+ this.triggerEvent('chooseavatar', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.d.ts
new file mode 100644
index 0000000..d58043b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.d.ts
@@ -0,0 +1 @@
+export declare const link: string;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.js
new file mode 100644
index 0000000..14cb7e8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/link.js
@@ -0,0 +1,27 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.link = void 0;
+exports.link = Behavior({
+ properties: {
+ url: String,
+ linkType: {
+ type: String,
+ value: 'navigateTo',
+ },
+ },
+ methods: {
+ jumpLink: function (urlKey) {
+ if (urlKey === void 0) { urlKey = 'url'; }
+ var url = this.data[urlKey];
+ if (url) {
+ if (this.data.linkType === 'navigateTo' &&
+ getCurrentPages().length > 9) {
+ wx.redirectTo({ url: url });
+ }
+ else {
+ wx[this.data.linkType]({ url: url });
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.d.ts
new file mode 100644
index 0000000..4625447
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.d.ts
@@ -0,0 +1,6 @@
+///
+///
+type IPageScrollOption = WechatMiniprogram.Page.IPageScrollOption;
+type Scroller = (this: WechatMiniprogram.Component.TrivialInstance, event?: IPageScrollOption) => void;
+export declare function pageScrollMixin(scroller: Scroller): string;
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.js
new file mode 100644
index 0000000..fce7049
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/page-scroll.js
@@ -0,0 +1,47 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.pageScrollMixin = void 0;
+var validator_1 = require("../common/validator");
+var utils_1 = require("../common/utils");
+function onPageScroll(event) {
+ var _a = (0, utils_1.getCurrentPage)().vanPageScroller, vanPageScroller = _a === void 0 ? [] : _a;
+ vanPageScroller.forEach(function (scroller) {
+ if (typeof scroller === 'function') {
+ // @ts-ignore
+ scroller(event);
+ }
+ });
+}
+function pageScrollMixin(scroller) {
+ return Behavior({
+ attached: function () {
+ var page = (0, utils_1.getCurrentPage)();
+ if (!(0, utils_1.isDef)(page)) {
+ return;
+ }
+ var _scroller = scroller.bind(this);
+ var _a = page.vanPageScroller, vanPageScroller = _a === void 0 ? [] : _a;
+ if ((0, validator_1.isFunction)(page.onPageScroll) && page.onPageScroll !== onPageScroll) {
+ vanPageScroller.push(page.onPageScroll.bind(page));
+ }
+ vanPageScroller.push(_scroller);
+ page.vanPageScroller = vanPageScroller;
+ page.onPageScroll = onPageScroll;
+ this._scroller = _scroller;
+ },
+ detached: function () {
+ var _this = this;
+ var page = (0, utils_1.getCurrentPage)();
+ if (!(0, utils_1.isDef)(page) || !(0, utils_1.isDef)(page.vanPageScroller)) {
+ return;
+ }
+ var vanPageScroller = page.vanPageScroller;
+ var index = vanPageScroller.findIndex(function (v) { return v === _this._scroller; });
+ if (index > -1) {
+ page.vanPageScroller.splice(index, 1);
+ }
+ this._scroller = undefined;
+ },
+ });
+}
+exports.pageScrollMixin = pageScrollMixin;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.d.ts
new file mode 100644
index 0000000..35ee831
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.d.ts
@@ -0,0 +1 @@
+export declare const touch: string;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.js
new file mode 100644
index 0000000..d762c2c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/touch.js
@@ -0,0 +1,40 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.touch = void 0;
+// @ts-nocheck
+var MIN_DISTANCE = 10;
+function getDirection(x, y) {
+ if (x > y && x > MIN_DISTANCE) {
+ return 'horizontal';
+ }
+ if (y > x && y > MIN_DISTANCE) {
+ return 'vertical';
+ }
+ return '';
+}
+exports.touch = Behavior({
+ methods: {
+ resetTouchStatus: function () {
+ this.direction = '';
+ this.deltaX = 0;
+ this.deltaY = 0;
+ this.offsetX = 0;
+ this.offsetY = 0;
+ },
+ touchStart: function (event) {
+ this.resetTouchStatus();
+ var touch = event.touches[0];
+ this.startX = touch.clientX;
+ this.startY = touch.clientY;
+ },
+ touchMove: function (event) {
+ var touch = event.touches[0];
+ this.deltaX = touch.clientX - this.startX;
+ this.deltaY = touch.clientY - this.startY;
+ this.offsetX = Math.abs(this.deltaX);
+ this.offsetY = Math.abs(this.deltaY);
+ this.direction =
+ this.direction || getDirection(this.offsetX, this.offsetY);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.d.ts
new file mode 100644
index 0000000..dd829e5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.d.ts
@@ -0,0 +1 @@
+export declare function transition(showDefaultValue: boolean): string;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.js
new file mode 100644
index 0000000..922900f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/mixins/transition.js
@@ -0,0 +1,131 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.transition = void 0;
+// @ts-nocheck
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var getClassNames = function (name) { return ({
+ enter: "van-".concat(name, "-enter van-").concat(name, "-enter-active enter-class enter-active-class"),
+ 'enter-to': "van-".concat(name, "-enter-to van-").concat(name, "-enter-active enter-to-class enter-active-class"),
+ leave: "van-".concat(name, "-leave van-").concat(name, "-leave-active leave-class leave-active-class"),
+ 'leave-to': "van-".concat(name, "-leave-to van-").concat(name, "-leave-active leave-to-class leave-active-class"),
+}); };
+function transition(showDefaultValue) {
+ return Behavior({
+ properties: {
+ customStyle: String,
+ // @ts-ignore
+ show: {
+ type: Boolean,
+ value: showDefaultValue,
+ observer: 'observeShow',
+ },
+ // @ts-ignore
+ duration: {
+ type: null,
+ value: 300,
+ observer: 'observeDuration',
+ },
+ name: {
+ type: String,
+ value: 'fade',
+ },
+ },
+ data: {
+ type: '',
+ inited: false,
+ display: false,
+ },
+ ready: function () {
+ if (this.data.show === true) {
+ this.observeShow(true, false);
+ }
+ },
+ methods: {
+ observeShow: function (value, old) {
+ if (value === old) {
+ return;
+ }
+ value ? this.enter() : this.leave();
+ },
+ enter: function () {
+ var _this = this;
+ this.waitEnterEndPromise = new Promise(function (resolve) {
+ var _a = _this.data, duration = _a.duration, name = _a.name;
+ var classNames = getClassNames(name);
+ var currentDuration = (0, validator_1.isObj)(duration) ? duration.enter : duration;
+ if (_this.status === 'enter') {
+ return;
+ }
+ _this.status = 'enter';
+ _this.$emit('before-enter');
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'enter') {
+ return;
+ }
+ _this.$emit('enter');
+ _this.setData({
+ inited: true,
+ display: true,
+ classes: classNames.enter,
+ currentDuration: currentDuration,
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'enter') {
+ return;
+ }
+ _this.transitionEnded = false;
+ _this.setData({ classes: classNames['enter-to'] });
+ resolve();
+ });
+ });
+ });
+ },
+ leave: function () {
+ var _this = this;
+ if (!this.waitEnterEndPromise)
+ return;
+ this.waitEnterEndPromise.then(function () {
+ if (!_this.data.display) {
+ return;
+ }
+ var _a = _this.data, duration = _a.duration, name = _a.name;
+ var classNames = getClassNames(name);
+ var currentDuration = (0, validator_1.isObj)(duration) ? duration.leave : duration;
+ _this.status = 'leave';
+ _this.$emit('before-leave');
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'leave') {
+ return;
+ }
+ _this.$emit('leave');
+ _this.setData({
+ classes: classNames.leave,
+ currentDuration: currentDuration,
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'leave') {
+ return;
+ }
+ _this.transitionEnded = false;
+ setTimeout(function () { return _this.onTransitionEnd(); }, currentDuration);
+ _this.setData({ classes: classNames['leave-to'] });
+ });
+ });
+ });
+ },
+ onTransitionEnd: function () {
+ if (this.transitionEnded) {
+ return;
+ }
+ this.transitionEnded = true;
+ this.$emit("after-".concat(this.status));
+ var _a = this.data, show = _a.show, display = _a.display;
+ if (!show && display) {
+ this.setData({ display: false });
+ }
+ },
+ },
+ });
+}
+exports.transition = transition;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.js
new file mode 100644
index 0000000..376b561
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ classes: ['title-class'],
+ props: {
+ title: String,
+ fixed: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ leftText: String,
+ rightText: String,
+ customStyle: String,
+ leftArrow: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 46,
+ },
+ created: function () {
+ var statusBarHeight = (0, utils_1.getSystemInfoSync)().statusBarHeight;
+ this.setData({
+ statusBarHeight: statusBarHeight,
+ height: 46 + statusBarHeight,
+ });
+ },
+ mounted: function () {
+ this.setHeight();
+ },
+ methods: {
+ onClickLeft: function () {
+ this.$emit('click-left');
+ },
+ onClickRight: function () {
+ this.$emit('click-right');
+ },
+ setHeight: function () {
+ var _this = this;
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(function () {
+ (0, utils_1.getRect)(_this, '.van-nav-bar').then(function (res) {
+ if (res && 'height' in res) {
+ _this.setData({ height: res.height });
+ }
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxml
new file mode 100644
index 0000000..b6405fd
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxml
@@ -0,0 +1,42 @@
+
+
+
+
+
+
+
+
+
+
+ {{ leftText }}
+
+
+
+
+ {{ title }}
+
+
+
+ {{ rightText }}
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxs
new file mode 100644
index 0000000..55b4158
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function barStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ 'padding-top': data.safeAreaInsetTop ? data.statusBarHeight + 'px' : 0,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxss
new file mode 100644
index 0000000..d11c31e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/nav-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-nav-bar{background-color:var(--nav-bar-background-color,#fff);box-sizing:initial;height:var(--nav-bar-height,46px);line-height:var(--nav-bar-height,46px);position:relative;text-align:center;-webkit-user-select:none;user-select:none}.van-nav-bar__content{height:100%;position:relative}.van-nav-bar__text{color:var(--nav-bar-text-color,#1989fa);display:inline-block;margin:0 calc(var(--padding-md, 16px)*-1);padding:0 var(--padding-md,16px);vertical-align:middle}.van-nav-bar__text--hover{background-color:#f2f3f5}.van-nav-bar__arrow{color:var(--nav-bar-icon-color,#1989fa)!important;font-size:var(--nav-bar-arrow-size,16px)!important;vertical-align:middle}.van-nav-bar__arrow+.van-nav-bar__text{margin-left:-20px;padding-left:25px}.van-nav-bar--fixed{left:0;position:fixed;top:0;width:100%}.van-nav-bar__title{color:var(--nav-bar-title-text-color,#323233);font-size:var(--nav-bar-title-font-size,16px);font-weight:var(--font-weight-bold,500);margin:0 auto;max-width:60%}.van-nav-bar__left,.van-nav-bar__right{align-items:center;bottom:0;display:flex;font-size:var(--font-size-md,14px);position:absolute;top:0}.van-nav-bar__left{left:var(--padding-md,16px)}.van-nav-bar__right{right:var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.js
new file mode 100644
index 0000000..db2a755
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.js
@@ -0,0 +1,125 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ text: {
+ type: String,
+ value: '',
+ observer: 'init',
+ },
+ mode: {
+ type: String,
+ value: '',
+ },
+ url: {
+ type: String,
+ value: '',
+ },
+ openType: {
+ type: String,
+ value: 'navigate',
+ },
+ delay: {
+ type: Number,
+ value: 1,
+ },
+ speed: {
+ type: Number,
+ value: 60,
+ observer: 'init',
+ },
+ scrollable: null,
+ leftIcon: {
+ type: String,
+ value: '',
+ },
+ color: String,
+ backgroundColor: String,
+ background: String,
+ wrapable: Boolean,
+ },
+ data: {
+ show: true,
+ },
+ created: function () {
+ this.resetAnimation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'linear',
+ });
+ },
+ destroyed: function () {
+ this.timer && clearTimeout(this.timer);
+ },
+ mounted: function () {
+ this.init();
+ },
+ methods: {
+ init: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ Promise.all([
+ (0, utils_1.getRect)(_this, '.van-notice-bar__content'),
+ (0, utils_1.getRect)(_this, '.van-notice-bar__wrap'),
+ ]).then(function (rects) {
+ var contentRect = rects[0], wrapRect = rects[1];
+ var _a = _this.data, speed = _a.speed, scrollable = _a.scrollable, delay = _a.delay;
+ if (contentRect == null ||
+ wrapRect == null ||
+ !contentRect.width ||
+ !wrapRect.width ||
+ scrollable === false) {
+ return;
+ }
+ if (scrollable || wrapRect.width < contentRect.width) {
+ var duration = ((wrapRect.width + contentRect.width) / speed) * 1000;
+ _this.wrapWidth = wrapRect.width;
+ _this.contentWidth = contentRect.width;
+ _this.duration = duration;
+ _this.animation = wx.createAnimation({
+ duration: duration,
+ timingFunction: 'linear',
+ delay: delay,
+ });
+ _this.scroll(true);
+ }
+ });
+ });
+ },
+ scroll: function (isInit) {
+ var _this = this;
+ if (isInit === void 0) { isInit = false; }
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({
+ animationData: this.resetAnimation
+ .translateX(isInit ? 0 : this.wrapWidth)
+ .step()
+ .export(),
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.setData({
+ animationData: _this.animation
+ .translateX(-_this.contentWidth)
+ .step()
+ .export(),
+ });
+ });
+ this.timer = setTimeout(function () {
+ _this.scroll();
+ }, this.duration + this.data.delay);
+ },
+ onClickIcon: function (event) {
+ if (this.data.mode === 'closeable') {
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({ show: false });
+ this.$emit('close', event.detail);
+ }
+ },
+ onClick: function (event) {
+ this.$emit('click', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxml
new file mode 100644
index 0000000..21b0973
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxml
@@ -0,0 +1,38 @@
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxs
new file mode 100644
index 0000000..11e6456
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ color: data.color,
+ 'background-color': data.backgroundColor,
+ background: data.background,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxss
new file mode 100644
index 0000000..497636c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notice-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notice-bar{align-items:center;background-color:var(--notice-bar-background-color,#fffbe8);color:var(--notice-bar-text-color,#ed6a0c);display:flex;font-size:var(--notice-bar-font-size,14px);height:var(--notice-bar-height,40px);line-height:var(--notice-bar-line-height,24px);padding:var(--notice-bar-padding,0 16px)}.van-notice-bar--withicon{padding-right:40px;position:relative}.van-notice-bar--wrapable{height:auto;padding:var(--notice-bar-wrapable-padding,8px 16px)}.van-notice-bar--wrapable .van-notice-bar__wrap{height:auto}.van-notice-bar--wrapable .van-notice-bar__content{position:relative;white-space:normal}.van-notice-bar__left-icon{align-items:center;display:flex;margin-right:4px;vertical-align:middle}.van-notice-bar__left-icon,.van-notice-bar__right-icon{font-size:var(--notice-bar-icon-size,16px);min-width:var(--notice-bar-icon-min-width,22px)}.van-notice-bar__right-icon{position:absolute;right:15px;top:10px}.van-notice-bar__wrap{flex:1;height:var(--notice-bar-line-height,24px);overflow:hidden;position:relative}.van-notice-bar__content{position:absolute;white-space:nowrap}.van-notice-bar__content.van-ellipsis{max-width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.js
new file mode 100644
index 0000000..a9526aa
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ message: String,
+ background: String,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ color: {
+ type: String,
+ value: color_1.WHITE,
+ },
+ duration: {
+ type: Number,
+ value: 3000,
+ },
+ zIndex: {
+ type: Number,
+ value: 110,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ top: null,
+ },
+ data: {
+ show: false,
+ onOpened: null,
+ onClose: null,
+ onClick: null,
+ },
+ created: function () {
+ var statusBarHeight = (0, utils_1.getSystemInfoSync)().statusBarHeight;
+ this.setData({ statusBarHeight: statusBarHeight });
+ },
+ methods: {
+ show: function () {
+ var _this = this;
+ var _a = this.data, duration = _a.duration, onOpened = _a.onOpened;
+ clearTimeout(this.timer);
+ this.setData({ show: true });
+ wx.nextTick(onOpened);
+ if (duration > 0 && duration !== Infinity) {
+ this.timer = setTimeout(function () {
+ _this.hide();
+ }, duration);
+ }
+ },
+ hide: function () {
+ var onClose = this.data.onClose;
+ clearTimeout(this.timer);
+ this.setData({ show: false });
+ wx.nextTick(onClose);
+ },
+ onTap: function (event) {
+ var onClick = this.data.onClick;
+ if (onClick) {
+ onClick(event.detail);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxml
new file mode 100644
index 0000000..42d913e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+ {{ message }}
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxs
new file mode 100644
index 0000000..bbb94c2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ top: addUnit(data.top),
+ });
+}
+
+function notifyStyle(data) {
+ return style({
+ background: data.background,
+ color: data.color,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ notifyStyle: notifyStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxss
new file mode 100644
index 0000000..c030e9b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notify{word-wrap:break-word;font-size:var(--notify-font-size,14px);line-height:var(--notify-line-height,20px);padding:var(--notify-padding,6px 15px);text-align:center}.van-notify__container{box-sizing:border-box;left:0;position:fixed;top:0;width:100%}.van-notify--primary{background-color:var(--notify-primary-background-color,#1989fa)}.van-notify--success{background-color:var(--notify-success-background-color,#07c160)}.van-notify--danger{background-color:var(--notify-danger-background-color,#ee0a24)}.van-notify--warning{background-color:var(--notify-warning-background-color,#ff976a)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.d.ts
new file mode 100644
index 0000000..d5213cb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.d.ts
@@ -0,0 +1,22 @@
+interface NotifyOptions {
+ type?: 'primary' | 'success' | 'danger' | 'warning';
+ color?: string;
+ zIndex?: number;
+ top?: number;
+ message: string;
+ context?: any;
+ duration?: number;
+ selector?: string;
+ background?: string;
+ safeAreaInsetTop?: boolean;
+ onClick?: () => void;
+ onOpened?: () => void;
+ onClose?: () => void;
+}
+declare function Notify(options: NotifyOptions | string): any;
+declare namespace Notify {
+ var clear: (options?: NotifyOptions | undefined) => void;
+ var setDefaultOptions: (options: NotifyOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Notify;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.js
new file mode 100644
index 0000000..d470431
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/notify/notify.js
@@ -0,0 +1,67 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var defaultOptions = {
+ selector: '#van-notify',
+ type: 'danger',
+ message: '',
+ background: '',
+ duration: 3000,
+ zIndex: 110,
+ top: 0,
+ color: color_1.WHITE,
+ safeAreaInsetTop: false,
+ onClick: function () { },
+ onOpened: function () { },
+ onClose: function () { },
+};
+var currentOptions = __assign({}, defaultOptions);
+function parseOptions(message) {
+ if (message == null) {
+ return {};
+ }
+ return typeof message === 'string' ? { message: message } : message;
+}
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+function Notify(options) {
+ options = __assign(__assign({}, currentOptions), parseOptions(options));
+ var context = options.context || getContext();
+ var notify = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (notify) {
+ notify.setData(options);
+ notify.show();
+ return notify;
+ }
+ console.warn('未找到 van-notify 节点,请确认 selector 及 context 是否正确');
+}
+exports.default = Notify;
+Notify.clear = function (options) {
+ options = __assign(__assign({}, defaultOptions), parseOptions(options));
+ var context = options.context || getContext();
+ var notify = context.selectComponent(options.selector);
+ if (notify) {
+ notify.hide();
+ }
+};
+Notify.setDefaultOptions = function (options) {
+ Object.assign(currentOptions, options);
+};
+Notify.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.js
new file mode 100644
index 0000000..9b58b5f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ show: Boolean,
+ customStyle: String,
+ duration: {
+ type: null,
+ value: 300,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClick: function () {
+ this.$emit('click');
+ },
+ // for prevent touchmove
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxml
new file mode 100644
index 0000000..17fc56f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxml
@@ -0,0 +1,7 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxss
new file mode 100644
index 0000000..d1ad81a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-overlay{background-color:var(--overlay-background-color,rgba(0,0,0,.7));height:100%;left:0;position:fixed;top:0;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/overlay.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/overlay.wxml
new file mode 100644
index 0000000..017e801
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/overlay/overlay.wxml
@@ -0,0 +1,10 @@
+
+
+
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.js
new file mode 100644
index 0000000..818b8c5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['header-class', 'footer-class'],
+ props: {
+ desc: String,
+ title: String,
+ status: String,
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxml
new file mode 100644
index 0000000..1843703
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxss
new file mode 100644
index 0000000..485edcd
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/panel/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-panel{background:var(--panel-background-color,#fff)}.van-panel__header-value{color:var(--panel-header-value-color,#ee0a24)}.van-panel__footer{padding:var(--panel-footer-padding,8px 16px)}.van-panel__footer:empty{display:none}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.js
new file mode 100644
index 0000000..9dbf17c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.js
@@ -0,0 +1,122 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var DEFAULT_DURATION = 200;
+(0, component_1.VantComponent)({
+ classes: ['active-class'],
+ props: {
+ valueKey: String,
+ className: String,
+ itemHeight: Number,
+ visibleItemCount: Number,
+ initialOptions: {
+ type: Array,
+ value: [],
+ },
+ defaultIndex: {
+ type: Number,
+ value: 0,
+ observer: function (value) {
+ this.setIndex(value);
+ },
+ },
+ },
+ data: {
+ startY: 0,
+ offset: 0,
+ duration: 0,
+ startOffset: 0,
+ options: [],
+ currentIndex: 0,
+ },
+ created: function () {
+ var _this = this;
+ var _a = this.data, defaultIndex = _a.defaultIndex, initialOptions = _a.initialOptions;
+ this.set({
+ currentIndex: defaultIndex,
+ options: initialOptions,
+ }).then(function () {
+ _this.setIndex(defaultIndex);
+ });
+ },
+ methods: {
+ getCount: function () {
+ return this.data.options.length;
+ },
+ onTouchStart: function (event) {
+ this.setData({
+ startY: event.touches[0].clientY,
+ startOffset: this.data.offset,
+ duration: 0,
+ });
+ },
+ onTouchMove: function (event) {
+ var data = this.data;
+ var deltaY = event.touches[0].clientY - data.startY;
+ this.setData({
+ offset: (0, utils_1.range)(data.startOffset + deltaY, -(this.getCount() * data.itemHeight), data.itemHeight),
+ });
+ },
+ onTouchEnd: function () {
+ var data = this.data;
+ if (data.offset !== data.startOffset) {
+ this.setData({ duration: DEFAULT_DURATION });
+ var index = (0, utils_1.range)(Math.round(-data.offset / data.itemHeight), 0, this.getCount() - 1);
+ this.setIndex(index, true);
+ }
+ },
+ onClickItem: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.setIndex(index, true);
+ },
+ adjustIndex: function (index) {
+ var data = this.data;
+ var count = this.getCount();
+ index = (0, utils_1.range)(index, 0, count);
+ for (var i = index; i < count; i++) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ for (var i = index - 1; i >= 0; i--) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ },
+ isDisabled: function (option) {
+ return (0, validator_1.isObj)(option) && option.disabled;
+ },
+ getOptionText: function (option) {
+ var data = this.data;
+ return (0, validator_1.isObj)(option) && data.valueKey in option
+ ? option[data.valueKey]
+ : option;
+ },
+ setIndex: function (index, userAction) {
+ var _this = this;
+ var data = this.data;
+ index = this.adjustIndex(index) || 0;
+ var offset = -index * data.itemHeight;
+ if (index !== data.currentIndex) {
+ return this.set({ offset: offset, currentIndex: index }).then(function () {
+ userAction && _this.$emit('change', index);
+ });
+ }
+ return this.set({ offset: offset });
+ },
+ setValue: function (value) {
+ var options = this.data.options;
+ for (var i = 0; i < options.length; i++) {
+ if (this.getOptionText(options[i]) === value) {
+ return this.setIndex(i);
+ }
+ }
+ return Promise.resolve();
+ },
+ getValue: function () {
+ var data = this.data;
+ return data.options[data.currentIndex];
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxml
new file mode 100644
index 0000000..f2c8da2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
+
+ {{ computed.optionText(option, valueKey) }}
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxs
new file mode 100644
index 0000000..2d5a611
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isObj(x) {
+ var type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+
+function optionText(option, valueKey) {
+ return isObj(option) && option[valueKey] != null ? option[valueKey] : option;
+}
+
+function rootStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function wrapperStyle(data) {
+ var offset = addUnit(
+ data.offset + (data.itemHeight * (data.visibleItemCount - 1)) / 2
+ );
+
+ return style({
+ transition: 'transform ' + data.duration + 'ms',
+ 'line-height': addUnit(data.itemHeight),
+ transform: 'translate3d(0, ' + offset + ', 0)',
+ });
+}
+
+module.exports = {
+ optionText: optionText,
+ rootStyle: rootStyle,
+ wrapperStyle: wrapperStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxss
new file mode 100644
index 0000000..519a438
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker-column/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker-column{color:var(--picker-option-text-color,#000);font-size:var(--picker-option-font-size,16px);overflow:hidden;text-align:center}.van-picker-column__item{padding:0 5px}.van-picker-column__item--selected{color:var(--picker-option-selected-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-picker-column__item--disabled{opacity:var(--picker-option-disabled-opacity,.3)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.js
new file mode 100644
index 0000000..06d1826
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.js
@@ -0,0 +1,161 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var shared_1 = require("./shared");
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { valueKey: {
+ type: String,
+ value: 'text',
+ }, toolbarPosition: {
+ type: String,
+ value: 'top',
+ }, defaultIndex: {
+ type: Number,
+ value: 0,
+ }, columns: {
+ type: Array,
+ value: [],
+ observer: function (columns) {
+ if (columns === void 0) { columns = []; }
+ this.simple = columns.length && !columns[0].values;
+ if (Array.isArray(this.children) && this.children.length) {
+ this.setColumns().catch(function () { });
+ }
+ },
+ } }),
+ beforeCreate: function () {
+ var _this = this;
+ Object.defineProperty(this, 'children', {
+ get: function () { return _this.selectAllComponents('.van-picker__column') || []; },
+ });
+ },
+ methods: {
+ noop: function () { },
+ setColumns: function () {
+ var _this = this;
+ var data = this.data;
+ var columns = this.simple ? [{ values: data.columns }] : data.columns;
+ var stack = columns.map(function (column, index) {
+ return _this.setColumnValues(index, column.values);
+ });
+ return Promise.all(stack);
+ },
+ emit: function (event) {
+ var type = event.currentTarget.dataset.type;
+ if (this.simple) {
+ this.$emit(type, {
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit(type, {
+ value: this.getValues(),
+ index: this.getIndexes(),
+ });
+ }
+ },
+ onChange: function (event) {
+ if (this.simple) {
+ this.$emit('change', {
+ picker: this,
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit('change', {
+ picker: this,
+ value: this.getValues(),
+ index: event.currentTarget.dataset.index,
+ });
+ }
+ },
+ // get column instance by index
+ getColumn: function (index) {
+ return this.children[index];
+ },
+ // get column value by index
+ getColumnValue: function (index) {
+ var column = this.getColumn(index);
+ return column && column.getValue();
+ },
+ // set column value by index
+ setColumnValue: function (index, value) {
+ var column = this.getColumn(index);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValue: 对应列不存在'));
+ }
+ return column.setValue(value);
+ },
+ // get column option index by column index
+ getColumnIndex: function (columnIndex) {
+ return (this.getColumn(columnIndex) || {}).data.currentIndex;
+ },
+ // set column option index by column index
+ setColumnIndex: function (columnIndex, optionIndex) {
+ var column = this.getColumn(columnIndex);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnIndex: 对应列不存在'));
+ }
+ return column.setIndex(optionIndex);
+ },
+ // get options of column by index
+ getColumnValues: function (index) {
+ return (this.children[index] || {}).data.options;
+ },
+ // set options of column by index
+ setColumnValues: function (index, options, needReset) {
+ if (needReset === void 0) { needReset = true; }
+ var column = this.children[index];
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValues: 对应列不存在'));
+ }
+ var isSame = JSON.stringify(column.data.options) === JSON.stringify(options);
+ if (isSame) {
+ return Promise.resolve();
+ }
+ return column.set({ options: options }).then(function () {
+ if (needReset) {
+ column.setIndex(0);
+ }
+ });
+ },
+ // get values of all columns
+ getValues: function () {
+ return this.children.map(function (child) { return child.getValue(); });
+ },
+ // set values of all columns
+ setValues: function (values) {
+ var _this = this;
+ var stack = values.map(function (value, index) {
+ return _this.setColumnValue(index, value);
+ });
+ return Promise.all(stack);
+ },
+ // get indexes of all columns
+ getIndexes: function () {
+ return this.children.map(function (child) { return child.data.currentIndex; });
+ },
+ // set indexes of all columns
+ setIndexes: function (indexes) {
+ var _this = this;
+ var stack = indexes.map(function (optionIndex, columnIndex) {
+ return _this.setColumnIndex(columnIndex, optionIndex);
+ });
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.json
new file mode 100644
index 0000000..2fcec89
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "picker-column": "../picker-column/index",
+ "loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxml
new file mode 100644
index 0000000..8564ccc
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxml
@@ -0,0 +1,37 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxs
new file mode 100644
index 0000000..0abbd10
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+var array = require('../wxs/array.wxs');
+
+function columnsStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function maskStyle(data) {
+ return style({
+ 'background-size':
+ '100% ' + addUnit((data.itemHeight * (data.visibleItemCount - 1)) / 2),
+ });
+}
+
+function frameStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight),
+ });
+}
+
+function columns(columns) {
+ if (!array.isArray(columns)) {
+ return [];
+ }
+
+ if (columns.length && !columns[0].values) {
+ return [{ values: columns }];
+ }
+
+ return columns;
+}
+
+module.exports = {
+ columnsStyle: columnsStyle,
+ frameStyle: frameStyle,
+ maskStyle: maskStyle,
+ columns: columns,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxss
new file mode 100644
index 0000000..d924abb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker{-webkit-text-size-adjust:100%;background-color:var(--picker-background-color,#fff);overflow:hidden;position:relative;-webkit-user-select:none;user-select:none}.van-picker__toolbar{display:flex;height:var(--picker-toolbar-height,44px);justify-content:space-between;line-height:var(--picker-toolbar-height,44px)}.van-picker__cancel,.van-picker__confirm{font-size:var(--picker-action-font-size,14px);padding:var(--picker-action-padding,0 16px)}.van-picker__cancel--hover,.van-picker__confirm--hover{opacity:.7}.van-picker__confirm{color:var(--picker-confirm-action-color,#576b95)}.van-picker__cancel{color:var(--picker-cancel-action-color,#969799)}.van-picker__title{font-size:var(--picker-option-font-size,16px);font-weight:var(--font-weight-bold,500);max-width:50%;text-align:center}.van-picker__columns{display:flex;position:relative}.van-picker__column{flex:1 1;width:0}.van-picker__loading{align-items:center;background-color:var(--picker-loading-mask-color,hsla(0,0%,100%,.9));bottom:0;display:flex;justify-content:center;left:0;position:absolute;right:0;top:0;z-index:4}.van-picker__mask{-webkit-backface-visibility:hidden;backface-visibility:hidden;background-image:linear-gradient(180deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4)),linear-gradient(0deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4));background-position:top,bottom;background-repeat:no-repeat;height:100%;left:0;top:0;width:100%;z-index:2}.van-picker__frame,.van-picker__mask{pointer-events:none;position:absolute}.van-picker__frame{left:16px;right:16px;top:50%;transform:translateY(-50%);z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.d.ts
new file mode 100644
index 0000000..c548045
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.d.ts
@@ -0,0 +1,21 @@
+export declare const pickerProps: {
+ title: StringConstructor;
+ loading: BooleanConstructor;
+ showToolbar: BooleanConstructor;
+ cancelButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ confirmButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ visibleItemCount: {
+ type: NumberConstructor;
+ value: number;
+ };
+ itemHeight: {
+ type: NumberConstructor;
+ value: number;
+ };
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.js
new file mode 100644
index 0000000..3d40a8c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/shared.js
@@ -0,0 +1,24 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.pickerProps = void 0;
+exports.pickerProps = {
+ title: String,
+ loading: Boolean,
+ showToolbar: Boolean,
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ visibleItemCount: {
+ type: Number,
+ value: 6,
+ },
+ itemHeight: {
+ type: Number,
+ value: 44,
+ },
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/toolbar.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/toolbar.wxml
new file mode 100644
index 0000000..414f612
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/picker/toolbar.wxml
@@ -0,0 +1,23 @@
+
+
+ {{ cancelButtonText }}
+
+ {{
+ title
+ }}
+
+ {{ confirmButtonText }}
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.js
new file mode 100644
index 0000000..18b08e6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.js
@@ -0,0 +1,99 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var transition_1 = require("../mixins/transition");
+(0, component_1.VantComponent)({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ 'close-icon-class',
+ ],
+ mixins: [(0, transition_1.transition)(false)],
+ props: {
+ round: Boolean,
+ closeable: Boolean,
+ customStyle: String,
+ overlayStyle: String,
+ transition: {
+ type: String,
+ observer: 'observeClass',
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ closeIconPosition: {
+ type: String,
+ value: 'top-right',
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ position: {
+ type: String,
+ value: 'center',
+ observer: 'observeClass',
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ created: function () {
+ this.observeClass();
+ },
+ methods: {
+ onClickCloseIcon: function () {
+ this.$emit('close');
+ },
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ if (this.data.closeOnClickOverlay) {
+ this.$emit('close');
+ }
+ },
+ observeClass: function () {
+ var _a = this.data, transition = _a.transition, position = _a.position, duration = _a.duration;
+ var updateData = {
+ name: transition || position,
+ };
+ if (transition === 'none') {
+ updateData.duration = 0;
+ this.originDuration = duration;
+ }
+ else if (this.originDuration != null) {
+ updateData.duration = this.originDuration;
+ }
+ this.setData(updateData);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.json
new file mode 100644
index 0000000..88a6eab
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-overlay": "../overlay/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxml
new file mode 100644
index 0000000..ab824b1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxs
new file mode 100644
index 0000000..8d59f24
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function popupStyle(data) {
+ return style([
+ {
+ 'z-index': data.zIndex,
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ popupStyle: popupStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxss
new file mode 100644
index 0000000..91983b4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-popup{-webkit-overflow-scrolling:touch;animation:ease both;background-color:var(--popup-background-color,#fff);box-sizing:border-box;max-height:100%;overflow-y:auto;position:fixed;transition-timing-function:ease}.van-popup--center{left:50%;top:50%;transform:translate3d(-50%,-50%,0)}.van-popup--center.van-popup--round{border-radius:var(--popup-round-border-radius,16px)}.van-popup--top{left:0;top:0;width:100%}.van-popup--top.van-popup--round{border-radius:0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--right{right:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--right.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--bottom{bottom:0;left:0;width:100%}.van-popup--bottom.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0}.van-popup--left{left:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--left.van-popup--round{border-radius:0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0}.van-popup--bottom.van-popup--safe{padding-bottom:env(safe-area-inset-bottom)}.van-popup--bottom.van-popup--safeTabBar,.van-popup--top.van-popup--safeTabBar{bottom:var(--tabbar-height,50px)}.van-popup--safeTop{padding-top:env(safe-area-inset-top)}.van-popup__close-icon{color:var(--popup-close-icon-color,#969799);font-size:var(--popup-close-icon-size,18px);position:absolute;z-index:var(--popup-close-icon-z-index,1)}.van-popup__close-icon--top-left{left:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--top-right{right:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-left{bottom:var(--popup-close-icon-margin,16px);left:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-right{bottom:var(--popup-close-icon-margin,16px);right:var(--popup-close-icon-margin,16px)}.van-popup__close-icon:active{opacity:.6}.van-scale-enter-active,.van-scale-leave-active{transition-property:opacity,transform}.van-scale-enter,.van-scale-leave-to{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-center-enter-active,.van-center-leave-active{transition-property:opacity}.van-center-enter,.van-center-leave-to{opacity:0}.van-bottom-enter-active,.van-bottom-leave-active,.van-left-enter-active,.van-left-leave-active,.van-right-enter-active,.van-right-leave-active,.van-top-enter-active,.van-top-leave-active{transition-property:transform}.van-bottom-enter,.van-bottom-leave-to{transform:translate3d(0,100%,0)}.van-top-enter,.van-top-leave-to{transform:translate3d(0,-100%,0)}.van-left-enter,.van-left-leave-to{transform:translate3d(-100%,-50%,0)}.van-right-enter,.van-right-leave-to{transform:translate3d(100%,-50%,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/popup.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/popup.wxml
new file mode 100644
index 0000000..bd8f508
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/popup/popup.wxml
@@ -0,0 +1,16 @@
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.js
new file mode 100644
index 0000000..3bca928
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.js
@@ -0,0 +1,55 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ inactive: Boolean,
+ percentage: {
+ type: Number,
+ observer: 'setLeft',
+ },
+ pivotText: String,
+ pivotColor: String,
+ trackColor: String,
+ showPivot: {
+ type: Boolean,
+ value: true,
+ },
+ color: {
+ type: String,
+ value: color_1.BLUE,
+ },
+ textColor: {
+ type: String,
+ value: '#fff',
+ },
+ strokeWidth: {
+ type: null,
+ value: 4,
+ },
+ },
+ data: {
+ right: 0,
+ },
+ mounted: function () {
+ this.setLeft();
+ },
+ methods: {
+ setLeft: function () {
+ var _this = this;
+ Promise.all([
+ (0, utils_1.getRect)(this, '.van-progress'),
+ (0, utils_1.getRect)(this, '.van-progress__pivot'),
+ ]).then(function (_a) {
+ var portion = _a[0], pivot = _a[1];
+ if (portion && pivot) {
+ _this.setData({
+ right: (pivot.width * (_this.data.percentage - 100)) / 100,
+ });
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxml
new file mode 100644
index 0000000..e81514d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+ {{ computed.pivotText(pivotText, percentage) }}
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxs
new file mode 100644
index 0000000..5b1e8e6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function pivotText(pivotText, percentage) {
+ return pivotText || percentage + '%';
+}
+
+function rootStyle(data) {
+ return style({
+ 'height': data.strokeWidth ? utils.addUnit(data.strokeWidth) : '',
+ 'background': data.trackColor,
+ });
+}
+
+function portionStyle(data) {
+ return style({
+ background: data.inactive ? '#cacaca' : data.color,
+ width: data.percentage ? data.percentage + '%' : '',
+ });
+}
+
+function pivotStyle(data) {
+ return style({
+ color: data.textColor,
+ right: data.right + 'px',
+ background: data.pivotColor ? data.pivotColor : data.inactive ? '#cacaca' : data.color,
+ });
+}
+
+module.exports = {
+ pivotText: pivotText,
+ rootStyle: rootStyle,
+ portionStyle: portionStyle,
+ pivotStyle: pivotStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxss
new file mode 100644
index 0000000..a08972a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/progress/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-progress{background:var(--progress-background-color,#ebedf0);border-radius:var(--progress-height,4px);height:var(--progress-height,4px);position:relative}.van-progress__portion{background:var(--progress-color,#1989fa);border-radius:inherit;height:100%;left:0;position:absolute}.van-progress__pivot{background-color:var(--progress-pivot-background-color,#1989fa);border-radius:1em;box-sizing:border-box;color:var(--progress-pivot-text-color,#fff);font-size:var(--progress-pivot-font-size,10px);line-height:var(--progress-pivot-line-height,1.6);min-width:3.6em;padding:var(--progress-pivot-padding,0 5px);position:absolute;text-align:center;top:50%;transform:translateY(-50%);word-break:keep-all}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.js
new file mode 100644
index 0000000..ddb2a60
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.js
@@ -0,0 +1,24 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useChildren)('radio'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ direction: String,
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ this.children.forEach(function (child) { return child.updateFromParent(); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxml
new file mode 100644
index 0000000..0ab17af
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxss
new file mode 100644
index 0000000..4e3b5d4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.js
new file mode 100644
index 0000000..61a86d5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var version_1 = require("../common/version");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useParent)('radio-group', function () {
+ this.updateFromParent();
+ }),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ name: null,
+ value: null,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ direction: '',
+ parentDisabled: false,
+ },
+ methods: {
+ updateFromParent: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent.data, value = _a.value, parentDisabled = _a.disabled, direction = _a.direction;
+ this.setData({
+ value: value,
+ direction: direction,
+ parentDisabled: parentDisabled,
+ });
+ },
+ emitChange: function (value) {
+ var instance = this.parent || this;
+ instance.$emit('input', value);
+ instance.$emit('change', value);
+ if ((0, version_1.canIUseModel)()) {
+ instance.setData({ value: value });
+ }
+ },
+ onChange: function () {
+ if (!this.data.disabled && !this.data.parentDisabled) {
+ this.emitChange(this.data.name);
+ }
+ },
+ onClickLabel: function () {
+ var _a = this.data, disabled = _a.disabled, parentDisabled = _a.parentDisabled, labelDisabled = _a.labelDisabled, name = _a.name;
+ if (!(disabled || parentDisabled) && !labelDisabled) {
+ this.emitChange(name);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxml
new file mode 100644
index 0000000..5f898c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxml
@@ -0,0 +1,30 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxs
new file mode 100644
index 0000000..a428aad
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxs
@@ -0,0 +1,33 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(data) {
+ var styles = {
+ 'font-size': addUnit(data.iconSize),
+ };
+
+ if (
+ data.checkedColor &&
+ !(data.disabled || data.parentDisabled) &&
+ data.value === data.name
+ ) {
+ styles['border-color'] = data.checkedColor;
+ styles['background-color'] = data.checkedColor;
+ }
+
+ return style(styles);
+}
+
+function iconCustomStyle(data) {
+ return style({
+ 'line-height': addUnit(data.iconSize),
+ 'font-size': '.8em',
+ display: 'block',
+ });
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+ iconCustomStyle: iconCustomStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxss
new file mode 100644
index 0000000..257b0c7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/radio/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-radio__icon-wrap{flex:none}.van-radio--horizontal{margin-right:var(--padding-sm,12px)}.van-radio__icon{align-items:center;border:1px solid var(--radio-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--radio-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--radio-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-radio__icon--round{border-radius:100%}.van-radio__icon--checked{background-color:var(--radio-checked-icon-color,#1989fa);border-color:var(--radio-checked-icon-color,#1989fa);color:#fff}.van-radio__icon--disabled{background-color:var(--radio-disabled-background-color,#ebedf0);border-color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__icon--disabled.van-radio__icon--checked{color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__label{word-wrap:break-word;color:var(--radio-label-color,#323233);line-height:var(--radio-size,20px);padding-left:var(--radio-label-margin,10px)}.van-radio__label--left{float:left;margin:0 var(--radio-label-margin,10px) 0 0}.van-radio__label--disabled{color:var(--radio-disabled-label-color,#c8c9cc)}.van-radio__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.js
new file mode 100644
index 0000000..30a96de
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.js
@@ -0,0 +1,93 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var version_1 = require("../common/version");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['icon-class'],
+ props: {
+ value: {
+ type: Number,
+ observer: function (value) {
+ if (value !== this.data.innerValue) {
+ this.setData({ innerValue: value });
+ }
+ },
+ },
+ readonly: Boolean,
+ disabled: Boolean,
+ allowHalf: Boolean,
+ size: null,
+ icon: {
+ type: String,
+ value: 'star',
+ },
+ voidIcon: {
+ type: String,
+ value: 'star-o',
+ },
+ color: String,
+ voidColor: String,
+ disabledColor: String,
+ count: {
+ type: Number,
+ value: 5,
+ observer: function (value) {
+ this.setData({ innerCountArray: Array.from({ length: value }) });
+ },
+ },
+ gutter: null,
+ touchable: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ innerValue: 0,
+ innerCountArray: Array.from({ length: 5 }),
+ },
+ methods: {
+ onSelect: function (event) {
+ var _this = this;
+ var data = this.data;
+ var score = event.currentTarget.dataset.score;
+ if (!data.disabled && !data.readonly) {
+ this.setData({ innerValue: score + 1 });
+ if ((0, version_1.canIUseModel)()) {
+ this.setData({ value: score + 1 });
+ }
+ wx.nextTick(function () {
+ _this.$emit('input', score + 1);
+ _this.$emit('change', score + 1);
+ });
+ }
+ },
+ onTouchMove: function (event) {
+ var _this = this;
+ var touchable = this.data.touchable;
+ if (!touchable)
+ return;
+ var clientX = event.touches[0].clientX;
+ (0, utils_1.getAllRect)(this, '.van-rate__icon').then(function (list) {
+ var target = list
+ .sort(function (cur, next) { return cur.dataset.score - next.dataset.score; })
+ .find(function (item) { return clientX >= item.left && clientX <= item.right; });
+ if (target != null) {
+ _this.onSelect(__assign(__assign({}, event), { currentTarget: target }));
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxml
new file mode 100644
index 0000000..049714c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxss
new file mode 100644
index 0000000..470e4f4
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/rate/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-rate{display:inline-flex;-webkit-user-select:none;user-select:none}.van-rate__item{padding:0 var(--rate-horizontal-padding,2px);position:relative}.van-rate__item:not(:last-child){padding-right:var(--rate-icon-gutter,4px)}.van-rate__icon{color:var(--rate-icon-void-color,#c8c9cc);display:block;font-size:var(--rate-icon-size,20px);height:100%}.van-rate__icon--half{left:var(--rate-horizontal-padding,2px);overflow:hidden;position:absolute;top:0;width:.5em}.van-rate__icon--full,.van-rate__icon--half{color:var(--rate-icon-full-color,#ee0a24)}.van-rate__icon--disabled{color:var(--rate-icon-disabled-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.js
new file mode 100644
index 0000000..c27acd6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.js
@@ -0,0 +1,26 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('col', function (target) {
+ var gutter = this.data.gutter;
+ if (gutter) {
+ target.setData({ gutter: gutter });
+ }
+ }),
+ props: {
+ gutter: {
+ type: Number,
+ observer: 'setGutter',
+ },
+ },
+ methods: {
+ setGutter: function () {
+ var _this = this;
+ this.children.forEach(function (col) {
+ col.setData(_this.data);
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxml
new file mode 100644
index 0000000..69a4359
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxs
new file mode 100644
index 0000000..f5c5958
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'margin-right': addUnit(-data.gutter / 2),
+ 'margin-left': addUnit(-data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxss
new file mode 100644
index 0000000..bb8946b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/row/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-row:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.js
new file mode 100644
index 0000000..b5348b0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.js
@@ -0,0 +1,100 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var version_1 = require("../common/version");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['field-class', 'input-class', 'cancel-class'],
+ props: {
+ value: {
+ type: String,
+ value: '',
+ },
+ label: String,
+ focus: Boolean,
+ error: Boolean,
+ disabled: Boolean,
+ readonly: Boolean,
+ inputAlign: String,
+ showAction: Boolean,
+ useActionSlot: Boolean,
+ useLeftIconSlot: Boolean,
+ useRightIconSlot: Boolean,
+ leftIcon: {
+ type: String,
+ value: 'search',
+ },
+ rightIcon: String,
+ placeholder: String,
+ placeholderStyle: String,
+ actionText: {
+ type: String,
+ value: '取消',
+ },
+ background: {
+ type: String,
+ value: '#ffffff',
+ },
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ shape: {
+ type: String,
+ value: 'square',
+ },
+ clearable: {
+ type: Boolean,
+ value: true,
+ },
+ clearTrigger: {
+ type: String,
+ value: 'focus',
+ },
+ clearIcon: {
+ type: String,
+ value: 'clear',
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 0,
+ },
+ },
+ methods: {
+ onChange: function (event) {
+ if ((0, version_1.canIUseModel)()) {
+ this.setData({ value: event.detail });
+ }
+ this.$emit('change', event.detail);
+ },
+ onCancel: function () {
+ var _this = this;
+ /**
+ * 修复修改输入框值时,输入框失焦和赋值同时触发,赋值失效
+ * https://github.com/youzan/vant-weapp/issues/1768
+ */
+ setTimeout(function () {
+ if ((0, version_1.canIUseModel)()) {
+ _this.setData({ value: '' });
+ }
+ _this.$emit('cancel');
+ _this.$emit('change', '');
+ }, 200);
+ },
+ onSearch: function (event) {
+ this.$emit('search', event.detail);
+ },
+ onFocus: function (event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ this.$emit('blur', event.detail);
+ },
+ onClear: function (event) {
+ this.$emit('clear', event.detail);
+ },
+ onClickInput: function (event) {
+ this.$emit('click-input', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.json
new file mode 100644
index 0000000..b4cfe91
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-field": "../field/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxml
new file mode 100644
index 0000000..cbcb47a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+ {{ label }}
+
+
+
+
+
+
+
+
+
+
+ {{ actionText }}
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxss
new file mode 100644
index 0000000..4f306b0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/search/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-search{align-items:center;box-sizing:border-box;display:flex;padding:var(--search-padding,10px 12px)}.van-search__content{background-color:var(--search-background-color,#f7f8fa);border-radius:2px;display:flex;flex:1;padding-left:var(--padding-sm,12px)}.van-search__content--round{border-radius:999px}.van-search__label{color:var(--search-label-color,#323233);font-size:var(--search-label-font-size,14px);line-height:var(--search-input-height,34px);padding:var(--search-label-padding,0 5px)}.van-search__field{flex:1}.van-search__field__left-icon{color:var(--search-left-icon-color,#969799)}.van-search--withaction{padding-right:0}.van-search__action{color:var(--search-action-text-color,#323233);font-size:var(--search-action-font-size,14px);line-height:var(--search-input-height,34px)}.van-search__action--hover{background-color:#f2f3f5}.van-search__action-button{padding:var(--search-action-padding,0 8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.js
new file mode 100644
index 0000000..11604a7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.js
@@ -0,0 +1,61 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ // whether to show popup
+ show: Boolean,
+ // overlay custom style
+ overlayStyle: String,
+ // z-index
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ title: String,
+ cancelText: {
+ type: String,
+ value: '取消',
+ },
+ description: String,
+ options: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: null,
+ value: 300,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ },
+ onCancel: function () {
+ this.onClose();
+ this.$emit('cancel');
+ },
+ onSelect: function (event) {
+ this.$emit('select', event.detail);
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.json
new file mode 100644
index 0000000..15a7c22
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "options": "./options"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxml
new file mode 100644
index 0000000..72a5b25
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxs
new file mode 100644
index 0000000..2149ee9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+function isMulti(options) {
+ if (options == null || options[0] == null) {
+ return false;
+ }
+
+ return "Array" === options.constructor && "Array" === options[0].constructor;
+}
+
+module.exports = {
+ isMulti: isMulti
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxss
new file mode 100644
index 0000000..e8d8dae
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__header{padding:12px 16px 4px;text-align:center}.van-share-sheet__title{color:#323233;font-size:14px;font-weight:400;line-height:20px;margin-top:8px}.van-share-sheet__title:empty,.van-share-sheet__title:not(:empty)+.van-share-sheet__title{display:none}.van-share-sheet__description{color:#969799;display:block;font-size:12px;line-height:16px;margin-top:8px}.van-share-sheet__description:empty,.van-share-sheet__description:not(:empty)+.van-share-sheet__description{display:none}.van-share-sheet__cancel{background:#fff;border:none;box-sizing:initial;display:block;font-size:16px;height:auto;line-height:48px;padding:0;text-align:center;width:100%}.van-share-sheet__cancel:before{background-color:#f7f8fa;content:" ";display:block;height:8px}.van-share-sheet__cancel:after{display:none}.van-share-sheet__cancel:active{background-color:#f2f3f5}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.js
new file mode 100644
index 0000000..0432d4f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.js
@@ -0,0 +1,27 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ options: Array,
+ showBorder: Boolean,
+ },
+ methods: {
+ onSelect: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var option = this.data.options[index];
+ this.$emit('select', __assign(__assign({}, option), { index: index }));
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxml
new file mode 100644
index 0000000..2983ebb
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxs
new file mode 100644
index 0000000..a116d32
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var PRESET_ICONS = ['qq', 'link', 'weibo', 'wechat', 'poster', 'qrcode', 'weapp-qrcode', 'wechat-moments'];
+
+function getIconURL(icon) {
+ if (PRESET_ICONS.indexOf(icon) !== -1) {
+ return 'https://img.yzcdn.cn/vant/share-sheet-' + icon + '.png';
+ }
+
+ return icon;
+}
+
+module.exports = {
+ getIconURL: getIconURL,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxss
new file mode 100644
index 0000000..b7f5455
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/share-sheet/options.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__options{-webkit-overflow-scrolling:touch;display:flex;overflow-x:auto;overflow-y:visible;padding:16px 0 16px 8px;position:relative}.van-share-sheet__options--border:before{border-top:1px solid #ebedf0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:0;top:0;transform:scaleY(.5);transform-origin:center}.van-share-sheet__options::-webkit-scrollbar{height:0}.van-share-sheet__option{align-items:center;display:flex;flex-direction:column;-webkit-user-select:none;user-select:none}.van-share-sheet__option:active{opacity:.7}.van-share-sheet__button{background-color:initial;border:0;height:auto;line-height:inherit;padding:0}.van-share-sheet__button:after{border:0}.van-share-sheet__icon{height:48px;margin:0 16px;width:48px}.van-share-sheet__name{color:#646566;font-size:12px;margin-top:8px;padding:0 4px}.van-share-sheet__option-description{color:#c8c9cc;font-size:12px;padding:0 4px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.js
new file mode 100644
index 0000000..eac568f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'disabled-class'],
+ relation: (0, relation_1.useParent)('sidebar'),
+ props: {
+ dot: Boolean,
+ badge: null,
+ info: null,
+ title: String,
+ disabled: Boolean,
+ },
+ methods: {
+ onClick: function () {
+ var _this = this;
+ var parent = this.parent;
+ if (!parent || this.data.disabled) {
+ return;
+ }
+ var index = parent.children.indexOf(this);
+ parent.setActive(index).then(function () {
+ _this.$emit('click', index);
+ parent.$emit('change', index);
+ });
+ },
+ setActive: function (selected) {
+ return this.setData({ selected: selected });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxml
new file mode 100644
index 0000000..c5c08a6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxml
@@ -0,0 +1,18 @@
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxss
new file mode 100644
index 0000000..f1ce421
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar-item{background-color:var(--sidebar-background-color,#f7f8fa);border-left:3px solid transparent;box-sizing:border-box;color:var(--sidebar-text-color,#323233);display:block;font-size:var(--sidebar-font-size,14px);line-height:var(--sidebar-line-height,20px);overflow:hidden;padding:var(--sidebar-padding,20px 12px 20px 8px);-webkit-user-select:none;user-select:none}.van-sidebar-item__text{display:inline-block;position:relative;word-break:break-all}.van-sidebar-item--hover:not(.van-sidebar-item--disabled){background-color:var(--sidebar-active-color,#f2f3f5)}.van-sidebar-item:after{border-bottom-width:1px}.van-sidebar-item--selected{border-color:var(--sidebar-selected-border-color,#ee0a24);color:var(--sidebar-selected-text-color,#323233);font-weight:var(--sidebar-selected-font-weight,500)}.van-sidebar-item--selected:after{border-right-width:1px}.van-sidebar-item--selected,.van-sidebar-item--selected.van-sidebar-item--hover{background-color:var(--sidebar-selected-background-color,#fff)}.van-sidebar-item--disabled{color:var(--sidebar-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.js
new file mode 100644
index 0000000..f3e0a58
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.js
@@ -0,0 +1,36 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('sidebar-item', function () {
+ this.setActive(this.data.activeKey);
+ }),
+ props: {
+ activeKey: {
+ type: Number,
+ value: 0,
+ observer: 'setActive',
+ },
+ },
+ beforeCreate: function () {
+ this.currentActive = -1;
+ },
+ methods: {
+ setActive: function (activeKey) {
+ var _a = this, children = _a.children, currentActive = _a.currentActive;
+ if (!children.length) {
+ return Promise.resolve();
+ }
+ this.currentActive = activeKey;
+ var stack = [];
+ if (currentActive !== activeKey && children[currentActive]) {
+ stack.push(children[currentActive].setActive(false));
+ }
+ if (children[activeKey]) {
+ stack.push(children[activeKey].setActive(true));
+ }
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxml
new file mode 100644
index 0000000..96b11c7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxml
@@ -0,0 +1,3 @@
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxss
new file mode 100644
index 0000000..5a2d44f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sidebar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar{width:var(--sidebar-width,80px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.js
new file mode 100644
index 0000000..2ab3175
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.js
@@ -0,0 +1,48 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['avatar-class', 'title-class', 'row-class'],
+ props: {
+ row: {
+ type: Number,
+ value: 0,
+ observer: function (value) {
+ this.setData({ rowArray: Array.from({ length: value }) });
+ },
+ },
+ title: Boolean,
+ avatar: Boolean,
+ loading: {
+ type: Boolean,
+ value: true,
+ },
+ animate: {
+ type: Boolean,
+ value: true,
+ },
+ avatarSize: {
+ type: String,
+ value: '32px',
+ },
+ avatarShape: {
+ type: String,
+ value: 'round',
+ },
+ titleWidth: {
+ type: String,
+ value: '40%',
+ },
+ rowWidth: {
+ type: null,
+ value: '100%',
+ observer: function (val) {
+ this.setData({ isArray: val instanceof Array });
+ },
+ },
+ },
+ data: {
+ isArray: false,
+ rowArray: [],
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxml
new file mode 100644
index 0000000..058e2ef
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxml
@@ -0,0 +1,29 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxss
new file mode 100644
index 0000000..d59a5ed
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/skeleton/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-skeleton{box-sizing:border-box;display:flex;padding:var(--skeleton-padding,0 16px);width:100%}.van-skeleton__avatar{background-color:var(--skeleton-avatar-background-color,#f2f3f5);flex-shrink:0;margin-right:var(--padding-md,16px)}.van-skeleton__avatar--round{border-radius:100%}.van-skeleton__content{flex:1}.van-skeleton__avatar+.van-skeleton__content{padding-top:var(--padding-xs,8px)}.van-skeleton__row,.van-skeleton__title{background-color:var(--skeleton-row-background-color,#f2f3f5);height:var(--skeleton-row-height,16px)}.van-skeleton__title{margin:0}.van-skeleton__row:not(:first-child){margin-top:var(--skeleton-row-margin-top,12px)}.van-skeleton__title+.van-skeleton__row{margin-top:20px}.van-skeleton--animate{animation:van-skeleton-blink 1.2s ease-in-out infinite}@keyframes van-skeleton-blink{50%{opacity:.6}}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.js
new file mode 100644
index 0000000..92adca8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.js
@@ -0,0 +1,206 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var version_1 = require("../common/version");
+var utils_1 = require("../common/utils");
+var DRAG_STATUS = {
+ START: 'start',
+ MOVING: 'moving',
+ END: 'end',
+};
+(0, component_1.VantComponent)({
+ mixins: [touch_1.touch],
+ props: {
+ range: Boolean,
+ disabled: Boolean,
+ useButtonSlot: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ max: {
+ type: Number,
+ value: 100,
+ },
+ min: {
+ type: Number,
+ value: 0,
+ },
+ step: {
+ type: Number,
+ value: 1,
+ },
+ value: {
+ type: null,
+ value: 0,
+ observer: function (val) {
+ if (val !== this.value) {
+ this.updateValue(val);
+ }
+ },
+ },
+ vertical: Boolean,
+ barHeight: null,
+ },
+ created: function () {
+ this.updateValue(this.data.value);
+ },
+ methods: {
+ onTouchStart: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ var index = event.currentTarget.dataset.index;
+ if (typeof index === 'number') {
+ this.buttonIndex = index;
+ }
+ this.touchStart(event);
+ this.startValue = this.format(this.value);
+ this.newValue = this.value;
+ if (this.isRange(this.newValue)) {
+ this.startValue = this.newValue.map(function (val) { return _this.format(val); });
+ }
+ else {
+ this.startValue = this.format(this.newValue);
+ }
+ this.dragStatus = DRAG_STATUS.START;
+ },
+ onTouchMove: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.START) {
+ this.$emit('drag-start');
+ }
+ this.touchMove(event);
+ this.dragStatus = DRAG_STATUS.MOVING;
+ (0, utils_1.getRect)(this, '.van-slider').then(function (rect) {
+ var vertical = _this.data.vertical;
+ var delta = vertical ? _this.deltaY : _this.deltaX;
+ var total = vertical ? rect.height : rect.width;
+ var diff = (delta / total) * _this.getRange();
+ if (_this.isRange(_this.startValue)) {
+ _this.newValue[_this.buttonIndex] =
+ _this.startValue[_this.buttonIndex] + diff;
+ }
+ else {
+ _this.newValue = _this.startValue + diff;
+ }
+ _this.updateValue(_this.newValue, false, true);
+ });
+ },
+ onTouchEnd: function () {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.MOVING) {
+ this.dragStatus = DRAG_STATUS.END;
+ (0, utils_1.nextTick)(function () {
+ _this.updateValue(_this.newValue, true);
+ _this.$emit('drag-end');
+ });
+ }
+ },
+ onClick: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ var min = this.data.min;
+ (0, utils_1.getRect)(this, '.van-slider').then(function (rect) {
+ var vertical = _this.data.vertical;
+ var touch = event.touches[0];
+ var delta = vertical
+ ? touch.clientY - rect.top
+ : touch.clientX - rect.left;
+ var total = vertical ? rect.height : rect.width;
+ var value = Number(min) + (delta / total) * _this.getRange();
+ if (_this.isRange(_this.value)) {
+ var _a = _this.value, left = _a[0], right = _a[1];
+ var middle = (left + right) / 2;
+ if (value <= middle) {
+ _this.updateValue([value, right], true);
+ }
+ else {
+ _this.updateValue([left, value], true);
+ }
+ }
+ else {
+ _this.updateValue(value, true);
+ }
+ });
+ },
+ isRange: function (val) {
+ var range = this.data.range;
+ return range && Array.isArray(val);
+ },
+ handleOverlap: function (value) {
+ if (value[0] > value[1]) {
+ return value.slice(0).reverse();
+ }
+ return value;
+ },
+ updateValue: function (value, end, drag) {
+ var _this = this;
+ if (this.isRange(value)) {
+ value = this.handleOverlap(value).map(function (val) { return _this.format(val); });
+ }
+ else {
+ value = this.format(value);
+ }
+ this.value = value;
+ var vertical = this.data.vertical;
+ var mainAxis = vertical ? 'height' : 'width';
+ this.setData({
+ wrapperStyle: "\n background: ".concat(this.data.inactiveColor || '', ";\n ").concat(vertical ? 'width' : 'height', ": ").concat((0, utils_1.addUnit)(this.data.barHeight) || '', ";\n "),
+ barStyle: "\n ".concat(mainAxis, ": ").concat(this.calcMainAxis(), ";\n left: ").concat(vertical ? 0 : this.calcOffset(), ";\n top: ").concat(vertical ? this.calcOffset() : 0, ";\n ").concat(drag ? 'transition: none;' : '', "\n "),
+ });
+ if (drag) {
+ this.$emit('drag', { value: value });
+ }
+ if (end) {
+ this.$emit('change', value);
+ }
+ if ((drag || end) && (0, version_1.canIUseModel)()) {
+ this.setData({ value: value });
+ }
+ },
+ getScope: function () {
+ return Number(this.data.max) - Number(this.data.min);
+ },
+ getRange: function () {
+ var _a = this.data, max = _a.max, min = _a.min;
+ return max - min;
+ },
+ getOffsetWidth: function (current, min) {
+ var scope = this.getScope();
+ // 避免最小值小于最小step时出现负数情况
+ return "".concat(Math.max(((current - min) * 100) / scope, 0), "%");
+ },
+ // 计算选中条的长度百分比
+ calcMainAxis: function () {
+ var value = this.value;
+ var min = this.data.min;
+ if (this.isRange(value)) {
+ return this.getOffsetWidth(value[1], value[0]);
+ }
+ return this.getOffsetWidth(value, Number(min));
+ },
+ // 计算选中条的开始位置的偏移量
+ calcOffset: function () {
+ var value = this.value;
+ var min = this.data.min;
+ var scope = this.getScope();
+ if (this.isRange(value)) {
+ return "".concat(((value[0] - Number(min)) * 100) / scope, "%");
+ }
+ return '0%';
+ },
+ format: function (value) {
+ var min = +this.data.min;
+ var max = +this.data.max;
+ var step = +this.data.step;
+ value = (0, utils_1.clamp)(value, min, max);
+ var diff = Math.round((value - min) / step) * step;
+ return (0, utils_1.addNumber)(min, diff);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxml
new file mode 100644
index 0000000..7c0184f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxml
@@ -0,0 +1,68 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxs
new file mode 100644
index 0000000..7c43e6e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function barStyle(barHeight, activeColor) {
+ return style({
+ height: addUnit(barHeight),
+ background: activeColor,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxss
new file mode 100644
index 0000000..d1587de
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/slider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-slider{background-color:var(--slider-inactive-background-color,#ebedf0);border-radius:999px;height:var(--slider-bar-height,2px);position:relative}.van-slider:before{bottom:calc(var(--padding-xs, 8px)*-1);content:"";left:0;position:absolute;right:0;top:calc(var(--padding-xs, 8px)*-1)}.van-slider__bar{background-color:var(--slider-active-background-color,#1989fa);border-radius:inherit;height:100%;position:relative;transition:all .2s;width:100%}.van-slider__button{background-color:var(--slider-button-background-color,#fff);border-radius:var(--slider-button-border-radius,50%);box-shadow:var(--slider-button-box-shadow,0 1px 2px rgba(0,0,0,.5));height:var(--slider-button-height,24px);width:var(--slider-button-width,24px)}.van-slider__button-wrapper,.van-slider__button-wrapper-right{position:absolute;right:0;top:50%;transform:translate3d(50%,-50%,0)}.van-slider__button-wrapper-left{left:0;position:absolute;top:50%;transform:translate3d(-50%,-50%,0)}.van-slider--disabled{opacity:var(--slider-disabled-opacity,.5)}.van-slider--vertical{display:inline-block;height:100%;width:var(--slider-bar-height,2px)}.van-slider--vertical .van-slider__button-wrapper,.van-slider--vertical .van-slider__button-wrapper-right{bottom:0;right:50%;top:auto;transform:translate3d(50%,50%,0)}.van-slider--vertical .van-slider__button-wrapper-left{left:auto;right:50%;top:0;transform:translate3d(50%,-50%,0)}.van-slider--vertical:before{bottom:0;left:-8px;right:-8px;top:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.js
new file mode 100644
index 0000000..1aa2c27
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.js
@@ -0,0 +1,203 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var LONG_PRESS_START_TIME = 600;
+var LONG_PRESS_INTERVAL = 200;
+// add num and avoid float number
+function add(num1, num2) {
+ var cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+function equal(value1, value2) {
+ return String(value1) === String(value2);
+}
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['input-class', 'plus-class', 'minus-class'],
+ props: {
+ value: {
+ type: null,
+ },
+ integer: {
+ type: Boolean,
+ observer: 'check',
+ },
+ disabled: Boolean,
+ inputWidth: String,
+ buttonSize: String,
+ asyncChange: Boolean,
+ disableInput: Boolean,
+ decimalLength: {
+ type: Number,
+ value: null,
+ observer: 'check',
+ },
+ min: {
+ type: null,
+ value: 1,
+ observer: 'check',
+ },
+ max: {
+ type: null,
+ value: Number.MAX_SAFE_INTEGER,
+ observer: 'check',
+ },
+ step: {
+ type: null,
+ value: 1,
+ },
+ showPlus: {
+ type: Boolean,
+ value: true,
+ },
+ showMinus: {
+ type: Boolean,
+ value: true,
+ },
+ disablePlus: Boolean,
+ disableMinus: Boolean,
+ longPress: {
+ type: Boolean,
+ value: true,
+ },
+ theme: String,
+ alwaysEmbed: Boolean,
+ },
+ data: {
+ currentValue: '',
+ },
+ watch: {
+ value: function () {
+ this.observeValue();
+ },
+ },
+ created: function () {
+ this.setData({
+ currentValue: this.format(this.data.value),
+ });
+ },
+ methods: {
+ observeValue: function () {
+ var value = this.data.value;
+ this.setData({ currentValue: this.format(value) });
+ },
+ check: function () {
+ var val = this.format(this.data.currentValue);
+ if (!equal(val, this.data.currentValue)) {
+ this.setData({ currentValue: val });
+ }
+ },
+ isDisabled: function (type) {
+ var _a = this.data, disabled = _a.disabled, disablePlus = _a.disablePlus, disableMinus = _a.disableMinus, currentValue = _a.currentValue, max = _a.max, min = _a.min;
+ if (type === 'plus') {
+ return disabled || disablePlus || +currentValue >= +max;
+ }
+ return disabled || disableMinus || +currentValue <= +min;
+ },
+ onFocus: function (event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ var value = this.format(event.detail.value);
+ this.setData({ currentValue: value });
+ this.emitChange(value);
+ this.$emit('blur', __assign(__assign({}, event.detail), { value: value }));
+ },
+ // filter illegal characters
+ filter: function (value) {
+ value = String(value).replace(/[^0-9.-]/g, '');
+ if (this.data.integer && value.indexOf('.') !== -1) {
+ value = value.split('.')[0];
+ }
+ return value;
+ },
+ // limit value range
+ format: function (value) {
+ value = this.filter(value);
+ // format range
+ value = value === '' ? 0 : +value;
+ value = Math.max(Math.min(this.data.max, value), this.data.min);
+ // format decimal
+ if ((0, validator_1.isDef)(this.data.decimalLength)) {
+ value = value.toFixed(this.data.decimalLength);
+ }
+ return value;
+ },
+ onInput: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ // allow input to be empty
+ if (value === '') {
+ return;
+ }
+ var formatted = this.filter(value);
+ // limit max decimal length
+ if ((0, validator_1.isDef)(this.data.decimalLength) && formatted.indexOf('.') !== -1) {
+ var pair = formatted.split('.');
+ formatted = "".concat(pair[0], ".").concat(pair[1].slice(0, this.data.decimalLength));
+ }
+ this.emitChange(formatted);
+ },
+ emitChange: function (value) {
+ if (!this.data.asyncChange) {
+ this.setData({ currentValue: value });
+ }
+ this.$emit('change', value);
+ },
+ onChange: function () {
+ var type = this.type;
+ if (this.isDisabled(type)) {
+ this.$emit('overlimit', type);
+ return;
+ }
+ var diff = type === 'minus' ? -this.data.step : +this.data.step;
+ var value = this.format(add(+this.data.currentValue, diff));
+ this.emitChange(value);
+ this.$emit(type);
+ },
+ longPressStep: function () {
+ var _this = this;
+ this.longPressTimer = setTimeout(function () {
+ _this.onChange();
+ _this.longPressStep();
+ }, LONG_PRESS_INTERVAL);
+ },
+ onTap: function (event) {
+ var type = event.currentTarget.dataset.type;
+ this.type = type;
+ this.onChange();
+ },
+ onTouchStart: function (event) {
+ var _this = this;
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ var type = event.currentTarget.dataset.type;
+ this.type = type;
+ this.isLongPress = false;
+ this.longPressTimer = setTimeout(function () {
+ _this.isLongPress = true;
+ _this.onChange();
+ _this.longPressStep();
+ }, LONG_PRESS_START_TIME);
+ },
+ onTouchEnd: function () {
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxml
new file mode 100644
index 0000000..6dd44bc
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxml
@@ -0,0 +1,43 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxs
new file mode 100644
index 0000000..a13e818
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function buttonStyle(data) {
+ return style({
+ width: addUnit(data.buttonSize),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+function inputStyle(data) {
+ return style({
+ width: addUnit(data.inputWidth),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+module.exports = {
+ buttonStyle: buttonStyle,
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxss
new file mode 100644
index 0000000..2561a7e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/stepper/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-stepper{font-size:0}.van-stepper__minus,.van-stepper__plus{background-color:var(--stepper-background-color,#f2f3f5);border:0;box-sizing:border-box;color:var(--stepper-button-icon-color,#323233);display:inline-block;height:var(--stepper-input-height,28px);margin:1px;padding:var(--padding-base,4px);position:relative;vertical-align:middle;width:var(--stepper-input-height,28px)}.van-stepper__minus:before,.van-stepper__plus:before{height:1px;width:9px}.van-stepper__minus:after,.van-stepper__plus:after{height:9px;width:1px}.van-stepper__minus:empty.van-stepper__minus:after,.van-stepper__minus:empty.van-stepper__minus:before,.van-stepper__minus:empty.van-stepper__plus:after,.van-stepper__minus:empty.van-stepper__plus:before,.van-stepper__plus:empty.van-stepper__minus:after,.van-stepper__plus:empty.van-stepper__minus:before,.van-stepper__plus:empty.van-stepper__plus:after,.van-stepper__plus:empty.van-stepper__plus:before{background-color:currentColor;bottom:0;content:"";left:0;margin:auto;position:absolute;right:0;top:0}.van-stepper__minus--hover,.van-stepper__plus--hover{background-color:var(--stepper-active-color,#e8e8e8)}.van-stepper__minus--disabled,.van-stepper__plus--disabled{color:var(--stepper-button-disabled-icon-color,#c8c9cc)}.van-stepper__minus--disabled,.van-stepper__minus--disabled.van-stepper__minus--hover,.van-stepper__minus--disabled.van-stepper__plus--hover,.van-stepper__plus--disabled,.van-stepper__plus--disabled.van-stepper__minus--hover,.van-stepper__plus--disabled.van-stepper__plus--hover{background-color:var(--stepper-button-disabled-color,#f7f8fa)}.van-stepper__minus{border-radius:var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0 0 var(--stepper-border-radius,var(--stepper-border-radius,4px))}.van-stepper__minus:after{display:none}.van-stepper__plus{border-radius:0 var(--stepper-border-radius,var(--stepper-border-radius,4px)) var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0}.van-stepper--round .van-stepper__input{background-color:initial!important}.van-stepper--round .van-stepper__minus,.van-stepper--round .van-stepper__plus{border-radius:100%}.van-stepper--round .van-stepper__minus:active,.van-stepper--round .van-stepper__plus:active{opacity:.7}.van-stepper--round .van-stepper__minus--disabled,.van-stepper--round .van-stepper__minus--disabled:active,.van-stepper--round .van-stepper__plus--disabled,.van-stepper--round .van-stepper__plus--disabled:active{opacity:.3}.van-stepper--round .van-stepper__plus{background-color:#ee0a24;color:#fff}.van-stepper--round .van-stepper__minus{background-color:#fff;border:1px solid #ee0a24;color:#ee0a24}.van-stepper__input{-webkit-appearance:none;background-color:var(--stepper-background-color,#f2f3f5);border:0;border-radius:0;border-width:1px 0;box-sizing:border-box;color:var(--stepper-input-text-color,#323233);display:inline-block;font-size:var(--stepper-input-font-size,14px);height:var(--stepper-input-height,28px);margin:1px;min-height:0;padding:1px;text-align:center;vertical-align:middle;width:var(--stepper-input-width,32px)}.van-stepper__input--disabled{background-color:var(--stepper-input-disabled-background-color,#f2f3f5);color:var(--stepper-input-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.js
new file mode 100644
index 0000000..1a9986a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.js
@@ -0,0 +1,35 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+(0, component_1.VantComponent)({
+ classes: ['desc-class'],
+ props: {
+ icon: String,
+ steps: Array,
+ active: Number,
+ direction: {
+ type: String,
+ value: 'horizontal',
+ },
+ activeColor: {
+ type: String,
+ value: color_1.GREEN,
+ },
+ inactiveColor: {
+ type: String,
+ value: color_1.GRAY_DARK,
+ },
+ activeIcon: {
+ type: String,
+ value: 'checked',
+ },
+ inactiveIcon: String,
+ },
+ methods: {
+ onClick: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.$emit('click-step', index);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxml
new file mode 100644
index 0000000..00c8e10
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+ {{ item.text }}
+ {{ item.desc }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+function get(index, active) {
+ if (index < active) {
+ return 'finish';
+ } else if (index === active) {
+ return 'process';
+ }
+
+ return 'inactive';
+}
+
+module.exports = get;
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxss
new file mode 100644
index 0000000..c653884
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/steps/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-steps{background-color:var(--steps-background-color,#fff);overflow:hidden}.van-steps--horizontal{padding:10px}.van-steps--horizontal .van-step__wrapper{display:flex;overflow:hidden;position:relative}.van-steps--vertical{padding-left:10px}.van-steps--vertical .van-step__wrapper{padding:0 0 0 20px}.van-step{color:var(--step-text-color,#969799);flex:1;font-size:var(--step-font-size,14px);position:relative}.van-step--finish{color:var(--step-finish-text-color,#323233)}.van-step__circle{background-color:var(--step-circle-color,#969799);border-radius:50%;height:var(--step-circle-size,5px);width:var(--step-circle-size,5px)}.van-step--horizontal{padding-bottom:14px}.van-step--horizontal:first-child .van-step__title{transform:none}.van-step--horizontal:first-child .van-step__circle-container{padding:0 8px 0 0;transform:translate3d(0,50%,0)}.van-step--horizontal:last-child{position:absolute;right:0;width:auto}.van-step--horizontal:last-child .van-step__title{text-align:right;transform:none}.van-step--horizontal:last-child .van-step__circle-container{padding:0 0 0 8px;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal .van-step__circle-container{background-color:#fff;bottom:6px;padding:0 var(--padding-xs,8px);position:absolute;transform:translate3d(-50%,50%,0);z-index:1}.van-step--horizontal .van-step__title{display:inline-block;font-size:var(--step-horizontal-title-font-size,12px);transform:translate3d(-50%,0,0)}.van-step--horizontal .van-step__line{background-color:var(--step-line-color,#ebedf0);bottom:6px;height:1px;left:0;position:absolute;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal.van-step--process{color:var(--step-process-text-color,#323233)}.van-step--horizontal.van-step--process .van-step__icon{display:block;font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical{line-height:18px;padding:10px 10px 10px 0}.van-step--vertical:after{border-bottom-width:1px}.van-step--vertical:last-child:after{border-bottom-width:none}.van-step--vertical:first-child:before{background-color:#fff;content:"";height:20px;left:-15px;position:absolute;top:0;width:1px;z-index:1}.van-step--vertical .van-step__circle,.van-step--vertical .van-step__icon,.van-step--vertical .van-step__line{left:-14px;position:absolute;top:19px;transform:translate3d(-50%,-50%,0);z-index:2}.van-step--vertical .van-step__icon{background-color:var(--steps-background-color,#fff);font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical .van-step__line{background-color:var(--step-line-color,#ebedf0);height:100%;transform:translate3d(-50%,0,0);width:1px;z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.js
new file mode 100644
index 0000000..ffeb125
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.js
@@ -0,0 +1,126 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var page_scroll_1 = require("../mixins/page-scroll");
+var ROOT_ELEMENT = '.van-sticky';
+(0, component_1.VantComponent)({
+ props: {
+ zIndex: {
+ type: Number,
+ value: 99,
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ observer: 'onScroll',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'onScroll',
+ },
+ container: {
+ type: null,
+ observer: 'onScroll',
+ },
+ scrollTop: {
+ type: null,
+ observer: function (val) {
+ this.onScroll({ scrollTop: val });
+ },
+ },
+ },
+ mixins: [
+ (0, page_scroll_1.pageScrollMixin)(function (event) {
+ if (this.data.scrollTop != null) {
+ return;
+ }
+ this.onScroll(event);
+ }),
+ ],
+ data: {
+ height: 0,
+ fixed: false,
+ transform: 0,
+ },
+ mounted: function () {
+ this.onScroll();
+ },
+ methods: {
+ onScroll: function (_a) {
+ var _this = this;
+ var _b = _a === void 0 ? {} : _a, scrollTop = _b.scrollTop;
+ var _c = this.data, container = _c.container, offsetTop = _c.offsetTop, disabled = _c.disabled;
+ if (disabled) {
+ this.setDataAfterDiff({
+ fixed: false,
+ transform: 0,
+ });
+ return;
+ }
+ this.scrollTop = scrollTop || this.scrollTop;
+ if (typeof container === 'function') {
+ Promise.all([(0, utils_1.getRect)(this, ROOT_ELEMENT), this.getContainerRect()])
+ .then(function (_a) {
+ var root = _a[0], container = _a[1];
+ if (offsetTop + root.height > container.height + container.top) {
+ _this.setDataAfterDiff({
+ fixed: false,
+ transform: container.height - root.height,
+ });
+ }
+ else if (offsetTop >= root.top) {
+ _this.setDataAfterDiff({
+ fixed: true,
+ height: root.height,
+ transform: 0,
+ });
+ }
+ else {
+ _this.setDataAfterDiff({ fixed: false, transform: 0 });
+ }
+ })
+ .catch(function () { });
+ return;
+ }
+ (0, utils_1.getRect)(this, ROOT_ELEMENT).then(function (root) {
+ if (!(0, validator_1.isDef)(root) || (!root.width && !root.height)) {
+ return;
+ }
+ if (offsetTop >= root.top) {
+ _this.setDataAfterDiff({ fixed: true, height: root.height });
+ _this.transform = 0;
+ }
+ else {
+ _this.setDataAfterDiff({ fixed: false });
+ }
+ });
+ },
+ setDataAfterDiff: function (data) {
+ var _this = this;
+ wx.nextTick(function () {
+ var diff = Object.keys(data).reduce(function (prev, key) {
+ if (data[key] !== _this.data[key]) {
+ prev[key] = data[key];
+ }
+ return prev;
+ }, {});
+ if (Object.keys(diff).length > 0) {
+ _this.setData(diff);
+ }
+ _this.$emit('scroll', {
+ scrollTop: _this.scrollTop,
+ isFixed: data.fixed || _this.data.fixed,
+ });
+ });
+ },
+ getContainerRect: function () {
+ var nodesRef = this.data.container();
+ if (!nodesRef) {
+ return Promise.reject(new Error('not found container'));
+ }
+ return new Promise(function (resolve) { return nodesRef.boundingClientRect(resolve).exec(); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxml
new file mode 100644
index 0000000..15e9f4a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxs
new file mode 100644
index 0000000..be99d89
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapStyle(data) {
+ return style({
+ transform: data.transform
+ ? 'translate3d(0, ' + data.transform + 'px, 0)'
+ : '',
+ top: data.fixed ? addUnit(data.offsetTop) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+function containerStyle(data) {
+ return style({
+ height: data.fixed ? addUnit(data.height) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+module.exports = {
+ wrapStyle: wrapStyle,
+ containerStyle: containerStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxss
new file mode 100644
index 0000000..34d76aa
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/sticky/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sticky{position:relative}.van-sticky-wrap--fixed{left:0;position:fixed;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.js
new file mode 100644
index 0000000..d3bfc25
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.js
@@ -0,0 +1,58 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['bar-class', 'price-class', 'button-class'],
+ props: {
+ tip: {
+ type: null,
+ observer: 'updateTip',
+ },
+ tipIcon: String,
+ type: Number,
+ price: {
+ type: null,
+ observer: 'updatePrice',
+ },
+ label: String,
+ loading: Boolean,
+ disabled: Boolean,
+ buttonText: String,
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ buttonType: {
+ type: String,
+ value: 'danger',
+ },
+ decimalLength: {
+ type: Number,
+ value: 2,
+ observer: 'updatePrice',
+ },
+ suffixLabel: String,
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updatePrice: function () {
+ var _a = this.data, price = _a.price, decimalLength = _a.decimalLength;
+ var priceStrArr = typeof price === 'number' &&
+ (price / 100).toFixed(decimalLength).split('.');
+ this.setData({
+ hasPrice: typeof price === 'number',
+ integerStr: priceStrArr && priceStrArr[0],
+ decimalStr: decimalLength && priceStrArr ? ".".concat(priceStrArr[1]) : '',
+ });
+ },
+ updateTip: function () {
+ this.setData({ hasTip: typeof this.data.tip === 'string' });
+ },
+ onSubmit: function (event) {
+ this.$emit('submit', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.json
new file mode 100644
index 0000000..bda9b8d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxml
new file mode 100644
index 0000000..a56dd46
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxml
@@ -0,0 +1,44 @@
+
+
+
+
+
+
+
+
+ {{ tip }}
+
+
+
+
+
+
+
+ {{ label || '合计:' }}
+
+ {{ currency }}
+ {{ integerStr }}{{decimalStr}}
+
+ {{ suffixLabel }}
+
+
+ {{ loading ? '' : buttonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxss
new file mode 100644
index 0000000..8379a30
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/submit-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-submit-bar{background-color:var(--submit-bar-background-color,#fff);bottom:0;left:0;position:fixed;-webkit-user-select:none;user-select:none;width:100%;z-index:var(--submit-bar-z-index,100)}.van-submit-bar__tip{background-color:var(--submit-bar-tip-background-color,#fff7cc);color:var(--submit-bar-tip-color,#f56723);font-size:var(--submit-bar-tip-font-size,12px);line-height:var(--submit-bar-tip-line-height,1.5);padding:var(--submit-bar-tip-padding,10px)}.van-submit-bar__tip:empty{display:none}.van-submit-bar__tip-icon{margin-right:4px;vertical-align:middle}.van-submit-bar__tip-text{display:inline;vertical-align:middle}.van-submit-bar__bar{align-items:center;background-color:var(--submit-bar-background-color,#fff);display:flex;font-size:var(--submit-bar-text-font-size,14px);height:var(--submit-bar-height,50px);justify-content:flex-end;padding:var(--submit-bar-padding,0 16px)}.van-submit-bar__safe{height:constant(safe-area-inset-bottom);height:env(safe-area-inset-bottom)}.van-submit-bar__text{color:var(--submit-bar-text-color,#323233);flex:1;font-weight:var(--font-weight-bold,500);padding-right:var(--padding-sm,12px);text-align:right}.van-submit-bar__price{color:var(--submit-bar-price-color,#ee0a24);font-size:var(--submit-bar-price-font-size,12px);font-weight:var(--font-weight-bold,500)}.van-submit-bar__price-integer{font-family:Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif;font-size:20px}.van-submit-bar__currency{font-size:var(--submit-bar-currency-font-size,12px)}.van-submit-bar__suffix-label{margin-left:5px}.van-submit-bar__button{--button-default-height:var(--submit-bar-button-height,40px)!important;--button-line-height:var(--submit-bar-button-height,40px)!important;font-weight:var(--font-weight-bold,500);width:var(--submit-bar-button-width,110px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.js
new file mode 100644
index 0000000..1582b6c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.js
@@ -0,0 +1,135 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var utils_1 = require("../common/utils");
+var THRESHOLD = 0.3;
+var ARRAY = [];
+(0, component_1.VantComponent)({
+ props: {
+ disabled: Boolean,
+ leftWidth: {
+ type: Number,
+ value: 0,
+ observer: function (leftWidth) {
+ if (leftWidth === void 0) { leftWidth = 0; }
+ if (this.offset > 0) {
+ this.swipeMove(leftWidth);
+ }
+ },
+ },
+ rightWidth: {
+ type: Number,
+ value: 0,
+ observer: function (rightWidth) {
+ if (rightWidth === void 0) { rightWidth = 0; }
+ if (this.offset < 0) {
+ this.swipeMove(-rightWidth);
+ }
+ },
+ },
+ asyncClose: Boolean,
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ mixins: [touch_1.touch],
+ data: {
+ catchMove: false,
+ wrapperStyle: '',
+ },
+ created: function () {
+ this.offset = 0;
+ ARRAY.push(this);
+ },
+ destroyed: function () {
+ var _this = this;
+ ARRAY = ARRAY.filter(function (item) { return item !== _this; });
+ },
+ methods: {
+ open: function (position) {
+ var _a = this.data, leftWidth = _a.leftWidth, rightWidth = _a.rightWidth;
+ var offset = position === 'left' ? leftWidth : -rightWidth;
+ this.swipeMove(offset);
+ this.$emit('open', {
+ position: position,
+ name: this.data.name,
+ });
+ },
+ close: function () {
+ this.swipeMove(0);
+ },
+ swipeMove: function (offset) {
+ if (offset === void 0) { offset = 0; }
+ this.offset = (0, utils_1.range)(offset, -this.data.rightWidth, this.data.leftWidth);
+ var transform = "translate3d(".concat(this.offset, "px, 0, 0)");
+ var transition = this.dragging
+ ? 'none'
+ : 'transform .6s cubic-bezier(0.18, 0.89, 0.32, 1)';
+ this.setData({
+ wrapperStyle: "\n -webkit-transform: ".concat(transform, ";\n -webkit-transition: ").concat(transition, ";\n transform: ").concat(transform, ";\n transition: ").concat(transition, ";\n "),
+ });
+ },
+ swipeLeaveTransition: function () {
+ var _a = this.data, leftWidth = _a.leftWidth, rightWidth = _a.rightWidth;
+ var offset = this.offset;
+ if (rightWidth > 0 && -offset > rightWidth * THRESHOLD) {
+ this.open('right');
+ }
+ else if (leftWidth > 0 && offset > leftWidth * THRESHOLD) {
+ this.open('left');
+ }
+ else {
+ this.swipeMove(0);
+ }
+ this.setData({ catchMove: false });
+ },
+ startDrag: function (event) {
+ if (this.data.disabled) {
+ return;
+ }
+ this.startOffset = this.offset;
+ this.touchStart(event);
+ },
+ noop: function () { },
+ onDrag: function (event) {
+ var _this = this;
+ if (this.data.disabled) {
+ return;
+ }
+ this.touchMove(event);
+ if (this.direction !== 'horizontal') {
+ return;
+ }
+ this.dragging = true;
+ ARRAY.filter(function (item) { return item !== _this && item.offset !== 0; }).forEach(function (item) { return item.close(); });
+ this.setData({ catchMove: true });
+ this.swipeMove(this.startOffset + this.deltaX);
+ },
+ endDrag: function () {
+ if (this.data.disabled) {
+ return;
+ }
+ this.dragging = false;
+ this.swipeLeaveTransition();
+ },
+ onClick: function (event) {
+ var _a = event.currentTarget.dataset.key, position = _a === void 0 ? 'outside' : _a;
+ this.$emit('click', position);
+ if (!this.offset) {
+ return;
+ }
+ if (this.data.asyncClose) {
+ this.$emit('close', {
+ position: position,
+ instance: this,
+ name: this.data.name,
+ });
+ }
+ else {
+ this.swipeMove(0);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxml
new file mode 100644
index 0000000..3f7f726
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxss
new file mode 100644
index 0000000..3a265bf
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/swipe-cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-swipe-cell{overflow:hidden;position:relative}.van-swipe-cell__left,.van-swipe-cell__right{height:100%;position:absolute;top:0}.van-swipe-cell__left{left:0;transform:translate3d(-100%,0,0)}.van-swipe-cell__right{right:0;transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.js
new file mode 100644
index 0000000..1d2317f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.js
@@ -0,0 +1,38 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['node-class'],
+ props: {
+ checked: null,
+ loading: Boolean,
+ disabled: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ size: {
+ type: String,
+ value: '30',
+ },
+ activeValue: {
+ type: null,
+ value: true,
+ },
+ inactiveValue: {
+ type: null,
+ value: false,
+ },
+ },
+ methods: {
+ onClick: function () {
+ var _a = this.data, activeValue = _a.activeValue, inactiveValue = _a.inactiveValue, disabled = _a.disabled, loading = _a.loading;
+ if (disabled || loading) {
+ return;
+ }
+ var checked = this.data.checked === activeValue;
+ var value = checked ? inactiveValue : activeValue;
+ this.$emit('input', value);
+ this.$emit('change', value);
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.json
new file mode 100644
index 0000000..01077f5
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxml
new file mode 100644
index 0000000..4e9789b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxml
@@ -0,0 +1,16 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxs
new file mode 100644
index 0000000..3ae387a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxs
@@ -0,0 +1,26 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ var currentColor = data.checked === data.activeValue ? data.activeColor : data.inactiveColor;
+
+ return style({
+ 'font-size': addUnit(data.size),
+ 'background-color': currentColor,
+ });
+}
+
+var BLUE = '#1989fa';
+var GRAY_DARK = '#969799';
+
+function loadingColor(data) {
+ return data.checked === data.activeValue
+ ? data.activeColor || BLUE
+ : data.inactiveColor || GRAY_DARK;
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxss
new file mode 100644
index 0000000..35929de
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/switch/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-switch{background-color:var(--switch-background-color,#fff);border:var(--switch-border,1px solid rgba(0,0,0,.1));border-radius:var(--switch-node-size,1em);box-sizing:initial;display:inline-block;height:var(--switch-height,1em);position:relative;transition:background-color var(--switch-transition-duration,.3s);width:var(--switch-width,2em)}.van-switch__node{background-color:var(--switch-node-background-color,#fff);border-radius:100%;box-shadow:var(--switch-node-box-shadow,0 3px 1px 0 rgba(0,0,0,.05),0 2px 2px 0 rgba(0,0,0,.1),0 3px 3px 0 rgba(0,0,0,.05));height:var(--switch-node-size,1em);left:0;position:absolute;top:0;transition:var(--switch-transition-duration,.3s) cubic-bezier(.3,1.05,.4,1.05);width:var(--switch-node-size,1em);z-index:var(--switch-node-z-index,1)}.van-switch__loading{height:50%;left:25%;position:absolute!important;top:25%;width:50%}.van-switch--on{background-color:var(--switch-on-background-color,#1989fa)}.van-switch--on .van-switch__node{transform:translateX(calc(var(--switch-width, 2em) - var(--switch-node-size, 1em)))}.van-switch--disabled{opacity:var(--switch-disabled-opacity,.4)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.js
new file mode 100644
index 0000000..ae4d06b
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.js
@@ -0,0 +1,58 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('tabs'),
+ props: {
+ dot: {
+ type: Boolean,
+ observer: 'update',
+ },
+ info: {
+ type: null,
+ observer: 'update',
+ },
+ title: {
+ type: String,
+ observer: 'update',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'update',
+ },
+ titleStyle: {
+ type: String,
+ observer: 'update',
+ },
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ data: {
+ active: false,
+ },
+ methods: {
+ getComputedName: function () {
+ if (this.data.name !== '') {
+ return this.data.name;
+ }
+ return this.index;
+ },
+ updateRender: function (active, parent) {
+ var parentData = parent.data;
+ this.inited = this.inited || active;
+ this.setData({
+ active: active,
+ shouldRender: this.inited || !parentData.lazyRender,
+ shouldShow: active || parentData.animated,
+ });
+ },
+ update: function () {
+ if (this.parent) {
+ this.parent.updateTabs();
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxml
new file mode 100644
index 0000000..f5e99f2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxss
new file mode 100644
index 0000000..1c90c88
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tab/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{box-sizing:border-box;flex-shrink:0;width:100%}.van-tab__pane{-webkit-overflow-scrolling:touch;box-sizing:border-box;overflow-y:auto}.van-tab__pane--active{height:auto}.van-tab__pane--inactive{height:0;overflow:visible}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.js
new file mode 100644
index 0000000..58a5065
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ props: {
+ info: null,
+ name: null,
+ icon: String,
+ dot: Boolean,
+ url: {
+ type: String,
+ value: '',
+ },
+ linkType: {
+ type: String,
+ value: 'redirectTo',
+ },
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ },
+ relation: (0, relation_1.useParent)('tabbar'),
+ data: {
+ active: false,
+ activeColor: '',
+ inactiveColor: '',
+ },
+ methods: {
+ onClick: function () {
+ var parent = this.parent;
+ if (parent) {
+ var index = parent.children.indexOf(this);
+ var active = this.data.name || index;
+ if (active !== this.data.active) {
+ parent.$emit('change', active);
+ }
+ }
+ var _a = this.data, url = _a.url, linkType = _a.linkType;
+ if (url && wx[linkType]) {
+ return wx[linkType]({ url: url });
+ }
+ this.$emit('click');
+ },
+ updateFromParent: function () {
+ var parent = this.parent;
+ if (!parent) {
+ return;
+ }
+ var index = parent.children.indexOf(this);
+ var parentData = parent.data;
+ var data = this.data;
+ var active = (data.name || index) === parentData.active;
+ var patch = {};
+ if (active !== data.active) {
+ patch.active = active;
+ }
+ if (parentData.activeColor !== data.activeColor) {
+ patch.activeColor = parentData.activeColor;
+ }
+ if (parentData.inactiveColor !== data.inactiveColor) {
+ patch.inactiveColor = parentData.inactiveColor;
+ }
+ if (Object.keys(patch).length > 0) {
+ this.setData(patch);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.json
new file mode 100644
index 0000000..16f174c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxml
new file mode 100644
index 0000000..524728f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxml
@@ -0,0 +1,28 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxss
new file mode 100644
index 0000000..21ee224
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-tabbar-item{align-items:center;color:var(--tabbar-item-text-color,#646566);display:flex;flex-direction:column;font-size:var(--tabbar-item-font-size,12px);height:100%;justify-content:center;line-height:var(--tabbar-item-line-height,1)}.van-tabbar-item__icon{font-size:var(--tabbar-item-icon-size,22px);margin-bottom:var(--tabbar-item-margin-bottom,4px);position:relative}.van-tabbar-item__icon__inner{display:block;min-width:1em}.van-tabbar-item--active{color:var(--tabbar-item-active-color,#1989fa)}.van-tabbar-item__info{margin-top:2px}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.js
new file mode 100644
index 0000000..3db793d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('tabbar-item', function () {
+ this.updateChildren();
+ }),
+ props: {
+ active: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ activeColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ inactiveColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ fixed: {
+ type: Boolean,
+ value: true,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 50,
+ },
+ methods: {
+ updateChildren: function () {
+ var children = this.children;
+ if (!Array.isArray(children) || !children.length) {
+ return;
+ }
+ children.forEach(function (child) { return child.updateFromParent(); });
+ },
+ setHeight: function () {
+ var _this = this;
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(function () {
+ (0, utils_1.getRect)(_this, '.van-tabbar').then(function (res) {
+ _this.setData({ height: res.height });
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxml
new file mode 100644
index 0000000..43bb111
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxss
new file mode 100644
index 0000000..42b6c1e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabbar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabbar{background-color:var(--tabbar-background-color,#fff);box-sizing:initial;display:flex;height:var(--tabbar-height,50px);width:100%}.van-tabbar--fixed{bottom:0;left:0;position:fixed}.van-tabbar--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.js
new file mode 100644
index 0000000..3121957
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.js
@@ -0,0 +1,327 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ mixins: [touch_1.touch],
+ classes: [
+ 'nav-class',
+ 'tab-class',
+ 'tab-active-class',
+ 'line-class',
+ 'wrap-class',
+ ],
+ relation: (0, relation_1.useChildren)('tab', function () {
+ this.updateTabs();
+ }),
+ props: {
+ sticky: Boolean,
+ border: Boolean,
+ swipeable: Boolean,
+ titleActiveColor: String,
+ titleInactiveColor: String,
+ color: String,
+ animated: {
+ type: Boolean,
+ observer: function () {
+ var _this = this;
+ this.children.forEach(function (child, index) {
+ return child.updateRender(index === _this.data.currentIndex, _this);
+ });
+ },
+ },
+ lineWidth: {
+ type: null,
+ value: 40,
+ observer: 'resize',
+ },
+ lineHeight: {
+ type: null,
+ value: -1,
+ },
+ active: {
+ type: null,
+ value: 0,
+ observer: function (name) {
+ if (name !== this.getCurrentName()) {
+ this.setCurrentIndexByName(name);
+ }
+ },
+ },
+ type: {
+ type: String,
+ value: 'line',
+ },
+ ellipsis: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: Number,
+ value: 0.3,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ swipeThreshold: {
+ type: Number,
+ value: 5,
+ observer: function (value) {
+ this.setData({
+ scrollable: this.children.length > value || !this.data.ellipsis,
+ });
+ },
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ },
+ lazyRender: {
+ type: Boolean,
+ value: true,
+ },
+ useBeforeChange: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ tabs: [],
+ scrollLeft: 0,
+ scrollable: false,
+ currentIndex: 0,
+ container: null,
+ skipTransition: true,
+ scrollWithAnimation: false,
+ lineOffsetLeft: 0,
+ inited: false,
+ },
+ mounted: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.swiping = true;
+ _this.setData({
+ container: function () { return _this.createSelectorQuery().select('.van-tabs'); },
+ });
+ _this.resize();
+ _this.scrollIntoView();
+ });
+ },
+ methods: {
+ updateTabs: function () {
+ var _a = this, _b = _a.children, children = _b === void 0 ? [] : _b, data = _a.data;
+ this.setData({
+ tabs: children.map(function (child) { return child.data; }),
+ scrollable: this.children.length > data.swipeThreshold || !data.ellipsis,
+ });
+ this.setCurrentIndexByName(data.active || this.getCurrentName());
+ },
+ trigger: function (eventName, child) {
+ var currentIndex = this.data.currentIndex;
+ var data = this.getChildData(currentIndex, child);
+ if (!(0, validator_1.isDef)(data)) {
+ return;
+ }
+ this.$emit(eventName, data);
+ },
+ onTap: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var child = this.children[index];
+ if (child.data.disabled) {
+ this.trigger('disabled', child);
+ return;
+ }
+ this.onBeforeChange(index).then(function () {
+ _this.setCurrentIndex(index);
+ (0, utils_1.nextTick)(function () {
+ _this.trigger('click');
+ });
+ });
+ },
+ // correct the index of active tab
+ setCurrentIndexByName: function (name) {
+ var _a = this.children, children = _a === void 0 ? [] : _a;
+ var matched = children.filter(function (child) { return child.getComputedName() === name; });
+ if (matched.length) {
+ this.setCurrentIndex(matched[0].index);
+ }
+ },
+ setCurrentIndex: function (currentIndex) {
+ var _this = this;
+ var _a = this, data = _a.data, _b = _a.children, children = _b === void 0 ? [] : _b;
+ if (!(0, validator_1.isDef)(currentIndex) ||
+ currentIndex >= children.length ||
+ currentIndex < 0) {
+ return;
+ }
+ (0, utils_1.groupSetData)(this, function () {
+ children.forEach(function (item, index) {
+ var active = index === currentIndex;
+ if (active !== item.data.active || !item.inited) {
+ item.updateRender(active, _this);
+ }
+ });
+ });
+ if (currentIndex === data.currentIndex) {
+ if (!data.inited) {
+ this.resize();
+ }
+ return;
+ }
+ var shouldEmitChange = data.currentIndex !== null;
+ this.setData({ currentIndex: currentIndex });
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.resize();
+ _this.scrollIntoView();
+ });
+ (0, utils_1.nextTick)(function () {
+ _this.trigger('input');
+ if (shouldEmitChange) {
+ _this.trigger('change');
+ }
+ });
+ },
+ getCurrentName: function () {
+ var activeTab = this.children[this.data.currentIndex];
+ if (activeTab) {
+ return activeTab.getComputedName();
+ }
+ },
+ resize: function () {
+ var _this = this;
+ if (this.data.type !== 'line') {
+ return;
+ }
+ var _a = this.data, currentIndex = _a.currentIndex, ellipsis = _a.ellipsis, skipTransition = _a.skipTransition;
+ Promise.all([
+ (0, utils_1.getAllRect)(this, '.van-tab'),
+ (0, utils_1.getRect)(this, '.van-tabs__line'),
+ ]).then(function (_a) {
+ var _b = _a[0], rects = _b === void 0 ? [] : _b, lineRect = _a[1];
+ var rect = rects[currentIndex];
+ if (rect == null) {
+ return;
+ }
+ var lineOffsetLeft = rects
+ .slice(0, currentIndex)
+ .reduce(function (prev, curr) { return prev + curr.width; }, 0);
+ lineOffsetLeft +=
+ (rect.width - lineRect.width) / 2 + (ellipsis ? 0 : 8);
+ _this.setData({ lineOffsetLeft: lineOffsetLeft, inited: true });
+ _this.swiping = true;
+ if (skipTransition) {
+ // waiting transition end
+ setTimeout(function () {
+ _this.setData({ skipTransition: false });
+ }, _this.data.duration);
+ }
+ });
+ },
+ // scroll active tab into view
+ scrollIntoView: function () {
+ var _this = this;
+ var _a = this.data, currentIndex = _a.currentIndex, scrollable = _a.scrollable, scrollWithAnimation = _a.scrollWithAnimation;
+ if (!scrollable) {
+ return;
+ }
+ Promise.all([
+ (0, utils_1.getAllRect)(this, '.van-tab'),
+ (0, utils_1.getRect)(this, '.van-tabs__nav'),
+ ]).then(function (_a) {
+ var tabRects = _a[0], navRect = _a[1];
+ var tabRect = tabRects[currentIndex];
+ var offsetLeft = tabRects
+ .slice(0, currentIndex)
+ .reduce(function (prev, curr) { return prev + curr.width; }, 0);
+ _this.setData({
+ scrollLeft: offsetLeft - (navRect.width - tabRect.width) / 2,
+ });
+ if (!scrollWithAnimation) {
+ (0, utils_1.nextTick)(function () {
+ _this.setData({ scrollWithAnimation: true });
+ });
+ }
+ });
+ },
+ onTouchScroll: function (event) {
+ this.$emit('scroll', event.detail);
+ },
+ onTouchStart: function (event) {
+ if (!this.data.swipeable)
+ return;
+ this.swiping = true;
+ this.touchStart(event);
+ },
+ onTouchMove: function (event) {
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ this.touchMove(event);
+ },
+ // watch swipe touch end
+ onTouchEnd: function () {
+ var _this = this;
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ var _a = this, direction = _a.direction, deltaX = _a.deltaX, offsetX = _a.offsetX;
+ var minSwipeDistance = 50;
+ if (direction === 'horizontal' && offsetX >= minSwipeDistance) {
+ var index_1 = this.getAvaiableTab(deltaX);
+ if (index_1 !== -1) {
+ this.onBeforeChange(index_1).then(function () { return _this.setCurrentIndex(index_1); });
+ }
+ }
+ this.swiping = false;
+ },
+ getAvaiableTab: function (direction) {
+ var _a = this.data, tabs = _a.tabs, currentIndex = _a.currentIndex;
+ var step = direction > 0 ? -1 : 1;
+ for (var i = step; currentIndex + i < tabs.length && currentIndex + i >= 0; i += step) {
+ var index = currentIndex + i;
+ if (index >= 0 &&
+ index < tabs.length &&
+ tabs[index] &&
+ !tabs[index].disabled) {
+ return index;
+ }
+ }
+ return -1;
+ },
+ onBeforeChange: function (index) {
+ var _this = this;
+ var useBeforeChange = this.data.useBeforeChange;
+ if (!useBeforeChange) {
+ return Promise.resolve();
+ }
+ return new Promise(function (resolve, reject) {
+ _this.$emit('before-change', __assign(__assign({}, _this.getChildData(index)), { callback: function (status) { return (status ? resolve() : reject()); } }));
+ });
+ },
+ getChildData: function (index, child) {
+ var currentChild = child || this.children[index];
+ if (!(0, validator_1.isDef)(currentChild)) {
+ return;
+ }
+ return {
+ index: currentChild.index,
+ name: currentChild.getComputedName(),
+ title: currentChild.data.title,
+ };
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.json
new file mode 100644
index 0000000..19c0bc3
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index",
+ "van-sticky": "../sticky/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxml
new file mode 100644
index 0000000..05bb1e1
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxml
@@ -0,0 +1,63 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.title }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxs
new file mode 100644
index 0000000..4059c7f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxs
@@ -0,0 +1,83 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function tabClass(active, ellipsis) {
+ var classes = ['tab-class'];
+
+ if (active) {
+ classes.push('tab-active-class');
+ }
+
+ if (ellipsis) {
+ classes.push('van-ellipsis');
+ }
+
+ return classes.join(' ');
+}
+
+function tabStyle(data) {
+ var titleColor = data.active
+ ? data.titleActiveColor
+ : data.titleInactiveColor;
+
+ var ellipsis = data.scrollable && data.ellipsis;
+
+ // card theme color
+ if (data.type === 'card') {
+ return style({
+ 'border-color': data.color,
+ 'background-color': !data.disabled && data.active ? data.color : null,
+ color: titleColor || (!data.disabled && !data.active ? data.color : null),
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+ }
+
+ return style({
+ color: titleColor,
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+}
+
+function navStyle(color, type) {
+ return style({
+ 'border-color': type === 'card' && color ? color : null,
+ });
+}
+
+function trackStyle(data) {
+ if (!data.animated) {
+ return '';
+ }
+
+ return style({
+ left: -100 * data.currentIndex + '%',
+ 'transition-duration': data.duration + 's',
+ '-webkit-transition-duration': data.duration + 's',
+ });
+}
+
+function lineStyle(data) {
+ return style({
+ width: utils.addUnit(data.lineWidth),
+ opacity: data.inited ? 1 : 0,
+ transform: 'translateX(' + data.lineOffsetLeft + 'px)',
+ '-webkit-transform': 'translateX(' + data.lineOffsetLeft + 'px)',
+ 'background-color': data.color,
+ height: data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'border-radius':
+ data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'transition-duration': !data.skipTransition ? data.duration + 's' : null,
+ '-webkit-transition-duration': !data.skipTransition
+ ? data.duration + 's'
+ : null,
+ });
+}
+
+module.exports = {
+ tabClass: tabClass,
+ tabStyle: tabStyle,
+ trackStyle: trackStyle,
+ lineStyle: lineStyle,
+ navStyle: navStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxss
new file mode 100644
index 0000000..09a93af
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tabs/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabs{-webkit-tap-highlight-color:transparent;position:relative}.van-tabs__wrap{display:flex;overflow:hidden}.van-tabs__wrap--scrollable .van-tab{flex:0 0 22%}.van-tabs__wrap--scrollable .van-tab--complete{flex:1 0 auto!important;padding:0 12px}.van-tabs__wrap--scrollable .van-tabs__nav--complete{padding-left:8px;padding-right:8px}.van-tabs__scroll{background-color:var(--tabs-nav-background-color,#fff);overflow:auto}.van-tabs__scroll--line{box-sizing:initial;height:calc(100% + 15px)}.van-tabs__scroll--card{border:1px solid var(--tabs-default-color,#ee0a24);border-radius:2px;box-sizing:border-box;margin:0 var(--padding-md,16px);width:calc(100% - var(--padding-md, 16px)*2)}.van-tabs__scroll::-webkit-scrollbar{display:none}.van-tabs__nav{display:flex;position:relative;-webkit-user-select:none;user-select:none}.van-tabs__nav--card{box-sizing:border-box;height:var(--tabs-card-height,30px)}.van-tabs__nav--card .van-tab{border-right:1px solid var(--tabs-default-color,#ee0a24);color:var(--tabs-default-color,#ee0a24);line-height:calc(var(--tabs-card-height, 30px) - 2px)}.van-tabs__nav--card .van-tab:last-child{border-right:none}.van-tabs__nav--card .van-tab.van-tab--active{background-color:var(--tabs-default-color,#ee0a24);color:#fff}.van-tabs__nav--card .van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tabs__line{background-color:var(--tabs-bottom-bar-color,#ee0a24);border-radius:var(--tabs-bottom-bar-height,3px);bottom:0;height:var(--tabs-bottom-bar-height,3px);left:0;opacity:0;position:absolute;z-index:1}.van-tabs__track{height:100%;position:relative;width:100%}.van-tabs__track--animated{display:flex;transition-property:left}.van-tabs__content{overflow:hidden}.van-tabs--line{height:var(--tabs-line-height,44px)}.van-tabs--card{height:var(--tabs-card-height,30px)}.van-tab{box-sizing:border-box;color:var(--tab-text-color,#646566);cursor:pointer;flex:1;font-size:var(--tab-font-size,14px);line-height:var(--tabs-line-height,44px);min-width:0;padding:0 5px;position:relative;text-align:center}.van-tab--active{color:var(--tab-active-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tab__title__info{position:relative!important;top:-1px!important;transform:translateX(0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.js
new file mode 100644
index 0000000..ec4069a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.js
@@ -0,0 +1,23 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ size: String,
+ mark: Boolean,
+ color: String,
+ plain: Boolean,
+ round: Boolean,
+ textColor: String,
+ type: {
+ type: String,
+ value: 'default',
+ },
+ closeable: Boolean,
+ },
+ methods: {
+ onClose: function () {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxml
new file mode 100644
index 0000000..59352dd
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxml
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxs
new file mode 100644
index 0000000..12d1668
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'background-color': data.plain ? '' : data.color,
+ color: data.textColor || data.plain ? data.textColor || data.color : '',
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxss
new file mode 100644
index 0000000..0f0cbae
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tag/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tag{align-items:center;border-radius:var(--tag-border-radius,2px);color:var(--tag-text-color,#fff);display:inline-flex;font-size:var(--tag-font-size,12px);line-height:var(--tag-line-height,16px);padding:var(--tag-padding,0 4px);position:relative}.van-tag--default{background-color:var(--tag-default-color,#969799)}.van-tag--default.van-tag--plain{color:var(--tag-default-color,#969799)}.van-tag--danger{background-color:var(--tag-danger-color,#ee0a24)}.van-tag--danger.van-tag--plain{color:var(--tag-danger-color,#ee0a24)}.van-tag--primary{background-color:var(--tag-primary-color,#1989fa)}.van-tag--primary.van-tag--plain{color:var(--tag-primary-color,#1989fa)}.van-tag--success{background-color:var(--tag-success-color,#07c160)}.van-tag--success.van-tag--plain{color:var(--tag-success-color,#07c160)}.van-tag--warning{background-color:var(--tag-warning-color,#ff976a)}.van-tag--warning.van-tag--plain{color:var(--tag-warning-color,#ff976a)}.van-tag--plain{background-color:var(--tag-plain-background-color,#fff)}.van-tag--plain:before{border:1px solid;border-radius:inherit;bottom:0;content:"";left:0;pointer-events:none;position:absolute;right:0;top:0}.van-tag--medium{padding:var(--tag-medium-padding,2px 6px)}.van-tag--large{border-radius:var(--tag-large-border-radius,4px);font-size:var(--tag-large-font-size,14px);padding:var(--tag-large-padding,4px 8px)}.van-tag--mark{border-radius:0 var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) 0}.van-tag--mark:after{content:"";display:block;width:2px}.van-tag--round{border-radius:var(--tag-round-border-radius,999px)}.van-tag__close{margin-left:2px;min-width:1em}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.js
new file mode 100644
index 0000000..0c01366
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.js
@@ -0,0 +1,31 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ show: Boolean,
+ mask: Boolean,
+ message: String,
+ forbidClick: Boolean,
+ zIndex: {
+ type: Number,
+ value: 1000,
+ },
+ type: {
+ type: String,
+ value: 'text',
+ },
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ position: {
+ type: String,
+ value: 'middle',
+ },
+ },
+ methods: {
+ // for prevent touchmove
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.json
new file mode 100644
index 0000000..9b1b78c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index",
+ "van-overlay": "../overlay/index",
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxml
new file mode 100644
index 0000000..69f143e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxml
@@ -0,0 +1,36 @@
+
+
+
+
+ {{ message }}
+
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxss
new file mode 100644
index 0000000..3b7a34e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-toast{word-wrap:break-word;align-items:center;background-color:var(--toast-background-color,rgba(0,0,0,.7));border-radius:var(--toast-border-radius,8px);box-sizing:initial;color:var(--toast-text-color,#fff);display:flex;flex-direction:column;font-size:var(--toast-font-size,14px);justify-content:center;line-height:var(--toast-line-height,20px);white-space:pre-wrap}.van-toast__container{left:50%;max-width:var(--toast-max-width,70%);position:fixed;top:50%;transform:translate(-50%,-50%);width:-webkit-fit-content;width:fit-content}.van-toast--text{min-width:var(--toast-text-min-width,96px);padding:var(--toast-text-padding,8px 12px)}.van-toast--icon{min-height:var(--toast-default-min-height,88px);padding:var(--toast-default-padding,16px);width:var(--toast-default-width,88px)}.van-toast--icon .van-toast__icon{font-size:var(--toast-icon-size,36px)}.van-toast--icon .van-toast__text{padding-top:8px}.van-toast__loading{margin:10px 0}.van-toast--top{transform:translateY(-30vh)}.van-toast--bottom{transform:translateY(30vh)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.d.ts
new file mode 100644
index 0000000..de2a4a2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.d.ts
@@ -0,0 +1,28 @@
+///
+///
+type ToastMessage = string | number;
+type ToastContext = WechatMiniprogram.Component.TrivialInstance | WechatMiniprogram.Page.TrivialInstance;
+interface ToastOptions {
+ show?: boolean;
+ type?: string;
+ mask?: boolean;
+ zIndex?: number;
+ context?: (() => ToastContext) | ToastContext;
+ position?: string;
+ duration?: number;
+ selector?: string;
+ forbidClick?: boolean;
+ loadingType?: string;
+ message?: ToastMessage;
+ onClose?: () => void;
+}
+declare function Toast(toastOptions: ToastOptions | ToastMessage): WechatMiniprogram.Component.TrivialInstance | undefined;
+declare namespace Toast {
+ var loading: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var success: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var fail: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var clear: () => void;
+ var setDefaultOptions: (options: ToastOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Toast;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.js
new file mode 100644
index 0000000..f51a89a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/toast/toast.js
@@ -0,0 +1,83 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var validator_1 = require("../common/validator");
+var defaultOptions = {
+ type: 'text',
+ mask: false,
+ message: '',
+ show: true,
+ zIndex: 1000,
+ duration: 2000,
+ position: 'middle',
+ forbidClick: false,
+ loadingType: 'circular',
+ selector: '#van-toast',
+};
+var queue = [];
+var currentOptions = __assign({}, defaultOptions);
+function parseOptions(message) {
+ return (0, validator_1.isObj)(message) ? message : { message: message };
+}
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+function Toast(toastOptions) {
+ var options = __assign(__assign({}, currentOptions), parseOptions(toastOptions));
+ var context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ var toast = context.selectComponent(options.selector);
+ if (!toast) {
+ console.warn('未找到 van-toast 节点,请确认 selector 及 context 是否正确');
+ return;
+ }
+ delete options.context;
+ delete options.selector;
+ toast.clear = function () {
+ toast.setData({ show: false });
+ if (options.onClose) {
+ options.onClose();
+ }
+ };
+ queue.push(toast);
+ toast.setData(options);
+ clearTimeout(toast.timer);
+ if (options.duration != null && options.duration > 0) {
+ toast.timer = setTimeout(function () {
+ toast.clear();
+ queue = queue.filter(function (item) { return item !== toast; });
+ }, options.duration);
+ }
+ return toast;
+}
+var createMethod = function (type) { return function (options) {
+ return Toast(__assign({ type: type }, parseOptions(options)));
+}; };
+Toast.loading = createMethod('loading');
+Toast.success = createMethod('success');
+Toast.fail = createMethod('fail');
+Toast.clear = function () {
+ queue.forEach(function (toast) {
+ toast.clear();
+ });
+ queue = [];
+};
+Toast.setDefaultOptions = function (options) {
+ Object.assign(currentOptions, options);
+};
+Toast.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+};
+exports.default = Toast;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.js
new file mode 100644
index 0000000..55fc8b8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.js
@@ -0,0 +1,15 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var transition_1 = require("../mixins/transition");
+(0, component_1.VantComponent)({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ ],
+ mixins: [(0, transition_1.transition)(true)],
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxml
new file mode 100644
index 0000000..2743785
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxs
new file mode 100644
index 0000000..e0babf6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxss
new file mode 100644
index 0000000..3a3d37f
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/transition/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-transition{transition-timing-function:ease}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-fade-down-enter-active,.van-fade-down-leave-active,.van-fade-left-enter-active,.van-fade-left-leave-active,.van-fade-right-enter-active,.van-fade-right-leave-active,.van-fade-up-enter-active,.van-fade-up-leave-active{transition-property:opacity,transform}.van-fade-up-enter,.van-fade-up-leave-to{opacity:0;transform:translate3d(0,100%,0)}.van-fade-down-enter,.van-fade-down-leave-to{opacity:0;transform:translate3d(0,-100%,0)}.van-fade-left-enter,.van-fade-left-leave-to{opacity:0;transform:translate3d(-100%,0,0)}.van-fade-right-enter,.van-fade-right-leave-to{opacity:0;transform:translate3d(100%,0,0)}.van-slide-down-enter-active,.van-slide-down-leave-active,.van-slide-left-enter-active,.van-slide-left-leave-active,.van-slide-right-enter-active,.van-slide-right-leave-active,.van-slide-up-enter-active,.van-slide-up-leave-active{transition-property:transform}.van-slide-up-enter,.van-slide-up-leave-to{transform:translate3d(0,100%,0)}.van-slide-down-enter,.van-slide-down-leave-to{transform:translate3d(0,-100%,0)}.van-slide-left-enter,.van-slide-left-leave-to{transform:translate3d(-100%,0,0)}.van-slide-right-enter,.van-slide-right-leave-to{transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.js
new file mode 100644
index 0000000..b6f69b2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'main-item-class',
+ 'content-item-class',
+ 'main-active-class',
+ 'content-active-class',
+ 'main-disabled-class',
+ 'content-disabled-class',
+ ],
+ props: {
+ items: {
+ type: Array,
+ observer: 'updateSubItems',
+ },
+ activeId: null,
+ mainActiveIndex: {
+ type: Number,
+ value: 0,
+ observer: 'updateSubItems',
+ },
+ height: {
+ type: null,
+ value: 300,
+ },
+ max: {
+ type: Number,
+ value: Infinity,
+ },
+ selectedIcon: {
+ type: String,
+ value: 'success',
+ },
+ },
+ data: {
+ subItems: [],
+ },
+ methods: {
+ // 当一个子项被选择时
+ onSelectItem: function (event) {
+ var item = event.currentTarget.dataset.item;
+ var isArray = Array.isArray(this.data.activeId);
+ // 判断有没有超出右侧选择的最大数
+ var isOverMax = isArray && this.data.activeId.length >= this.data.max;
+ // 判断该项有没有被选中, 如果有被选中,则忽视是否超出的条件
+ var isSelected = isArray
+ ? this.data.activeId.indexOf(item.id) > -1
+ : this.data.activeId === item.id;
+ if (!item.disabled && (!isOverMax || isSelected)) {
+ this.$emit('click-item', item);
+ }
+ },
+ // 当一个导航被点击时
+ onClickNav: function (event) {
+ var index = event.detail;
+ var item = this.data.items[index];
+ if (!item.disabled) {
+ this.$emit('click-nav', { index: index });
+ }
+ },
+ // 更新子项列表
+ updateSubItems: function () {
+ var _a = this.data, items = _a.items, mainActiveIndex = _a.mainActiveIndex;
+ var _b = (items[mainActiveIndex] || {}).children, children = _b === void 0 ? [] : _b;
+ this.setData({ subItems: children });
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.json
new file mode 100644
index 0000000..42991a2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-sidebar": "../sidebar/index",
+ "van-sidebar-item": "../sidebar-item/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxml
new file mode 100644
index 0000000..2663e52
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxs
new file mode 100644
index 0000000..b1cbb39
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var array = require('../wxs/array.wxs');
+
+function isActive (activeList, itemId) {
+ if (array.isArray(activeList)) {
+ return activeList.indexOf(itemId) > -1;
+ }
+
+ return activeList === itemId;
+}
+
+module.exports.isActive = isActive;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxss
new file mode 100644
index 0000000..5bef0ac
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/tree-select/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tree-select{display:flex;font-size:var(--tree-select-font-size,14px);position:relative;-webkit-user-select:none;user-select:none}.van-tree-select__nav{--sidebar-padding:12px 8px 12px 12px;background-color:var(--tree-select-nav-background-color,#f7f8fa);flex:1}.van-tree-select__nav__inner{height:100%;width:100%!important}.van-tree-select__content{background-color:var(--tree-select-content-background-color,#fff);flex:2}.van-tree-select__item{font-weight:700;line-height:var(--tree-select-item-height,44px);padding:0 32px 0 var(--padding-md,16px);position:relative}.van-tree-select__item--active{color:var(--tree-select-item-active-color,#ee0a24)}.van-tree-select__item--disabled{color:var(--tree-select-item-disabled-color,#c8c9cc)}.van-tree-select__selected{position:absolute;right:var(--padding-md,16px);top:50%;transform:translateY(-50%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.js
new file mode 100644
index 0000000..5492d40
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.js
@@ -0,0 +1,183 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+var shared_1 = require("./shared");
+var validator_1 = require("../common/validator");
+(0, component_1.VantComponent)({
+ props: __assign(__assign(__assign(__assign({ disabled: Boolean, multiple: Boolean, uploadText: String, useBeforeRead: Boolean, afterRead: null, beforeRead: null, previewSize: {
+ type: null,
+ value: 80,
+ }, name: {
+ type: null,
+ value: '',
+ }, accept: {
+ type: String,
+ value: 'image',
+ }, fileList: {
+ type: Array,
+ value: [],
+ observer: 'formatFileList',
+ }, maxSize: {
+ type: Number,
+ value: Number.MAX_VALUE,
+ }, maxCount: {
+ type: Number,
+ value: 100,
+ }, deletable: {
+ type: Boolean,
+ value: true,
+ }, showUpload: {
+ type: Boolean,
+ value: true,
+ }, previewImage: {
+ type: Boolean,
+ value: true,
+ }, previewFullImage: {
+ type: Boolean,
+ value: true,
+ }, videoFit: {
+ type: String,
+ value: 'contain',
+ }, imageFit: {
+ type: String,
+ value: 'scaleToFill',
+ }, uploadIcon: {
+ type: String,
+ value: 'photograph',
+ } }, shared_1.imageProps), shared_1.videoProps), shared_1.mediaProps), shared_1.messageFileProps),
+ data: {
+ lists: [],
+ isInCount: true,
+ },
+ methods: {
+ formatFileList: function () {
+ var _a = this.data, _b = _a.fileList, fileList = _b === void 0 ? [] : _b, maxCount = _a.maxCount;
+ var lists = fileList.map(function (item) { return (__assign(__assign({}, item), { isImage: (0, utils_1.isImageFile)(item), isVideo: (0, utils_1.isVideoFile)(item), deletable: (0, validator_1.isBoolean)(item.deletable) ? item.deletable : true })); });
+ this.setData({ lists: lists, isInCount: lists.length < maxCount });
+ },
+ getDetail: function (index) {
+ return {
+ name: this.data.name,
+ index: index == null ? this.data.fileList.length : index,
+ };
+ },
+ startUpload: function () {
+ var _this = this;
+ var _a = this.data, maxCount = _a.maxCount, multiple = _a.multiple, lists = _a.lists, disabled = _a.disabled;
+ if (disabled)
+ return;
+ (0, utils_1.chooseFile)(__assign(__assign({}, this.data), { maxCount: maxCount - lists.length }))
+ .then(function (res) {
+ _this.onBeforeRead(multiple ? res : res[0]);
+ })
+ .catch(function (error) {
+ _this.$emit('error', error);
+ });
+ },
+ onBeforeRead: function (file) {
+ var _this = this;
+ var _a = this.data, beforeRead = _a.beforeRead, useBeforeRead = _a.useBeforeRead;
+ var res = true;
+ if (typeof beforeRead === 'function') {
+ res = beforeRead(file, this.getDetail());
+ }
+ if (useBeforeRead) {
+ res = new Promise(function (resolve, reject) {
+ _this.$emit('before-read', __assign(__assign({ file: file }, _this.getDetail()), { callback: function (ok) {
+ ok ? resolve() : reject();
+ } }));
+ });
+ }
+ if (!res) {
+ return;
+ }
+ if ((0, validator_1.isPromise)(res)) {
+ res.then(function (data) { return _this.onAfterRead(data || file); });
+ }
+ else {
+ this.onAfterRead(file);
+ }
+ },
+ onAfterRead: function (file) {
+ var _a = this.data, maxSize = _a.maxSize, afterRead = _a.afterRead;
+ var oversize = Array.isArray(file)
+ ? file.some(function (item) { return item.size > maxSize; })
+ : file.size > maxSize;
+ if (oversize) {
+ this.$emit('oversize', __assign({ file: file }, this.getDetail()));
+ return;
+ }
+ if (typeof afterRead === 'function') {
+ afterRead(file, this.getDetail());
+ }
+ this.$emit('after-read', __assign({ file: file }, this.getDetail()));
+ },
+ deleteItem: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.$emit('delete', __assign(__assign({}, this.getDetail(index)), { file: this.data.fileList[index] }));
+ },
+ onPreviewImage: function (event) {
+ if (!this.data.previewFullImage)
+ return;
+ var index = event.currentTarget.dataset.index;
+ var _a = this.data, lists = _a.lists, showmenu = _a.showmenu;
+ var item = lists[index];
+ wx.previewImage({
+ urls: lists.filter(function (item) { return (0, utils_1.isImageFile)(item); }).map(function (item) { return item.url; }),
+ current: item.url,
+ showmenu: showmenu,
+ fail: function () {
+ wx.showToast({ title: '预览图片失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewVideo: function (event) {
+ if (!this.data.previewFullImage)
+ return;
+ var index = event.currentTarget.dataset.index;
+ var lists = this.data.lists;
+ var sources = [];
+ var current = lists.reduce(function (sum, cur, curIndex) {
+ if (!(0, utils_1.isVideoFile)(cur)) {
+ return sum;
+ }
+ sources.push(__assign(__assign({}, cur), { type: 'video' }));
+ if (curIndex < index) {
+ sum++;
+ }
+ return sum;
+ }, 0);
+ wx.previewMedia({
+ sources: sources,
+ current: current,
+ fail: function () {
+ wx.showToast({ title: '预览视频失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewFile: function (event) {
+ var index = event.currentTarget.dataset.index;
+ wx.openDocument({
+ filePath: this.data.lists[index].url,
+ showMenu: true,
+ });
+ },
+ onClickPreview: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var item = this.data.lists[index];
+ this.$emit('click-preview', __assign(__assign({}, item), this.getDetail(index)));
+ },
+ },
+});
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.json b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxml b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxml
new file mode 100644
index 0000000..3e61fd9
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxml
@@ -0,0 +1,84 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.name || item.url }}
+
+
+
+
+ {{ item.message }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ uploadText }}
+
+
+
+
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxs
new file mode 100644
index 0000000..c567ec2
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function sizeStyle(data) {
+ return "Array" === data.previewSize.constructor ? style({
+ width: addUnit(data.previewSize[0]),
+ height: addUnit(data.previewSize[1]),
+ }) : style({
+ width: addUnit(data.previewSize),
+ height: addUnit(data.previewSize),
+ });
+}
+
+module.exports = {
+ sizeStyle: sizeStyle,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxss b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxss
new file mode 100644
index 0000000..11f8696
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-uploader{display:inline-block;position:relative}.van-uploader__wrapper{display:flex;flex-wrap:wrap}.van-uploader__slot:empty{display:none}.van-uploader__slot:not(:empty)+.van-uploader__upload{display:none!important}.van-uploader__upload{align-items:center;background-color:var(--uploader-upload-background-color,#f7f8fa);box-sizing:border-box;display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;margin:0 8px 8px 0;position:relative;width:var(--uploader-size,80px)}.van-uploader__upload:active{background-color:var(--uploader-upload-active-color,#f2f3f5)}.van-uploader__upload-icon{color:var(--uploader-icon-color,#dcdee0);font-size:var(--uploader-icon-size,24px)}.van-uploader__upload-text{color:var(--uploader-text-color,#969799);font-size:var(--uploader-text-font-size,12px);margin-top:var(--padding-xs,8px)}.van-uploader__upload--disabled{opacity:var(--uploader-disabled-opacity,.5)}.van-uploader__preview{cursor:pointer;margin:0 8px 8px 0;position:relative}.van-uploader__preview-image{display:block;height:var(--uploader-size,80px);overflow:hidden;width:var(--uploader-size,80px)}.van-uploader__preview-delete,.van-uploader__preview-delete:after{height:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;width:var(--uploader-delete-icon-size,14px)}.van-uploader__preview-delete:after{background-color:var(--uploader-delete-background-color,rgba(0,0,0,.7));border-radius:0 0 0 12px;content:""}.van-uploader__preview-delete-icon{color:var(--uploader-delete-color,#fff);font-size:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;transform:scale(.7) translate(10%,-10%);z-index:1}.van-uploader__file{align-items:center;background-color:var(--uploader-file-background-color,#f7f8fa);display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;width:var(--uploader-size,80px)}.van-uploader__file-icon{color:var(--uploader-file-icon-color,#646566);font-size:var(--uploader-file-icon-size,20px)}.van-uploader__file-name{box-sizing:border-box;color:var(--uploader-file-name-text-color,#646566);font-size:var(--uploader-file-name-font-size,12px);margin-top:var(--uploader-file-name-margin-top,8px);padding:var(--uploader-file-name-padding,0 4px);text-align:center;width:100%}.van-uploader__mask{align-items:center;background-color:var(--uploader-mask-background-color,rgba(50,50,51,.88));bottom:0;color:#fff;display:flex;flex-direction:column;justify-content:center;left:0;position:absolute;right:0;top:0}.van-uploader__mask-icon{font-size:var(--uploader-mask-icon-size,22px)}.van-uploader__mask-message{font-size:var(--uploader-mask-message-font-size,12px);line-height:var(--uploader-mask-message-line-height,14px);margin-top:6px;padding:0 var(--padding-base,4px)}.van-uploader__loading{color:var(--uploader-loading-icon-color,#fff)!important;height:var(--uploader-loading-icon-size,22px);width:var(--uploader-loading-icon-size,22px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.d.ts
new file mode 100644
index 0000000..e0a0d7e
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.d.ts
@@ -0,0 +1,53 @@
+export declare const imageProps: {
+ sizeType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ showmenu: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+};
+export declare const videoProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ compressed: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const mediaProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ mediaType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const messageFileProps: {
+ extension: null;
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.js
new file mode 100644
index 0000000..b88fea7
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/shared.js
@@ -0,0 +1,60 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.messageFileProps = exports.mediaProps = exports.videoProps = exports.imageProps = void 0;
+// props for image
+exports.imageProps = {
+ sizeType: {
+ type: Array,
+ value: ['original', 'compressed'],
+ },
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ showmenu: {
+ type: Boolean,
+ value: true,
+ },
+};
+// props for video
+exports.videoProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ compressed: {
+ type: Boolean,
+ value: true,
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for media
+exports.mediaProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ mediaType: {
+ type: Array,
+ value: ['image', 'video', 'mix'],
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for file
+exports.messageFileProps = {
+ extension: null,
+};
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.d.ts b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.d.ts
new file mode 100644
index 0000000..1e76ee6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.d.ts
@@ -0,0 +1,24 @@
+export interface File {
+ url: string;
+ size?: number;
+ name?: string;
+ type: string;
+ duration?: number;
+ time?: number;
+ isImage?: boolean;
+ isVideo?: boolean;
+}
+export declare function isImageFile(item: File): boolean;
+export declare function isVideoFile(item: File): boolean;
+export declare function chooseFile({ accept, multiple, capture, compressed, maxDuration, sizeType, camera, maxCount, mediaType, extension, }: {
+ accept: any;
+ multiple: any;
+ capture: any;
+ compressed: any;
+ maxDuration: any;
+ sizeType: any;
+ camera: any;
+ maxCount: any;
+ mediaType: any;
+ extension: any;
+}): Promise;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.js b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.js
new file mode 100644
index 0000000..a5d49c6
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/uploader/utils.js
@@ -0,0 +1,112 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.chooseFile = exports.isVideoFile = exports.isImageFile = void 0;
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+function isImageFile(item) {
+ if (item.isImage != null) {
+ return item.isImage;
+ }
+ if (item.type) {
+ return item.type === 'image';
+ }
+ if (item.url) {
+ return (0, validator_1.isImageUrl)(item.url);
+ }
+ return false;
+}
+exports.isImageFile = isImageFile;
+function isVideoFile(item) {
+ if (item.isVideo != null) {
+ return item.isVideo;
+ }
+ if (item.type) {
+ return item.type === 'video';
+ }
+ if (item.url) {
+ return (0, validator_1.isVideoUrl)(item.url);
+ }
+ return false;
+}
+exports.isVideoFile = isVideoFile;
+function formatImage(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['path'])), { type: 'image', url: item.tempFilePath || item.path, thumb: item.tempFilePath || item.path })); });
+}
+function formatVideo(res) {
+ return [
+ __assign(__assign({}, (0, utils_1.pickExclude)(res, ['tempFilePath', 'thumbTempFilePath', 'errMsg'])), { type: 'video', url: res.tempFilePath, thumb: res.thumbTempFilePath }),
+ ];
+}
+function formatMedia(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['fileType', 'thumbTempFilePath', 'tempFilePath'])), { type: item.fileType, url: item.tempFilePath, thumb: item.fileType === 'video' ? item.thumbTempFilePath : item.tempFilePath })); });
+}
+function formatFile(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['path'])), { url: item.path })); });
+}
+function chooseFile(_a) {
+ var accept = _a.accept, multiple = _a.multiple, capture = _a.capture, compressed = _a.compressed, maxDuration = _a.maxDuration, sizeType = _a.sizeType, camera = _a.camera, maxCount = _a.maxCount, mediaType = _a.mediaType, extension = _a.extension;
+ return new Promise(function (resolve, reject) {
+ switch (accept) {
+ case 'image':
+ if (utils_1.isPC || utils_1.isWxWork) {
+ wx.chooseImage({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ sourceType: capture,
+ sizeType: sizeType,
+ success: function (res) { return resolve(formatImage(res)); },
+ fail: reject,
+ });
+ }
+ else {
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType: ['image'],
+ sourceType: capture,
+ maxDuration: maxDuration,
+ sizeType: sizeType,
+ camera: camera,
+ success: function (res) { return resolve(formatImage(res)); },
+ fail: reject,
+ });
+ }
+ break;
+ case 'media':
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType: mediaType,
+ sourceType: capture,
+ maxDuration: maxDuration,
+ sizeType: sizeType,
+ camera: camera,
+ success: function (res) { return resolve(formatMedia(res)); },
+ fail: reject,
+ });
+ break;
+ case 'video':
+ wx.chooseVideo({
+ sourceType: capture,
+ compressed: compressed,
+ maxDuration: maxDuration,
+ camera: camera,
+ success: function (res) { return resolve(formatVideo(res)); },
+ fail: reject,
+ });
+ break;
+ default:
+ wx.chooseMessageFile(__assign(__assign({ count: multiple ? maxCount : 1, type: accept }, (extension ? { extension: extension } : {})), { success: function (res) { return resolve(formatFile(res)); }, fail: reject }));
+ break;
+ }
+ });
+}
+exports.chooseFile = chooseFile;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/add-unit.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/add-unit.wxs
new file mode 100644
index 0000000..4f33462
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/add-unit.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var REGEXP = getRegExp('^-?\d+(\.\d+)?$');
+
+function addUnit(value) {
+ if (value == null) {
+ return undefined;
+ }
+
+ return REGEXP.test('' + value) ? value + 'px' : value;
+}
+
+module.exports = addUnit;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/array.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/array.wxs
new file mode 100644
index 0000000..610089c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/array.wxs
@@ -0,0 +1,5 @@
+function isArray(array) {
+ return array && array.constructor === 'Array';
+}
+
+module.exports.isArray = isArray;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/bem.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/bem.wxs
new file mode 100644
index 0000000..1efa129
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/bem.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var array = require('./array.wxs');
+var object = require('./object.wxs');
+var PREFIX = 'van-';
+
+function join(name, mods) {
+ name = PREFIX + name;
+ mods = mods.map(function(mod) {
+ return name + '--' + mod;
+ });
+ mods.unshift(name);
+ return mods.join(' ');
+}
+
+function traversing(mods, conf) {
+ if (!conf) {
+ return;
+ }
+
+ if (typeof conf === 'string' || typeof conf === 'number') {
+ mods.push(conf);
+ } else if (array.isArray(conf)) {
+ conf.forEach(function(item) {
+ traversing(mods, item);
+ });
+ } else if (typeof conf === 'object') {
+ object.keys(conf).forEach(function(key) {
+ conf[key] && mods.push(key);
+ });
+ }
+}
+
+function bem(name, conf) {
+ var mods = [];
+ traversing(mods, conf);
+ return join(name, mods);
+}
+
+module.exports = bem;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/memoize.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/memoize.wxs
new file mode 100644
index 0000000..8f7f46d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/memoize.wxs
@@ -0,0 +1,55 @@
+/**
+ * Simple memoize
+ * wxs doesn't support fn.apply, so this memoize only support up to 2 args
+ */
+/* eslint-disable */
+
+function isPrimitive(value) {
+ var type = typeof value;
+ return (
+ type === 'boolean' ||
+ type === 'number' ||
+ type === 'string' ||
+ type === 'undefined' ||
+ value === null
+ );
+}
+
+// mock simple fn.call in wxs
+function call(fn, args) {
+ if (args.length === 2) {
+ return fn(args[0], args[1]);
+ }
+
+ if (args.length === 1) {
+ return fn(args[0]);
+ }
+
+ return fn();
+}
+
+function serializer(args) {
+ if (args.length === 1 && isPrimitive(args[0])) {
+ return args[0];
+ }
+ var obj = {};
+ for (var i = 0; i < args.length; i++) {
+ obj['key' + i] = args[i];
+ }
+ return JSON.stringify(obj);
+}
+
+function memoize(fn) {
+ var cache = {};
+
+ return function() {
+ var key = serializer(arguments);
+ if (cache[key] === undefined) {
+ cache[key] = call(fn, arguments);
+ }
+
+ return cache[key];
+ };
+}
+
+module.exports = memoize;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/object.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/object.wxs
new file mode 100644
index 0000000..e077107
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/object.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var REGEXP = getRegExp('{|}|"', 'g');
+
+function keys(obj) {
+ return JSON.stringify(obj)
+ .replace(REGEXP, '')
+ .split(',')
+ .map(function(item) {
+ return item.split(':')[0];
+ });
+}
+
+module.exports.keys = keys;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/style.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/style.wxs
new file mode 100644
index 0000000..d88ca7c
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/style.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var object = require('./object.wxs');
+var array = require('./array.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+
+ return newWord;
+}
+
+function style(styles) {
+ if (array.isArray(styles)) {
+ return styles
+ .filter(function (item) {
+ return item != null && item !== '';
+ })
+ .map(function (item) {
+ return style(item);
+ })
+ .join(';');
+ }
+
+ if ('Object' === styles.constructor) {
+ return object
+ .keys(styles)
+ .filter(function (key) {
+ return styles[key] != null && styles[key] !== '';
+ })
+ .map(function (key) {
+ return [kebabCase(key), [styles[key]]].join(':');
+ })
+ .join(';');
+ }
+
+ return styles;
+}
+
+module.exports = style;
diff --git a/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/utils.wxs b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/utils.wxs
new file mode 100644
index 0000000..f66d33a
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/@vant/weapp/wxs/utils.wxs
@@ -0,0 +1,10 @@
+/* eslint-disable */
+var bem = require('./bem.wxs');
+var memoize = require('./memoize.wxs');
+var addUnit = require('./add-unit.wxs');
+
+module.exports = {
+ bem: memoize(bem),
+ memoize: memoize,
+ addUnit: addUnit
+};
diff --git a/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js
new file mode 100644
index 0000000..109e62d
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js
@@ -0,0 +1,292 @@
+module.exports =
+/******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+/******/
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+/******/
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId]) {
+/******/ return installedModules[moduleId].exports;
+/******/ }
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ i: moduleId,
+/******/ l: false,
+/******/ exports: {}
+/******/ };
+/******/
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+/******/
+/******/ // Flag the module as loaded
+/******/ module.l = true;
+/******/
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+/******/
+/******/
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+/******/
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+/******/
+/******/ // define getter function for harmony exports
+/******/ __webpack_require__.d = function(exports, name, getter) {
+/******/ if(!__webpack_require__.o(exports, name)) {
+/******/ Object.defineProperty(exports, name, { enumerable: true, get: getter });
+/******/ }
+/******/ };
+/******/
+/******/ // define __esModule on exports
+/******/ __webpack_require__.r = function(exports) {
+/******/ if(typeof Symbol !== 'undefined' && Symbol.toStringTag) {
+/******/ Object.defineProperty(exports, Symbol.toStringTag, { value: 'Module' });
+/******/ }
+/******/ Object.defineProperty(exports, '__esModule', { value: true });
+/******/ };
+/******/
+/******/ // create a fake namespace object
+/******/ // mode & 1: value is a module id, require it
+/******/ // mode & 2: merge all properties of value into the ns
+/******/ // mode & 4: return value when already ns object
+/******/ // mode & 8|1: behave like require
+/******/ __webpack_require__.t = function(value, mode) {
+/******/ if(mode & 1) value = __webpack_require__(value);
+/******/ if(mode & 8) return value;
+/******/ if((mode & 4) && typeof value === 'object' && value && value.__esModule) return value;
+/******/ var ns = Object.create(null);
+/******/ __webpack_require__.r(ns);
+/******/ Object.defineProperty(ns, 'default', { enumerable: true, value: value });
+/******/ if(mode & 2 && typeof value != 'string') for(var key in value) __webpack_require__.d(ns, key, function(key) { return value[key]; }.bind(null, key));
+/******/ return ns;
+/******/ };
+/******/
+/******/ // getDefaultExport function for compatibility with non-harmony modules
+/******/ __webpack_require__.n = function(module) {
+/******/ var getter = module && module.__esModule ?
+/******/ function getDefault() { return module['default']; } :
+/******/ function getModuleExports() { return module; };
+/******/ __webpack_require__.d(getter, 'a', getter);
+/******/ return getter;
+/******/ };
+/******/
+/******/ // Object.prototype.hasOwnProperty.call
+/******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };
+/******/
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+/******/
+/******/
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(__webpack_require__.s = 0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ (function(module, exports, __webpack_require__) {
+
+"use strict";
+
+
+exports.__esModule = true;
+exports.storeBindingsBehavior = undefined;
+
+var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
+
+exports.createStoreBindings = createStoreBindings;
+
+var _mobxMiniprogram = __webpack_require__(1);
+
+function _createActions(methods, options) {
+ var store = options.store,
+ actions = options.actions;
+
+
+ if (!actions) return;
+
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+
+ if (actions instanceof Array) {
+ // eslint-disable-next-line arrow-body-style
+ actions.forEach(function (field) {
+ methods[field] = function () {
+ return store[field].apply(store, arguments);
+ };
+ });
+ } else if ((typeof actions === 'undefined' ? 'undefined' : _typeof(actions)) === 'object') {
+ // for object-typed fields definition
+ Object.keys(actions).forEach(function (field) {
+ var def = actions[field];
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition');
+ }
+ methods[field] = function () {
+ return store[def].apply(store, arguments);
+ };
+ });
+ }
+}
+
+function _createDataFieldsReactions(target, options) {
+ var store = options.store,
+ fields = options.fields;
+
+ // setData combination
+
+ var pendingSetData = null;
+ function applySetData() {
+ if (pendingSetData === null) return;
+ var data = pendingSetData;
+ pendingSetData = null;
+ target.setData(data);
+ }
+ function scheduleSetData(field, value) {
+ if (!pendingSetData) {
+ pendingSetData = {};
+ wx.nextTick(applySetData);
+ }
+ pendingSetData[field] = value;
+ }
+
+ // handling fields
+ var reactions = [];
+ if (fields instanceof Array) {
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+ // eslint-disable-next-line arrow-body-style
+ reactions = fields.map(function (field) {
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return store[field];
+ }, function (value) {
+ scheduleSetData(field, value);
+ }, {
+ fireImmediately: true
+ });
+ });
+ } else if ((typeof fields === 'undefined' ? 'undefined' : _typeof(fields)) === 'object' && fields) {
+ // for object-typed fields definition
+ reactions = Object.keys(fields).map(function (field) {
+ var def = fields[field];
+ if (typeof def === 'function') {
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return def.call(target, store);
+ }, function (value) {
+ scheduleSetData(field, value);
+ }, {
+ fireImmediately: true
+ });
+ }
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition');
+ }
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return store[def];
+ }, function (value) {
+ scheduleSetData(String(field), value);
+ }, {
+ fireImmediately: true
+ });
+ });
+ }
+
+ var destroyStoreBindings = function destroyStoreBindings() {
+ reactions.forEach(function (reaction) {
+ return reaction();
+ });
+ };
+
+ return {
+ updateStoreBindings: applySetData,
+ destroyStoreBindings: destroyStoreBindings
+ };
+}
+
+function createStoreBindings(target, options) {
+ _createActions(target, options);
+ return _createDataFieldsReactions(target, options);
+}
+
+var storeBindingsBehavior = exports.storeBindingsBehavior = Behavior({
+ definitionFilter: function definitionFilter(defFields) {
+ if (!defFields.methods) {
+ defFields.methods = {};
+ }
+ var storeBindings = defFields.storeBindings;
+
+ defFields.methods._mobxMiniprogramBindings = function () {
+ return storeBindings;
+ };
+ if (storeBindings) {
+ if (Array.isArray(storeBindings)) {
+ storeBindings.forEach(function (binding) {
+ _createActions(defFields.methods, binding);
+ });
+ } else {
+ _createActions(defFields.methods, storeBindings);
+ }
+ }
+ },
+ attached: function attached() {
+ if (typeof this._mobxMiniprogramBindings !== 'function') return;
+ var storeBindings = this._mobxMiniprogramBindings();
+ if (!storeBindings) {
+ this._mobxMiniprogramBindings = null;
+ return;
+ }
+ if (Array.isArray(storeBindings)) {
+ var that = this;
+ this._mobxMiniprogramBindings = storeBindings.map(function (item) {
+ return _createDataFieldsReactions(that, item);
+ });
+ } else {
+ this._mobxMiniprogramBindings = _createDataFieldsReactions(this, storeBindings);
+ }
+ },
+ detached: function detached() {
+ if (this._mobxMiniprogramBindings) {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.destroyStoreBindings();
+ });
+ } else {
+ this._mobxMiniprogramBindings.destroyStoreBindings();
+ }
+ }
+ },
+
+ methods: {
+ updateStoreBindings: function updateStoreBindings() {
+ if (this._mobxMiniprogramBindings && typeof this._mobxMiniprogramBindings !== 'function') {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.updateStoreBindings();
+ });
+ } else {
+ this._mobxMiniprogramBindings.updateStoreBindings();
+ }
+ }
+ }
+ }
+});
+
+/***/ }),
+/* 1 */
+/***/ (function(module, exports) {
+
+module.exports = require("mobx-miniprogram");
+
+/***/ })
+/******/ ]);
+//# sourceMappingURL=index.js.map
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js.map b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js.map
new file mode 100644
index 0000000..eeb46a3
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram-bindings/index.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["webpack:///webpack/bootstrap","webpack:///./src/index.js","webpack:///external \"mobx-miniprogram\""],"names":["createStoreBindings","_createActions","methods","options","store","actions","Error","Array","forEach","field","Object","keys","def","_createDataFieldsReactions","target","fields","pendingSetData","applySetData","data","setData","scheduleSetData","value","wx","nextTick","reactions","map","fireImmediately","call","String","destroyStoreBindings","reaction","updateStoreBindings","storeBindingsBehavior","Behavior","definitionFilter","defFields","storeBindings","_mobxMiniprogramBindings","isArray","binding","attached","that","item","detached","bd"],"mappings":";;QAAA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;;QAEA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;;;QAGA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;QACA,0CAA0C,gCAAgC;QAC1E;QACA;;QAEA;QACA;QACA;QACA,wDAAwD,kBAAkB;QAC1E;QACA,iDAAiD,cAAc;QAC/D;;QAEA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA,yCAAyC,iCAAiC;QAC1E,gHAAgH,mBAAmB,EAAE;QACrI;QACA;;QAEA;QACA;QACA;QACA,2BAA2B,0BAA0B,EAAE;QACvD,iCAAiC,eAAe;QAChD;QACA;QACA;;QAEA;QACA,sDAAsD,+DAA+D;;QAErH;QACA;;;QAGA;QACA;;;;;;;;;;;;;;;QCoBgBA,mB,GAAAA,mB;;AAtGhB;;AAEA,SAASC,cAAT,CAAwBC,OAAxB,EAAiCC,OAAjC,EAA0C;AAAA,MACjCC,KADiC,GACfD,OADe,CACjCC,KADiC;AAAA,MAC1BC,OAD0B,GACfF,OADe,CAC1BE,OAD0B;;;AAGxC,MAAI,CAACA,OAAL,EAAc;;AAEd;AACA,MAAI,OAAOD,KAAP,KAAiB,WAArB,EAAkC;AAChC,UAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;;AAED,MAAID,mBAAmBE,KAAvB,EAA8B;AAC5B;AACAF,YAAQG,OAAR,CAAgB,UAACC,KAAD,EAAW;AACzBP,cAAQO,KAAR,IAAiB,YAAmB;AAClC,eAAOL,MAAMK,KAAN,yBAAP;AACD,OAFD;AAGD,KAJD;AAKD,GAPD,MAOO,IAAI,QAAOJ,OAAP,yCAAOA,OAAP,OAAmB,QAAvB,EAAiC;AACtC;AACAK,WAAOC,IAAP,CAAYN,OAAZ,EAAqBG,OAArB,CAA6B,UAACC,KAAD,EAAW;AACtC,UAAMG,MAAMP,QAAQI,KAAR,CAAZ;AACA,UAAI,OAAOA,KAAP,KAAiB,QAAjB,IAA6B,OAAOA,KAAP,KAAiB,QAAlD,EAA4D;AAC1D,cAAM,IAAIH,KAAJ,CAAU,kDAAV,CAAN;AACD;AACDJ,cAAQO,KAAR,IAAiB,YAAmB;AAClC,eAAOL,MAAMQ,GAAN,yBAAP;AACD,OAFD;AAGD,KARD;AASD;AACF;;AAED,SAASC,0BAAT,CAAoCC,MAApC,EAA4CX,OAA5C,EAAqD;AAAA,MAC5CC,KAD4C,GAC3BD,OAD2B,CAC5CC,KAD4C;AAAA,MACrCW,MADqC,GAC3BZ,OAD2B,CACrCY,MADqC;;AAGnD;;AACA,MAAIC,iBAAiB,IAArB;AACA,WAASC,YAAT,GAAwB;AACtB,QAAID,mBAAmB,IAAvB,EAA6B;AAC7B,QAAME,OAAOF,cAAb;AACAA,qBAAiB,IAAjB;AACAF,WAAOK,OAAP,CAAeD,IAAf;AACD;AACD,WAASE,eAAT,CAAyBX,KAAzB,EAAgCY,KAAhC,EAAuC;AACrC,QAAI,CAACL,cAAL,EAAqB;AACnBA,uBAAiB,EAAjB;AACAM,SAAGC,QAAH,CAAYN,YAAZ;AACD;AACDD,mBAAeP,KAAf,IAAwBY,KAAxB;AACD;;AAED;AACA,MAAIG,YAAY,EAAhB;AACA,MAAIT,kBAAkBR,KAAtB,EAA6B;AAC3B;AACA,QAAI,OAAOH,KAAP,KAAiB,WAArB,EAAkC;AAChC,YAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;AACD;AACAkB,gBAAYT,OAAOU,GAAP,CAAW,UAAChB,KAAD,EAAW;AAChC,aAAO,+BAAS;AAAA,eAAML,MAAMK,KAAN,CAAN;AAAA,OAAT,EAA6B,UAACY,KAAD,EAAW;AAC7CD,wBAAgBX,KAAhB,EAAuBY,KAAvB;AACD,OAFM,EAEJ;AACDK,yBAAiB;AADhB,OAFI,CAAP;AAKD,KANW,CAAZ;AAOD,GAbD,MAaO,IAAI,QAAOX,MAAP,yCAAOA,MAAP,OAAkB,QAAlB,IAA8BA,MAAlC,EAA0C;AAC/C;AACAS,gBAAYd,OAAOC,IAAP,CAAYI,MAAZ,EAAoBU,GAApB,CAAwB,UAAChB,KAAD,EAAW;AAC7C,UAAMG,MAAMG,OAAON,KAAP,CAAZ;AACA,UAAI,OAAOG,GAAP,KAAe,UAAnB,EAA+B;AAC7B,eAAO,+BAAS;AAAA,iBAAMA,IAAIe,IAAJ,CAASb,MAAT,EAAiBV,KAAjB,CAAN;AAAA,SAAT,EAAwC,UAACiB,KAAD,EAAW;AACxDD,0BAAgBX,KAAhB,EAAuBY,KAAvB;AACD,SAFM,EAEJ;AACDK,2BAAiB;AADhB,SAFI,CAAP;AAKD;AACD,UAAI,OAAOjB,KAAP,KAAiB,QAAjB,IAA6B,OAAOA,KAAP,KAAiB,QAAlD,EAA4D;AAC1D,cAAM,IAAIH,KAAJ,CAAU,kDAAV,CAAN;AACD;AACD,UAAI,OAAOF,KAAP,KAAiB,WAArB,EAAkC;AAChC,cAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;AACD,aAAO,+BAAS;AAAA,eAAMF,MAAMQ,GAAN,CAAN;AAAA,OAAT,EAA2B,UAACS,KAAD,EAAW;AAC3CD,wBAAgBQ,OAAOnB,KAAP,CAAhB,EAA+BY,KAA/B;AACD,OAFM,EAEJ;AACDK,yBAAiB;AADhB,OAFI,CAAP;AAKD,KApBW,CAAZ;AAqBD;;AAED,MAAMG,uBAAuB,SAAvBA,oBAAuB,GAAM;AACjCL,cAAUhB,OAAV,CAAkB,UAACsB,QAAD;AAAA,aAAcA,UAAd;AAAA,KAAlB;AACD,GAFD;;AAIA,SAAO;AACLC,yBAAqBd,YADhB;AAELY;AAFK,GAAP;AAID;;AAEM,SAAS7B,mBAAT,CAA6Bc,MAA7B,EAAqCX,OAArC,EAA8C;AACnDF,iBAAea,MAAf,EAAuBX,OAAvB;AACA,SAAOU,2BAA2BC,MAA3B,EAAmCX,OAAnC,CAAP;AACD;;AAEM,IAAM6B,wDAAwBC,SAAS;AAC5CC,oBAAkB,0BAACC,SAAD,EAAe;AAC/B,QAAI,CAACA,UAAUjC,OAAf,EAAwB;AACtBiC,gBAAUjC,OAAV,GAAoB,EAApB;AACD;AAH8B,QAIxBkC,aAJwB,GAIPD,SAJO,CAIxBC,aAJwB;;AAK/BD,cAAUjC,OAAV,CAAkBmC,wBAAlB,GAA6C,YAAY;AACvD,aAAOD,aAAP;AACD,KAFD;AAGA,QAAIA,aAAJ,EAAmB;AACjB,UAAI7B,MAAM+B,OAAN,CAAcF,aAAd,CAAJ,EAAkC;AAChCA,sBAAc5B,OAAd,CAAsB,UAAU+B,OAAV,EAAmB;AACvCtC,yBAAekC,UAAUjC,OAAzB,EAAkCqC,OAAlC;AACD,SAFD;AAGD,OAJD,MAIO;AACLtC,uBAAekC,UAAUjC,OAAzB,EAAkCkC,aAAlC;AACD;AACF;AACF,GAlB2C;AAmB5CI,UAnB4C,sBAmBjC;AACT,QAAI,OAAO,KAAKH,wBAAZ,KAAyC,UAA7C,EAAyD;AACzD,QAAMD,gBAAgB,KAAKC,wBAAL,EAAtB;AACA,QAAI,CAACD,aAAL,EAAoB;AAClB,WAAKC,wBAAL,GAAgC,IAAhC;AACA;AACD;AACD,QAAI9B,MAAM+B,OAAN,CAAcF,aAAd,CAAJ,EAAkC;AAChC,UAAMK,OAAO,IAAb;AACA,WAAKJ,wBAAL,GAAgCD,cAAcX,GAAd,CAAkB,UAAUiB,IAAV,EAAgB;AAChE,eAAO7B,2BAA2B4B,IAA3B,EAAiCC,IAAjC,CAAP;AACD,OAF+B,CAAhC;AAGD,KALD,MAKO;AACL,WAAKL,wBAAL,GAAgCxB,2BAA2B,IAA3B,EAAiCuB,aAAjC,CAAhC;AACD;AACF,GAlC2C;AAmC5CO,UAnC4C,sBAmCjC;AACT,QAAI,KAAKN,wBAAT,EAAmC;AACjC,UAAI9B,MAAM+B,OAAN,CAAc,KAAKD,wBAAnB,CAAJ,EAAkD;AAChD,aAAKA,wBAAL,CAA8B7B,OAA9B,CAAsC,UAAUoC,EAAV,EAAc;AAClDA,aAAGf,oBAAH;AACD,SAFD;AAGD,OAJD,MAIO;AACL,aAAKQ,wBAAL,CAA8BR,oBAA9B;AACD;AACF;AACF,GA7C2C;;AA8C5C3B,WAAS;AACP6B,uBADO,iCACe;AACpB,UAAI,KAAKM,wBAAL,IAAiC,OAAO,KAAKA,wBAAZ,KAAyC,UAA9E,EAA0F;AACxF,YAAI9B,MAAM+B,OAAN,CAAc,KAAKD,wBAAnB,CAAJ,EAAkD;AAChD,eAAKA,wBAAL,CAA8B7B,OAA9B,CAAsC,UAAUoC,EAAV,EAAc;AAClDA,eAAGb,mBAAH;AACD,WAFD;AAGD,SAJD,MAIO;AACL,eAAKM,wBAAL,CAA8BN,mBAA9B;AACD;AACF;AACF;AAXM;AA9CmC,CAAT,CAA9B,C;;;;;;AC3GP,6C","file":"index.js","sourceRoot":""}
\ No newline at end of file
diff --git a/src/miniprogram-4/miniprogram_npm/mobx-miniprogram/index.js b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram/index.js
new file mode 100644
index 0000000..b3b99d8
--- /dev/null
+++ b/src/miniprogram-4/miniprogram_npm/mobx-miniprogram/index.js
@@ -0,0 +1 @@
+"use strict";Object.defineProperty(exports,"__esModule",{value:!0});var extendStatics=function(e,t){return(extendStatics=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(e,t){e.__proto__=t}||function(e,t){for(var r in t)t.hasOwnProperty(r)&&(e[r]=t[r])})(e,t)};function __extends(e,t){function r(){this.constructor=e}extendStatics(e,t),e.prototype=null===t?Object.create(t):(r.prototype=t.prototype,new r)}var __assign=function(){return(__assign=Object.assign||function(e){for(var t,r=1,n=arguments.length;r0)&&!(n=a.next()).done;)i.push(n.value)}catch(e){o={error:e}}finally{try{n&&!n.done&&(r=a.return)&&r.call(a)}finally{if(o)throw o.error}}return i}function __spread(){for(var e=[],t=0;t2&&incorrectlyUsedAsDecorator("box");var r=asCreateObservableOptions(t);return new ObservableValue(e,getEnhancerFromOptions(r),r.name,!0,r.equals)},shallowBox:function(e,t){return arguments.length>2&&incorrectlyUsedAsDecorator("shallowBox"),deprecated("observable.shallowBox","observable.box(value, { deep: false })"),observable.box(e,{name:t,deep:!1})},array:function(e,t){arguments.length>2&&incorrectlyUsedAsDecorator("array");var r=asCreateObservableOptions(t);return new ObservableArray(e,getEnhancerFromOptions(r),r.name)},shallowArray:function(e,t){return arguments.length>2&&incorrectlyUsedAsDecorator("shallowArray"),deprecated("observable.shallowArray","observable.array(values, { deep: false })"),observable.array(e,{name:t,deep:!1})},map:function(e,t){arguments.length>2&&incorrectlyUsedAsDecorator("map");var r=asCreateObservableOptions(t);return new ObservableMap(e,getEnhancerFromOptions(r),r.name)},shallowMap:function(e,t){return arguments.length>2&&incorrectlyUsedAsDecorator("shallowMap"),deprecated("observable.shallowMap","observable.map(values, { deep: false })"),observable.map(e,{name:t,deep:!1})},set:function(e,t){arguments.length>2&&incorrectlyUsedAsDecorator("set");var r=asCreateObservableOptions(t);return new ObservableSet(e,getEnhancerFromOptions(r),r.name)},object:function(e,t,r){return"string"==typeof arguments[1]&&incorrectlyUsedAsDecorator("object"),extendObservable({},e,t,asCreateObservableOptions(r))},shallowObject:function(e,t){return"string"==typeof arguments[1]&&incorrectlyUsedAsDecorator("shallowObject"),deprecated("observable.shallowObject","observable.object(values, {}, { deep: false })"),observable.object(e,{},{name:t,deep:!1})},ref:refDecorator,shallow:shallowDecorator,deep:deepDecorator,struct:refStructDecorator},observable=createObservable;function incorrectlyUsedAsDecorator(e){fail("Expected one or two arguments to observable."+e+". Did you accidentally try to use observable."+e+" as decorator?")}Object.keys(observableFactories).forEach(function(e){return observable[e]=observableFactories[e]});var computedDecorator=createPropDecorator(!1,function(e,t,r,n,o){var a=r.get,i=r.set,s=o[0]||{};defineComputedProperty(e,t,__assign({get:a,set:i},s))}),computedStructDecorator=computedDecorator({equals:comparer.structural}),computed=function(e,t,r){if("string"==typeof t)return computedDecorator.apply(null,arguments);if(null!==e&&"object"==typeof e&&1===arguments.length)return computedDecorator.apply(null,arguments);var n="object"==typeof t?t:{};return n.get=e,n.set="function"==typeof t?t:n.set,n.name=n.name||e.name||"",new ComputedValue(n)};function createAction(e,t){var r=function(){return executeAction(e,t,this,arguments)};return r.isMobxAction=!0,r}function executeAction(e,t,r,n){var o=startAction(e,t,r,n),a=!0;try{var i=t.apply(r,n);return a=!1,i}finally{a?(globalState.suppressReactionErrors=a,endAction(o),globalState.suppressReactionErrors=!1):endAction(o)}}function startAction(e,t,r,n){var o=isSpyEnabled()&&!!e,a=0;if(o){a=Date.now();var i=n&&n.length||0,s=new Array(i);if(i>0)for(var c=0;c0;globalState.computationDepth>0&&t&&fail(!1),globalState.allowStateChanges||!t&&"strict"!==globalState.enforceActions||fail(!1)}function trackDerivedFunction(e,t,r){changeDependenciesStateTo0(e),e.newObserving=new Array(e.observing.length+100),e.unboundDepsCount=0,e.runId=++globalState.runId;var n,o=globalState.trackingDerivation;if(globalState.trackingDerivation=e,!0===globalState.disableErrorBoundaries)n=t.call(r);else try{n=t.call(r)}catch(e){n=new CaughtException(e)}return globalState.trackingDerivation=o,bindDependencies(e),n}function bindDependencies(e){for(var t=e.observing,r=e.observing=e.newObserving,n=exports.IDerivationState.UP_TO_DATE,o=0,a=e.unboundDepsCount,i=0;in&&(n=s.dependenciesState)}for(r.length=o,e.newObserving=null,a=t.length;a--;){0===(s=t[a]).diffValue&&removeObserver(s,e),s.diffValue=0}for(;o--;){var s;1===(s=r[o]).diffValue&&(s.diffValue=0,addObserver(s,e))}n!==exports.IDerivationState.UP_TO_DATE&&(e.dependenciesState=n,e.onBecomeStale())}function clearObserving(e){var t=e.observing;e.observing=[];for(var r=t.length;r--;)removeObserver(t[r],e);e.dependenciesState=exports.IDerivationState.NOT_TRACKING}function untracked(e){var t=untrackedStart(),r=e();return untrackedEnd(t),r}function untrackedStart(){var e=globalState.trackingDerivation;return globalState.trackingDerivation=null,e}function untrackedEnd(e){globalState.trackingDerivation=e}function changeDependenciesStateTo0(e){if(e.dependenciesState!==exports.IDerivationState.UP_TO_DATE){e.dependenciesState=exports.IDerivationState.UP_TO_DATE;for(var t=e.observing,r=t.length;r--;)t[r].lowestObserverState=exports.IDerivationState.UP_TO_DATE}}var persistentKeys=["mobxGuid","spyListeners","enforceActions","computedRequiresReaction","disableErrorBoundaries","runId","UNCHANGED"],MobXGlobals=function(){return function(){this.version=5,this.UNCHANGED={},this.trackingDerivation=null,this.computationDepth=0,this.runId=0,this.mobxGuid=0,this.inBatch=0,this.pendingUnobservations=[],this.pendingReactions=[],this.isRunningReactions=!1,this.allowStateChanges=!0,this.enforceActions=!1,this.spyListeners=[],this.globalReactionErrorHandlers=[],this.computedRequiresReaction=!1,this.computedConfigurable=!1,this.disableErrorBoundaries=!1,this.suppressReactionErrors=!1}}(),canMergeGlobalState=!0,isolateCalled=!1,globalState=function(){var e=getGlobal();return e.__mobxInstanceCount>0&&!e.__mobxGlobals&&(canMergeGlobalState=!1),e.__mobxGlobals&&e.__mobxGlobals.version!==(new MobXGlobals).version&&(canMergeGlobalState=!1),canMergeGlobalState?e.__mobxGlobals?(e.__mobxInstanceCount+=1,e.__mobxGlobals.UNCHANGED||(e.__mobxGlobals.UNCHANGED={}),e.__mobxGlobals):(e.__mobxInstanceCount=1,e.__mobxGlobals=new MobXGlobals):(setTimeout(function(){isolateCalled||fail("There are multiple, different versions of MobX active. Make sure MobX is loaded only once or use `configure({ isolateGlobalState: true })`")},1),new MobXGlobals)}();function isolateGlobalState(){(globalState.pendingReactions.length||globalState.inBatch||globalState.isRunningReactions)&&fail("isolateGlobalState should be called before MobX is running any reactions"),isolateCalled=!0,canMergeGlobalState&&(0==--getGlobal().__mobxInstanceCount&&(getGlobal().__mobxGlobals=void 0),globalState=new MobXGlobals)}function getGlobalState(){return globalState}function resetGlobalState(){var e=new MobXGlobals;for(var t in e)-1===persistentKeys.indexOf(t)&&(globalState[t]=e[t]);globalState.allowStateChanges=!globalState.enforceActions}function hasObservers(e){return e.observers&&e.observers.length>0}function getObservers(e){return e.observers}function addObserver(e,t){var r=e.observers.length;r&&(e.observersIndexes[t.__mapid]=r),e.observers[r]=t,e.lowestObserverState>t.dependenciesState&&(e.lowestObserverState=t.dependenciesState)}function removeObserver(e,t){if(1===e.observers.length)e.observers.length=0,queueForUnobservation(e);else{var r=e.observers,n=e.observersIndexes,o=r.pop();if(o!==t){var a=n[t.__mapid]||0;a?n[o.__mapid]=a:delete n[o.__mapid],r[a]=o}delete n[t.__mapid]}}function queueForUnobservation(e){!1===e.isPendingUnobservation&&(e.isPendingUnobservation=!0,globalState.pendingUnobservations.push(e))}function startBatch(){globalState.inBatch++}function endBatch(){if(0==--globalState.inBatch){runReactions();for(var e=globalState.pendingUnobservations,t=0;t0&&queueForUnobservation(e),!1)}function propagateChanged(e){if(e.lowestObserverState!==exports.IDerivationState.STALE){e.lowestObserverState=exports.IDerivationState.STALE;for(var t=e.observers,r=t.length;r--;){var n=t[r];n.dependenciesState===exports.IDerivationState.UP_TO_DATE&&(n.isTracing!==TraceMode.NONE&&logTraceInfo(n,e),n.onBecomeStale()),n.dependenciesState=exports.IDerivationState.STALE}}}function propagateChangeConfirmed(e){if(e.lowestObserverState!==exports.IDerivationState.STALE){e.lowestObserverState=exports.IDerivationState.STALE;for(var t=e.observers,r=t.length;r--;){var n=t[r];n.dependenciesState===exports.IDerivationState.POSSIBLY_STALE?n.dependenciesState=exports.IDerivationState.STALE:n.dependenciesState===exports.IDerivationState.UP_TO_DATE&&(e.lowestObserverState=exports.IDerivationState.UP_TO_DATE)}}}function propagateMaybeChanged(e){if(e.lowestObserverState===exports.IDerivationState.UP_TO_DATE){e.lowestObserverState=exports.IDerivationState.POSSIBLY_STALE;for(var t=e.observers,r=t.length;r--;){var n=t[r];n.dependenciesState===exports.IDerivationState.UP_TO_DATE&&(n.dependenciesState=exports.IDerivationState.POSSIBLY_STALE,n.isTracing!==TraceMode.NONE&&logTraceInfo(n,e),n.onBecomeStale())}}}function logTraceInfo(e,t){if(console.log("[mobx.trace] '"+e.name+"' is invalidated due to a change in: '"+t.name+"'"),e.isTracing===TraceMode.BREAK){var r=[];printDepTree(getDependencyTree(e),r,1),new Function("debugger;\n/*\nTracing '"+e.name+"'\n\nYou are entering this break point because derivation '"+e.name+"' is being traced and '"+t.name+"' is now forcing it to update.\nJust follow the stacktrace you should now see in the devtools to see precisely what piece of your code is causing this update\nThe stackframe you are looking for is at least ~6-8 stack-frames up.\n\n"+(e instanceof ComputedValue?e.derivation.toString().replace(/[*]\//g,"/"):"")+"\n\nThe dependencies for this derivation are:\n\n"+r.join("\n")+"\n*/\n ")()}}function printDepTree(e,t,r){t.length>=1e3?t.push("(and many more)"):(t.push(""+new Array(r).join("\t")+e.name),e.dependencies&&e.dependencies.forEach(function(e){return printDepTree(e,t,r+1)}))}var Reaction=function(){function e(e,t,r){void 0===e&&(e="Reaction@"+getNextId()),this.name=e,this.onInvalidate=t,this.errorHandler=r,this.observing=[],this.newObserving=[],this.dependenciesState=exports.IDerivationState.NOT_TRACKING,this.diffValue=0,this.runId=0,this.unboundDepsCount=0,this.__mapid="#"+getNextId(),this.isDisposed=!1,this._isScheduled=!1,this._isTrackPending=!1,this._isRunning=!1,this.isTracing=TraceMode.NONE}return e.prototype.onBecomeStale=function(){this.schedule()},e.prototype.schedule=function(){this._isScheduled||(this._isScheduled=!0,globalState.pendingReactions.push(this),runReactions())},e.prototype.isScheduled=function(){return this._isScheduled},e.prototype.runReaction=function(){if(!this.isDisposed){if(startBatch(),this._isScheduled=!1,shouldCompute(this)){this._isTrackPending=!0;try{this.onInvalidate(),this._isTrackPending&&isSpyEnabled()&&spyReport({name:this.name,type:"scheduled-reaction"})}catch(e){this.reportExceptionInDerivation(e)}}endBatch()}},e.prototype.track=function(e){startBatch();var t,r=isSpyEnabled();r&&(t=Date.now(),spyReportStart({name:this.name,type:"reaction"})),this._isRunning=!0;var n=trackDerivedFunction(this,e,void 0);this._isRunning=!1,this._isTrackPending=!1,this.isDisposed&&clearObserving(this),isCaughtException(n)&&this.reportExceptionInDerivation(n.cause),r&&spyReportEnd({time:Date.now()-t}),endBatch()},e.prototype.reportExceptionInDerivation=function(e){var t=this;if(this.errorHandler)this.errorHandler(e,this);else{if(globalState.disableErrorBoundaries)throw e;var r="[mobx] Encountered an uncaught exception that was thrown by a reaction or observer component, in: '"+this+"'";globalState.suppressReactionErrors?console.warn("[mobx] (error in reaction '"+this.name+"' suppressed, fix error of causing action below)"):console.error(r,e),isSpyEnabled()&&spyReport({type:"error",name:this.name,message:r,error:""+e}),globalState.globalReactionErrorHandlers.forEach(function(r){return r(e,t)})}},e.prototype.dispose=function(){this.isDisposed||(this.isDisposed=!0,this._isRunning||(startBatch(),clearObserving(this),endBatch()))},e.prototype.getDisposer=function(){var e=this.dispose.bind(this);return e.$mobx=this,e},e.prototype.toString=function(){return"Reaction["+this.name+"]"},e.prototype.trace=function(e){void 0===e&&(e=!1),trace(this,e)},e}();function onReactionError(e){return globalState.globalReactionErrorHandlers.push(e),function(){var t=globalState.globalReactionErrorHandlers.indexOf(e);t>=0&&globalState.globalReactionErrorHandlers.splice(t,1)}}var MAX_REACTION_ITERATIONS=100,reactionScheduler=function(e){return e()};function runReactions(){globalState.inBatch>0||globalState.isRunningReactions||reactionScheduler(runReactionsHelper)}function runReactionsHelper(){globalState.isRunningReactions=!0;for(var e=globalState.pendingReactions,t=0;e.length>0;){++t===MAX_REACTION_ITERATIONS&&(console.error("Reaction doesn't converge to a stable state after "+MAX_REACTION_ITERATIONS+" iterations. Probably there is a cycle in the reactive function: "+e[0]),e.splice(0));for(var r=e.splice(0),n=0,o=r.length;n",e):2===arguments.length&&"function"==typeof t?createAction(e,t):1===arguments.length&&"string"==typeof e?namedActionDecorator(e):!0!==n?namedActionDecorator(t).apply(null,arguments):void(e[t]=createAction(e.name||t,r.value))};function runInAction(e,t){return executeAction("string"==typeof e?e:e.name||"","function"==typeof e?e:t,this,void 0)}function isAction(e){return"function"==typeof e&&!0===e.isMobxAction}function defineBoundAction(e,t,r){addHiddenProp(e,t,createAction(t,r.bind(e)))}function autorun(e,t){void 0===t&&(t=EMPTY_OBJECT);var r,n=t&&t.name||e.name||"Autorun@"+getNextId();if(!t.scheduler&&!t.delay)r=new Reaction(n,function(){this.track(i)},t.onError);else{var o=createSchedulerFromOptions(t),a=!1;r=new Reaction(n,function(){a||(a=!0,o(function(){a=!1,r.isDisposed||r.track(i)}))},t.onError)}function i(){e(r)}return r.schedule(),r.getDisposer()}action.bound=boundActionDecorator;var run=function(e){return e()};function createSchedulerFromOptions(e){return e.scheduler?e.scheduler:e.delay?function(t){return setTimeout(t,e.delay)}:run}function reaction(e,t,r){void 0===r&&(r=EMPTY_OBJECT),"boolean"==typeof r&&(r={fireImmediately:r},deprecated("Using fireImmediately as argument is deprecated. Use '{ fireImmediately: true }' instead"));var n,o=r.name||"Reaction@"+getNextId(),a=action(o,r.onError?wrapErrorHandler(r.onError,t):t),i=!r.scheduler&&!r.delay,s=createSchedulerFromOptions(r),c=!0,l=!1,u=r.compareStructural?comparer.structural:r.equals||comparer.default,p=new Reaction(o,function(){c||i?b():l||(l=!0,s(b))},r.onError);function b(){if(l=!1,!p.isDisposed){var t=!1;p.track(function(){var r=e(p);t=c||!u(n,r),n=r}),c&&r.fireImmediately&&a(n,p),c||!0!==t||a(n,p),c&&(c=!1)}}return p.schedule(),p.getDisposer()}function wrapErrorHandler(e,t){return function(){try{return t.apply(this,arguments)}catch(t){e.call(this,t)}}}function onBecomeObserved(e,t,r){return interceptHook("onBecomeObserved",e,t,r)}function onBecomeUnobserved(e,t,r){return interceptHook("onBecomeUnobserved",e,t,r)}function interceptHook(e,t,r,n){var o="string"==typeof r?getAtom(t,r):getAtom(t),a="string"==typeof r?n:r,i=o[e];return"function"!=typeof i?fail(!1):(o[e]=function(){i.call(this),a.call(this)},function(){o[e]=i})}function configure(e){var t=e.enforceActions,r=e.computedRequiresReaction,n=e.computedConfigurable,o=e.disableErrorBoundaries,a=e.arrayBuffer,i=e.reactionScheduler;if(!0===e.isolateGlobalState&&isolateGlobalState(),void 0!==t){"boolean"!=typeof t&&"strict"!==t||deprecated("Deprecated value for 'enforceActions', use 'false' => '\"never\"', 'true' => '\"observed\"', '\"strict\"' => \"'always'\" instead");var s=void 0;switch(t){case!0:case"observed":s=!0;break;case!1:case"never":s=!1;break;case"strict":case"always":s="strict";break;default:fail("Invalid value for 'enforceActions': '"+t+"', expected 'never', 'always' or 'observed'")}globalState.enforceActions=s,globalState.allowStateChanges=!0!==s&&"strict"!==s}void 0!==r&&(globalState.computedRequiresReaction=!!r),void 0!==n&&(globalState.computedConfigurable=!!n),void 0!==o&&(!0===o&&console.warn("WARNING: Debug feature only. MobX will NOT recover from errors if this is on."),globalState.disableErrorBoundaries=!!o),"number"==typeof a&&reserveArrayBuffer(a),i&&setReactionScheduler(i)}function decorate(e,t){var r="function"==typeof e?e.prototype:e,n=function(e){var n=t[e];Array.isArray(n)||(n=[n]);var o=Object.getOwnPropertyDescriptor(r,e),a=n.reduce(function(t,n){return n(r,e,t)},o);a&&Object.defineProperty(r,e,a)};for(var o in t)n(o);return e}function extendShallowObservable(e,t,r){return deprecated("'extendShallowObservable' is deprecated, use 'extendObservable(target, props, { deep: false })' instead"),extendObservable(e,t,r,shallowCreateObservableOptions)}function extendObservable(e,t,r,n){var o=(n=asCreateObservableOptions(n)).defaultDecorator||(!1===n.deep?refDecorator:deepDecorator);initializeInstance(e),asObservableObject(e,n.name,o.enhancer),startBatch();try{for(var a in t){var i=Object.getOwnPropertyDescriptor(t,a);0;var s=r&&a in r?r[a]:i.get?computedDecorator:o;0;var c=s(e,a,i,!0);c&&Object.defineProperty(e,a,c)}}finally{endBatch()}return e}function getDependencyTree(e,t){return nodeToDependencyTree(getAtom(e,t))}function nodeToDependencyTree(e){var t={name:e.name};return e.observing&&e.observing.length>0&&(t.dependencies=unique(e.observing).map(nodeToDependencyTree)),t}function getObserverTree(e,t){return nodeToObserverTree(getAtom(e,t))}function nodeToObserverTree(e){var t={name:e.name};return hasObservers(e)&&(t.observers=getObservers(e).map(nodeToObserverTree)),t}var generatorId=0;function flow(e){1!==arguments.length&&fail("Flow expects one 1 argument and cannot be used as decorator");var t=e.name||"";return function(){var r,n=arguments,o=++generatorId,a=action(t+" - runid: "+o+" - init",e).apply(this,n),i=void 0,s=new Promise(function(e,n){var s=0;function c(e){var r;i=void 0;try{r=action(t+" - runid: "+o+" - yield "+s++,a.next).call(a,e)}catch(e){return n(e)}u(r)}function l(e){var r;i=void 0;try{r=action(t+" - runid: "+o+" - yield "+s++,a.throw).call(a,e)}catch(e){return n(e)}u(r)}function u(t){if(!t||"function"!=typeof t.then)return t.done?e(t.value):(i=Promise.resolve(t.value)).then(c,l);t.then(u,n)}r=n,c(void 0)});return s.cancel=action(t+" - runid: "+o+" - cancel",function(){try{i&&cancelPromise(i);var e=a.return(),t=Promise.resolve(e.value);t.then(noop,noop),cancelPromise(t),r(new Error("FLOW_CANCELLED"))}catch(e){r(e)}}),s}}function cancelPromise(e){"function"==typeof e.cancel&&e.cancel()}function interceptReads(e,t,r){var n;if(isObservableMap(e)||isObservableArray(e)||isObservableValue(e))n=getAdministration(e);else{if(!isObservableObject(e))return fail(!1);if("string"!=typeof t)return fail(!1);n=getAdministration(e,t)}return void 0!==n.dehancer?fail(!1):(n.dehancer="function"==typeof t?t:r,function(){n.dehancer=void 0})}function intercept(e,t,r){return"function"==typeof r?interceptProperty(e,t,r):interceptInterceptable(e,t)}function interceptInterceptable(e,t){return getAdministration(e).intercept(t)}function interceptProperty(e,t,r){return getAdministration(e,t).intercept(r)}function _isComputed(e,t){if(null==e)return!1;if(void 0!==t){if(!1===isObservableObject(e))return!1;if(!e.$mobx.values[t])return!1;var r=getAtom(e,t);return isComputedValue(r)}return isComputedValue(e)}function isComputed(e){return arguments.length>1?fail(!1):_isComputed(e)}function isComputedProp(e,t){return"string"!=typeof t?fail(!1):_isComputed(e,t)}function _isObservable(e,t){if(null==e)return!1;if(void 0!==t){if(isObservableObject(e)){var r=e.$mobx;return r.values&&!!r.values[t]}return!1}return isObservableObject(e)||!!e.$mobx||isAtom(e)||isReaction(e)||isComputedValue(e)}function isObservable(e){return 1!==arguments.length&&fail(!1),_isObservable(e)}function isObservableProp(e,t){return"string"!=typeof t?fail(!1):_isObservable(e,t)}function keys(e){return isObservableObject(e)?e.$mobx.getKeys():isObservableMap(e)?e._keys.slice():isObservableSet(e)?iteratorToArray(e.keys()):isObservableArray(e)?e.map(function(e,t){return t}):fail(!1)}function values(e){return isObservableObject(e)?keys(e).map(function(t){return e[t]}):isObservableMap(e)?keys(e).map(function(t){return e.get(t)}):isObservableSet(e)?iteratorToArray(e.values()):isObservableArray(e)?e.slice():fail(!1)}function entries(e){return isObservableObject(e)?keys(e).map(function(t){return[t,e[t]]}):isObservableMap(e)?keys(e).map(function(t){return[t,e.get(t)]}):isObservableSet(e)?iteratorToArray(e.entries()):isObservableArray(e)?e.map(function(e,t){return[t,e]}):fail(!1)}function set(e,t,r){if(2!==arguments.length||isObservableSet(e))if(isObservableObject(e)){var n=e.$mobx;n.values[t]?n.write(e,t,r):defineObservableProperty(e,t,r,n.defaultEnhancer)}else if(isObservableMap(e))e.set(t,r);else if(isObservableSet(e))e.add(t);else{if(!isObservableArray(e))return fail(!1);"number"!=typeof t&&(t=parseInt(t,10)),invariant(t>=0,"Not a valid index: '"+t+"'"),startBatch(),t>=e.length&&(e.length=t+1),e[t]=r,endBatch()}else{startBatch();var o=t;try{for(var a in o)set(e,a,o[a])}finally{endBatch()}}}function remove(e,t){if(isObservableObject(e))e.$mobx.remove(t);else if(isObservableMap(e))e.delete(t);else if(isObservableSet(e))e.delete(t);else{if(!isObservableArray(e))return fail(!1);"number"!=typeof t&&(t=parseInt(t,10)),invariant(t>=0,"Not a valid index: '"+t+"'"),e.splice(t,1)}}function has(e,t){if(isObservableObject(e)){var r=getAdministration(e);return r.getKeys(),!!r.values[t]}return isObservableMap(e)?e.has(t):isObservableSet(e)?e.has(t):isObservableArray(e)?t>=0&&t0}function registerInterceptor(e,t){var r=e.interceptors||(e.interceptors=[]);return r.push(t),once(function(){var e=r.indexOf(t);-1!==e&&r.splice(e,1)})}function interceptChange(e,t){var r=untrackedStart();try{var n=e.interceptors;if(n)for(var o=0,a=n.length;o0}function registerListener(e,t){var r=e.changeListeners||(e.changeListeners=[]);return r.push(t),once(function(){var e=r.indexOf(t);-1!==e&&r.splice(e,1)})}function notifyListeners(e,t){var r=untrackedStart(),n=e.changeListeners;if(n){for(var o=0,a=(n=n.slice()).length;o0?e.map(this.dehancer):e},e.prototype.intercept=function(e){return registerInterceptor(this,e)},e.prototype.observe=function(e,t){return void 0===t&&(t=!1),t&&e({object:this.array,type:"splice",index:0,added:this.values.slice(),addedCount:this.values.length,removed:[],removedCount:0}),registerListener(this,e)},e.prototype.getArrayLength=function(){return this.atom.reportObserved(),this.values.length},e.prototype.setArrayLength=function(e){if("number"!=typeof e||e<0)throw new Error("[mobx.array] Out of range: "+e);var t=this.values.length;if(e!==t)if(e>t){for(var r=new Array(e-t),n=0;n0&&e+t+1>OBSERVABLE_ARRAY_BUFFER_SIZE&&reserveArrayBuffer(e+t+1)},e.prototype.spliceWithArray=function(e,t,r){var n=this;checkIfStateModificationsAreAllowed(this.atom);var o=this.values.length;if(void 0===e?e=0:e>o?e=o:e<0&&(e=Math.max(0,o+e)),t=1===arguments.length?o-e:null==t?0:Math.max(0,Math.min(t,o-e)),void 0===r&&(r=EMPTY_ARRAY),hasInterceptors(this)){var a=interceptChange(this,{object:this.array,type:"splice",index:e,removedCount:t,added:r});if(!a)return EMPTY_ARRAY;t=a.removedCount,r=a.added}var i=(r=0===r.length?r:r.map(function(e){return n.enhancer(e,void 0)})).length-t;this.updateArrayLength(o,i);var s=this.spliceItemsIntoValues(e,t,r);return 0===t&&0===r.length||this.notifyArraySplice(e,r,s),this.dehanceValues(s)},e.prototype.spliceItemsIntoValues=function(e,t,r){var n;if(r.length-1&&(this.splice(t,1),!0)},t.prototype.move=function(e,t){function r(e){if(e<0)throw new Error("[mobx.array] Index out of bounds: "+e+" is negative");var t=this.$mobx.values.length;if(e>=t)throw new Error("[mobx.array] Index out of bounds: "+e+" is not smaller than "+t)}if(deprecated("observableArray.move is deprecated, use .slice() & .replace() instead"),r.call(this,e),r.call(this,t),e!==t){var n,o=this.$mobx.values;n=e
+
+
+轻量、可靠的小程序 UI 组件库
+
+
+
+
+
+
+
+
+
+ 🔥 文档网站(国内)
+
+ 🌈 文档网站(GitHub)
+
+ 🚀 Vue 版
+
+
+---
+
+### 介绍
+
+Vant 是一个**轻量、可靠的移动端组件库**,于 2017 年开源。
+
+目前 Vant 官方提供了 [Vue 2 版本](https://vant-contrib.gitee.io/vant/v2)、[Vue 3 版本](https://vant-contrib.gitee.io/vant)和[微信小程序版本](http://vant-contrib.gitee.io/vant-weapp),并由社区团队维护 [React 版本](https://github.com/3lang3/react-vant)和[支付宝小程序版本](https://github.com/ant-move/Vant-Aliapp)。
+
+## 预览
+
+扫描下方小程序二维码,体验组件库示例。注意:因微信审核机制限制,目前示例小程序不是最新版本,可以 clone 代码到本地开发工具预览
+
+
+
+## 使用之前
+
+使用 Vant Weapp 前,请确保你已经学习过微信官方的 [小程序简易教程](https://developers.weixin.qq.com/miniprogram/dev/framework/) 和 [自定义组件介绍](https://developers.weixin.qq.com/miniprogram/dev/framework/custom-component/)。
+
+## 安装
+
+### 方式一. 通过 npm 安装 (推荐)
+
+小程序已经支持使用 npm 安装第三方包,详见 [npm 支持](https://developers.weixin.qq.com/miniprogram/dev/devtools/npm.html?search-key=npm)
+
+```bash
+# 通过 npm 安装
+npm i @vant/weapp -S --production
+
+# 通过 yarn 安装
+yarn add @vant/weapp --production
+
+# 安装 0.x 版本
+npm i vant-weapp -S --production
+```
+
+### 方式二. 下载代码
+
+直接通过 git 下载 Vant Weapp 源代码,并将 `dist` 目录拷贝到自己的项目中。
+
+```bash
+git clone https://github.com/youzan/vant-weapp.git
+```
+
+## 使用组件
+
+以按钮组件为例,只需要在 json 文件中引入按钮对应的自定义组件即可
+
+```json
+{
+ "usingComponents": {
+ "van-button": "/path/to/vant-weapp/dist/button/index"
+ }
+}
+```
+
+接着就可以在 wxml 中直接使用组件
+
+```html
+按钮
+```
+
+## 在开发者工具中预览
+
+```bash
+# 安装项目依赖
+npm install
+
+# 执行组件编译
+npm run dev
+```
+
+打开[微信开发者工具](https://developers.weixin.qq.com/miniprogram/dev/devtools/download.html),把`vant-weapp/example`目录添加进去就可以预览示例了。
+
+PS:关于 `van-area` Area 省市区选择组件,地区数据初始化可以直接在云开发环境中导入`vant-weapp/example/database_area.JSON` 文件使用。
+
+## 基础库版本
+
+Vant Weapp 最低支持到小程序基础库 2.6.5 版本。
+
+## 链接
+
+- [意见反馈](https://github.com/youzan/vant-weapp/issues)
+- [设计资源](https://vant-contrib.gitee.io/vant/#/zh-CN/design)
+- [更新日志](https://vant-contrib.gitee.io/vant-weapp/#/changelog)
+- [官方示例](https://github.com/vant-ui/vant-demo)
+
+## 核心团队
+
+以下是 Vant 和 Vant Weapp 的核心贡献者们:
+
+| [](https://github.com/chenjiahan/) | [](https://github.com/cookfront/) | [](https://github.com/w91/) | [](https://github.com/pangxie1991/) | [](https://github.com/rex-zsd/) | [](https://github.com/nemo-shen/) |
+| :-: | :-: | :-: | :-: | :-: | :-: |
+| [chenjiahan](https://github.com/chenjiahan/) | [cookfront](https://github.com/cookfront/) | [wangnaiyi](https://github.com/w91/) | [pangxie](https://github.com/pangxie1991/) | [rex-zsd](https://github.com/rex-zsd/) | [nemo-shen](https://github.com/nemo-shen/) |
+
+| [](https://github.com/Lindysen/) | [](https://github.com/JakeLaoyu/) | [](https://github.com/landluck/) | [](https://github.com/wjw-gavin/) | [](https://github.com/inottn/) | [](https://github.com/zhousg/) |
+| :-: | :-: | :-: | :-: | :-: | :-: |
+| [Lindysen](https://github.com/Lindysen/) | [JakeLaoyu](https://github.com/JakeLaoyu/) | [landluck](https://github.com/landluck/) | [wjw-gavin](https://github.com/wjw-gavin/) | [inottn](https://github.com/inottn/) | [zhousg](https://github.com/zhousg/) |
+
+## 贡献者们
+
+感谢以下小伙伴们为 Vant Weapp 发展做出的贡献:
+
+
+
+
+
+## 开源协议
+
+本项目基于 [MIT](https://zh.wikipedia.org/wiki/MIT%E8%A8%B1%E5%8F%AF%E8%AD%89)协议,请自由地享受和参与开源。
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.js
new file mode 100644
index 0000000..9c4879c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.js
@@ -0,0 +1,75 @@
+import { VantComponent } from '../common/component';
+import { button } from '../mixins/button';
+VantComponent({
+ classes: ['list-class'],
+ mixins: [button],
+ props: {
+ show: Boolean,
+ title: String,
+ cancelText: String,
+ description: String,
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ actions: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickAction: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onSelect(event) {
+ const { index } = event.currentTarget.dataset;
+ const { actions, closeOnClickAction, canIUseGetUserProfile } = this.data;
+ const item = actions[index];
+ if (item) {
+ this.$emit('select', item);
+ if (closeOnClickAction) {
+ this.onClose();
+ }
+ if (item.openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: item.getUserProfileDesc || ' ',
+ complete: (userProfile) => {
+ this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ }
+ },
+ onCancel() {
+ this.$emit('cancel');
+ },
+ onClose() {
+ this.$emit('close');
+ },
+ onClickOverlay() {
+ this.$emit('click-overlay');
+ this.onClose();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.json
new file mode 100644
index 0000000..19bf989
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-popup": "../popup/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxml
new file mode 100644
index 0000000..6311e33
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
+
+
+
+ {{ cancelText }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxss
new file mode 100644
index 0000000..eedd361
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/action-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-action-sheet{color:var(--action-sheet-item-text-color,#323233);max-height:var(--action-sheet-max-height,90%)!important}.van-action-sheet__cancel,.van-action-sheet__item{background-color:var(--action-sheet-item-background,#fff);font-size:var(--action-sheet-item-font-size,16px);line-height:var(--action-sheet-item-line-height,22px);padding:14px 16px;text-align:center}.van-action-sheet__cancel--hover,.van-action-sheet__item--hover{background-color:#f2f3f5}.van-action-sheet__cancel:after,.van-action-sheet__item:after{border-width:0}.van-action-sheet__cancel{color:var(--action-sheet-cancel-text-color,#646566)}.van-action-sheet__gap{background-color:var(--action-sheet-cancel-padding-color,#f7f8fa);display:block;height:var(--action-sheet-cancel-padding-top,8px)}.van-action-sheet__item--disabled{color:var(--action-sheet-item-disabled-text-color,#c8c9cc)}.van-action-sheet__item--disabled.van-action-sheet__item--hover{background-color:var(--action-sheet-item-background,#fff)}.van-action-sheet__subname{color:var(--action-sheet-subname-color,#969799);font-size:var(--action-sheet-subname-font-size,12px);line-height:var(--action-sheet-subname-line-height,20px);margin-top:var(--padding-xs,8px)}.van-action-sheet__header{font-size:var(--action-sheet-header-font-size,16px);font-weight:var(--font-weight-bold,500);line-height:var(--action-sheet-header-height,48px);text-align:center}.van-action-sheet__description{color:var(--action-sheet-description-color,#969799);font-size:var(--action-sheet-description-font-size,14px);line-height:var(--action-sheet-description-line-height,20px);padding:20px var(--padding-md,16px);text-align:center}.van-action-sheet__close{color:var(--action-sheet-close-icon-color,#c8c9cc);font-size:var(--action-sheet-close-icon-size,22px)!important;line-height:inherit!important;padding:var(--action-sheet-close-icon-padding,0 16px);position:absolute!important;right:0;top:0}.van-action-sheet__loading{display:flex!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.js
new file mode 100644
index 0000000..9cf1edd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.js
@@ -0,0 +1,220 @@
+import { VantComponent } from '../common/component';
+import { pickerProps } from '../picker/shared';
+import { requestAnimationFrame } from '../common/utils';
+const EMPTY_CODE = '000000';
+VantComponent({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: Object.assign(Object.assign({}, pickerProps), { showToolbar: {
+ type: Boolean,
+ value: true,
+ }, value: {
+ type: String,
+ observer(value) {
+ this.code = value;
+ this.setValues();
+ },
+ }, areaList: {
+ type: Object,
+ value: {},
+ observer: 'setValues',
+ }, columnsNum: {
+ type: null,
+ value: 3,
+ }, columnsPlaceholder: {
+ type: Array,
+ observer(val) {
+ this.setData({
+ typeToColumnsPlaceholder: {
+ province: val[0] || '',
+ city: val[1] || '',
+ county: val[2] || '',
+ },
+ });
+ },
+ } }),
+ data: {
+ columns: [{ values: [] }, { values: [] }, { values: [] }],
+ typeToColumnsPlaceholder: {},
+ },
+ mounted() {
+ requestAnimationFrame(() => {
+ this.setValues();
+ });
+ },
+ methods: {
+ getPicker() {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-area__picker');
+ }
+ return this.picker;
+ },
+ onCancel(event) {
+ this.emit('cancel', event.detail);
+ },
+ onConfirm(event) {
+ const { index } = event.detail;
+ let { value } = event.detail;
+ value = this.parseValues(value);
+ this.emit('confirm', { value, index });
+ },
+ emit(type, detail) {
+ detail.values = detail.value;
+ delete detail.value;
+ this.$emit(type, detail);
+ },
+ parseValues(values) {
+ const { columnsPlaceholder } = this.data;
+ return values.map((value, index) => {
+ if (value &&
+ (!value.code || value.name === columnsPlaceholder[index])) {
+ return Object.assign(Object.assign({}, value), { code: '', name: '' });
+ }
+ return value;
+ });
+ },
+ onChange(event) {
+ var _a;
+ const { index, picker, value } = event.detail;
+ this.code = value[index].code;
+ (_a = this.setValues()) === null || _a === void 0 ? void 0 : _a.then(() => {
+ this.$emit('change', {
+ picker,
+ values: this.parseValues(picker.getValues()),
+ index,
+ });
+ });
+ },
+ getConfig(type) {
+ const { areaList } = this.data;
+ return (areaList && areaList[`${type}_list`]) || {};
+ },
+ getList(type, code) {
+ if (type !== 'province' && !code) {
+ return [];
+ }
+ const { typeToColumnsPlaceholder } = this.data;
+ const list = this.getConfig(type);
+ let result = Object.keys(list).map((code) => ({
+ code,
+ name: list[code],
+ }));
+ if (code != null) {
+ // oversea code
+ if (code[0] === '9' && type === 'city') {
+ code = '9';
+ }
+ result = result.filter((item) => item.code.indexOf(code) === 0);
+ }
+ if (typeToColumnsPlaceholder[type] && result.length) {
+ // set columns placeholder
+ const codeFill = type === 'province'
+ ? ''
+ : type === 'city'
+ ? EMPTY_CODE.slice(2, 4)
+ : EMPTY_CODE.slice(4, 6);
+ result.unshift({
+ code: `${code}${codeFill}`,
+ name: typeToColumnsPlaceholder[type],
+ });
+ }
+ return result;
+ },
+ getIndex(type, code) {
+ let compareNum = type === 'province' ? 2 : type === 'city' ? 4 : 6;
+ const list = this.getList(type, code.slice(0, compareNum - 2));
+ // oversea code
+ if (code[0] === '9' && type === 'province') {
+ compareNum = 1;
+ }
+ code = code.slice(0, compareNum);
+ for (let i = 0; i < list.length; i++) {
+ if (list[i].code.slice(0, compareNum) === code) {
+ return i;
+ }
+ }
+ return 0;
+ },
+ setValues() {
+ const picker = this.getPicker();
+ if (!picker) {
+ return;
+ }
+ let code = this.code || this.getDefaultCode();
+ const provinceList = this.getList('province');
+ const cityList = this.getList('city', code.slice(0, 2));
+ const stack = [];
+ const indexes = [];
+ const { columnsNum } = this.data;
+ if (columnsNum >= 1) {
+ stack.push(picker.setColumnValues(0, provinceList, false));
+ indexes.push(this.getIndex('province', code));
+ }
+ if (columnsNum >= 2) {
+ stack.push(picker.setColumnValues(1, cityList, false));
+ indexes.push(this.getIndex('city', code));
+ if (cityList.length && code.slice(2, 4) === '00') {
+ [{ code }] = cityList;
+ }
+ }
+ if (columnsNum === 3) {
+ stack.push(picker.setColumnValues(2, this.getList('county', code.slice(0, 4)), false));
+ indexes.push(this.getIndex('county', code));
+ }
+ return Promise.all(stack)
+ .catch(() => { })
+ .then(() => picker.setIndexes(indexes))
+ .catch(() => { });
+ },
+ getDefaultCode() {
+ const { columnsPlaceholder } = this.data;
+ if (columnsPlaceholder.length) {
+ return EMPTY_CODE;
+ }
+ const countyCodes = Object.keys(this.getConfig('county'));
+ if (countyCodes[0]) {
+ return countyCodes[0];
+ }
+ const cityCodes = Object.keys(this.getConfig('city'));
+ if (cityCodes[0]) {
+ return cityCodes[0];
+ }
+ return '';
+ },
+ getValues() {
+ const picker = this.getPicker();
+ if (!picker) {
+ return [];
+ }
+ return this.parseValues(picker.getValues().filter((value) => !!value));
+ },
+ getDetail() {
+ const values = this.getValues();
+ const area = {
+ code: '',
+ country: '',
+ province: '',
+ city: '',
+ county: '',
+ };
+ if (!values.length) {
+ return area;
+ }
+ const names = values.map((item) => item.name);
+ area.code = values[values.length - 1].code;
+ if (area.code[0] === '9') {
+ area.country = names[1] || '';
+ area.province = names[2] || '';
+ }
+ else {
+ area.province = names[0] || '';
+ area.city = names[1] || '';
+ area.county = names[2] || '';
+ }
+ return area;
+ },
+ reset(code) {
+ this.code = code || '';
+ return this.setValues();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxml
new file mode 100644
index 0000000..3a437b7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxml
@@ -0,0 +1,20 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxs
new file mode 100644
index 0000000..07723c1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxs
@@ -0,0 +1,8 @@
+/* eslint-disable */
+function displayColumns(columns, columnsNum) {
+ return columns.slice(0, +columnsNum);
+}
+
+module.exports = {
+ displayColumns: displayColumns,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/area/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.js
new file mode 100644
index 0000000..0e3c134
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.js
@@ -0,0 +1,64 @@
+import { VantComponent } from '../common/component';
+import { button } from '../mixins/button';
+import { canIUseFormFieldButton } from '../common/version';
+const mixins = [button];
+if (canIUseFormFieldButton()) {
+ mixins.push('wx://form-field-button');
+}
+VantComponent({
+ mixins,
+ classes: ['hover-class', 'loading-class'],
+ data: {
+ baseStyle: '',
+ },
+ props: {
+ formType: String,
+ icon: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ plain: Boolean,
+ block: Boolean,
+ round: Boolean,
+ square: Boolean,
+ loading: Boolean,
+ hairline: Boolean,
+ disabled: Boolean,
+ loadingText: String,
+ customStyle: String,
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ type: {
+ type: String,
+ value: 'default',
+ },
+ dataset: null,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loadingSize: {
+ type: String,
+ value: '20px',
+ },
+ color: String,
+ },
+ methods: {
+ onClick(event) {
+ this.$emit('click', event);
+ const { canIUseGetUserProfile, openType, getUserProfileDesc, lang, } = this.data;
+ if (openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: getUserProfileDesc || ' ',
+ lang: lang || 'en',
+ complete: (userProfile) => {
+ this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxml
new file mode 100644
index 0000000..e7f60f1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxs
new file mode 100644
index 0000000..8b649fe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ if (!data.color) {
+ return data.customStyle;
+ }
+
+ var properties = {
+ color: data.plain ? data.color : '#fff',
+ background: data.plain ? null : data.color,
+ };
+
+ // hide border when color is linear-gradient
+ if (data.color.indexOf('gradient') !== -1) {
+ properties.border = 0;
+ } else {
+ properties['border-color'] = data.color;
+ }
+
+ return style([properties, data.customStyle]);
+}
+
+function loadingColor(data) {
+ if (data.plain) {
+ return data.color ? data.color : '#c9c9c9';
+ }
+
+ if (data.type === 'default') {
+ return '#c9c9c9';
+ }
+
+ return '#fff';
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxss
new file mode 100644
index 0000000..bd8bb5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-button{-webkit-text-size-adjust:100%;align-items:center;-webkit-appearance:none;border-radius:var(--button-border-radius,2px);box-sizing:border-box;display:inline-flex;font-size:var(--button-default-font-size,16px);height:var(--button-default-height,44px);justify-content:center;line-height:var(--button-line-height,20px);padding:0;position:relative;text-align:center;transition:opacity .2s;vertical-align:middle}.van-button:before{background-color:#000;border:inherit;border-color:#000;border-radius:inherit;content:" ";height:100%;left:50%;opacity:0;position:absolute;top:50%;transform:translate(-50%,-50%);width:100%}.van-button:after{border-width:0}.van-button--active:before{opacity:.15}.van-button--unclickable:after{display:none}.van-button--default{background:var(--button-default-background-color,#fff);border:var(--button-border-width,1px) solid var(--button-default-border-color,#ebedf0);color:var(--button-default-color,#323233)}.van-button--primary{background:var(--button-primary-background-color,#07c160);border:var(--button-border-width,1px) solid var(--button-primary-border-color,#07c160);color:var(--button-primary-color,#fff)}.van-button--info{background:var(--button-info-background-color,#1989fa);border:var(--button-border-width,1px) solid var(--button-info-border-color,#1989fa);color:var(--button-info-color,#fff)}.van-button--danger{background:var(--button-danger-background-color,#ee0a24);border:var(--button-border-width,1px) solid var(--button-danger-border-color,#ee0a24);color:var(--button-danger-color,#fff)}.van-button--warning{background:var(--button-warning-background-color,#ff976a);border:var(--button-border-width,1px) solid var(--button-warning-border-color,#ff976a);color:var(--button-warning-color,#fff)}.van-button--plain{background:var(--button-plain-background-color,#fff)}.van-button--plain.van-button--primary{color:var(--button-primary-background-color,#07c160)}.van-button--plain.van-button--info{color:var(--button-info-background-color,#1989fa)}.van-button--plain.van-button--danger{color:var(--button-danger-background-color,#ee0a24)}.van-button--plain.van-button--warning{color:var(--button-warning-background-color,#ff976a)}.van-button--large{height:var(--button-large-height,50px);width:100%}.van-button--normal{font-size:var(--button-normal-font-size,14px);padding:0 15px}.van-button--small{font-size:var(--button-small-font-size,12px);height:var(--button-small-height,30px);min-width:var(--button-small-min-width,60px);padding:0 var(--padding-xs,8px)}.van-button--mini{display:inline-block;font-size:var(--button-mini-font-size,10px);height:var(--button-mini-height,22px);min-width:var(--button-mini-min-width,50px)}.van-button--mini+.van-button--mini{margin-left:5px}.van-button--block{display:flex;width:100%}.van-button--round{border-radius:var(--button-round-border-radius,999px)}.van-button--square{border-radius:0}.van-button--disabled{opacity:var(--button-disabled-opacity,.5)}.van-button__text{display:inline}.van-button__icon+.van-button__text:not(:empty),.van-button__loading-text{margin-left:4px}.van-button__icon{line-height:inherit!important;min-width:1em;vertical-align:top}.van-button--hairline{border-width:0;padding-top:1px}.van-button--hairline:after{border-color:inherit;border-radius:calc(var(--button-border-radius, 2px)*2);border-width:1px}.van-button--hairline.van-button--round:after{border-radius:var(--button-round-border-radius,999px)}.van-button--hairline.van-button--square:after{border-radius:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/calendar.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/calendar.wxml
new file mode 100644
index 0000000..2ddb048
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/calendar.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.js
new file mode 100644
index 0000000..8fb3682
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.js
@@ -0,0 +1,37 @@
+import { VantComponent } from '../../../common/component';
+VantComponent({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ subtitle: String,
+ showTitle: Boolean,
+ showSubtitle: Boolean,
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'initWeekDay',
+ },
+ },
+ data: {
+ weekdays: [],
+ },
+ created() {
+ this.initWeekDay();
+ },
+ methods: {
+ initWeekDay() {
+ const defaultWeeks = ['日', '一', '二', '三', '四', '五', '六'];
+ const firstDayOfWeek = this.data.firstDayOfWeek || 0;
+ this.setData({
+ weekdays: [
+ ...defaultWeeks.slice(firstDayOfWeek, 7),
+ ...defaultWeeks.slice(0, firstDayOfWeek),
+ ],
+ });
+ },
+ onClickSubtitle(event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxml
new file mode 100644
index 0000000..7e56c83
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxss
new file mode 100644
index 0000000..272537e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/header/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar__header{box-shadow:var(--calendar-header-box-shadow,0 2px 10px hsla(220,1%,50%,.16));flex-shrink:0}.van-calendar__header-subtitle,.van-calendar__header-title{font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__header-title+.van-calendar__header-title,.van-calendar__header-title:empty{display:none}.van-calendar__header-title:empty+.van-calendar__header-title{display:block!important}.van-calendar__weekdays{display:flex}.van-calendar__weekday{flex:1;font-size:var(--calendar-weekdays-font-size,12px);line-height:var(--calendar-weekdays-height,30px);text-align:center}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.d.ts
new file mode 100644
index 0000000..3ccf85a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.d.ts
@@ -0,0 +1,6 @@
+export interface Day {
+ date: Date;
+ type: string;
+ text: number;
+ bottomInfo?: string;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.js
new file mode 100644
index 0000000..d04c0fe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.js
@@ -0,0 +1,154 @@
+import { VantComponent } from '../../../common/component';
+import { getMonthEndDay, compareDay, getPrevDay, getNextDay, } from '../../utils';
+VantComponent({
+ props: {
+ date: {
+ type: null,
+ observer: 'setDays',
+ },
+ type: {
+ type: String,
+ observer: 'setDays',
+ },
+ color: String,
+ minDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ maxDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ showMark: Boolean,
+ rowHeight: null,
+ formatter: {
+ type: null,
+ observer: 'setDays',
+ },
+ currentDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'setDays',
+ },
+ allowSameDay: Boolean,
+ showSubtitle: Boolean,
+ showMonthTitle: Boolean,
+ },
+ data: {
+ visible: true,
+ days: [],
+ },
+ methods: {
+ onClick(event) {
+ const { index } = event.currentTarget.dataset;
+ const item = this.data.days[index];
+ if (item.type !== 'disabled') {
+ this.$emit('click', item);
+ }
+ },
+ setDays() {
+ const days = [];
+ const startDate = new Date(this.data.date);
+ const year = startDate.getFullYear();
+ const month = startDate.getMonth();
+ const totalDay = getMonthEndDay(startDate.getFullYear(), startDate.getMonth() + 1);
+ for (let day = 1; day <= totalDay; day++) {
+ const date = new Date(year, month, day);
+ const type = this.getDayType(date);
+ let config = {
+ date,
+ type,
+ text: day,
+ bottomInfo: this.getBottomInfo(type),
+ };
+ if (this.data.formatter) {
+ config = this.data.formatter(config);
+ }
+ days.push(config);
+ }
+ this.setData({ days });
+ },
+ getMultipleDayType(day) {
+ const { currentDate } = this.data;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ const isSelected = (date) => currentDate.some((item) => compareDay(item, date) === 0);
+ if (isSelected(day)) {
+ const prevDay = getPrevDay(day);
+ const nextDay = getNextDay(day);
+ const prevSelected = isSelected(prevDay);
+ const nextSelected = isSelected(nextDay);
+ if (prevSelected && nextSelected) {
+ return 'multiple-middle';
+ }
+ if (prevSelected) {
+ return 'end';
+ }
+ return nextSelected ? 'start' : 'multiple-selected';
+ }
+ return '';
+ },
+ getRangeDayType(day) {
+ const { currentDate, allowSameDay } = this.data;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ const [startDay, endDay] = currentDate;
+ if (!startDay) {
+ return '';
+ }
+ const compareToStart = compareDay(day, startDay);
+ if (!endDay) {
+ return compareToStart === 0 ? 'start' : '';
+ }
+ const compareToEnd = compareDay(day, endDay);
+ if (compareToStart === 0 && compareToEnd === 0 && allowSameDay) {
+ return 'start-end';
+ }
+ if (compareToStart === 0) {
+ return 'start';
+ }
+ if (compareToEnd === 0) {
+ return 'end';
+ }
+ if (compareToStart > 0 && compareToEnd < 0) {
+ return 'middle';
+ }
+ return '';
+ },
+ getDayType(day) {
+ const { type, minDate, maxDate, currentDate } = this.data;
+ if (compareDay(day, minDate) < 0 || compareDay(day, maxDate) > 0) {
+ return 'disabled';
+ }
+ if (type === 'single') {
+ return compareDay(day, currentDate) === 0 ? 'selected' : '';
+ }
+ if (type === 'multiple') {
+ return this.getMultipleDayType(day);
+ }
+ /* istanbul ignore else */
+ if (type === 'range') {
+ return this.getRangeDayType(day);
+ }
+ return '';
+ },
+ getBottomInfo(type) {
+ if (this.data.type === 'range') {
+ if (type === 'start') {
+ return '开始';
+ }
+ if (type === 'end') {
+ return '结束';
+ }
+ if (type === 'start-end') {
+ return '开始/结束';
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxml
new file mode 100644
index 0000000..0c73b2f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxml
@@ -0,0 +1,39 @@
+
+
+
+
+
+ {{ computed.formatMonthTitle(date) }}
+
+
+
+
+ {{ computed.getMark(date) }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxs
new file mode 100644
index 0000000..55e45a5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxs
@@ -0,0 +1,71 @@
+/* eslint-disable */
+var utils = require('../../utils.wxs');
+
+function getMark(date) {
+ return getDate(date).getMonth() + 1;
+}
+
+var ROW_HEIGHT = 64;
+
+function getDayStyle(type, index, date, rowHeight, color, firstDayOfWeek) {
+ var style = [];
+ var current = getDate(date).getDay() || 7;
+ var offset = current < firstDayOfWeek ? (7 - firstDayOfWeek + current) :
+ current === 7 && firstDayOfWeek === 0 ? 0 :
+ (current - firstDayOfWeek);
+
+ if (index === 0) {
+ style.push(['margin-left', (100 * offset) / 7 + '%']);
+ }
+
+ if (rowHeight !== ROW_HEIGHT) {
+ style.push(['height', rowHeight + 'px']);
+ }
+
+ if (color) {
+ if (
+ type === 'start' ||
+ type === 'end' ||
+ type === 'start-end' ||
+ type === 'multiple-selected' ||
+ type === 'multiple-middle'
+ ) {
+ style.push(['background', color]);
+ } else if (type === 'middle') {
+ style.push(['color', color]);
+ }
+ }
+
+ return style
+ .map(function(item) {
+ return item.join(':');
+ })
+ .join(';');
+}
+
+function formatMonthTitle(date) {
+ date = getDate(date);
+ return date.getFullYear() + '年' + (date.getMonth() + 1) + '月';
+}
+
+function getMonthStyle(visible, date, rowHeight) {
+ if (!visible) {
+ date = getDate(date);
+
+ var totalDay = utils.getMonthEndDay(
+ date.getFullYear(),
+ date.getMonth() + 1
+ );
+ var offset = getDate(date).getDay();
+ var padding = Math.ceil((totalDay + offset) / 7) * rowHeight;
+
+ return 'padding-bottom:' + padding + 'px';
+ }
+}
+
+module.exports = {
+ getMark: getMark,
+ getDayStyle: getDayStyle,
+ formatMonthTitle: formatMonthTitle,
+ getMonthStyle: getMonthStyle
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxss
new file mode 100644
index 0000000..9aee73d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/components/month/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:100%}.van-calendar__month-title{font-size:var(--calendar-month-title-font-size,14px);font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__days{display:flex;flex-wrap:wrap;position:relative;-webkit-user-select:none;user-select:none}.van-calendar__month-mark{color:var(--calendar-month-mark-color,rgba(242,243,245,.8));font-size:var(--calendar-month-mark-font-size,160px);left:50%;pointer-events:none;position:absolute;top:50%;transform:translate(-50%,-50%);z-index:0}.van-calendar__day,.van-calendar__selected-day{align-items:center;display:flex;justify-content:center;text-align:center}.van-calendar__day{font-size:var(--calendar-day-font-size,16px);height:var(--calendar-day-height,64px);position:relative;width:14.285%}.van-calendar__day--end,.van-calendar__day--multiple-middle,.van-calendar__day--multiple-selected,.van-calendar__day--start,.van-calendar__day--start-end{background-color:var(--calendar-range-edge-background-color,#ee0a24);color:var(--calendar-range-edge-color,#fff)}.van-calendar__day--start{border-radius:4px 0 0 4px}.van-calendar__day--end{border-radius:0 4px 4px 0}.van-calendar__day--multiple-selected,.van-calendar__day--start-end{border-radius:4px}.van-calendar__day--middle{color:var(--calendar-range-middle-color,#ee0a24)}.van-calendar__day--middle:after{background-color:currentColor;bottom:0;content:"";left:0;opacity:var(--calendar-range-middle-background-opacity,.1);position:absolute;right:0;top:0}.van-calendar__day--disabled{color:var(--calendar-day-disabled-color,#c8c9cc);cursor:default}.van-calendar__bottom-info,.van-calendar__top-info{font-size:var(--calendar-info-font-size,10px);left:0;line-height:var(--calendar-info-line-height,14px);position:absolute;right:0}@media (max-width:350px){.van-calendar__bottom-info,.van-calendar__top-info{font-size:9px}}.van-calendar__top-info{top:6px}.van-calendar__bottom-info{bottom:6px}.van-calendar__selected-day{background-color:var(--calendar-selected-day-background-color,#ee0a24);border-radius:4px;color:var(--calendar-selected-day-color,#fff);height:var(--calendar-selected-day-size,54px);width:var(--calendar-selected-day-size,54px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.js
new file mode 100644
index 0000000..515de42
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.js
@@ -0,0 +1,360 @@
+import { VantComponent } from '../common/component';
+import { ROW_HEIGHT, getPrevDay, getNextDay, getToday, compareDay, copyDates, calcDateNum, formatMonthTitle, compareMonth, getMonths, getDayByOffset, } from './utils';
+import Toast from '../toast/toast';
+import { requestAnimationFrame } from '../common/utils';
+const initialMinDate = getToday().getTime();
+const initialMaxDate = (() => {
+ const now = getToday();
+ return new Date(now.getFullYear(), now.getMonth() + 6, now.getDate()).getTime();
+})();
+const getTime = (date) => date instanceof Date ? date.getTime() : date;
+VantComponent({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ color: String,
+ show: {
+ type: Boolean,
+ observer(val) {
+ if (val) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ },
+ formatter: null,
+ confirmText: {
+ type: String,
+ value: '确定',
+ },
+ confirmDisabledText: {
+ type: String,
+ value: '确定',
+ },
+ rangePrompt: String,
+ showRangePrompt: {
+ type: Boolean,
+ value: true,
+ },
+ defaultDate: {
+ type: null,
+ value: getToday().getTime(),
+ observer(val) {
+ this.setData({ currentDate: val });
+ this.scrollIntoView();
+ },
+ },
+ allowSameDay: Boolean,
+ type: {
+ type: String,
+ value: 'single',
+ observer: 'reset',
+ },
+ minDate: {
+ type: Number,
+ value: initialMinDate,
+ },
+ maxDate: {
+ type: Number,
+ value: initialMaxDate,
+ },
+ position: {
+ type: String,
+ value: 'bottom',
+ },
+ rowHeight: {
+ type: null,
+ value: ROW_HEIGHT,
+ },
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ poppable: {
+ type: Boolean,
+ value: true,
+ },
+ showMark: {
+ type: Boolean,
+ value: true,
+ },
+ showTitle: {
+ type: Boolean,
+ value: true,
+ },
+ showConfirm: {
+ type: Boolean,
+ value: true,
+ },
+ showSubtitle: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ maxRange: {
+ type: null,
+ value: null,
+ },
+ minRange: {
+ type: Number,
+ value: 1,
+ },
+ firstDayOfWeek: {
+ type: Number,
+ value: 0,
+ },
+ readonly: Boolean,
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ subtitle: '',
+ currentDate: null,
+ scrollIntoView: '',
+ },
+ watch: {
+ minDate() {
+ this.initRect();
+ },
+ maxDate() {
+ this.initRect();
+ },
+ },
+ created() {
+ this.setData({
+ currentDate: this.getInitialDate(this.data.defaultDate),
+ });
+ },
+ mounted() {
+ if (this.data.show || !this.data.poppable) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ methods: {
+ reset() {
+ this.setData({ currentDate: this.getInitialDate(this.data.defaultDate) });
+ this.scrollIntoView();
+ },
+ initRect() {
+ if (this.contentObserver != null) {
+ this.contentObserver.disconnect();
+ }
+ const contentObserver = this.createIntersectionObserver({
+ thresholds: [0, 0.1, 0.9, 1],
+ observeAll: true,
+ });
+ this.contentObserver = contentObserver;
+ contentObserver.relativeTo('.van-calendar__body');
+ contentObserver.observe('.month', (res) => {
+ if (res.boundingClientRect.top <= res.relativeRect.top) {
+ // @ts-ignore
+ this.setData({ subtitle: formatMonthTitle(res.dataset.date) });
+ }
+ });
+ },
+ limitDateRange(date, minDate = null, maxDate = null) {
+ minDate = minDate || this.data.minDate;
+ maxDate = maxDate || this.data.maxDate;
+ if (compareDay(date, minDate) === -1) {
+ return minDate;
+ }
+ if (compareDay(date, maxDate) === 1) {
+ return maxDate;
+ }
+ return date;
+ },
+ getInitialDate(defaultDate = null) {
+ const { type, minDate, maxDate, allowSameDay } = this.data;
+ if (!defaultDate)
+ return [];
+ const now = getToday().getTime();
+ if (type === 'range') {
+ if (!Array.isArray(defaultDate)) {
+ defaultDate = [];
+ }
+ const [startDay, endDay] = defaultDate || [];
+ const startDate = getTime(startDay || now);
+ const start = this.limitDateRange(startDate, minDate, allowSameDay ? startDate : getPrevDay(new Date(maxDate)).getTime());
+ const date = getTime(endDay || now);
+ const end = this.limitDateRange(date, allowSameDay ? date : getNextDay(new Date(minDate)).getTime());
+ return [start, end];
+ }
+ if (type === 'multiple') {
+ if (Array.isArray(defaultDate)) {
+ return defaultDate.map((date) => this.limitDateRange(date));
+ }
+ return [this.limitDateRange(now)];
+ }
+ if (!defaultDate || Array.isArray(defaultDate)) {
+ defaultDate = now;
+ }
+ return this.limitDateRange(defaultDate);
+ },
+ scrollIntoView() {
+ requestAnimationFrame(() => {
+ const { currentDate, type, show, poppable, minDate, maxDate } = this.data;
+ if (!currentDate)
+ return;
+ // @ts-ignore
+ const targetDate = type === 'single' ? currentDate : currentDate[0];
+ const displayed = show || !poppable;
+ if (!targetDate || !displayed) {
+ return;
+ }
+ const months = getMonths(minDate, maxDate);
+ months.some((month, index) => {
+ if (compareMonth(month, targetDate) === 0) {
+ this.setData({ scrollIntoView: `month${index}` });
+ return true;
+ }
+ return false;
+ });
+ });
+ },
+ onOpen() {
+ this.$emit('open');
+ },
+ onOpened() {
+ this.$emit('opened');
+ },
+ onClose() {
+ this.$emit('close');
+ },
+ onClosed() {
+ this.$emit('closed');
+ },
+ onClickDay(event) {
+ if (this.data.readonly) {
+ return;
+ }
+ let { date } = event.detail;
+ const { type, currentDate, allowSameDay } = this.data;
+ if (type === 'range') {
+ // @ts-ignore
+ const [startDay, endDay] = currentDate;
+ if (startDay && !endDay) {
+ const compareToStart = compareDay(date, startDay);
+ if (compareToStart === 1) {
+ const { days } = this.selectComponent('.month').data;
+ days.some((day, index) => {
+ const isDisabled = day.type === 'disabled' &&
+ getTime(startDay) < getTime(day.date) &&
+ getTime(day.date) < getTime(date);
+ if (isDisabled) {
+ ({ date } = days[index - 1]);
+ }
+ return isDisabled;
+ });
+ this.select([startDay, date], true);
+ }
+ else if (compareToStart === -1) {
+ this.select([date, null]);
+ }
+ else if (allowSameDay) {
+ this.select([date, date], true);
+ }
+ }
+ else {
+ this.select([date, null]);
+ }
+ }
+ else if (type === 'multiple') {
+ let selectedIndex;
+ // @ts-ignore
+ const selected = currentDate.some((dateItem, index) => {
+ const equal = compareDay(dateItem, date) === 0;
+ if (equal) {
+ selectedIndex = index;
+ }
+ return equal;
+ });
+ if (selected) {
+ // @ts-ignore
+ const cancelDate = currentDate.splice(selectedIndex, 1);
+ this.setData({ currentDate });
+ this.unselect(cancelDate);
+ }
+ else {
+ // @ts-ignore
+ this.select([...currentDate, date]);
+ }
+ }
+ else {
+ this.select(date, true);
+ }
+ },
+ unselect(dateArray) {
+ const date = dateArray[0];
+ if (date) {
+ this.$emit('unselect', copyDates(date));
+ }
+ },
+ select(date, complete) {
+ if (complete && this.data.type === 'range') {
+ const valid = this.checkRange(date);
+ if (!valid) {
+ // auto selected to max range if showConfirm
+ if (this.data.showConfirm) {
+ this.emit([
+ date[0],
+ getDayByOffset(date[0], this.data.maxRange - 1),
+ ]);
+ }
+ else {
+ this.emit(date);
+ }
+ return;
+ }
+ }
+ this.emit(date);
+ if (complete && !this.data.showConfirm) {
+ this.onConfirm();
+ }
+ },
+ emit(date) {
+ this.setData({
+ currentDate: Array.isArray(date) ? date.map(getTime) : getTime(date),
+ });
+ this.$emit('select', copyDates(date));
+ },
+ checkRange(date) {
+ const { maxRange, rangePrompt, showRangePrompt } = this.data;
+ if (maxRange && calcDateNum(date) > maxRange) {
+ if (showRangePrompt) {
+ Toast({
+ context: this,
+ message: rangePrompt || `选择天数不能超过 ${maxRange} 天`,
+ });
+ }
+ this.$emit('over-range');
+ return false;
+ }
+ return true;
+ },
+ onConfirm() {
+ if (this.data.type === 'range' &&
+ !this.checkRange(this.data.currentDate)) {
+ return;
+ }
+ wx.nextTick(() => {
+ // @ts-ignore
+ this.$emit('confirm', copyDates(this.data.currentDate));
+ });
+ },
+ onClickSubtitle(event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.json
new file mode 100644
index 0000000..397d5ae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.json
@@ -0,0 +1,10 @@
+{
+ "component": true,
+ "usingComponents": {
+ "header": "./components/header/index",
+ "month": "./components/month/index",
+ "van-button": "../button/index",
+ "van-popup": "../popup/index",
+ "van-toast": "../toast/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxml
new file mode 100644
index 0000000..9d0fc6b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxs
new file mode 100644
index 0000000..0a56646
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxs
@@ -0,0 +1,37 @@
+/* eslint-disable */
+var utils = require('./utils.wxs');
+
+function getMonths(minDate, maxDate) {
+ var months = [];
+ var cursor = getDate(minDate);
+
+ cursor.setDate(1);
+
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (utils.compareMonth(cursor, getDate(maxDate)) !== 1);
+
+ return months;
+}
+
+function getButtonDisabled(type, currentDate, minRange) {
+ if (currentDate == null) {
+ return true;
+ }
+
+ if (type === 'range') {
+ return !currentDate[0] || !currentDate[1];
+ }
+
+ if (type === 'multiple') {
+ return currentDate.length < minRange;
+ }
+
+ return !currentDate;
+}
+
+module.exports = {
+ getMonths: getMonths,
+ getButtonDisabled: getButtonDisabled
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxss
new file mode 100644
index 0000000..a1f1cf0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:var(--calendar-height,100%)}.van-calendar__close-icon{top:11px}.van-calendar__popup--bottom,.van-calendar__popup--top{height:var(--calendar-popup-height,90%)}.van-calendar__popup--left,.van-calendar__popup--right{height:100%}.van-calendar__body{-webkit-overflow-scrolling:touch;flex:1;overflow:auto}.van-calendar__footer{flex-shrink:0;padding:0 var(--padding-md,16px)}.van-calendar__footer--safe-area-inset-bottom{padding-bottom:env(safe-area-inset-bottom)}.van-calendar__footer+.van-calendar__footer,.van-calendar__footer:empty{display:none}.van-calendar__footer:empty+.van-calendar__footer{display:block!important}.van-calendar__confirm{height:var(--calendar-confirm-button-height,36px)!important;line-height:var(--calendar-confirm-button-line-height,34px)!important;margin:var(--calendar-confirm-button-margin,7px 0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.d.ts
new file mode 100644
index 0000000..889e6e7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.d.ts
@@ -0,0 +1,12 @@
+export declare const ROW_HEIGHT = 64;
+export declare function formatMonthTitle(date: Date): string;
+export declare function compareMonth(date1: Date | number, date2: Date | number): 0 | 1 | -1;
+export declare function compareDay(day1: Date | number, day2: Date | number): 0 | 1 | -1;
+export declare function getDayByOffset(date: Date, offset: number): Date;
+export declare function getPrevDay(date: Date): Date;
+export declare function getNextDay(date: Date): Date;
+export declare function getToday(): Date;
+export declare function calcDateNum(date: [Date, Date]): number;
+export declare function copyDates(dates: Date | Date[]): Date | Date[];
+export declare function getMonthEndDay(year: number, month: number): number;
+export declare function getMonths(minDate: number, maxDate: number): number[];
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.js
new file mode 100644
index 0000000..83d6971
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.js
@@ -0,0 +1,83 @@
+export const ROW_HEIGHT = 64;
+export function formatMonthTitle(date) {
+ if (!(date instanceof Date)) {
+ date = new Date(date);
+ }
+ return `${date.getFullYear()}年${date.getMonth() + 1}月`;
+}
+export function compareMonth(date1, date2) {
+ if (!(date1 instanceof Date)) {
+ date1 = new Date(date1);
+ }
+ if (!(date2 instanceof Date)) {
+ date2 = new Date(date2);
+ }
+ const year1 = date1.getFullYear();
+ const year2 = date2.getFullYear();
+ const month1 = date1.getMonth();
+ const month2 = date2.getMonth();
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+ return year1 > year2 ? 1 : -1;
+}
+export function compareDay(day1, day2) {
+ if (!(day1 instanceof Date)) {
+ day1 = new Date(day1);
+ }
+ if (!(day2 instanceof Date)) {
+ day2 = new Date(day2);
+ }
+ const compareMonthResult = compareMonth(day1, day2);
+ if (compareMonthResult === 0) {
+ const date1 = day1.getDate();
+ const date2 = day2.getDate();
+ return date1 === date2 ? 0 : date1 > date2 ? 1 : -1;
+ }
+ return compareMonthResult;
+}
+export function getDayByOffset(date, offset) {
+ date = new Date(date);
+ date.setDate(date.getDate() + offset);
+ return date;
+}
+export function getPrevDay(date) {
+ return getDayByOffset(date, -1);
+}
+export function getNextDay(date) {
+ return getDayByOffset(date, 1);
+}
+export function getToday() {
+ const today = new Date();
+ today.setHours(0, 0, 0, 0);
+ return today;
+}
+export function calcDateNum(date) {
+ const day1 = new Date(date[0]).getTime();
+ const day2 = new Date(date[1]).getTime();
+ return (day2 - day1) / (1000 * 60 * 60 * 24) + 1;
+}
+export function copyDates(dates) {
+ if (Array.isArray(dates)) {
+ return dates.map((date) => {
+ if (date === null) {
+ return date;
+ }
+ return new Date(date);
+ });
+ }
+ return new Date(dates);
+}
+export function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+export function getMonths(minDate, maxDate) {
+ const months = [];
+ const cursor = new Date(minDate);
+ cursor.setDate(1);
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (compareMonth(cursor, maxDate) !== 1);
+ return months;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.wxs
new file mode 100644
index 0000000..e57f6b3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/calendar/utils.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+function getMonthEndDay(year, month) {
+ return 32 - getDate(year, month - 1, 32).getDate();
+}
+
+function compareMonth(date1, date2) {
+ date1 = getDate(date1);
+ date2 = getDate(date2);
+
+ var year1 = date1.getFullYear();
+ var year2 = date2.getFullYear();
+ var month1 = date1.getMonth();
+ var month2 = date2.getMonth();
+
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+
+ return year1 > year2 ? 1 : -1;
+}
+
+module.exports = {
+ getMonthEndDay: getMonthEndDay,
+ compareMonth: compareMonth
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.js
new file mode 100644
index 0000000..5bbd212
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.js
@@ -0,0 +1,49 @@
+import { link } from '../mixins/link';
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: [
+ 'num-class',
+ 'desc-class',
+ 'thumb-class',
+ 'title-class',
+ 'price-class',
+ 'origin-price-class',
+ ],
+ mixins: [link],
+ props: {
+ tag: String,
+ num: String,
+ desc: String,
+ thumb: String,
+ title: String,
+ price: {
+ type: String,
+ observer: 'updatePrice',
+ },
+ centered: Boolean,
+ lazyLoad: Boolean,
+ thumbLink: String,
+ originPrice: String,
+ thumbMode: {
+ type: String,
+ value: 'aspectFit',
+ },
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ },
+ methods: {
+ updatePrice() {
+ const { price } = this.data;
+ const priceArr = price.toString().split('.');
+ this.setData({
+ integerStr: priceArr[0],
+ decimalStr: priceArr[1] ? `.${priceArr[1]}` : '',
+ });
+ },
+ onClickThumb() {
+ this.jumpLink('thumbLink');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.json
new file mode 100644
index 0000000..e917407
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-tag": "../tag/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxml
new file mode 100644
index 0000000..62173e4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxss
new file mode 100644
index 0000000..0f4d7c5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/card/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-card{background-color:var(--card-background-color,#fafafa);box-sizing:border-box;color:var(--card-text-color,#323233);font-size:var(--card-font-size,12px);padding:var(--card-padding,8px 16px);position:relative}.van-card__header{display:flex}.van-card__header--center{align-items:center;justify-content:center}.van-card__thumb{flex:none;height:var(--card-thumb-size,88px);margin-right:var(--padding-xs,8px);position:relative;width:var(--card-thumb-size,88px)}.van-card__thumb:empty{display:none}.van-card__img{border-radius:8px;height:100%;width:100%}.van-card__content{display:flex;flex:1;flex-direction:column;justify-content:space-between;min-height:var(--card-thumb-size,88px);min-width:0;position:relative}.van-card__content--center{justify-content:center}.van-card__desc,.van-card__title{word-wrap:break-word}.van-card__title{font-weight:700;line-height:var(--card-title-line-height,16px)}.van-card__desc{color:var(--card-desc-color,#646566);line-height:var(--card-desc-line-height,20px)}.van-card__bottom{line-height:20px}.van-card__price{color:var(--card-price-color,#ee0a24);display:inline-block;font-size:var(--card-price-font-size,12px);font-weight:700}.van-card__price-integer{font-size:var(--card-price-integer-font-size,16px)}.van-card__price-decimal,.van-card__price-integer{font-family:var(--card-price-font-family,Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif)}.van-card__origin-price{color:var(--card-origin-price-color,#646566);display:inline-block;font-size:var(--card-origin-price-font-size,10px);margin-left:5px;text-decoration:line-through}.van-card__num{float:right}.van-card__tag{left:0;position:absolute!important;top:2px}.van-card__footer{flex:none;text-align:right;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.js
new file mode 100644
index 0000000..03680f5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.js
@@ -0,0 +1,209 @@
+import { VantComponent } from '../common/component';
+var FieldName;
+(function (FieldName) {
+ FieldName["TEXT"] = "text";
+ FieldName["VALUE"] = "value";
+ FieldName["CHILDREN"] = "children";
+})(FieldName || (FieldName = {}));
+const defaultFieldNames = {
+ text: FieldName.TEXT,
+ value: FieldName.VALUE,
+ children: FieldName.CHILDREN,
+};
+VantComponent({
+ props: {
+ title: String,
+ value: {
+ type: String,
+ },
+ placeholder: {
+ type: String,
+ value: '请选择',
+ },
+ activeColor: {
+ type: String,
+ value: '#1989fa',
+ },
+ options: {
+ type: Array,
+ value: [],
+ },
+ swipeable: {
+ type: Boolean,
+ value: false,
+ },
+ closeable: {
+ type: Boolean,
+ value: true,
+ },
+ showHeader: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ fieldNames: {
+ type: Object,
+ value: defaultFieldNames,
+ observer: 'updateFieldNames',
+ },
+ useTitleSlot: Boolean,
+ },
+ data: {
+ tabs: [],
+ activeTab: 0,
+ textKey: FieldName.TEXT,
+ valueKey: FieldName.VALUE,
+ childrenKey: FieldName.CHILDREN,
+ innerValue: '',
+ },
+ watch: {
+ options() {
+ this.updateTabs();
+ },
+ value(newVal) {
+ this.updateValue(newVal);
+ },
+ },
+ created() {
+ this.updateTabs();
+ },
+ methods: {
+ updateValue(val) {
+ if (val !== undefined) {
+ const values = this.data.tabs.map((tab) => tab.selected && tab.selected[this.data.valueKey]);
+ if (values.indexOf(val) > -1) {
+ return;
+ }
+ }
+ this.innerValue = val;
+ this.updateTabs();
+ },
+ updateFieldNames() {
+ const { text = 'text', value = 'value', children = 'children', } = this.data.fieldNames || defaultFieldNames;
+ this.setData({
+ textKey: text,
+ valueKey: value,
+ childrenKey: children,
+ });
+ },
+ getSelectedOptionsByValue(options, value) {
+ for (let i = 0; i < options.length; i++) {
+ const option = options[i];
+ if (option[this.data.valueKey] === value) {
+ return [option];
+ }
+ if (option[this.data.childrenKey]) {
+ const selectedOptions = this.getSelectedOptionsByValue(option[this.data.childrenKey], value);
+ if (selectedOptions) {
+ return [option, ...selectedOptions];
+ }
+ }
+ }
+ },
+ updateTabs() {
+ const { options } = this.data;
+ const { innerValue } = this;
+ if (!options.length) {
+ return;
+ }
+ if (innerValue !== undefined) {
+ const selectedOptions = this.getSelectedOptionsByValue(options, innerValue);
+ if (selectedOptions) {
+ let optionsCursor = options;
+ const tabs = selectedOptions.map((option) => {
+ const tab = {
+ options: optionsCursor,
+ selected: option,
+ };
+ const next = optionsCursor.find((item) => item[this.data.valueKey] === option[this.data.valueKey]);
+ if (next) {
+ optionsCursor = next[this.data.childrenKey];
+ }
+ return tab;
+ });
+ if (optionsCursor) {
+ tabs.push({
+ options: optionsCursor,
+ selected: null,
+ });
+ }
+ this.setData({
+ tabs,
+ });
+ wx.nextTick(() => {
+ this.setData({
+ activeTab: tabs.length - 1,
+ });
+ });
+ return;
+ }
+ }
+ this.setData({
+ tabs: [
+ {
+ options,
+ selected: null,
+ },
+ ],
+ });
+ },
+ onClose() {
+ this.$emit('close');
+ },
+ onClickTab(e) {
+ const { index: tabIndex, title } = e.detail;
+ this.$emit('click-tab', { title, tabIndex });
+ this.setData({
+ activeTab: tabIndex,
+ });
+ },
+ // 选中
+ onSelect(e) {
+ const { option, tabIndex } = e.currentTarget.dataset;
+ if (option && option.disabled) {
+ return;
+ }
+ const { valueKey, childrenKey } = this.data;
+ let { tabs } = this.data;
+ tabs[tabIndex].selected = option;
+ if (tabs.length > tabIndex + 1) {
+ tabs = tabs.slice(0, tabIndex + 1);
+ }
+ if (option[childrenKey]) {
+ const nextTab = {
+ options: option[childrenKey],
+ selected: null,
+ };
+ if (tabs[tabIndex + 1]) {
+ tabs[tabIndex + 1] = nextTab;
+ }
+ else {
+ tabs.push(nextTab);
+ }
+ wx.nextTick(() => {
+ this.setData({
+ activeTab: tabIndex + 1,
+ });
+ });
+ }
+ this.setData({
+ tabs,
+ });
+ const selectedOptions = tabs.map((tab) => tab.selected).filter(Boolean);
+ const value = option[valueKey];
+ const params = {
+ value,
+ tabIndex,
+ selectedOptions,
+ };
+ this.innerValue = value;
+ this.$emit('change', params);
+ if (!option[childrenKey]) {
+ this.$emit('finish', params);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.json
new file mode 100644
index 0000000..d0f75eb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-tab": "../tab/index",
+ "van-tabs": "../tabs/index"
+ }
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxml
new file mode 100644
index 0000000..9417234
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ option[textKey] }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxs
new file mode 100644
index 0000000..b1aab58
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxs
@@ -0,0 +1,24 @@
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function isSelected(tab, valueKey, option) {
+ return tab.selected && tab.selected[valueKey] === option[valueKey]
+}
+
+function optionClass(tab, valueKey, option) {
+ return utils.bem('cascader__option', { selected: isSelected(tab, valueKey, option), disabled: option.disabled })
+}
+
+function optionStyle(data) {
+ var color = data.option.color || (isSelected(data.tab, data.valueKey, data.option) ? data.activeColor : undefined);
+ return style({
+ color
+ });
+}
+
+
+module.exports = {
+ isSelected: isSelected,
+ optionClass: optionClass,
+ optionStyle: optionStyle,
+};
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxss
new file mode 100644
index 0000000..7062486
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cascader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cascader__header{align-items:center;display:flex;height:48px;justify-content:space-between;padding:0 16px}.van-cascader__title{font-size:16px;font-weight:600;line-height:20px}.van-cascader__close-icon{color:#c8c9cc;font-size:22px;height:22px}.van-cascader__tabs-wrap{height:48px!important;padding:0 8px}.van-cascader__tab{color:#323233!important;flex:none!important;font-weight:600!important;padding:0 8px!important}.van-cascader__tab--unselected{color:#969799!important;font-weight:400!important}.van-cascader__option{align-items:center;cursor:pointer;display:flex;font-size:14px;justify-content:space-between;line-height:20px;padding:10px 16px}.van-cascader__option:active{background-color:#f2f3f5}.van-cascader__option--selected{color:#1989fa;font-weight:600}.van-cascader__option--disabled{color:#c8c9cc;cursor:not-allowed}.van-cascader__option--disabled:active{background-color:initial}.van-cascader__options{-webkit-overflow-scrolling:touch;box-sizing:border-box;height:384px;overflow-y:auto;padding-top:6px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.js
new file mode 100644
index 0000000..170760f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.js
@@ -0,0 +1,11 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ title: String,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ inset: Boolean,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxml
new file mode 100644
index 0000000..311e064
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxml
@@ -0,0 +1,11 @@
+
+
+
+ {{ title }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxss
new file mode 100644
index 0000000..08b252f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell-group--inset{border-radius:var(--cell-group-inset-border-radius,8px);margin:var(--cell-group-inset-padding,0 16px);overflow:hidden}.van-cell-group__title{color:var(--cell-group-title-color,#969799);font-size:var(--cell-group-title-font-size,14px);line-height:var(--cell-group-title-line-height,16px);padding:var(--cell-group-title-padding,16px 16px 8px)}.van-cell-group__title--inset{padding:var(--cell-group-inset-title-padding,16px 16px 8px 32px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.js
new file mode 100644
index 0000000..35548b9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.js
@@ -0,0 +1,38 @@
+import { link } from '../mixins/link';
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: [
+ 'title-class',
+ 'label-class',
+ 'value-class',
+ 'right-icon-class',
+ 'hover-class',
+ ],
+ mixins: [link],
+ props: {
+ title: null,
+ value: null,
+ icon: String,
+ size: String,
+ label: String,
+ center: Boolean,
+ isLink: Boolean,
+ required: Boolean,
+ clickable: Boolean,
+ titleWidth: String,
+ customStyle: String,
+ arrowDirection: String,
+ useLabelSlot: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ titleStyle: String,
+ },
+ methods: {
+ onClick(event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxml
new file mode 100644
index 0000000..8387c3c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+ {{ title }}
+
+
+
+
+ {{ label }}
+
+
+
+
+ {{ value }}
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxs
new file mode 100644
index 0000000..e3500c4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function titleStyle(data) {
+ return style([
+ {
+ 'max-width': addUnit(data.titleWidth),
+ 'min-width': addUnit(data.titleWidth),
+ },
+ data.titleStyle,
+ ]);
+}
+
+module.exports = {
+ titleStyle: titleStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxss
new file mode 100644
index 0000000..1802f8e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell{background-color:var(--cell-background-color,#fff);box-sizing:border-box;color:var(--cell-text-color,#323233);display:flex;font-size:var(--cell-font-size,14px);line-height:var(--cell-line-height,24px);padding:var(--cell-vertical-padding,10px) var(--cell-horizontal-padding,16px);position:relative;width:100%}.van-cell:after{border-bottom:1px solid #ebedf0;bottom:0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:16px;transform:scaleY(.5);transform-origin:center}.van-cell--borderless:after{display:none}.van-cell-group{background-color:var(--cell-background-color,#fff)}.van-cell__label{color:var(--cell-label-color,#969799);font-size:var(--cell-label-font-size,12px);line-height:var(--cell-label-line-height,18px);margin-top:var(--cell-label-margin-top,3px)}.van-cell__value{color:var(--cell-value-color,#969799);overflow:hidden;text-align:right;vertical-align:middle}.van-cell__title,.van-cell__value{flex:1}.van-cell__title:empty,.van-cell__value:empty{display:none}.van-cell__left-icon-wrap,.van-cell__right-icon-wrap{align-items:center;display:flex;font-size:var(--cell-icon-size,16px);height:var(--cell-line-height,24px)}.van-cell__left-icon-wrap{margin-right:var(--padding-base,4px)}.van-cell__right-icon-wrap{color:var(--cell-right-icon-color,#969799);margin-left:var(--padding-base,4px)}.van-cell__left-icon{vertical-align:middle}.van-cell__left-icon,.van-cell__right-icon{line-height:var(--cell-line-height,24px)}.van-cell--clickable.van-cell--hover{background-color:var(--cell-active-color,#f2f3f5)}.van-cell--required{overflow:visible}.van-cell--required:before{color:var(--cell-required-color,#ee0a24);content:"*";font-size:var(--cell-font-size,14px);left:var(--padding-xs,8px);position:absolute}.van-cell--center{align-items:center}.van-cell--large{padding-bottom:var(--cell-large-vertical-padding,12px);padding-top:var(--cell-large-vertical-padding,12px)}.van-cell--large .van-cell__title{font-size:var(--cell-large-title-font-size,16px)}.van-cell--large .van-cell__value{font-size:var(--cell-large-value-font-size,16px)}.van-cell--large .van-cell__label{font-size:var(--cell-large-label-font-size,14px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.js
new file mode 100644
index 0000000..c47d97d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.js
@@ -0,0 +1,36 @@
+import { useChildren } from '../common/relation';
+import { VantComponent } from '../common/component';
+VantComponent({
+ field: true,
+ relation: useChildren('checkbox', function (target) {
+ this.updateChild(target);
+ }),
+ props: {
+ max: Number,
+ value: {
+ type: Array,
+ observer: 'updateChildren',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ value: 'vertical',
+ },
+ },
+ methods: {
+ updateChildren() {
+ this.children.forEach((child) => this.updateChild(child));
+ },
+ updateChild(child) {
+ const { value, disabled, direction } = this.data;
+ child.setData({
+ value: value.indexOf(child.data.name) !== -1,
+ parentDisabled: disabled,
+ direction,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxml
new file mode 100644
index 0000000..638bf9d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxss
new file mode 100644
index 0000000..c5666d7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.js
new file mode 100644
index 0000000..e3b78ab
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.js
@@ -0,0 +1,77 @@
+import { useParent } from '../common/relation';
+import { VantComponent } from '../common/component';
+function emit(target, value) {
+ target.$emit('input', value);
+ target.$emit('change', value);
+}
+VantComponent({
+ field: true,
+ relation: useParent('checkbox-group'),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ value: Boolean,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ parentDisabled: false,
+ direction: 'vertical',
+ },
+ methods: {
+ emitChange(value) {
+ if (this.parent) {
+ this.setParentValue(this.parent, value);
+ }
+ else {
+ emit(this, value);
+ }
+ },
+ toggle() {
+ const { parentDisabled, disabled, value } = this.data;
+ if (!disabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ onClickLabel() {
+ const { labelDisabled, parentDisabled, disabled, value } = this.data;
+ if (!disabled && !labelDisabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ setParentValue(parent, value) {
+ const parentValue = parent.data.value.slice();
+ const { name } = this.data;
+ const { max } = parent.data;
+ if (value) {
+ if (max && parentValue.length >= max) {
+ return;
+ }
+ if (parentValue.indexOf(name) === -1) {
+ parentValue.push(name);
+ emit(parent, parentValue);
+ }
+ }
+ else {
+ const index = parentValue.indexOf(name);
+ if (index !== -1) {
+ parentValue.splice(index, 1);
+ emit(parent, parentValue);
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxml
new file mode 100644
index 0000000..39a7bb0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxml
@@ -0,0 +1,31 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxs
new file mode 100644
index 0000000..eb9c772
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxs
@@ -0,0 +1,20 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(checkedColor, value, disabled, parentDisabled, iconSize) {
+ var styles = {
+ 'font-size': addUnit(iconSize),
+ };
+
+ if (checkedColor && value && !disabled && !parentDisabled) {
+ styles['border-color'] = checkedColor;
+ styles['background-color'] = checkedColor;
+ }
+
+ return style(styles);
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxss
new file mode 100644
index 0000000..da2272a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/checkbox/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-checkbox--horizontal{margin-right:12px}.van-checkbox__icon-wrap,.van-checkbox__label{line-height:var(--checkbox-size,20px)}.van-checkbox__icon-wrap{flex:none}.van-checkbox__icon{align-items:center;border:1px solid var(--checkbox-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--checkbox-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--checkbox-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-checkbox__icon--round{border-radius:100%}.van-checkbox__icon--checked{background-color:var(--checkbox-checked-icon-color,#1989fa);border-color:var(--checkbox-checked-icon-color,#1989fa);color:#fff}.van-checkbox__icon--disabled{background-color:var(--checkbox-disabled-background-color,#ebedf0);border-color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__icon--disabled.van-checkbox__icon--checked{color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__label{word-wrap:break-word;color:var(--checkbox-label-color,#323233);padding-left:var(--checkbox-label-margin,10px)}.van-checkbox__label--left{float:left;margin:0 var(--checkbox-label-margin,10px) 0 0}.van-checkbox__label--disabled{color:var(--checkbox-disabled-label-color,#c8c9cc)}.van-checkbox__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.d.ts
new file mode 100644
index 0000000..8a0b71e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.d.ts
@@ -0,0 +1,4 @@
+///
+type CanvasContext = WechatMiniprogram.CanvasContext;
+export declare function adaptor(ctx: CanvasContext & Record): CanvasContext;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.js
new file mode 100644
index 0000000..3ade4cd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/canvas.js
@@ -0,0 +1,43 @@
+export function adaptor(ctx) {
+ // @ts-ignore
+ return Object.assign(ctx, {
+ setStrokeStyle(val) {
+ ctx.strokeStyle = val;
+ },
+ setLineWidth(val) {
+ ctx.lineWidth = val;
+ },
+ setLineCap(val) {
+ ctx.lineCap = val;
+ },
+ setFillStyle(val) {
+ ctx.fillStyle = val;
+ },
+ setFontSize(val) {
+ ctx.font = String(val);
+ },
+ setGlobalAlpha(val) {
+ ctx.globalAlpha = val;
+ },
+ setLineJoin(val) {
+ ctx.lineJoin = val;
+ },
+ setTextAlign(val) {
+ ctx.textAlign = val;
+ },
+ setMiterLimit(val) {
+ ctx.miterLimit = val;
+ },
+ setShadow(offsetX, offsetY, blur, color) {
+ ctx.shadowOffsetX = offsetX;
+ ctx.shadowOffsetY = offsetY;
+ ctx.shadowBlur = blur;
+ ctx.shadowColor = color;
+ },
+ setTextBaseline(val) {
+ ctx.textBaseline = val;
+ },
+ createCircularGradient() { },
+ draw() { },
+ });
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.js
new file mode 100644
index 0000000..0b60888
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.js
@@ -0,0 +1,197 @@
+import { BLUE, WHITE } from '../common/color';
+import { VantComponent } from '../common/component';
+import { getSystemInfoSync } from '../common/utils';
+import { isObj } from '../common/validator';
+import { canIUseCanvas2d } from '../common/version';
+import { adaptor } from './canvas';
+function format(rate) {
+ return Math.min(Math.max(rate, 0), 100);
+}
+const PERIMETER = 2 * Math.PI;
+const BEGIN_ANGLE = -Math.PI / 2;
+const STEP = 1;
+VantComponent({
+ props: {
+ text: String,
+ lineCap: {
+ type: String,
+ value: 'round',
+ },
+ value: {
+ type: Number,
+ value: 0,
+ observer: 'reRender',
+ },
+ speed: {
+ type: Number,
+ value: 50,
+ },
+ size: {
+ type: Number,
+ value: 100,
+ observer() {
+ this.drawCircle(this.currentValue);
+ },
+ },
+ fill: String,
+ layerColor: {
+ type: String,
+ value: WHITE,
+ },
+ color: {
+ type: null,
+ value: BLUE,
+ observer() {
+ this.setHoverColor().then(() => {
+ this.drawCircle(this.currentValue);
+ });
+ },
+ },
+ type: {
+ type: String,
+ value: '',
+ },
+ strokeWidth: {
+ type: Number,
+ value: 4,
+ },
+ clockwise: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ hoverColor: BLUE,
+ },
+ methods: {
+ getContext() {
+ const { type, size } = this.data;
+ if (type === '' || !canIUseCanvas2d()) {
+ const ctx = wx.createCanvasContext('van-circle', this);
+ return Promise.resolve(ctx);
+ }
+ const dpr = getSystemInfoSync().pixelRatio;
+ return new Promise((resolve) => {
+ wx.createSelectorQuery()
+ .in(this)
+ .select('#van-circle')
+ .node()
+ .exec((res) => {
+ const canvas = res[0].node;
+ const ctx = canvas.getContext(type);
+ if (!this.inited) {
+ this.inited = true;
+ canvas.width = size * dpr;
+ canvas.height = size * dpr;
+ ctx.scale(dpr, dpr);
+ }
+ resolve(adaptor(ctx));
+ });
+ });
+ },
+ setHoverColor() {
+ const { color, size } = this.data;
+ if (isObj(color)) {
+ return this.getContext().then((context) => {
+ if (!context)
+ return;
+ const LinearColor = context.createLinearGradient(size, 0, 0, 0);
+ Object.keys(color)
+ .sort((a, b) => parseFloat(a) - parseFloat(b))
+ .map((key) => LinearColor.addColorStop(parseFloat(key) / 100, color[key]));
+ this.hoverColor = LinearColor;
+ });
+ }
+ this.hoverColor = color;
+ return Promise.resolve();
+ },
+ presetCanvas(context, strokeStyle, beginAngle, endAngle, fill) {
+ const { strokeWidth, lineCap, clockwise, size } = this.data;
+ const position = size / 2;
+ const radius = position - strokeWidth / 2;
+ context.setStrokeStyle(strokeStyle);
+ context.setLineWidth(strokeWidth);
+ context.setLineCap(lineCap);
+ context.beginPath();
+ context.arc(position, position, radius, beginAngle, endAngle, !clockwise);
+ context.stroke();
+ if (fill) {
+ context.setFillStyle(fill);
+ context.fill();
+ }
+ },
+ renderLayerCircle(context) {
+ const { layerColor, fill } = this.data;
+ this.presetCanvas(context, layerColor, 0, PERIMETER, fill);
+ },
+ renderHoverCircle(context, formatValue) {
+ const { clockwise } = this.data;
+ // 结束角度
+ const progress = PERIMETER * (formatValue / 100);
+ const endAngle = clockwise
+ ? BEGIN_ANGLE + progress
+ : 3 * Math.PI - (BEGIN_ANGLE + progress);
+ this.presetCanvas(context, this.hoverColor, BEGIN_ANGLE, endAngle);
+ },
+ drawCircle(currentValue) {
+ const { size } = this.data;
+ this.getContext().then((context) => {
+ if (!context)
+ return;
+ context.clearRect(0, 0, size, size);
+ this.renderLayerCircle(context);
+ const formatValue = format(currentValue);
+ if (formatValue !== 0) {
+ this.renderHoverCircle(context, formatValue);
+ }
+ context.draw();
+ });
+ },
+ reRender() {
+ // tofector 动画暂时没有想到好的解决方案
+ const { value, speed } = this.data;
+ if (speed <= 0 || speed > 1000) {
+ this.drawCircle(value);
+ return;
+ }
+ this.clearMockInterval();
+ this.currentValue = this.currentValue || 0;
+ const run = () => {
+ this.interval = setTimeout(() => {
+ if (this.currentValue !== value) {
+ if (Math.abs(this.currentValue - value) < STEP) {
+ this.currentValue = value;
+ }
+ else if (this.currentValue < value) {
+ this.currentValue += STEP;
+ }
+ else {
+ this.currentValue -= STEP;
+ }
+ this.drawCircle(this.currentValue);
+ run();
+ }
+ else {
+ this.clearMockInterval();
+ }
+ }, 1000 / speed);
+ };
+ run();
+ },
+ clearMockInterval() {
+ if (this.interval) {
+ clearTimeout(this.interval);
+ this.interval = null;
+ }
+ },
+ },
+ mounted() {
+ this.currentValue = this.data.value;
+ this.setHoverColor().then(() => {
+ this.drawCircle(this.currentValue);
+ });
+ },
+ destroyed() {
+ this.clearMockInterval();
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxml
new file mode 100644
index 0000000..52bc59f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxss
new file mode 100644
index 0000000..2200751
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/circle/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-circle{display:inline-block;position:relative;text-align:center}.van-circle__text{color:var(--circle-text-color,#323233);left:0;position:absolute;top:50%;transform:translateY(-50%);width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.js
new file mode 100644
index 0000000..02bb78d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.js
@@ -0,0 +1,9 @@
+import { useParent } from '../common/relation';
+import { VantComponent } from '../common/component';
+VantComponent({
+ relation: useParent('row'),
+ props: {
+ span: Number,
+ offset: Number,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxml
new file mode 100644
index 0000000..975348b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxs
new file mode 100644
index 0000000..507c1cb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'padding-right': addUnit(data.gutter / 2),
+ 'padding-left': addUnit(data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxss
new file mode 100644
index 0000000..2fa265e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/col/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-col{box-sizing:border-box;float:left}.van-col--1{width:4.16666667%}.van-col--offset-1{margin-left:4.16666667%}.van-col--2{width:8.33333333%}.van-col--offset-2{margin-left:8.33333333%}.van-col--3{width:12.5%}.van-col--offset-3{margin-left:12.5%}.van-col--4{width:16.66666667%}.van-col--offset-4{margin-left:16.66666667%}.van-col--5{width:20.83333333%}.van-col--offset-5{margin-left:20.83333333%}.van-col--6{width:25%}.van-col--offset-6{margin-left:25%}.van-col--7{width:29.16666667%}.van-col--offset-7{margin-left:29.16666667%}.van-col--8{width:33.33333333%}.van-col--offset-8{margin-left:33.33333333%}.van-col--9{width:37.5%}.van-col--offset-9{margin-left:37.5%}.van-col--10{width:41.66666667%}.van-col--offset-10{margin-left:41.66666667%}.van-col--11{width:45.83333333%}.van-col--offset-11{margin-left:45.83333333%}.van-col--12{width:50%}.van-col--offset-12{margin-left:50%}.van-col--13{width:54.16666667%}.van-col--offset-13{margin-left:54.16666667%}.van-col--14{width:58.33333333%}.van-col--offset-14{margin-left:58.33333333%}.van-col--15{width:62.5%}.van-col--offset-15{margin-left:62.5%}.van-col--16{width:66.66666667%}.van-col--offset-16{margin-left:66.66666667%}.van-col--17{width:70.83333333%}.van-col--offset-17{margin-left:70.83333333%}.van-col--18{width:75%}.van-col--offset-18{margin-left:75%}.van-col--19{width:79.16666667%}.van-col--offset-19{margin-left:79.16666667%}.van-col--20{width:83.33333333%}.van-col--offset-20{margin-left:83.33333333%}.van-col--21{width:87.5%}.van-col--offset-21{margin-left:87.5%}.van-col--22{width:91.66666667%}.van-col--offset-22{margin-left:91.66666667%}.van-col--23{width:95.83333333%}.van-col--offset-23{margin-left:95.83333333%}.van-col--24{width:100%}.van-col--offset-24{margin-left:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.d.ts
new file mode 100644
index 0000000..32157b6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.d.ts
@@ -0,0 +1,2 @@
+///
+export declare function setContentAnimate(context: WechatMiniprogram.Component.TrivialInstance, expanded: boolean, mounted: boolean): void;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.js
new file mode 100644
index 0000000..f966ac5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/animate.js
@@ -0,0 +1,39 @@
+import { getRect } from '../common/utils';
+function useAnimation(context, expanded, mounted, height) {
+ const animation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'ease-in-out',
+ });
+ if (expanded) {
+ if (height === 0) {
+ animation.height('auto').top(1).step();
+ }
+ else {
+ animation
+ .height(height)
+ .top(1)
+ .step({
+ duration: mounted ? 300 : 1,
+ })
+ .height('auto')
+ .step();
+ }
+ context.setData({
+ animation: animation.export(),
+ });
+ return;
+ }
+ animation.height(height).top(0).step({ duration: 1 }).height(0).step({
+ duration: 300,
+ });
+ context.setData({
+ animation: animation.export(),
+ });
+}
+export function setContentAnimate(context, expanded, mounted) {
+ getRect(context, '.van-collapse-item__content')
+ .then((rect) => rect.height)
+ .then((height) => {
+ useAnimation(context, expanded, mounted, height);
+ });
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.js
new file mode 100644
index 0000000..5bac368
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.js
@@ -0,0 +1,60 @@
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+import { setContentAnimate } from './animate';
+VantComponent({
+ classes: ['title-class', 'content-class'],
+ relation: useParent('collapse'),
+ props: {
+ size: String,
+ name: null,
+ title: null,
+ value: null,
+ icon: String,
+ label: String,
+ disabled: Boolean,
+ clickable: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ isLink: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ expanded: false,
+ },
+ mounted() {
+ this.updateExpanded();
+ this.mounted = true;
+ },
+ methods: {
+ updateExpanded() {
+ if (!this.parent) {
+ return;
+ }
+ const { value, accordion } = this.parent.data;
+ const { children = [] } = this.parent;
+ const { name } = this.data;
+ const index = children.indexOf(this);
+ const currentName = name == null ? index : name;
+ const expanded = accordion
+ ? value === currentName
+ : (value || []).some((name) => name === currentName);
+ if (expanded !== this.data.expanded) {
+ setContentAnimate(this, expanded, this.mounted);
+ }
+ this.setData({ index, expanded });
+ },
+ onClick() {
+ if (this.data.disabled) {
+ return;
+ }
+ const { name, expanded } = this.data;
+ const index = this.parent.children.indexOf(this);
+ const currentName = name == null ? index : name;
+ this.parent.switch(currentName, !expanded);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxml
new file mode 100644
index 0000000..f11d0d4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxml
@@ -0,0 +1,45 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxss
new file mode 100644
index 0000000..4a65b5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-collapse-item__title .van-cell__right-icon{transform:rotate(90deg);transition:transform var(--collapse-item-transition-duration,.3s)}.van-collapse-item__title--expanded .van-cell__right-icon{transform:rotate(-90deg)}.van-collapse-item__title--disabled .van-cell,.van-collapse-item__title--disabled .van-cell__right-icon{color:var(--collapse-item-title-disabled-color,#c8c9cc)!important}.van-collapse-item__title--disabled .van-cell--hover{background-color:#fff!important}.van-collapse-item__wrapper{overflow:hidden}.van-collapse-item__content{background-color:var(--collapse-item-content-background-color,#fff);color:var(--collapse-item-content-text-color,#969799);font-size:var(--collapse-item-content-font-size,13px);line-height:var(--collapse-item-content-line-height,1.5);padding:var(--collapse-item-content-padding,15px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.js
new file mode 100644
index 0000000..3616087
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.js
@@ -0,0 +1,46 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ relation: useChildren('collapse-item'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateExpanded',
+ },
+ accordion: {
+ type: Boolean,
+ observer: 'updateExpanded',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updateExpanded() {
+ this.children.forEach((child) => {
+ child.updateExpanded();
+ });
+ },
+ switch(name, expanded) {
+ const { accordion, value } = this.data;
+ const changeItem = name;
+ if (!accordion) {
+ name = expanded
+ ? (value || []).concat(name)
+ : (value || []).filter((activeName) => activeName !== name);
+ }
+ else {
+ name = expanded ? name : '';
+ }
+ if (expanded) {
+ this.$emit('open', changeItem);
+ }
+ else {
+ this.$emit('close', changeItem);
+ }
+ this.$emit('change', name);
+ this.$emit('input', name);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxml
new file mode 100644
index 0000000..fd4e171
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxml
@@ -0,0 +1,3 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/collapse/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.d.ts
new file mode 100644
index 0000000..386f307
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.d.ts
@@ -0,0 +1,7 @@
+export declare const RED = "#ee0a24";
+export declare const BLUE = "#1989fa";
+export declare const WHITE = "#fff";
+export declare const GREEN = "#07c160";
+export declare const ORANGE = "#ff976a";
+export declare const GRAY = "#323233";
+export declare const GRAY_DARK = "#969799";
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.js
new file mode 100644
index 0000000..6b285bd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/color.js
@@ -0,0 +1,7 @@
+export const RED = '#ee0a24';
+export const BLUE = '#1989fa';
+export const WHITE = '#fff';
+export const GREEN = '#07c160';
+export const ORANGE = '#ff976a';
+export const GRAY = '#323233';
+export const GRAY_DARK = '#969799';
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.d.ts
new file mode 100644
index 0000000..1d0fd27
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.d.ts
@@ -0,0 +1,4 @@
+///
+import { VantComponentOptions } from 'definitions/index';
+declare function VantComponent(vantOptions: VantComponentOptions): void;
+export { VantComponent };
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.js
new file mode 100644
index 0000000..938d96b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/component.js
@@ -0,0 +1,46 @@
+import { basic } from '../mixins/basic';
+function mapKeys(source, target, map) {
+ Object.keys(map).forEach((key) => {
+ if (source[key]) {
+ target[map[key]] = source[key];
+ }
+ });
+}
+function VantComponent(vantOptions) {
+ const options = {};
+ mapKeys(vantOptions, options, {
+ data: 'data',
+ props: 'properties',
+ watch: 'observers',
+ mixins: 'behaviors',
+ methods: 'methods',
+ beforeCreate: 'created',
+ created: 'attached',
+ mounted: 'ready',
+ destroyed: 'detached',
+ classes: 'externalClasses',
+ });
+ // add default externalClasses
+ options.externalClasses = options.externalClasses || [];
+ options.externalClasses.push('custom-class');
+ // add default behaviors
+ options.behaviors = options.behaviors || [];
+ options.behaviors.push(basic);
+ // add relations
+ const { relation } = vantOptions;
+ if (relation) {
+ options.relations = relation.relations;
+ options.behaviors.push(relation.mixin);
+ }
+ // map field to form-field behavior
+ if (vantOptions.field) {
+ options.behaviors.push('wx://form-field');
+ }
+ // add default options
+ options.options = {
+ multipleSlots: true,
+ addGlobalClass: true,
+ };
+ Component(options);
+}
+export { VantComponent };
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/index.wxss
new file mode 100644
index 0000000..a73bb7a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/index.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}.van-clearfix:after{clear:both;content:"";display:table}.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.d.ts
new file mode 100644
index 0000000..10193fa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.d.ts
@@ -0,0 +1,15 @@
+///
+type TrivialInstance = WechatMiniprogram.Component.TrivialInstance;
+export declare function useParent(name: string, onEffect?: (this: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export declare function useChildren(name: string, onEffect?: (this: TrivialInstance, target: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.js
new file mode 100644
index 0000000..04e2934
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/relation.js
@@ -0,0 +1,56 @@
+export function useParent(name, onEffect) {
+ const path = `../${name}/index`;
+ return {
+ relations: {
+ [path]: {
+ type: 'ancestor',
+ linked() {
+ onEffect && onEffect.call(this);
+ },
+ linkChanged() {
+ onEffect && onEffect.call(this);
+ },
+ unlinked() {
+ onEffect && onEffect.call(this);
+ },
+ },
+ },
+ mixin: Behavior({
+ created() {
+ Object.defineProperty(this, 'parent', {
+ get: () => this.getRelationNodes(path)[0],
+ });
+ Object.defineProperty(this, 'index', {
+ // @ts-ignore
+ get: () => { var _a, _b; return (_b = (_a = this.parent) === null || _a === void 0 ? void 0 : _a.children) === null || _b === void 0 ? void 0 : _b.indexOf(this); },
+ });
+ },
+ }),
+ };
+}
+export function useChildren(name, onEffect) {
+ const path = `../${name}/index`;
+ return {
+ relations: {
+ [path]: {
+ type: 'descendant',
+ linked(target) {
+ onEffect && onEffect.call(this, target);
+ },
+ linkChanged(target) {
+ onEffect && onEffect.call(this, target);
+ },
+ unlinked(target) {
+ onEffect && onEffect.call(this, target);
+ },
+ },
+ },
+ mixin: Behavior({
+ created() {
+ Object.defineProperty(this, 'children', {
+ get: () => this.getRelationNodes(path) || [],
+ });
+ },
+ }),
+ };
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/clearfix.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/clearfix.wxss
new file mode 100644
index 0000000..442246f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/clearfix.wxss
@@ -0,0 +1 @@
+.van-clearfix:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/ellipsis.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/ellipsis.wxss
new file mode 100644
index 0000000..ee701df
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/ellipsis.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/hairline.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/hairline.wxss
new file mode 100644
index 0000000..f7c6260
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/hairline.wxss
@@ -0,0 +1 @@
+.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/clearfix.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/clearfix.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/ellipsis.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/ellipsis.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/hairline.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/mixins/hairline.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/var.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/style/var.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.d.ts
new file mode 100644
index 0000000..a77d8c6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.d.ts
@@ -0,0 +1,21 @@
+///
+///
+///
+///
+///
+export { isDef } from './validator';
+export { getSystemInfoSync } from './version';
+export declare function range(num: number, min: number, max: number): number;
+export declare function nextTick(cb: (...args: any[]) => void): void;
+export declare function addUnit(value?: string | number): string | undefined;
+export declare function requestAnimationFrame(cb: () => void): NodeJS.Timeout;
+export declare function pickExclude(obj: unknown, keys: string[]): {};
+export declare function getRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function getAllRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function groupSetData(context: WechatMiniprogram.Component.TrivialInstance, cb: () => void): void;
+export declare function toPromise(promiseLike: Promise | unknown): Promise;
+export declare function addNumber(num1: any, num2: any): number;
+export declare const clamp: (num: any, min: any, max: any) => number;
+export declare function getCurrentPage(): T & WechatMiniprogram.OptionalInterface & WechatMiniprogram.Page.InstanceProperties & WechatMiniprogram.Page.InstanceMethods & WechatMiniprogram.Page.Data & WechatMiniprogram.IAnyObject;
+export declare const isPC: boolean;
+export declare const isWxWork: boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.js
new file mode 100644
index 0000000..c84e32b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/utils.js
@@ -0,0 +1,86 @@
+import { isDef, isNumber, isPlainObject, isPromise } from './validator';
+import { canIUseGroupSetData, canIUseNextTick, getSystemInfoSync, } from './version';
+export { isDef } from './validator';
+export { getSystemInfoSync } from './version';
+export function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+export function nextTick(cb) {
+ if (canIUseNextTick()) {
+ wx.nextTick(cb);
+ }
+ else {
+ setTimeout(() => {
+ cb();
+ }, 1000 / 30);
+ }
+}
+export function addUnit(value) {
+ if (!isDef(value)) {
+ return undefined;
+ }
+ value = String(value);
+ return isNumber(value) ? `${value}px` : value;
+}
+export function requestAnimationFrame(cb) {
+ return setTimeout(() => {
+ cb();
+ }, 1000 / 30);
+}
+export function pickExclude(obj, keys) {
+ if (!isPlainObject(obj)) {
+ return {};
+ }
+ return Object.keys(obj).reduce((prev, key) => {
+ if (!keys.includes(key)) {
+ prev[key] = obj[key];
+ }
+ return prev;
+ }, {});
+}
+export function getRect(context, selector) {
+ return new Promise((resolve) => {
+ wx.createSelectorQuery()
+ .in(context)
+ .select(selector)
+ .boundingClientRect()
+ .exec((rect = []) => resolve(rect[0]));
+ });
+}
+export function getAllRect(context, selector) {
+ return new Promise((resolve) => {
+ wx.createSelectorQuery()
+ .in(context)
+ .selectAll(selector)
+ .boundingClientRect()
+ .exec((rect = []) => resolve(rect[0]));
+ });
+}
+export function groupSetData(context, cb) {
+ if (canIUseGroupSetData()) {
+ context.groupSetData(cb);
+ }
+ else {
+ cb();
+ }
+}
+export function toPromise(promiseLike) {
+ if (isPromise(promiseLike)) {
+ return promiseLike;
+ }
+ return Promise.resolve(promiseLike);
+}
+// 浮点数精度处理
+export function addNumber(num1, num2) {
+ const cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+// 限制value在[min, max]之间
+export const clamp = (num, min, max) => Math.min(Math.max(num, min), max);
+export function getCurrentPage() {
+ const pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+export const isPC = ['mac', 'windows'].includes(getSystemInfoSync().platform);
+// 是否企业微信
+export const isWxWork = getSystemInfoSync().environment === 'wxwork';
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.d.ts
new file mode 100644
index 0000000..152894a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.d.ts
@@ -0,0 +1,9 @@
+export declare function isFunction(val: unknown): val is Function;
+export declare function isPlainObject(val: unknown): val is Record;
+export declare function isPromise(val: unknown): val is Promise;
+export declare function isDef(value: unknown): boolean;
+export declare function isObj(x: unknown): x is Record;
+export declare function isNumber(value: string): boolean;
+export declare function isBoolean(value: unknown): value is boolean;
+export declare function isImageUrl(url: string): boolean;
+export declare function isVideoUrl(url: string): boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.js
new file mode 100644
index 0000000..f11f844
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/validator.js
@@ -0,0 +1,31 @@
+// eslint-disable-next-line @typescript-eslint/ban-types
+export function isFunction(val) {
+ return typeof val === 'function';
+}
+export function isPlainObject(val) {
+ return val !== null && typeof val === 'object' && !Array.isArray(val);
+}
+export function isPromise(val) {
+ return isPlainObject(val) && isFunction(val.then) && isFunction(val.catch);
+}
+export function isDef(value) {
+ return value !== undefined && value !== null;
+}
+export function isObj(x) {
+ const type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+export function isNumber(value) {
+ return /^\d+(\.\d+)?$/.test(value);
+}
+export function isBoolean(value) {
+ return typeof value === 'boolean';
+}
+const IMAGE_REGEXP = /\.(jpeg|jpg|gif|png|svg|webp|jfif|bmp|dpg)/i;
+const VIDEO_REGEXP = /\.(mp4|mpg|mpeg|dat|asf|avi|rm|rmvb|mov|wmv|flv|mkv)/i;
+export function isImageUrl(url) {
+ return IMAGE_REGEXP.test(url);
+}
+export function isVideoUrl(url) {
+ return VIDEO_REGEXP.test(url);
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.d.ts
new file mode 100644
index 0000000..3393221
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.d.ts
@@ -0,0 +1,15 @@
+///
+interface WxWorkSystemInfo extends WechatMiniprogram.SystemInfo {
+ environment?: 'wxwork';
+}
+interface SystemInfo extends WxWorkSystemInfo, WechatMiniprogram.SystemInfo {
+}
+export declare function getSystemInfoSync(): SystemInfo;
+export declare function canIUseModel(): boolean;
+export declare function canIUseFormFieldButton(): boolean;
+export declare function canIUseAnimate(): boolean;
+export declare function canIUseGroupSetData(): boolean;
+export declare function canIUseNextTick(): boolean;
+export declare function canIUseCanvas2d(): boolean;
+export declare function canIUseGetUserProfile(): boolean;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.js
new file mode 100644
index 0000000..c675e1f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/common/version.js
@@ -0,0 +1,59 @@
+let systemInfo;
+export function getSystemInfoSync() {
+ if (systemInfo == null) {
+ systemInfo = wx.getSystemInfoSync();
+ }
+ return systemInfo;
+}
+function compareVersion(v1, v2) {
+ v1 = v1.split('.');
+ v2 = v2.split('.');
+ const len = Math.max(v1.length, v2.length);
+ while (v1.length < len) {
+ v1.push('0');
+ }
+ while (v2.length < len) {
+ v2.push('0');
+ }
+ for (let i = 0; i < len; i++) {
+ const num1 = parseInt(v1[i], 10);
+ const num2 = parseInt(v2[i], 10);
+ if (num1 > num2) {
+ return 1;
+ }
+ if (num1 < num2) {
+ return -1;
+ }
+ }
+ return 0;
+}
+function gte(version) {
+ const system = getSystemInfoSync();
+ return compareVersion(system.SDKVersion, version) >= 0;
+}
+export function canIUseModel() {
+ return gte('2.9.3');
+}
+export function canIUseFormFieldButton() {
+ return gte('2.10.3');
+}
+export function canIUseAnimate() {
+ return gte('2.9.0');
+}
+export function canIUseGroupSetData() {
+ return gte('2.4.0');
+}
+export function canIUseNextTick() {
+ try {
+ return wx.canIUse('nextTick');
+ }
+ catch (e) {
+ return gte('2.7.1');
+ }
+}
+export function canIUseCanvas2d() {
+ return gte('2.9.0');
+}
+export function canIUseGetUserProfile() {
+ return !!wx.getUserProfile;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.js
new file mode 100644
index 0000000..0cb23f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.js
@@ -0,0 +1,9 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ themeVars: {
+ type: Object,
+ value: {},
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxml
new file mode 100644
index 0000000..3cfb461
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxs
new file mode 100644
index 0000000..7ca0203
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/config-provider/index.wxs
@@ -0,0 +1,29 @@
+/* eslint-disable */
+var object = require('../wxs/object.wxs');
+var style = require('../wxs/style.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+ .replace(getRegExp("^-"), '');
+
+ return newWord;
+}
+
+function mapThemeVarsToCSSVars(themeVars) {
+ var cssVars = {};
+ object.keys(themeVars).forEach(function (key) {
+ var cssVarsKey = '--' + kebabCase(key);
+ cssVars[cssVarsKey] = themeVars[key];
+ });
+
+ return style(cssVars);
+}
+
+module.exports = {
+ kebabCase: kebabCase,
+ mapThemeVarsToCSSVars: mapThemeVarsToCSSVars,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.js
new file mode 100644
index 0000000..da24145
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.js
@@ -0,0 +1,100 @@
+import { VantComponent } from '../common/component';
+import { isSameSecond, parseFormat, parseTimeData } from './utils';
+function simpleTick(fn) {
+ return setTimeout(fn, 30);
+}
+VantComponent({
+ props: {
+ useSlot: Boolean,
+ millisecond: Boolean,
+ time: {
+ type: Number,
+ observer: 'reset',
+ },
+ format: {
+ type: String,
+ value: 'HH:mm:ss',
+ },
+ autoStart: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ timeData: parseTimeData(0),
+ formattedTime: '0',
+ },
+ destroyed() {
+ clearTimeout(this.tid);
+ this.tid = null;
+ },
+ methods: {
+ // 开始
+ start() {
+ if (this.counting) {
+ return;
+ }
+ this.counting = true;
+ this.endTime = Date.now() + this.remain;
+ this.tick();
+ },
+ // 暂停
+ pause() {
+ this.counting = false;
+ clearTimeout(this.tid);
+ },
+ // 重置
+ reset() {
+ this.pause();
+ this.remain = this.data.time;
+ this.setRemain(this.remain);
+ if (this.data.autoStart) {
+ this.start();
+ }
+ },
+ tick() {
+ if (this.data.millisecond) {
+ this.microTick();
+ }
+ else {
+ this.macroTick();
+ }
+ },
+ microTick() {
+ this.tid = simpleTick(() => {
+ this.setRemain(this.getRemain());
+ if (this.remain !== 0) {
+ this.microTick();
+ }
+ });
+ },
+ macroTick() {
+ this.tid = simpleTick(() => {
+ const remain = this.getRemain();
+ if (!isSameSecond(remain, this.remain) || remain === 0) {
+ this.setRemain(remain);
+ }
+ if (this.remain !== 0) {
+ this.macroTick();
+ }
+ });
+ },
+ getRemain() {
+ return Math.max(this.endTime - Date.now(), 0);
+ },
+ setRemain(remain) {
+ this.remain = remain;
+ const timeData = parseTimeData(remain);
+ if (this.data.useSlot) {
+ this.$emit('change', timeData);
+ }
+ this.setData({
+ formattedTime: parseFormat(this.data.format, timeData),
+ });
+ if (remain === 0) {
+ this.pause();
+ this.$emit('finish');
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxml
new file mode 100644
index 0000000..e206e16
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxml
@@ -0,0 +1,4 @@
+
+
+ {{ formattedTime }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxss
new file mode 100644
index 0000000..8b957f7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-count-down{color:var(--count-down-text-color,#323233);font-size:var(--count-down-font-size,14px);line-height:var(--count-down-line-height,20px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.d.ts
new file mode 100644
index 0000000..876a6c1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.d.ts
@@ -0,0 +1,10 @@
+export type TimeData = {
+ days: number;
+ hours: number;
+ minutes: number;
+ seconds: number;
+ milliseconds: number;
+};
+export declare function parseTimeData(time: number): TimeData;
+export declare function parseFormat(format: string, timeData: TimeData): string;
+export declare function isSameSecond(time1: number, time2: number): boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.js
new file mode 100644
index 0000000..cbdbd79
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/count-down/utils.js
@@ -0,0 +1,57 @@
+function padZero(num, targetLength = 2) {
+ let str = num + '';
+ while (str.length < targetLength) {
+ str = '0' + str;
+ }
+ return str;
+}
+const SECOND = 1000;
+const MINUTE = 60 * SECOND;
+const HOUR = 60 * MINUTE;
+const DAY = 24 * HOUR;
+export function parseTimeData(time) {
+ const days = Math.floor(time / DAY);
+ const hours = Math.floor((time % DAY) / HOUR);
+ const minutes = Math.floor((time % HOUR) / MINUTE);
+ const seconds = Math.floor((time % MINUTE) / SECOND);
+ const milliseconds = Math.floor(time % SECOND);
+ return {
+ days,
+ hours,
+ minutes,
+ seconds,
+ milliseconds,
+ };
+}
+export function parseFormat(format, timeData) {
+ const { days } = timeData;
+ let { hours, minutes, seconds, milliseconds } = timeData;
+ if (format.indexOf('DD') === -1) {
+ hours += days * 24;
+ }
+ else {
+ format = format.replace('DD', padZero(days));
+ }
+ if (format.indexOf('HH') === -1) {
+ minutes += hours * 60;
+ }
+ else {
+ format = format.replace('HH', padZero(hours));
+ }
+ if (format.indexOf('mm') === -1) {
+ seconds += minutes * 60;
+ }
+ else {
+ format = format.replace('mm', padZero(minutes));
+ }
+ if (format.indexOf('ss') === -1) {
+ milliseconds += seconds * 1000;
+ }
+ else {
+ format = format.replace('ss', padZero(seconds));
+ }
+ return format.replace('SSS', padZero(milliseconds, 3));
+}
+export function isSameSecond(time1, time2) {
+ return Math.floor(time1 / 1000) === Math.floor(time2 / 1000);
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.js
new file mode 100644
index 0000000..3334170
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.js
@@ -0,0 +1,295 @@
+import { VantComponent } from '../common/component';
+import { isDef } from '../common/validator';
+import { pickerProps } from '../picker/shared';
+const currentYear = new Date().getFullYear();
+function isValidDate(date) {
+ return isDef(date) && !isNaN(new Date(date).getTime());
+}
+function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+function padZero(val) {
+ return `00${val}`.slice(-2);
+}
+function times(n, iteratee) {
+ let index = -1;
+ const result = Array(n < 0 ? 0 : n);
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+function getTrueValue(formattedValue) {
+ if (formattedValue === undefined) {
+ formattedValue = '1';
+ }
+ while (isNaN(parseInt(formattedValue, 10))) {
+ formattedValue = formattedValue.slice(1);
+ }
+ return parseInt(formattedValue, 10);
+}
+function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+const defaultFormatter = (type, value) => value;
+VantComponent({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: Object.assign(Object.assign({}, pickerProps), { value: {
+ type: null,
+ observer: 'updateValue',
+ }, filter: null, type: {
+ type: String,
+ value: 'datetime',
+ observer: 'updateValue',
+ }, showToolbar: {
+ type: Boolean,
+ value: true,
+ }, formatter: {
+ type: null,
+ value: defaultFormatter,
+ }, minDate: {
+ type: Number,
+ value: new Date(currentYear - 10, 0, 1).getTime(),
+ observer: 'updateValue',
+ }, maxDate: {
+ type: Number,
+ value: new Date(currentYear + 10, 11, 31).getTime(),
+ observer: 'updateValue',
+ }, minHour: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxHour: {
+ type: Number,
+ value: 23,
+ observer: 'updateValue',
+ }, minMinute: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxMinute: {
+ type: Number,
+ value: 59,
+ observer: 'updateValue',
+ } }),
+ data: {
+ innerValue: Date.now(),
+ columns: [],
+ },
+ methods: {
+ updateValue() {
+ const { data } = this;
+ const val = this.correctValue(data.value);
+ const isEqual = val === data.innerValue;
+ this.updateColumnValue(val).then(() => {
+ if (!isEqual) {
+ this.$emit('input', val);
+ }
+ });
+ },
+ getPicker() {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-datetime-picker');
+ const { picker } = this;
+ const { setColumnValues } = picker;
+ picker.setColumnValues = (...args) => setColumnValues.apply(picker, [...args, false]);
+ }
+ return this.picker;
+ },
+ updateColumns() {
+ const { formatter = defaultFormatter } = this.data;
+ const results = this.getOriginColumns().map((column) => ({
+ values: column.values.map((value) => formatter(column.type, value)),
+ }));
+ return this.set({ columns: results });
+ },
+ getOriginColumns() {
+ const { filter } = this.data;
+ const results = this.getRanges().map(({ type, range }) => {
+ let values = times(range[1] - range[0] + 1, (index) => {
+ const value = range[0] + index;
+ return type === 'year' ? `${value}` : padZero(value);
+ });
+ if (filter) {
+ values = filter(type, values);
+ }
+ return { type, values };
+ });
+ return results;
+ },
+ getRanges() {
+ const { data } = this;
+ if (data.type === 'time') {
+ return [
+ {
+ type: 'hour',
+ range: [data.minHour, data.maxHour],
+ },
+ {
+ type: 'minute',
+ range: [data.minMinute, data.maxMinute],
+ },
+ ];
+ }
+ const { maxYear, maxDate, maxMonth, maxHour, maxMinute, } = this.getBoundary('max', data.innerValue);
+ const { minYear, minDate, minMonth, minHour, minMinute, } = this.getBoundary('min', data.innerValue);
+ const result = [
+ {
+ type: 'year',
+ range: [minYear, maxYear],
+ },
+ {
+ type: 'month',
+ range: [minMonth, maxMonth],
+ },
+ {
+ type: 'day',
+ range: [minDate, maxDate],
+ },
+ {
+ type: 'hour',
+ range: [minHour, maxHour],
+ },
+ {
+ type: 'minute',
+ range: [minMinute, maxMinute],
+ },
+ ];
+ if (data.type === 'date')
+ result.splice(3, 2);
+ if (data.type === 'year-month')
+ result.splice(2, 3);
+ return result;
+ },
+ correctValue(value) {
+ const { data } = this;
+ // validate value
+ const isDateType = data.type !== 'time';
+ if (isDateType && !isValidDate(value)) {
+ value = data.minDate;
+ }
+ else if (!isDateType && !value) {
+ const { minHour } = data;
+ value = `${padZero(minHour)}:00`;
+ }
+ // time type
+ if (!isDateType) {
+ let [hour, minute] = value.split(':');
+ hour = padZero(range(hour, data.minHour, data.maxHour));
+ minute = padZero(range(minute, data.minMinute, data.maxMinute));
+ return `${hour}:${minute}`;
+ }
+ // date type
+ value = Math.max(value, data.minDate);
+ value = Math.min(value, data.maxDate);
+ return value;
+ },
+ getBoundary(type, innerValue) {
+ const value = new Date(innerValue);
+ const boundary = new Date(this.data[`${type}Date`]);
+ const year = boundary.getFullYear();
+ let month = 1;
+ let date = 1;
+ let hour = 0;
+ let minute = 0;
+ if (type === 'max') {
+ month = 12;
+ date = getMonthEndDay(value.getFullYear(), value.getMonth() + 1);
+ hour = 23;
+ minute = 59;
+ }
+ if (value.getFullYear() === year) {
+ month = boundary.getMonth() + 1;
+ if (value.getMonth() + 1 === month) {
+ date = boundary.getDate();
+ if (value.getDate() === date) {
+ hour = boundary.getHours();
+ if (value.getHours() === hour) {
+ minute = boundary.getMinutes();
+ }
+ }
+ }
+ }
+ return {
+ [`${type}Year`]: year,
+ [`${type}Month`]: month,
+ [`${type}Date`]: date,
+ [`${type}Hour`]: hour,
+ [`${type}Minute`]: minute,
+ };
+ },
+ onCancel() {
+ this.$emit('cancel');
+ },
+ onConfirm() {
+ this.$emit('confirm', this.data.innerValue);
+ },
+ onChange() {
+ const { data } = this;
+ let value;
+ const picker = this.getPicker();
+ const originColumns = this.getOriginColumns();
+ if (data.type === 'time') {
+ const indexes = picker.getIndexes();
+ value = `${+originColumns[0].values[indexes[0]]}:${+originColumns[1]
+ .values[indexes[1]]}`;
+ }
+ else {
+ const indexes = picker.getIndexes();
+ const values = indexes.map((value, index) => originColumns[index].values[value]);
+ const year = getTrueValue(values[0]);
+ const month = getTrueValue(values[1]);
+ const maxDate = getMonthEndDay(year, month);
+ let date = getTrueValue(values[2]);
+ if (data.type === 'year-month') {
+ date = 1;
+ }
+ date = date > maxDate ? maxDate : date;
+ let hour = 0;
+ let minute = 0;
+ if (data.type === 'datetime') {
+ hour = getTrueValue(values[3]);
+ minute = getTrueValue(values[4]);
+ }
+ value = new Date(year, month - 1, date, hour, minute);
+ }
+ value = this.correctValue(value);
+ this.updateColumnValue(value).then(() => {
+ this.$emit('input', value);
+ this.$emit('change', picker);
+ });
+ },
+ updateColumnValue(value) {
+ let values = [];
+ const { type } = this.data;
+ const formatter = this.data.formatter || defaultFormatter;
+ const picker = this.getPicker();
+ if (type === 'time') {
+ const pair = value.split(':');
+ values = [formatter('hour', pair[0]), formatter('minute', pair[1])];
+ }
+ else {
+ const date = new Date(value);
+ values = [
+ formatter('year', `${date.getFullYear()}`),
+ formatter('month', padZero(date.getMonth() + 1)),
+ ];
+ if (type === 'date') {
+ values.push(formatter('day', padZero(date.getDate())));
+ }
+ if (type === 'datetime') {
+ values.push(formatter('day', padZero(date.getDate())), formatter('hour', padZero(date.getHours())), formatter('minute', padZero(date.getMinutes())));
+ }
+ }
+ return this.set({ innerValue: value })
+ .then(() => this.updateColumns())
+ .then(() => picker.setValues(values));
+ },
+ },
+ created() {
+ const innerValue = this.correctValue(this.data.value);
+ this.updateColumnValue(innerValue).then(() => {
+ this.$emit('input', innerValue);
+ });
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxml
new file mode 100644
index 0000000..ade2202
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/datetime-picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.d.ts
new file mode 100644
index 0000000..d0554f6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.d.ts
@@ -0,0 +1,28 @@
+///
+interface VantComponentInstance {
+ parent: WechatMiniprogram.Component.TrivialInstance;
+ children: WechatMiniprogram.Component.TrivialInstance[];
+ index: number;
+ $emit: (name: string, detail?: unknown, options?: WechatMiniprogram.Component.TriggerEventOption) => void;
+}
+export type VantComponentOptions = {
+ data?: Data;
+ field?: boolean;
+ classes?: string[];
+ mixins?: string[];
+ props?: Props;
+ relation?: {
+ relations: Record;
+ mixin: string;
+ };
+ watch?: Record any>;
+ methods?: Methods;
+ beforeCreate?: () => void;
+ created?: () => void;
+ mounted?: () => void;
+ destroyed?: () => void;
+} & ThisType, Props, Methods> & Record>;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.js
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/definitions/index.js
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.d.ts
new file mode 100644
index 0000000..db2da5f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.d.ts
@@ -0,0 +1,55 @@
+///
+///
+export type Action = 'confirm' | 'cancel' | 'overlay';
+type DialogContext = WechatMiniprogram.Page.TrivialInstance | WechatMiniprogram.Component.TrivialInstance;
+interface DialogOptions {
+ lang?: string;
+ show?: boolean;
+ title?: string;
+ width?: string | number | null;
+ zIndex?: number;
+ theme?: string;
+ context?: (() => DialogContext) | DialogContext;
+ message?: string;
+ overlay?: boolean;
+ selector?: string;
+ ariaLabel?: string;
+ /**
+ * @deprecated use custom-class instead
+ */
+ className?: string;
+ customStyle?: string;
+ transition?: string;
+ /**
+ * @deprecated use beforeClose instead
+ */
+ asyncClose?: boolean;
+ beforeClose?: null | ((action: Action) => Promise | void);
+ businessId?: number;
+ sessionFrom?: string;
+ overlayStyle?: string;
+ appParameter?: string;
+ messageAlign?: string;
+ sendMessageImg?: string;
+ showMessageCard?: boolean;
+ sendMessagePath?: string;
+ sendMessageTitle?: string;
+ confirmButtonText?: string;
+ cancelButtonText?: string;
+ showConfirmButton?: boolean;
+ showCancelButton?: boolean;
+ closeOnClickOverlay?: boolean;
+ confirmButtonOpenType?: string;
+}
+declare const Dialog: {
+ (options: DialogOptions): Promise;
+ alert(options: DialogOptions): Promise;
+ confirm(options: DialogOptions): Promise;
+ close(): void;
+ stopLoading(): void;
+ currentOptions: DialogOptions;
+ defaultOptions: DialogOptions;
+ setDefaultOptions(options: DialogOptions): void;
+ resetDefaultOptions(): void;
+};
+export default Dialog;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.js
new file mode 100644
index 0000000..a96ec08
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/dialog.js
@@ -0,0 +1,77 @@
+let queue = [];
+const defaultOptions = {
+ show: false,
+ title: '',
+ width: null,
+ theme: 'default',
+ message: '',
+ zIndex: 100,
+ overlay: true,
+ selector: '#van-dialog',
+ className: '',
+ asyncClose: false,
+ beforeClose: null,
+ transition: 'scale',
+ customStyle: '',
+ messageAlign: '',
+ overlayStyle: '',
+ confirmButtonText: '确认',
+ cancelButtonText: '取消',
+ showConfirmButton: true,
+ showCancelButton: false,
+ closeOnClickOverlay: false,
+ confirmButtonOpenType: '',
+};
+let currentOptions = Object.assign({}, defaultOptions);
+function getContext() {
+ const pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+const Dialog = (options) => {
+ options = Object.assign(Object.assign({}, currentOptions), options);
+ return new Promise((resolve, reject) => {
+ const context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ const dialog = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (dialog) {
+ dialog.setData(Object.assign({ callback: (action, instance) => {
+ action === 'confirm' ? resolve(instance) : reject(instance);
+ } }, options));
+ wx.nextTick(() => {
+ dialog.setData({ show: true });
+ });
+ queue.push(dialog);
+ }
+ else {
+ console.warn('未找到 van-dialog 节点,请确认 selector 及 context 是否正确');
+ }
+ });
+};
+Dialog.alert = (options) => Dialog(options);
+Dialog.confirm = (options) => Dialog(Object.assign({ showCancelButton: true }, options));
+Dialog.close = () => {
+ queue.forEach((dialog) => {
+ dialog.close();
+ });
+ queue = [];
+};
+Dialog.stopLoading = () => {
+ queue.forEach((dialog) => {
+ dialog.stopLoading();
+ });
+};
+Dialog.currentOptions = currentOptions;
+Dialog.defaultOptions = defaultOptions;
+Dialog.setDefaultOptions = (options) => {
+ currentOptions = Object.assign(Object.assign({}, currentOptions), options);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions = () => {
+ currentOptions = Object.assign({}, defaultOptions);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions();
+export default Dialog;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.js
new file mode 100644
index 0000000..e2cc450
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.js
@@ -0,0 +1,130 @@
+import { VantComponent } from '../common/component';
+import { button } from '../mixins/button';
+import { GRAY, RED } from '../common/color';
+import { toPromise } from '../common/utils';
+VantComponent({
+ mixins: [button],
+ classes: ['cancle-button-class', 'confirm-button-class'],
+ props: {
+ show: {
+ type: Boolean,
+ observer(show) {
+ !show && this.stopLoading();
+ },
+ },
+ title: String,
+ message: String,
+ theme: {
+ type: String,
+ value: 'default',
+ },
+ confirmButtonId: String,
+ className: String,
+ customStyle: String,
+ asyncClose: Boolean,
+ messageAlign: String,
+ beforeClose: null,
+ overlayStyle: String,
+ useSlot: Boolean,
+ useTitleSlot: Boolean,
+ useConfirmButtonSlot: Boolean,
+ useCancelButtonSlot: Boolean,
+ showCancelButton: Boolean,
+ closeOnClickOverlay: Boolean,
+ confirmButtonOpenType: String,
+ width: null,
+ zIndex: {
+ type: Number,
+ value: 2000,
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonColor: {
+ type: String,
+ value: RED,
+ },
+ cancelButtonColor: {
+ type: String,
+ value: GRAY,
+ },
+ showConfirmButton: {
+ type: Boolean,
+ value: true,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ transition: {
+ type: String,
+ value: 'scale',
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ callback: (() => { }),
+ },
+ methods: {
+ onConfirm() {
+ this.handleAction('confirm');
+ },
+ onCancel() {
+ this.handleAction('cancel');
+ },
+ onClickOverlay() {
+ this.close('overlay');
+ },
+ close(action) {
+ this.setData({ show: false });
+ wx.nextTick(() => {
+ this.$emit('close', action);
+ const { callback } = this.data;
+ if (callback) {
+ callback(action, this);
+ }
+ });
+ },
+ stopLoading() {
+ this.setData({
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ });
+ },
+ handleAction(action) {
+ this.$emit(action, { dialog: this });
+ const { asyncClose, beforeClose } = this.data;
+ if (!asyncClose && !beforeClose) {
+ this.close(action);
+ return;
+ }
+ this.setData({
+ [`loading.${action}`]: true,
+ });
+ if (beforeClose) {
+ toPromise(beforeClose(action)).then((value) => {
+ if (value) {
+ this.close(action);
+ }
+ else {
+ this.stopLoading();
+ }
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.json
new file mode 100644
index 0000000..43417fc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-button": "../button/index",
+ "van-goods-action": "../goods-action/index",
+ "van-goods-action-button": "../goods-action-button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxml
new file mode 100644
index 0000000..a1d8e3c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxml
@@ -0,0 +1,125 @@
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+ {{ cancelButtonText }}
+
+
+ {{ confirmButtonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxss
new file mode 100644
index 0000000..507a789
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dialog/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dialog{background-color:var(--dialog-background-color,#fff);border-radius:var(--dialog-border-radius,16px);font-size:var(--dialog-font-size,16px);overflow:hidden;top:45%!important;width:var(--dialog-width,320px)}@media (max-width:321px){.van-dialog{width:var(--dialog-small-screen-width,90%)}}.van-dialog__header{font-weight:var(--dialog-header-font-weight,500);line-height:var(--dialog-header-line-height,24px);padding-top:var(--dialog-header-padding-top,24px);text-align:center}.van-dialog__header--isolated{padding:var(--dialog-header-isolated-padding,24px 0)}.van-dialog__message{-webkit-overflow-scrolling:touch;font-size:var(--dialog-message-font-size,14px);line-height:var(--dialog-message-line-height,20px);max-height:var(--dialog-message-max-height,60vh);overflow-y:auto;padding:var(--dialog-message-padding,24px);text-align:center}.van-dialog__message-text{word-wrap:break-word}.van-dialog__message--hasTitle{color:var(--dialog-has-title-message-text-color,#646566);padding-top:var(--dialog-has-title-message-padding-top,8px)}.van-dialog__message--round-button{color:#323233;padding-bottom:16px}.van-dialog__message--left{text-align:left}.van-dialog__message--right{text-align:right}.van-dialog__message--justify{text-align:justify}.van-dialog__footer{display:flex}.van-dialog__footer--round-button{padding:8px 24px 16px!important;position:relative!important}.van-dialog__button{flex:1}.van-dialog__cancel,.van-dialog__confirm{border:0!important}.van-dialog-bounce-enter{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-dialog-bounce-leave-active{opacity:0;transform:translate3d(-50%,-50%,0) scale(.9)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.js
new file mode 100644
index 0000000..9596edd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.js
@@ -0,0 +1,12 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ dashed: Boolean,
+ hairline: Boolean,
+ contentPosition: String,
+ fontSize: String,
+ borderColor: String,
+ textColor: String,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxml
new file mode 100644
index 0000000..f6a5a45
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxs
new file mode 100644
index 0000000..215b14f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ 'border-color': data.borderColor,
+ color: data.textColor,
+ 'font-size': addUnit(data.fontSize),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxss
new file mode 100644
index 0000000..e91dc44
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/divider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-divider{align-items:center;border:0 solid var(--divider-border-color,#ebedf0);color:var(--divider-text-color,#969799);display:flex;font-size:var(--divider-font-size,14px);line-height:var(--divider-line-height,24px);margin:var(--divider-margin,16px 0)}.van-divider:after,.van-divider:before{border-color:inherit;border-style:inherit;border-width:1px 0 0;box-sizing:border-box;display:block;flex:1;height:1px}.van-divider:before{content:""}.van-divider--hairline:after,.van-divider--hairline:before{transform:scaleY(.5)}.van-divider--dashed{border-style:dashed}.van-divider--center:before,.van-divider--left:before,.van-divider--right:before{margin-right:var(--divider-content-padding,16px)}.van-divider--center:after,.van-divider--left:after,.van-divider--right:after{content:"";margin-left:var(--divider-content-padding,16px)}.van-divider--left:before{max-width:var(--divider-content-left-width,10%)}.van-divider--right:after{max-width:var(--divider-content-right-width,10%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.js
new file mode 100644
index 0000000..fd61a47
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.js
@@ -0,0 +1,130 @@
+import { useParent } from '../common/relation';
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: ['item-title-class'],
+ field: true,
+ relation: useParent('dropdown-menu', function () {
+ this.updateDataFromParent();
+ }),
+ props: {
+ value: {
+ type: null,
+ observer: 'rerender',
+ },
+ title: {
+ type: String,
+ observer: 'rerender',
+ },
+ disabled: Boolean,
+ titleClass: {
+ type: String,
+ observer: 'rerender',
+ },
+ options: {
+ type: Array,
+ value: [],
+ observer: 'rerender',
+ },
+ popupStyle: String,
+ useBeforeToggle: {
+ type: Boolean,
+ value: false,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ transition: true,
+ showPopup: false,
+ showWrapper: false,
+ displayTitle: '',
+ safeAreaTabBar: false,
+ },
+ methods: {
+ rerender() {
+ wx.nextTick(() => {
+ var _a;
+ (_a = this.parent) === null || _a === void 0 ? void 0 : _a.updateItemListData();
+ });
+ },
+ updateDataFromParent() {
+ if (this.parent) {
+ const { overlay, duration, activeColor, closeOnClickOverlay, direction, safeAreaTabBar, } = this.parent.data;
+ this.setData({
+ overlay,
+ duration,
+ activeColor,
+ closeOnClickOverlay,
+ direction,
+ safeAreaTabBar,
+ });
+ }
+ },
+ onOpen() {
+ this.$emit('open');
+ },
+ onOpened() {
+ this.$emit('opened');
+ },
+ onClose() {
+ this.$emit('close');
+ },
+ onClosed() {
+ this.$emit('closed');
+ this.setData({ showWrapper: false });
+ },
+ onOptionTap(event) {
+ const { option } = event.currentTarget.dataset;
+ const { value } = option;
+ const shouldEmitChange = this.data.value !== value;
+ this.setData({ showPopup: false, value });
+ this.$emit('close');
+ this.rerender();
+ if (shouldEmitChange) {
+ this.$emit('change', value);
+ }
+ },
+ toggle(show, options = {}) {
+ const { showPopup } = this.data;
+ if (typeof show !== 'boolean') {
+ show = !showPopup;
+ }
+ if (show === showPopup) {
+ return;
+ }
+ this.onBeforeToggle(show).then((status) => {
+ var _a;
+ if (!status) {
+ return;
+ }
+ this.setData({
+ transition: !options.immediate,
+ showPopup: show,
+ });
+ if (show) {
+ (_a = this.parent) === null || _a === void 0 ? void 0 : _a.getChildWrapperStyle().then((wrapperStyle) => {
+ this.setData({ wrapperStyle, showWrapper: true });
+ this.rerender();
+ });
+ }
+ else {
+ this.rerender();
+ }
+ });
+ },
+ onBeforeToggle(status) {
+ const { useBeforeToggle } = this.data;
+ if (!useBeforeToggle) {
+ return Promise.resolve(true);
+ }
+ return new Promise((resolve) => {
+ this.$emit('before-toggle', {
+ status,
+ callback: (value) => resolve(value),
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.json
new file mode 100644
index 0000000..88d5409
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxml
new file mode 100644
index 0000000..63904f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxml
@@ -0,0 +1,50 @@
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxss
new file mode 100644
index 0000000..80505e9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-item{left:0;overflow:hidden;position:fixed;right:0}.van-dropdown-item__option{text-align:left}.van-dropdown-item__option--active .van-dropdown-item__icon,.van-dropdown-item__option--active .van-dropdown-item__title{color:var(--dropdown-menu-option-active-color,#ee0a24)}.van-dropdown-item--up{top:0}.van-dropdown-item--down{bottom:0}.van-dropdown-item__icon{display:block;line-height:inherit}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.d.ts
new file mode 100644
index 0000000..774eb4c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.d.ts
@@ -0,0 +1,5 @@
+export interface Option {
+ text: string;
+ value: string | number;
+ icon: string;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.js
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-item/shared.js
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.js
new file mode 100644
index 0000000..9858ab3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.js
@@ -0,0 +1,117 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+import { addUnit, getRect, getSystemInfoSync } from '../common/utils';
+let ARRAY = [];
+VantComponent({
+ field: true,
+ classes: ['title-class'],
+ relation: useChildren('dropdown-item', function () {
+ this.updateItemListData();
+ }),
+ props: {
+ activeColor: {
+ type: String,
+ observer: 'updateChildrenData',
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ zIndex: {
+ type: Number,
+ value: 10,
+ },
+ duration: {
+ type: Number,
+ value: 200,
+ observer: 'updateChildrenData',
+ },
+ direction: {
+ type: String,
+ value: 'down',
+ observer: 'updateChildrenData',
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ closeOnClickOutside: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ itemListData: [],
+ },
+ beforeCreate() {
+ const { windowHeight } = getSystemInfoSync();
+ this.windowHeight = windowHeight;
+ ARRAY.push(this);
+ },
+ destroyed() {
+ ARRAY = ARRAY.filter((item) => item !== this);
+ },
+ methods: {
+ updateItemListData() {
+ this.setData({
+ itemListData: this.children.map((child) => child.data),
+ });
+ },
+ updateChildrenData() {
+ this.children.forEach((child) => {
+ child.updateDataFromParent();
+ });
+ },
+ toggleItem(active) {
+ this.children.forEach((item, index) => {
+ const { showPopup } = item.data;
+ if (index === active) {
+ item.toggle();
+ }
+ else if (showPopup) {
+ item.toggle(false, { immediate: true });
+ }
+ });
+ },
+ close() {
+ this.children.forEach((child) => {
+ child.toggle(false, { immediate: true });
+ });
+ },
+ getChildWrapperStyle() {
+ const { zIndex, direction } = this.data;
+ return getRect(this, '.van-dropdown-menu').then((rect) => {
+ const { top = 0, bottom = 0 } = rect;
+ const offset = direction === 'down' ? bottom : this.windowHeight - top;
+ let wrapperStyle = `z-index: ${zIndex};`;
+ if (direction === 'down') {
+ wrapperStyle += `top: ${addUnit(offset)};`;
+ }
+ else {
+ wrapperStyle += `bottom: ${addUnit(offset)};`;
+ }
+ return wrapperStyle;
+ });
+ },
+ onTitleTap(event) {
+ const { index } = event.currentTarget.dataset;
+ const child = this.children[index];
+ if (!child.data.disabled) {
+ ARRAY.forEach((menuItem) => {
+ if (menuItem &&
+ menuItem.data.closeOnClickOutside &&
+ menuItem !== this) {
+ menuItem.close();
+ }
+ });
+ this.toggleItem(index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxml
new file mode 100644
index 0000000..ec165a9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxs
new file mode 100644
index 0000000..6538854
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxs
@@ -0,0 +1,16 @@
+/* eslint-disable */
+function displayTitle(item) {
+ if (item.title) {
+ return item.title;
+ }
+
+ var match = item.options.filter(function(option) {
+ return option.value === item.value;
+ });
+ var displayTitle = match.length ? match[0].text : '';
+ return displayTitle;
+}
+
+module.exports = {
+ displayTitle: displayTitle
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxss
new file mode 100644
index 0000000..dba000e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/dropdown-menu/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-menu{background-color:var(--dropdown-menu-background-color,#fff);box-shadow:var(--dropdown-menu-box-shadow,0 2px 12px hsla(210,1%,40%,.12));display:flex;height:var(--dropdown-menu-height,50px);-webkit-user-select:none;user-select:none}.van-dropdown-menu__item{align-items:center;display:flex;flex:1;justify-content:center;min-width:0}.van-dropdown-menu__item:active{opacity:.7}.van-dropdown-menu__item--disabled:active{opacity:1}.van-dropdown-menu__item--disabled .van-dropdown-menu__title{color:var(--dropdown-menu-title-disabled-text-color,#969799)}.van-dropdown-menu__title{box-sizing:border-box;color:var(--dropdown-menu-title-text-color,#323233);font-size:var(--dropdown-menu-title-font-size,15px);line-height:var(--dropdown-menu-title-line-height,18px);max-width:100%;padding:var(--dropdown-menu-title-padding,0 24px 0 8px);position:relative}.van-dropdown-menu__title:after{border-color:transparent transparent currentcolor currentcolor;border-style:solid;border-width:3px;content:"";margin-top:-5px;opacity:.8;position:absolute;right:11px;top:50%;transform:rotate(-45deg)}.van-dropdown-menu__title--active{color:var(--dropdown-menu-title-active-text-color,#ee0a24)}.van-dropdown-menu__title--down:after{margin-top:-1px;transform:rotate(135deg)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.js
new file mode 100644
index 0000000..842e1bb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.js
@@ -0,0 +1,10 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ description: String,
+ image: {
+ type: String,
+ value: 'default',
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxml
new file mode 100644
index 0000000..9c7b719
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxs
new file mode 100644
index 0000000..cf92ece
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var PRESETS = ['error', 'search', 'default', 'network'];
+
+function imageUrl(image) {
+ if (PRESETS.indexOf(image) !== -1) {
+ return 'https://img.yzcdn.cn/vant/empty-image-' + image + '.png';
+ }
+
+ return image;
+}
+
+module.exports = {
+ imageUrl: imageUrl,
+};
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxss
new file mode 100644
index 0000000..0fb74fe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/empty/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-empty{align-items:center;box-sizing:border-box;display:flex;flex-direction:column;justify-content:center;padding:32px 0}.van-empty__image{height:160px;width:160px}.van-empty__image:empty{display:none}.van-empty__image__img{height:100%;width:100%}.van-empty__image:not(:empty)+.van-empty__image{display:none}.van-empty__description{color:#969799;font-size:14px;line-height:20px;margin-top:16px;padding:0 60px}.van-empty__description:empty,.van-empty__description:not(:empty)+.van-empty__description{display:none}.van-empty__bottom{margin-top:24px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.js
new file mode 100644
index 0000000..dba92e0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.js
@@ -0,0 +1,123 @@
+import { nextTick } from '../common/utils';
+import { VantComponent } from '../common/component';
+import { commonProps, inputProps, textareaProps } from './props';
+VantComponent({
+ field: true,
+ classes: ['input-class', 'right-icon-class', 'label-class'],
+ props: Object.assign(Object.assign(Object.assign(Object.assign({}, commonProps), inputProps), textareaProps), { size: String, icon: String, label: String, error: Boolean, center: Boolean, isLink: Boolean, leftIcon: String, rightIcon: String, autosize: null, required: Boolean, iconClass: String, clickable: Boolean, inputAlign: String, customStyle: String, errorMessage: String, arrowDirection: String, showWordLimit: Boolean, errorMessageAlign: String, readonly: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearable: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearTrigger: {
+ type: String,
+ value: 'focus',
+ }, border: {
+ type: Boolean,
+ value: true,
+ }, titleWidth: {
+ type: String,
+ value: '6.2em',
+ }, clearIcon: {
+ type: String,
+ value: 'clear',
+ }, extraEventParams: {
+ type: Boolean,
+ value: false,
+ } }),
+ data: {
+ focused: false,
+ innerValue: '',
+ showClear: false,
+ },
+ created() {
+ this.value = this.data.value;
+ this.setData({ innerValue: this.value });
+ },
+ methods: {
+ formatValue(value) {
+ const { maxlength } = this.data;
+ if (maxlength !== -1 && value.length > maxlength) {
+ return value.slice(0, maxlength);
+ }
+ return value;
+ },
+ onInput(event) {
+ const { value = '' } = event.detail || {};
+ const formatValue = this.formatValue(value);
+ this.value = formatValue;
+ this.setShowClear();
+ return this.emitChange(Object.assign(Object.assign({}, event.detail), { value: formatValue }));
+ },
+ onFocus(event) {
+ this.focused = true;
+ this.setShowClear();
+ this.$emit('focus', event.detail);
+ },
+ onBlur(event) {
+ this.focused = false;
+ this.setShowClear();
+ this.$emit('blur', event.detail);
+ },
+ onClickIcon() {
+ this.$emit('click-icon');
+ },
+ onClickInput(event) {
+ this.$emit('click-input', event.detail);
+ },
+ onClear() {
+ this.setData({ innerValue: '' });
+ this.value = '';
+ this.setShowClear();
+ nextTick(() => {
+ this.emitChange({ value: '' });
+ this.$emit('clear', '');
+ });
+ },
+ onConfirm(event) {
+ const { value = '' } = event.detail || {};
+ this.value = value;
+ this.setShowClear();
+ this.$emit('confirm', value);
+ },
+ setValue(value) {
+ this.value = value;
+ this.setShowClear();
+ if (value === '') {
+ this.setData({ innerValue: '' });
+ }
+ this.emitChange({ value });
+ },
+ onLineChange(event) {
+ this.$emit('linechange', event.detail);
+ },
+ onKeyboardHeightChange(event) {
+ this.$emit('keyboardheightchange', event.detail);
+ },
+ emitChange(detail) {
+ const { extraEventParams } = this.data;
+ this.setData({ value: detail.value });
+ let result;
+ const data = extraEventParams
+ ? Object.assign(Object.assign({}, detail), { callback: (data) => {
+ result = data;
+ } }) : detail.value;
+ this.$emit('input', data);
+ this.$emit('change', data);
+ return result;
+ },
+ setShowClear() {
+ const { clearable, readonly, clearTrigger } = this.data;
+ const { focused, value } = this;
+ let showClear = false;
+ if (clearable && !readonly) {
+ const hasValue = !!value;
+ const trigger = clearTrigger === 'always' || (clearTrigger === 'focus' && focused);
+ showClear = hasValue && trigger;
+ }
+ this.setData({ showClear });
+ },
+ noop() { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.json
new file mode 100644
index 0000000..5906c50
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxml
new file mode 100644
index 0000000..6018993
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxs
new file mode 100644
index 0000000..78575b9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function inputStyle(autosize) {
+ if (autosize && autosize.constructor === 'Object') {
+ return style({
+ 'min-height': addUnit(autosize.minHeight),
+ 'max-height': addUnit(autosize.maxHeight),
+ });
+ }
+
+ return '';
+}
+
+module.exports = {
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxss
new file mode 100644
index 0000000..5f7d306
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-field{--cell-icon-size:var(--field-icon-size,16px)}.van-field__label{color:var(--field-label-color,#646566)}.van-field__label--disabled{color:var(--field-disabled-text-color,#c8c9cc)}.van-field__body{align-items:center;display:flex}.van-field__body--textarea{box-sizing:border-box;line-height:1.2em;min-height:var(--cell-line-height,24px);padding:3.6px 0}.van-field__control:empty+.van-field__control{display:block}.van-field__control{background-color:initial;border:0;box-sizing:border-box;color:var(--field-input-text-color,#323233);display:none;height:var(--cell-line-height,24px);line-height:inherit;margin:0;min-height:var(--cell-line-height,24px);padding:0;position:relative;resize:none;text-align:left;width:100%}.van-field__control:empty{display:none}.van-field__control--textarea{height:var(--field-text-area-min-height,18px);min-height:var(--field-text-area-min-height,18px)}.van-field__control--error{color:var(--field-input-error-text-color,#ee0a24)}.van-field__control--disabled{background-color:initial;color:var(--field-input-disabled-text-color,#c8c9cc);opacity:1}.van-field__control--center{text-align:center}.van-field__control--right{text-align:right}.van-field__control--custom{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__placeholder{color:var(--field-placeholder-text-color,#c8c9cc);left:0;pointer-events:none;position:absolute;right:0;top:0}.van-field__placeholder--error{color:var(--field-error-message-color,#ee0a24)}.van-field__icon-root{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__clear-root,.van-field__icon-container{line-height:inherit;margin-right:calc(var(--padding-xs, 8px)*-1);padding:0 var(--padding-xs,8px);vertical-align:middle}.van-field__button,.van-field__clear-root,.van-field__icon-container{flex-shrink:0}.van-field__clear-root{color:var(--field-clear-icon-color,#c8c9cc);font-size:var(--field-clear-icon-size,16px)}.van-field__icon-container{color:var(--field-icon-container-color,#969799);font-size:var(--field-icon-size,16px)}.van-field__icon-container:empty{display:none}.van-field__button{padding-left:var(--padding-xs,8px)}.van-field__button:empty{display:none}.van-field__error-message{color:var(--field-error-message-color,#ee0a24);display:block;font-size:var(--field-error-message-text-font-size,12px);text-align:left}.van-field__error-message--center{text-align:center}.van-field__error-message--right{text-align:right}.van-field__word-limit{color:var(--field-word-limit-color,#646566);font-size:var(--field-word-limit-font-size,12px);line-height:var(--field-word-limit-line-height,16px);margin-top:var(--padding-base,4px);text-align:right}.van-field__word-num{display:inline}.van-field__word-num--full{color:var(--field-word-num-full-color,#ee0a24)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/input.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/input.wxml
new file mode 100644
index 0000000..c10f4c4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/input.wxml
@@ -0,0 +1,30 @@
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.d.ts
new file mode 100644
index 0000000..5cd130a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.d.ts
@@ -0,0 +1,4 @@
+///
+export declare const commonProps: WechatMiniprogram.Component.PropertyOption;
+export declare const inputProps: WechatMiniprogram.Component.PropertyOption;
+export declare const textareaProps: WechatMiniprogram.Component.PropertyOption;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.js
new file mode 100644
index 0000000..ae405b3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/props.js
@@ -0,0 +1,64 @@
+export const commonProps = {
+ value: {
+ type: String,
+ observer(value) {
+ if (value !== this.value) {
+ this.setData({ innerValue: value });
+ this.value = value;
+ }
+ },
+ },
+ placeholder: String,
+ placeholderStyle: String,
+ placeholderClass: String,
+ disabled: Boolean,
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 50,
+ },
+ autoFocus: Boolean,
+ focus: Boolean,
+ cursor: {
+ type: Number,
+ value: -1,
+ },
+ selectionStart: {
+ type: Number,
+ value: -1,
+ },
+ selectionEnd: {
+ type: Number,
+ value: -1,
+ },
+ adjustPosition: {
+ type: Boolean,
+ value: true,
+ },
+ holdKeyboard: Boolean,
+};
+export const inputProps = {
+ type: {
+ type: String,
+ value: 'text',
+ },
+ password: Boolean,
+ confirmType: String,
+ confirmHold: Boolean,
+ alwaysEmbed: Boolean,
+};
+export const textareaProps = {
+ autoHeight: Boolean,
+ fixed: Boolean,
+ showConfirmBar: {
+ type: Boolean,
+ value: true,
+ },
+ disableDefaultPadding: {
+ type: Boolean,
+ value: true,
+ },
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/textarea.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/textarea.wxml
new file mode 100644
index 0000000..945d03e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/textarea.wxml
@@ -0,0 +1,32 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.d.ts
new file mode 100644
index 0000000..357ccbe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.d.ts
@@ -0,0 +1,8 @@
+export interface InputDetails {
+ /** 输入框内容 */
+ value: string;
+ /** 光标位置 */
+ cursor?: number;
+ /** keyCode 为键值 (目前工具还不支持返回keyCode参数) `2.1.0` 起支持 */
+ keyCode?: number;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.js
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/field/types.js
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.js
new file mode 100644
index 0000000..10a219a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.js
@@ -0,0 +1,44 @@
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+import { button } from '../mixins/button';
+import { link } from '../mixins/link';
+VantComponent({
+ mixins: [link, button],
+ relation: useParent('goods-action'),
+ props: {
+ text: String,
+ color: String,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loading: Boolean,
+ disabled: Boolean,
+ plain: Boolean,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ customStyle: {
+ type: String,
+ value: '',
+ },
+ },
+ methods: {
+ onClick(event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ updateStyle() {
+ if (this.parent == null) {
+ return;
+ }
+ const { index } = this;
+ const { children = [] } = this.parent;
+ this.setData({
+ isFirst: index === 0,
+ isLast: index === children.length - 1,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.json
new file mode 100644
index 0000000..b567686
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxml
new file mode 100644
index 0000000..4530ab8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxml
@@ -0,0 +1,35 @@
+
+
+ {{ text }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxss
new file mode 100644
index 0000000..759a1d9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-goods-action-button{--button-warning-background-color:var(--goods-action-button-warning-color,linear-gradient(to right,#ffd01e,#ff8917));--button-danger-background-color:var(--goods-action-button-danger-color,linear-gradient(to right,#ff6034,#ee0a24));--button-default-height:var(--goods-action-button-height,40px);--button-line-height:var(--goods-action-button-line-height,20px);--button-plain-background-color:var(--goods-action-button-plain-color,#fff);--button-border-width:0;display:block}.van-goods-action-button--first{--button-border-radius:999px 0 0 var(--goods-action-button-border-radius,999px);margin-left:5px}.van-goods-action-button--last{--button-border-radius:0 999px var(--goods-action-button-border-radius,999px) 0;margin-right:5px}.van-goods-action-button--first.van-goods-action-button--last{--button-border-radius:var(--goods-action-button-border-radius,999px)}.van-goods-action-button--plain{--button-border-width:1px}.van-goods-action-button__inner{font-weight:var(--font-weight-bold,500)!important;width:100%}@media (max-width:321px){.van-goods-action-button{font-size:13px}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.js
new file mode 100644
index 0000000..98ed74f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.js
@@ -0,0 +1,27 @@
+import { VantComponent } from '../common/component';
+import { button } from '../mixins/button';
+import { link } from '../mixins/link';
+VantComponent({
+ classes: ['icon-class', 'text-class', 'info-class'],
+ mixins: [link, button],
+ props: {
+ text: String,
+ dot: Boolean,
+ info: String,
+ icon: String,
+ size: String,
+ color: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ disabled: Boolean,
+ loading: Boolean,
+ },
+ methods: {
+ onClick(event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.json
new file mode 100644
index 0000000..93bfe8a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxml
new file mode 100644
index 0000000..30c1a8c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxss
new file mode 100644
index 0000000..6e4758d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action-icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action-icon{border:none!important;color:var(--goods-action-icon-text-color,#646566)!important;display:flex!important;flex-direction:column;font-size:var(--goods-action-icon-font-size,10px)!important;height:var(--goods-action-icon-height,50px)!important;justify-content:center!important;line-height:1!important;min-width:var(--goods-action-icon-width,48px)}.van-goods-action-icon__icon{color:var(--goods-action-icon-color,#323233);display:flex;font-size:var(--goods-action-icon-size,18px);margin:0 auto 5px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.js
new file mode 100644
index 0000000..6b2ed74
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.js
@@ -0,0 +1,15 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ relation: useChildren('goods-action-button', function () {
+ this.children.forEach((item) => {
+ item.updateStyle();
+ });
+ }),
+ props: {
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxml
new file mode 100644
index 0000000..569450c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxss
new file mode 100644
index 0000000..7793e77
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/goods-action/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action{align-items:center;background-color:var(--goods-action-background-color,#fff);bottom:0;box-sizing:initial;display:flex;height:var(--goods-action-height,50px);left:0;position:fixed;right:0}.van-goods-action--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.js
new file mode 100644
index 0000000..dbeb18a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.js
@@ -0,0 +1,52 @@
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+import { link } from '../mixins/link';
+VantComponent({
+ relation: useParent('grid'),
+ classes: ['content-class', 'icon-class', 'text-class'],
+ mixins: [link],
+ props: {
+ icon: String,
+ iconColor: String,
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ dot: Boolean,
+ info: null,
+ badge: null,
+ text: String,
+ useSlot: Boolean,
+ },
+ data: {
+ viewStyle: '',
+ },
+ mounted() {
+ this.updateStyle();
+ },
+ methods: {
+ updateStyle() {
+ if (!this.parent) {
+ return;
+ }
+ const { data, children } = this.parent;
+ const { columnNum, border, square, gutter, clickable, center, direction, reverse, iconSize, } = data;
+ this.setData({
+ center,
+ border,
+ square,
+ gutter,
+ clickable,
+ direction,
+ reverse,
+ iconSize,
+ index: children.indexOf(this),
+ columnNum,
+ });
+ },
+ onClick() {
+ this.$emit('click');
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxml
new file mode 100644
index 0000000..e95087d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxs
new file mode 100644
index 0000000..2cfe37d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapperStyle(data) {
+ var width = 100 / data.columnNum + '%';
+
+ return style({
+ width: width,
+ 'padding-top': data.square ? width : null,
+ 'padding-right': addUnit(data.gutter),
+ 'margin-top':
+ data.index >= data.columnNum && !data.square
+ ? addUnit(data.gutter)
+ : null,
+ });
+}
+
+function contentStyle(data) {
+ return data.square
+ ? style({
+ right: addUnit(data.gutter),
+ bottom: addUnit(data.gutter),
+ height: 'auto',
+ })
+ : '';
+}
+
+module.exports = {
+ wrapperStyle: wrapperStyle,
+ contentStyle: contentStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxss
new file mode 100644
index 0000000..acaea84
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid-item{box-sizing:border-box;float:left;position:relative}.van-grid-item--square{height:0}.van-grid-item__content{background-color:var(--grid-item-content-background-color,#fff);box-sizing:border-box;display:flex;flex-direction:column;height:100%;padding:var(--grid-item-content-padding,16px 8px)}.van-grid-item__content:after{border-width:0 1px 1px 0;z-index:1}.van-grid-item__content--surround:after{border-width:1px}.van-grid-item__content--center{align-items:center;justify-content:center}.van-grid-item__content--square{left:0;position:absolute;right:0;top:0}.van-grid-item__content--horizontal{flex-direction:row}.van-grid-item__content--horizontal .van-grid-item__text{margin:0 0 0 8px}.van-grid-item__content--reverse{flex-direction:column-reverse}.van-grid-item__content--reverse .van-grid-item__text{margin:0 0 8px}.van-grid-item__content--horizontal.van-grid-item__content--reverse{flex-direction:row-reverse}.van-grid-item__content--horizontal.van-grid-item__content--reverse .van-grid-item__text{margin:0 8px 0 0}.van-grid-item__content--clickable:active{background-color:var(--grid-item-content-active-color,#f2f3f5)}.van-grid-item__icon{align-items:center;display:flex;font-size:var(--grid-item-icon-size,26px);height:var(--grid-item-icon-size,26px)}.van-grid-item__text{word-wrap:break-word;color:var(--grid-item-text-color,#646566);font-size:var(--grid-item-text-font-size,12px)}.van-grid-item__icon+.van-grid-item__text{margin-top:8px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.js
new file mode 100644
index 0000000..41dfa4c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.js
@@ -0,0 +1,55 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ relation: useChildren('grid-item'),
+ props: {
+ square: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ gutter: {
+ type: null,
+ value: 0,
+ observer: 'updateChildren',
+ },
+ clickable: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ columnNum: {
+ type: Number,
+ value: 4,
+ observer: 'updateChildren',
+ },
+ center: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ iconSize: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ reverse: {
+ type: Boolean,
+ value: false,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren() {
+ this.children.forEach((child) => {
+ child.updateStyle();
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxml
new file mode 100644
index 0000000..2e4118f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxs
new file mode 100644
index 0000000..cd3b1bd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'padding-left': addUnit(data.gutter),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxss
new file mode 100644
index 0000000..e347440
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/grid/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid{box-sizing:border-box;overflow:hidden;position:relative}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.js
new file mode 100644
index 0000000..01c9073
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.js
@@ -0,0 +1,21 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: ['info-class'],
+ props: {
+ dot: Boolean,
+ info: null,
+ size: null,
+ color: String,
+ customStyle: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ name: String,
+ },
+ methods: {
+ onClick() {
+ this.$emit('click');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxml
new file mode 100644
index 0000000..91b47f9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxs
new file mode 100644
index 0000000..a906f76
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxs
@@ -0,0 +1,43 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isImage(name) {
+ return name.indexOf('/') !== -1;
+}
+
+function rootClass(data) {
+ var classes = ['custom-class'];
+
+ if (data.classPrefix !== 'van-icon') {
+ classes.push('van-icon--custom')
+ }
+
+ if (data.classPrefix != null) {
+ classes.push(data.classPrefix);
+ }
+
+ if (isImage(data.name)) {
+ classes.push('van-icon--image');
+ } else if (data.classPrefix != null) {
+ classes.push(data.classPrefix + '-' + data.name);
+ }
+
+ return classes.join(' ');
+}
+
+function rootStyle(data) {
+ return style([
+ {
+ color: data.color,
+ 'font-size': addUnit(data.size),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ isImage: isImage,
+ rootClass: rootClass,
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxss
new file mode 100644
index 0000000..feb3d7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-icon{text-rendering:auto;-webkit-font-smoothing:antialiased;font:normal normal normal 14px/1 vant-icon;font:normal normal normal 14px/1 var(--van-icon-font-family,"vant-icon");font-size:inherit;position:relative}.van-icon,.van-icon:before{display:inline-block}.van-icon-contact:before{content:"\e753"}.van-icon-notes:before{content:"\e63c"}.van-icon-records:before{content:"\e63d"}.van-icon-cash-back-record:before{content:"\e63e"}.van-icon-newspaper:before{content:"\e63f"}.van-icon-discount:before{content:"\e640"}.van-icon-completed:before{content:"\e641"}.van-icon-user:before{content:"\e642"}.van-icon-description:before{content:"\e643"}.van-icon-list-switch:before{content:"\e6ad"}.van-icon-list-switching:before{content:"\e65a"}.van-icon-link-o:before{content:"\e751"}.van-icon-miniprogram-o:before{content:"\e752"}.van-icon-qq:before{content:"\e74e"}.van-icon-wechat-moments:before{content:"\e74f"}.van-icon-weibo:before{content:"\e750"}.van-icon-cash-o:before{content:"\e74d"}.van-icon-guide-o:before{content:"\e74c"}.van-icon-invitation:before{content:"\e6d6"}.van-icon-shield-o:before{content:"\e74b"}.van-icon-exchange:before{content:"\e6af"}.van-icon-eye:before{content:"\e6b0"}.van-icon-enlarge:before{content:"\e6b1"}.van-icon-expand-o:before{content:"\e6b2"}.van-icon-eye-o:before{content:"\e6b3"}.van-icon-expand:before{content:"\e6b4"}.van-icon-filter-o:before{content:"\e6b5"}.van-icon-fire:before{content:"\e6b6"}.van-icon-fail:before{content:"\e6b7"}.van-icon-failure:before{content:"\e6b8"}.van-icon-fire-o:before{content:"\e6b9"}.van-icon-flag-o:before{content:"\e6ba"}.van-icon-font:before{content:"\e6bb"}.van-icon-font-o:before{content:"\e6bc"}.van-icon-gem-o:before{content:"\e6bd"}.van-icon-flower-o:before{content:"\e6be"}.van-icon-gem:before{content:"\e6bf"}.van-icon-gift-card:before{content:"\e6c0"}.van-icon-friends:before{content:"\e6c1"}.van-icon-friends-o:before{content:"\e6c2"}.van-icon-gold-coin:before{content:"\e6c3"}.van-icon-gold-coin-o:before{content:"\e6c4"}.van-icon-good-job-o:before{content:"\e6c5"}.van-icon-gift:before{content:"\e6c6"}.van-icon-gift-o:before{content:"\e6c7"}.van-icon-gift-card-o:before{content:"\e6c8"}.van-icon-good-job:before{content:"\e6c9"}.van-icon-home-o:before{content:"\e6ca"}.van-icon-goods-collect:before{content:"\e6cb"}.van-icon-graphic:before{content:"\e6cc"}.van-icon-goods-collect-o:before{content:"\e6cd"}.van-icon-hot-o:before{content:"\e6ce"}.van-icon-info:before{content:"\e6cf"}.van-icon-hotel-o:before{content:"\e6d0"}.van-icon-info-o:before{content:"\e6d1"}.van-icon-hot-sale-o:before{content:"\e6d2"}.van-icon-hot:before{content:"\e6d3"}.van-icon-like:before{content:"\e6d4"}.van-icon-idcard:before{content:"\e6d5"}.van-icon-like-o:before{content:"\e6d7"}.van-icon-hot-sale:before{content:"\e6d8"}.van-icon-location-o:before{content:"\e6d9"}.van-icon-location:before{content:"\e6da"}.van-icon-label:before{content:"\e6db"}.van-icon-lock:before{content:"\e6dc"}.van-icon-label-o:before{content:"\e6dd"}.van-icon-map-marked:before{content:"\e6de"}.van-icon-logistics:before{content:"\e6df"}.van-icon-manager:before{content:"\e6e0"}.van-icon-more:before{content:"\e6e1"}.van-icon-live:before{content:"\e6e2"}.van-icon-manager-o:before{content:"\e6e3"}.van-icon-medal:before{content:"\e6e4"}.van-icon-more-o:before{content:"\e6e5"}.van-icon-music-o:before{content:"\e6e6"}.van-icon-music:before{content:"\e6e7"}.van-icon-new-arrival-o:before{content:"\e6e8"}.van-icon-medal-o:before{content:"\e6e9"}.van-icon-new-o:before{content:"\e6ea"}.van-icon-free-postage:before{content:"\e6eb"}.van-icon-newspaper-o:before{content:"\e6ec"}.van-icon-new-arrival:before{content:"\e6ed"}.van-icon-minus:before{content:"\e6ee"}.van-icon-orders-o:before{content:"\e6ef"}.van-icon-new:before{content:"\e6f0"}.van-icon-paid:before{content:"\e6f1"}.van-icon-notes-o:before{content:"\e6f2"}.van-icon-other-pay:before{content:"\e6f3"}.van-icon-pause-circle:before{content:"\e6f4"}.van-icon-pause:before{content:"\e6f5"}.van-icon-pause-circle-o:before{content:"\e6f6"}.van-icon-peer-pay:before{content:"\e6f7"}.van-icon-pending-payment:before{content:"\e6f8"}.van-icon-passed:before{content:"\e6f9"}.van-icon-plus:before{content:"\e6fa"}.van-icon-phone-circle-o:before{content:"\e6fb"}.van-icon-phone-o:before{content:"\e6fc"}.van-icon-printer:before{content:"\e6fd"}.van-icon-photo-fail:before{content:"\e6fe"}.van-icon-phone:before{content:"\e6ff"}.van-icon-photo-o:before{content:"\e700"}.van-icon-play-circle:before{content:"\e701"}.van-icon-play:before{content:"\e702"}.van-icon-phone-circle:before{content:"\e703"}.van-icon-point-gift-o:before{content:"\e704"}.van-icon-point-gift:before{content:"\e705"}.van-icon-play-circle-o:before{content:"\e706"}.van-icon-shrink:before{content:"\e707"}.van-icon-photo:before{content:"\e708"}.van-icon-qr:before{content:"\e709"}.van-icon-qr-invalid:before{content:"\e70a"}.van-icon-question-o:before{content:"\e70b"}.van-icon-revoke:before{content:"\e70c"}.van-icon-replay:before{content:"\e70d"}.van-icon-service:before{content:"\e70e"}.van-icon-question:before{content:"\e70f"}.van-icon-search:before{content:"\e710"}.van-icon-refund-o:before{content:"\e711"}.van-icon-service-o:before{content:"\e712"}.van-icon-scan:before{content:"\e713"}.van-icon-share:before{content:"\e714"}.van-icon-send-gift-o:before{content:"\e715"}.van-icon-share-o:before{content:"\e716"}.van-icon-setting:before{content:"\e717"}.van-icon-points:before{content:"\e718"}.van-icon-photograph:before{content:"\e719"}.van-icon-shop:before{content:"\e71a"}.van-icon-shop-o:before{content:"\e71b"}.van-icon-shop-collect-o:before{content:"\e71c"}.van-icon-shop-collect:before{content:"\e71d"}.van-icon-smile:before{content:"\e71e"}.van-icon-shopping-cart-o:before{content:"\e71f"}.van-icon-sign:before{content:"\e720"}.van-icon-sort:before{content:"\e721"}.van-icon-star-o:before{content:"\e722"}.van-icon-smile-comment-o:before{content:"\e723"}.van-icon-stop:before{content:"\e724"}.van-icon-stop-circle-o:before{content:"\e725"}.van-icon-smile-o:before{content:"\e726"}.van-icon-star:before{content:"\e727"}.van-icon-success:before{content:"\e728"}.van-icon-stop-circle:before{content:"\e729"}.van-icon-records-o:before{content:"\e72a"}.van-icon-shopping-cart:before{content:"\e72b"}.van-icon-tosend:before{content:"\e72c"}.van-icon-todo-list:before{content:"\e72d"}.van-icon-thumb-circle-o:before{content:"\e72e"}.van-icon-thumb-circle:before{content:"\e72f"}.van-icon-umbrella-circle:before{content:"\e730"}.van-icon-underway:before{content:"\e731"}.van-icon-upgrade:before{content:"\e732"}.van-icon-todo-list-o:before{content:"\e733"}.van-icon-tv-o:before{content:"\e734"}.van-icon-underway-o:before{content:"\e735"}.van-icon-user-o:before{content:"\e736"}.van-icon-vip-card-o:before{content:"\e737"}.van-icon-vip-card:before{content:"\e738"}.van-icon-send-gift:before{content:"\e739"}.van-icon-wap-home:before{content:"\e73a"}.van-icon-wap-nav:before{content:"\e73b"}.van-icon-volume-o:before{content:"\e73c"}.van-icon-video:before{content:"\e73d"}.van-icon-wap-home-o:before{content:"\e73e"}.van-icon-volume:before{content:"\e73f"}.van-icon-warning:before{content:"\e740"}.van-icon-weapp-nav:before{content:"\e741"}.van-icon-wechat-pay:before{content:"\e742"}.van-icon-warning-o:before{content:"\e743"}.van-icon-wechat:before{content:"\e744"}.van-icon-setting-o:before{content:"\e745"}.van-icon-youzan-shield:before{content:"\e746"}.van-icon-warn-o:before{content:"\e747"}.van-icon-smile-comment:before{content:"\e748"}.van-icon-user-circle-o:before{content:"\e749"}.van-icon-video-o:before{content:"\e74a"}.van-icon-add-square:before{content:"\e65c"}.van-icon-add:before{content:"\e65d"}.van-icon-arrow-down:before{content:"\e65e"}.van-icon-arrow-up:before{content:"\e65f"}.van-icon-arrow:before{content:"\e660"}.van-icon-after-sale:before{content:"\e661"}.van-icon-add-o:before{content:"\e662"}.van-icon-alipay:before{content:"\e663"}.van-icon-ascending:before{content:"\e664"}.van-icon-apps-o:before{content:"\e665"}.van-icon-aim:before{content:"\e666"}.van-icon-award:before{content:"\e667"}.van-icon-arrow-left:before{content:"\e668"}.van-icon-award-o:before{content:"\e669"}.van-icon-audio:before{content:"\e66a"}.van-icon-bag-o:before{content:"\e66b"}.van-icon-balance-list:before{content:"\e66c"}.van-icon-back-top:before{content:"\e66d"}.van-icon-bag:before{content:"\e66e"}.van-icon-balance-pay:before{content:"\e66f"}.van-icon-balance-o:before{content:"\e670"}.van-icon-bar-chart-o:before{content:"\e671"}.van-icon-bars:before{content:"\e672"}.van-icon-balance-list-o:before{content:"\e673"}.van-icon-birthday-cake-o:before{content:"\e674"}.van-icon-bookmark:before{content:"\e675"}.van-icon-bill:before{content:"\e676"}.van-icon-bell:before{content:"\e677"}.van-icon-browsing-history-o:before{content:"\e678"}.van-icon-browsing-history:before{content:"\e679"}.van-icon-bookmark-o:before{content:"\e67a"}.van-icon-bulb-o:before{content:"\e67b"}.van-icon-bullhorn-o:before{content:"\e67c"}.van-icon-bill-o:before{content:"\e67d"}.van-icon-calendar-o:before{content:"\e67e"}.van-icon-brush-o:before{content:"\e67f"}.van-icon-card:before{content:"\e680"}.van-icon-cart-o:before{content:"\e681"}.van-icon-cart-circle:before{content:"\e682"}.van-icon-cart-circle-o:before{content:"\e683"}.van-icon-cart:before{content:"\e684"}.van-icon-cash-on-deliver:before{content:"\e685"}.van-icon-cash-back-record-o:before{content:"\e686"}.van-icon-cashier-o:before{content:"\e687"}.van-icon-chart-trending-o:before{content:"\e688"}.van-icon-certificate:before{content:"\e689"}.van-icon-chat:before{content:"\e68a"}.van-icon-clear:before{content:"\e68b"}.van-icon-chat-o:before{content:"\e68c"}.van-icon-checked:before{content:"\e68d"}.van-icon-clock:before{content:"\e68e"}.van-icon-clock-o:before{content:"\e68f"}.van-icon-close:before{content:"\e690"}.van-icon-closed-eye:before{content:"\e691"}.van-icon-circle:before{content:"\e692"}.van-icon-cluster-o:before{content:"\e693"}.van-icon-column:before{content:"\e694"}.van-icon-comment-circle-o:before{content:"\e695"}.van-icon-cluster:before{content:"\e696"}.van-icon-comment:before{content:"\e697"}.van-icon-comment-o:before{content:"\e698"}.van-icon-comment-circle:before{content:"\e699"}.van-icon-completed-o:before{content:"\e69a"}.van-icon-credit-pay:before{content:"\e69b"}.van-icon-coupon:before{content:"\e69c"}.van-icon-debit-pay:before{content:"\e69d"}.van-icon-coupon-o:before{content:"\e69e"}.van-icon-contact-o:before{content:"\e69f"}.van-icon-descending:before{content:"\e6a0"}.van-icon-desktop-o:before{content:"\e6a1"}.van-icon-diamond-o:before{content:"\e6a2"}.van-icon-description-o:before{content:"\e6a3"}.van-icon-delete:before{content:"\e6a4"}.van-icon-diamond:before{content:"\e6a5"}.van-icon-delete-o:before{content:"\e6a6"}.van-icon-cross:before{content:"\e6a7"}.van-icon-edit:before{content:"\e6a8"}.van-icon-ellipsis:before{content:"\e6a9"}.van-icon-down:before{content:"\e6aa"}.van-icon-discount-o:before{content:"\e6ab"}.van-icon-ecard-pay:before{content:"\e6ac"}.van-icon-envelop-o:before{content:"\e6ae"}@font-face{font-display:auto;font-family:vant-icon;font-style:normal;font-weight:400;src:url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff2?t=1694918397022) format("woff2"),url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff?t=1694918397022) format("woff")}:host{align-items:center;display:inline-flex;justify-content:center}.van-icon--custom{position:relative}.van-icon--image{height:1em;width:1em}.van-icon__image{height:100%;width:100%}.van-icon__info{z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.js
new file mode 100644
index 0000000..a9d007e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.js
@@ -0,0 +1,64 @@
+import { VantComponent } from '../common/component';
+import { button } from '../mixins/button';
+VantComponent({
+ mixins: [button],
+ classes: ['custom-class', 'loading-class', 'error-class', 'image-class'],
+ props: {
+ src: {
+ type: String,
+ observer() {
+ this.setData({
+ error: false,
+ loading: true,
+ });
+ },
+ },
+ round: Boolean,
+ width: null,
+ height: null,
+ radius: null,
+ lazyLoad: Boolean,
+ useErrorSlot: Boolean,
+ useLoadingSlot: Boolean,
+ showMenuByLongpress: Boolean,
+ fit: {
+ type: String,
+ value: 'fill',
+ },
+ webp: {
+ type: Boolean,
+ value: false,
+ },
+ showError: {
+ type: Boolean,
+ value: true,
+ },
+ showLoading: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ error: false,
+ loading: true,
+ viewStyle: '',
+ },
+ methods: {
+ onLoad(event) {
+ this.setData({
+ loading: false,
+ });
+ this.$emit('load', event.detail);
+ },
+ onError(event) {
+ this.setData({
+ loading: false,
+ error: true,
+ });
+ this.$emit('error', event.detail);
+ },
+ onClick(event) {
+ this.$emit('click', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxml
new file mode 100644
index 0000000..a6f573c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxs
new file mode 100644
index 0000000..cec14b8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ width: addUnit(data.width),
+ height: addUnit(data.height),
+ 'border-radius': addUnit(data.radius),
+ },
+ data.radius ? 'overflow: hidden' : null,
+ ]);
+}
+
+var FIT_MODE_MAP = {
+ none: 'center',
+ fill: 'scaleToFill',
+ cover: 'aspectFill',
+ contain: 'aspectFit',
+ widthFix: 'widthFix',
+ heightFix: 'heightFix',
+};
+
+function mode(fit) {
+ return FIT_MODE_MAP[fit];
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ mode: mode,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxss
new file mode 100644
index 0000000..a9c6ebb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/image/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-image{display:inline-block;position:relative}.van-image--round{border-radius:50%;overflow:hidden}.van-image--round .van-image__img{border-radius:inherit}.van-image__error,.van-image__img,.van-image__loading{display:block;height:100%;width:100%}.van-image__error,.van-image__loading{align-items:center;background-color:var(--image-placeholder-background-color,#f7f8fa);color:var(--image-placeholder-text-color,#969799);display:flex;flex-direction:column;font-size:var(--image-placeholder-font-size,14px);justify-content:center;left:0;position:absolute;top:0}.van-image__loading-icon{color:var(--image-loading-icon-color,#dcdee0);font-size:var(--image-loading-icon-size,32px)!important}.van-image__error-icon{color:var(--image-error-icon-color,#dcdee0);font-size:var(--image-error-icon-size,32px)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.js
new file mode 100644
index 0000000..85265e9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.js
@@ -0,0 +1,25 @@
+import { getRect } from '../common/utils';
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+VantComponent({
+ relation: useParent('index-bar'),
+ props: {
+ useSlot: Boolean,
+ index: null,
+ },
+ data: {
+ active: false,
+ wrapperStyle: '',
+ anchorStyle: '',
+ },
+ methods: {
+ scrollIntoView(scrollTop) {
+ getRect(this, '.van-index-anchor-wrapper').then((rect) => {
+ wx.pageScrollTo({
+ duration: 0,
+ scrollTop: scrollTop + rect.top - this.parent.data.stickyOffsetTop,
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxml
new file mode 100644
index 0000000..49affa7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxml
@@ -0,0 +1,14 @@
+
+
+
+
+ {{ index }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxss
new file mode 100644
index 0000000..4b91560
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-anchor/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-anchor{background-color:var(--index-anchor-background-color,transparent);color:var(--index-anchor-text-color,#323233);font-size:var(--index-anchor-font-size,14px);font-weight:var(--index-anchor-font-weight,500);line-height:var(--index-anchor-line-height,32px);padding:var(--index-anchor-padding,0 16px)}.van-index-anchor--active{background-color:var(--index-anchor-active-background-color,#fff);color:var(--index-anchor-active-text-color,#07c160);left:0;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.js
new file mode 100644
index 0000000..c541b89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.js
@@ -0,0 +1,246 @@
+import { GREEN } from '../common/color';
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+import { getRect, isDef } from '../common/utils';
+import { pageScrollMixin } from '../mixins/page-scroll';
+const indexList = () => {
+ const indexList = [];
+ const charCodeOfA = 'A'.charCodeAt(0);
+ for (let i = 0; i < 26; i++) {
+ indexList.push(String.fromCharCode(charCodeOfA + i));
+ }
+ return indexList;
+};
+VantComponent({
+ relation: useChildren('index-anchor', function () {
+ this.updateData();
+ }),
+ props: {
+ sticky: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ highlightColor: {
+ type: String,
+ value: GREEN,
+ },
+ stickyOffsetTop: {
+ type: Number,
+ value: 0,
+ },
+ indexList: {
+ type: Array,
+ value: indexList(),
+ },
+ },
+ mixins: [
+ pageScrollMixin(function (event) {
+ this.scrollTop = (event === null || event === void 0 ? void 0 : event.scrollTop) || 0;
+ this.onScroll();
+ }),
+ ],
+ data: {
+ activeAnchorIndex: null,
+ showSidebar: false,
+ },
+ created() {
+ this.scrollTop = 0;
+ },
+ methods: {
+ updateData() {
+ wx.nextTick(() => {
+ if (this.timer != null) {
+ clearTimeout(this.timer);
+ }
+ this.timer = setTimeout(() => {
+ this.setData({
+ showSidebar: !!this.children.length,
+ });
+ this.setRect().then(() => {
+ this.onScroll();
+ });
+ }, 0);
+ });
+ },
+ setRect() {
+ return Promise.all([
+ this.setAnchorsRect(),
+ this.setListRect(),
+ this.setSiderbarRect(),
+ ]);
+ },
+ setAnchorsRect() {
+ return Promise.all(this.children.map((anchor) => getRect(anchor, '.van-index-anchor-wrapper').then((rect) => {
+ Object.assign(anchor, {
+ height: rect.height,
+ top: rect.top + this.scrollTop,
+ });
+ })));
+ },
+ setListRect() {
+ return getRect(this, '.van-index-bar').then((rect) => {
+ if (!isDef(rect)) {
+ return;
+ }
+ Object.assign(this, {
+ height: rect.height,
+ top: rect.top + this.scrollTop,
+ });
+ });
+ },
+ setSiderbarRect() {
+ return getRect(this, '.van-index-bar__sidebar').then((res) => {
+ if (!isDef(res)) {
+ return;
+ }
+ this.sidebar = {
+ height: res.height,
+ top: res.top,
+ };
+ });
+ },
+ setDiffData({ target, data }) {
+ const diffData = {};
+ Object.keys(data).forEach((key) => {
+ if (target.data[key] !== data[key]) {
+ diffData[key] = data[key];
+ }
+ });
+ if (Object.keys(diffData).length) {
+ target.setData(diffData);
+ }
+ },
+ getAnchorRect(anchor) {
+ return getRect(anchor, '.van-index-anchor-wrapper').then((rect) => ({
+ height: rect.height,
+ top: rect.top,
+ }));
+ },
+ getActiveAnchorIndex() {
+ const { children, scrollTop } = this;
+ const { sticky, stickyOffsetTop } = this.data;
+ for (let i = this.children.length - 1; i >= 0; i--) {
+ const preAnchorHeight = i > 0 ? children[i - 1].height : 0;
+ const reachTop = sticky ? preAnchorHeight + stickyOffsetTop : 0;
+ if (reachTop + scrollTop >= children[i].top) {
+ return i;
+ }
+ }
+ return -1;
+ },
+ onScroll() {
+ const { children = [], scrollTop } = this;
+ if (!children.length) {
+ return;
+ }
+ const { sticky, stickyOffsetTop, zIndex, highlightColor } = this.data;
+ const active = this.getActiveAnchorIndex();
+ this.setDiffData({
+ target: this,
+ data: {
+ activeAnchorIndex: active,
+ },
+ });
+ if (sticky) {
+ let isActiveAnchorSticky = false;
+ if (active !== -1) {
+ isActiveAnchorSticky =
+ children[active].top <= stickyOffsetTop + scrollTop;
+ }
+ children.forEach((item, index) => {
+ if (index === active) {
+ let wrapperStyle = '';
+ let anchorStyle = `
+ color: ${highlightColor};
+ `;
+ if (isActiveAnchorSticky) {
+ wrapperStyle = `
+ height: ${children[index].height}px;
+ `;
+ anchorStyle = `
+ position: fixed;
+ top: ${stickyOffsetTop}px;
+ z-index: ${zIndex};
+ color: ${highlightColor};
+ `;
+ }
+ this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle,
+ wrapperStyle,
+ },
+ });
+ }
+ else if (index === active - 1) {
+ const currentAnchor = children[index];
+ const currentOffsetTop = currentAnchor.top;
+ const targetOffsetTop = index === children.length - 1
+ ? this.top
+ : children[index + 1].top;
+ const parentOffsetHeight = targetOffsetTop - currentOffsetTop;
+ const translateY = parentOffsetHeight - currentAnchor.height;
+ const anchorStyle = `
+ position: relative;
+ transform: translate3d(0, ${translateY}px, 0);
+ z-index: ${zIndex};
+ color: ${highlightColor};
+ `;
+ this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle,
+ },
+ });
+ }
+ else {
+ this.setDiffData({
+ target: item,
+ data: {
+ active: false,
+ anchorStyle: '',
+ wrapperStyle: '',
+ },
+ });
+ }
+ });
+ }
+ },
+ onClick(event) {
+ this.scrollToAnchor(event.target.dataset.index);
+ },
+ onTouchMove(event) {
+ const sidebarLength = this.children.length;
+ const touch = event.touches[0];
+ const itemHeight = this.sidebar.height / sidebarLength;
+ let index = Math.floor((touch.clientY - this.sidebar.top) / itemHeight);
+ if (index < 0) {
+ index = 0;
+ }
+ else if (index > sidebarLength - 1) {
+ index = sidebarLength - 1;
+ }
+ this.scrollToAnchor(index);
+ },
+ onTouchStop() {
+ this.scrollToAnchorIndex = null;
+ },
+ scrollToAnchor(index) {
+ if (typeof index !== 'number' || this.scrollToAnchorIndex === index) {
+ return;
+ }
+ this.scrollToAnchorIndex = index;
+ const anchor = this.children.find((item) => item.data.index === this.data.indexList[index]);
+ if (anchor) {
+ anchor.scrollIntoView(this.scrollTop);
+ this.$emit('select', anchor.data.index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxml
new file mode 100644
index 0000000..19a59cf
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxss
new file mode 100644
index 0000000..8568801
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/index-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-bar{position:relative}.van-index-bar__sidebar{display:flex;flex-direction:column;position:fixed;right:0;text-align:center;top:50%;transform:translateY(-50%);-webkit-user-select:none;user-select:none}.van-index-bar__index{font-size:var(--index-bar-index-font-size,10px);font-weight:500;line-height:var(--index-bar-index-line-height,14px);padding:0 var(--padding-base,4px) 0 var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.js
new file mode 100644
index 0000000..6eac8f8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.js
@@ -0,0 +1,8 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ dot: Boolean,
+ info: null,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxml
new file mode 100644
index 0000000..b39b524
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxml
@@ -0,0 +1,7 @@
+
+
+{{ dot ? '' : info }}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxss
new file mode 100644
index 0000000..375ed5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/info/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-info{align-items:center;background-color:var(--info-background-color,#ee0a24);border:var(--info-border-width,1px) solid #fff;border-radius:var(--info-size,16px);box-sizing:border-box;color:var(--info-color,#fff);display:inline-flex;font-family:var(--info-font-family,-apple-system-font,Helvetica Neue,Arial,sans-serif);font-size:var(--info-font-size,12px);font-weight:var(--info-font-weight,500);height:var(--info-size,16px);justify-content:center;min-width:var(--info-size,16px);padding:var(--info-padding,0 3px);position:absolute;right:0;top:0;transform:translate(50%,-50%);transform-origin:100%;white-space:nowrap}.van-info--dot{background-color:var(--info-dot-color,#ee0a24);border-radius:100%;height:var(--info-dot-size,8px);min-width:0;width:var(--info-dot-size,8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.js
new file mode 100644
index 0000000..f5f96ba
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.js
@@ -0,0 +1,16 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ color: String,
+ vertical: Boolean,
+ type: {
+ type: String,
+ value: 'circular',
+ },
+ size: String,
+ textSize: String,
+ },
+ data: {
+ array12: Array.from({ length: 12 }),
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxml
new file mode 100644
index 0000000..7d4a539
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxs
new file mode 100644
index 0000000..02a0b80
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function spinnerStyle(data) {
+ return style({
+ color: data.color,
+ width: addUnit(data.size),
+ height: addUnit(data.size),
+ });
+}
+
+function textStyle(data) {
+ return style({
+ 'font-size': addUnit(data.textSize),
+ });
+}
+
+module.exports = {
+ spinnerStyle: spinnerStyle,
+ textStyle: textStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxss
new file mode 100644
index 0000000..fc84e84
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/loading/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{font-size:0;line-height:1}.van-loading{align-items:center;color:var(--loading-spinner-color,#c8c9cc);display:inline-flex;justify-content:center}.van-loading__spinner{animation:van-rotate var(--loading-spinner-animation-duration,.8s) linear infinite;box-sizing:border-box;height:var(--loading-spinner-size,30px);max-height:100%;max-width:100%;position:relative;width:var(--loading-spinner-size,30px)}.van-loading__spinner--spinner{animation-timing-function:steps(12)}.van-loading__spinner--circular{border:1px solid transparent;border-radius:100%;border-top-color:initial}.van-loading__text{color:var(--loading-text-color,#969799);font-size:var(--loading-text-font-size,14px);line-height:var(--loading-text-line-height,20px);margin-left:var(--padding-xs,8px)}.van-loading__text:empty{display:none}.van-loading--vertical{flex-direction:column}.van-loading--vertical .van-loading__text{margin:var(--padding-xs,8px) 0 0}.van-loading__dot{height:100%;left:0;position:absolute;top:0;width:100%}.van-loading__dot:before{background-color:currentColor;border-radius:40%;content:" ";display:block;height:25%;margin:0 auto;width:2px}.van-loading__dot:first-of-type{opacity:1;transform:rotate(30deg)}.van-loading__dot:nth-of-type(2){opacity:.9375;transform:rotate(60deg)}.van-loading__dot:nth-of-type(3){opacity:.875;transform:rotate(90deg)}.van-loading__dot:nth-of-type(4){opacity:.8125;transform:rotate(120deg)}.van-loading__dot:nth-of-type(5){opacity:.75;transform:rotate(150deg)}.van-loading__dot:nth-of-type(6){opacity:.6875;transform:rotate(180deg)}.van-loading__dot:nth-of-type(7){opacity:.625;transform:rotate(210deg)}.van-loading__dot:nth-of-type(8){opacity:.5625;transform:rotate(240deg)}.van-loading__dot:nth-of-type(9){opacity:.5;transform:rotate(270deg)}.van-loading__dot:nth-of-type(10){opacity:.4375;transform:rotate(300deg)}.van-loading__dot:nth-of-type(11){opacity:.375;transform:rotate(330deg)}.van-loading__dot:nth-of-type(12){opacity:.3125;transform:rotate(1turn)}@keyframes van-rotate{0%{transform:rotate(0deg)}to{transform:rotate(1turn)}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.d.ts
new file mode 100644
index 0000000..b273369
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.d.ts
@@ -0,0 +1 @@
+export declare const basic: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.js
new file mode 100644
index 0000000..617cc07
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/basic.js
@@ -0,0 +1,11 @@
+export const basic = Behavior({
+ methods: {
+ $emit(name, detail, options) {
+ this.triggerEvent(name, detail, options);
+ },
+ set(data) {
+ this.setData(data);
+ return new Promise((resolve) => wx.nextTick(resolve));
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.d.ts
new file mode 100644
index 0000000..b51db87
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.d.ts
@@ -0,0 +1 @@
+export declare const button: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.js
new file mode 100644
index 0000000..ce22c93
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/button.js
@@ -0,0 +1,51 @@
+import { canIUseGetUserProfile } from '../common/version';
+export const button = Behavior({
+ externalClasses: ['hover-class'],
+ properties: {
+ id: String,
+ buttonId: String,
+ lang: String,
+ businessId: Number,
+ sessionFrom: String,
+ sendMessageTitle: String,
+ sendMessagePath: String,
+ sendMessageImg: String,
+ showMessageCard: Boolean,
+ appParameter: String,
+ ariaLabel: String,
+ openType: String,
+ getUserProfileDesc: String,
+ },
+ data: {
+ canIUseGetUserProfile: canIUseGetUserProfile(),
+ },
+ methods: {
+ onGetUserInfo(event) {
+ this.triggerEvent('getuserinfo', event.detail);
+ },
+ onContact(event) {
+ this.triggerEvent('contact', event.detail);
+ },
+ onGetPhoneNumber(event) {
+ this.triggerEvent('getphonenumber', event.detail);
+ },
+ onGetRealTimePhoneNumber(event) {
+ this.triggerEvent('getrealtimephonenumber', event.detail);
+ },
+ onError(event) {
+ this.triggerEvent('error', event.detail);
+ },
+ onLaunchApp(event) {
+ this.triggerEvent('launchapp', event.detail);
+ },
+ onOpenSetting(event) {
+ this.triggerEvent('opensetting', event.detail);
+ },
+ onAgreePrivacyAuthorization(event) {
+ this.triggerEvent('agreeprivacyauthorization', event.detail);
+ },
+ onChooseAvatar(event) {
+ this.triggerEvent('chooseavatar', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.d.ts
new file mode 100644
index 0000000..d58043b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.d.ts
@@ -0,0 +1 @@
+export declare const link: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.js
new file mode 100644
index 0000000..8c274e1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/link.js
@@ -0,0 +1,23 @@
+export const link = Behavior({
+ properties: {
+ url: String,
+ linkType: {
+ type: String,
+ value: 'navigateTo',
+ },
+ },
+ methods: {
+ jumpLink(urlKey = 'url') {
+ const url = this.data[urlKey];
+ if (url) {
+ if (this.data.linkType === 'navigateTo' &&
+ getCurrentPages().length > 9) {
+ wx.redirectTo({ url });
+ }
+ else {
+ wx[this.data.linkType]({ url });
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.d.ts
new file mode 100644
index 0000000..4625447
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.d.ts
@@ -0,0 +1,6 @@
+///
+///
+type IPageScrollOption = WechatMiniprogram.Page.IPageScrollOption;
+type Scroller = (this: WechatMiniprogram.Component.TrivialInstance, event?: IPageScrollOption) => void;
+export declare function pageScrollMixin(scroller: Scroller): string;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.js
new file mode 100644
index 0000000..e08fb6e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/page-scroll.js
@@ -0,0 +1,42 @@
+import { isFunction } from '../common/validator';
+import { getCurrentPage, isDef } from '../common/utils';
+function onPageScroll(event) {
+ const { vanPageScroller = [] } = getCurrentPage();
+ vanPageScroller.forEach((scroller) => {
+ if (typeof scroller === 'function') {
+ // @ts-ignore
+ scroller(event);
+ }
+ });
+}
+export function pageScrollMixin(scroller) {
+ return Behavior({
+ attached() {
+ const page = getCurrentPage();
+ if (!isDef(page)) {
+ return;
+ }
+ const _scroller = scroller.bind(this);
+ const { vanPageScroller = [] } = page;
+ if (isFunction(page.onPageScroll) && page.onPageScroll !== onPageScroll) {
+ vanPageScroller.push(page.onPageScroll.bind(page));
+ }
+ vanPageScroller.push(_scroller);
+ page.vanPageScroller = vanPageScroller;
+ page.onPageScroll = onPageScroll;
+ this._scroller = _scroller;
+ },
+ detached() {
+ const page = getCurrentPage();
+ if (!isDef(page) || !isDef(page.vanPageScroller)) {
+ return;
+ }
+ const { vanPageScroller } = page;
+ const index = vanPageScroller.findIndex((v) => v === this._scroller);
+ if (index > -1) {
+ page.vanPageScroller.splice(index, 1);
+ }
+ this._scroller = undefined;
+ },
+ });
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.d.ts
new file mode 100644
index 0000000..35ee831
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.d.ts
@@ -0,0 +1 @@
+export declare const touch: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.js
new file mode 100644
index 0000000..ecefae8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/touch.js
@@ -0,0 +1,37 @@
+// @ts-nocheck
+const MIN_DISTANCE = 10;
+function getDirection(x, y) {
+ if (x > y && x > MIN_DISTANCE) {
+ return 'horizontal';
+ }
+ if (y > x && y > MIN_DISTANCE) {
+ return 'vertical';
+ }
+ return '';
+}
+export const touch = Behavior({
+ methods: {
+ resetTouchStatus() {
+ this.direction = '';
+ this.deltaX = 0;
+ this.deltaY = 0;
+ this.offsetX = 0;
+ this.offsetY = 0;
+ },
+ touchStart(event) {
+ this.resetTouchStatus();
+ const touch = event.touches[0];
+ this.startX = touch.clientX;
+ this.startY = touch.clientY;
+ },
+ touchMove(event) {
+ const touch = event.touches[0];
+ this.deltaX = touch.clientX - this.startX;
+ this.deltaY = touch.clientY - this.startY;
+ this.offsetX = Math.abs(this.deltaX);
+ this.offsetY = Math.abs(this.deltaY);
+ this.direction =
+ this.direction || getDirection(this.offsetX, this.offsetY);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.d.ts
new file mode 100644
index 0000000..dd829e5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.d.ts
@@ -0,0 +1 @@
+export declare function transition(showDefaultValue: boolean): string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.js
new file mode 100644
index 0000000..add9575
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/mixins/transition.js
@@ -0,0 +1,125 @@
+// @ts-nocheck
+import { requestAnimationFrame } from '../common/utils';
+import { isObj } from '../common/validator';
+const getClassNames = (name) => ({
+ enter: `van-${name}-enter van-${name}-enter-active enter-class enter-active-class`,
+ 'enter-to': `van-${name}-enter-to van-${name}-enter-active enter-to-class enter-active-class`,
+ leave: `van-${name}-leave van-${name}-leave-active leave-class leave-active-class`,
+ 'leave-to': `van-${name}-leave-to van-${name}-leave-active leave-to-class leave-active-class`,
+});
+export function transition(showDefaultValue) {
+ return Behavior({
+ properties: {
+ customStyle: String,
+ // @ts-ignore
+ show: {
+ type: Boolean,
+ value: showDefaultValue,
+ observer: 'observeShow',
+ },
+ // @ts-ignore
+ duration: {
+ type: null,
+ value: 300,
+ observer: 'observeDuration',
+ },
+ name: {
+ type: String,
+ value: 'fade',
+ },
+ },
+ data: {
+ type: '',
+ inited: false,
+ display: false,
+ },
+ ready() {
+ if (this.data.show === true) {
+ this.observeShow(true, false);
+ }
+ },
+ methods: {
+ observeShow(value, old) {
+ if (value === old) {
+ return;
+ }
+ value ? this.enter() : this.leave();
+ },
+ enter() {
+ this.waitEnterEndPromise = new Promise((resolve) => {
+ const { duration, name } = this.data;
+ const classNames = getClassNames(name);
+ const currentDuration = isObj(duration) ? duration.enter : duration;
+ if (this.status === 'enter') {
+ return;
+ }
+ this.status = 'enter';
+ this.$emit('before-enter');
+ requestAnimationFrame(() => {
+ if (this.status !== 'enter') {
+ return;
+ }
+ this.$emit('enter');
+ this.setData({
+ inited: true,
+ display: true,
+ classes: classNames.enter,
+ currentDuration,
+ });
+ requestAnimationFrame(() => {
+ if (this.status !== 'enter') {
+ return;
+ }
+ this.transitionEnded = false;
+ this.setData({ classes: classNames['enter-to'] });
+ resolve();
+ });
+ });
+ });
+ },
+ leave() {
+ if (!this.waitEnterEndPromise)
+ return;
+ this.waitEnterEndPromise.then(() => {
+ if (!this.data.display) {
+ return;
+ }
+ const { duration, name } = this.data;
+ const classNames = getClassNames(name);
+ const currentDuration = isObj(duration) ? duration.leave : duration;
+ this.status = 'leave';
+ this.$emit('before-leave');
+ requestAnimationFrame(() => {
+ if (this.status !== 'leave') {
+ return;
+ }
+ this.$emit('leave');
+ this.setData({
+ classes: classNames.leave,
+ currentDuration,
+ });
+ requestAnimationFrame(() => {
+ if (this.status !== 'leave') {
+ return;
+ }
+ this.transitionEnded = false;
+ setTimeout(() => this.onTransitionEnd(), currentDuration);
+ this.setData({ classes: classNames['leave-to'] });
+ });
+ });
+ });
+ },
+ onTransitionEnd() {
+ if (this.transitionEnded) {
+ return;
+ }
+ this.transitionEnded = true;
+ this.$emit(`after-${this.status}`);
+ const { show, display } = this.data;
+ if (!show && display) {
+ this.setData({ display: false });
+ }
+ },
+ },
+ });
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.js
new file mode 100644
index 0000000..30fc5aa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.js
@@ -0,0 +1,65 @@
+import { VantComponent } from '../common/component';
+import { getRect, getSystemInfoSync } from '../common/utils';
+VantComponent({
+ classes: ['title-class'],
+ props: {
+ title: String,
+ fixed: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ leftText: String,
+ rightText: String,
+ customStyle: String,
+ leftArrow: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 46,
+ },
+ created() {
+ const { statusBarHeight } = getSystemInfoSync();
+ this.setData({
+ statusBarHeight,
+ height: 46 + statusBarHeight,
+ });
+ },
+ mounted() {
+ this.setHeight();
+ },
+ methods: {
+ onClickLeft() {
+ this.$emit('click-left');
+ },
+ onClickRight() {
+ this.$emit('click-right');
+ },
+ setHeight() {
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(() => {
+ getRect(this, '.van-nav-bar').then((res) => {
+ if (res && 'height' in res) {
+ this.setData({ height: res.height });
+ }
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxml
new file mode 100644
index 0000000..b6405fd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxml
@@ -0,0 +1,42 @@
+
+
+
+
+
+
+
+
+
+
+ {{ leftText }}
+
+
+
+
+ {{ title }}
+
+
+
+ {{ rightText }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxs
new file mode 100644
index 0000000..55b4158
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function barStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ 'padding-top': data.safeAreaInsetTop ? data.statusBarHeight + 'px' : 0,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxss
new file mode 100644
index 0000000..d11c31e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/nav-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-nav-bar{background-color:var(--nav-bar-background-color,#fff);box-sizing:initial;height:var(--nav-bar-height,46px);line-height:var(--nav-bar-height,46px);position:relative;text-align:center;-webkit-user-select:none;user-select:none}.van-nav-bar__content{height:100%;position:relative}.van-nav-bar__text{color:var(--nav-bar-text-color,#1989fa);display:inline-block;margin:0 calc(var(--padding-md, 16px)*-1);padding:0 var(--padding-md,16px);vertical-align:middle}.van-nav-bar__text--hover{background-color:#f2f3f5}.van-nav-bar__arrow{color:var(--nav-bar-icon-color,#1989fa)!important;font-size:var(--nav-bar-arrow-size,16px)!important;vertical-align:middle}.van-nav-bar__arrow+.van-nav-bar__text{margin-left:-20px;padding-left:25px}.van-nav-bar--fixed{left:0;position:fixed;top:0;width:100%}.van-nav-bar__title{color:var(--nav-bar-title-text-color,#323233);font-size:var(--nav-bar-title-font-size,16px);font-weight:var(--font-weight-bold,500);margin:0 auto;max-width:60%}.van-nav-bar__left,.van-nav-bar__right{align-items:center;bottom:0;display:flex;font-size:var(--font-size-md,14px);position:absolute;top:0}.van-nav-bar__left{left:var(--padding-md,16px)}.van-nav-bar__right{right:var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.js
new file mode 100644
index 0000000..ee55e59
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.js
@@ -0,0 +1,120 @@
+import { VantComponent } from '../common/component';
+import { getRect, requestAnimationFrame } from '../common/utils';
+VantComponent({
+ props: {
+ text: {
+ type: String,
+ value: '',
+ observer: 'init',
+ },
+ mode: {
+ type: String,
+ value: '',
+ },
+ url: {
+ type: String,
+ value: '',
+ },
+ openType: {
+ type: String,
+ value: 'navigate',
+ },
+ delay: {
+ type: Number,
+ value: 1,
+ },
+ speed: {
+ type: Number,
+ value: 60,
+ observer: 'init',
+ },
+ scrollable: null,
+ leftIcon: {
+ type: String,
+ value: '',
+ },
+ color: String,
+ backgroundColor: String,
+ background: String,
+ wrapable: Boolean,
+ },
+ data: {
+ show: true,
+ },
+ created() {
+ this.resetAnimation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'linear',
+ });
+ },
+ destroyed() {
+ this.timer && clearTimeout(this.timer);
+ },
+ mounted() {
+ this.init();
+ },
+ methods: {
+ init() {
+ requestAnimationFrame(() => {
+ Promise.all([
+ getRect(this, '.van-notice-bar__content'),
+ getRect(this, '.van-notice-bar__wrap'),
+ ]).then((rects) => {
+ const [contentRect, wrapRect] = rects;
+ const { speed, scrollable, delay } = this.data;
+ if (contentRect == null ||
+ wrapRect == null ||
+ !contentRect.width ||
+ !wrapRect.width ||
+ scrollable === false) {
+ return;
+ }
+ if (scrollable || wrapRect.width < contentRect.width) {
+ const duration = ((wrapRect.width + contentRect.width) / speed) * 1000;
+ this.wrapWidth = wrapRect.width;
+ this.contentWidth = contentRect.width;
+ this.duration = duration;
+ this.animation = wx.createAnimation({
+ duration,
+ timingFunction: 'linear',
+ delay,
+ });
+ this.scroll(true);
+ }
+ });
+ });
+ },
+ scroll(isInit = false) {
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({
+ animationData: this.resetAnimation
+ .translateX(isInit ? 0 : this.wrapWidth)
+ .step()
+ .export(),
+ });
+ requestAnimationFrame(() => {
+ this.setData({
+ animationData: this.animation
+ .translateX(-this.contentWidth)
+ .step()
+ .export(),
+ });
+ });
+ this.timer = setTimeout(() => {
+ this.scroll();
+ }, this.duration + this.data.delay);
+ },
+ onClickIcon(event) {
+ if (this.data.mode === 'closeable') {
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({ show: false });
+ this.$emit('close', event.detail);
+ }
+ },
+ onClick(event) {
+ this.$emit('click', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxml
new file mode 100644
index 0000000..21b0973
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxml
@@ -0,0 +1,38 @@
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxs
new file mode 100644
index 0000000..11e6456
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ color: data.color,
+ 'background-color': data.backgroundColor,
+ background: data.background,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxss
new file mode 100644
index 0000000..497636c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notice-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notice-bar{align-items:center;background-color:var(--notice-bar-background-color,#fffbe8);color:var(--notice-bar-text-color,#ed6a0c);display:flex;font-size:var(--notice-bar-font-size,14px);height:var(--notice-bar-height,40px);line-height:var(--notice-bar-line-height,24px);padding:var(--notice-bar-padding,0 16px)}.van-notice-bar--withicon{padding-right:40px;position:relative}.van-notice-bar--wrapable{height:auto;padding:var(--notice-bar-wrapable-padding,8px 16px)}.van-notice-bar--wrapable .van-notice-bar__wrap{height:auto}.van-notice-bar--wrapable .van-notice-bar__content{position:relative;white-space:normal}.van-notice-bar__left-icon{align-items:center;display:flex;margin-right:4px;vertical-align:middle}.van-notice-bar__left-icon,.van-notice-bar__right-icon{font-size:var(--notice-bar-icon-size,16px);min-width:var(--notice-bar-icon-min-width,22px)}.van-notice-bar__right-icon{position:absolute;right:15px;top:10px}.van-notice-bar__wrap{flex:1;height:var(--notice-bar-line-height,24px);overflow:hidden;position:relative}.van-notice-bar__content{position:absolute;white-space:nowrap}.van-notice-bar__content.van-ellipsis{max-width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.js
new file mode 100644
index 0000000..d4aba2d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.js
@@ -0,0 +1,65 @@
+import { VantComponent } from '../common/component';
+import { WHITE } from '../common/color';
+import { getSystemInfoSync } from '../common/utils';
+VantComponent({
+ props: {
+ message: String,
+ background: String,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ color: {
+ type: String,
+ value: WHITE,
+ },
+ duration: {
+ type: Number,
+ value: 3000,
+ },
+ zIndex: {
+ type: Number,
+ value: 110,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ top: null,
+ },
+ data: {
+ show: false,
+ onOpened: null,
+ onClose: null,
+ onClick: null,
+ },
+ created() {
+ const { statusBarHeight } = getSystemInfoSync();
+ this.setData({ statusBarHeight });
+ },
+ methods: {
+ show() {
+ const { duration, onOpened } = this.data;
+ clearTimeout(this.timer);
+ this.setData({ show: true });
+ wx.nextTick(onOpened);
+ if (duration > 0 && duration !== Infinity) {
+ this.timer = setTimeout(() => {
+ this.hide();
+ }, duration);
+ }
+ },
+ hide() {
+ const { onClose } = this.data;
+ clearTimeout(this.timer);
+ this.setData({ show: false });
+ wx.nextTick(onClose);
+ },
+ onTap(event) {
+ const { onClick } = this.data;
+ if (onClick) {
+ onClick(event.detail);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxml
new file mode 100644
index 0000000..42d913e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+ {{ message }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxs
new file mode 100644
index 0000000..bbb94c2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ top: addUnit(data.top),
+ });
+}
+
+function notifyStyle(data) {
+ return style({
+ background: data.background,
+ color: data.color,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ notifyStyle: notifyStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxss
new file mode 100644
index 0000000..c030e9b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notify{word-wrap:break-word;font-size:var(--notify-font-size,14px);line-height:var(--notify-line-height,20px);padding:var(--notify-padding,6px 15px);text-align:center}.van-notify__container{box-sizing:border-box;left:0;position:fixed;top:0;width:100%}.van-notify--primary{background-color:var(--notify-primary-background-color,#1989fa)}.van-notify--success{background-color:var(--notify-success-background-color,#07c160)}.van-notify--danger{background-color:var(--notify-danger-background-color,#ee0a24)}.van-notify--warning{background-color:var(--notify-warning-background-color,#ff976a)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.d.ts
new file mode 100644
index 0000000..d5213cb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.d.ts
@@ -0,0 +1,22 @@
+interface NotifyOptions {
+ type?: 'primary' | 'success' | 'danger' | 'warning';
+ color?: string;
+ zIndex?: number;
+ top?: number;
+ message: string;
+ context?: any;
+ duration?: number;
+ selector?: string;
+ background?: string;
+ safeAreaInsetTop?: boolean;
+ onClick?: () => void;
+ onOpened?: () => void;
+ onClose?: () => void;
+}
+declare function Notify(options: NotifyOptions | string): any;
+declare namespace Notify {
+ var clear: (options?: NotifyOptions | undefined) => void;
+ var setDefaultOptions: (options: NotifyOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Notify;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.js
new file mode 100644
index 0000000..306d7fd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/notify/notify.js
@@ -0,0 +1,53 @@
+import { WHITE } from '../common/color';
+const defaultOptions = {
+ selector: '#van-notify',
+ type: 'danger',
+ message: '',
+ background: '',
+ duration: 3000,
+ zIndex: 110,
+ top: 0,
+ color: WHITE,
+ safeAreaInsetTop: false,
+ onClick: () => { },
+ onOpened: () => { },
+ onClose: () => { },
+};
+let currentOptions = Object.assign({}, defaultOptions);
+function parseOptions(message) {
+ if (message == null) {
+ return {};
+ }
+ return typeof message === 'string' ? { message } : message;
+}
+function getContext() {
+ const pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+export default function Notify(options) {
+ options = Object.assign(Object.assign({}, currentOptions), parseOptions(options));
+ const context = options.context || getContext();
+ const notify = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (notify) {
+ notify.setData(options);
+ notify.show();
+ return notify;
+ }
+ console.warn('未找到 van-notify 节点,请确认 selector 及 context 是否正确');
+}
+Notify.clear = function (options) {
+ options = Object.assign(Object.assign({}, defaultOptions), parseOptions(options));
+ const context = options.context || getContext();
+ const notify = context.selectComponent(options.selector);
+ if (notify) {
+ notify.hide();
+ }
+};
+Notify.setDefaultOptions = (options) => {
+ Object.assign(currentOptions, options);
+};
+Notify.resetDefaultOptions = () => {
+ currentOptions = Object.assign({}, defaultOptions);
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.js
new file mode 100644
index 0000000..d6647dd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.js
@@ -0,0 +1,30 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ show: Boolean,
+ customStyle: String,
+ duration: {
+ type: null,
+ value: 300,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClick() {
+ this.$emit('click');
+ },
+ // for prevent touchmove
+ noop() { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxml
new file mode 100644
index 0000000..17fc56f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxml
@@ -0,0 +1,7 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxss
new file mode 100644
index 0000000..d1ad81a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-overlay{background-color:var(--overlay-background-color,rgba(0,0,0,.7));height:100%;left:0;position:fixed;top:0;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/overlay.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/overlay.wxml
new file mode 100644
index 0000000..017e801
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/overlay/overlay.wxml
@@ -0,0 +1,10 @@
+
+
+
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.js
new file mode 100644
index 0000000..7b6a99a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.js
@@ -0,0 +1,9 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: ['header-class', 'footer-class'],
+ props: {
+ desc: String,
+ title: String,
+ status: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxml
new file mode 100644
index 0000000..1843703
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxss
new file mode 100644
index 0000000..485edcd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/panel/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-panel{background:var(--panel-background-color,#fff)}.van-panel__header-value{color:var(--panel-header-value-color,#ee0a24)}.van-panel__footer{padding:var(--panel-footer-padding,8px 16px)}.van-panel__footer:empty{display:none}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.js
new file mode 100644
index 0000000..abcc520
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.js
@@ -0,0 +1,118 @@
+import { VantComponent } from '../common/component';
+import { range } from '../common/utils';
+import { isObj } from '../common/validator';
+const DEFAULT_DURATION = 200;
+VantComponent({
+ classes: ['active-class'],
+ props: {
+ valueKey: String,
+ className: String,
+ itemHeight: Number,
+ visibleItemCount: Number,
+ initialOptions: {
+ type: Array,
+ value: [],
+ },
+ defaultIndex: {
+ type: Number,
+ value: 0,
+ observer(value) {
+ this.setIndex(value);
+ },
+ },
+ },
+ data: {
+ startY: 0,
+ offset: 0,
+ duration: 0,
+ startOffset: 0,
+ options: [],
+ currentIndex: 0,
+ },
+ created() {
+ const { defaultIndex, initialOptions } = this.data;
+ this.set({
+ currentIndex: defaultIndex,
+ options: initialOptions,
+ }).then(() => {
+ this.setIndex(defaultIndex);
+ });
+ },
+ methods: {
+ getCount() {
+ return this.data.options.length;
+ },
+ onTouchStart(event) {
+ this.setData({
+ startY: event.touches[0].clientY,
+ startOffset: this.data.offset,
+ duration: 0,
+ });
+ },
+ onTouchMove(event) {
+ const { data } = this;
+ const deltaY = event.touches[0].clientY - data.startY;
+ this.setData({
+ offset: range(data.startOffset + deltaY, -(this.getCount() * data.itemHeight), data.itemHeight),
+ });
+ },
+ onTouchEnd() {
+ const { data } = this;
+ if (data.offset !== data.startOffset) {
+ this.setData({ duration: DEFAULT_DURATION });
+ const index = range(Math.round(-data.offset / data.itemHeight), 0, this.getCount() - 1);
+ this.setIndex(index, true);
+ }
+ },
+ onClickItem(event) {
+ const { index } = event.currentTarget.dataset;
+ this.setIndex(index, true);
+ },
+ adjustIndex(index) {
+ const { data } = this;
+ const count = this.getCount();
+ index = range(index, 0, count);
+ for (let i = index; i < count; i++) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ for (let i = index - 1; i >= 0; i--) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ },
+ isDisabled(option) {
+ return isObj(option) && option.disabled;
+ },
+ getOptionText(option) {
+ const { data } = this;
+ return isObj(option) && data.valueKey in option
+ ? option[data.valueKey]
+ : option;
+ },
+ setIndex(index, userAction) {
+ const { data } = this;
+ index = this.adjustIndex(index) || 0;
+ const offset = -index * data.itemHeight;
+ if (index !== data.currentIndex) {
+ return this.set({ offset, currentIndex: index }).then(() => {
+ userAction && this.$emit('change', index);
+ });
+ }
+ return this.set({ offset });
+ },
+ setValue(value) {
+ const { options } = this.data;
+ for (let i = 0; i < options.length; i++) {
+ if (this.getOptionText(options[i]) === value) {
+ return this.setIndex(i);
+ }
+ }
+ return Promise.resolve();
+ },
+ getValue() {
+ const { data } = this;
+ return data.options[data.currentIndex];
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxml
new file mode 100644
index 0000000..f2c8da2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
+
+ {{ computed.optionText(option, valueKey) }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxs
new file mode 100644
index 0000000..2d5a611
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isObj(x) {
+ var type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+
+function optionText(option, valueKey) {
+ return isObj(option) && option[valueKey] != null ? option[valueKey] : option;
+}
+
+function rootStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function wrapperStyle(data) {
+ var offset = addUnit(
+ data.offset + (data.itemHeight * (data.visibleItemCount - 1)) / 2
+ );
+
+ return style({
+ transition: 'transform ' + data.duration + 'ms',
+ 'line-height': addUnit(data.itemHeight),
+ transform: 'translate3d(0, ' + offset + ', 0)',
+ });
+}
+
+module.exports = {
+ optionText: optionText,
+ rootStyle: rootStyle,
+ wrapperStyle: wrapperStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxss
new file mode 100644
index 0000000..519a438
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker-column/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker-column{color:var(--picker-option-text-color,#000);font-size:var(--picker-option-font-size,16px);overflow:hidden;text-align:center}.van-picker-column__item{padding:0 5px}.van-picker-column__item--selected{color:var(--picker-option-selected-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-picker-column__item--disabled{opacity:var(--picker-option-disabled-opacity,.3)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.js
new file mode 100644
index 0000000..cef057d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.js
@@ -0,0 +1,136 @@
+import { VantComponent } from '../common/component';
+import { pickerProps } from './shared';
+VantComponent({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: Object.assign(Object.assign({}, pickerProps), { valueKey: {
+ type: String,
+ value: 'text',
+ }, toolbarPosition: {
+ type: String,
+ value: 'top',
+ }, defaultIndex: {
+ type: Number,
+ value: 0,
+ }, columns: {
+ type: Array,
+ value: [],
+ observer(columns = []) {
+ this.simple = columns.length && !columns[0].values;
+ if (Array.isArray(this.children) && this.children.length) {
+ this.setColumns().catch(() => { });
+ }
+ },
+ } }),
+ beforeCreate() {
+ Object.defineProperty(this, 'children', {
+ get: () => this.selectAllComponents('.van-picker__column') || [],
+ });
+ },
+ methods: {
+ noop() { },
+ setColumns() {
+ const { data } = this;
+ const columns = this.simple ? [{ values: data.columns }] : data.columns;
+ const stack = columns.map((column, index) => this.setColumnValues(index, column.values));
+ return Promise.all(stack);
+ },
+ emit(event) {
+ const { type } = event.currentTarget.dataset;
+ if (this.simple) {
+ this.$emit(type, {
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit(type, {
+ value: this.getValues(),
+ index: this.getIndexes(),
+ });
+ }
+ },
+ onChange(event) {
+ if (this.simple) {
+ this.$emit('change', {
+ picker: this,
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit('change', {
+ picker: this,
+ value: this.getValues(),
+ index: event.currentTarget.dataset.index,
+ });
+ }
+ },
+ // get column instance by index
+ getColumn(index) {
+ return this.children[index];
+ },
+ // get column value by index
+ getColumnValue(index) {
+ const column = this.getColumn(index);
+ return column && column.getValue();
+ },
+ // set column value by index
+ setColumnValue(index, value) {
+ const column = this.getColumn(index);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValue: 对应列不存在'));
+ }
+ return column.setValue(value);
+ },
+ // get column option index by column index
+ getColumnIndex(columnIndex) {
+ return (this.getColumn(columnIndex) || {}).data.currentIndex;
+ },
+ // set column option index by column index
+ setColumnIndex(columnIndex, optionIndex) {
+ const column = this.getColumn(columnIndex);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnIndex: 对应列不存在'));
+ }
+ return column.setIndex(optionIndex);
+ },
+ // get options of column by index
+ getColumnValues(index) {
+ return (this.children[index] || {}).data.options;
+ },
+ // set options of column by index
+ setColumnValues(index, options, needReset = true) {
+ const column = this.children[index];
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValues: 对应列不存在'));
+ }
+ const isSame = JSON.stringify(column.data.options) === JSON.stringify(options);
+ if (isSame) {
+ return Promise.resolve();
+ }
+ return column.set({ options }).then(() => {
+ if (needReset) {
+ column.setIndex(0);
+ }
+ });
+ },
+ // get values of all columns
+ getValues() {
+ return this.children.map((child) => child.getValue());
+ },
+ // set values of all columns
+ setValues(values) {
+ const stack = values.map((value, index) => this.setColumnValue(index, value));
+ return Promise.all(stack);
+ },
+ // get indexes of all columns
+ getIndexes() {
+ return this.children.map((child) => child.data.currentIndex);
+ },
+ // set indexes of all columns
+ setIndexes(indexes) {
+ const stack = indexes.map((optionIndex, columnIndex) => this.setColumnIndex(columnIndex, optionIndex));
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.json
new file mode 100644
index 0000000..2fcec89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "picker-column": "../picker-column/index",
+ "loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxml
new file mode 100644
index 0000000..8564ccc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxml
@@ -0,0 +1,37 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxs
new file mode 100644
index 0000000..0abbd10
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+var array = require('../wxs/array.wxs');
+
+function columnsStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function maskStyle(data) {
+ return style({
+ 'background-size':
+ '100% ' + addUnit((data.itemHeight * (data.visibleItemCount - 1)) / 2),
+ });
+}
+
+function frameStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight),
+ });
+}
+
+function columns(columns) {
+ if (!array.isArray(columns)) {
+ return [];
+ }
+
+ if (columns.length && !columns[0].values) {
+ return [{ values: columns }];
+ }
+
+ return columns;
+}
+
+module.exports = {
+ columnsStyle: columnsStyle,
+ frameStyle: frameStyle,
+ maskStyle: maskStyle,
+ columns: columns,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxss
new file mode 100644
index 0000000..d924abb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker{-webkit-text-size-adjust:100%;background-color:var(--picker-background-color,#fff);overflow:hidden;position:relative;-webkit-user-select:none;user-select:none}.van-picker__toolbar{display:flex;height:var(--picker-toolbar-height,44px);justify-content:space-between;line-height:var(--picker-toolbar-height,44px)}.van-picker__cancel,.van-picker__confirm{font-size:var(--picker-action-font-size,14px);padding:var(--picker-action-padding,0 16px)}.van-picker__cancel--hover,.van-picker__confirm--hover{opacity:.7}.van-picker__confirm{color:var(--picker-confirm-action-color,#576b95)}.van-picker__cancel{color:var(--picker-cancel-action-color,#969799)}.van-picker__title{font-size:var(--picker-option-font-size,16px);font-weight:var(--font-weight-bold,500);max-width:50%;text-align:center}.van-picker__columns{display:flex;position:relative}.van-picker__column{flex:1 1;width:0}.van-picker__loading{align-items:center;background-color:var(--picker-loading-mask-color,hsla(0,0%,100%,.9));bottom:0;display:flex;justify-content:center;left:0;position:absolute;right:0;top:0;z-index:4}.van-picker__mask{-webkit-backface-visibility:hidden;backface-visibility:hidden;background-image:linear-gradient(180deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4)),linear-gradient(0deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4));background-position:top,bottom;background-repeat:no-repeat;height:100%;left:0;top:0;width:100%;z-index:2}.van-picker__frame,.van-picker__mask{pointer-events:none;position:absolute}.van-picker__frame{left:16px;right:16px;top:50%;transform:translateY(-50%);z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.d.ts
new file mode 100644
index 0000000..c548045
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.d.ts
@@ -0,0 +1,21 @@
+export declare const pickerProps: {
+ title: StringConstructor;
+ loading: BooleanConstructor;
+ showToolbar: BooleanConstructor;
+ cancelButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ confirmButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ visibleItemCount: {
+ type: NumberConstructor;
+ value: number;
+ };
+ itemHeight: {
+ type: NumberConstructor;
+ value: number;
+ };
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.js
new file mode 100644
index 0000000..5f21f32
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/shared.js
@@ -0,0 +1,21 @@
+export const pickerProps = {
+ title: String,
+ loading: Boolean,
+ showToolbar: Boolean,
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ visibleItemCount: {
+ type: Number,
+ value: 6,
+ },
+ itemHeight: {
+ type: Number,
+ value: 44,
+ },
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/toolbar.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/toolbar.wxml
new file mode 100644
index 0000000..414f612
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/picker/toolbar.wxml
@@ -0,0 +1,23 @@
+
+
+ {{ cancelButtonText }}
+
+ {{
+ title
+ }}
+
+ {{ confirmButtonText }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.js
new file mode 100644
index 0000000..fd3dd8e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.js
@@ -0,0 +1,97 @@
+import { VantComponent } from '../common/component';
+import { transition } from '../mixins/transition';
+VantComponent({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ 'close-icon-class',
+ ],
+ mixins: [transition(false)],
+ props: {
+ round: Boolean,
+ closeable: Boolean,
+ customStyle: String,
+ overlayStyle: String,
+ transition: {
+ type: String,
+ observer: 'observeClass',
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ closeIconPosition: {
+ type: String,
+ value: 'top-right',
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ position: {
+ type: String,
+ value: 'center',
+ observer: 'observeClass',
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ created() {
+ this.observeClass();
+ },
+ methods: {
+ onClickCloseIcon() {
+ this.$emit('close');
+ },
+ onClickOverlay() {
+ this.$emit('click-overlay');
+ if (this.data.closeOnClickOverlay) {
+ this.$emit('close');
+ }
+ },
+ observeClass() {
+ const { transition, position, duration } = this.data;
+ const updateData = {
+ name: transition || position,
+ };
+ if (transition === 'none') {
+ updateData.duration = 0;
+ this.originDuration = duration;
+ }
+ else if (this.originDuration != null) {
+ updateData.duration = this.originDuration;
+ }
+ this.setData(updateData);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.json
new file mode 100644
index 0000000..88a6eab
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-overlay": "../overlay/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxml
new file mode 100644
index 0000000..ab824b1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxs
new file mode 100644
index 0000000..8d59f24
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function popupStyle(data) {
+ return style([
+ {
+ 'z-index': data.zIndex,
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ popupStyle: popupStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxss
new file mode 100644
index 0000000..91983b4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-popup{-webkit-overflow-scrolling:touch;animation:ease both;background-color:var(--popup-background-color,#fff);box-sizing:border-box;max-height:100%;overflow-y:auto;position:fixed;transition-timing-function:ease}.van-popup--center{left:50%;top:50%;transform:translate3d(-50%,-50%,0)}.van-popup--center.van-popup--round{border-radius:var(--popup-round-border-radius,16px)}.van-popup--top{left:0;top:0;width:100%}.van-popup--top.van-popup--round{border-radius:0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--right{right:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--right.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--bottom{bottom:0;left:0;width:100%}.van-popup--bottom.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0}.van-popup--left{left:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--left.van-popup--round{border-radius:0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0}.van-popup--bottom.van-popup--safe{padding-bottom:env(safe-area-inset-bottom)}.van-popup--bottom.van-popup--safeTabBar,.van-popup--top.van-popup--safeTabBar{bottom:var(--tabbar-height,50px)}.van-popup--safeTop{padding-top:env(safe-area-inset-top)}.van-popup__close-icon{color:var(--popup-close-icon-color,#969799);font-size:var(--popup-close-icon-size,18px);position:absolute;z-index:var(--popup-close-icon-z-index,1)}.van-popup__close-icon--top-left{left:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--top-right{right:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-left{bottom:var(--popup-close-icon-margin,16px);left:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-right{bottom:var(--popup-close-icon-margin,16px);right:var(--popup-close-icon-margin,16px)}.van-popup__close-icon:active{opacity:.6}.van-scale-enter-active,.van-scale-leave-active{transition-property:opacity,transform}.van-scale-enter,.van-scale-leave-to{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-center-enter-active,.van-center-leave-active{transition-property:opacity}.van-center-enter,.van-center-leave-to{opacity:0}.van-bottom-enter-active,.van-bottom-leave-active,.van-left-enter-active,.van-left-leave-active,.van-right-enter-active,.van-right-leave-active,.van-top-enter-active,.van-top-leave-active{transition-property:transform}.van-bottom-enter,.van-bottom-leave-to{transform:translate3d(0,100%,0)}.van-top-enter,.van-top-leave-to{transform:translate3d(0,-100%,0)}.van-left-enter,.van-left-leave-to{transform:translate3d(-100%,-50%,0)}.van-right-enter,.van-right-leave-to{transform:translate3d(100%,-50%,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/popup.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/popup.wxml
new file mode 100644
index 0000000..bd8f508
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/popup/popup.wxml
@@ -0,0 +1,16 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.js
new file mode 100644
index 0000000..0780c43
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.js
@@ -0,0 +1,51 @@
+import { VantComponent } from '../common/component';
+import { BLUE } from '../common/color';
+import { getRect } from '../common/utils';
+VantComponent({
+ props: {
+ inactive: Boolean,
+ percentage: {
+ type: Number,
+ observer: 'setLeft',
+ },
+ pivotText: String,
+ pivotColor: String,
+ trackColor: String,
+ showPivot: {
+ type: Boolean,
+ value: true,
+ },
+ color: {
+ type: String,
+ value: BLUE,
+ },
+ textColor: {
+ type: String,
+ value: '#fff',
+ },
+ strokeWidth: {
+ type: null,
+ value: 4,
+ },
+ },
+ data: {
+ right: 0,
+ },
+ mounted() {
+ this.setLeft();
+ },
+ methods: {
+ setLeft() {
+ Promise.all([
+ getRect(this, '.van-progress'),
+ getRect(this, '.van-progress__pivot'),
+ ]).then(([portion, pivot]) => {
+ if (portion && pivot) {
+ this.setData({
+ right: (pivot.width * (this.data.percentage - 100)) / 100,
+ });
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxml
new file mode 100644
index 0000000..e81514d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+ {{ computed.pivotText(pivotText, percentage) }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxs
new file mode 100644
index 0000000..5b1e8e6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function pivotText(pivotText, percentage) {
+ return pivotText || percentage + '%';
+}
+
+function rootStyle(data) {
+ return style({
+ 'height': data.strokeWidth ? utils.addUnit(data.strokeWidth) : '',
+ 'background': data.trackColor,
+ });
+}
+
+function portionStyle(data) {
+ return style({
+ background: data.inactive ? '#cacaca' : data.color,
+ width: data.percentage ? data.percentage + '%' : '',
+ });
+}
+
+function pivotStyle(data) {
+ return style({
+ color: data.textColor,
+ right: data.right + 'px',
+ background: data.pivotColor ? data.pivotColor : data.inactive ? '#cacaca' : data.color,
+ });
+}
+
+module.exports = {
+ pivotText: pivotText,
+ rootStyle: rootStyle,
+ portionStyle: portionStyle,
+ pivotStyle: pivotStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxss
new file mode 100644
index 0000000..a08972a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/progress/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-progress{background:var(--progress-background-color,#ebedf0);border-radius:var(--progress-height,4px);height:var(--progress-height,4px);position:relative}.van-progress__portion{background:var(--progress-color,#1989fa);border-radius:inherit;height:100%;left:0;position:absolute}.van-progress__pivot{background-color:var(--progress-pivot-background-color,#1989fa);border-radius:1em;box-sizing:border-box;color:var(--progress-pivot-text-color,#fff);font-size:var(--progress-pivot-font-size,10px);line-height:var(--progress-pivot-line-height,1.6);min-width:3.6em;padding:var(--progress-pivot-padding,0 5px);position:absolute;text-align:center;top:50%;transform:translateY(-50%);word-break:keep-all}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.js
new file mode 100644
index 0000000..2846fdd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.js
@@ -0,0 +1,22 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ field: true,
+ relation: useChildren('radio'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ direction: String,
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren() {
+ this.children.forEach((child) => child.updateFromParent());
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxml
new file mode 100644
index 0000000..0ab17af
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxss
new file mode 100644
index 0000000..4e3b5d4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.js
new file mode 100644
index 0000000..9eb1258
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.js
@@ -0,0 +1,66 @@
+import { canIUseModel } from '../common/version';
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+VantComponent({
+ field: true,
+ relation: useParent('radio-group', function () {
+ this.updateFromParent();
+ }),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ name: null,
+ value: null,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ direction: '',
+ parentDisabled: false,
+ },
+ methods: {
+ updateFromParent() {
+ if (!this.parent) {
+ return;
+ }
+ const { value, disabled: parentDisabled, direction } = this.parent.data;
+ this.setData({
+ value,
+ direction,
+ parentDisabled,
+ });
+ },
+ emitChange(value) {
+ const instance = this.parent || this;
+ instance.$emit('input', value);
+ instance.$emit('change', value);
+ if (canIUseModel()) {
+ instance.setData({ value });
+ }
+ },
+ onChange() {
+ if (!this.data.disabled && !this.data.parentDisabled) {
+ this.emitChange(this.data.name);
+ }
+ },
+ onClickLabel() {
+ const { disabled, parentDisabled, labelDisabled, name } = this.data;
+ if (!(disabled || parentDisabled) && !labelDisabled) {
+ this.emitChange(name);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxml
new file mode 100644
index 0000000..5f898c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxml
@@ -0,0 +1,30 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxs
new file mode 100644
index 0000000..a428aad
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxs
@@ -0,0 +1,33 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(data) {
+ var styles = {
+ 'font-size': addUnit(data.iconSize),
+ };
+
+ if (
+ data.checkedColor &&
+ !(data.disabled || data.parentDisabled) &&
+ data.value === data.name
+ ) {
+ styles['border-color'] = data.checkedColor;
+ styles['background-color'] = data.checkedColor;
+ }
+
+ return style(styles);
+}
+
+function iconCustomStyle(data) {
+ return style({
+ 'line-height': addUnit(data.iconSize),
+ 'font-size': '.8em',
+ display: 'block',
+ });
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+ iconCustomStyle: iconCustomStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxss
new file mode 100644
index 0000000..257b0c7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/radio/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-radio__icon-wrap{flex:none}.van-radio--horizontal{margin-right:var(--padding-sm,12px)}.van-radio__icon{align-items:center;border:1px solid var(--radio-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--radio-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--radio-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-radio__icon--round{border-radius:100%}.van-radio__icon--checked{background-color:var(--radio-checked-icon-color,#1989fa);border-color:var(--radio-checked-icon-color,#1989fa);color:#fff}.van-radio__icon--disabled{background-color:var(--radio-disabled-background-color,#ebedf0);border-color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__icon--disabled.van-radio__icon--checked{color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__label{word-wrap:break-word;color:var(--radio-label-color,#323233);line-height:var(--radio-size,20px);padding-left:var(--radio-label-margin,10px)}.van-radio__label--left{float:left;margin:0 var(--radio-label-margin,10px) 0 0}.van-radio__label--disabled{color:var(--radio-disabled-label-color,#c8c9cc)}.van-radio__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.js
new file mode 100644
index 0000000..23b7345
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.js
@@ -0,0 +1,78 @@
+import { getAllRect } from '../common/utils';
+import { VantComponent } from '../common/component';
+import { canIUseModel } from '../common/version';
+VantComponent({
+ field: true,
+ classes: ['icon-class'],
+ props: {
+ value: {
+ type: Number,
+ observer(value) {
+ if (value !== this.data.innerValue) {
+ this.setData({ innerValue: value });
+ }
+ },
+ },
+ readonly: Boolean,
+ disabled: Boolean,
+ allowHalf: Boolean,
+ size: null,
+ icon: {
+ type: String,
+ value: 'star',
+ },
+ voidIcon: {
+ type: String,
+ value: 'star-o',
+ },
+ color: String,
+ voidColor: String,
+ disabledColor: String,
+ count: {
+ type: Number,
+ value: 5,
+ observer(value) {
+ this.setData({ innerCountArray: Array.from({ length: value }) });
+ },
+ },
+ gutter: null,
+ touchable: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ innerValue: 0,
+ innerCountArray: Array.from({ length: 5 }),
+ },
+ methods: {
+ onSelect(event) {
+ const { data } = this;
+ const { score } = event.currentTarget.dataset;
+ if (!data.disabled && !data.readonly) {
+ this.setData({ innerValue: score + 1 });
+ if (canIUseModel()) {
+ this.setData({ value: score + 1 });
+ }
+ wx.nextTick(() => {
+ this.$emit('input', score + 1);
+ this.$emit('change', score + 1);
+ });
+ }
+ },
+ onTouchMove(event) {
+ const { touchable } = this.data;
+ if (!touchable)
+ return;
+ const { clientX } = event.touches[0];
+ getAllRect(this, '.van-rate__icon').then((list) => {
+ const target = list
+ .sort((cur, next) => cur.dataset.score - next.dataset.score)
+ .find((item) => clientX >= item.left && clientX <= item.right);
+ if (target != null) {
+ this.onSelect(Object.assign(Object.assign({}, event), { currentTarget: target }));
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxml
new file mode 100644
index 0000000..049714c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxss
new file mode 100644
index 0000000..470e4f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/rate/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-rate{display:inline-flex;-webkit-user-select:none;user-select:none}.van-rate__item{padding:0 var(--rate-horizontal-padding,2px);position:relative}.van-rate__item:not(:last-child){padding-right:var(--rate-icon-gutter,4px)}.van-rate__icon{color:var(--rate-icon-void-color,#c8c9cc);display:block;font-size:var(--rate-icon-size,20px);height:100%}.van-rate__icon--half{left:var(--rate-horizontal-padding,2px);overflow:hidden;position:absolute;top:0;width:.5em}.van-rate__icon--full,.van-rate__icon--half{color:var(--rate-icon-full-color,#ee0a24)}.van-rate__icon--disabled{color:var(--rate-icon-disabled-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.js
new file mode 100644
index 0000000..cc844f8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.js
@@ -0,0 +1,23 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ relation: useChildren('col', function (target) {
+ const { gutter } = this.data;
+ if (gutter) {
+ target.setData({ gutter });
+ }
+ }),
+ props: {
+ gutter: {
+ type: Number,
+ observer: 'setGutter',
+ },
+ },
+ methods: {
+ setGutter() {
+ this.children.forEach((col) => {
+ col.setData(this.data);
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxml
new file mode 100644
index 0000000..69a4359
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxs
new file mode 100644
index 0000000..f5c5958
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'margin-right': addUnit(-data.gutter / 2),
+ 'margin-left': addUnit(-data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxss
new file mode 100644
index 0000000..bb8946b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/row/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-row:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.js
new file mode 100644
index 0000000..d3c1bcd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.js
@@ -0,0 +1,97 @@
+import { VantComponent } from '../common/component';
+import { canIUseModel } from '../common/version';
+VantComponent({
+ field: true,
+ classes: ['field-class', 'input-class', 'cancel-class'],
+ props: {
+ value: {
+ type: String,
+ value: '',
+ },
+ label: String,
+ focus: Boolean,
+ error: Boolean,
+ disabled: Boolean,
+ readonly: Boolean,
+ inputAlign: String,
+ showAction: Boolean,
+ useActionSlot: Boolean,
+ useLeftIconSlot: Boolean,
+ useRightIconSlot: Boolean,
+ leftIcon: {
+ type: String,
+ value: 'search',
+ },
+ rightIcon: String,
+ placeholder: String,
+ placeholderStyle: String,
+ actionText: {
+ type: String,
+ value: '取消',
+ },
+ background: {
+ type: String,
+ value: '#ffffff',
+ },
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ shape: {
+ type: String,
+ value: 'square',
+ },
+ clearable: {
+ type: Boolean,
+ value: true,
+ },
+ clearTrigger: {
+ type: String,
+ value: 'focus',
+ },
+ clearIcon: {
+ type: String,
+ value: 'clear',
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 0,
+ },
+ },
+ methods: {
+ onChange(event) {
+ if (canIUseModel()) {
+ this.setData({ value: event.detail });
+ }
+ this.$emit('change', event.detail);
+ },
+ onCancel() {
+ /**
+ * 修复修改输入框值时,输入框失焦和赋值同时触发,赋值失效
+ * https://github.com/youzan/vant-weapp/issues/1768
+ */
+ setTimeout(() => {
+ if (canIUseModel()) {
+ this.setData({ value: '' });
+ }
+ this.$emit('cancel');
+ this.$emit('change', '');
+ }, 200);
+ },
+ onSearch(event) {
+ this.$emit('search', event.detail);
+ },
+ onFocus(event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur(event) {
+ this.$emit('blur', event.detail);
+ },
+ onClear(event) {
+ this.$emit('clear', event.detail);
+ },
+ onClickInput(event) {
+ this.$emit('click-input', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.json
new file mode 100644
index 0000000..b4cfe91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-field": "../field/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxml
new file mode 100644
index 0000000..cbcb47a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+ {{ label }}
+
+
+
+
+
+
+
+
+
+
+ {{ actionText }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxss
new file mode 100644
index 0000000..4f306b0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/search/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-search{align-items:center;box-sizing:border-box;display:flex;padding:var(--search-padding,10px 12px)}.van-search__content{background-color:var(--search-background-color,#f7f8fa);border-radius:2px;display:flex;flex:1;padding-left:var(--padding-sm,12px)}.van-search__content--round{border-radius:999px}.van-search__label{color:var(--search-label-color,#323233);font-size:var(--search-label-font-size,14px);line-height:var(--search-input-height,34px);padding:var(--search-label-padding,0 5px)}.van-search__field{flex:1}.van-search__field__left-icon{color:var(--search-left-icon-color,#969799)}.van-search--withaction{padding-right:0}.van-search__action{color:var(--search-action-text-color,#323233);font-size:var(--search-action-font-size,14px);line-height:var(--search-input-height,34px)}.van-search__action--hover{background-color:#f2f3f5}.van-search__action-button{padding:var(--search-action-padding,0 8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.js
new file mode 100644
index 0000000..ad2ccf4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.js
@@ -0,0 +1,59 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ // whether to show popup
+ show: Boolean,
+ // overlay custom style
+ overlayStyle: String,
+ // z-index
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ title: String,
+ cancelText: {
+ type: String,
+ value: '取消',
+ },
+ description: String,
+ options: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: null,
+ value: 300,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClickOverlay() {
+ this.$emit('click-overlay');
+ },
+ onCancel() {
+ this.onClose();
+ this.$emit('cancel');
+ },
+ onSelect(event) {
+ this.$emit('select', event.detail);
+ },
+ onClose() {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.json
new file mode 100644
index 0000000..15a7c22
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "options": "./options"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxml
new file mode 100644
index 0000000..72a5b25
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxs
new file mode 100644
index 0000000..2149ee9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+function isMulti(options) {
+ if (options == null || options[0] == null) {
+ return false;
+ }
+
+ return "Array" === options.constructor && "Array" === options[0].constructor;
+}
+
+module.exports = {
+ isMulti: isMulti
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxss
new file mode 100644
index 0000000..e8d8dae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__header{padding:12px 16px 4px;text-align:center}.van-share-sheet__title{color:#323233;font-size:14px;font-weight:400;line-height:20px;margin-top:8px}.van-share-sheet__title:empty,.van-share-sheet__title:not(:empty)+.van-share-sheet__title{display:none}.van-share-sheet__description{color:#969799;display:block;font-size:12px;line-height:16px;margin-top:8px}.van-share-sheet__description:empty,.van-share-sheet__description:not(:empty)+.van-share-sheet__description{display:none}.van-share-sheet__cancel{background:#fff;border:none;box-sizing:initial;display:block;font-size:16px;height:auto;line-height:48px;padding:0;text-align:center;width:100%}.van-share-sheet__cancel:before{background-color:#f7f8fa;content:" ";display:block;height:8px}.van-share-sheet__cancel:after{display:none}.van-share-sheet__cancel:active{background-color:#f2f3f5}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.js
new file mode 100644
index 0000000..5a29ad7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.js
@@ -0,0 +1,14 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ options: Array,
+ showBorder: Boolean,
+ },
+ methods: {
+ onSelect(event) {
+ const { index } = event.currentTarget.dataset;
+ const option = this.data.options[index];
+ this.$emit('select', Object.assign(Object.assign({}, option), { index }));
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxml
new file mode 100644
index 0000000..2983ebb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxs
new file mode 100644
index 0000000..a116d32
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var PRESET_ICONS = ['qq', 'link', 'weibo', 'wechat', 'poster', 'qrcode', 'weapp-qrcode', 'wechat-moments'];
+
+function getIconURL(icon) {
+ if (PRESET_ICONS.indexOf(icon) !== -1) {
+ return 'https://img.yzcdn.cn/vant/share-sheet-' + icon + '.png';
+ }
+
+ return icon;
+}
+
+module.exports = {
+ getIconURL: getIconURL,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxss
new file mode 100644
index 0000000..b7f5455
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/share-sheet/options.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__options{-webkit-overflow-scrolling:touch;display:flex;overflow-x:auto;overflow-y:visible;padding:16px 0 16px 8px;position:relative}.van-share-sheet__options--border:before{border-top:1px solid #ebedf0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:0;top:0;transform:scaleY(.5);transform-origin:center}.van-share-sheet__options::-webkit-scrollbar{height:0}.van-share-sheet__option{align-items:center;display:flex;flex-direction:column;-webkit-user-select:none;user-select:none}.van-share-sheet__option:active{opacity:.7}.van-share-sheet__button{background-color:initial;border:0;height:auto;line-height:inherit;padding:0}.van-share-sheet__button:after{border:0}.van-share-sheet__icon{height:48px;margin:0 16px;width:48px}.van-share-sheet__name{color:#646566;font-size:12px;margin-top:8px;padding:0 4px}.van-share-sheet__option-description{color:#c8c9cc;font-size:12px;padding:0 4px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.js
new file mode 100644
index 0000000..63ea57d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.js
@@ -0,0 +1,29 @@
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+VantComponent({
+ classes: ['active-class', 'disabled-class'],
+ relation: useParent('sidebar'),
+ props: {
+ dot: Boolean,
+ badge: null,
+ info: null,
+ title: String,
+ disabled: Boolean,
+ },
+ methods: {
+ onClick() {
+ const { parent } = this;
+ if (!parent || this.data.disabled) {
+ return;
+ }
+ const index = parent.children.indexOf(this);
+ parent.setActive(index).then(() => {
+ this.$emit('click', index);
+ parent.$emit('change', index);
+ });
+ },
+ setActive(selected) {
+ return this.setData({ selected });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxml
new file mode 100644
index 0000000..c5c08a6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxml
@@ -0,0 +1,18 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxss
new file mode 100644
index 0000000..f1ce421
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar-item{background-color:var(--sidebar-background-color,#f7f8fa);border-left:3px solid transparent;box-sizing:border-box;color:var(--sidebar-text-color,#323233);display:block;font-size:var(--sidebar-font-size,14px);line-height:var(--sidebar-line-height,20px);overflow:hidden;padding:var(--sidebar-padding,20px 12px 20px 8px);-webkit-user-select:none;user-select:none}.van-sidebar-item__text{display:inline-block;position:relative;word-break:break-all}.van-sidebar-item--hover:not(.van-sidebar-item--disabled){background-color:var(--sidebar-active-color,#f2f3f5)}.van-sidebar-item:after{border-bottom-width:1px}.van-sidebar-item--selected{border-color:var(--sidebar-selected-border-color,#ee0a24);color:var(--sidebar-selected-text-color,#323233);font-weight:var(--sidebar-selected-font-weight,500)}.van-sidebar-item--selected:after{border-right-width:1px}.van-sidebar-item--selected,.van-sidebar-item--selected.van-sidebar-item--hover{background-color:var(--sidebar-selected-background-color,#fff)}.van-sidebar-item--disabled{color:var(--sidebar-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.js
new file mode 100644
index 0000000..d763e06
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.js
@@ -0,0 +1,34 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+VantComponent({
+ relation: useChildren('sidebar-item', function () {
+ this.setActive(this.data.activeKey);
+ }),
+ props: {
+ activeKey: {
+ type: Number,
+ value: 0,
+ observer: 'setActive',
+ },
+ },
+ beforeCreate() {
+ this.currentActive = -1;
+ },
+ methods: {
+ setActive(activeKey) {
+ const { children, currentActive } = this;
+ if (!children.length) {
+ return Promise.resolve();
+ }
+ this.currentActive = activeKey;
+ const stack = [];
+ if (currentActive !== activeKey && children[currentActive]) {
+ stack.push(children[currentActive].setActive(false));
+ }
+ if (children[activeKey]) {
+ stack.push(children[activeKey].setActive(true));
+ }
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxml
new file mode 100644
index 0000000..96b11c7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxml
@@ -0,0 +1,3 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxss
new file mode 100644
index 0000000..5a2d44f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sidebar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar{width:var(--sidebar-width,80px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.js
new file mode 100644
index 0000000..33b1141
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.js
@@ -0,0 +1,46 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: ['avatar-class', 'title-class', 'row-class'],
+ props: {
+ row: {
+ type: Number,
+ value: 0,
+ observer(value) {
+ this.setData({ rowArray: Array.from({ length: value }) });
+ },
+ },
+ title: Boolean,
+ avatar: Boolean,
+ loading: {
+ type: Boolean,
+ value: true,
+ },
+ animate: {
+ type: Boolean,
+ value: true,
+ },
+ avatarSize: {
+ type: String,
+ value: '32px',
+ },
+ avatarShape: {
+ type: String,
+ value: 'round',
+ },
+ titleWidth: {
+ type: String,
+ value: '40%',
+ },
+ rowWidth: {
+ type: null,
+ value: '100%',
+ observer(val) {
+ this.setData({ isArray: val instanceof Array });
+ },
+ },
+ },
+ data: {
+ isArray: false,
+ rowArray: [],
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxml
new file mode 100644
index 0000000..058e2ef
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxml
@@ -0,0 +1,29 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxss
new file mode 100644
index 0000000..d59a5ed
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/skeleton/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-skeleton{box-sizing:border-box;display:flex;padding:var(--skeleton-padding,0 16px);width:100%}.van-skeleton__avatar{background-color:var(--skeleton-avatar-background-color,#f2f3f5);flex-shrink:0;margin-right:var(--padding-md,16px)}.van-skeleton__avatar--round{border-radius:100%}.van-skeleton__content{flex:1}.van-skeleton__avatar+.van-skeleton__content{padding-top:var(--padding-xs,8px)}.van-skeleton__row,.van-skeleton__title{background-color:var(--skeleton-row-background-color,#f2f3f5);height:var(--skeleton-row-height,16px)}.van-skeleton__title{margin:0}.van-skeleton__row:not(:first-child){margin-top:var(--skeleton-row-margin-top,12px)}.van-skeleton__title+.van-skeleton__row{margin-top:20px}.van-skeleton--animate{animation:van-skeleton-blink 1.2s ease-in-out infinite}@keyframes van-skeleton-blink{50%{opacity:.6}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.js
new file mode 100644
index 0000000..26f83ba
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.js
@@ -0,0 +1,207 @@
+import { VantComponent } from '../common/component';
+import { touch } from '../mixins/touch';
+import { canIUseModel } from '../common/version';
+import { getRect, addUnit, nextTick, addNumber, clamp } from '../common/utils';
+const DRAG_STATUS = {
+ START: 'start',
+ MOVING: 'moving',
+ END: 'end',
+};
+VantComponent({
+ mixins: [touch],
+ props: {
+ range: Boolean,
+ disabled: Boolean,
+ useButtonSlot: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ max: {
+ type: Number,
+ value: 100,
+ },
+ min: {
+ type: Number,
+ value: 0,
+ },
+ step: {
+ type: Number,
+ value: 1,
+ },
+ value: {
+ type: null,
+ value: 0,
+ observer(val) {
+ if (val !== this.value) {
+ this.updateValue(val);
+ }
+ },
+ },
+ vertical: Boolean,
+ barHeight: null,
+ },
+ created() {
+ this.updateValue(this.data.value);
+ },
+ methods: {
+ onTouchStart(event) {
+ if (this.data.disabled)
+ return;
+ const { index } = event.currentTarget.dataset;
+ if (typeof index === 'number') {
+ this.buttonIndex = index;
+ }
+ this.touchStart(event);
+ this.startValue = this.format(this.value);
+ this.newValue = this.value;
+ if (this.isRange(this.newValue)) {
+ this.startValue = this.newValue.map((val) => this.format(val));
+ }
+ else {
+ this.startValue = this.format(this.newValue);
+ }
+ this.dragStatus = DRAG_STATUS.START;
+ },
+ onTouchMove(event) {
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.START) {
+ this.$emit('drag-start');
+ }
+ this.touchMove(event);
+ this.dragStatus = DRAG_STATUS.MOVING;
+ getRect(this, '.van-slider').then((rect) => {
+ const { vertical } = this.data;
+ const delta = vertical ? this.deltaY : this.deltaX;
+ const total = vertical ? rect.height : rect.width;
+ const diff = (delta / total) * this.getRange();
+ if (this.isRange(this.startValue)) {
+ this.newValue[this.buttonIndex] =
+ this.startValue[this.buttonIndex] + diff;
+ }
+ else {
+ this.newValue = this.startValue + diff;
+ }
+ this.updateValue(this.newValue, false, true);
+ });
+ },
+ onTouchEnd() {
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.MOVING) {
+ this.dragStatus = DRAG_STATUS.END;
+ nextTick(() => {
+ this.updateValue(this.newValue, true);
+ this.$emit('drag-end');
+ });
+ }
+ },
+ onClick(event) {
+ if (this.data.disabled)
+ return;
+ const { min } = this.data;
+ getRect(this, '.van-slider').then((rect) => {
+ const { vertical } = this.data;
+ const touch = event.touches[0];
+ const delta = vertical
+ ? touch.clientY - rect.top
+ : touch.clientX - rect.left;
+ const total = vertical ? rect.height : rect.width;
+ const value = Number(min) + (delta / total) * this.getRange();
+ if (this.isRange(this.value)) {
+ const [left, right] = this.value;
+ const middle = (left + right) / 2;
+ if (value <= middle) {
+ this.updateValue([value, right], true);
+ }
+ else {
+ this.updateValue([left, value], true);
+ }
+ }
+ else {
+ this.updateValue(value, true);
+ }
+ });
+ },
+ isRange(val) {
+ const { range } = this.data;
+ return range && Array.isArray(val);
+ },
+ handleOverlap(value) {
+ if (value[0] > value[1]) {
+ return value.slice(0).reverse();
+ }
+ return value;
+ },
+ updateValue(value, end, drag) {
+ if (this.isRange(value)) {
+ value = this.handleOverlap(value).map((val) => this.format(val));
+ }
+ else {
+ value = this.format(value);
+ }
+ this.value = value;
+ const { vertical } = this.data;
+ const mainAxis = vertical ? 'height' : 'width';
+ this.setData({
+ wrapperStyle: `
+ background: ${this.data.inactiveColor || ''};
+ ${vertical ? 'width' : 'height'}: ${addUnit(this.data.barHeight) || ''};
+ `,
+ barStyle: `
+ ${mainAxis}: ${this.calcMainAxis()};
+ left: ${vertical ? 0 : this.calcOffset()};
+ top: ${vertical ? this.calcOffset() : 0};
+ ${drag ? 'transition: none;' : ''}
+ `,
+ });
+ if (drag) {
+ this.$emit('drag', { value });
+ }
+ if (end) {
+ this.$emit('change', value);
+ }
+ if ((drag || end) && canIUseModel()) {
+ this.setData({ value });
+ }
+ },
+ getScope() {
+ return Number(this.data.max) - Number(this.data.min);
+ },
+ getRange() {
+ const { max, min } = this.data;
+ return max - min;
+ },
+ getOffsetWidth(current, min) {
+ const scope = this.getScope();
+ // 避免最小值小于最小step时出现负数情况
+ return `${Math.max(((current - min) * 100) / scope, 0)}%`;
+ },
+ // 计算选中条的长度百分比
+ calcMainAxis() {
+ const { value } = this;
+ const { min } = this.data;
+ if (this.isRange(value)) {
+ return this.getOffsetWidth(value[1], value[0]);
+ }
+ return this.getOffsetWidth(value, Number(min));
+ },
+ // 计算选中条的开始位置的偏移量
+ calcOffset() {
+ const { value } = this;
+ const { min } = this.data;
+ const scope = this.getScope();
+ if (this.isRange(value)) {
+ return `${((value[0] - Number(min)) * 100) / scope}%`;
+ }
+ return '0%';
+ },
+ format(value) {
+ const min = +this.data.min;
+ const max = +this.data.max;
+ const step = +this.data.step;
+ value = clamp(value, min, max);
+ const diff = Math.round((value - min) / step) * step;
+ return addNumber(min, diff);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxml
new file mode 100644
index 0000000..7c0184f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxml
@@ -0,0 +1,68 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxs
new file mode 100644
index 0000000..7c43e6e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function barStyle(barHeight, activeColor) {
+ return style({
+ height: addUnit(barHeight),
+ background: activeColor,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxss
new file mode 100644
index 0000000..d1587de
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/slider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-slider{background-color:var(--slider-inactive-background-color,#ebedf0);border-radius:999px;height:var(--slider-bar-height,2px);position:relative}.van-slider:before{bottom:calc(var(--padding-xs, 8px)*-1);content:"";left:0;position:absolute;right:0;top:calc(var(--padding-xs, 8px)*-1)}.van-slider__bar{background-color:var(--slider-active-background-color,#1989fa);border-radius:inherit;height:100%;position:relative;transition:all .2s;width:100%}.van-slider__button{background-color:var(--slider-button-background-color,#fff);border-radius:var(--slider-button-border-radius,50%);box-shadow:var(--slider-button-box-shadow,0 1px 2px rgba(0,0,0,.5));height:var(--slider-button-height,24px);width:var(--slider-button-width,24px)}.van-slider__button-wrapper,.van-slider__button-wrapper-right{position:absolute;right:0;top:50%;transform:translate3d(50%,-50%,0)}.van-slider__button-wrapper-left{left:0;position:absolute;top:50%;transform:translate3d(-50%,-50%,0)}.van-slider--disabled{opacity:var(--slider-disabled-opacity,.5)}.van-slider--vertical{display:inline-block;height:100%;width:var(--slider-bar-height,2px)}.van-slider--vertical .van-slider__button-wrapper,.van-slider--vertical .van-slider__button-wrapper-right{bottom:0;right:50%;top:auto;transform:translate3d(50%,50%,0)}.van-slider--vertical .van-slider__button-wrapper-left{left:auto;right:50%;top:0;transform:translate3d(50%,-50%,0)}.van-slider--vertical:before{bottom:0;left:-8px;right:-8px;top:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.js
new file mode 100644
index 0000000..937ae6e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.js
@@ -0,0 +1,188 @@
+import { VantComponent } from '../common/component';
+import { isDef } from '../common/validator';
+const LONG_PRESS_START_TIME = 600;
+const LONG_PRESS_INTERVAL = 200;
+// add num and avoid float number
+function add(num1, num2) {
+ const cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+function equal(value1, value2) {
+ return String(value1) === String(value2);
+}
+VantComponent({
+ field: true,
+ classes: ['input-class', 'plus-class', 'minus-class'],
+ props: {
+ value: {
+ type: null,
+ },
+ integer: {
+ type: Boolean,
+ observer: 'check',
+ },
+ disabled: Boolean,
+ inputWidth: String,
+ buttonSize: String,
+ asyncChange: Boolean,
+ disableInput: Boolean,
+ decimalLength: {
+ type: Number,
+ value: null,
+ observer: 'check',
+ },
+ min: {
+ type: null,
+ value: 1,
+ observer: 'check',
+ },
+ max: {
+ type: null,
+ value: Number.MAX_SAFE_INTEGER,
+ observer: 'check',
+ },
+ step: {
+ type: null,
+ value: 1,
+ },
+ showPlus: {
+ type: Boolean,
+ value: true,
+ },
+ showMinus: {
+ type: Boolean,
+ value: true,
+ },
+ disablePlus: Boolean,
+ disableMinus: Boolean,
+ longPress: {
+ type: Boolean,
+ value: true,
+ },
+ theme: String,
+ alwaysEmbed: Boolean,
+ },
+ data: {
+ currentValue: '',
+ },
+ watch: {
+ value() {
+ this.observeValue();
+ },
+ },
+ created() {
+ this.setData({
+ currentValue: this.format(this.data.value),
+ });
+ },
+ methods: {
+ observeValue() {
+ const { value } = this.data;
+ this.setData({ currentValue: this.format(value) });
+ },
+ check() {
+ const val = this.format(this.data.currentValue);
+ if (!equal(val, this.data.currentValue)) {
+ this.setData({ currentValue: val });
+ }
+ },
+ isDisabled(type) {
+ const { disabled, disablePlus, disableMinus, currentValue, max, min } = this.data;
+ if (type === 'plus') {
+ return disabled || disablePlus || +currentValue >= +max;
+ }
+ return disabled || disableMinus || +currentValue <= +min;
+ },
+ onFocus(event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur(event) {
+ const value = this.format(event.detail.value);
+ this.setData({ currentValue: value });
+ this.emitChange(value);
+ this.$emit('blur', Object.assign(Object.assign({}, event.detail), { value }));
+ },
+ // filter illegal characters
+ filter(value) {
+ value = String(value).replace(/[^0-9.-]/g, '');
+ if (this.data.integer && value.indexOf('.') !== -1) {
+ value = value.split('.')[0];
+ }
+ return value;
+ },
+ // limit value range
+ format(value) {
+ value = this.filter(value);
+ // format range
+ value = value === '' ? 0 : +value;
+ value = Math.max(Math.min(this.data.max, value), this.data.min);
+ // format decimal
+ if (isDef(this.data.decimalLength)) {
+ value = value.toFixed(this.data.decimalLength);
+ }
+ return value;
+ },
+ onInput(event) {
+ const { value = '' } = event.detail || {};
+ // allow input to be empty
+ if (value === '') {
+ return;
+ }
+ let formatted = this.filter(value);
+ // limit max decimal length
+ if (isDef(this.data.decimalLength) && formatted.indexOf('.') !== -1) {
+ const pair = formatted.split('.');
+ formatted = `${pair[0]}.${pair[1].slice(0, this.data.decimalLength)}`;
+ }
+ this.emitChange(formatted);
+ },
+ emitChange(value) {
+ if (!this.data.asyncChange) {
+ this.setData({ currentValue: value });
+ }
+ this.$emit('change', value);
+ },
+ onChange() {
+ const { type } = this;
+ if (this.isDisabled(type)) {
+ this.$emit('overlimit', type);
+ return;
+ }
+ const diff = type === 'minus' ? -this.data.step : +this.data.step;
+ const value = this.format(add(+this.data.currentValue, diff));
+ this.emitChange(value);
+ this.$emit(type);
+ },
+ longPressStep() {
+ this.longPressTimer = setTimeout(() => {
+ this.onChange();
+ this.longPressStep();
+ }, LONG_PRESS_INTERVAL);
+ },
+ onTap(event) {
+ const { type } = event.currentTarget.dataset;
+ this.type = type;
+ this.onChange();
+ },
+ onTouchStart(event) {
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ const { type } = event.currentTarget.dataset;
+ this.type = type;
+ this.isLongPress = false;
+ this.longPressTimer = setTimeout(() => {
+ this.isLongPress = true;
+ this.onChange();
+ this.longPressStep();
+ }, LONG_PRESS_START_TIME);
+ },
+ onTouchEnd() {
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxml
new file mode 100644
index 0000000..6dd44bc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxml
@@ -0,0 +1,43 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxs
new file mode 100644
index 0000000..a13e818
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function buttonStyle(data) {
+ return style({
+ width: addUnit(data.buttonSize),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+function inputStyle(data) {
+ return style({
+ width: addUnit(data.inputWidth),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+module.exports = {
+ buttonStyle: buttonStyle,
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxss
new file mode 100644
index 0000000..2561a7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/stepper/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-stepper{font-size:0}.van-stepper__minus,.van-stepper__plus{background-color:var(--stepper-background-color,#f2f3f5);border:0;box-sizing:border-box;color:var(--stepper-button-icon-color,#323233);display:inline-block;height:var(--stepper-input-height,28px);margin:1px;padding:var(--padding-base,4px);position:relative;vertical-align:middle;width:var(--stepper-input-height,28px)}.van-stepper__minus:before,.van-stepper__plus:before{height:1px;width:9px}.van-stepper__minus:after,.van-stepper__plus:after{height:9px;width:1px}.van-stepper__minus:empty.van-stepper__minus:after,.van-stepper__minus:empty.van-stepper__minus:before,.van-stepper__minus:empty.van-stepper__plus:after,.van-stepper__minus:empty.van-stepper__plus:before,.van-stepper__plus:empty.van-stepper__minus:after,.van-stepper__plus:empty.van-stepper__minus:before,.van-stepper__plus:empty.van-stepper__plus:after,.van-stepper__plus:empty.van-stepper__plus:before{background-color:currentColor;bottom:0;content:"";left:0;margin:auto;position:absolute;right:0;top:0}.van-stepper__minus--hover,.van-stepper__plus--hover{background-color:var(--stepper-active-color,#e8e8e8)}.van-stepper__minus--disabled,.van-stepper__plus--disabled{color:var(--stepper-button-disabled-icon-color,#c8c9cc)}.van-stepper__minus--disabled,.van-stepper__minus--disabled.van-stepper__minus--hover,.van-stepper__minus--disabled.van-stepper__plus--hover,.van-stepper__plus--disabled,.van-stepper__plus--disabled.van-stepper__minus--hover,.van-stepper__plus--disabled.van-stepper__plus--hover{background-color:var(--stepper-button-disabled-color,#f7f8fa)}.van-stepper__minus{border-radius:var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0 0 var(--stepper-border-radius,var(--stepper-border-radius,4px))}.van-stepper__minus:after{display:none}.van-stepper__plus{border-radius:0 var(--stepper-border-radius,var(--stepper-border-radius,4px)) var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0}.van-stepper--round .van-stepper__input{background-color:initial!important}.van-stepper--round .van-stepper__minus,.van-stepper--round .van-stepper__plus{border-radius:100%}.van-stepper--round .van-stepper__minus:active,.van-stepper--round .van-stepper__plus:active{opacity:.7}.van-stepper--round .van-stepper__minus--disabled,.van-stepper--round .van-stepper__minus--disabled:active,.van-stepper--round .van-stepper__plus--disabled,.van-stepper--round .van-stepper__plus--disabled:active{opacity:.3}.van-stepper--round .van-stepper__plus{background-color:#ee0a24;color:#fff}.van-stepper--round .van-stepper__minus{background-color:#fff;border:1px solid #ee0a24;color:#ee0a24}.van-stepper__input{-webkit-appearance:none;background-color:var(--stepper-background-color,#f2f3f5);border:0;border-radius:0;border-width:1px 0;box-sizing:border-box;color:var(--stepper-input-text-color,#323233);display:inline-block;font-size:var(--stepper-input-font-size,14px);height:var(--stepper-input-height,28px);margin:1px;min-height:0;padding:1px;text-align:center;vertical-align:middle;width:var(--stepper-input-width,32px)}.van-stepper__input--disabled{background-color:var(--stepper-input-disabled-background-color,#f2f3f5);color:var(--stepper-input-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.js
new file mode 100644
index 0000000..b47be76
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.js
@@ -0,0 +1,33 @@
+import { VantComponent } from '../common/component';
+import { GREEN, GRAY_DARK } from '../common/color';
+VantComponent({
+ classes: ['desc-class'],
+ props: {
+ icon: String,
+ steps: Array,
+ active: Number,
+ direction: {
+ type: String,
+ value: 'horizontal',
+ },
+ activeColor: {
+ type: String,
+ value: GREEN,
+ },
+ inactiveColor: {
+ type: String,
+ value: GRAY_DARK,
+ },
+ activeIcon: {
+ type: String,
+ value: 'checked',
+ },
+ inactiveIcon: String,
+ },
+ methods: {
+ onClick(event) {
+ const { index } = event.currentTarget.dataset;
+ this.$emit('click-step', index);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxml
new file mode 100644
index 0000000..00c8e10
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+ {{ item.text }}
+ {{ item.desc }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+function get(index, active) {
+ if (index < active) {
+ return 'finish';
+ } else if (index === active) {
+ return 'process';
+ }
+
+ return 'inactive';
+}
+
+module.exports = get;
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxss
new file mode 100644
index 0000000..c653884
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/steps/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-steps{background-color:var(--steps-background-color,#fff);overflow:hidden}.van-steps--horizontal{padding:10px}.van-steps--horizontal .van-step__wrapper{display:flex;overflow:hidden;position:relative}.van-steps--vertical{padding-left:10px}.van-steps--vertical .van-step__wrapper{padding:0 0 0 20px}.van-step{color:var(--step-text-color,#969799);flex:1;font-size:var(--step-font-size,14px);position:relative}.van-step--finish{color:var(--step-finish-text-color,#323233)}.van-step__circle{background-color:var(--step-circle-color,#969799);border-radius:50%;height:var(--step-circle-size,5px);width:var(--step-circle-size,5px)}.van-step--horizontal{padding-bottom:14px}.van-step--horizontal:first-child .van-step__title{transform:none}.van-step--horizontal:first-child .van-step__circle-container{padding:0 8px 0 0;transform:translate3d(0,50%,0)}.van-step--horizontal:last-child{position:absolute;right:0;width:auto}.van-step--horizontal:last-child .van-step__title{text-align:right;transform:none}.van-step--horizontal:last-child .van-step__circle-container{padding:0 0 0 8px;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal .van-step__circle-container{background-color:#fff;bottom:6px;padding:0 var(--padding-xs,8px);position:absolute;transform:translate3d(-50%,50%,0);z-index:1}.van-step--horizontal .van-step__title{display:inline-block;font-size:var(--step-horizontal-title-font-size,12px);transform:translate3d(-50%,0,0)}.van-step--horizontal .van-step__line{background-color:var(--step-line-color,#ebedf0);bottom:6px;height:1px;left:0;position:absolute;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal.van-step--process{color:var(--step-process-text-color,#323233)}.van-step--horizontal.van-step--process .van-step__icon{display:block;font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical{line-height:18px;padding:10px 10px 10px 0}.van-step--vertical:after{border-bottom-width:1px}.van-step--vertical:last-child:after{border-bottom-width:none}.van-step--vertical:first-child:before{background-color:#fff;content:"";height:20px;left:-15px;position:absolute;top:0;width:1px;z-index:1}.van-step--vertical .van-step__circle,.van-step--vertical .van-step__icon,.van-step--vertical .van-step__line{left:-14px;position:absolute;top:19px;transform:translate3d(-50%,-50%,0);z-index:2}.van-step--vertical .van-step__icon{background-color:var(--steps-background-color,#fff);font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical .van-step__line{background-color:var(--step-line-color,#ebedf0);height:100%;transform:translate3d(-50%,0,0);width:1px;z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.js
new file mode 100644
index 0000000..df45e3c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.js
@@ -0,0 +1,120 @@
+import { getRect } from '../common/utils';
+import { VantComponent } from '../common/component';
+import { isDef } from '../common/validator';
+import { pageScrollMixin } from '../mixins/page-scroll';
+const ROOT_ELEMENT = '.van-sticky';
+VantComponent({
+ props: {
+ zIndex: {
+ type: Number,
+ value: 99,
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ observer: 'onScroll',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'onScroll',
+ },
+ container: {
+ type: null,
+ observer: 'onScroll',
+ },
+ scrollTop: {
+ type: null,
+ observer(val) {
+ this.onScroll({ scrollTop: val });
+ },
+ },
+ },
+ mixins: [
+ pageScrollMixin(function (event) {
+ if (this.data.scrollTop != null) {
+ return;
+ }
+ this.onScroll(event);
+ }),
+ ],
+ data: {
+ height: 0,
+ fixed: false,
+ transform: 0,
+ },
+ mounted() {
+ this.onScroll();
+ },
+ methods: {
+ onScroll({ scrollTop } = {}) {
+ const { container, offsetTop, disabled } = this.data;
+ if (disabled) {
+ this.setDataAfterDiff({
+ fixed: false,
+ transform: 0,
+ });
+ return;
+ }
+ this.scrollTop = scrollTop || this.scrollTop;
+ if (typeof container === 'function') {
+ Promise.all([getRect(this, ROOT_ELEMENT), this.getContainerRect()])
+ .then(([root, container]) => {
+ if (offsetTop + root.height > container.height + container.top) {
+ this.setDataAfterDiff({
+ fixed: false,
+ transform: container.height - root.height,
+ });
+ }
+ else if (offsetTop >= root.top) {
+ this.setDataAfterDiff({
+ fixed: true,
+ height: root.height,
+ transform: 0,
+ });
+ }
+ else {
+ this.setDataAfterDiff({ fixed: false, transform: 0 });
+ }
+ })
+ .catch(() => { });
+ return;
+ }
+ getRect(this, ROOT_ELEMENT).then((root) => {
+ if (!isDef(root) || (!root.width && !root.height)) {
+ return;
+ }
+ if (offsetTop >= root.top) {
+ this.setDataAfterDiff({ fixed: true, height: root.height });
+ this.transform = 0;
+ }
+ else {
+ this.setDataAfterDiff({ fixed: false });
+ }
+ });
+ },
+ setDataAfterDiff(data) {
+ wx.nextTick(() => {
+ const diff = Object.keys(data).reduce((prev, key) => {
+ if (data[key] !== this.data[key]) {
+ prev[key] = data[key];
+ }
+ return prev;
+ }, {});
+ if (Object.keys(diff).length > 0) {
+ this.setData(diff);
+ }
+ this.$emit('scroll', {
+ scrollTop: this.scrollTop,
+ isFixed: data.fixed || this.data.fixed,
+ });
+ });
+ },
+ getContainerRect() {
+ const nodesRef = this.data.container();
+ if (!nodesRef) {
+ return Promise.reject(new Error('not found container'));
+ }
+ return new Promise((resolve) => nodesRef.boundingClientRect(resolve).exec());
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxml
new file mode 100644
index 0000000..15e9f4a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxs
new file mode 100644
index 0000000..be99d89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapStyle(data) {
+ return style({
+ transform: data.transform
+ ? 'translate3d(0, ' + data.transform + 'px, 0)'
+ : '',
+ top: data.fixed ? addUnit(data.offsetTop) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+function containerStyle(data) {
+ return style({
+ height: data.fixed ? addUnit(data.height) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+module.exports = {
+ wrapStyle: wrapStyle,
+ containerStyle: containerStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxss
new file mode 100644
index 0000000..34d76aa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/sticky/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sticky{position:relative}.van-sticky-wrap--fixed{left:0;position:fixed;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.js
new file mode 100644
index 0000000..decf459
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.js
@@ -0,0 +1,56 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: ['bar-class', 'price-class', 'button-class'],
+ props: {
+ tip: {
+ type: null,
+ observer: 'updateTip',
+ },
+ tipIcon: String,
+ type: Number,
+ price: {
+ type: null,
+ observer: 'updatePrice',
+ },
+ label: String,
+ loading: Boolean,
+ disabled: Boolean,
+ buttonText: String,
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ buttonType: {
+ type: String,
+ value: 'danger',
+ },
+ decimalLength: {
+ type: Number,
+ value: 2,
+ observer: 'updatePrice',
+ },
+ suffixLabel: String,
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updatePrice() {
+ const { price, decimalLength } = this.data;
+ const priceStrArr = typeof price === 'number' &&
+ (price / 100).toFixed(decimalLength).split('.');
+ this.setData({
+ hasPrice: typeof price === 'number',
+ integerStr: priceStrArr && priceStrArr[0],
+ decimalStr: decimalLength && priceStrArr ? `.${priceStrArr[1]}` : '',
+ });
+ },
+ updateTip() {
+ this.setData({ hasTip: typeof this.data.tip === 'string' });
+ },
+ onSubmit(event) {
+ this.$emit('submit', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.json
new file mode 100644
index 0000000..bda9b8d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxml
new file mode 100644
index 0000000..a56dd46
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxml
@@ -0,0 +1,44 @@
+
+
+
+
+
+
+
+
+ {{ tip }}
+
+
+
+
+
+
+
+ {{ label || '合计:' }}
+
+ {{ currency }}
+ {{ integerStr }}{{decimalStr}}
+
+ {{ suffixLabel }}
+
+
+ {{ loading ? '' : buttonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxss
new file mode 100644
index 0000000..8379a30
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/submit-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-submit-bar{background-color:var(--submit-bar-background-color,#fff);bottom:0;left:0;position:fixed;-webkit-user-select:none;user-select:none;width:100%;z-index:var(--submit-bar-z-index,100)}.van-submit-bar__tip{background-color:var(--submit-bar-tip-background-color,#fff7cc);color:var(--submit-bar-tip-color,#f56723);font-size:var(--submit-bar-tip-font-size,12px);line-height:var(--submit-bar-tip-line-height,1.5);padding:var(--submit-bar-tip-padding,10px)}.van-submit-bar__tip:empty{display:none}.van-submit-bar__tip-icon{margin-right:4px;vertical-align:middle}.van-submit-bar__tip-text{display:inline;vertical-align:middle}.van-submit-bar__bar{align-items:center;background-color:var(--submit-bar-background-color,#fff);display:flex;font-size:var(--submit-bar-text-font-size,14px);height:var(--submit-bar-height,50px);justify-content:flex-end;padding:var(--submit-bar-padding,0 16px)}.van-submit-bar__safe{height:constant(safe-area-inset-bottom);height:env(safe-area-inset-bottom)}.van-submit-bar__text{color:var(--submit-bar-text-color,#323233);flex:1;font-weight:var(--font-weight-bold,500);padding-right:var(--padding-sm,12px);text-align:right}.van-submit-bar__price{color:var(--submit-bar-price-color,#ee0a24);font-size:var(--submit-bar-price-font-size,12px);font-weight:var(--font-weight-bold,500)}.van-submit-bar__price-integer{font-family:Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif;font-size:20px}.van-submit-bar__currency{font-size:var(--submit-bar-currency-font-size,12px)}.van-submit-bar__suffix-label{margin-left:5px}.van-submit-bar__button{--button-default-height:var(--submit-bar-button-height,40px)!important;--button-line-height:var(--submit-bar-button-height,40px)!important;font-weight:var(--font-weight-bold,500);width:var(--submit-bar-button-width,110px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.js
new file mode 100644
index 0000000..501088f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.js
@@ -0,0 +1,133 @@
+import { VantComponent } from '../common/component';
+import { touch } from '../mixins/touch';
+import { range } from '../common/utils';
+const THRESHOLD = 0.3;
+let ARRAY = [];
+VantComponent({
+ props: {
+ disabled: Boolean,
+ leftWidth: {
+ type: Number,
+ value: 0,
+ observer(leftWidth = 0) {
+ if (this.offset > 0) {
+ this.swipeMove(leftWidth);
+ }
+ },
+ },
+ rightWidth: {
+ type: Number,
+ value: 0,
+ observer(rightWidth = 0) {
+ if (this.offset < 0) {
+ this.swipeMove(-rightWidth);
+ }
+ },
+ },
+ asyncClose: Boolean,
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ mixins: [touch],
+ data: {
+ catchMove: false,
+ wrapperStyle: '',
+ },
+ created() {
+ this.offset = 0;
+ ARRAY.push(this);
+ },
+ destroyed() {
+ ARRAY = ARRAY.filter((item) => item !== this);
+ },
+ methods: {
+ open(position) {
+ const { leftWidth, rightWidth } = this.data;
+ const offset = position === 'left' ? leftWidth : -rightWidth;
+ this.swipeMove(offset);
+ this.$emit('open', {
+ position,
+ name: this.data.name,
+ });
+ },
+ close() {
+ this.swipeMove(0);
+ },
+ swipeMove(offset = 0) {
+ this.offset = range(offset, -this.data.rightWidth, this.data.leftWidth);
+ const transform = `translate3d(${this.offset}px, 0, 0)`;
+ const transition = this.dragging
+ ? 'none'
+ : 'transform .6s cubic-bezier(0.18, 0.89, 0.32, 1)';
+ this.setData({
+ wrapperStyle: `
+ -webkit-transform: ${transform};
+ -webkit-transition: ${transition};
+ transform: ${transform};
+ transition: ${transition};
+ `,
+ });
+ },
+ swipeLeaveTransition() {
+ const { leftWidth, rightWidth } = this.data;
+ const { offset } = this;
+ if (rightWidth > 0 && -offset > rightWidth * THRESHOLD) {
+ this.open('right');
+ }
+ else if (leftWidth > 0 && offset > leftWidth * THRESHOLD) {
+ this.open('left');
+ }
+ else {
+ this.swipeMove(0);
+ }
+ this.setData({ catchMove: false });
+ },
+ startDrag(event) {
+ if (this.data.disabled) {
+ return;
+ }
+ this.startOffset = this.offset;
+ this.touchStart(event);
+ },
+ noop() { },
+ onDrag(event) {
+ if (this.data.disabled) {
+ return;
+ }
+ this.touchMove(event);
+ if (this.direction !== 'horizontal') {
+ return;
+ }
+ this.dragging = true;
+ ARRAY.filter((item) => item !== this && item.offset !== 0).forEach((item) => item.close());
+ this.setData({ catchMove: true });
+ this.swipeMove(this.startOffset + this.deltaX);
+ },
+ endDrag() {
+ if (this.data.disabled) {
+ return;
+ }
+ this.dragging = false;
+ this.swipeLeaveTransition();
+ },
+ onClick(event) {
+ const { key: position = 'outside' } = event.currentTarget.dataset;
+ this.$emit('click', position);
+ if (!this.offset) {
+ return;
+ }
+ if (this.data.asyncClose) {
+ this.$emit('close', {
+ position,
+ instance: this,
+ name: this.data.name,
+ });
+ }
+ else {
+ this.swipeMove(0);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxml
new file mode 100644
index 0000000..3f7f726
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxss
new file mode 100644
index 0000000..3a265bf
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/swipe-cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-swipe-cell{overflow:hidden;position:relative}.van-swipe-cell__left,.van-swipe-cell__right{height:100%;position:absolute;top:0}.van-swipe-cell__left{left:0;transform:translate3d(-100%,0,0)}.van-swipe-cell__right{right:0;transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.js
new file mode 100644
index 0000000..4cad09c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.js
@@ -0,0 +1,36 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ field: true,
+ classes: ['node-class'],
+ props: {
+ checked: null,
+ loading: Boolean,
+ disabled: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ size: {
+ type: String,
+ value: '30',
+ },
+ activeValue: {
+ type: null,
+ value: true,
+ },
+ inactiveValue: {
+ type: null,
+ value: false,
+ },
+ },
+ methods: {
+ onClick() {
+ const { activeValue, inactiveValue, disabled, loading } = this.data;
+ if (disabled || loading) {
+ return;
+ }
+ const checked = this.data.checked === activeValue;
+ const value = checked ? inactiveValue : activeValue;
+ this.$emit('input', value);
+ this.$emit('change', value);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.json
new file mode 100644
index 0000000..01077f5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxml
new file mode 100644
index 0000000..4e9789b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxml
@@ -0,0 +1,16 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxs
new file mode 100644
index 0000000..3ae387a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxs
@@ -0,0 +1,26 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ var currentColor = data.checked === data.activeValue ? data.activeColor : data.inactiveColor;
+
+ return style({
+ 'font-size': addUnit(data.size),
+ 'background-color': currentColor,
+ });
+}
+
+var BLUE = '#1989fa';
+var GRAY_DARK = '#969799';
+
+function loadingColor(data) {
+ return data.checked === data.activeValue
+ ? data.activeColor || BLUE
+ : data.inactiveColor || GRAY_DARK;
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxss
new file mode 100644
index 0000000..35929de
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/switch/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-switch{background-color:var(--switch-background-color,#fff);border:var(--switch-border,1px solid rgba(0,0,0,.1));border-radius:var(--switch-node-size,1em);box-sizing:initial;display:inline-block;height:var(--switch-height,1em);position:relative;transition:background-color var(--switch-transition-duration,.3s);width:var(--switch-width,2em)}.van-switch__node{background-color:var(--switch-node-background-color,#fff);border-radius:100%;box-shadow:var(--switch-node-box-shadow,0 3px 1px 0 rgba(0,0,0,.05),0 2px 2px 0 rgba(0,0,0,.1),0 3px 3px 0 rgba(0,0,0,.05));height:var(--switch-node-size,1em);left:0;position:absolute;top:0;transition:var(--switch-transition-duration,.3s) cubic-bezier(.3,1.05,.4,1.05);width:var(--switch-node-size,1em);z-index:var(--switch-node-z-index,1)}.van-switch__loading{height:50%;left:25%;position:absolute!important;top:25%;width:50%}.van-switch--on{background-color:var(--switch-on-background-color,#1989fa)}.van-switch--on .van-switch__node{transform:translateX(calc(var(--switch-width, 2em) - var(--switch-node-size, 1em)))}.van-switch--disabled{opacity:var(--switch-disabled-opacity,.4)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.js
new file mode 100644
index 0000000..9ada62e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.js
@@ -0,0 +1,56 @@
+import { useParent } from '../common/relation';
+import { VantComponent } from '../common/component';
+VantComponent({
+ relation: useParent('tabs'),
+ props: {
+ dot: {
+ type: Boolean,
+ observer: 'update',
+ },
+ info: {
+ type: null,
+ observer: 'update',
+ },
+ title: {
+ type: String,
+ observer: 'update',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'update',
+ },
+ titleStyle: {
+ type: String,
+ observer: 'update',
+ },
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ data: {
+ active: false,
+ },
+ methods: {
+ getComputedName() {
+ if (this.data.name !== '') {
+ return this.data.name;
+ }
+ return this.index;
+ },
+ updateRender(active, parent) {
+ const { data: parentData } = parent;
+ this.inited = this.inited || active;
+ this.setData({
+ active,
+ shouldRender: this.inited || !parentData.lazyRender,
+ shouldShow: active || parentData.animated,
+ });
+ },
+ update() {
+ if (this.parent) {
+ this.parent.updateTabs();
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxml
new file mode 100644
index 0000000..f5e99f2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxss
new file mode 100644
index 0000000..1c90c88
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tab/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{box-sizing:border-box;flex-shrink:0;width:100%}.van-tab__pane{-webkit-overflow-scrolling:touch;box-sizing:border-box;overflow-y:auto}.van-tab__pane--active{height:auto}.van-tab__pane--inactive{height:0;overflow:visible}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.js
new file mode 100644
index 0000000..64275ea
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.js
@@ -0,0 +1,68 @@
+import { VantComponent } from '../common/component';
+import { useParent } from '../common/relation';
+VantComponent({
+ props: {
+ info: null,
+ name: null,
+ icon: String,
+ dot: Boolean,
+ url: {
+ type: String,
+ value: '',
+ },
+ linkType: {
+ type: String,
+ value: 'redirectTo',
+ },
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ },
+ relation: useParent('tabbar'),
+ data: {
+ active: false,
+ activeColor: '',
+ inactiveColor: '',
+ },
+ methods: {
+ onClick() {
+ const { parent } = this;
+ if (parent) {
+ const index = parent.children.indexOf(this);
+ const active = this.data.name || index;
+ if (active !== this.data.active) {
+ parent.$emit('change', active);
+ }
+ }
+ const { url, linkType } = this.data;
+ if (url && wx[linkType]) {
+ return wx[linkType]({ url });
+ }
+ this.$emit('click');
+ },
+ updateFromParent() {
+ const { parent } = this;
+ if (!parent) {
+ return;
+ }
+ const index = parent.children.indexOf(this);
+ const parentData = parent.data;
+ const { data } = this;
+ const active = (data.name || index) === parentData.active;
+ const patch = {};
+ if (active !== data.active) {
+ patch.active = active;
+ }
+ if (parentData.activeColor !== data.activeColor) {
+ patch.activeColor = parentData.activeColor;
+ }
+ if (parentData.inactiveColor !== data.inactiveColor) {
+ patch.inactiveColor = parentData.inactiveColor;
+ }
+ if (Object.keys(patch).length > 0) {
+ this.setData(patch);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.json
new file mode 100644
index 0000000..16f174c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxml
new file mode 100644
index 0000000..524728f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxml
@@ -0,0 +1,28 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxss
new file mode 100644
index 0000000..21ee224
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-tabbar-item{align-items:center;color:var(--tabbar-item-text-color,#646566);display:flex;flex-direction:column;font-size:var(--tabbar-item-font-size,12px);height:100%;justify-content:center;line-height:var(--tabbar-item-line-height,1)}.van-tabbar-item__icon{font-size:var(--tabbar-item-icon-size,22px);margin-bottom:var(--tabbar-item-margin-bottom,4px);position:relative}.van-tabbar-item__icon__inner{display:block;min-width:1em}.van-tabbar-item--active{color:var(--tabbar-item-active-color,#1989fa)}.van-tabbar-item__info{margin-top:2px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.js
new file mode 100644
index 0000000..05a39d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.js
@@ -0,0 +1,65 @@
+import { VantComponent } from '../common/component';
+import { useChildren } from '../common/relation';
+import { getRect } from '../common/utils';
+VantComponent({
+ relation: useChildren('tabbar-item', function () {
+ this.updateChildren();
+ }),
+ props: {
+ active: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ activeColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ inactiveColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ fixed: {
+ type: Boolean,
+ value: true,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 50,
+ },
+ methods: {
+ updateChildren() {
+ const { children } = this;
+ if (!Array.isArray(children) || !children.length) {
+ return;
+ }
+ children.forEach((child) => child.updateFromParent());
+ },
+ setHeight() {
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(() => {
+ getRect(this, '.van-tabbar').then((res) => {
+ this.setData({ height: res.height });
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxml
new file mode 100644
index 0000000..43bb111
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxss
new file mode 100644
index 0000000..42b6c1e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabbar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabbar{background-color:var(--tabbar-background-color,#fff);box-sizing:initial;display:flex;height:var(--tabbar-height,50px);width:100%}.van-tabbar--fixed{bottom:0;left:0;position:fixed}.van-tabbar--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.js
new file mode 100644
index 0000000..3be2db9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.js
@@ -0,0 +1,302 @@
+import { VantComponent } from '../common/component';
+import { touch } from '../mixins/touch';
+import { getAllRect, getRect, groupSetData, nextTick, requestAnimationFrame, } from '../common/utils';
+import { isDef } from '../common/validator';
+import { useChildren } from '../common/relation';
+VantComponent({
+ mixins: [touch],
+ classes: [
+ 'nav-class',
+ 'tab-class',
+ 'tab-active-class',
+ 'line-class',
+ 'wrap-class',
+ ],
+ relation: useChildren('tab', function () {
+ this.updateTabs();
+ }),
+ props: {
+ sticky: Boolean,
+ border: Boolean,
+ swipeable: Boolean,
+ titleActiveColor: String,
+ titleInactiveColor: String,
+ color: String,
+ animated: {
+ type: Boolean,
+ observer() {
+ this.children.forEach((child, index) => child.updateRender(index === this.data.currentIndex, this));
+ },
+ },
+ lineWidth: {
+ type: null,
+ value: 40,
+ observer: 'resize',
+ },
+ lineHeight: {
+ type: null,
+ value: -1,
+ },
+ active: {
+ type: null,
+ value: 0,
+ observer(name) {
+ if (name !== this.getCurrentName()) {
+ this.setCurrentIndexByName(name);
+ }
+ },
+ },
+ type: {
+ type: String,
+ value: 'line',
+ },
+ ellipsis: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: Number,
+ value: 0.3,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ swipeThreshold: {
+ type: Number,
+ value: 5,
+ observer(value) {
+ this.setData({
+ scrollable: this.children.length > value || !this.data.ellipsis,
+ });
+ },
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ },
+ lazyRender: {
+ type: Boolean,
+ value: true,
+ },
+ useBeforeChange: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ tabs: [],
+ scrollLeft: 0,
+ scrollable: false,
+ currentIndex: 0,
+ container: null,
+ skipTransition: true,
+ scrollWithAnimation: false,
+ lineOffsetLeft: 0,
+ inited: false,
+ },
+ mounted() {
+ requestAnimationFrame(() => {
+ this.swiping = true;
+ this.setData({
+ container: () => this.createSelectorQuery().select('.van-tabs'),
+ });
+ this.resize();
+ this.scrollIntoView();
+ });
+ },
+ methods: {
+ updateTabs() {
+ const { children = [], data } = this;
+ this.setData({
+ tabs: children.map((child) => child.data),
+ scrollable: this.children.length > data.swipeThreshold || !data.ellipsis,
+ });
+ this.setCurrentIndexByName(data.active || this.getCurrentName());
+ },
+ trigger(eventName, child) {
+ const { currentIndex } = this.data;
+ const data = this.getChildData(currentIndex, child);
+ if (!isDef(data)) {
+ return;
+ }
+ this.$emit(eventName, data);
+ },
+ onTap(event) {
+ const { index } = event.currentTarget.dataset;
+ const child = this.children[index];
+ if (child.data.disabled) {
+ this.trigger('disabled', child);
+ return;
+ }
+ this.onBeforeChange(index).then(() => {
+ this.setCurrentIndex(index);
+ nextTick(() => {
+ this.trigger('click');
+ });
+ });
+ },
+ // correct the index of active tab
+ setCurrentIndexByName(name) {
+ const { children = [] } = this;
+ const matched = children.filter((child) => child.getComputedName() === name);
+ if (matched.length) {
+ this.setCurrentIndex(matched[0].index);
+ }
+ },
+ setCurrentIndex(currentIndex) {
+ const { data, children = [] } = this;
+ if (!isDef(currentIndex) ||
+ currentIndex >= children.length ||
+ currentIndex < 0) {
+ return;
+ }
+ groupSetData(this, () => {
+ children.forEach((item, index) => {
+ const active = index === currentIndex;
+ if (active !== item.data.active || !item.inited) {
+ item.updateRender(active, this);
+ }
+ });
+ });
+ if (currentIndex === data.currentIndex) {
+ if (!data.inited) {
+ this.resize();
+ }
+ return;
+ }
+ const shouldEmitChange = data.currentIndex !== null;
+ this.setData({ currentIndex });
+ requestAnimationFrame(() => {
+ this.resize();
+ this.scrollIntoView();
+ });
+ nextTick(() => {
+ this.trigger('input');
+ if (shouldEmitChange) {
+ this.trigger('change');
+ }
+ });
+ },
+ getCurrentName() {
+ const activeTab = this.children[this.data.currentIndex];
+ if (activeTab) {
+ return activeTab.getComputedName();
+ }
+ },
+ resize() {
+ if (this.data.type !== 'line') {
+ return;
+ }
+ const { currentIndex, ellipsis, skipTransition } = this.data;
+ Promise.all([
+ getAllRect(this, '.van-tab'),
+ getRect(this, '.van-tabs__line'),
+ ]).then(([rects = [], lineRect]) => {
+ const rect = rects[currentIndex];
+ if (rect == null) {
+ return;
+ }
+ let lineOffsetLeft = rects
+ .slice(0, currentIndex)
+ .reduce((prev, curr) => prev + curr.width, 0);
+ lineOffsetLeft +=
+ (rect.width - lineRect.width) / 2 + (ellipsis ? 0 : 8);
+ this.setData({ lineOffsetLeft, inited: true });
+ this.swiping = true;
+ if (skipTransition) {
+ // waiting transition end
+ setTimeout(() => {
+ this.setData({ skipTransition: false });
+ }, this.data.duration);
+ }
+ });
+ },
+ // scroll active tab into view
+ scrollIntoView() {
+ const { currentIndex, scrollable, scrollWithAnimation } = this.data;
+ if (!scrollable) {
+ return;
+ }
+ Promise.all([
+ getAllRect(this, '.van-tab'),
+ getRect(this, '.van-tabs__nav'),
+ ]).then(([tabRects, navRect]) => {
+ const tabRect = tabRects[currentIndex];
+ const offsetLeft = tabRects
+ .slice(0, currentIndex)
+ .reduce((prev, curr) => prev + curr.width, 0);
+ this.setData({
+ scrollLeft: offsetLeft - (navRect.width - tabRect.width) / 2,
+ });
+ if (!scrollWithAnimation) {
+ nextTick(() => {
+ this.setData({ scrollWithAnimation: true });
+ });
+ }
+ });
+ },
+ onTouchScroll(event) {
+ this.$emit('scroll', event.detail);
+ },
+ onTouchStart(event) {
+ if (!this.data.swipeable)
+ return;
+ this.swiping = true;
+ this.touchStart(event);
+ },
+ onTouchMove(event) {
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ this.touchMove(event);
+ },
+ // watch swipe touch end
+ onTouchEnd() {
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ const { direction, deltaX, offsetX } = this;
+ const minSwipeDistance = 50;
+ if (direction === 'horizontal' && offsetX >= minSwipeDistance) {
+ const index = this.getAvaiableTab(deltaX);
+ if (index !== -1) {
+ this.onBeforeChange(index).then(() => this.setCurrentIndex(index));
+ }
+ }
+ this.swiping = false;
+ },
+ getAvaiableTab(direction) {
+ const { tabs, currentIndex } = this.data;
+ const step = direction > 0 ? -1 : 1;
+ for (let i = step; currentIndex + i < tabs.length && currentIndex + i >= 0; i += step) {
+ const index = currentIndex + i;
+ if (index >= 0 &&
+ index < tabs.length &&
+ tabs[index] &&
+ !tabs[index].disabled) {
+ return index;
+ }
+ }
+ return -1;
+ },
+ onBeforeChange(index) {
+ const { useBeforeChange } = this.data;
+ if (!useBeforeChange) {
+ return Promise.resolve();
+ }
+ return new Promise((resolve, reject) => {
+ this.$emit('before-change', Object.assign(Object.assign({}, this.getChildData(index)), { callback: (status) => (status ? resolve() : reject()) }));
+ });
+ },
+ getChildData(index, child) {
+ const currentChild = child || this.children[index];
+ if (!isDef(currentChild)) {
+ return;
+ }
+ return {
+ index: currentChild.index,
+ name: currentChild.getComputedName(),
+ title: currentChild.data.title,
+ };
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.json
new file mode 100644
index 0000000..19c0bc3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index",
+ "van-sticky": "../sticky/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxml
new file mode 100644
index 0000000..05bb1e1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxml
@@ -0,0 +1,63 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.title }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxs
new file mode 100644
index 0000000..4059c7f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxs
@@ -0,0 +1,83 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function tabClass(active, ellipsis) {
+ var classes = ['tab-class'];
+
+ if (active) {
+ classes.push('tab-active-class');
+ }
+
+ if (ellipsis) {
+ classes.push('van-ellipsis');
+ }
+
+ return classes.join(' ');
+}
+
+function tabStyle(data) {
+ var titleColor = data.active
+ ? data.titleActiveColor
+ : data.titleInactiveColor;
+
+ var ellipsis = data.scrollable && data.ellipsis;
+
+ // card theme color
+ if (data.type === 'card') {
+ return style({
+ 'border-color': data.color,
+ 'background-color': !data.disabled && data.active ? data.color : null,
+ color: titleColor || (!data.disabled && !data.active ? data.color : null),
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+ }
+
+ return style({
+ color: titleColor,
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+}
+
+function navStyle(color, type) {
+ return style({
+ 'border-color': type === 'card' && color ? color : null,
+ });
+}
+
+function trackStyle(data) {
+ if (!data.animated) {
+ return '';
+ }
+
+ return style({
+ left: -100 * data.currentIndex + '%',
+ 'transition-duration': data.duration + 's',
+ '-webkit-transition-duration': data.duration + 's',
+ });
+}
+
+function lineStyle(data) {
+ return style({
+ width: utils.addUnit(data.lineWidth),
+ opacity: data.inited ? 1 : 0,
+ transform: 'translateX(' + data.lineOffsetLeft + 'px)',
+ '-webkit-transform': 'translateX(' + data.lineOffsetLeft + 'px)',
+ 'background-color': data.color,
+ height: data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'border-radius':
+ data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'transition-duration': !data.skipTransition ? data.duration + 's' : null,
+ '-webkit-transition-duration': !data.skipTransition
+ ? data.duration + 's'
+ : null,
+ });
+}
+
+module.exports = {
+ tabClass: tabClass,
+ tabStyle: tabStyle,
+ trackStyle: trackStyle,
+ lineStyle: lineStyle,
+ navStyle: navStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxss
new file mode 100644
index 0000000..09a93af
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tabs/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabs{-webkit-tap-highlight-color:transparent;position:relative}.van-tabs__wrap{display:flex;overflow:hidden}.van-tabs__wrap--scrollable .van-tab{flex:0 0 22%}.van-tabs__wrap--scrollable .van-tab--complete{flex:1 0 auto!important;padding:0 12px}.van-tabs__wrap--scrollable .van-tabs__nav--complete{padding-left:8px;padding-right:8px}.van-tabs__scroll{background-color:var(--tabs-nav-background-color,#fff);overflow:auto}.van-tabs__scroll--line{box-sizing:initial;height:calc(100% + 15px)}.van-tabs__scroll--card{border:1px solid var(--tabs-default-color,#ee0a24);border-radius:2px;box-sizing:border-box;margin:0 var(--padding-md,16px);width:calc(100% - var(--padding-md, 16px)*2)}.van-tabs__scroll::-webkit-scrollbar{display:none}.van-tabs__nav{display:flex;position:relative;-webkit-user-select:none;user-select:none}.van-tabs__nav--card{box-sizing:border-box;height:var(--tabs-card-height,30px)}.van-tabs__nav--card .van-tab{border-right:1px solid var(--tabs-default-color,#ee0a24);color:var(--tabs-default-color,#ee0a24);line-height:calc(var(--tabs-card-height, 30px) - 2px)}.van-tabs__nav--card .van-tab:last-child{border-right:none}.van-tabs__nav--card .van-tab.van-tab--active{background-color:var(--tabs-default-color,#ee0a24);color:#fff}.van-tabs__nav--card .van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tabs__line{background-color:var(--tabs-bottom-bar-color,#ee0a24);border-radius:var(--tabs-bottom-bar-height,3px);bottom:0;height:var(--tabs-bottom-bar-height,3px);left:0;opacity:0;position:absolute;z-index:1}.van-tabs__track{height:100%;position:relative;width:100%}.van-tabs__track--animated{display:flex;transition-property:left}.van-tabs__content{overflow:hidden}.van-tabs--line{height:var(--tabs-line-height,44px)}.van-tabs--card{height:var(--tabs-card-height,30px)}.van-tab{box-sizing:border-box;color:var(--tab-text-color,#646566);cursor:pointer;flex:1;font-size:var(--tab-font-size,14px);line-height:var(--tabs-line-height,44px);min-width:0;padding:0 5px;position:relative;text-align:center}.van-tab--active{color:var(--tab-active-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tab__title__info{position:relative!important;top:-1px!important;transform:translateX(0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.js
new file mode 100644
index 0000000..9704ef0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.js
@@ -0,0 +1,21 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ size: String,
+ mark: Boolean,
+ color: String,
+ plain: Boolean,
+ round: Boolean,
+ textColor: String,
+ type: {
+ type: String,
+ value: 'default',
+ },
+ closeable: Boolean,
+ },
+ methods: {
+ onClose() {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxml
new file mode 100644
index 0000000..59352dd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxml
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxs
new file mode 100644
index 0000000..12d1668
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'background-color': data.plain ? '' : data.color,
+ color: data.textColor || data.plain ? data.textColor || data.color : '',
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxss
new file mode 100644
index 0000000..0f0cbae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tag/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tag{align-items:center;border-radius:var(--tag-border-radius,2px);color:var(--tag-text-color,#fff);display:inline-flex;font-size:var(--tag-font-size,12px);line-height:var(--tag-line-height,16px);padding:var(--tag-padding,0 4px);position:relative}.van-tag--default{background-color:var(--tag-default-color,#969799)}.van-tag--default.van-tag--plain{color:var(--tag-default-color,#969799)}.van-tag--danger{background-color:var(--tag-danger-color,#ee0a24)}.van-tag--danger.van-tag--plain{color:var(--tag-danger-color,#ee0a24)}.van-tag--primary{background-color:var(--tag-primary-color,#1989fa)}.van-tag--primary.van-tag--plain{color:var(--tag-primary-color,#1989fa)}.van-tag--success{background-color:var(--tag-success-color,#07c160)}.van-tag--success.van-tag--plain{color:var(--tag-success-color,#07c160)}.van-tag--warning{background-color:var(--tag-warning-color,#ff976a)}.van-tag--warning.van-tag--plain{color:var(--tag-warning-color,#ff976a)}.van-tag--plain{background-color:var(--tag-plain-background-color,#fff)}.van-tag--plain:before{border:1px solid;border-radius:inherit;bottom:0;content:"";left:0;pointer-events:none;position:absolute;right:0;top:0}.van-tag--medium{padding:var(--tag-medium-padding,2px 6px)}.van-tag--large{border-radius:var(--tag-large-border-radius,4px);font-size:var(--tag-large-font-size,14px);padding:var(--tag-large-padding,4px 8px)}.van-tag--mark{border-radius:0 var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) 0}.van-tag--mark:after{content:"";display:block;width:2px}.van-tag--round{border-radius:var(--tag-round-border-radius,999px)}.van-tag__close{margin-left:2px;min-width:1em}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.js
new file mode 100644
index 0000000..414e746
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.js
@@ -0,0 +1,29 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ props: {
+ show: Boolean,
+ mask: Boolean,
+ message: String,
+ forbidClick: Boolean,
+ zIndex: {
+ type: Number,
+ value: 1000,
+ },
+ type: {
+ type: String,
+ value: 'text',
+ },
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ position: {
+ type: String,
+ value: 'middle',
+ },
+ },
+ methods: {
+ // for prevent touchmove
+ noop() { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.json
new file mode 100644
index 0000000..9b1b78c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index",
+ "van-overlay": "../overlay/index",
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxml
new file mode 100644
index 0000000..69f143e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxml
@@ -0,0 +1,36 @@
+
+
+
+
+ {{ message }}
+
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxss
new file mode 100644
index 0000000..3b7a34e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-toast{word-wrap:break-word;align-items:center;background-color:var(--toast-background-color,rgba(0,0,0,.7));border-radius:var(--toast-border-radius,8px);box-sizing:initial;color:var(--toast-text-color,#fff);display:flex;flex-direction:column;font-size:var(--toast-font-size,14px);justify-content:center;line-height:var(--toast-line-height,20px);white-space:pre-wrap}.van-toast__container{left:50%;max-width:var(--toast-max-width,70%);position:fixed;top:50%;transform:translate(-50%,-50%);width:-webkit-fit-content;width:fit-content}.van-toast--text{min-width:var(--toast-text-min-width,96px);padding:var(--toast-text-padding,8px 12px)}.van-toast--icon{min-height:var(--toast-default-min-height,88px);padding:var(--toast-default-padding,16px);width:var(--toast-default-width,88px)}.van-toast--icon .van-toast__icon{font-size:var(--toast-icon-size,36px)}.van-toast--icon .van-toast__text{padding-top:8px}.van-toast__loading{margin:10px 0}.van-toast--top{transform:translateY(-30vh)}.van-toast--bottom{transform:translateY(30vh)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.d.ts
new file mode 100644
index 0000000..de2a4a2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.d.ts
@@ -0,0 +1,28 @@
+///
+///
+type ToastMessage = string | number;
+type ToastContext = WechatMiniprogram.Component.TrivialInstance | WechatMiniprogram.Page.TrivialInstance;
+interface ToastOptions {
+ show?: boolean;
+ type?: string;
+ mask?: boolean;
+ zIndex?: number;
+ context?: (() => ToastContext) | ToastContext;
+ position?: string;
+ duration?: number;
+ selector?: string;
+ forbidClick?: boolean;
+ loadingType?: string;
+ message?: ToastMessage;
+ onClose?: () => void;
+}
+declare function Toast(toastOptions: ToastOptions | ToastMessage): WechatMiniprogram.Component.TrivialInstance | undefined;
+declare namespace Toast {
+ var loading: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var success: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var fail: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var clear: () => void;
+ var setDefaultOptions: (options: ToastOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Toast;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.js
new file mode 100644
index 0000000..51d1426
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/toast/toast.js
@@ -0,0 +1,68 @@
+import { isObj } from '../common/validator';
+const defaultOptions = {
+ type: 'text',
+ mask: false,
+ message: '',
+ show: true,
+ zIndex: 1000,
+ duration: 2000,
+ position: 'middle',
+ forbidClick: false,
+ loadingType: 'circular',
+ selector: '#van-toast',
+};
+let queue = [];
+let currentOptions = Object.assign({}, defaultOptions);
+function parseOptions(message) {
+ return isObj(message) ? message : { message };
+}
+function getContext() {
+ const pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+function Toast(toastOptions) {
+ const options = Object.assign(Object.assign({}, currentOptions), parseOptions(toastOptions));
+ const context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ const toast = context.selectComponent(options.selector);
+ if (!toast) {
+ console.warn('未找到 van-toast 节点,请确认 selector 及 context 是否正确');
+ return;
+ }
+ delete options.context;
+ delete options.selector;
+ toast.clear = () => {
+ toast.setData({ show: false });
+ if (options.onClose) {
+ options.onClose();
+ }
+ };
+ queue.push(toast);
+ toast.setData(options);
+ clearTimeout(toast.timer);
+ if (options.duration != null && options.duration > 0) {
+ toast.timer = setTimeout(() => {
+ toast.clear();
+ queue = queue.filter((item) => item !== toast);
+ }, options.duration);
+ }
+ return toast;
+}
+const createMethod = (type) => (options) => Toast(Object.assign({ type }, parseOptions(options)));
+Toast.loading = createMethod('loading');
+Toast.success = createMethod('success');
+Toast.fail = createMethod('fail');
+Toast.clear = () => {
+ queue.forEach((toast) => {
+ toast.clear();
+ });
+ queue = [];
+};
+Toast.setDefaultOptions = (options) => {
+ Object.assign(currentOptions, options);
+};
+Toast.resetDefaultOptions = () => {
+ currentOptions = Object.assign({}, defaultOptions);
+};
+export default Toast;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.js
new file mode 100644
index 0000000..59bb984
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.js
@@ -0,0 +1,13 @@
+import { VantComponent } from '../common/component';
+import { transition } from '../mixins/transition';
+VantComponent({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ ],
+ mixins: [transition(true)],
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxml
new file mode 100644
index 0000000..2743785
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxs
new file mode 100644
index 0000000..e0babf6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxss
new file mode 100644
index 0000000..3a3d37f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/transition/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-transition{transition-timing-function:ease}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-fade-down-enter-active,.van-fade-down-leave-active,.van-fade-left-enter-active,.van-fade-left-leave-active,.van-fade-right-enter-active,.van-fade-right-leave-active,.van-fade-up-enter-active,.van-fade-up-leave-active{transition-property:opacity,transform}.van-fade-up-enter,.van-fade-up-leave-to{opacity:0;transform:translate3d(0,100%,0)}.van-fade-down-enter,.van-fade-down-leave-to{opacity:0;transform:translate3d(0,-100%,0)}.van-fade-left-enter,.van-fade-left-leave-to{opacity:0;transform:translate3d(-100%,0,0)}.van-fade-right-enter,.van-fade-right-leave-to{opacity:0;transform:translate3d(100%,0,0)}.van-slide-down-enter-active,.van-slide-down-leave-active,.van-slide-left-enter-active,.van-slide-left-leave-active,.van-slide-right-enter-active,.van-slide-right-leave-active,.van-slide-up-enter-active,.van-slide-up-leave-active{transition-property:transform}.van-slide-up-enter,.van-slide-up-leave-to{transform:translate3d(0,100%,0)}.van-slide-down-enter,.van-slide-down-leave-to{transform:translate3d(0,-100%,0)}.van-slide-left-enter,.van-slide-left-leave-to{transform:translate3d(-100%,0,0)}.van-slide-right-enter,.van-slide-right-leave-to{transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.js
new file mode 100644
index 0000000..a850ed6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.js
@@ -0,0 +1,68 @@
+import { VantComponent } from '../common/component';
+VantComponent({
+ classes: [
+ 'main-item-class',
+ 'content-item-class',
+ 'main-active-class',
+ 'content-active-class',
+ 'main-disabled-class',
+ 'content-disabled-class',
+ ],
+ props: {
+ items: {
+ type: Array,
+ observer: 'updateSubItems',
+ },
+ activeId: null,
+ mainActiveIndex: {
+ type: Number,
+ value: 0,
+ observer: 'updateSubItems',
+ },
+ height: {
+ type: null,
+ value: 300,
+ },
+ max: {
+ type: Number,
+ value: Infinity,
+ },
+ selectedIcon: {
+ type: String,
+ value: 'success',
+ },
+ },
+ data: {
+ subItems: [],
+ },
+ methods: {
+ // 当一个子项被选择时
+ onSelectItem(event) {
+ const { item } = event.currentTarget.dataset;
+ const isArray = Array.isArray(this.data.activeId);
+ // 判断有没有超出右侧选择的最大数
+ const isOverMax = isArray && this.data.activeId.length >= this.data.max;
+ // 判断该项有没有被选中, 如果有被选中,则忽视是否超出的条件
+ const isSelected = isArray
+ ? this.data.activeId.indexOf(item.id) > -1
+ : this.data.activeId === item.id;
+ if (!item.disabled && (!isOverMax || isSelected)) {
+ this.$emit('click-item', item);
+ }
+ },
+ // 当一个导航被点击时
+ onClickNav(event) {
+ const index = event.detail;
+ const item = this.data.items[index];
+ if (!item.disabled) {
+ this.$emit('click-nav', { index });
+ }
+ },
+ // 更新子项列表
+ updateSubItems() {
+ const { items, mainActiveIndex } = this.data;
+ const { children = [] } = items[mainActiveIndex] || {};
+ this.setData({ subItems: children });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.json
new file mode 100644
index 0000000..42991a2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-sidebar": "../sidebar/index",
+ "van-sidebar-item": "../sidebar-item/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxml
new file mode 100644
index 0000000..2663e52
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxs
new file mode 100644
index 0000000..b1cbb39
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var array = require('../wxs/array.wxs');
+
+function isActive (activeList, itemId) {
+ if (array.isArray(activeList)) {
+ return activeList.indexOf(itemId) > -1;
+ }
+
+ return activeList === itemId;
+}
+
+module.exports.isActive = isActive;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxss
new file mode 100644
index 0000000..5bef0ac
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/tree-select/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tree-select{display:flex;font-size:var(--tree-select-font-size,14px);position:relative;-webkit-user-select:none;user-select:none}.van-tree-select__nav{--sidebar-padding:12px 8px 12px 12px;background-color:var(--tree-select-nav-background-color,#f7f8fa);flex:1}.van-tree-select__nav__inner{height:100%;width:100%!important}.van-tree-select__content{background-color:var(--tree-select-content-background-color,#fff);flex:2}.van-tree-select__item{font-weight:700;line-height:var(--tree-select-item-height,44px);padding:0 32px 0 var(--padding-md,16px);position:relative}.van-tree-select__item--active{color:var(--tree-select-item-active-color,#ee0a24)}.van-tree-select__item--disabled{color:var(--tree-select-item-disabled-color,#c8c9cc)}.van-tree-select__selected{position:absolute;right:var(--padding-md,16px);top:50%;transform:translateY(-50%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.js
new file mode 100644
index 0000000..5379c1b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.js
@@ -0,0 +1,168 @@
+import { VantComponent } from '../common/component';
+import { isImageFile, chooseFile, isVideoFile } from './utils';
+import { imageProps, videoProps, mediaProps, messageFileProps } from './shared';
+import { isBoolean, isPromise } from '../common/validator';
+VantComponent({
+ props: Object.assign(Object.assign(Object.assign(Object.assign({ disabled: Boolean, multiple: Boolean, uploadText: String, useBeforeRead: Boolean, afterRead: null, beforeRead: null, previewSize: {
+ type: null,
+ value: 80,
+ }, name: {
+ type: null,
+ value: '',
+ }, accept: {
+ type: String,
+ value: 'image',
+ }, fileList: {
+ type: Array,
+ value: [],
+ observer: 'formatFileList',
+ }, maxSize: {
+ type: Number,
+ value: Number.MAX_VALUE,
+ }, maxCount: {
+ type: Number,
+ value: 100,
+ }, deletable: {
+ type: Boolean,
+ value: true,
+ }, showUpload: {
+ type: Boolean,
+ value: true,
+ }, previewImage: {
+ type: Boolean,
+ value: true,
+ }, previewFullImage: {
+ type: Boolean,
+ value: true,
+ }, videoFit: {
+ type: String,
+ value: 'contain',
+ }, imageFit: {
+ type: String,
+ value: 'scaleToFill',
+ }, uploadIcon: {
+ type: String,
+ value: 'photograph',
+ } }, imageProps), videoProps), mediaProps), messageFileProps),
+ data: {
+ lists: [],
+ isInCount: true,
+ },
+ methods: {
+ formatFileList() {
+ const { fileList = [], maxCount } = this.data;
+ const lists = fileList.map((item) => (Object.assign(Object.assign({}, item), { isImage: isImageFile(item), isVideo: isVideoFile(item), deletable: isBoolean(item.deletable) ? item.deletable : true })));
+ this.setData({ lists, isInCount: lists.length < maxCount });
+ },
+ getDetail(index) {
+ return {
+ name: this.data.name,
+ index: index == null ? this.data.fileList.length : index,
+ };
+ },
+ startUpload() {
+ const { maxCount, multiple, lists, disabled } = this.data;
+ if (disabled)
+ return;
+ chooseFile(Object.assign(Object.assign({}, this.data), { maxCount: maxCount - lists.length }))
+ .then((res) => {
+ this.onBeforeRead(multiple ? res : res[0]);
+ })
+ .catch((error) => {
+ this.$emit('error', error);
+ });
+ },
+ onBeforeRead(file) {
+ const { beforeRead, useBeforeRead } = this.data;
+ let res = true;
+ if (typeof beforeRead === 'function') {
+ res = beforeRead(file, this.getDetail());
+ }
+ if (useBeforeRead) {
+ res = new Promise((resolve, reject) => {
+ this.$emit('before-read', Object.assign(Object.assign({ file }, this.getDetail()), { callback: (ok) => {
+ ok ? resolve() : reject();
+ } }));
+ });
+ }
+ if (!res) {
+ return;
+ }
+ if (isPromise(res)) {
+ res.then((data) => this.onAfterRead(data || file));
+ }
+ else {
+ this.onAfterRead(file);
+ }
+ },
+ onAfterRead(file) {
+ const { maxSize, afterRead } = this.data;
+ const oversize = Array.isArray(file)
+ ? file.some((item) => item.size > maxSize)
+ : file.size > maxSize;
+ if (oversize) {
+ this.$emit('oversize', Object.assign({ file }, this.getDetail()));
+ return;
+ }
+ if (typeof afterRead === 'function') {
+ afterRead(file, this.getDetail());
+ }
+ this.$emit('after-read', Object.assign({ file }, this.getDetail()));
+ },
+ deleteItem(event) {
+ const { index } = event.currentTarget.dataset;
+ this.$emit('delete', Object.assign(Object.assign({}, this.getDetail(index)), { file: this.data.fileList[index] }));
+ },
+ onPreviewImage(event) {
+ if (!this.data.previewFullImage)
+ return;
+ const { index } = event.currentTarget.dataset;
+ const { lists, showmenu } = this.data;
+ const item = lists[index];
+ wx.previewImage({
+ urls: lists.filter((item) => isImageFile(item)).map((item) => item.url),
+ current: item.url,
+ showmenu,
+ fail() {
+ wx.showToast({ title: '预览图片失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewVideo(event) {
+ if (!this.data.previewFullImage)
+ return;
+ const { index } = event.currentTarget.dataset;
+ const { lists } = this.data;
+ const sources = [];
+ const current = lists.reduce((sum, cur, curIndex) => {
+ if (!isVideoFile(cur)) {
+ return sum;
+ }
+ sources.push(Object.assign(Object.assign({}, cur), { type: 'video' }));
+ if (curIndex < index) {
+ sum++;
+ }
+ return sum;
+ }, 0);
+ wx.previewMedia({
+ sources,
+ current,
+ fail() {
+ wx.showToast({ title: '预览视频失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewFile(event) {
+ const { index } = event.currentTarget.dataset;
+ wx.openDocument({
+ filePath: this.data.lists[index].url,
+ showMenu: true,
+ });
+ },
+ onClickPreview(event) {
+ const { index } = event.currentTarget.dataset;
+ const item = this.data.lists[index];
+ this.$emit('click-preview', Object.assign(Object.assign({}, item), this.getDetail(index)));
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.json b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxml
new file mode 100644
index 0000000..3e61fd9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxml
@@ -0,0 +1,84 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.name || item.url }}
+
+
+
+
+ {{ item.message }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ uploadText }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxs
new file mode 100644
index 0000000..c567ec2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function sizeStyle(data) {
+ return "Array" === data.previewSize.constructor ? style({
+ width: addUnit(data.previewSize[0]),
+ height: addUnit(data.previewSize[1]),
+ }) : style({
+ width: addUnit(data.previewSize),
+ height: addUnit(data.previewSize),
+ });
+}
+
+module.exports = {
+ sizeStyle: sizeStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxss
new file mode 100644
index 0000000..11f8696
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-uploader{display:inline-block;position:relative}.van-uploader__wrapper{display:flex;flex-wrap:wrap}.van-uploader__slot:empty{display:none}.van-uploader__slot:not(:empty)+.van-uploader__upload{display:none!important}.van-uploader__upload{align-items:center;background-color:var(--uploader-upload-background-color,#f7f8fa);box-sizing:border-box;display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;margin:0 8px 8px 0;position:relative;width:var(--uploader-size,80px)}.van-uploader__upload:active{background-color:var(--uploader-upload-active-color,#f2f3f5)}.van-uploader__upload-icon{color:var(--uploader-icon-color,#dcdee0);font-size:var(--uploader-icon-size,24px)}.van-uploader__upload-text{color:var(--uploader-text-color,#969799);font-size:var(--uploader-text-font-size,12px);margin-top:var(--padding-xs,8px)}.van-uploader__upload--disabled{opacity:var(--uploader-disabled-opacity,.5)}.van-uploader__preview{cursor:pointer;margin:0 8px 8px 0;position:relative}.van-uploader__preview-image{display:block;height:var(--uploader-size,80px);overflow:hidden;width:var(--uploader-size,80px)}.van-uploader__preview-delete,.van-uploader__preview-delete:after{height:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;width:var(--uploader-delete-icon-size,14px)}.van-uploader__preview-delete:after{background-color:var(--uploader-delete-background-color,rgba(0,0,0,.7));border-radius:0 0 0 12px;content:""}.van-uploader__preview-delete-icon{color:var(--uploader-delete-color,#fff);font-size:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;transform:scale(.7) translate(10%,-10%);z-index:1}.van-uploader__file{align-items:center;background-color:var(--uploader-file-background-color,#f7f8fa);display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;width:var(--uploader-size,80px)}.van-uploader__file-icon{color:var(--uploader-file-icon-color,#646566);font-size:var(--uploader-file-icon-size,20px)}.van-uploader__file-name{box-sizing:border-box;color:var(--uploader-file-name-text-color,#646566);font-size:var(--uploader-file-name-font-size,12px);margin-top:var(--uploader-file-name-margin-top,8px);padding:var(--uploader-file-name-padding,0 4px);text-align:center;width:100%}.van-uploader__mask{align-items:center;background-color:var(--uploader-mask-background-color,rgba(50,50,51,.88));bottom:0;color:#fff;display:flex;flex-direction:column;justify-content:center;left:0;position:absolute;right:0;top:0}.van-uploader__mask-icon{font-size:var(--uploader-mask-icon-size,22px)}.van-uploader__mask-message{font-size:var(--uploader-mask-message-font-size,12px);line-height:var(--uploader-mask-message-line-height,14px);margin-top:6px;padding:0 var(--padding-base,4px)}.van-uploader__loading{color:var(--uploader-loading-icon-color,#fff)!important;height:var(--uploader-loading-icon-size,22px);width:var(--uploader-loading-icon-size,22px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.d.ts
new file mode 100644
index 0000000..e0a0d7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.d.ts
@@ -0,0 +1,53 @@
+export declare const imageProps: {
+ sizeType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ showmenu: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+};
+export declare const videoProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ compressed: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const mediaProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ mediaType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const messageFileProps: {
+ extension: null;
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.js
new file mode 100644
index 0000000..c481c23
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/shared.js
@@ -0,0 +1,57 @@
+// props for image
+export const imageProps = {
+ sizeType: {
+ type: Array,
+ value: ['original', 'compressed'],
+ },
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ showmenu: {
+ type: Boolean,
+ value: true,
+ },
+};
+// props for video
+export const videoProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ compressed: {
+ type: Boolean,
+ value: true,
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for media
+export const mediaProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ mediaType: {
+ type: Array,
+ value: ['image', 'video', 'mix'],
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for file
+export const messageFileProps = {
+ extension: null,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.d.ts
new file mode 100644
index 0000000..1e76ee6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.d.ts
@@ -0,0 +1,24 @@
+export interface File {
+ url: string;
+ size?: number;
+ name?: string;
+ type: string;
+ duration?: number;
+ time?: number;
+ isImage?: boolean;
+ isVideo?: boolean;
+}
+export declare function isImageFile(item: File): boolean;
+export declare function isVideoFile(item: File): boolean;
+export declare function chooseFile({ accept, multiple, capture, compressed, maxDuration, sizeType, camera, maxCount, mediaType, extension, }: {
+ accept: any;
+ multiple: any;
+ capture: any;
+ compressed: any;
+ maxDuration: any;
+ sizeType: any;
+ camera: any;
+ maxCount: any;
+ mediaType: any;
+ extension: any;
+}): Promise;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.js
new file mode 100644
index 0000000..f2aa889
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/uploader/utils.js
@@ -0,0 +1,94 @@
+import { pickExclude, isPC, isWxWork } from '../common/utils';
+import { isImageUrl, isVideoUrl } from '../common/validator';
+export function isImageFile(item) {
+ if (item.isImage != null) {
+ return item.isImage;
+ }
+ if (item.type) {
+ return item.type === 'image';
+ }
+ if (item.url) {
+ return isImageUrl(item.url);
+ }
+ return false;
+}
+export function isVideoFile(item) {
+ if (item.isVideo != null) {
+ return item.isVideo;
+ }
+ if (item.type) {
+ return item.type === 'video';
+ }
+ if (item.url) {
+ return isVideoUrl(item.url);
+ }
+ return false;
+}
+function formatImage(res) {
+ return res.tempFiles.map((item) => (Object.assign(Object.assign({}, pickExclude(item, ['path'])), { type: 'image', url: item.tempFilePath || item.path, thumb: item.tempFilePath || item.path })));
+}
+function formatVideo(res) {
+ return [
+ Object.assign(Object.assign({}, pickExclude(res, ['tempFilePath', 'thumbTempFilePath', 'errMsg'])), { type: 'video', url: res.tempFilePath, thumb: res.thumbTempFilePath }),
+ ];
+}
+function formatMedia(res) {
+ return res.tempFiles.map((item) => (Object.assign(Object.assign({}, pickExclude(item, ['fileType', 'thumbTempFilePath', 'tempFilePath'])), { type: item.fileType, url: item.tempFilePath, thumb: item.fileType === 'video' ? item.thumbTempFilePath : item.tempFilePath })));
+}
+function formatFile(res) {
+ return res.tempFiles.map((item) => (Object.assign(Object.assign({}, pickExclude(item, ['path'])), { url: item.path })));
+}
+export function chooseFile({ accept, multiple, capture, compressed, maxDuration, sizeType, camera, maxCount, mediaType, extension, }) {
+ return new Promise((resolve, reject) => {
+ switch (accept) {
+ case 'image':
+ if (isPC || isWxWork) {
+ wx.chooseImage({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ sourceType: capture,
+ sizeType,
+ success: (res) => resolve(formatImage(res)),
+ fail: reject,
+ });
+ }
+ else {
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType: ['image'],
+ sourceType: capture,
+ maxDuration,
+ sizeType,
+ camera,
+ success: (res) => resolve(formatImage(res)),
+ fail: reject,
+ });
+ }
+ break;
+ case 'media':
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType,
+ sourceType: capture,
+ maxDuration,
+ sizeType,
+ camera,
+ success: (res) => resolve(formatMedia(res)),
+ fail: reject,
+ });
+ break;
+ case 'video':
+ wx.chooseVideo({
+ sourceType: capture,
+ compressed,
+ maxDuration,
+ camera,
+ success: (res) => resolve(formatVideo(res)),
+ fail: reject,
+ });
+ break;
+ default:
+ wx.chooseMessageFile(Object.assign(Object.assign({ count: multiple ? maxCount : 1, type: accept }, (extension ? { extension } : {})), { success: (res) => resolve(formatFile(res)), fail: reject }));
+ break;
+ }
+ });
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/add-unit.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/add-unit.wxs
new file mode 100644
index 0000000..4f33462
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/add-unit.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var REGEXP = getRegExp('^-?\d+(\.\d+)?$');
+
+function addUnit(value) {
+ if (value == null) {
+ return undefined;
+ }
+
+ return REGEXP.test('' + value) ? value + 'px' : value;
+}
+
+module.exports = addUnit;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/array.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/array.wxs
new file mode 100644
index 0000000..610089c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/array.wxs
@@ -0,0 +1,5 @@
+function isArray(array) {
+ return array && array.constructor === 'Array';
+}
+
+module.exports.isArray = isArray;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/bem.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/bem.wxs
new file mode 100644
index 0000000..1efa129
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/bem.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var array = require('./array.wxs');
+var object = require('./object.wxs');
+var PREFIX = 'van-';
+
+function join(name, mods) {
+ name = PREFIX + name;
+ mods = mods.map(function(mod) {
+ return name + '--' + mod;
+ });
+ mods.unshift(name);
+ return mods.join(' ');
+}
+
+function traversing(mods, conf) {
+ if (!conf) {
+ return;
+ }
+
+ if (typeof conf === 'string' || typeof conf === 'number') {
+ mods.push(conf);
+ } else if (array.isArray(conf)) {
+ conf.forEach(function(item) {
+ traversing(mods, item);
+ });
+ } else if (typeof conf === 'object') {
+ object.keys(conf).forEach(function(key) {
+ conf[key] && mods.push(key);
+ });
+ }
+}
+
+function bem(name, conf) {
+ var mods = [];
+ traversing(mods, conf);
+ return join(name, mods);
+}
+
+module.exports = bem;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/memoize.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/memoize.wxs
new file mode 100644
index 0000000..8f7f46d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/memoize.wxs
@@ -0,0 +1,55 @@
+/**
+ * Simple memoize
+ * wxs doesn't support fn.apply, so this memoize only support up to 2 args
+ */
+/* eslint-disable */
+
+function isPrimitive(value) {
+ var type = typeof value;
+ return (
+ type === 'boolean' ||
+ type === 'number' ||
+ type === 'string' ||
+ type === 'undefined' ||
+ value === null
+ );
+}
+
+// mock simple fn.call in wxs
+function call(fn, args) {
+ if (args.length === 2) {
+ return fn(args[0], args[1]);
+ }
+
+ if (args.length === 1) {
+ return fn(args[0]);
+ }
+
+ return fn();
+}
+
+function serializer(args) {
+ if (args.length === 1 && isPrimitive(args[0])) {
+ return args[0];
+ }
+ var obj = {};
+ for (var i = 0; i < args.length; i++) {
+ obj['key' + i] = args[i];
+ }
+ return JSON.stringify(obj);
+}
+
+function memoize(fn) {
+ var cache = {};
+
+ return function() {
+ var key = serializer(arguments);
+ if (cache[key] === undefined) {
+ cache[key] = call(fn, arguments);
+ }
+
+ return cache[key];
+ };
+}
+
+module.exports = memoize;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/object.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/object.wxs
new file mode 100644
index 0000000..e077107
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/object.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var REGEXP = getRegExp('{|}|"', 'g');
+
+function keys(obj) {
+ return JSON.stringify(obj)
+ .replace(REGEXP, '')
+ .split(',')
+ .map(function(item) {
+ return item.split(':')[0];
+ });
+}
+
+module.exports.keys = keys;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/style.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/style.wxs
new file mode 100644
index 0000000..d88ca7c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/style.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var object = require('./object.wxs');
+var array = require('./array.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+
+ return newWord;
+}
+
+function style(styles) {
+ if (array.isArray(styles)) {
+ return styles
+ .filter(function (item) {
+ return item != null && item !== '';
+ })
+ .map(function (item) {
+ return style(item);
+ })
+ .join(';');
+ }
+
+ if ('Object' === styles.constructor) {
+ return object
+ .keys(styles)
+ .filter(function (key) {
+ return styles[key] != null && styles[key] !== '';
+ })
+ .map(function (key) {
+ return [kebabCase(key), [styles[key]]].join(':');
+ })
+ .join(';');
+ }
+
+ return styles;
+}
+
+module.exports = style;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/utils.wxs b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/utils.wxs
new file mode 100644
index 0000000..f66d33a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/dist/wxs/utils.wxs
@@ -0,0 +1,10 @@
+/* eslint-disable */
+var bem = require('./bem.wxs');
+var memoize = require('./memoize.wxs');
+var addUnit = require('./add-unit.wxs');
+
+module.exports = {
+ bem: memoize(bem),
+ memoize: memoize,
+ addUnit: addUnit
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.js
new file mode 100644
index 0000000..8403b68
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.js
@@ -0,0 +1,78 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+(0, component_1.VantComponent)({
+ classes: ['list-class'],
+ mixins: [button_1.button],
+ props: {
+ show: Boolean,
+ title: String,
+ cancelText: String,
+ description: String,
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ actions: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickAction: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onSelect: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var _a = this.data, actions = _a.actions, closeOnClickAction = _a.closeOnClickAction, canIUseGetUserProfile = _a.canIUseGetUserProfile;
+ var item = actions[index];
+ if (item) {
+ this.$emit('select', item);
+ if (closeOnClickAction) {
+ this.onClose();
+ }
+ if (item.openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: item.getUserProfileDesc || ' ',
+ complete: function (userProfile) {
+ _this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ }
+ },
+ onCancel: function () {
+ this.$emit('cancel');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ this.onClose();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.json
new file mode 100644
index 0000000..19bf989
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-popup": "../popup/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxml
new file mode 100644
index 0000000..6311e33
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
+
+
+
+ {{ cancelText }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxss
new file mode 100644
index 0000000..eedd361
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/action-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-action-sheet{color:var(--action-sheet-item-text-color,#323233);max-height:var(--action-sheet-max-height,90%)!important}.van-action-sheet__cancel,.van-action-sheet__item{background-color:var(--action-sheet-item-background,#fff);font-size:var(--action-sheet-item-font-size,16px);line-height:var(--action-sheet-item-line-height,22px);padding:14px 16px;text-align:center}.van-action-sheet__cancel--hover,.van-action-sheet__item--hover{background-color:#f2f3f5}.van-action-sheet__cancel:after,.van-action-sheet__item:after{border-width:0}.van-action-sheet__cancel{color:var(--action-sheet-cancel-text-color,#646566)}.van-action-sheet__gap{background-color:var(--action-sheet-cancel-padding-color,#f7f8fa);display:block;height:var(--action-sheet-cancel-padding-top,8px)}.van-action-sheet__item--disabled{color:var(--action-sheet-item-disabled-text-color,#c8c9cc)}.van-action-sheet__item--disabled.van-action-sheet__item--hover{background-color:var(--action-sheet-item-background,#fff)}.van-action-sheet__subname{color:var(--action-sheet-subname-color,#969799);font-size:var(--action-sheet-subname-font-size,12px);line-height:var(--action-sheet-subname-line-height,20px);margin-top:var(--padding-xs,8px)}.van-action-sheet__header{font-size:var(--action-sheet-header-font-size,16px);font-weight:var(--font-weight-bold,500);line-height:var(--action-sheet-header-height,48px);text-align:center}.van-action-sheet__description{color:var(--action-sheet-description-color,#969799);font-size:var(--action-sheet-description-font-size,14px);line-height:var(--action-sheet-description-line-height,20px);padding:20px var(--padding-md,16px);text-align:center}.van-action-sheet__close{color:var(--action-sheet-close-icon-color,#c8c9cc);font-size:var(--action-sheet-close-icon-size,22px)!important;line-height:inherit!important;padding:var(--action-sheet-close-icon-padding,0 16px);position:absolute!important;right:0;top:0}.van-action-sheet__loading{display:flex!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.js
new file mode 100644
index 0000000..73de66d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.js
@@ -0,0 +1,235 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var shared_1 = require("../picker/shared");
+var utils_1 = require("../common/utils");
+var EMPTY_CODE = '000000';
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { showToolbar: {
+ type: Boolean,
+ value: true,
+ }, value: {
+ type: String,
+ observer: function (value) {
+ this.code = value;
+ this.setValues();
+ },
+ }, areaList: {
+ type: Object,
+ value: {},
+ observer: 'setValues',
+ }, columnsNum: {
+ type: null,
+ value: 3,
+ }, columnsPlaceholder: {
+ type: Array,
+ observer: function (val) {
+ this.setData({
+ typeToColumnsPlaceholder: {
+ province: val[0] || '',
+ city: val[1] || '',
+ county: val[2] || '',
+ },
+ });
+ },
+ } }),
+ data: {
+ columns: [{ values: [] }, { values: [] }, { values: [] }],
+ typeToColumnsPlaceholder: {},
+ },
+ mounted: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.setValues();
+ });
+ },
+ methods: {
+ getPicker: function () {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-area__picker');
+ }
+ return this.picker;
+ },
+ onCancel: function (event) {
+ this.emit('cancel', event.detail);
+ },
+ onConfirm: function (event) {
+ var index = event.detail.index;
+ var value = event.detail.value;
+ value = this.parseValues(value);
+ this.emit('confirm', { value: value, index: index });
+ },
+ emit: function (type, detail) {
+ detail.values = detail.value;
+ delete detail.value;
+ this.$emit(type, detail);
+ },
+ parseValues: function (values) {
+ var columnsPlaceholder = this.data.columnsPlaceholder;
+ return values.map(function (value, index) {
+ if (value &&
+ (!value.code || value.name === columnsPlaceholder[index])) {
+ return __assign(__assign({}, value), { code: '', name: '' });
+ }
+ return value;
+ });
+ },
+ onChange: function (event) {
+ var _this = this;
+ var _a;
+ var _b = event.detail, index = _b.index, picker = _b.picker, value = _b.value;
+ this.code = value[index].code;
+ (_a = this.setValues()) === null || _a === void 0 ? void 0 : _a.then(function () {
+ _this.$emit('change', {
+ picker: picker,
+ values: _this.parseValues(picker.getValues()),
+ index: index,
+ });
+ });
+ },
+ getConfig: function (type) {
+ var areaList = this.data.areaList;
+ return (areaList && areaList["".concat(type, "_list")]) || {};
+ },
+ getList: function (type, code) {
+ if (type !== 'province' && !code) {
+ return [];
+ }
+ var typeToColumnsPlaceholder = this.data.typeToColumnsPlaceholder;
+ var list = this.getConfig(type);
+ var result = Object.keys(list).map(function (code) { return ({
+ code: code,
+ name: list[code],
+ }); });
+ if (code != null) {
+ // oversea code
+ if (code[0] === '9' && type === 'city') {
+ code = '9';
+ }
+ result = result.filter(function (item) { return item.code.indexOf(code) === 0; });
+ }
+ if (typeToColumnsPlaceholder[type] && result.length) {
+ // set columns placeholder
+ var codeFill = type === 'province'
+ ? ''
+ : type === 'city'
+ ? EMPTY_CODE.slice(2, 4)
+ : EMPTY_CODE.slice(4, 6);
+ result.unshift({
+ code: "".concat(code).concat(codeFill),
+ name: typeToColumnsPlaceholder[type],
+ });
+ }
+ return result;
+ },
+ getIndex: function (type, code) {
+ var compareNum = type === 'province' ? 2 : type === 'city' ? 4 : 6;
+ var list = this.getList(type, code.slice(0, compareNum - 2));
+ // oversea code
+ if (code[0] === '9' && type === 'province') {
+ compareNum = 1;
+ }
+ code = code.slice(0, compareNum);
+ for (var i = 0; i < list.length; i++) {
+ if (list[i].code.slice(0, compareNum) === code) {
+ return i;
+ }
+ }
+ return 0;
+ },
+ setValues: function () {
+ var picker = this.getPicker();
+ if (!picker) {
+ return;
+ }
+ var code = this.code || this.getDefaultCode();
+ var provinceList = this.getList('province');
+ var cityList = this.getList('city', code.slice(0, 2));
+ var stack = [];
+ var indexes = [];
+ var columnsNum = this.data.columnsNum;
+ if (columnsNum >= 1) {
+ stack.push(picker.setColumnValues(0, provinceList, false));
+ indexes.push(this.getIndex('province', code));
+ }
+ if (columnsNum >= 2) {
+ stack.push(picker.setColumnValues(1, cityList, false));
+ indexes.push(this.getIndex('city', code));
+ if (cityList.length && code.slice(2, 4) === '00') {
+ code = cityList[0].code;
+ }
+ }
+ if (columnsNum === 3) {
+ stack.push(picker.setColumnValues(2, this.getList('county', code.slice(0, 4)), false));
+ indexes.push(this.getIndex('county', code));
+ }
+ return Promise.all(stack)
+ .catch(function () { })
+ .then(function () { return picker.setIndexes(indexes); })
+ .catch(function () { });
+ },
+ getDefaultCode: function () {
+ var columnsPlaceholder = this.data.columnsPlaceholder;
+ if (columnsPlaceholder.length) {
+ return EMPTY_CODE;
+ }
+ var countyCodes = Object.keys(this.getConfig('county'));
+ if (countyCodes[0]) {
+ return countyCodes[0];
+ }
+ var cityCodes = Object.keys(this.getConfig('city'));
+ if (cityCodes[0]) {
+ return cityCodes[0];
+ }
+ return '';
+ },
+ getValues: function () {
+ var picker = this.getPicker();
+ if (!picker) {
+ return [];
+ }
+ return this.parseValues(picker.getValues().filter(function (value) { return !!value; }));
+ },
+ getDetail: function () {
+ var values = this.getValues();
+ var area = {
+ code: '',
+ country: '',
+ province: '',
+ city: '',
+ county: '',
+ };
+ if (!values.length) {
+ return area;
+ }
+ var names = values.map(function (item) { return item.name; });
+ area.code = values[values.length - 1].code;
+ if (area.code[0] === '9') {
+ area.country = names[1] || '';
+ area.province = names[2] || '';
+ }
+ else {
+ area.province = names[0] || '';
+ area.city = names[1] || '';
+ area.county = names[2] || '';
+ }
+ return area;
+ },
+ reset: function (code) {
+ this.code = code || '';
+ return this.setValues();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxml
new file mode 100644
index 0000000..3a437b7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxml
@@ -0,0 +1,20 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxs
new file mode 100644
index 0000000..07723c1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxs
@@ -0,0 +1,8 @@
+/* eslint-disable */
+function displayColumns(columns, columnsNum) {
+ return columns.slice(0, +columnsNum);
+}
+
+module.exports = {
+ displayColumns: displayColumns,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/area/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.js
new file mode 100644
index 0000000..984135c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.js
@@ -0,0 +1,67 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var version_1 = require("../common/version");
+var mixins = [button_1.button];
+if ((0, version_1.canIUseFormFieldButton)()) {
+ mixins.push('wx://form-field-button');
+}
+(0, component_1.VantComponent)({
+ mixins: mixins,
+ classes: ['hover-class', 'loading-class'],
+ data: {
+ baseStyle: '',
+ },
+ props: {
+ formType: String,
+ icon: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ plain: Boolean,
+ block: Boolean,
+ round: Boolean,
+ square: Boolean,
+ loading: Boolean,
+ hairline: Boolean,
+ disabled: Boolean,
+ loadingText: String,
+ customStyle: String,
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ type: {
+ type: String,
+ value: 'default',
+ },
+ dataset: null,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loadingSize: {
+ type: String,
+ value: '20px',
+ },
+ color: String,
+ },
+ methods: {
+ onClick: function (event) {
+ var _this = this;
+ this.$emit('click', event);
+ var _a = this.data, canIUseGetUserProfile = _a.canIUseGetUserProfile, openType = _a.openType, getUserProfileDesc = _a.getUserProfileDesc, lang = _a.lang;
+ if (openType === 'getUserInfo' && canIUseGetUserProfile) {
+ wx.getUserProfile({
+ desc: getUserProfileDesc || ' ',
+ lang: lang || 'en',
+ complete: function (userProfile) {
+ _this.$emit('getuserinfo', userProfile);
+ },
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxml
new file mode 100644
index 0000000..e7f60f1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxs
new file mode 100644
index 0000000..8b649fe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ if (!data.color) {
+ return data.customStyle;
+ }
+
+ var properties = {
+ color: data.plain ? data.color : '#fff',
+ background: data.plain ? null : data.color,
+ };
+
+ // hide border when color is linear-gradient
+ if (data.color.indexOf('gradient') !== -1) {
+ properties.border = 0;
+ } else {
+ properties['border-color'] = data.color;
+ }
+
+ return style([properties, data.customStyle]);
+}
+
+function loadingColor(data) {
+ if (data.plain) {
+ return data.color ? data.color : '#c9c9c9';
+ }
+
+ if (data.type === 'default') {
+ return '#c9c9c9';
+ }
+
+ return '#fff';
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxss
new file mode 100644
index 0000000..bd8bb5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-button{-webkit-text-size-adjust:100%;align-items:center;-webkit-appearance:none;border-radius:var(--button-border-radius,2px);box-sizing:border-box;display:inline-flex;font-size:var(--button-default-font-size,16px);height:var(--button-default-height,44px);justify-content:center;line-height:var(--button-line-height,20px);padding:0;position:relative;text-align:center;transition:opacity .2s;vertical-align:middle}.van-button:before{background-color:#000;border:inherit;border-color:#000;border-radius:inherit;content:" ";height:100%;left:50%;opacity:0;position:absolute;top:50%;transform:translate(-50%,-50%);width:100%}.van-button:after{border-width:0}.van-button--active:before{opacity:.15}.van-button--unclickable:after{display:none}.van-button--default{background:var(--button-default-background-color,#fff);border:var(--button-border-width,1px) solid var(--button-default-border-color,#ebedf0);color:var(--button-default-color,#323233)}.van-button--primary{background:var(--button-primary-background-color,#07c160);border:var(--button-border-width,1px) solid var(--button-primary-border-color,#07c160);color:var(--button-primary-color,#fff)}.van-button--info{background:var(--button-info-background-color,#1989fa);border:var(--button-border-width,1px) solid var(--button-info-border-color,#1989fa);color:var(--button-info-color,#fff)}.van-button--danger{background:var(--button-danger-background-color,#ee0a24);border:var(--button-border-width,1px) solid var(--button-danger-border-color,#ee0a24);color:var(--button-danger-color,#fff)}.van-button--warning{background:var(--button-warning-background-color,#ff976a);border:var(--button-border-width,1px) solid var(--button-warning-border-color,#ff976a);color:var(--button-warning-color,#fff)}.van-button--plain{background:var(--button-plain-background-color,#fff)}.van-button--plain.van-button--primary{color:var(--button-primary-background-color,#07c160)}.van-button--plain.van-button--info{color:var(--button-info-background-color,#1989fa)}.van-button--plain.van-button--danger{color:var(--button-danger-background-color,#ee0a24)}.van-button--plain.van-button--warning{color:var(--button-warning-background-color,#ff976a)}.van-button--large{height:var(--button-large-height,50px);width:100%}.van-button--normal{font-size:var(--button-normal-font-size,14px);padding:0 15px}.van-button--small{font-size:var(--button-small-font-size,12px);height:var(--button-small-height,30px);min-width:var(--button-small-min-width,60px);padding:0 var(--padding-xs,8px)}.van-button--mini{display:inline-block;font-size:var(--button-mini-font-size,10px);height:var(--button-mini-height,22px);min-width:var(--button-mini-min-width,50px)}.van-button--mini+.van-button--mini{margin-left:5px}.van-button--block{display:flex;width:100%}.van-button--round{border-radius:var(--button-round-border-radius,999px)}.van-button--square{border-radius:0}.van-button--disabled{opacity:var(--button-disabled-opacity,.5)}.van-button__text{display:inline}.van-button__icon+.van-button__text:not(:empty),.van-button__loading-text{margin-left:4px}.van-button__icon{line-height:inherit!important;min-width:1em;vertical-align:top}.van-button--hairline{border-width:0;padding-top:1px}.van-button--hairline:after{border-color:inherit;border-radius:calc(var(--button-border-radius, 2px)*2);border-width:1px}.van-button--hairline.van-button--round:after{border-radius:var(--button-round-border-radius,999px)}.van-button--hairline.van-button--square:after{border-radius:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/calendar.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/calendar.wxml
new file mode 100644
index 0000000..2ddb048
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/calendar.wxml
@@ -0,0 +1,70 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.js
new file mode 100644
index 0000000..544b3a4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.js
@@ -0,0 +1,45 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../../../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ subtitle: String,
+ showTitle: Boolean,
+ showSubtitle: Boolean,
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'initWeekDay',
+ },
+ },
+ data: {
+ weekdays: [],
+ },
+ created: function () {
+ this.initWeekDay();
+ },
+ methods: {
+ initWeekDay: function () {
+ var defaultWeeks = ['日', '一', '二', '三', '四', '五', '六'];
+ var firstDayOfWeek = this.data.firstDayOfWeek || 0;
+ this.setData({
+ weekdays: __spreadArray(__spreadArray([], defaultWeeks.slice(firstDayOfWeek, 7), true), defaultWeeks.slice(0, firstDayOfWeek), true),
+ });
+ },
+ onClickSubtitle: function (event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxml
new file mode 100644
index 0000000..7e56c83
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxss
new file mode 100644
index 0000000..272537e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/header/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar__header{box-shadow:var(--calendar-header-box-shadow,0 2px 10px hsla(220,1%,50%,.16));flex-shrink:0}.van-calendar__header-subtitle,.van-calendar__header-title{font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__header-title+.van-calendar__header-title,.van-calendar__header-title:empty{display:none}.van-calendar__header-title:empty+.van-calendar__header-title{display:block!important}.van-calendar__weekdays{display:flex}.van-calendar__weekday{flex:1;font-size:var(--calendar-weekdays-font-size,12px);line-height:var(--calendar-weekdays-height,30px);text-align:center}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.d.ts
new file mode 100644
index 0000000..3ccf85a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.d.ts
@@ -0,0 +1,6 @@
+export interface Day {
+ date: Date;
+ type: string;
+ text: number;
+ bottomInfo?: string;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.js
new file mode 100644
index 0000000..4d137f5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.js
@@ -0,0 +1,158 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../../../common/component");
+var utils_1 = require("../../utils");
+(0, component_1.VantComponent)({
+ props: {
+ date: {
+ type: null,
+ observer: 'setDays',
+ },
+ type: {
+ type: String,
+ observer: 'setDays',
+ },
+ color: String,
+ minDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ maxDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ showMark: Boolean,
+ rowHeight: null,
+ formatter: {
+ type: null,
+ observer: 'setDays',
+ },
+ currentDate: {
+ type: null,
+ observer: 'setDays',
+ },
+ firstDayOfWeek: {
+ type: Number,
+ observer: 'setDays',
+ },
+ allowSameDay: Boolean,
+ showSubtitle: Boolean,
+ showMonthTitle: Boolean,
+ },
+ data: {
+ visible: true,
+ days: [],
+ },
+ methods: {
+ onClick: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var item = this.data.days[index];
+ if (item.type !== 'disabled') {
+ this.$emit('click', item);
+ }
+ },
+ setDays: function () {
+ var days = [];
+ var startDate = new Date(this.data.date);
+ var year = startDate.getFullYear();
+ var month = startDate.getMonth();
+ var totalDay = (0, utils_1.getMonthEndDay)(startDate.getFullYear(), startDate.getMonth() + 1);
+ for (var day = 1; day <= totalDay; day++) {
+ var date = new Date(year, month, day);
+ var type = this.getDayType(date);
+ var config = {
+ date: date,
+ type: type,
+ text: day,
+ bottomInfo: this.getBottomInfo(type),
+ };
+ if (this.data.formatter) {
+ config = this.data.formatter(config);
+ }
+ days.push(config);
+ }
+ this.setData({ days: days });
+ },
+ getMultipleDayType: function (day) {
+ var currentDate = this.data.currentDate;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ var isSelected = function (date) {
+ return currentDate.some(function (item) { return (0, utils_1.compareDay)(item, date) === 0; });
+ };
+ if (isSelected(day)) {
+ var prevDay = (0, utils_1.getPrevDay)(day);
+ var nextDay = (0, utils_1.getNextDay)(day);
+ var prevSelected = isSelected(prevDay);
+ var nextSelected = isSelected(nextDay);
+ if (prevSelected && nextSelected) {
+ return 'multiple-middle';
+ }
+ if (prevSelected) {
+ return 'end';
+ }
+ return nextSelected ? 'start' : 'multiple-selected';
+ }
+ return '';
+ },
+ getRangeDayType: function (day) {
+ var _a = this.data, currentDate = _a.currentDate, allowSameDay = _a.allowSameDay;
+ if (!Array.isArray(currentDate)) {
+ return '';
+ }
+ var startDay = currentDate[0], endDay = currentDate[1];
+ if (!startDay) {
+ return '';
+ }
+ var compareToStart = (0, utils_1.compareDay)(day, startDay);
+ if (!endDay) {
+ return compareToStart === 0 ? 'start' : '';
+ }
+ var compareToEnd = (0, utils_1.compareDay)(day, endDay);
+ if (compareToStart === 0 && compareToEnd === 0 && allowSameDay) {
+ return 'start-end';
+ }
+ if (compareToStart === 0) {
+ return 'start';
+ }
+ if (compareToEnd === 0) {
+ return 'end';
+ }
+ if (compareToStart > 0 && compareToEnd < 0) {
+ return 'middle';
+ }
+ return '';
+ },
+ getDayType: function (day) {
+ var _a = this.data, type = _a.type, minDate = _a.minDate, maxDate = _a.maxDate, currentDate = _a.currentDate;
+ if ((0, utils_1.compareDay)(day, minDate) < 0 || (0, utils_1.compareDay)(day, maxDate) > 0) {
+ return 'disabled';
+ }
+ if (type === 'single') {
+ return (0, utils_1.compareDay)(day, currentDate) === 0 ? 'selected' : '';
+ }
+ if (type === 'multiple') {
+ return this.getMultipleDayType(day);
+ }
+ /* istanbul ignore else */
+ if (type === 'range') {
+ return this.getRangeDayType(day);
+ }
+ return '';
+ },
+ getBottomInfo: function (type) {
+ if (this.data.type === 'range') {
+ if (type === 'start') {
+ return '开始';
+ }
+ if (type === 'end') {
+ return '结束';
+ }
+ if (type === 'start-end') {
+ return '开始/结束';
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxml
new file mode 100644
index 0000000..0c73b2f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxml
@@ -0,0 +1,39 @@
+
+
+
+
+
+ {{ computed.formatMonthTitle(date) }}
+
+
+
+
+ {{ computed.getMark(date) }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+ {{ item.topInfo }}
+ {{ item.text }}
+
+ {{ item.bottomInfo }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxs
new file mode 100644
index 0000000..55e45a5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxs
@@ -0,0 +1,71 @@
+/* eslint-disable */
+var utils = require('../../utils.wxs');
+
+function getMark(date) {
+ return getDate(date).getMonth() + 1;
+}
+
+var ROW_HEIGHT = 64;
+
+function getDayStyle(type, index, date, rowHeight, color, firstDayOfWeek) {
+ var style = [];
+ var current = getDate(date).getDay() || 7;
+ var offset = current < firstDayOfWeek ? (7 - firstDayOfWeek + current) :
+ current === 7 && firstDayOfWeek === 0 ? 0 :
+ (current - firstDayOfWeek);
+
+ if (index === 0) {
+ style.push(['margin-left', (100 * offset) / 7 + '%']);
+ }
+
+ if (rowHeight !== ROW_HEIGHT) {
+ style.push(['height', rowHeight + 'px']);
+ }
+
+ if (color) {
+ if (
+ type === 'start' ||
+ type === 'end' ||
+ type === 'start-end' ||
+ type === 'multiple-selected' ||
+ type === 'multiple-middle'
+ ) {
+ style.push(['background', color]);
+ } else if (type === 'middle') {
+ style.push(['color', color]);
+ }
+ }
+
+ return style
+ .map(function(item) {
+ return item.join(':');
+ })
+ .join(';');
+}
+
+function formatMonthTitle(date) {
+ date = getDate(date);
+ return date.getFullYear() + '年' + (date.getMonth() + 1) + '月';
+}
+
+function getMonthStyle(visible, date, rowHeight) {
+ if (!visible) {
+ date = getDate(date);
+
+ var totalDay = utils.getMonthEndDay(
+ date.getFullYear(),
+ date.getMonth() + 1
+ );
+ var offset = getDate(date).getDay();
+ var padding = Math.ceil((totalDay + offset) / 7) * rowHeight;
+
+ return 'padding-bottom:' + padding + 'px';
+ }
+}
+
+module.exports = {
+ getMark: getMark,
+ getDayStyle: getDayStyle,
+ formatMonthTitle: formatMonthTitle,
+ getMonthStyle: getMonthStyle
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxss
new file mode 100644
index 0000000..9aee73d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/components/month/index.wxss
@@ -0,0 +1 @@
+@import '../../../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:100%}.van-calendar__month-title{font-size:var(--calendar-month-title-font-size,14px);font-weight:var(--font-weight-bold,500);height:var(--calendar-header-title-height,44px);line-height:var(--calendar-header-title-height,44px);text-align:center}.van-calendar__days{display:flex;flex-wrap:wrap;position:relative;-webkit-user-select:none;user-select:none}.van-calendar__month-mark{color:var(--calendar-month-mark-color,rgba(242,243,245,.8));font-size:var(--calendar-month-mark-font-size,160px);left:50%;pointer-events:none;position:absolute;top:50%;transform:translate(-50%,-50%);z-index:0}.van-calendar__day,.van-calendar__selected-day{align-items:center;display:flex;justify-content:center;text-align:center}.van-calendar__day{font-size:var(--calendar-day-font-size,16px);height:var(--calendar-day-height,64px);position:relative;width:14.285%}.van-calendar__day--end,.van-calendar__day--multiple-middle,.van-calendar__day--multiple-selected,.van-calendar__day--start,.van-calendar__day--start-end{background-color:var(--calendar-range-edge-background-color,#ee0a24);color:var(--calendar-range-edge-color,#fff)}.van-calendar__day--start{border-radius:4px 0 0 4px}.van-calendar__day--end{border-radius:0 4px 4px 0}.van-calendar__day--multiple-selected,.van-calendar__day--start-end{border-radius:4px}.van-calendar__day--middle{color:var(--calendar-range-middle-color,#ee0a24)}.van-calendar__day--middle:after{background-color:currentColor;bottom:0;content:"";left:0;opacity:var(--calendar-range-middle-background-opacity,.1);position:absolute;right:0;top:0}.van-calendar__day--disabled{color:var(--calendar-day-disabled-color,#c8c9cc);cursor:default}.van-calendar__bottom-info,.van-calendar__top-info{font-size:var(--calendar-info-font-size,10px);left:0;line-height:var(--calendar-info-line-height,14px);position:absolute;right:0}@media (max-width:350px){.van-calendar__bottom-info,.van-calendar__top-info{font-size:9px}}.van-calendar__top-info{top:6px}.van-calendar__bottom-info{bottom:6px}.van-calendar__selected-day{background-color:var(--calendar-selected-day-background-color,#ee0a24);border-radius:4px;color:var(--calendar-selected-day-color,#fff);height:var(--calendar-selected-day-size,54px);width:var(--calendar-selected-day-size,54px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.js
new file mode 100644
index 0000000..7a7324d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.js
@@ -0,0 +1,383 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+var __importDefault = (this && this.__importDefault) || function (mod) {
+ return (mod && mod.__esModule) ? mod : { "default": mod };
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+var toast_1 = __importDefault(require("../toast/toast"));
+var utils_2 = require("../common/utils");
+var initialMinDate = (0, utils_1.getToday)().getTime();
+var initialMaxDate = (function () {
+ var now = (0, utils_1.getToday)();
+ return new Date(now.getFullYear(), now.getMonth() + 6, now.getDate()).getTime();
+})();
+var getTime = function (date) {
+ return date instanceof Date ? date.getTime() : date;
+};
+(0, component_1.VantComponent)({
+ props: {
+ title: {
+ type: String,
+ value: '日期选择',
+ },
+ color: String,
+ show: {
+ type: Boolean,
+ observer: function (val) {
+ if (val) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ },
+ formatter: null,
+ confirmText: {
+ type: String,
+ value: '确定',
+ },
+ confirmDisabledText: {
+ type: String,
+ value: '确定',
+ },
+ rangePrompt: String,
+ showRangePrompt: {
+ type: Boolean,
+ value: true,
+ },
+ defaultDate: {
+ type: null,
+ value: (0, utils_1.getToday)().getTime(),
+ observer: function (val) {
+ this.setData({ currentDate: val });
+ this.scrollIntoView();
+ },
+ },
+ allowSameDay: Boolean,
+ type: {
+ type: String,
+ value: 'single',
+ observer: 'reset',
+ },
+ minDate: {
+ type: Number,
+ value: initialMinDate,
+ },
+ maxDate: {
+ type: Number,
+ value: initialMaxDate,
+ },
+ position: {
+ type: String,
+ value: 'bottom',
+ },
+ rowHeight: {
+ type: null,
+ value: utils_1.ROW_HEIGHT,
+ },
+ round: {
+ type: Boolean,
+ value: true,
+ },
+ poppable: {
+ type: Boolean,
+ value: true,
+ },
+ showMark: {
+ type: Boolean,
+ value: true,
+ },
+ showTitle: {
+ type: Boolean,
+ value: true,
+ },
+ showConfirm: {
+ type: Boolean,
+ value: true,
+ },
+ showSubtitle: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ maxRange: {
+ type: null,
+ value: null,
+ },
+ minRange: {
+ type: Number,
+ value: 1,
+ },
+ firstDayOfWeek: {
+ type: Number,
+ value: 0,
+ },
+ readonly: Boolean,
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ subtitle: '',
+ currentDate: null,
+ scrollIntoView: '',
+ },
+ watch: {
+ minDate: function () {
+ this.initRect();
+ },
+ maxDate: function () {
+ this.initRect();
+ },
+ },
+ created: function () {
+ this.setData({
+ currentDate: this.getInitialDate(this.data.defaultDate),
+ });
+ },
+ mounted: function () {
+ if (this.data.show || !this.data.poppable) {
+ this.initRect();
+ this.scrollIntoView();
+ }
+ },
+ methods: {
+ reset: function () {
+ this.setData({ currentDate: this.getInitialDate(this.data.defaultDate) });
+ this.scrollIntoView();
+ },
+ initRect: function () {
+ var _this = this;
+ if (this.contentObserver != null) {
+ this.contentObserver.disconnect();
+ }
+ var contentObserver = this.createIntersectionObserver({
+ thresholds: [0, 0.1, 0.9, 1],
+ observeAll: true,
+ });
+ this.contentObserver = contentObserver;
+ contentObserver.relativeTo('.van-calendar__body');
+ contentObserver.observe('.month', function (res) {
+ if (res.boundingClientRect.top <= res.relativeRect.top) {
+ // @ts-ignore
+ _this.setData({ subtitle: (0, utils_1.formatMonthTitle)(res.dataset.date) });
+ }
+ });
+ },
+ limitDateRange: function (date, minDate, maxDate) {
+ if (minDate === void 0) { minDate = null; }
+ if (maxDate === void 0) { maxDate = null; }
+ minDate = minDate || this.data.minDate;
+ maxDate = maxDate || this.data.maxDate;
+ if ((0, utils_1.compareDay)(date, minDate) === -1) {
+ return minDate;
+ }
+ if ((0, utils_1.compareDay)(date, maxDate) === 1) {
+ return maxDate;
+ }
+ return date;
+ },
+ getInitialDate: function (defaultDate) {
+ var _this = this;
+ if (defaultDate === void 0) { defaultDate = null; }
+ var _a = this.data, type = _a.type, minDate = _a.minDate, maxDate = _a.maxDate, allowSameDay = _a.allowSameDay;
+ if (!defaultDate)
+ return [];
+ var now = (0, utils_1.getToday)().getTime();
+ if (type === 'range') {
+ if (!Array.isArray(defaultDate)) {
+ defaultDate = [];
+ }
+ var _b = defaultDate || [], startDay = _b[0], endDay = _b[1];
+ var startDate = getTime(startDay || now);
+ var start = this.limitDateRange(startDate, minDate, allowSameDay ? startDate : (0, utils_1.getPrevDay)(new Date(maxDate)).getTime());
+ var date = getTime(endDay || now);
+ var end = this.limitDateRange(date, allowSameDay ? date : (0, utils_1.getNextDay)(new Date(minDate)).getTime());
+ return [start, end];
+ }
+ if (type === 'multiple') {
+ if (Array.isArray(defaultDate)) {
+ return defaultDate.map(function (date) { return _this.limitDateRange(date); });
+ }
+ return [this.limitDateRange(now)];
+ }
+ if (!defaultDate || Array.isArray(defaultDate)) {
+ defaultDate = now;
+ }
+ return this.limitDateRange(defaultDate);
+ },
+ scrollIntoView: function () {
+ var _this = this;
+ (0, utils_2.requestAnimationFrame)(function () {
+ var _a = _this.data, currentDate = _a.currentDate, type = _a.type, show = _a.show, poppable = _a.poppable, minDate = _a.minDate, maxDate = _a.maxDate;
+ if (!currentDate)
+ return;
+ // @ts-ignore
+ var targetDate = type === 'single' ? currentDate : currentDate[0];
+ var displayed = show || !poppable;
+ if (!targetDate || !displayed) {
+ return;
+ }
+ var months = (0, utils_1.getMonths)(minDate, maxDate);
+ months.some(function (month, index) {
+ if ((0, utils_1.compareMonth)(month, targetDate) === 0) {
+ _this.setData({ scrollIntoView: "month".concat(index) });
+ return true;
+ }
+ return false;
+ });
+ });
+ },
+ onOpen: function () {
+ this.$emit('open');
+ },
+ onOpened: function () {
+ this.$emit('opened');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClosed: function () {
+ this.$emit('closed');
+ },
+ onClickDay: function (event) {
+ if (this.data.readonly) {
+ return;
+ }
+ var date = event.detail.date;
+ var _a = this.data, type = _a.type, currentDate = _a.currentDate, allowSameDay = _a.allowSameDay;
+ if (type === 'range') {
+ // @ts-ignore
+ var startDay_1 = currentDate[0], endDay = currentDate[1];
+ if (startDay_1 && !endDay) {
+ var compareToStart = (0, utils_1.compareDay)(date, startDay_1);
+ if (compareToStart === 1) {
+ var days_1 = this.selectComponent('.month').data.days;
+ days_1.some(function (day, index) {
+ var isDisabled = day.type === 'disabled' &&
+ getTime(startDay_1) < getTime(day.date) &&
+ getTime(day.date) < getTime(date);
+ if (isDisabled) {
+ (date = days_1[index - 1].date);
+ }
+ return isDisabled;
+ });
+ this.select([startDay_1, date], true);
+ }
+ else if (compareToStart === -1) {
+ this.select([date, null]);
+ }
+ else if (allowSameDay) {
+ this.select([date, date], true);
+ }
+ }
+ else {
+ this.select([date, null]);
+ }
+ }
+ else if (type === 'multiple') {
+ var selectedIndex_1;
+ // @ts-ignore
+ var selected = currentDate.some(function (dateItem, index) {
+ var equal = (0, utils_1.compareDay)(dateItem, date) === 0;
+ if (equal) {
+ selectedIndex_1 = index;
+ }
+ return equal;
+ });
+ if (selected) {
+ // @ts-ignore
+ var cancelDate = currentDate.splice(selectedIndex_1, 1);
+ this.setData({ currentDate: currentDate });
+ this.unselect(cancelDate);
+ }
+ else {
+ // @ts-ignore
+ this.select(__spreadArray(__spreadArray([], currentDate, true), [date], false));
+ }
+ }
+ else {
+ this.select(date, true);
+ }
+ },
+ unselect: function (dateArray) {
+ var date = dateArray[0];
+ if (date) {
+ this.$emit('unselect', (0, utils_1.copyDates)(date));
+ }
+ },
+ select: function (date, complete) {
+ if (complete && this.data.type === 'range') {
+ var valid = this.checkRange(date);
+ if (!valid) {
+ // auto selected to max range if showConfirm
+ if (this.data.showConfirm) {
+ this.emit([
+ date[0],
+ (0, utils_1.getDayByOffset)(date[0], this.data.maxRange - 1),
+ ]);
+ }
+ else {
+ this.emit(date);
+ }
+ return;
+ }
+ }
+ this.emit(date);
+ if (complete && !this.data.showConfirm) {
+ this.onConfirm();
+ }
+ },
+ emit: function (date) {
+ this.setData({
+ currentDate: Array.isArray(date) ? date.map(getTime) : getTime(date),
+ });
+ this.$emit('select', (0, utils_1.copyDates)(date));
+ },
+ checkRange: function (date) {
+ var _a = this.data, maxRange = _a.maxRange, rangePrompt = _a.rangePrompt, showRangePrompt = _a.showRangePrompt;
+ if (maxRange && (0, utils_1.calcDateNum)(date) > maxRange) {
+ if (showRangePrompt) {
+ (0, toast_1.default)({
+ context: this,
+ message: rangePrompt || "\u9009\u62E9\u5929\u6570\u4E0D\u80FD\u8D85\u8FC7 ".concat(maxRange, " \u5929"),
+ });
+ }
+ this.$emit('over-range');
+ return false;
+ }
+ return true;
+ },
+ onConfirm: function () {
+ var _this = this;
+ if (this.data.type === 'range' &&
+ !this.checkRange(this.data.currentDate)) {
+ return;
+ }
+ wx.nextTick(function () {
+ // @ts-ignore
+ _this.$emit('confirm', (0, utils_1.copyDates)(_this.data.currentDate));
+ });
+ },
+ onClickSubtitle: function (event) {
+ this.$emit('click-subtitle', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.json
new file mode 100644
index 0000000..397d5ae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.json
@@ -0,0 +1,10 @@
+{
+ "component": true,
+ "usingComponents": {
+ "header": "./components/header/index",
+ "month": "./components/month/index",
+ "van-button": "../button/index",
+ "van-popup": "../popup/index",
+ "van-toast": "../toast/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxml
new file mode 100644
index 0000000..9d0fc6b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxs
new file mode 100644
index 0000000..0a56646
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxs
@@ -0,0 +1,37 @@
+/* eslint-disable */
+var utils = require('./utils.wxs');
+
+function getMonths(minDate, maxDate) {
+ var months = [];
+ var cursor = getDate(minDate);
+
+ cursor.setDate(1);
+
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (utils.compareMonth(cursor, getDate(maxDate)) !== 1);
+
+ return months;
+}
+
+function getButtonDisabled(type, currentDate, minRange) {
+ if (currentDate == null) {
+ return true;
+ }
+
+ if (type === 'range') {
+ return !currentDate[0] || !currentDate[1];
+ }
+
+ if (type === 'multiple') {
+ return currentDate.length < minRange;
+ }
+
+ return !currentDate;
+}
+
+module.exports = {
+ getMonths: getMonths,
+ getButtonDisabled: getButtonDisabled
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxss
new file mode 100644
index 0000000..a1f1cf0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-calendar{background-color:var(--calendar-background-color,#fff);display:flex;flex-direction:column;height:var(--calendar-height,100%)}.van-calendar__close-icon{top:11px}.van-calendar__popup--bottom,.van-calendar__popup--top{height:var(--calendar-popup-height,90%)}.van-calendar__popup--left,.van-calendar__popup--right{height:100%}.van-calendar__body{-webkit-overflow-scrolling:touch;flex:1;overflow:auto}.van-calendar__footer{flex-shrink:0;padding:0 var(--padding-md,16px)}.van-calendar__footer--safe-area-inset-bottom{padding-bottom:env(safe-area-inset-bottom)}.van-calendar__footer+.van-calendar__footer,.van-calendar__footer:empty{display:none}.van-calendar__footer:empty+.van-calendar__footer{display:block!important}.van-calendar__confirm{height:var(--calendar-confirm-button-height,36px)!important;line-height:var(--calendar-confirm-button-line-height,34px)!important;margin:var(--calendar-confirm-button-margin,7px 0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.d.ts
new file mode 100644
index 0000000..889e6e7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.d.ts
@@ -0,0 +1,12 @@
+export declare const ROW_HEIGHT = 64;
+export declare function formatMonthTitle(date: Date): string;
+export declare function compareMonth(date1: Date | number, date2: Date | number): 0 | 1 | -1;
+export declare function compareDay(day1: Date | number, day2: Date | number): 0 | 1 | -1;
+export declare function getDayByOffset(date: Date, offset: number): Date;
+export declare function getPrevDay(date: Date): Date;
+export declare function getNextDay(date: Date): Date;
+export declare function getToday(): Date;
+export declare function calcDateNum(date: [Date, Date]): number;
+export declare function copyDates(dates: Date | Date[]): Date | Date[];
+export declare function getMonthEndDay(year: number, month: number): number;
+export declare function getMonths(minDate: number, maxDate: number): number[];
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.js
new file mode 100644
index 0000000..c9e5df7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.js
@@ -0,0 +1,97 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.getMonths = exports.getMonthEndDay = exports.copyDates = exports.calcDateNum = exports.getToday = exports.getNextDay = exports.getPrevDay = exports.getDayByOffset = exports.compareDay = exports.compareMonth = exports.formatMonthTitle = exports.ROW_HEIGHT = void 0;
+exports.ROW_HEIGHT = 64;
+function formatMonthTitle(date) {
+ if (!(date instanceof Date)) {
+ date = new Date(date);
+ }
+ return "".concat(date.getFullYear(), "\u5E74").concat(date.getMonth() + 1, "\u6708");
+}
+exports.formatMonthTitle = formatMonthTitle;
+function compareMonth(date1, date2) {
+ if (!(date1 instanceof Date)) {
+ date1 = new Date(date1);
+ }
+ if (!(date2 instanceof Date)) {
+ date2 = new Date(date2);
+ }
+ var year1 = date1.getFullYear();
+ var year2 = date2.getFullYear();
+ var month1 = date1.getMonth();
+ var month2 = date2.getMonth();
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+ return year1 > year2 ? 1 : -1;
+}
+exports.compareMonth = compareMonth;
+function compareDay(day1, day2) {
+ if (!(day1 instanceof Date)) {
+ day1 = new Date(day1);
+ }
+ if (!(day2 instanceof Date)) {
+ day2 = new Date(day2);
+ }
+ var compareMonthResult = compareMonth(day1, day2);
+ if (compareMonthResult === 0) {
+ var date1 = day1.getDate();
+ var date2 = day2.getDate();
+ return date1 === date2 ? 0 : date1 > date2 ? 1 : -1;
+ }
+ return compareMonthResult;
+}
+exports.compareDay = compareDay;
+function getDayByOffset(date, offset) {
+ date = new Date(date);
+ date.setDate(date.getDate() + offset);
+ return date;
+}
+exports.getDayByOffset = getDayByOffset;
+function getPrevDay(date) {
+ return getDayByOffset(date, -1);
+}
+exports.getPrevDay = getPrevDay;
+function getNextDay(date) {
+ return getDayByOffset(date, 1);
+}
+exports.getNextDay = getNextDay;
+function getToday() {
+ var today = new Date();
+ today.setHours(0, 0, 0, 0);
+ return today;
+}
+exports.getToday = getToday;
+function calcDateNum(date) {
+ var day1 = new Date(date[0]).getTime();
+ var day2 = new Date(date[1]).getTime();
+ return (day2 - day1) / (1000 * 60 * 60 * 24) + 1;
+}
+exports.calcDateNum = calcDateNum;
+function copyDates(dates) {
+ if (Array.isArray(dates)) {
+ return dates.map(function (date) {
+ if (date === null) {
+ return date;
+ }
+ return new Date(date);
+ });
+ }
+ return new Date(dates);
+}
+exports.copyDates = copyDates;
+function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+exports.getMonthEndDay = getMonthEndDay;
+function getMonths(minDate, maxDate) {
+ var months = [];
+ var cursor = new Date(minDate);
+ cursor.setDate(1);
+ do {
+ months.push(cursor.getTime());
+ cursor.setMonth(cursor.getMonth() + 1);
+ } while (compareMonth(cursor, maxDate) !== 1);
+ return months;
+}
+exports.getMonths = getMonths;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.wxs
new file mode 100644
index 0000000..e57f6b3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/calendar/utils.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+function getMonthEndDay(year, month) {
+ return 32 - getDate(year, month - 1, 32).getDate();
+}
+
+function compareMonth(date1, date2) {
+ date1 = getDate(date1);
+ date2 = getDate(date2);
+
+ var year1 = date1.getFullYear();
+ var year2 = date2.getFullYear();
+ var month1 = date1.getMonth();
+ var month2 = date2.getMonth();
+
+ if (year1 === year2) {
+ return month1 === month2 ? 0 : month1 > month2 ? 1 : -1;
+ }
+
+ return year1 > year2 ? 1 : -1;
+}
+
+module.exports = {
+ getMonthEndDay: getMonthEndDay,
+ compareMonth: compareMonth
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.js
new file mode 100644
index 0000000..2815655
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.js
@@ -0,0 +1,51 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var link_1 = require("../mixins/link");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'num-class',
+ 'desc-class',
+ 'thumb-class',
+ 'title-class',
+ 'price-class',
+ 'origin-price-class',
+ ],
+ mixins: [link_1.link],
+ props: {
+ tag: String,
+ num: String,
+ desc: String,
+ thumb: String,
+ title: String,
+ price: {
+ type: String,
+ observer: 'updatePrice',
+ },
+ centered: Boolean,
+ lazyLoad: Boolean,
+ thumbLink: String,
+ originPrice: String,
+ thumbMode: {
+ type: String,
+ value: 'aspectFit',
+ },
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ },
+ methods: {
+ updatePrice: function () {
+ var price = this.data.price;
+ var priceArr = price.toString().split('.');
+ this.setData({
+ integerStr: priceArr[0],
+ decimalStr: priceArr[1] ? ".".concat(priceArr[1]) : '',
+ });
+ },
+ onClickThumb: function () {
+ this.jumpLink('thumbLink');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.json
new file mode 100644
index 0000000..e917407
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-tag": "../tag/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxml
new file mode 100644
index 0000000..62173e4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxss
new file mode 100644
index 0000000..0f4d7c5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/card/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-card{background-color:var(--card-background-color,#fafafa);box-sizing:border-box;color:var(--card-text-color,#323233);font-size:var(--card-font-size,12px);padding:var(--card-padding,8px 16px);position:relative}.van-card__header{display:flex}.van-card__header--center{align-items:center;justify-content:center}.van-card__thumb{flex:none;height:var(--card-thumb-size,88px);margin-right:var(--padding-xs,8px);position:relative;width:var(--card-thumb-size,88px)}.van-card__thumb:empty{display:none}.van-card__img{border-radius:8px;height:100%;width:100%}.van-card__content{display:flex;flex:1;flex-direction:column;justify-content:space-between;min-height:var(--card-thumb-size,88px);min-width:0;position:relative}.van-card__content--center{justify-content:center}.van-card__desc,.van-card__title{word-wrap:break-word}.van-card__title{font-weight:700;line-height:var(--card-title-line-height,16px)}.van-card__desc{color:var(--card-desc-color,#646566);line-height:var(--card-desc-line-height,20px)}.van-card__bottom{line-height:20px}.van-card__price{color:var(--card-price-color,#ee0a24);display:inline-block;font-size:var(--card-price-font-size,12px);font-weight:700}.van-card__price-integer{font-size:var(--card-price-integer-font-size,16px)}.van-card__price-decimal,.van-card__price-integer{font-family:var(--card-price-font-family,Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif)}.van-card__origin-price{color:var(--card-origin-price-color,#646566);display:inline-block;font-size:var(--card-origin-price-font-size,10px);margin-left:5px;text-decoration:line-through}.van-card__num{float:right}.van-card__tag{left:0;position:absolute!important;top:2px}.van-card__footer{flex:none;text-align:right;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.js
new file mode 100644
index 0000000..1b45831
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.js
@@ -0,0 +1,223 @@
+"use strict";
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var FieldName;
+(function (FieldName) {
+ FieldName["TEXT"] = "text";
+ FieldName["VALUE"] = "value";
+ FieldName["CHILDREN"] = "children";
+})(FieldName || (FieldName = {}));
+var defaultFieldNames = {
+ text: FieldName.TEXT,
+ value: FieldName.VALUE,
+ children: FieldName.CHILDREN,
+};
+(0, component_1.VantComponent)({
+ props: {
+ title: String,
+ value: {
+ type: String,
+ },
+ placeholder: {
+ type: String,
+ value: '请选择',
+ },
+ activeColor: {
+ type: String,
+ value: '#1989fa',
+ },
+ options: {
+ type: Array,
+ value: [],
+ },
+ swipeable: {
+ type: Boolean,
+ value: false,
+ },
+ closeable: {
+ type: Boolean,
+ value: true,
+ },
+ showHeader: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ fieldNames: {
+ type: Object,
+ value: defaultFieldNames,
+ observer: 'updateFieldNames',
+ },
+ useTitleSlot: Boolean,
+ },
+ data: {
+ tabs: [],
+ activeTab: 0,
+ textKey: FieldName.TEXT,
+ valueKey: FieldName.VALUE,
+ childrenKey: FieldName.CHILDREN,
+ innerValue: '',
+ },
+ watch: {
+ options: function () {
+ this.updateTabs();
+ },
+ value: function (newVal) {
+ this.updateValue(newVal);
+ },
+ },
+ created: function () {
+ this.updateTabs();
+ },
+ methods: {
+ updateValue: function (val) {
+ var _this = this;
+ if (val !== undefined) {
+ var values = this.data.tabs.map(function (tab) { return tab.selected && tab.selected[_this.data.valueKey]; });
+ if (values.indexOf(val) > -1) {
+ return;
+ }
+ }
+ this.innerValue = val;
+ this.updateTabs();
+ },
+ updateFieldNames: function () {
+ var _a = this.data.fieldNames || defaultFieldNames, _b = _a.text, text = _b === void 0 ? 'text' : _b, _c = _a.value, value = _c === void 0 ? 'value' : _c, _d = _a.children, children = _d === void 0 ? 'children' : _d;
+ this.setData({
+ textKey: text,
+ valueKey: value,
+ childrenKey: children,
+ });
+ },
+ getSelectedOptionsByValue: function (options, value) {
+ for (var i = 0; i < options.length; i++) {
+ var option = options[i];
+ if (option[this.data.valueKey] === value) {
+ return [option];
+ }
+ if (option[this.data.childrenKey]) {
+ var selectedOptions = this.getSelectedOptionsByValue(option[this.data.childrenKey], value);
+ if (selectedOptions) {
+ return __spreadArray([option], selectedOptions, true);
+ }
+ }
+ }
+ },
+ updateTabs: function () {
+ var _this = this;
+ var options = this.data.options;
+ var innerValue = this.innerValue;
+ if (!options.length) {
+ return;
+ }
+ if (innerValue !== undefined) {
+ var selectedOptions = this.getSelectedOptionsByValue(options, innerValue);
+ if (selectedOptions) {
+ var optionsCursor_1 = options;
+ var tabs_1 = selectedOptions.map(function (option) {
+ var tab = {
+ options: optionsCursor_1,
+ selected: option,
+ };
+ var next = optionsCursor_1.find(function (item) { return item[_this.data.valueKey] === option[_this.data.valueKey]; });
+ if (next) {
+ optionsCursor_1 = next[_this.data.childrenKey];
+ }
+ return tab;
+ });
+ if (optionsCursor_1) {
+ tabs_1.push({
+ options: optionsCursor_1,
+ selected: null,
+ });
+ }
+ this.setData({
+ tabs: tabs_1,
+ });
+ wx.nextTick(function () {
+ _this.setData({
+ activeTab: tabs_1.length - 1,
+ });
+ });
+ return;
+ }
+ }
+ this.setData({
+ tabs: [
+ {
+ options: options,
+ selected: null,
+ },
+ ],
+ });
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClickTab: function (e) {
+ var _a = e.detail, tabIndex = _a.index, title = _a.title;
+ this.$emit('click-tab', { title: title, tabIndex: tabIndex });
+ this.setData({
+ activeTab: tabIndex,
+ });
+ },
+ // 选中
+ onSelect: function (e) {
+ var _this = this;
+ var _a = e.currentTarget.dataset, option = _a.option, tabIndex = _a.tabIndex;
+ if (option && option.disabled) {
+ return;
+ }
+ var _b = this.data, valueKey = _b.valueKey, childrenKey = _b.childrenKey;
+ var tabs = this.data.tabs;
+ tabs[tabIndex].selected = option;
+ if (tabs.length > tabIndex + 1) {
+ tabs = tabs.slice(0, tabIndex + 1);
+ }
+ if (option[childrenKey]) {
+ var nextTab = {
+ options: option[childrenKey],
+ selected: null,
+ };
+ if (tabs[tabIndex + 1]) {
+ tabs[tabIndex + 1] = nextTab;
+ }
+ else {
+ tabs.push(nextTab);
+ }
+ wx.nextTick(function () {
+ _this.setData({
+ activeTab: tabIndex + 1,
+ });
+ });
+ }
+ this.setData({
+ tabs: tabs,
+ });
+ var selectedOptions = tabs.map(function (tab) { return tab.selected; }).filter(Boolean);
+ var value = option[valueKey];
+ var params = {
+ value: value,
+ tabIndex: tabIndex,
+ selectedOptions: selectedOptions,
+ };
+ this.innerValue = value;
+ this.$emit('change', params);
+ if (!option[childrenKey]) {
+ this.$emit('finish', params);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.json
new file mode 100644
index 0000000..d0f75eb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-tab": "../tab/index",
+ "van-tabs": "../tabs/index"
+ }
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxml
new file mode 100644
index 0000000..9417234
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ option[textKey] }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxs
new file mode 100644
index 0000000..b1aab58
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxs
@@ -0,0 +1,24 @@
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function isSelected(tab, valueKey, option) {
+ return tab.selected && tab.selected[valueKey] === option[valueKey]
+}
+
+function optionClass(tab, valueKey, option) {
+ return utils.bem('cascader__option', { selected: isSelected(tab, valueKey, option), disabled: option.disabled })
+}
+
+function optionStyle(data) {
+ var color = data.option.color || (isSelected(data.tab, data.valueKey, data.option) ? data.activeColor : undefined);
+ return style({
+ color
+ });
+}
+
+
+module.exports = {
+ isSelected: isSelected,
+ optionClass: optionClass,
+ optionStyle: optionStyle,
+};
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxss
new file mode 100644
index 0000000..7062486
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cascader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cascader__header{align-items:center;display:flex;height:48px;justify-content:space-between;padding:0 16px}.van-cascader__title{font-size:16px;font-weight:600;line-height:20px}.van-cascader__close-icon{color:#c8c9cc;font-size:22px;height:22px}.van-cascader__tabs-wrap{height:48px!important;padding:0 8px}.van-cascader__tab{color:#323233!important;flex:none!important;font-weight:600!important;padding:0 8px!important}.van-cascader__tab--unselected{color:#969799!important;font-weight:400!important}.van-cascader__option{align-items:center;cursor:pointer;display:flex;font-size:14px;justify-content:space-between;line-height:20px;padding:10px 16px}.van-cascader__option:active{background-color:#f2f3f5}.van-cascader__option--selected{color:#1989fa;font-weight:600}.van-cascader__option--disabled{color:#c8c9cc;cursor:not-allowed}.van-cascader__option--disabled:active{background-color:initial}.van-cascader__options{-webkit-overflow-scrolling:touch;box-sizing:border-box;height:384px;overflow-y:auto;padding-top:6px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.js
new file mode 100644
index 0000000..34a93a6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.js
@@ -0,0 +1,13 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ title: String,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ inset: Boolean,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxml
new file mode 100644
index 0000000..311e064
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxml
@@ -0,0 +1,11 @@
+
+
+
+ {{ title }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxss
new file mode 100644
index 0000000..08b252f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell-group--inset{border-radius:var(--cell-group-inset-border-radius,8px);margin:var(--cell-group-inset-padding,0 16px);overflow:hidden}.van-cell-group__title{color:var(--cell-group-title-color,#969799);font-size:var(--cell-group-title-font-size,14px);line-height:var(--cell-group-title-line-height,16px);padding:var(--cell-group-title-padding,16px 16px 8px)}.van-cell-group__title--inset{padding:var(--cell-group-inset-title-padding,16px 16px 8px 32px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.js
new file mode 100644
index 0000000..80f3039
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.js
@@ -0,0 +1,40 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var link_1 = require("../mixins/link");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'title-class',
+ 'label-class',
+ 'value-class',
+ 'right-icon-class',
+ 'hover-class',
+ ],
+ mixins: [link_1.link],
+ props: {
+ title: null,
+ value: null,
+ icon: String,
+ size: String,
+ label: String,
+ center: Boolean,
+ isLink: Boolean,
+ required: Boolean,
+ clickable: Boolean,
+ titleWidth: String,
+ customStyle: String,
+ arrowDirection: String,
+ useLabelSlot: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ titleStyle: String,
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxml
new file mode 100644
index 0000000..8387c3c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+ {{ title }}
+
+
+
+
+ {{ label }}
+
+
+
+
+ {{ value }}
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxs
new file mode 100644
index 0000000..e3500c4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function titleStyle(data) {
+ return style([
+ {
+ 'max-width': addUnit(data.titleWidth),
+ 'min-width': addUnit(data.titleWidth),
+ },
+ data.titleStyle,
+ ]);
+}
+
+module.exports = {
+ titleStyle: titleStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxss
new file mode 100644
index 0000000..1802f8e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-cell{background-color:var(--cell-background-color,#fff);box-sizing:border-box;color:var(--cell-text-color,#323233);display:flex;font-size:var(--cell-font-size,14px);line-height:var(--cell-line-height,24px);padding:var(--cell-vertical-padding,10px) var(--cell-horizontal-padding,16px);position:relative;width:100%}.van-cell:after{border-bottom:1px solid #ebedf0;bottom:0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:16px;transform:scaleY(.5);transform-origin:center}.van-cell--borderless:after{display:none}.van-cell-group{background-color:var(--cell-background-color,#fff)}.van-cell__label{color:var(--cell-label-color,#969799);font-size:var(--cell-label-font-size,12px);line-height:var(--cell-label-line-height,18px);margin-top:var(--cell-label-margin-top,3px)}.van-cell__value{color:var(--cell-value-color,#969799);overflow:hidden;text-align:right;vertical-align:middle}.van-cell__title,.van-cell__value{flex:1}.van-cell__title:empty,.van-cell__value:empty{display:none}.van-cell__left-icon-wrap,.van-cell__right-icon-wrap{align-items:center;display:flex;font-size:var(--cell-icon-size,16px);height:var(--cell-line-height,24px)}.van-cell__left-icon-wrap{margin-right:var(--padding-base,4px)}.van-cell__right-icon-wrap{color:var(--cell-right-icon-color,#969799);margin-left:var(--padding-base,4px)}.van-cell__left-icon{vertical-align:middle}.van-cell__left-icon,.van-cell__right-icon{line-height:var(--cell-line-height,24px)}.van-cell--clickable.van-cell--hover{background-color:var(--cell-active-color,#f2f3f5)}.van-cell--required{overflow:visible}.van-cell--required:before{color:var(--cell-required-color,#ee0a24);content:"*";font-size:var(--cell-font-size,14px);left:var(--padding-xs,8px);position:absolute}.van-cell--center{align-items:center}.van-cell--large{padding-bottom:var(--cell-large-vertical-padding,12px);padding-top:var(--cell-large-vertical-padding,12px)}.van-cell--large .van-cell__title{font-size:var(--cell-large-title-font-size,16px)}.van-cell--large .van-cell__value{font-size:var(--cell-large-value-font-size,16px)}.van-cell--large .van-cell__label{font-size:var(--cell-large-label-font-size,14px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.js
new file mode 100644
index 0000000..80c93a1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.js
@@ -0,0 +1,39 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useChildren)('checkbox', function (target) {
+ this.updateChild(target);
+ }),
+ props: {
+ max: Number,
+ value: {
+ type: Array,
+ observer: 'updateChildren',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ value: 'vertical',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ var _this = this;
+ this.children.forEach(function (child) { return _this.updateChild(child); });
+ },
+ updateChild: function (child) {
+ var _a = this.data, value = _a.value, disabled = _a.disabled, direction = _a.direction;
+ child.setData({
+ value: value.indexOf(child.data.name) !== -1,
+ parentDisabled: disabled,
+ direction: direction,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxml
new file mode 100644
index 0000000..638bf9d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxss
new file mode 100644
index 0000000..c5666d7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.js
new file mode 100644
index 0000000..6247365
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.js
@@ -0,0 +1,79 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+function emit(target, value) {
+ target.$emit('input', value);
+ target.$emit('change', value);
+}
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useParent)('checkbox-group'),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ value: Boolean,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ parentDisabled: false,
+ direction: 'vertical',
+ },
+ methods: {
+ emitChange: function (value) {
+ if (this.parent) {
+ this.setParentValue(this.parent, value);
+ }
+ else {
+ emit(this, value);
+ }
+ },
+ toggle: function () {
+ var _a = this.data, parentDisabled = _a.parentDisabled, disabled = _a.disabled, value = _a.value;
+ if (!disabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ onClickLabel: function () {
+ var _a = this.data, labelDisabled = _a.labelDisabled, parentDisabled = _a.parentDisabled, disabled = _a.disabled, value = _a.value;
+ if (!disabled && !labelDisabled && !parentDisabled) {
+ this.emitChange(!value);
+ }
+ },
+ setParentValue: function (parent, value) {
+ var parentValue = parent.data.value.slice();
+ var name = this.data.name;
+ var max = parent.data.max;
+ if (value) {
+ if (max && parentValue.length >= max) {
+ return;
+ }
+ if (parentValue.indexOf(name) === -1) {
+ parentValue.push(name);
+ emit(parent, parentValue);
+ }
+ }
+ else {
+ var index = parentValue.indexOf(name);
+ if (index !== -1) {
+ parentValue.splice(index, 1);
+ emit(parent, parentValue);
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxml
new file mode 100644
index 0000000..39a7bb0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxml
@@ -0,0 +1,31 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxs
new file mode 100644
index 0000000..eb9c772
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxs
@@ -0,0 +1,20 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(checkedColor, value, disabled, parentDisabled, iconSize) {
+ var styles = {
+ 'font-size': addUnit(iconSize),
+ };
+
+ if (checkedColor && value && !disabled && !parentDisabled) {
+ styles['border-color'] = checkedColor;
+ styles['background-color'] = checkedColor;
+ }
+
+ return style(styles);
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxss
new file mode 100644
index 0000000..da2272a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/checkbox/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-checkbox{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-checkbox--horizontal{margin-right:12px}.van-checkbox__icon-wrap,.van-checkbox__label{line-height:var(--checkbox-size,20px)}.van-checkbox__icon-wrap{flex:none}.van-checkbox__icon{align-items:center;border:1px solid var(--checkbox-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--checkbox-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--checkbox-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-checkbox__icon--round{border-radius:100%}.van-checkbox__icon--checked{background-color:var(--checkbox-checked-icon-color,#1989fa);border-color:var(--checkbox-checked-icon-color,#1989fa);color:#fff}.van-checkbox__icon--disabled{background-color:var(--checkbox-disabled-background-color,#ebedf0);border-color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__icon--disabled.van-checkbox__icon--checked{color:var(--checkbox-disabled-icon-color,#c8c9cc)}.van-checkbox__label{word-wrap:break-word;color:var(--checkbox-label-color,#323233);padding-left:var(--checkbox-label-margin,10px)}.van-checkbox__label--left{float:left;margin:0 var(--checkbox-label-margin,10px) 0 0}.van-checkbox__label--disabled{color:var(--checkbox-disabled-label-color,#c8c9cc)}.van-checkbox__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.d.ts
new file mode 100644
index 0000000..8a0b71e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.d.ts
@@ -0,0 +1,4 @@
+///
+type CanvasContext = WechatMiniprogram.CanvasContext;
+export declare function adaptor(ctx: CanvasContext & Record): CanvasContext;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.js
new file mode 100644
index 0000000..d81df74
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/canvas.js
@@ -0,0 +1,47 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.adaptor = void 0;
+function adaptor(ctx) {
+ // @ts-ignore
+ return Object.assign(ctx, {
+ setStrokeStyle: function (val) {
+ ctx.strokeStyle = val;
+ },
+ setLineWidth: function (val) {
+ ctx.lineWidth = val;
+ },
+ setLineCap: function (val) {
+ ctx.lineCap = val;
+ },
+ setFillStyle: function (val) {
+ ctx.fillStyle = val;
+ },
+ setFontSize: function (val) {
+ ctx.font = String(val);
+ },
+ setGlobalAlpha: function (val) {
+ ctx.globalAlpha = val;
+ },
+ setLineJoin: function (val) {
+ ctx.lineJoin = val;
+ },
+ setTextAlign: function (val) {
+ ctx.textAlign = val;
+ },
+ setMiterLimit: function (val) {
+ ctx.miterLimit = val;
+ },
+ setShadow: function (offsetX, offsetY, blur, color) {
+ ctx.shadowOffsetX = offsetX;
+ ctx.shadowOffsetY = offsetY;
+ ctx.shadowBlur = blur;
+ ctx.shadowColor = color;
+ },
+ setTextBaseline: function (val) {
+ ctx.textBaseline = val;
+ },
+ createCircularGradient: function () { },
+ draw: function () { },
+ });
+}
+exports.adaptor = adaptor;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.js
new file mode 100644
index 0000000..e131e4b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.js
@@ -0,0 +1,207 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var version_1 = require("../common/version");
+var canvas_1 = require("./canvas");
+function format(rate) {
+ return Math.min(Math.max(rate, 0), 100);
+}
+var PERIMETER = 2 * Math.PI;
+var BEGIN_ANGLE = -Math.PI / 2;
+var STEP = 1;
+(0, component_1.VantComponent)({
+ props: {
+ text: String,
+ lineCap: {
+ type: String,
+ value: 'round',
+ },
+ value: {
+ type: Number,
+ value: 0,
+ observer: 'reRender',
+ },
+ speed: {
+ type: Number,
+ value: 50,
+ },
+ size: {
+ type: Number,
+ value: 100,
+ observer: function () {
+ this.drawCircle(this.currentValue);
+ },
+ },
+ fill: String,
+ layerColor: {
+ type: String,
+ value: color_1.WHITE,
+ },
+ color: {
+ type: null,
+ value: color_1.BLUE,
+ observer: function () {
+ var _this = this;
+ this.setHoverColor().then(function () {
+ _this.drawCircle(_this.currentValue);
+ });
+ },
+ },
+ type: {
+ type: String,
+ value: '',
+ },
+ strokeWidth: {
+ type: Number,
+ value: 4,
+ },
+ clockwise: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ hoverColor: color_1.BLUE,
+ },
+ methods: {
+ getContext: function () {
+ var _this = this;
+ var _a = this.data, type = _a.type, size = _a.size;
+ if (type === '' || !(0, version_1.canIUseCanvas2d)()) {
+ var ctx = wx.createCanvasContext('van-circle', this);
+ return Promise.resolve(ctx);
+ }
+ var dpr = (0, utils_1.getSystemInfoSync)().pixelRatio;
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(_this)
+ .select('#van-circle')
+ .node()
+ .exec(function (res) {
+ var canvas = res[0].node;
+ var ctx = canvas.getContext(type);
+ if (!_this.inited) {
+ _this.inited = true;
+ canvas.width = size * dpr;
+ canvas.height = size * dpr;
+ ctx.scale(dpr, dpr);
+ }
+ resolve((0, canvas_1.adaptor)(ctx));
+ });
+ });
+ },
+ setHoverColor: function () {
+ var _this = this;
+ var _a = this.data, color = _a.color, size = _a.size;
+ if ((0, validator_1.isObj)(color)) {
+ return this.getContext().then(function (context) {
+ if (!context)
+ return;
+ var LinearColor = context.createLinearGradient(size, 0, 0, 0);
+ Object.keys(color)
+ .sort(function (a, b) { return parseFloat(a) - parseFloat(b); })
+ .map(function (key) {
+ return LinearColor.addColorStop(parseFloat(key) / 100, color[key]);
+ });
+ _this.hoverColor = LinearColor;
+ });
+ }
+ this.hoverColor = color;
+ return Promise.resolve();
+ },
+ presetCanvas: function (context, strokeStyle, beginAngle, endAngle, fill) {
+ var _a = this.data, strokeWidth = _a.strokeWidth, lineCap = _a.lineCap, clockwise = _a.clockwise, size = _a.size;
+ var position = size / 2;
+ var radius = position - strokeWidth / 2;
+ context.setStrokeStyle(strokeStyle);
+ context.setLineWidth(strokeWidth);
+ context.setLineCap(lineCap);
+ context.beginPath();
+ context.arc(position, position, radius, beginAngle, endAngle, !clockwise);
+ context.stroke();
+ if (fill) {
+ context.setFillStyle(fill);
+ context.fill();
+ }
+ },
+ renderLayerCircle: function (context) {
+ var _a = this.data, layerColor = _a.layerColor, fill = _a.fill;
+ this.presetCanvas(context, layerColor, 0, PERIMETER, fill);
+ },
+ renderHoverCircle: function (context, formatValue) {
+ var clockwise = this.data.clockwise;
+ // 结束角度
+ var progress = PERIMETER * (formatValue / 100);
+ var endAngle = clockwise
+ ? BEGIN_ANGLE + progress
+ : 3 * Math.PI - (BEGIN_ANGLE + progress);
+ this.presetCanvas(context, this.hoverColor, BEGIN_ANGLE, endAngle);
+ },
+ drawCircle: function (currentValue) {
+ var _this = this;
+ var size = this.data.size;
+ this.getContext().then(function (context) {
+ if (!context)
+ return;
+ context.clearRect(0, 0, size, size);
+ _this.renderLayerCircle(context);
+ var formatValue = format(currentValue);
+ if (formatValue !== 0) {
+ _this.renderHoverCircle(context, formatValue);
+ }
+ context.draw();
+ });
+ },
+ reRender: function () {
+ var _this = this;
+ // tofector 动画暂时没有想到好的解决方案
+ var _a = this.data, value = _a.value, speed = _a.speed;
+ if (speed <= 0 || speed > 1000) {
+ this.drawCircle(value);
+ return;
+ }
+ this.clearMockInterval();
+ this.currentValue = this.currentValue || 0;
+ var run = function () {
+ _this.interval = setTimeout(function () {
+ if (_this.currentValue !== value) {
+ if (Math.abs(_this.currentValue - value) < STEP) {
+ _this.currentValue = value;
+ }
+ else if (_this.currentValue < value) {
+ _this.currentValue += STEP;
+ }
+ else {
+ _this.currentValue -= STEP;
+ }
+ _this.drawCircle(_this.currentValue);
+ run();
+ }
+ else {
+ _this.clearMockInterval();
+ }
+ }, 1000 / speed);
+ };
+ run();
+ },
+ clearMockInterval: function () {
+ if (this.interval) {
+ clearTimeout(this.interval);
+ this.interval = null;
+ }
+ },
+ },
+ mounted: function () {
+ var _this = this;
+ this.currentValue = this.data.value;
+ this.setHoverColor().then(function () {
+ _this.drawCircle(_this.currentValue);
+ });
+ },
+ destroyed: function () {
+ this.clearMockInterval();
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxml
new file mode 100644
index 0000000..52bc59f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxss
new file mode 100644
index 0000000..2200751
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/circle/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-circle{display:inline-block;position:relative;text-align:center}.van-circle__text{color:var(--circle-text-color,#323233);left:0;position:absolute;top:50%;transform:translateY(-50%);width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.js
new file mode 100644
index 0000000..63c56eb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('row'),
+ props: {
+ span: Number,
+ offset: Number,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxml
new file mode 100644
index 0000000..975348b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxs
new file mode 100644
index 0000000..507c1cb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'padding-right': addUnit(data.gutter / 2),
+ 'padding-left': addUnit(data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxss
new file mode 100644
index 0000000..2fa265e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/col/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-col{box-sizing:border-box;float:left}.van-col--1{width:4.16666667%}.van-col--offset-1{margin-left:4.16666667%}.van-col--2{width:8.33333333%}.van-col--offset-2{margin-left:8.33333333%}.van-col--3{width:12.5%}.van-col--offset-3{margin-left:12.5%}.van-col--4{width:16.66666667%}.van-col--offset-4{margin-left:16.66666667%}.van-col--5{width:20.83333333%}.van-col--offset-5{margin-left:20.83333333%}.van-col--6{width:25%}.van-col--offset-6{margin-left:25%}.van-col--7{width:29.16666667%}.van-col--offset-7{margin-left:29.16666667%}.van-col--8{width:33.33333333%}.van-col--offset-8{margin-left:33.33333333%}.van-col--9{width:37.5%}.van-col--offset-9{margin-left:37.5%}.van-col--10{width:41.66666667%}.van-col--offset-10{margin-left:41.66666667%}.van-col--11{width:45.83333333%}.van-col--offset-11{margin-left:45.83333333%}.van-col--12{width:50%}.van-col--offset-12{margin-left:50%}.van-col--13{width:54.16666667%}.van-col--offset-13{margin-left:54.16666667%}.van-col--14{width:58.33333333%}.van-col--offset-14{margin-left:58.33333333%}.van-col--15{width:62.5%}.van-col--offset-15{margin-left:62.5%}.van-col--16{width:66.66666667%}.van-col--offset-16{margin-left:66.66666667%}.van-col--17{width:70.83333333%}.van-col--offset-17{margin-left:70.83333333%}.van-col--18{width:75%}.van-col--offset-18{margin-left:75%}.van-col--19{width:79.16666667%}.van-col--offset-19{margin-left:79.16666667%}.van-col--20{width:83.33333333%}.van-col--offset-20{margin-left:83.33333333%}.van-col--21{width:87.5%}.van-col--offset-21{margin-left:87.5%}.van-col--22{width:91.66666667%}.van-col--offset-22{margin-left:91.66666667%}.van-col--23{width:95.83333333%}.van-col--offset-23{margin-left:95.83333333%}.van-col--24{width:100%}.van-col--offset-24{margin-left:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.d.ts
new file mode 100644
index 0000000..32157b6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.d.ts
@@ -0,0 +1,2 @@
+///
+export declare function setContentAnimate(context: WechatMiniprogram.Component.TrivialInstance, expanded: boolean, mounted: boolean): void;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.js
new file mode 100644
index 0000000..5734087
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/animate.js
@@ -0,0 +1,43 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.setContentAnimate = void 0;
+var utils_1 = require("../common/utils");
+function useAnimation(context, expanded, mounted, height) {
+ var animation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'ease-in-out',
+ });
+ if (expanded) {
+ if (height === 0) {
+ animation.height('auto').top(1).step();
+ }
+ else {
+ animation
+ .height(height)
+ .top(1)
+ .step({
+ duration: mounted ? 300 : 1,
+ })
+ .height('auto')
+ .step();
+ }
+ context.setData({
+ animation: animation.export(),
+ });
+ return;
+ }
+ animation.height(height).top(0).step({ duration: 1 }).height(0).step({
+ duration: 300,
+ });
+ context.setData({
+ animation: animation.export(),
+ });
+}
+function setContentAnimate(context, expanded, mounted) {
+ (0, utils_1.getRect)(context, '.van-collapse-item__content')
+ .then(function (rect) { return rect.height; })
+ .then(function (height) {
+ useAnimation(context, expanded, mounted, height);
+ });
+}
+exports.setContentAnimate = setContentAnimate;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.js
new file mode 100644
index 0000000..982490e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.js
@@ -0,0 +1,62 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var animate_1 = require("./animate");
+(0, component_1.VantComponent)({
+ classes: ['title-class', 'content-class'],
+ relation: (0, relation_1.useParent)('collapse'),
+ props: {
+ size: String,
+ name: null,
+ title: null,
+ value: null,
+ icon: String,
+ label: String,
+ disabled: Boolean,
+ clickable: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ isLink: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ expanded: false,
+ },
+ mounted: function () {
+ this.updateExpanded();
+ this.mounted = true;
+ },
+ methods: {
+ updateExpanded: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent.data, value = _a.value, accordion = _a.accordion;
+ var _b = this.parent.children, children = _b === void 0 ? [] : _b;
+ var name = this.data.name;
+ var index = children.indexOf(this);
+ var currentName = name == null ? index : name;
+ var expanded = accordion
+ ? value === currentName
+ : (value || []).some(function (name) { return name === currentName; });
+ if (expanded !== this.data.expanded) {
+ (0, animate_1.setContentAnimate)(this, expanded, this.mounted);
+ }
+ this.setData({ index: index, expanded: expanded });
+ },
+ onClick: function () {
+ if (this.data.disabled) {
+ return;
+ }
+ var _a = this.data, name = _a.name, expanded = _a.expanded;
+ var index = this.parent.children.indexOf(this);
+ var currentName = name == null ? index : name;
+ this.parent.switch(currentName, !expanded);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxml
new file mode 100644
index 0000000..f11d0d4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxml
@@ -0,0 +1,45 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxss
new file mode 100644
index 0000000..4a65b5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-collapse-item__title .van-cell__right-icon{transform:rotate(90deg);transition:transform var(--collapse-item-transition-duration,.3s)}.van-collapse-item__title--expanded .van-cell__right-icon{transform:rotate(-90deg)}.van-collapse-item__title--disabled .van-cell,.van-collapse-item__title--disabled .van-cell__right-icon{color:var(--collapse-item-title-disabled-color,#c8c9cc)!important}.van-collapse-item__title--disabled .van-cell--hover{background-color:#fff!important}.van-collapse-item__wrapper{overflow:hidden}.van-collapse-item__content{background-color:var(--collapse-item-content-background-color,#fff);color:var(--collapse-item-content-text-color,#969799);font-size:var(--collapse-item-content-font-size,13px);line-height:var(--collapse-item-content-line-height,1.5);padding:var(--collapse-item-content-padding,15px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.js
new file mode 100644
index 0000000..943d542
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.js
@@ -0,0 +1,48 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('collapse-item'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateExpanded',
+ },
+ accordion: {
+ type: Boolean,
+ observer: 'updateExpanded',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updateExpanded: function () {
+ this.children.forEach(function (child) {
+ child.updateExpanded();
+ });
+ },
+ switch: function (name, expanded) {
+ var _a = this.data, accordion = _a.accordion, value = _a.value;
+ var changeItem = name;
+ if (!accordion) {
+ name = expanded
+ ? (value || []).concat(name)
+ : (value || []).filter(function (activeName) { return activeName !== name; });
+ }
+ else {
+ name = expanded ? name : '';
+ }
+ if (expanded) {
+ this.$emit('open', changeItem);
+ }
+ else {
+ this.$emit('close', changeItem);
+ }
+ this.$emit('change', name);
+ this.$emit('input', name);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxml
new file mode 100644
index 0000000..fd4e171
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxml
@@ -0,0 +1,3 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/collapse/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.d.ts
new file mode 100644
index 0000000..386f307
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.d.ts
@@ -0,0 +1,7 @@
+export declare const RED = "#ee0a24";
+export declare const BLUE = "#1989fa";
+export declare const WHITE = "#fff";
+export declare const GREEN = "#07c160";
+export declare const ORANGE = "#ff976a";
+export declare const GRAY = "#323233";
+export declare const GRAY_DARK = "#969799";
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.js
new file mode 100644
index 0000000..008a45a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/color.js
@@ -0,0 +1,10 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.GRAY_DARK = exports.GRAY = exports.ORANGE = exports.GREEN = exports.WHITE = exports.BLUE = exports.RED = void 0;
+exports.RED = '#ee0a24';
+exports.BLUE = '#1989fa';
+exports.WHITE = '#fff';
+exports.GREEN = '#07c160';
+exports.ORANGE = '#ff976a';
+exports.GRAY = '#323233';
+exports.GRAY_DARK = '#969799';
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.d.ts
new file mode 100644
index 0000000..1d0fd27
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.d.ts
@@ -0,0 +1,4 @@
+///
+import { VantComponentOptions } from 'definitions/index';
+declare function VantComponent(vantOptions: VantComponentOptions): void;
+export { VantComponent };
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.js
new file mode 100644
index 0000000..66da00e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/component.js
@@ -0,0 +1,49 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.VantComponent = void 0;
+var basic_1 = require("../mixins/basic");
+function mapKeys(source, target, map) {
+ Object.keys(map).forEach(function (key) {
+ if (source[key]) {
+ target[map[key]] = source[key];
+ }
+ });
+}
+function VantComponent(vantOptions) {
+ var options = {};
+ mapKeys(vantOptions, options, {
+ data: 'data',
+ props: 'properties',
+ watch: 'observers',
+ mixins: 'behaviors',
+ methods: 'methods',
+ beforeCreate: 'created',
+ created: 'attached',
+ mounted: 'ready',
+ destroyed: 'detached',
+ classes: 'externalClasses',
+ });
+ // add default externalClasses
+ options.externalClasses = options.externalClasses || [];
+ options.externalClasses.push('custom-class');
+ // add default behaviors
+ options.behaviors = options.behaviors || [];
+ options.behaviors.push(basic_1.basic);
+ // add relations
+ var relation = vantOptions.relation;
+ if (relation) {
+ options.relations = relation.relations;
+ options.behaviors.push(relation.mixin);
+ }
+ // map field to form-field behavior
+ if (vantOptions.field) {
+ options.behaviors.push('wx://form-field');
+ }
+ // add default options
+ options.options = {
+ multipleSlots: true,
+ addGlobalClass: true,
+ };
+ Component(options);
+}
+exports.VantComponent = VantComponent;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/index.wxss
new file mode 100644
index 0000000..a73bb7a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/index.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}.van-clearfix:after{clear:both;content:"";display:table}.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.d.ts
new file mode 100644
index 0000000..10193fa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.d.ts
@@ -0,0 +1,15 @@
+///
+type TrivialInstance = WechatMiniprogram.Component.TrivialInstance;
+export declare function useParent(name: string, onEffect?: (this: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export declare function useChildren(name: string, onEffect?: (this: TrivialInstance, target: TrivialInstance) => void): {
+ relations: {
+ [x: string]: WechatMiniprogram.Component.RelationOption;
+ };
+ mixin: string;
+};
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.js
new file mode 100644
index 0000000..008256c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/relation.js
@@ -0,0 +1,65 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.useChildren = exports.useParent = void 0;
+function useParent(name, onEffect) {
+ var _a;
+ var path = "../".concat(name, "/index");
+ return {
+ relations: (_a = {},
+ _a[path] = {
+ type: 'ancestor',
+ linked: function () {
+ onEffect && onEffect.call(this);
+ },
+ linkChanged: function () {
+ onEffect && onEffect.call(this);
+ },
+ unlinked: function () {
+ onEffect && onEffect.call(this);
+ },
+ },
+ _a),
+ mixin: Behavior({
+ created: function () {
+ var _this = this;
+ Object.defineProperty(this, 'parent', {
+ get: function () { return _this.getRelationNodes(path)[0]; },
+ });
+ Object.defineProperty(this, 'index', {
+ // @ts-ignore
+ get: function () { var _a, _b; return (_b = (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.children) === null || _b === void 0 ? void 0 : _b.indexOf(_this); },
+ });
+ },
+ }),
+ };
+}
+exports.useParent = useParent;
+function useChildren(name, onEffect) {
+ var _a;
+ var path = "../".concat(name, "/index");
+ return {
+ relations: (_a = {},
+ _a[path] = {
+ type: 'descendant',
+ linked: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ linkChanged: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ unlinked: function (target) {
+ onEffect && onEffect.call(this, target);
+ },
+ },
+ _a),
+ mixin: Behavior({
+ created: function () {
+ var _this = this;
+ Object.defineProperty(this, 'children', {
+ get: function () { return _this.getRelationNodes(path) || []; },
+ });
+ },
+ }),
+ };
+}
+exports.useChildren = useChildren;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/clearfix.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/clearfix.wxss
new file mode 100644
index 0000000..442246f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/clearfix.wxss
@@ -0,0 +1 @@
+.van-clearfix:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/ellipsis.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/ellipsis.wxss
new file mode 100644
index 0000000..ee701df
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/ellipsis.wxss
@@ -0,0 +1 @@
+.van-ellipsis{overflow:hidden;text-overflow:ellipsis;white-space:nowrap}.van-multi-ellipsis--l2{-webkit-line-clamp:2}.van-multi-ellipsis--l2,.van-multi-ellipsis--l3{-webkit-box-orient:vertical;display:-webkit-box;overflow:hidden;text-overflow:ellipsis}.van-multi-ellipsis--l3{-webkit-line-clamp:3}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/hairline.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/hairline.wxss
new file mode 100644
index 0000000..f7c6260
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/hairline.wxss
@@ -0,0 +1 @@
+.van-hairline,.van-hairline--bottom,.van-hairline--left,.van-hairline--right,.van-hairline--surround,.van-hairline--top,.van-hairline--top-bottom{position:relative}.van-hairline--bottom:after,.van-hairline--left:after,.van-hairline--right:after,.van-hairline--surround:after,.van-hairline--top-bottom:after,.van-hairline--top:after,.van-hairline:after{border:0 solid #ebedf0;bottom:-50%;box-sizing:border-box;content:" ";left:-50%;pointer-events:none;position:absolute;right:-50%;top:-50%;transform:scale(.5);transform-origin:center}.van-hairline--top:after{border-top-width:1px}.van-hairline--left:after{border-left-width:1px}.van-hairline--right:after{border-right-width:1px}.van-hairline--bottom:after{border-bottom-width:1px}.van-hairline--top-bottom:after{border-width:1px 0}.van-hairline--surround:after{border-width:1px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/clearfix.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/clearfix.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/ellipsis.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/ellipsis.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/hairline.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/mixins/hairline.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/var.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/style/var.wxss
new file mode 100644
index 0000000..e69de29
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.d.ts
new file mode 100644
index 0000000..a77d8c6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.d.ts
@@ -0,0 +1,21 @@
+///
+///
+///
+///
+///
+export { isDef } from './validator';
+export { getSystemInfoSync } from './version';
+export declare function range(num: number, min: number, max: number): number;
+export declare function nextTick(cb: (...args: any[]) => void): void;
+export declare function addUnit(value?: string | number): string | undefined;
+export declare function requestAnimationFrame(cb: () => void): NodeJS.Timeout;
+export declare function pickExclude(obj: unknown, keys: string[]): {};
+export declare function getRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function getAllRect(context: WechatMiniprogram.Component.TrivialInstance, selector: string): Promise;
+export declare function groupSetData(context: WechatMiniprogram.Component.TrivialInstance, cb: () => void): void;
+export declare function toPromise(promiseLike: Promise | unknown): Promise;
+export declare function addNumber(num1: any, num2: any): number;
+export declare const clamp: (num: any, min: any, max: any) => number;
+export declare function getCurrentPage(): T & WechatMiniprogram.OptionalInterface & WechatMiniprogram.Page.InstanceProperties & WechatMiniprogram.Page.InstanceMethods & WechatMiniprogram.Page.Data & WechatMiniprogram.IAnyObject;
+export declare const isPC: boolean;
+export declare const isWxWork: boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.js
new file mode 100644
index 0000000..1727628
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/utils.js
@@ -0,0 +1,109 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isWxWork = exports.isPC = exports.getCurrentPage = exports.clamp = exports.addNumber = exports.toPromise = exports.groupSetData = exports.getAllRect = exports.getRect = exports.pickExclude = exports.requestAnimationFrame = exports.addUnit = exports.nextTick = exports.range = exports.getSystemInfoSync = exports.isDef = void 0;
+var validator_1 = require("./validator");
+var version_1 = require("./version");
+var validator_2 = require("./validator");
+Object.defineProperty(exports, "isDef", { enumerable: true, get: function () { return validator_2.isDef; } });
+var version_2 = require("./version");
+Object.defineProperty(exports, "getSystemInfoSync", { enumerable: true, get: function () { return version_2.getSystemInfoSync; } });
+function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+exports.range = range;
+function nextTick(cb) {
+ if ((0, version_1.canIUseNextTick)()) {
+ wx.nextTick(cb);
+ }
+ else {
+ setTimeout(function () {
+ cb();
+ }, 1000 / 30);
+ }
+}
+exports.nextTick = nextTick;
+function addUnit(value) {
+ if (!(0, validator_1.isDef)(value)) {
+ return undefined;
+ }
+ value = String(value);
+ return (0, validator_1.isNumber)(value) ? "".concat(value, "px") : value;
+}
+exports.addUnit = addUnit;
+function requestAnimationFrame(cb) {
+ return setTimeout(function () {
+ cb();
+ }, 1000 / 30);
+}
+exports.requestAnimationFrame = requestAnimationFrame;
+function pickExclude(obj, keys) {
+ if (!(0, validator_1.isPlainObject)(obj)) {
+ return {};
+ }
+ return Object.keys(obj).reduce(function (prev, key) {
+ if (!keys.includes(key)) {
+ prev[key] = obj[key];
+ }
+ return prev;
+ }, {});
+}
+exports.pickExclude = pickExclude;
+function getRect(context, selector) {
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(context)
+ .select(selector)
+ .boundingClientRect()
+ .exec(function (rect) {
+ if (rect === void 0) { rect = []; }
+ return resolve(rect[0]);
+ });
+ });
+}
+exports.getRect = getRect;
+function getAllRect(context, selector) {
+ return new Promise(function (resolve) {
+ wx.createSelectorQuery()
+ .in(context)
+ .selectAll(selector)
+ .boundingClientRect()
+ .exec(function (rect) {
+ if (rect === void 0) { rect = []; }
+ return resolve(rect[0]);
+ });
+ });
+}
+exports.getAllRect = getAllRect;
+function groupSetData(context, cb) {
+ if ((0, version_1.canIUseGroupSetData)()) {
+ context.groupSetData(cb);
+ }
+ else {
+ cb();
+ }
+}
+exports.groupSetData = groupSetData;
+function toPromise(promiseLike) {
+ if ((0, validator_1.isPromise)(promiseLike)) {
+ return promiseLike;
+ }
+ return Promise.resolve(promiseLike);
+}
+exports.toPromise = toPromise;
+// 浮点数精度处理
+function addNumber(num1, num2) {
+ var cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+exports.addNumber = addNumber;
+// 限制value在[min, max]之间
+var clamp = function (num, min, max) { return Math.min(Math.max(num, min), max); };
+exports.clamp = clamp;
+function getCurrentPage() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+exports.getCurrentPage = getCurrentPage;
+exports.isPC = ['mac', 'windows'].includes((0, version_1.getSystemInfoSync)().platform);
+// 是否企业微信
+exports.isWxWork = (0, version_1.getSystemInfoSync)().environment === 'wxwork';
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.d.ts
new file mode 100644
index 0000000..152894a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.d.ts
@@ -0,0 +1,9 @@
+export declare function isFunction(val: unknown): val is Function;
+export declare function isPlainObject(val: unknown): val is Record;
+export declare function isPromise(val: unknown): val is Promise;
+export declare function isDef(value: unknown): boolean;
+export declare function isObj(x: unknown): x is Record;
+export declare function isNumber(value: string): boolean;
+export declare function isBoolean(value: unknown): value is boolean;
+export declare function isImageUrl(url: string): boolean;
+export declare function isVideoUrl(url: string): boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.js
new file mode 100644
index 0000000..169e796
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/validator.js
@@ -0,0 +1,43 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isVideoUrl = exports.isImageUrl = exports.isBoolean = exports.isNumber = exports.isObj = exports.isDef = exports.isPromise = exports.isPlainObject = exports.isFunction = void 0;
+// eslint-disable-next-line @typescript-eslint/ban-types
+function isFunction(val) {
+ return typeof val === 'function';
+}
+exports.isFunction = isFunction;
+function isPlainObject(val) {
+ return val !== null && typeof val === 'object' && !Array.isArray(val);
+}
+exports.isPlainObject = isPlainObject;
+function isPromise(val) {
+ return isPlainObject(val) && isFunction(val.then) && isFunction(val.catch);
+}
+exports.isPromise = isPromise;
+function isDef(value) {
+ return value !== undefined && value !== null;
+}
+exports.isDef = isDef;
+function isObj(x) {
+ var type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+exports.isObj = isObj;
+function isNumber(value) {
+ return /^\d+(\.\d+)?$/.test(value);
+}
+exports.isNumber = isNumber;
+function isBoolean(value) {
+ return typeof value === 'boolean';
+}
+exports.isBoolean = isBoolean;
+var IMAGE_REGEXP = /\.(jpeg|jpg|gif|png|svg|webp|jfif|bmp|dpg)/i;
+var VIDEO_REGEXP = /\.(mp4|mpg|mpeg|dat|asf|avi|rm|rmvb|mov|wmv|flv|mkv)/i;
+function isImageUrl(url) {
+ return IMAGE_REGEXP.test(url);
+}
+exports.isImageUrl = isImageUrl;
+function isVideoUrl(url) {
+ return VIDEO_REGEXP.test(url);
+}
+exports.isVideoUrl = isVideoUrl;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.d.ts
new file mode 100644
index 0000000..3393221
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.d.ts
@@ -0,0 +1,15 @@
+///
+interface WxWorkSystemInfo extends WechatMiniprogram.SystemInfo {
+ environment?: 'wxwork';
+}
+interface SystemInfo extends WxWorkSystemInfo, WechatMiniprogram.SystemInfo {
+}
+export declare function getSystemInfoSync(): SystemInfo;
+export declare function canIUseModel(): boolean;
+export declare function canIUseFormFieldButton(): boolean;
+export declare function canIUseAnimate(): boolean;
+export declare function canIUseGroupSetData(): boolean;
+export declare function canIUseNextTick(): boolean;
+export declare function canIUseCanvas2d(): boolean;
+export declare function canIUseGetUserProfile(): boolean;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.js
new file mode 100644
index 0000000..5937008
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/common/version.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.canIUseGetUserProfile = exports.canIUseCanvas2d = exports.canIUseNextTick = exports.canIUseGroupSetData = exports.canIUseAnimate = exports.canIUseFormFieldButton = exports.canIUseModel = exports.getSystemInfoSync = void 0;
+var systemInfo;
+function getSystemInfoSync() {
+ if (systemInfo == null) {
+ systemInfo = wx.getSystemInfoSync();
+ }
+ return systemInfo;
+}
+exports.getSystemInfoSync = getSystemInfoSync;
+function compareVersion(v1, v2) {
+ v1 = v1.split('.');
+ v2 = v2.split('.');
+ var len = Math.max(v1.length, v2.length);
+ while (v1.length < len) {
+ v1.push('0');
+ }
+ while (v2.length < len) {
+ v2.push('0');
+ }
+ for (var i = 0; i < len; i++) {
+ var num1 = parseInt(v1[i], 10);
+ var num2 = parseInt(v2[i], 10);
+ if (num1 > num2) {
+ return 1;
+ }
+ if (num1 < num2) {
+ return -1;
+ }
+ }
+ return 0;
+}
+function gte(version) {
+ var system = getSystemInfoSync();
+ return compareVersion(system.SDKVersion, version) >= 0;
+}
+function canIUseModel() {
+ return gte('2.9.3');
+}
+exports.canIUseModel = canIUseModel;
+function canIUseFormFieldButton() {
+ return gte('2.10.3');
+}
+exports.canIUseFormFieldButton = canIUseFormFieldButton;
+function canIUseAnimate() {
+ return gte('2.9.0');
+}
+exports.canIUseAnimate = canIUseAnimate;
+function canIUseGroupSetData() {
+ return gte('2.4.0');
+}
+exports.canIUseGroupSetData = canIUseGroupSetData;
+function canIUseNextTick() {
+ try {
+ return wx.canIUse('nextTick');
+ }
+ catch (e) {
+ return gte('2.7.1');
+ }
+}
+exports.canIUseNextTick = canIUseNextTick;
+function canIUseCanvas2d() {
+ return gte('2.9.0');
+}
+exports.canIUseCanvas2d = canIUseCanvas2d;
+function canIUseGetUserProfile() {
+ return !!wx.getUserProfile;
+}
+exports.canIUseGetUserProfile = canIUseGetUserProfile;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.js
new file mode 100644
index 0000000..21fb1c4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ themeVars: {
+ type: Object,
+ value: {},
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxml
new file mode 100644
index 0000000..3cfb461
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxs
new file mode 100644
index 0000000..7ca0203
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/config-provider/index.wxs
@@ -0,0 +1,29 @@
+/* eslint-disable */
+var object = require('../wxs/object.wxs');
+var style = require('../wxs/style.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+ .replace(getRegExp("^-"), '');
+
+ return newWord;
+}
+
+function mapThemeVarsToCSSVars(themeVars) {
+ var cssVars = {};
+ object.keys(themeVars).forEach(function (key) {
+ var cssVarsKey = '--' + kebabCase(key);
+ cssVars[cssVarsKey] = themeVars[key];
+ });
+
+ return style(cssVars);
+}
+
+module.exports = {
+ kebabCase: kebabCase,
+ mapThemeVarsToCSSVars: mapThemeVarsToCSSVars,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.js
new file mode 100644
index 0000000..afc780b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.js
@@ -0,0 +1,104 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+function simpleTick(fn) {
+ return setTimeout(fn, 30);
+}
+(0, component_1.VantComponent)({
+ props: {
+ useSlot: Boolean,
+ millisecond: Boolean,
+ time: {
+ type: Number,
+ observer: 'reset',
+ },
+ format: {
+ type: String,
+ value: 'HH:mm:ss',
+ },
+ autoStart: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ timeData: (0, utils_1.parseTimeData)(0),
+ formattedTime: '0',
+ },
+ destroyed: function () {
+ clearTimeout(this.tid);
+ this.tid = null;
+ },
+ methods: {
+ // 开始
+ start: function () {
+ if (this.counting) {
+ return;
+ }
+ this.counting = true;
+ this.endTime = Date.now() + this.remain;
+ this.tick();
+ },
+ // 暂停
+ pause: function () {
+ this.counting = false;
+ clearTimeout(this.tid);
+ },
+ // 重置
+ reset: function () {
+ this.pause();
+ this.remain = this.data.time;
+ this.setRemain(this.remain);
+ if (this.data.autoStart) {
+ this.start();
+ }
+ },
+ tick: function () {
+ if (this.data.millisecond) {
+ this.microTick();
+ }
+ else {
+ this.macroTick();
+ }
+ },
+ microTick: function () {
+ var _this = this;
+ this.tid = simpleTick(function () {
+ _this.setRemain(_this.getRemain());
+ if (_this.remain !== 0) {
+ _this.microTick();
+ }
+ });
+ },
+ macroTick: function () {
+ var _this = this;
+ this.tid = simpleTick(function () {
+ var remain = _this.getRemain();
+ if (!(0, utils_1.isSameSecond)(remain, _this.remain) || remain === 0) {
+ _this.setRemain(remain);
+ }
+ if (_this.remain !== 0) {
+ _this.macroTick();
+ }
+ });
+ },
+ getRemain: function () {
+ return Math.max(this.endTime - Date.now(), 0);
+ },
+ setRemain: function (remain) {
+ this.remain = remain;
+ var timeData = (0, utils_1.parseTimeData)(remain);
+ if (this.data.useSlot) {
+ this.$emit('change', timeData);
+ }
+ this.setData({
+ formattedTime: (0, utils_1.parseFormat)(this.data.format, timeData),
+ });
+ if (remain === 0) {
+ this.pause();
+ this.$emit('finish');
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxml
new file mode 100644
index 0000000..e206e16
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxml
@@ -0,0 +1,4 @@
+
+
+ {{ formattedTime }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxss
new file mode 100644
index 0000000..8b957f7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-count-down{color:var(--count-down-text-color,#323233);font-size:var(--count-down-font-size,14px);line-height:var(--count-down-line-height,20px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.d.ts
new file mode 100644
index 0000000..876a6c1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.d.ts
@@ -0,0 +1,10 @@
+export type TimeData = {
+ days: number;
+ hours: number;
+ minutes: number;
+ seconds: number;
+ milliseconds: number;
+};
+export declare function parseTimeData(time: number): TimeData;
+export declare function parseFormat(format: string, timeData: TimeData): string;
+export declare function isSameSecond(time1: number, time2: number): boolean;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.js
new file mode 100644
index 0000000..a7cfa5f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/count-down/utils.js
@@ -0,0 +1,64 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.isSameSecond = exports.parseFormat = exports.parseTimeData = void 0;
+function padZero(num, targetLength) {
+ if (targetLength === void 0) { targetLength = 2; }
+ var str = num + '';
+ while (str.length < targetLength) {
+ str = '0' + str;
+ }
+ return str;
+}
+var SECOND = 1000;
+var MINUTE = 60 * SECOND;
+var HOUR = 60 * MINUTE;
+var DAY = 24 * HOUR;
+function parseTimeData(time) {
+ var days = Math.floor(time / DAY);
+ var hours = Math.floor((time % DAY) / HOUR);
+ var minutes = Math.floor((time % HOUR) / MINUTE);
+ var seconds = Math.floor((time % MINUTE) / SECOND);
+ var milliseconds = Math.floor(time % SECOND);
+ return {
+ days: days,
+ hours: hours,
+ minutes: minutes,
+ seconds: seconds,
+ milliseconds: milliseconds,
+ };
+}
+exports.parseTimeData = parseTimeData;
+function parseFormat(format, timeData) {
+ var days = timeData.days;
+ var hours = timeData.hours, minutes = timeData.minutes, seconds = timeData.seconds, milliseconds = timeData.milliseconds;
+ if (format.indexOf('DD') === -1) {
+ hours += days * 24;
+ }
+ else {
+ format = format.replace('DD', padZero(days));
+ }
+ if (format.indexOf('HH') === -1) {
+ minutes += hours * 60;
+ }
+ else {
+ format = format.replace('HH', padZero(hours));
+ }
+ if (format.indexOf('mm') === -1) {
+ seconds += minutes * 60;
+ }
+ else {
+ format = format.replace('mm', padZero(minutes));
+ }
+ if (format.indexOf('ss') === -1) {
+ milliseconds += seconds * 1000;
+ }
+ else {
+ format = format.replace('ss', padZero(seconds));
+ }
+ return format.replace('SSS', padZero(milliseconds, 3));
+}
+exports.parseFormat = parseFormat;
+function isSameSecond(time1, time2) {
+ return Math.floor(time1 / 1000) === Math.floor(time2 / 1000);
+}
+exports.isSameSecond = isSameSecond;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.js
new file mode 100644
index 0000000..e30afef
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.js
@@ -0,0 +1,329 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) {
+ if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
+ if (ar || !(i in from)) {
+ if (!ar) ar = Array.prototype.slice.call(from, 0, i);
+ ar[i] = from[i];
+ }
+ }
+ return to.concat(ar || Array.prototype.slice.call(from));
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var shared_1 = require("../picker/shared");
+var currentYear = new Date().getFullYear();
+function isValidDate(date) {
+ return (0, validator_1.isDef)(date) && !isNaN(new Date(date).getTime());
+}
+function range(num, min, max) {
+ return Math.min(Math.max(num, min), max);
+}
+function padZero(val) {
+ return "00".concat(val).slice(-2);
+}
+function times(n, iteratee) {
+ var index = -1;
+ var result = Array(n < 0 ? 0 : n);
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+function getTrueValue(formattedValue) {
+ if (formattedValue === undefined) {
+ formattedValue = '1';
+ }
+ while (isNaN(parseInt(formattedValue, 10))) {
+ formattedValue = formattedValue.slice(1);
+ }
+ return parseInt(formattedValue, 10);
+}
+function getMonthEndDay(year, month) {
+ return 32 - new Date(year, month - 1, 32).getDate();
+}
+var defaultFormatter = function (type, value) { return value; };
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { value: {
+ type: null,
+ observer: 'updateValue',
+ }, filter: null, type: {
+ type: String,
+ value: 'datetime',
+ observer: 'updateValue',
+ }, showToolbar: {
+ type: Boolean,
+ value: true,
+ }, formatter: {
+ type: null,
+ value: defaultFormatter,
+ }, minDate: {
+ type: Number,
+ value: new Date(currentYear - 10, 0, 1).getTime(),
+ observer: 'updateValue',
+ }, maxDate: {
+ type: Number,
+ value: new Date(currentYear + 10, 11, 31).getTime(),
+ observer: 'updateValue',
+ }, minHour: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxHour: {
+ type: Number,
+ value: 23,
+ observer: 'updateValue',
+ }, minMinute: {
+ type: Number,
+ value: 0,
+ observer: 'updateValue',
+ }, maxMinute: {
+ type: Number,
+ value: 59,
+ observer: 'updateValue',
+ } }),
+ data: {
+ innerValue: Date.now(),
+ columns: [],
+ },
+ methods: {
+ updateValue: function () {
+ var _this = this;
+ var data = this.data;
+ var val = this.correctValue(data.value);
+ var isEqual = val === data.innerValue;
+ this.updateColumnValue(val).then(function () {
+ if (!isEqual) {
+ _this.$emit('input', val);
+ }
+ });
+ },
+ getPicker: function () {
+ if (this.picker == null) {
+ this.picker = this.selectComponent('.van-datetime-picker');
+ var picker_1 = this.picker;
+ var setColumnValues_1 = picker_1.setColumnValues;
+ picker_1.setColumnValues = function () {
+ var args = [];
+ for (var _i = 0; _i < arguments.length; _i++) {
+ args[_i] = arguments[_i];
+ }
+ return setColumnValues_1.apply(picker_1, __spreadArray(__spreadArray([], args, true), [false], false));
+ };
+ }
+ return this.picker;
+ },
+ updateColumns: function () {
+ var _a = this.data.formatter, formatter = _a === void 0 ? defaultFormatter : _a;
+ var results = this.getOriginColumns().map(function (column) { return ({
+ values: column.values.map(function (value) { return formatter(column.type, value); }),
+ }); });
+ return this.set({ columns: results });
+ },
+ getOriginColumns: function () {
+ var filter = this.data.filter;
+ var results = this.getRanges().map(function (_a) {
+ var type = _a.type, range = _a.range;
+ var values = times(range[1] - range[0] + 1, function (index) {
+ var value = range[0] + index;
+ return type === 'year' ? "".concat(value) : padZero(value);
+ });
+ if (filter) {
+ values = filter(type, values);
+ }
+ return { type: type, values: values };
+ });
+ return results;
+ },
+ getRanges: function () {
+ var data = this.data;
+ if (data.type === 'time') {
+ return [
+ {
+ type: 'hour',
+ range: [data.minHour, data.maxHour],
+ },
+ {
+ type: 'minute',
+ range: [data.minMinute, data.maxMinute],
+ },
+ ];
+ }
+ var _a = this.getBoundary('max', data.innerValue), maxYear = _a.maxYear, maxDate = _a.maxDate, maxMonth = _a.maxMonth, maxHour = _a.maxHour, maxMinute = _a.maxMinute;
+ var _b = this.getBoundary('min', data.innerValue), minYear = _b.minYear, minDate = _b.minDate, minMonth = _b.minMonth, minHour = _b.minHour, minMinute = _b.minMinute;
+ var result = [
+ {
+ type: 'year',
+ range: [minYear, maxYear],
+ },
+ {
+ type: 'month',
+ range: [minMonth, maxMonth],
+ },
+ {
+ type: 'day',
+ range: [minDate, maxDate],
+ },
+ {
+ type: 'hour',
+ range: [minHour, maxHour],
+ },
+ {
+ type: 'minute',
+ range: [minMinute, maxMinute],
+ },
+ ];
+ if (data.type === 'date')
+ result.splice(3, 2);
+ if (data.type === 'year-month')
+ result.splice(2, 3);
+ return result;
+ },
+ correctValue: function (value) {
+ var data = this.data;
+ // validate value
+ var isDateType = data.type !== 'time';
+ if (isDateType && !isValidDate(value)) {
+ value = data.minDate;
+ }
+ else if (!isDateType && !value) {
+ var minHour = data.minHour;
+ value = "".concat(padZero(minHour), ":00");
+ }
+ // time type
+ if (!isDateType) {
+ var _a = value.split(':'), hour = _a[0], minute = _a[1];
+ hour = padZero(range(hour, data.minHour, data.maxHour));
+ minute = padZero(range(minute, data.minMinute, data.maxMinute));
+ return "".concat(hour, ":").concat(minute);
+ }
+ // date type
+ value = Math.max(value, data.minDate);
+ value = Math.min(value, data.maxDate);
+ return value;
+ },
+ getBoundary: function (type, innerValue) {
+ var _a;
+ var value = new Date(innerValue);
+ var boundary = new Date(this.data["".concat(type, "Date")]);
+ var year = boundary.getFullYear();
+ var month = 1;
+ var date = 1;
+ var hour = 0;
+ var minute = 0;
+ if (type === 'max') {
+ month = 12;
+ date = getMonthEndDay(value.getFullYear(), value.getMonth() + 1);
+ hour = 23;
+ minute = 59;
+ }
+ if (value.getFullYear() === year) {
+ month = boundary.getMonth() + 1;
+ if (value.getMonth() + 1 === month) {
+ date = boundary.getDate();
+ if (value.getDate() === date) {
+ hour = boundary.getHours();
+ if (value.getHours() === hour) {
+ minute = boundary.getMinutes();
+ }
+ }
+ }
+ }
+ return _a = {},
+ _a["".concat(type, "Year")] = year,
+ _a["".concat(type, "Month")] = month,
+ _a["".concat(type, "Date")] = date,
+ _a["".concat(type, "Hour")] = hour,
+ _a["".concat(type, "Minute")] = minute,
+ _a;
+ },
+ onCancel: function () {
+ this.$emit('cancel');
+ },
+ onConfirm: function () {
+ this.$emit('confirm', this.data.innerValue);
+ },
+ onChange: function () {
+ var _this = this;
+ var data = this.data;
+ var value;
+ var picker = this.getPicker();
+ var originColumns = this.getOriginColumns();
+ if (data.type === 'time') {
+ var indexes = picker.getIndexes();
+ value = "".concat(+originColumns[0].values[indexes[0]], ":").concat(+originColumns[1]
+ .values[indexes[1]]);
+ }
+ else {
+ var indexes = picker.getIndexes();
+ var values = indexes.map(function (value, index) { return originColumns[index].values[value]; });
+ var year = getTrueValue(values[0]);
+ var month = getTrueValue(values[1]);
+ var maxDate = getMonthEndDay(year, month);
+ var date = getTrueValue(values[2]);
+ if (data.type === 'year-month') {
+ date = 1;
+ }
+ date = date > maxDate ? maxDate : date;
+ var hour = 0;
+ var minute = 0;
+ if (data.type === 'datetime') {
+ hour = getTrueValue(values[3]);
+ minute = getTrueValue(values[4]);
+ }
+ value = new Date(year, month - 1, date, hour, minute);
+ }
+ value = this.correctValue(value);
+ this.updateColumnValue(value).then(function () {
+ _this.$emit('input', value);
+ _this.$emit('change', picker);
+ });
+ },
+ updateColumnValue: function (value) {
+ var _this = this;
+ var values = [];
+ var type = this.data.type;
+ var formatter = this.data.formatter || defaultFormatter;
+ var picker = this.getPicker();
+ if (type === 'time') {
+ var pair = value.split(':');
+ values = [formatter('hour', pair[0]), formatter('minute', pair[1])];
+ }
+ else {
+ var date = new Date(value);
+ values = [
+ formatter('year', "".concat(date.getFullYear())),
+ formatter('month', padZero(date.getMonth() + 1)),
+ ];
+ if (type === 'date') {
+ values.push(formatter('day', padZero(date.getDate())));
+ }
+ if (type === 'datetime') {
+ values.push(formatter('day', padZero(date.getDate())), formatter('hour', padZero(date.getHours())), formatter('minute', padZero(date.getMinutes())));
+ }
+ }
+ return this.set({ innerValue: value })
+ .then(function () { return _this.updateColumns(); })
+ .then(function () { return picker.setValues(values); });
+ },
+ },
+ created: function () {
+ var _this = this;
+ var innerValue = this.correctValue(this.data.value);
+ this.updateColumnValue(innerValue).then(function () {
+ _this.$emit('input', innerValue);
+ });
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.json
new file mode 100644
index 0000000..a778e91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-picker": "../picker/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxml
new file mode 100644
index 0000000..ade2202
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxml
@@ -0,0 +1,16 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxss
new file mode 100644
index 0000000..99694d6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/datetime-picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.d.ts
new file mode 100644
index 0000000..d0554f6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.d.ts
@@ -0,0 +1,28 @@
+///
+interface VantComponentInstance {
+ parent: WechatMiniprogram.Component.TrivialInstance;
+ children: WechatMiniprogram.Component.TrivialInstance[];
+ index: number;
+ $emit: (name: string, detail?: unknown, options?: WechatMiniprogram.Component.TriggerEventOption) => void;
+}
+export type VantComponentOptions = {
+ data?: Data;
+ field?: boolean;
+ classes?: string[];
+ mixins?: string[];
+ props?: Props;
+ relation?: {
+ relations: Record;
+ mixin: string;
+ };
+ watch?: Record any>;
+ methods?: Methods;
+ beforeCreate?: () => void;
+ created?: () => void;
+ mounted?: () => void;
+ destroyed?: () => void;
+} & ThisType, Props, Methods> & Record>;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/definitions/index.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.d.ts
new file mode 100644
index 0000000..db2da5f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.d.ts
@@ -0,0 +1,55 @@
+///
+///
+export type Action = 'confirm' | 'cancel' | 'overlay';
+type DialogContext = WechatMiniprogram.Page.TrivialInstance | WechatMiniprogram.Component.TrivialInstance;
+interface DialogOptions {
+ lang?: string;
+ show?: boolean;
+ title?: string;
+ width?: string | number | null;
+ zIndex?: number;
+ theme?: string;
+ context?: (() => DialogContext) | DialogContext;
+ message?: string;
+ overlay?: boolean;
+ selector?: string;
+ ariaLabel?: string;
+ /**
+ * @deprecated use custom-class instead
+ */
+ className?: string;
+ customStyle?: string;
+ transition?: string;
+ /**
+ * @deprecated use beforeClose instead
+ */
+ asyncClose?: boolean;
+ beforeClose?: null | ((action: Action) => Promise | void);
+ businessId?: number;
+ sessionFrom?: string;
+ overlayStyle?: string;
+ appParameter?: string;
+ messageAlign?: string;
+ sendMessageImg?: string;
+ showMessageCard?: boolean;
+ sendMessagePath?: string;
+ sendMessageTitle?: string;
+ confirmButtonText?: string;
+ cancelButtonText?: string;
+ showConfirmButton?: boolean;
+ showCancelButton?: boolean;
+ closeOnClickOverlay?: boolean;
+ confirmButtonOpenType?: string;
+}
+declare const Dialog: {
+ (options: DialogOptions): Promise;
+ alert(options: DialogOptions): Promise;
+ confirm(options: DialogOptions): Promise;
+ close(): void;
+ stopLoading(): void;
+ currentOptions: DialogOptions;
+ defaultOptions: DialogOptions;
+ setDefaultOptions(options: DialogOptions): void;
+ resetDefaultOptions(): void;
+};
+export default Dialog;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.js
new file mode 100644
index 0000000..400f4f1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/dialog.js
@@ -0,0 +1,92 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var queue = [];
+var defaultOptions = {
+ show: false,
+ title: '',
+ width: null,
+ theme: 'default',
+ message: '',
+ zIndex: 100,
+ overlay: true,
+ selector: '#van-dialog',
+ className: '',
+ asyncClose: false,
+ beforeClose: null,
+ transition: 'scale',
+ customStyle: '',
+ messageAlign: '',
+ overlayStyle: '',
+ confirmButtonText: '确认',
+ cancelButtonText: '取消',
+ showConfirmButton: true,
+ showCancelButton: false,
+ closeOnClickOverlay: false,
+ confirmButtonOpenType: '',
+};
+var currentOptions = __assign({}, defaultOptions);
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+var Dialog = function (options) {
+ options = __assign(__assign({}, currentOptions), options);
+ return new Promise(function (resolve, reject) {
+ var context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ var dialog = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (dialog) {
+ dialog.setData(__assign({ callback: function (action, instance) {
+ action === 'confirm' ? resolve(instance) : reject(instance);
+ } }, options));
+ wx.nextTick(function () {
+ dialog.setData({ show: true });
+ });
+ queue.push(dialog);
+ }
+ else {
+ console.warn('未找到 van-dialog 节点,请确认 selector 及 context 是否正确');
+ }
+ });
+};
+Dialog.alert = function (options) { return Dialog(options); };
+Dialog.confirm = function (options) {
+ return Dialog(__assign({ showCancelButton: true }, options));
+};
+Dialog.close = function () {
+ queue.forEach(function (dialog) {
+ dialog.close();
+ });
+ queue = [];
+};
+Dialog.stopLoading = function () {
+ queue.forEach(function (dialog) {
+ dialog.stopLoading();
+ });
+};
+Dialog.currentOptions = currentOptions;
+Dialog.defaultOptions = defaultOptions;
+Dialog.setDefaultOptions = function (options) {
+ currentOptions = __assign(__assign({}, currentOptions), options);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+ Dialog.currentOptions = currentOptions;
+};
+Dialog.resetDefaultOptions();
+exports.default = Dialog;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.js
new file mode 100644
index 0000000..b0acfa0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.js
@@ -0,0 +1,135 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ mixins: [button_1.button],
+ classes: ['cancle-button-class', 'confirm-button-class'],
+ props: {
+ show: {
+ type: Boolean,
+ observer: function (show) {
+ !show && this.stopLoading();
+ },
+ },
+ title: String,
+ message: String,
+ theme: {
+ type: String,
+ value: 'default',
+ },
+ confirmButtonId: String,
+ className: String,
+ customStyle: String,
+ asyncClose: Boolean,
+ messageAlign: String,
+ beforeClose: null,
+ overlayStyle: String,
+ useSlot: Boolean,
+ useTitleSlot: Boolean,
+ useConfirmButtonSlot: Boolean,
+ useCancelButtonSlot: Boolean,
+ showCancelButton: Boolean,
+ closeOnClickOverlay: Boolean,
+ confirmButtonOpenType: String,
+ width: null,
+ zIndex: {
+ type: Number,
+ value: 2000,
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonColor: {
+ type: String,
+ value: color_1.RED,
+ },
+ cancelButtonColor: {
+ type: String,
+ value: color_1.GRAY,
+ },
+ showConfirmButton: {
+ type: Boolean,
+ value: true,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ transition: {
+ type: String,
+ value: 'scale',
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ callback: (function () { }),
+ },
+ methods: {
+ onConfirm: function () {
+ this.handleAction('confirm');
+ },
+ onCancel: function () {
+ this.handleAction('cancel');
+ },
+ onClickOverlay: function () {
+ this.close('overlay');
+ },
+ close: function (action) {
+ var _this = this;
+ this.setData({ show: false });
+ wx.nextTick(function () {
+ _this.$emit('close', action);
+ var callback = _this.data.callback;
+ if (callback) {
+ callback(action, _this);
+ }
+ });
+ },
+ stopLoading: function () {
+ this.setData({
+ loading: {
+ confirm: false,
+ cancel: false,
+ },
+ });
+ },
+ handleAction: function (action) {
+ var _a;
+ var _this = this;
+ this.$emit(action, { dialog: this });
+ var _b = this.data, asyncClose = _b.asyncClose, beforeClose = _b.beforeClose;
+ if (!asyncClose && !beforeClose) {
+ this.close(action);
+ return;
+ }
+ this.setData((_a = {},
+ _a["loading.".concat(action)] = true,
+ _a));
+ if (beforeClose) {
+ (0, utils_1.toPromise)(beforeClose(action)).then(function (value) {
+ if (value) {
+ _this.close(action);
+ }
+ else {
+ _this.stopLoading();
+ }
+ });
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.json
new file mode 100644
index 0000000..43417fc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-button": "../button/index",
+ "van-goods-action": "../goods-action/index",
+ "van-goods-action-button": "../goods-action-button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxml
new file mode 100644
index 0000000..a1d8e3c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxml
@@ -0,0 +1,125 @@
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+ {{ cancelButtonText }}
+
+
+ {{ confirmButtonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxss
new file mode 100644
index 0000000..507a789
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dialog/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dialog{background-color:var(--dialog-background-color,#fff);border-radius:var(--dialog-border-radius,16px);font-size:var(--dialog-font-size,16px);overflow:hidden;top:45%!important;width:var(--dialog-width,320px)}@media (max-width:321px){.van-dialog{width:var(--dialog-small-screen-width,90%)}}.van-dialog__header{font-weight:var(--dialog-header-font-weight,500);line-height:var(--dialog-header-line-height,24px);padding-top:var(--dialog-header-padding-top,24px);text-align:center}.van-dialog__header--isolated{padding:var(--dialog-header-isolated-padding,24px 0)}.van-dialog__message{-webkit-overflow-scrolling:touch;font-size:var(--dialog-message-font-size,14px);line-height:var(--dialog-message-line-height,20px);max-height:var(--dialog-message-max-height,60vh);overflow-y:auto;padding:var(--dialog-message-padding,24px);text-align:center}.van-dialog__message-text{word-wrap:break-word}.van-dialog__message--hasTitle{color:var(--dialog-has-title-message-text-color,#646566);padding-top:var(--dialog-has-title-message-padding-top,8px)}.van-dialog__message--round-button{color:#323233;padding-bottom:16px}.van-dialog__message--left{text-align:left}.van-dialog__message--right{text-align:right}.van-dialog__message--justify{text-align:justify}.van-dialog__footer{display:flex}.van-dialog__footer--round-button{padding:8px 24px 16px!important;position:relative!important}.van-dialog__button{flex:1}.van-dialog__cancel,.van-dialog__confirm{border:0!important}.van-dialog-bounce-enter{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-dialog-bounce-leave-active{opacity:0;transform:translate3d(-50%,-50%,0) scale(.9)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.js
new file mode 100644
index 0000000..5c63844
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.js
@@ -0,0 +1,14 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ dashed: Boolean,
+ hairline: Boolean,
+ contentPosition: String,
+ fontSize: String,
+ borderColor: String,
+ textColor: String,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxml
new file mode 100644
index 0000000..f6a5a45
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxml
@@ -0,0 +1,9 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxs
new file mode 100644
index 0000000..215b14f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ 'border-color': data.borderColor,
+ color: data.textColor,
+ 'font-size': addUnit(data.fontSize),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxss
new file mode 100644
index 0000000..e91dc44
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/divider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-divider{align-items:center;border:0 solid var(--divider-border-color,#ebedf0);color:var(--divider-text-color,#969799);display:flex;font-size:var(--divider-font-size,14px);line-height:var(--divider-line-height,24px);margin:var(--divider-margin,16px 0)}.van-divider:after,.van-divider:before{border-color:inherit;border-style:inherit;border-width:1px 0 0;box-sizing:border-box;display:block;flex:1;height:1px}.van-divider:before{content:""}.van-divider--hairline:after,.van-divider--hairline:before{transform:scaleY(.5)}.van-divider--dashed{border-style:dashed}.van-divider--center:before,.van-divider--left:before,.van-divider--right:before{margin-right:var(--divider-content-padding,16px)}.van-divider--center:after,.van-divider--left:after,.van-divider--right:after{content:"";margin-left:var(--divider-content-padding,16px)}.van-divider--left:before{max-width:var(--divider-content-left-width,10%)}.van-divider--right:after{max-width:var(--divider-content-right-width,10%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.js
new file mode 100644
index 0000000..826c26a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.js
@@ -0,0 +1,136 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['item-title-class'],
+ field: true,
+ relation: (0, relation_1.useParent)('dropdown-menu', function () {
+ this.updateDataFromParent();
+ }),
+ props: {
+ value: {
+ type: null,
+ observer: 'rerender',
+ },
+ title: {
+ type: String,
+ observer: 'rerender',
+ },
+ disabled: Boolean,
+ titleClass: {
+ type: String,
+ observer: 'rerender',
+ },
+ options: {
+ type: Array,
+ value: [],
+ observer: 'rerender',
+ },
+ popupStyle: String,
+ useBeforeToggle: {
+ type: Boolean,
+ value: false,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ transition: true,
+ showPopup: false,
+ showWrapper: false,
+ displayTitle: '',
+ safeAreaTabBar: false,
+ },
+ methods: {
+ rerender: function () {
+ var _this = this;
+ wx.nextTick(function () {
+ var _a;
+ (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.updateItemListData();
+ });
+ },
+ updateDataFromParent: function () {
+ if (this.parent) {
+ var _a = this.parent.data, overlay = _a.overlay, duration = _a.duration, activeColor = _a.activeColor, closeOnClickOverlay = _a.closeOnClickOverlay, direction = _a.direction, safeAreaTabBar = _a.safeAreaTabBar;
+ this.setData({
+ overlay: overlay,
+ duration: duration,
+ activeColor: activeColor,
+ closeOnClickOverlay: closeOnClickOverlay,
+ direction: direction,
+ safeAreaTabBar: safeAreaTabBar,
+ });
+ }
+ },
+ onOpen: function () {
+ this.$emit('open');
+ },
+ onOpened: function () {
+ this.$emit('opened');
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ onClosed: function () {
+ this.$emit('closed');
+ this.setData({ showWrapper: false });
+ },
+ onOptionTap: function (event) {
+ var option = event.currentTarget.dataset.option;
+ var value = option.value;
+ var shouldEmitChange = this.data.value !== value;
+ this.setData({ showPopup: false, value: value });
+ this.$emit('close');
+ this.rerender();
+ if (shouldEmitChange) {
+ this.$emit('change', value);
+ }
+ },
+ toggle: function (show, options) {
+ var _this = this;
+ if (options === void 0) { options = {}; }
+ var showPopup = this.data.showPopup;
+ if (typeof show !== 'boolean') {
+ show = !showPopup;
+ }
+ if (show === showPopup) {
+ return;
+ }
+ this.onBeforeToggle(show).then(function (status) {
+ var _a;
+ if (!status) {
+ return;
+ }
+ _this.setData({
+ transition: !options.immediate,
+ showPopup: show,
+ });
+ if (show) {
+ (_a = _this.parent) === null || _a === void 0 ? void 0 : _a.getChildWrapperStyle().then(function (wrapperStyle) {
+ _this.setData({ wrapperStyle: wrapperStyle, showWrapper: true });
+ _this.rerender();
+ });
+ }
+ else {
+ _this.rerender();
+ }
+ });
+ },
+ onBeforeToggle: function (status) {
+ var _this = this;
+ var useBeforeToggle = this.data.useBeforeToggle;
+ if (!useBeforeToggle) {
+ return Promise.resolve(true);
+ }
+ return new Promise(function (resolve) {
+ _this.$emit('before-toggle', {
+ status: status,
+ callback: function (value) { return resolve(value); },
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.json
new file mode 100644
index 0000000..88d5409
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxml
new file mode 100644
index 0000000..63904f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxml
@@ -0,0 +1,50 @@
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxss
new file mode 100644
index 0000000..80505e9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-item{left:0;overflow:hidden;position:fixed;right:0}.van-dropdown-item__option{text-align:left}.van-dropdown-item__option--active .van-dropdown-item__icon,.van-dropdown-item__option--active .van-dropdown-item__title{color:var(--dropdown-menu-option-active-color,#ee0a24)}.van-dropdown-item--up{top:0}.van-dropdown-item--down{bottom:0}.van-dropdown-item__icon{display:block;line-height:inherit}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.d.ts
new file mode 100644
index 0000000..774eb4c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.d.ts
@@ -0,0 +1,5 @@
+export interface Option {
+ text: string;
+ value: string | number;
+ icon: string;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-item/shared.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.js
new file mode 100644
index 0000000..aed2921
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.js
@@ -0,0 +1,122 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+var ARRAY = [];
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['title-class'],
+ relation: (0, relation_1.useChildren)('dropdown-item', function () {
+ this.updateItemListData();
+ }),
+ props: {
+ activeColor: {
+ type: String,
+ observer: 'updateChildrenData',
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ zIndex: {
+ type: Number,
+ value: 10,
+ },
+ duration: {
+ type: Number,
+ value: 200,
+ observer: 'updateChildrenData',
+ },
+ direction: {
+ type: String,
+ value: 'down',
+ observer: 'updateChildrenData',
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildrenData',
+ },
+ closeOnClickOutside: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ itemListData: [],
+ },
+ beforeCreate: function () {
+ var windowHeight = (0, utils_1.getSystemInfoSync)().windowHeight;
+ this.windowHeight = windowHeight;
+ ARRAY.push(this);
+ },
+ destroyed: function () {
+ var _this = this;
+ ARRAY = ARRAY.filter(function (item) { return item !== _this; });
+ },
+ methods: {
+ updateItemListData: function () {
+ this.setData({
+ itemListData: this.children.map(function (child) { return child.data; }),
+ });
+ },
+ updateChildrenData: function () {
+ this.children.forEach(function (child) {
+ child.updateDataFromParent();
+ });
+ },
+ toggleItem: function (active) {
+ this.children.forEach(function (item, index) {
+ var showPopup = item.data.showPopup;
+ if (index === active) {
+ item.toggle();
+ }
+ else if (showPopup) {
+ item.toggle(false, { immediate: true });
+ }
+ });
+ },
+ close: function () {
+ this.children.forEach(function (child) {
+ child.toggle(false, { immediate: true });
+ });
+ },
+ getChildWrapperStyle: function () {
+ var _this = this;
+ var _a = this.data, zIndex = _a.zIndex, direction = _a.direction;
+ return (0, utils_1.getRect)(this, '.van-dropdown-menu').then(function (rect) {
+ var _a = rect.top, top = _a === void 0 ? 0 : _a, _b = rect.bottom, bottom = _b === void 0 ? 0 : _b;
+ var offset = direction === 'down' ? bottom : _this.windowHeight - top;
+ var wrapperStyle = "z-index: ".concat(zIndex, ";");
+ if (direction === 'down') {
+ wrapperStyle += "top: ".concat((0, utils_1.addUnit)(offset), ";");
+ }
+ else {
+ wrapperStyle += "bottom: ".concat((0, utils_1.addUnit)(offset), ";");
+ }
+ return wrapperStyle;
+ });
+ },
+ onTitleTap: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var child = this.children[index];
+ if (!child.data.disabled) {
+ ARRAY.forEach(function (menuItem) {
+ if (menuItem &&
+ menuItem.data.closeOnClickOutside &&
+ menuItem !== _this) {
+ menuItem.close();
+ }
+ });
+ this.toggleItem(index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxml
new file mode 100644
index 0000000..ec165a9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxs
new file mode 100644
index 0000000..6538854
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxs
@@ -0,0 +1,16 @@
+/* eslint-disable */
+function displayTitle(item) {
+ if (item.title) {
+ return item.title;
+ }
+
+ var match = item.options.filter(function(option) {
+ return option.value === item.value;
+ });
+ var displayTitle = match.length ? match[0].text : '';
+ return displayTitle;
+}
+
+module.exports = {
+ displayTitle: displayTitle
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxss
new file mode 100644
index 0000000..dba000e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/dropdown-menu/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-dropdown-menu{background-color:var(--dropdown-menu-background-color,#fff);box-shadow:var(--dropdown-menu-box-shadow,0 2px 12px hsla(210,1%,40%,.12));display:flex;height:var(--dropdown-menu-height,50px);-webkit-user-select:none;user-select:none}.van-dropdown-menu__item{align-items:center;display:flex;flex:1;justify-content:center;min-width:0}.van-dropdown-menu__item:active{opacity:.7}.van-dropdown-menu__item--disabled:active{opacity:1}.van-dropdown-menu__item--disabled .van-dropdown-menu__title{color:var(--dropdown-menu-title-disabled-text-color,#969799)}.van-dropdown-menu__title{box-sizing:border-box;color:var(--dropdown-menu-title-text-color,#323233);font-size:var(--dropdown-menu-title-font-size,15px);line-height:var(--dropdown-menu-title-line-height,18px);max-width:100%;padding:var(--dropdown-menu-title-padding,0 24px 0 8px);position:relative}.van-dropdown-menu__title:after{border-color:transparent transparent currentcolor currentcolor;border-style:solid;border-width:3px;content:"";margin-top:-5px;opacity:.8;position:absolute;right:11px;top:50%;transform:rotate(-45deg)}.van-dropdown-menu__title--active{color:var(--dropdown-menu-title-active-text-color,#ee0a24)}.van-dropdown-menu__title--down:after{margin-top:-1px;transform:rotate(135deg)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.js
new file mode 100644
index 0000000..755e638
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.js
@@ -0,0 +1,12 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ description: String,
+ image: {
+ type: String,
+ value: 'default',
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxml
new file mode 100644
index 0000000..9c7b719
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ description }}
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxs
new file mode 100644
index 0000000..cf92ece
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var PRESETS = ['error', 'search', 'default', 'network'];
+
+function imageUrl(image) {
+ if (PRESETS.indexOf(image) !== -1) {
+ return 'https://img.yzcdn.cn/vant/empty-image-' + image + '.png';
+ }
+
+ return image;
+}
+
+module.exports = {
+ imageUrl: imageUrl,
+};
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxss
new file mode 100644
index 0000000..0fb74fe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/empty/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-empty{align-items:center;box-sizing:border-box;display:flex;flex-direction:column;justify-content:center;padding:32px 0}.van-empty__image{height:160px;width:160px}.van-empty__image:empty{display:none}.van-empty__image__img{height:100%;width:100%}.van-empty__image:not(:empty)+.van-empty__image{display:none}.van-empty__description{color:#969799;font-size:14px;line-height:20px;margin-top:16px;padding:0 60px}.van-empty__description:empty,.van-empty__description:not(:empty)+.van-empty__description{display:none}.van-empty__bottom{margin-top:24px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.js
new file mode 100644
index 0000000..c20d266
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.js
@@ -0,0 +1,137 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var props_1 = require("./props");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['input-class', 'right-icon-class', 'label-class'],
+ props: __assign(__assign(__assign(__assign({}, props_1.commonProps), props_1.inputProps), props_1.textareaProps), { size: String, icon: String, label: String, error: Boolean, center: Boolean, isLink: Boolean, leftIcon: String, rightIcon: String, autosize: null, required: Boolean, iconClass: String, clickable: Boolean, inputAlign: String, customStyle: String, errorMessage: String, arrowDirection: String, showWordLimit: Boolean, errorMessageAlign: String, readonly: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearable: {
+ type: Boolean,
+ observer: 'setShowClear',
+ }, clearTrigger: {
+ type: String,
+ value: 'focus',
+ }, border: {
+ type: Boolean,
+ value: true,
+ }, titleWidth: {
+ type: String,
+ value: '6.2em',
+ }, clearIcon: {
+ type: String,
+ value: 'clear',
+ }, extraEventParams: {
+ type: Boolean,
+ value: false,
+ } }),
+ data: {
+ focused: false,
+ innerValue: '',
+ showClear: false,
+ },
+ created: function () {
+ this.value = this.data.value;
+ this.setData({ innerValue: this.value });
+ },
+ methods: {
+ formatValue: function (value) {
+ var maxlength = this.data.maxlength;
+ if (maxlength !== -1 && value.length > maxlength) {
+ return value.slice(0, maxlength);
+ }
+ return value;
+ },
+ onInput: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ var formatValue = this.formatValue(value);
+ this.value = formatValue;
+ this.setShowClear();
+ return this.emitChange(__assign(__assign({}, event.detail), { value: formatValue }));
+ },
+ onFocus: function (event) {
+ this.focused = true;
+ this.setShowClear();
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ this.focused = false;
+ this.setShowClear();
+ this.$emit('blur', event.detail);
+ },
+ onClickIcon: function () {
+ this.$emit('click-icon');
+ },
+ onClickInput: function (event) {
+ this.$emit('click-input', event.detail);
+ },
+ onClear: function () {
+ var _this = this;
+ this.setData({ innerValue: '' });
+ this.value = '';
+ this.setShowClear();
+ (0, utils_1.nextTick)(function () {
+ _this.emitChange({ value: '' });
+ _this.$emit('clear', '');
+ });
+ },
+ onConfirm: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ this.value = value;
+ this.setShowClear();
+ this.$emit('confirm', value);
+ },
+ setValue: function (value) {
+ this.value = value;
+ this.setShowClear();
+ if (value === '') {
+ this.setData({ innerValue: '' });
+ }
+ this.emitChange({ value: value });
+ },
+ onLineChange: function (event) {
+ this.$emit('linechange', event.detail);
+ },
+ onKeyboardHeightChange: function (event) {
+ this.$emit('keyboardheightchange', event.detail);
+ },
+ emitChange: function (detail) {
+ var extraEventParams = this.data.extraEventParams;
+ this.setData({ value: detail.value });
+ var result;
+ var data = extraEventParams
+ ? __assign(__assign({}, detail), { callback: function (data) {
+ result = data;
+ } }) : detail.value;
+ this.$emit('input', data);
+ this.$emit('change', data);
+ return result;
+ },
+ setShowClear: function () {
+ var _a = this.data, clearable = _a.clearable, readonly = _a.readonly, clearTrigger = _a.clearTrigger;
+ var _b = this, focused = _b.focused, value = _b.value;
+ var showClear = false;
+ if (clearable && !readonly) {
+ var hasValue = !!value;
+ var trigger = clearTrigger === 'always' || (clearTrigger === 'focus' && focused);
+ showClear = hasValue && trigger;
+ }
+ this.setData({ showClear: showClear });
+ },
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.json
new file mode 100644
index 0000000..5906c50
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxml
new file mode 100644
index 0000000..6018993
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxml
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxs
new file mode 100644
index 0000000..78575b9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function inputStyle(autosize) {
+ if (autosize && autosize.constructor === 'Object') {
+ return style({
+ 'min-height': addUnit(autosize.minHeight),
+ 'max-height': addUnit(autosize.maxHeight),
+ });
+ }
+
+ return '';
+}
+
+module.exports = {
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxss
new file mode 100644
index 0000000..5f7d306
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-field{--cell-icon-size:var(--field-icon-size,16px)}.van-field__label{color:var(--field-label-color,#646566)}.van-field__label--disabled{color:var(--field-disabled-text-color,#c8c9cc)}.van-field__body{align-items:center;display:flex}.van-field__body--textarea{box-sizing:border-box;line-height:1.2em;min-height:var(--cell-line-height,24px);padding:3.6px 0}.van-field__control:empty+.van-field__control{display:block}.van-field__control{background-color:initial;border:0;box-sizing:border-box;color:var(--field-input-text-color,#323233);display:none;height:var(--cell-line-height,24px);line-height:inherit;margin:0;min-height:var(--cell-line-height,24px);padding:0;position:relative;resize:none;text-align:left;width:100%}.van-field__control:empty{display:none}.van-field__control--textarea{height:var(--field-text-area-min-height,18px);min-height:var(--field-text-area-min-height,18px)}.van-field__control--error{color:var(--field-input-error-text-color,#ee0a24)}.van-field__control--disabled{background-color:initial;color:var(--field-input-disabled-text-color,#c8c9cc);opacity:1}.van-field__control--center{text-align:center}.van-field__control--right{text-align:right}.van-field__control--custom{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__placeholder{color:var(--field-placeholder-text-color,#c8c9cc);left:0;pointer-events:none;position:absolute;right:0;top:0}.van-field__placeholder--error{color:var(--field-error-message-color,#ee0a24)}.van-field__icon-root{align-items:center;display:flex;min-height:var(--cell-line-height,24px)}.van-field__clear-root,.van-field__icon-container{line-height:inherit;margin-right:calc(var(--padding-xs, 8px)*-1);padding:0 var(--padding-xs,8px);vertical-align:middle}.van-field__button,.van-field__clear-root,.van-field__icon-container{flex-shrink:0}.van-field__clear-root{color:var(--field-clear-icon-color,#c8c9cc);font-size:var(--field-clear-icon-size,16px)}.van-field__icon-container{color:var(--field-icon-container-color,#969799);font-size:var(--field-icon-size,16px)}.van-field__icon-container:empty{display:none}.van-field__button{padding-left:var(--padding-xs,8px)}.van-field__button:empty{display:none}.van-field__error-message{color:var(--field-error-message-color,#ee0a24);display:block;font-size:var(--field-error-message-text-font-size,12px);text-align:left}.van-field__error-message--center{text-align:center}.van-field__error-message--right{text-align:right}.van-field__word-limit{color:var(--field-word-limit-color,#646566);font-size:var(--field-word-limit-font-size,12px);line-height:var(--field-word-limit-line-height,16px);margin-top:var(--padding-base,4px);text-align:right}.van-field__word-num{display:inline}.van-field__word-num--full{color:var(--field-word-num-full-color,#ee0a24)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/input.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/input.wxml
new file mode 100644
index 0000000..c10f4c4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/input.wxml
@@ -0,0 +1,30 @@
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.d.ts
new file mode 100644
index 0000000..5cd130a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.d.ts
@@ -0,0 +1,4 @@
+///
+export declare const commonProps: WechatMiniprogram.Component.PropertyOption;
+export declare const inputProps: WechatMiniprogram.Component.PropertyOption;
+export declare const textareaProps: WechatMiniprogram.Component.PropertyOption;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.js
new file mode 100644
index 0000000..3cb8dca
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/props.js
@@ -0,0 +1,67 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.textareaProps = exports.inputProps = exports.commonProps = void 0;
+exports.commonProps = {
+ value: {
+ type: String,
+ observer: function (value) {
+ if (value !== this.value) {
+ this.setData({ innerValue: value });
+ this.value = value;
+ }
+ },
+ },
+ placeholder: String,
+ placeholderStyle: String,
+ placeholderClass: String,
+ disabled: Boolean,
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 50,
+ },
+ autoFocus: Boolean,
+ focus: Boolean,
+ cursor: {
+ type: Number,
+ value: -1,
+ },
+ selectionStart: {
+ type: Number,
+ value: -1,
+ },
+ selectionEnd: {
+ type: Number,
+ value: -1,
+ },
+ adjustPosition: {
+ type: Boolean,
+ value: true,
+ },
+ holdKeyboard: Boolean,
+};
+exports.inputProps = {
+ type: {
+ type: String,
+ value: 'text',
+ },
+ password: Boolean,
+ confirmType: String,
+ confirmHold: Boolean,
+ alwaysEmbed: Boolean,
+};
+exports.textareaProps = {
+ autoHeight: Boolean,
+ fixed: Boolean,
+ showConfirmBar: {
+ type: Boolean,
+ value: true,
+ },
+ disableDefaultPadding: {
+ type: Boolean,
+ value: true,
+ },
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/textarea.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/textarea.wxml
new file mode 100644
index 0000000..945d03e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/textarea.wxml
@@ -0,0 +1,32 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.d.ts
new file mode 100644
index 0000000..357ccbe
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.d.ts
@@ -0,0 +1,8 @@
+export interface InputDetails {
+ /** 输入框内容 */
+ value: string;
+ /** 光标位置 */
+ cursor?: number;
+ /** keyCode 为键值 (目前工具还不支持返回keyCode参数) `2.1.0` 起支持 */
+ keyCode?: number;
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.js
new file mode 100644
index 0000000..c8ad2e5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/field/types.js
@@ -0,0 +1,2 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.js
new file mode 100644
index 0000000..8179e89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.js
@@ -0,0 +1,46 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var button_1 = require("../mixins/button");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ mixins: [link_1.link, button_1.button],
+ relation: (0, relation_1.useParent)('goods-action'),
+ props: {
+ text: String,
+ color: String,
+ size: {
+ type: String,
+ value: 'normal',
+ },
+ loading: Boolean,
+ disabled: Boolean,
+ plain: Boolean,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ customStyle: {
+ type: String,
+ value: '',
+ },
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ updateStyle: function () {
+ if (this.parent == null) {
+ return;
+ }
+ var index = this.index;
+ var _a = this.parent.children, children = _a === void 0 ? [] : _a;
+ this.setData({
+ isFirst: index === 0,
+ isLast: index === children.length - 1,
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.json
new file mode 100644
index 0000000..b567686
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxml
new file mode 100644
index 0000000..4530ab8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxml
@@ -0,0 +1,35 @@
+
+
+ {{ text }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxss
new file mode 100644
index 0000000..759a1d9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-button/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-goods-action-button{--button-warning-background-color:var(--goods-action-button-warning-color,linear-gradient(to right,#ffd01e,#ff8917));--button-danger-background-color:var(--goods-action-button-danger-color,linear-gradient(to right,#ff6034,#ee0a24));--button-default-height:var(--goods-action-button-height,40px);--button-line-height:var(--goods-action-button-line-height,20px);--button-plain-background-color:var(--goods-action-button-plain-color,#fff);--button-border-width:0;display:block}.van-goods-action-button--first{--button-border-radius:999px 0 0 var(--goods-action-button-border-radius,999px);margin-left:5px}.van-goods-action-button--last{--button-border-radius:0 999px var(--goods-action-button-border-radius,999px) 0;margin-right:5px}.van-goods-action-button--first.van-goods-action-button--last{--button-border-radius:var(--goods-action-button-border-radius,999px)}.van-goods-action-button--plain{--button-border-width:1px}.van-goods-action-button__inner{font-weight:var(--font-weight-bold,500)!important;width:100%}@media (max-width:321px){.van-goods-action-button{font-size:13px}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.js
new file mode 100644
index 0000000..828e1f5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.js
@@ -0,0 +1,29 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ classes: ['icon-class', 'text-class', 'info-class'],
+ mixins: [link_1.link, button_1.button],
+ props: {
+ text: String,
+ dot: Boolean,
+ info: String,
+ icon: String,
+ size: String,
+ color: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ disabled: Boolean,
+ loading: Boolean,
+ },
+ methods: {
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.json
new file mode 100644
index 0000000..93bfe8a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-button": "../button/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxml
new file mode 100644
index 0000000..30c1a8c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+ {{ text }}
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxss
new file mode 100644
index 0000000..6e4758d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action-icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action-icon{border:none!important;color:var(--goods-action-icon-text-color,#646566)!important;display:flex!important;flex-direction:column;font-size:var(--goods-action-icon-font-size,10px)!important;height:var(--goods-action-icon-height,50px)!important;justify-content:center!important;line-height:1!important;min-width:var(--goods-action-icon-width,48px)}.van-goods-action-icon__icon{color:var(--goods-action-icon-color,#323233);display:flex;font-size:var(--goods-action-icon-size,18px);margin:0 auto 5px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.js
new file mode 100644
index 0000000..e49bcbc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.js
@@ -0,0 +1,17 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('goods-action-button', function () {
+ this.children.forEach(function (item) {
+ item.updateStyle();
+ });
+ }),
+ props: {
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxml
new file mode 100644
index 0000000..569450c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxss
new file mode 100644
index 0000000..7793e77
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/goods-action/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-goods-action{align-items:center;background-color:var(--goods-action-background-color,#fff);bottom:0;box-sizing:initial;display:flex;height:var(--goods-action-height,50px);left:0;position:fixed;right:0}.van-goods-action--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.js
new file mode 100644
index 0000000..a7d47a2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.js
@@ -0,0 +1,54 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var link_1 = require("../mixins/link");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('grid'),
+ classes: ['content-class', 'icon-class', 'text-class'],
+ mixins: [link_1.link],
+ props: {
+ icon: String,
+ iconColor: String,
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ dot: Boolean,
+ info: null,
+ badge: null,
+ text: String,
+ useSlot: Boolean,
+ },
+ data: {
+ viewStyle: '',
+ },
+ mounted: function () {
+ this.updateStyle();
+ },
+ methods: {
+ updateStyle: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent, data = _a.data, children = _a.children;
+ var columnNum = data.columnNum, border = data.border, square = data.square, gutter = data.gutter, clickable = data.clickable, center = data.center, direction = data.direction, reverse = data.reverse, iconSize = data.iconSize;
+ this.setData({
+ center: center,
+ border: border,
+ square: square,
+ gutter: gutter,
+ clickable: clickable,
+ direction: direction,
+ reverse: reverse,
+ iconSize: iconSize,
+ index: children.indexOf(this),
+ columnNum: columnNum,
+ });
+ },
+ onClick: function () {
+ this.$emit('click');
+ this.jumpLink();
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxml
new file mode 100644
index 0000000..e95087d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxml
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxs
new file mode 100644
index 0000000..2cfe37d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapperStyle(data) {
+ var width = 100 / data.columnNum + '%';
+
+ return style({
+ width: width,
+ 'padding-top': data.square ? width : null,
+ 'padding-right': addUnit(data.gutter),
+ 'margin-top':
+ data.index >= data.columnNum && !data.square
+ ? addUnit(data.gutter)
+ : null,
+ });
+}
+
+function contentStyle(data) {
+ return data.square
+ ? style({
+ right: addUnit(data.gutter),
+ bottom: addUnit(data.gutter),
+ height: 'auto',
+ })
+ : '';
+}
+
+module.exports = {
+ wrapperStyle: wrapperStyle,
+ contentStyle: contentStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxss
new file mode 100644
index 0000000..acaea84
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid-item{box-sizing:border-box;float:left;position:relative}.van-grid-item--square{height:0}.van-grid-item__content{background-color:var(--grid-item-content-background-color,#fff);box-sizing:border-box;display:flex;flex-direction:column;height:100%;padding:var(--grid-item-content-padding,16px 8px)}.van-grid-item__content:after{border-width:0 1px 1px 0;z-index:1}.van-grid-item__content--surround:after{border-width:1px}.van-grid-item__content--center{align-items:center;justify-content:center}.van-grid-item__content--square{left:0;position:absolute;right:0;top:0}.van-grid-item__content--horizontal{flex-direction:row}.van-grid-item__content--horizontal .van-grid-item__text{margin:0 0 0 8px}.van-grid-item__content--reverse{flex-direction:column-reverse}.van-grid-item__content--reverse .van-grid-item__text{margin:0 0 8px}.van-grid-item__content--horizontal.van-grid-item__content--reverse{flex-direction:row-reverse}.van-grid-item__content--horizontal.van-grid-item__content--reverse .van-grid-item__text{margin:0 8px 0 0}.van-grid-item__content--clickable:active{background-color:var(--grid-item-content-active-color,#f2f3f5)}.van-grid-item__icon{align-items:center;display:flex;font-size:var(--grid-item-icon-size,26px);height:var(--grid-item-icon-size,26px)}.van-grid-item__text{word-wrap:break-word;color:var(--grid-item-text-color,#646566);font-size:var(--grid-item-text-font-size,12px)}.van-grid-item__icon+.van-grid-item__text{margin-top:8px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.js
new file mode 100644
index 0000000..28d14f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.js
@@ -0,0 +1,57 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('grid-item'),
+ props: {
+ square: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ gutter: {
+ type: null,
+ value: 0,
+ observer: 'updateChildren',
+ },
+ clickable: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ columnNum: {
+ type: Number,
+ value: 4,
+ observer: 'updateChildren',
+ },
+ center: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ observer: 'updateChildren',
+ },
+ direction: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ iconSize: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ reverse: {
+ type: Boolean,
+ value: false,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ this.children.forEach(function (child) {
+ child.updateStyle();
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxml
new file mode 100644
index 0000000..2e4118f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxs
new file mode 100644
index 0000000..cd3b1bd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'padding-left': addUnit(data.gutter),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxss
new file mode 100644
index 0000000..e347440
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/grid/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-grid{box-sizing:border-box;overflow:hidden;position:relative}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.js
new file mode 100644
index 0000000..6758092
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.js
@@ -0,0 +1,23 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['info-class'],
+ props: {
+ dot: Boolean,
+ info: null,
+ size: null,
+ color: String,
+ customStyle: String,
+ classPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ name: String,
+ },
+ methods: {
+ onClick: function () {
+ this.$emit('click');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxml
new file mode 100644
index 0000000..91b47f9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxs
new file mode 100644
index 0000000..a906f76
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxs
@@ -0,0 +1,43 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isImage(name) {
+ return name.indexOf('/') !== -1;
+}
+
+function rootClass(data) {
+ var classes = ['custom-class'];
+
+ if (data.classPrefix !== 'van-icon') {
+ classes.push('van-icon--custom')
+ }
+
+ if (data.classPrefix != null) {
+ classes.push(data.classPrefix);
+ }
+
+ if (isImage(data.name)) {
+ classes.push('van-icon--image');
+ } else if (data.classPrefix != null) {
+ classes.push(data.classPrefix + '-' + data.name);
+ }
+
+ return classes.join(' ');
+}
+
+function rootStyle(data) {
+ return style([
+ {
+ color: data.color,
+ 'font-size': addUnit(data.size),
+ },
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ isImage: isImage,
+ rootClass: rootClass,
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxss
new file mode 100644
index 0000000..feb3d7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/icon/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-icon{text-rendering:auto;-webkit-font-smoothing:antialiased;font:normal normal normal 14px/1 vant-icon;font:normal normal normal 14px/1 var(--van-icon-font-family,"vant-icon");font-size:inherit;position:relative}.van-icon,.van-icon:before{display:inline-block}.van-icon-contact:before{content:"\e753"}.van-icon-notes:before{content:"\e63c"}.van-icon-records:before{content:"\e63d"}.van-icon-cash-back-record:before{content:"\e63e"}.van-icon-newspaper:before{content:"\e63f"}.van-icon-discount:before{content:"\e640"}.van-icon-completed:before{content:"\e641"}.van-icon-user:before{content:"\e642"}.van-icon-description:before{content:"\e643"}.van-icon-list-switch:before{content:"\e6ad"}.van-icon-list-switching:before{content:"\e65a"}.van-icon-link-o:before{content:"\e751"}.van-icon-miniprogram-o:before{content:"\e752"}.van-icon-qq:before{content:"\e74e"}.van-icon-wechat-moments:before{content:"\e74f"}.van-icon-weibo:before{content:"\e750"}.van-icon-cash-o:before{content:"\e74d"}.van-icon-guide-o:before{content:"\e74c"}.van-icon-invitation:before{content:"\e6d6"}.van-icon-shield-o:before{content:"\e74b"}.van-icon-exchange:before{content:"\e6af"}.van-icon-eye:before{content:"\e6b0"}.van-icon-enlarge:before{content:"\e6b1"}.van-icon-expand-o:before{content:"\e6b2"}.van-icon-eye-o:before{content:"\e6b3"}.van-icon-expand:before{content:"\e6b4"}.van-icon-filter-o:before{content:"\e6b5"}.van-icon-fire:before{content:"\e6b6"}.van-icon-fail:before{content:"\e6b7"}.van-icon-failure:before{content:"\e6b8"}.van-icon-fire-o:before{content:"\e6b9"}.van-icon-flag-o:before{content:"\e6ba"}.van-icon-font:before{content:"\e6bb"}.van-icon-font-o:before{content:"\e6bc"}.van-icon-gem-o:before{content:"\e6bd"}.van-icon-flower-o:before{content:"\e6be"}.van-icon-gem:before{content:"\e6bf"}.van-icon-gift-card:before{content:"\e6c0"}.van-icon-friends:before{content:"\e6c1"}.van-icon-friends-o:before{content:"\e6c2"}.van-icon-gold-coin:before{content:"\e6c3"}.van-icon-gold-coin-o:before{content:"\e6c4"}.van-icon-good-job-o:before{content:"\e6c5"}.van-icon-gift:before{content:"\e6c6"}.van-icon-gift-o:before{content:"\e6c7"}.van-icon-gift-card-o:before{content:"\e6c8"}.van-icon-good-job:before{content:"\e6c9"}.van-icon-home-o:before{content:"\e6ca"}.van-icon-goods-collect:before{content:"\e6cb"}.van-icon-graphic:before{content:"\e6cc"}.van-icon-goods-collect-o:before{content:"\e6cd"}.van-icon-hot-o:before{content:"\e6ce"}.van-icon-info:before{content:"\e6cf"}.van-icon-hotel-o:before{content:"\e6d0"}.van-icon-info-o:before{content:"\e6d1"}.van-icon-hot-sale-o:before{content:"\e6d2"}.van-icon-hot:before{content:"\e6d3"}.van-icon-like:before{content:"\e6d4"}.van-icon-idcard:before{content:"\e6d5"}.van-icon-like-o:before{content:"\e6d7"}.van-icon-hot-sale:before{content:"\e6d8"}.van-icon-location-o:before{content:"\e6d9"}.van-icon-location:before{content:"\e6da"}.van-icon-label:before{content:"\e6db"}.van-icon-lock:before{content:"\e6dc"}.van-icon-label-o:before{content:"\e6dd"}.van-icon-map-marked:before{content:"\e6de"}.van-icon-logistics:before{content:"\e6df"}.van-icon-manager:before{content:"\e6e0"}.van-icon-more:before{content:"\e6e1"}.van-icon-live:before{content:"\e6e2"}.van-icon-manager-o:before{content:"\e6e3"}.van-icon-medal:before{content:"\e6e4"}.van-icon-more-o:before{content:"\e6e5"}.van-icon-music-o:before{content:"\e6e6"}.van-icon-music:before{content:"\e6e7"}.van-icon-new-arrival-o:before{content:"\e6e8"}.van-icon-medal-o:before{content:"\e6e9"}.van-icon-new-o:before{content:"\e6ea"}.van-icon-free-postage:before{content:"\e6eb"}.van-icon-newspaper-o:before{content:"\e6ec"}.van-icon-new-arrival:before{content:"\e6ed"}.van-icon-minus:before{content:"\e6ee"}.van-icon-orders-o:before{content:"\e6ef"}.van-icon-new:before{content:"\e6f0"}.van-icon-paid:before{content:"\e6f1"}.van-icon-notes-o:before{content:"\e6f2"}.van-icon-other-pay:before{content:"\e6f3"}.van-icon-pause-circle:before{content:"\e6f4"}.van-icon-pause:before{content:"\e6f5"}.van-icon-pause-circle-o:before{content:"\e6f6"}.van-icon-peer-pay:before{content:"\e6f7"}.van-icon-pending-payment:before{content:"\e6f8"}.van-icon-passed:before{content:"\e6f9"}.van-icon-plus:before{content:"\e6fa"}.van-icon-phone-circle-o:before{content:"\e6fb"}.van-icon-phone-o:before{content:"\e6fc"}.van-icon-printer:before{content:"\e6fd"}.van-icon-photo-fail:before{content:"\e6fe"}.van-icon-phone:before{content:"\e6ff"}.van-icon-photo-o:before{content:"\e700"}.van-icon-play-circle:before{content:"\e701"}.van-icon-play:before{content:"\e702"}.van-icon-phone-circle:before{content:"\e703"}.van-icon-point-gift-o:before{content:"\e704"}.van-icon-point-gift:before{content:"\e705"}.van-icon-play-circle-o:before{content:"\e706"}.van-icon-shrink:before{content:"\e707"}.van-icon-photo:before{content:"\e708"}.van-icon-qr:before{content:"\e709"}.van-icon-qr-invalid:before{content:"\e70a"}.van-icon-question-o:before{content:"\e70b"}.van-icon-revoke:before{content:"\e70c"}.van-icon-replay:before{content:"\e70d"}.van-icon-service:before{content:"\e70e"}.van-icon-question:before{content:"\e70f"}.van-icon-search:before{content:"\e710"}.van-icon-refund-o:before{content:"\e711"}.van-icon-service-o:before{content:"\e712"}.van-icon-scan:before{content:"\e713"}.van-icon-share:before{content:"\e714"}.van-icon-send-gift-o:before{content:"\e715"}.van-icon-share-o:before{content:"\e716"}.van-icon-setting:before{content:"\e717"}.van-icon-points:before{content:"\e718"}.van-icon-photograph:before{content:"\e719"}.van-icon-shop:before{content:"\e71a"}.van-icon-shop-o:before{content:"\e71b"}.van-icon-shop-collect-o:before{content:"\e71c"}.van-icon-shop-collect:before{content:"\e71d"}.van-icon-smile:before{content:"\e71e"}.van-icon-shopping-cart-o:before{content:"\e71f"}.van-icon-sign:before{content:"\e720"}.van-icon-sort:before{content:"\e721"}.van-icon-star-o:before{content:"\e722"}.van-icon-smile-comment-o:before{content:"\e723"}.van-icon-stop:before{content:"\e724"}.van-icon-stop-circle-o:before{content:"\e725"}.van-icon-smile-o:before{content:"\e726"}.van-icon-star:before{content:"\e727"}.van-icon-success:before{content:"\e728"}.van-icon-stop-circle:before{content:"\e729"}.van-icon-records-o:before{content:"\e72a"}.van-icon-shopping-cart:before{content:"\e72b"}.van-icon-tosend:before{content:"\e72c"}.van-icon-todo-list:before{content:"\e72d"}.van-icon-thumb-circle-o:before{content:"\e72e"}.van-icon-thumb-circle:before{content:"\e72f"}.van-icon-umbrella-circle:before{content:"\e730"}.van-icon-underway:before{content:"\e731"}.van-icon-upgrade:before{content:"\e732"}.van-icon-todo-list-o:before{content:"\e733"}.van-icon-tv-o:before{content:"\e734"}.van-icon-underway-o:before{content:"\e735"}.van-icon-user-o:before{content:"\e736"}.van-icon-vip-card-o:before{content:"\e737"}.van-icon-vip-card:before{content:"\e738"}.van-icon-send-gift:before{content:"\e739"}.van-icon-wap-home:before{content:"\e73a"}.van-icon-wap-nav:before{content:"\e73b"}.van-icon-volume-o:before{content:"\e73c"}.van-icon-video:before{content:"\e73d"}.van-icon-wap-home-o:before{content:"\e73e"}.van-icon-volume:before{content:"\e73f"}.van-icon-warning:before{content:"\e740"}.van-icon-weapp-nav:before{content:"\e741"}.van-icon-wechat-pay:before{content:"\e742"}.van-icon-warning-o:before{content:"\e743"}.van-icon-wechat:before{content:"\e744"}.van-icon-setting-o:before{content:"\e745"}.van-icon-youzan-shield:before{content:"\e746"}.van-icon-warn-o:before{content:"\e747"}.van-icon-smile-comment:before{content:"\e748"}.van-icon-user-circle-o:before{content:"\e749"}.van-icon-video-o:before{content:"\e74a"}.van-icon-add-square:before{content:"\e65c"}.van-icon-add:before{content:"\e65d"}.van-icon-arrow-down:before{content:"\e65e"}.van-icon-arrow-up:before{content:"\e65f"}.van-icon-arrow:before{content:"\e660"}.van-icon-after-sale:before{content:"\e661"}.van-icon-add-o:before{content:"\e662"}.van-icon-alipay:before{content:"\e663"}.van-icon-ascending:before{content:"\e664"}.van-icon-apps-o:before{content:"\e665"}.van-icon-aim:before{content:"\e666"}.van-icon-award:before{content:"\e667"}.van-icon-arrow-left:before{content:"\e668"}.van-icon-award-o:before{content:"\e669"}.van-icon-audio:before{content:"\e66a"}.van-icon-bag-o:before{content:"\e66b"}.van-icon-balance-list:before{content:"\e66c"}.van-icon-back-top:before{content:"\e66d"}.van-icon-bag:before{content:"\e66e"}.van-icon-balance-pay:before{content:"\e66f"}.van-icon-balance-o:before{content:"\e670"}.van-icon-bar-chart-o:before{content:"\e671"}.van-icon-bars:before{content:"\e672"}.van-icon-balance-list-o:before{content:"\e673"}.van-icon-birthday-cake-o:before{content:"\e674"}.van-icon-bookmark:before{content:"\e675"}.van-icon-bill:before{content:"\e676"}.van-icon-bell:before{content:"\e677"}.van-icon-browsing-history-o:before{content:"\e678"}.van-icon-browsing-history:before{content:"\e679"}.van-icon-bookmark-o:before{content:"\e67a"}.van-icon-bulb-o:before{content:"\e67b"}.van-icon-bullhorn-o:before{content:"\e67c"}.van-icon-bill-o:before{content:"\e67d"}.van-icon-calendar-o:before{content:"\e67e"}.van-icon-brush-o:before{content:"\e67f"}.van-icon-card:before{content:"\e680"}.van-icon-cart-o:before{content:"\e681"}.van-icon-cart-circle:before{content:"\e682"}.van-icon-cart-circle-o:before{content:"\e683"}.van-icon-cart:before{content:"\e684"}.van-icon-cash-on-deliver:before{content:"\e685"}.van-icon-cash-back-record-o:before{content:"\e686"}.van-icon-cashier-o:before{content:"\e687"}.van-icon-chart-trending-o:before{content:"\e688"}.van-icon-certificate:before{content:"\e689"}.van-icon-chat:before{content:"\e68a"}.van-icon-clear:before{content:"\e68b"}.van-icon-chat-o:before{content:"\e68c"}.van-icon-checked:before{content:"\e68d"}.van-icon-clock:before{content:"\e68e"}.van-icon-clock-o:before{content:"\e68f"}.van-icon-close:before{content:"\e690"}.van-icon-closed-eye:before{content:"\e691"}.van-icon-circle:before{content:"\e692"}.van-icon-cluster-o:before{content:"\e693"}.van-icon-column:before{content:"\e694"}.van-icon-comment-circle-o:before{content:"\e695"}.van-icon-cluster:before{content:"\e696"}.van-icon-comment:before{content:"\e697"}.van-icon-comment-o:before{content:"\e698"}.van-icon-comment-circle:before{content:"\e699"}.van-icon-completed-o:before{content:"\e69a"}.van-icon-credit-pay:before{content:"\e69b"}.van-icon-coupon:before{content:"\e69c"}.van-icon-debit-pay:before{content:"\e69d"}.van-icon-coupon-o:before{content:"\e69e"}.van-icon-contact-o:before{content:"\e69f"}.van-icon-descending:before{content:"\e6a0"}.van-icon-desktop-o:before{content:"\e6a1"}.van-icon-diamond-o:before{content:"\e6a2"}.van-icon-description-o:before{content:"\e6a3"}.van-icon-delete:before{content:"\e6a4"}.van-icon-diamond:before{content:"\e6a5"}.van-icon-delete-o:before{content:"\e6a6"}.van-icon-cross:before{content:"\e6a7"}.van-icon-edit:before{content:"\e6a8"}.van-icon-ellipsis:before{content:"\e6a9"}.van-icon-down:before{content:"\e6aa"}.van-icon-discount-o:before{content:"\e6ab"}.van-icon-ecard-pay:before{content:"\e6ac"}.van-icon-envelop-o:before{content:"\e6ae"}@font-face{font-display:auto;font-family:vant-icon;font-style:normal;font-weight:400;src:url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff2?t=1694918397022) format("woff2"),url(//at.alicdn.com/t/c/font_2553510_kfwma2yq1rs.woff?t=1694918397022) format("woff")}:host{align-items:center;display:inline-flex;justify-content:center}.van-icon--custom{position:relative}.van-icon--image{height:1em;width:1em}.van-icon__image{height:100%;width:100%}.van-icon__info{z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.js
new file mode 100644
index 0000000..40f6812
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.js
@@ -0,0 +1,66 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var button_1 = require("../mixins/button");
+(0, component_1.VantComponent)({
+ mixins: [button_1.button],
+ classes: ['custom-class', 'loading-class', 'error-class', 'image-class'],
+ props: {
+ src: {
+ type: String,
+ observer: function () {
+ this.setData({
+ error: false,
+ loading: true,
+ });
+ },
+ },
+ round: Boolean,
+ width: null,
+ height: null,
+ radius: null,
+ lazyLoad: Boolean,
+ useErrorSlot: Boolean,
+ useLoadingSlot: Boolean,
+ showMenuByLongpress: Boolean,
+ fit: {
+ type: String,
+ value: 'fill',
+ },
+ webp: {
+ type: Boolean,
+ value: false,
+ },
+ showError: {
+ type: Boolean,
+ value: true,
+ },
+ showLoading: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ error: false,
+ loading: true,
+ viewStyle: '',
+ },
+ methods: {
+ onLoad: function (event) {
+ this.setData({
+ loading: false,
+ });
+ this.$emit('load', event.detail);
+ },
+ onError: function (event) {
+ this.setData({
+ loading: false,
+ error: true,
+ });
+ this.$emit('error', event.detail);
+ },
+ onClick: function (event) {
+ this.$emit('click', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxml
new file mode 100644
index 0000000..a6f573c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxs
new file mode 100644
index 0000000..cec14b8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxs
@@ -0,0 +1,32 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ width: addUnit(data.width),
+ height: addUnit(data.height),
+ 'border-radius': addUnit(data.radius),
+ },
+ data.radius ? 'overflow: hidden' : null,
+ ]);
+}
+
+var FIT_MODE_MAP = {
+ none: 'center',
+ fill: 'scaleToFill',
+ cover: 'aspectFill',
+ contain: 'aspectFit',
+ widthFix: 'widthFix',
+ heightFix: 'heightFix',
+};
+
+function mode(fit) {
+ return FIT_MODE_MAP[fit];
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ mode: mode,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxss
new file mode 100644
index 0000000..a9c6ebb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/image/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-image{display:inline-block;position:relative}.van-image--round{border-radius:50%;overflow:hidden}.van-image--round .van-image__img{border-radius:inherit}.van-image__error,.van-image__img,.van-image__loading{display:block;height:100%;width:100%}.van-image__error,.van-image__loading{align-items:center;background-color:var(--image-placeholder-background-color,#f7f8fa);color:var(--image-placeholder-text-color,#969799);display:flex;flex-direction:column;font-size:var(--image-placeholder-font-size,14px);justify-content:center;left:0;position:absolute;top:0}.van-image__loading-icon{color:var(--image-loading-icon-color,#dcdee0);font-size:var(--image-loading-icon-size,32px)!important}.van-image__error-icon{color:var(--image-error-icon-color,#dcdee0);font-size:var(--image-error-icon-size,32px)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.js
new file mode 100644
index 0000000..9a361a9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.js
@@ -0,0 +1,28 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('index-bar'),
+ props: {
+ useSlot: Boolean,
+ index: null,
+ },
+ data: {
+ active: false,
+ wrapperStyle: '',
+ anchorStyle: '',
+ },
+ methods: {
+ scrollIntoView: function (scrollTop) {
+ var _this = this;
+ (0, utils_1.getRect)(this, '.van-index-anchor-wrapper').then(function (rect) {
+ wx.pageScrollTo({
+ duration: 0,
+ scrollTop: scrollTop + rect.top - _this.parent.data.stickyOffsetTop,
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxml
new file mode 100644
index 0000000..49affa7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxml
@@ -0,0 +1,14 @@
+
+
+
+
+ {{ index }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxss
new file mode 100644
index 0000000..4b91560
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-anchor/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-anchor{background-color:var(--index-anchor-background-color,transparent);color:var(--index-anchor-text-color,#323233);font-size:var(--index-anchor-font-size,14px);font-weight:var(--index-anchor-font-weight,500);line-height:var(--index-anchor-line-height,32px);padding:var(--index-anchor-padding,0 16px)}.van-index-anchor--active{background-color:var(--index-anchor-active-background-color,#fff);color:var(--index-anchor-active-text-color,#07c160);left:0;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.js
new file mode 100644
index 0000000..afc5412
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.js
@@ -0,0 +1,243 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+var page_scroll_1 = require("../mixins/page-scroll");
+var indexList = function () {
+ var indexList = [];
+ var charCodeOfA = 'A'.charCodeAt(0);
+ for (var i = 0; i < 26; i++) {
+ indexList.push(String.fromCharCode(charCodeOfA + i));
+ }
+ return indexList;
+};
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('index-anchor', function () {
+ this.updateData();
+ }),
+ props: {
+ sticky: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ highlightColor: {
+ type: String,
+ value: color_1.GREEN,
+ },
+ stickyOffsetTop: {
+ type: Number,
+ value: 0,
+ },
+ indexList: {
+ type: Array,
+ value: indexList(),
+ },
+ },
+ mixins: [
+ (0, page_scroll_1.pageScrollMixin)(function (event) {
+ this.scrollTop = (event === null || event === void 0 ? void 0 : event.scrollTop) || 0;
+ this.onScroll();
+ }),
+ ],
+ data: {
+ activeAnchorIndex: null,
+ showSidebar: false,
+ },
+ created: function () {
+ this.scrollTop = 0;
+ },
+ methods: {
+ updateData: function () {
+ var _this = this;
+ wx.nextTick(function () {
+ if (_this.timer != null) {
+ clearTimeout(_this.timer);
+ }
+ _this.timer = setTimeout(function () {
+ _this.setData({
+ showSidebar: !!_this.children.length,
+ });
+ _this.setRect().then(function () {
+ _this.onScroll();
+ });
+ }, 0);
+ });
+ },
+ setRect: function () {
+ return Promise.all([
+ this.setAnchorsRect(),
+ this.setListRect(),
+ this.setSiderbarRect(),
+ ]);
+ },
+ setAnchorsRect: function () {
+ var _this = this;
+ return Promise.all(this.children.map(function (anchor) {
+ return (0, utils_1.getRect)(anchor, '.van-index-anchor-wrapper').then(function (rect) {
+ Object.assign(anchor, {
+ height: rect.height,
+ top: rect.top + _this.scrollTop,
+ });
+ });
+ }));
+ },
+ setListRect: function () {
+ var _this = this;
+ return (0, utils_1.getRect)(this, '.van-index-bar').then(function (rect) {
+ if (!(0, utils_1.isDef)(rect)) {
+ return;
+ }
+ Object.assign(_this, {
+ height: rect.height,
+ top: rect.top + _this.scrollTop,
+ });
+ });
+ },
+ setSiderbarRect: function () {
+ var _this = this;
+ return (0, utils_1.getRect)(this, '.van-index-bar__sidebar').then(function (res) {
+ if (!(0, utils_1.isDef)(res)) {
+ return;
+ }
+ _this.sidebar = {
+ height: res.height,
+ top: res.top,
+ };
+ });
+ },
+ setDiffData: function (_a) {
+ var target = _a.target, data = _a.data;
+ var diffData = {};
+ Object.keys(data).forEach(function (key) {
+ if (target.data[key] !== data[key]) {
+ diffData[key] = data[key];
+ }
+ });
+ if (Object.keys(diffData).length) {
+ target.setData(diffData);
+ }
+ },
+ getAnchorRect: function (anchor) {
+ return (0, utils_1.getRect)(anchor, '.van-index-anchor-wrapper').then(function (rect) { return ({
+ height: rect.height,
+ top: rect.top,
+ }); });
+ },
+ getActiveAnchorIndex: function () {
+ var _a = this, children = _a.children, scrollTop = _a.scrollTop;
+ var _b = this.data, sticky = _b.sticky, stickyOffsetTop = _b.stickyOffsetTop;
+ for (var i = this.children.length - 1; i >= 0; i--) {
+ var preAnchorHeight = i > 0 ? children[i - 1].height : 0;
+ var reachTop = sticky ? preAnchorHeight + stickyOffsetTop : 0;
+ if (reachTop + scrollTop >= children[i].top) {
+ return i;
+ }
+ }
+ return -1;
+ },
+ onScroll: function () {
+ var _this = this;
+ var _a = this, _b = _a.children, children = _b === void 0 ? [] : _b, scrollTop = _a.scrollTop;
+ if (!children.length) {
+ return;
+ }
+ var _c = this.data, sticky = _c.sticky, stickyOffsetTop = _c.stickyOffsetTop, zIndex = _c.zIndex, highlightColor = _c.highlightColor;
+ var active = this.getActiveAnchorIndex();
+ this.setDiffData({
+ target: this,
+ data: {
+ activeAnchorIndex: active,
+ },
+ });
+ if (sticky) {
+ var isActiveAnchorSticky_1 = false;
+ if (active !== -1) {
+ isActiveAnchorSticky_1 =
+ children[active].top <= stickyOffsetTop + scrollTop;
+ }
+ children.forEach(function (item, index) {
+ if (index === active) {
+ var wrapperStyle = '';
+ var anchorStyle = "\n color: ".concat(highlightColor, ";\n ");
+ if (isActiveAnchorSticky_1) {
+ wrapperStyle = "\n height: ".concat(children[index].height, "px;\n ");
+ anchorStyle = "\n position: fixed;\n top: ".concat(stickyOffsetTop, "px;\n z-index: ").concat(zIndex, ";\n color: ").concat(highlightColor, ";\n ");
+ }
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle: anchorStyle,
+ wrapperStyle: wrapperStyle,
+ },
+ });
+ }
+ else if (index === active - 1) {
+ var currentAnchor = children[index];
+ var currentOffsetTop = currentAnchor.top;
+ var targetOffsetTop = index === children.length - 1
+ ? _this.top
+ : children[index + 1].top;
+ var parentOffsetHeight = targetOffsetTop - currentOffsetTop;
+ var translateY = parentOffsetHeight - currentAnchor.height;
+ var anchorStyle = "\n position: relative;\n transform: translate3d(0, ".concat(translateY, "px, 0);\n z-index: ").concat(zIndex, ";\n color: ").concat(highlightColor, ";\n ");
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: true,
+ anchorStyle: anchorStyle,
+ },
+ });
+ }
+ else {
+ _this.setDiffData({
+ target: item,
+ data: {
+ active: false,
+ anchorStyle: '',
+ wrapperStyle: '',
+ },
+ });
+ }
+ });
+ }
+ },
+ onClick: function (event) {
+ this.scrollToAnchor(event.target.dataset.index);
+ },
+ onTouchMove: function (event) {
+ var sidebarLength = this.children.length;
+ var touch = event.touches[0];
+ var itemHeight = this.sidebar.height / sidebarLength;
+ var index = Math.floor((touch.clientY - this.sidebar.top) / itemHeight);
+ if (index < 0) {
+ index = 0;
+ }
+ else if (index > sidebarLength - 1) {
+ index = sidebarLength - 1;
+ }
+ this.scrollToAnchor(index);
+ },
+ onTouchStop: function () {
+ this.scrollToAnchorIndex = null;
+ },
+ scrollToAnchor: function (index) {
+ var _this = this;
+ if (typeof index !== 'number' || this.scrollToAnchorIndex === index) {
+ return;
+ }
+ this.scrollToAnchorIndex = index;
+ var anchor = this.children.find(function (item) { return item.data.index === _this.data.indexList[index]; });
+ if (anchor) {
+ anchor.scrollIntoView(this.scrollTop);
+ this.$emit('select', anchor.data.index);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxml
new file mode 100644
index 0000000..19a59cf
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxml
@@ -0,0 +1,22 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxss
new file mode 100644
index 0000000..8568801
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/index-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-index-bar{position:relative}.van-index-bar__sidebar{display:flex;flex-direction:column;position:fixed;right:0;text-align:center;top:50%;transform:translateY(-50%);-webkit-user-select:none;user-select:none}.van-index-bar__index{font-size:var(--index-bar-index-font-size,10px);font-weight:500;line-height:var(--index-bar-index-line-height,14px);padding:0 var(--padding-base,4px) 0 var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.js
new file mode 100644
index 0000000..e61af73
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.js
@@ -0,0 +1,10 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ dot: Boolean,
+ info: null,
+ customStyle: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxml
new file mode 100644
index 0000000..b39b524
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxml
@@ -0,0 +1,7 @@
+
+
+{{ dot ? '' : info }}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxss
new file mode 100644
index 0000000..375ed5a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/info/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-info{align-items:center;background-color:var(--info-background-color,#ee0a24);border:var(--info-border-width,1px) solid #fff;border-radius:var(--info-size,16px);box-sizing:border-box;color:var(--info-color,#fff);display:inline-flex;font-family:var(--info-font-family,-apple-system-font,Helvetica Neue,Arial,sans-serif);font-size:var(--info-font-size,12px);font-weight:var(--info-font-weight,500);height:var(--info-size,16px);justify-content:center;min-width:var(--info-size,16px);padding:var(--info-padding,0 3px);position:absolute;right:0;top:0;transform:translate(50%,-50%);transform-origin:100%;white-space:nowrap}.van-info--dot{background-color:var(--info-dot-color,#ee0a24);border-radius:100%;height:var(--info-dot-size,8px);min-width:0;width:var(--info-dot-size,8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.js
new file mode 100644
index 0000000..be9c0ef
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.js
@@ -0,0 +1,18 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ color: String,
+ vertical: Boolean,
+ type: {
+ type: String,
+ value: 'circular',
+ },
+ size: String,
+ textSize: String,
+ },
+ data: {
+ array12: Array.from({ length: 12 }),
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxml
new file mode 100644
index 0000000..7d4a539
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxs
new file mode 100644
index 0000000..02a0b80
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function spinnerStyle(data) {
+ return style({
+ color: data.color,
+ width: addUnit(data.size),
+ height: addUnit(data.size),
+ });
+}
+
+function textStyle(data) {
+ return style({
+ 'font-size': addUnit(data.textSize),
+ });
+}
+
+module.exports = {
+ spinnerStyle: spinnerStyle,
+ textStyle: textStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxss
new file mode 100644
index 0000000..fc84e84
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/loading/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{font-size:0;line-height:1}.van-loading{align-items:center;color:var(--loading-spinner-color,#c8c9cc);display:inline-flex;justify-content:center}.van-loading__spinner{animation:van-rotate var(--loading-spinner-animation-duration,.8s) linear infinite;box-sizing:border-box;height:var(--loading-spinner-size,30px);max-height:100%;max-width:100%;position:relative;width:var(--loading-spinner-size,30px)}.van-loading__spinner--spinner{animation-timing-function:steps(12)}.van-loading__spinner--circular{border:1px solid transparent;border-radius:100%;border-top-color:initial}.van-loading__text{color:var(--loading-text-color,#969799);font-size:var(--loading-text-font-size,14px);line-height:var(--loading-text-line-height,20px);margin-left:var(--padding-xs,8px)}.van-loading__text:empty{display:none}.van-loading--vertical{flex-direction:column}.van-loading--vertical .van-loading__text{margin:var(--padding-xs,8px) 0 0}.van-loading__dot{height:100%;left:0;position:absolute;top:0;width:100%}.van-loading__dot:before{background-color:currentColor;border-radius:40%;content:" ";display:block;height:25%;margin:0 auto;width:2px}.van-loading__dot:first-of-type{opacity:1;transform:rotate(30deg)}.van-loading__dot:nth-of-type(2){opacity:.9375;transform:rotate(60deg)}.van-loading__dot:nth-of-type(3){opacity:.875;transform:rotate(90deg)}.van-loading__dot:nth-of-type(4){opacity:.8125;transform:rotate(120deg)}.van-loading__dot:nth-of-type(5){opacity:.75;transform:rotate(150deg)}.van-loading__dot:nth-of-type(6){opacity:.6875;transform:rotate(180deg)}.van-loading__dot:nth-of-type(7){opacity:.625;transform:rotate(210deg)}.van-loading__dot:nth-of-type(8){opacity:.5625;transform:rotate(240deg)}.van-loading__dot:nth-of-type(9){opacity:.5;transform:rotate(270deg)}.van-loading__dot:nth-of-type(10){opacity:.4375;transform:rotate(300deg)}.van-loading__dot:nth-of-type(11){opacity:.375;transform:rotate(330deg)}.van-loading__dot:nth-of-type(12){opacity:.3125;transform:rotate(1turn)}@keyframes van-rotate{0%{transform:rotate(0deg)}to{transform:rotate(1turn)}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.d.ts
new file mode 100644
index 0000000..b273369
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.d.ts
@@ -0,0 +1 @@
+export declare const basic: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.js
new file mode 100644
index 0000000..4373ad4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/basic.js
@@ -0,0 +1,14 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.basic = void 0;
+exports.basic = Behavior({
+ methods: {
+ $emit: function (name, detail, options) {
+ this.triggerEvent(name, detail, options);
+ },
+ set: function (data) {
+ this.setData(data);
+ return new Promise(function (resolve) { return wx.nextTick(resolve); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.d.ts
new file mode 100644
index 0000000..b51db87
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.d.ts
@@ -0,0 +1 @@
+export declare const button: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.js
new file mode 100644
index 0000000..8c1c499
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/button.js
@@ -0,0 +1,54 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.button = void 0;
+var version_1 = require("../common/version");
+exports.button = Behavior({
+ externalClasses: ['hover-class'],
+ properties: {
+ id: String,
+ buttonId: String,
+ lang: String,
+ businessId: Number,
+ sessionFrom: String,
+ sendMessageTitle: String,
+ sendMessagePath: String,
+ sendMessageImg: String,
+ showMessageCard: Boolean,
+ appParameter: String,
+ ariaLabel: String,
+ openType: String,
+ getUserProfileDesc: String,
+ },
+ data: {
+ canIUseGetUserProfile: (0, version_1.canIUseGetUserProfile)(),
+ },
+ methods: {
+ onGetUserInfo: function (event) {
+ this.triggerEvent('getuserinfo', event.detail);
+ },
+ onContact: function (event) {
+ this.triggerEvent('contact', event.detail);
+ },
+ onGetPhoneNumber: function (event) {
+ this.triggerEvent('getphonenumber', event.detail);
+ },
+ onGetRealTimePhoneNumber: function (event) {
+ this.triggerEvent('getrealtimephonenumber', event.detail);
+ },
+ onError: function (event) {
+ this.triggerEvent('error', event.detail);
+ },
+ onLaunchApp: function (event) {
+ this.triggerEvent('launchapp', event.detail);
+ },
+ onOpenSetting: function (event) {
+ this.triggerEvent('opensetting', event.detail);
+ },
+ onAgreePrivacyAuthorization: function (event) {
+ this.triggerEvent('agreeprivacyauthorization', event.detail);
+ },
+ onChooseAvatar: function (event) {
+ this.triggerEvent('chooseavatar', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.d.ts
new file mode 100644
index 0000000..d58043b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.d.ts
@@ -0,0 +1 @@
+export declare const link: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.js
new file mode 100644
index 0000000..14cb7e8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/link.js
@@ -0,0 +1,27 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.link = void 0;
+exports.link = Behavior({
+ properties: {
+ url: String,
+ linkType: {
+ type: String,
+ value: 'navigateTo',
+ },
+ },
+ methods: {
+ jumpLink: function (urlKey) {
+ if (urlKey === void 0) { urlKey = 'url'; }
+ var url = this.data[urlKey];
+ if (url) {
+ if (this.data.linkType === 'navigateTo' &&
+ getCurrentPages().length > 9) {
+ wx.redirectTo({ url: url });
+ }
+ else {
+ wx[this.data.linkType]({ url: url });
+ }
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.d.ts
new file mode 100644
index 0000000..4625447
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.d.ts
@@ -0,0 +1,6 @@
+///
+///
+type IPageScrollOption = WechatMiniprogram.Page.IPageScrollOption;
+type Scroller = (this: WechatMiniprogram.Component.TrivialInstance, event?: IPageScrollOption) => void;
+export declare function pageScrollMixin(scroller: Scroller): string;
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.js
new file mode 100644
index 0000000..fce7049
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/page-scroll.js
@@ -0,0 +1,47 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.pageScrollMixin = void 0;
+var validator_1 = require("../common/validator");
+var utils_1 = require("../common/utils");
+function onPageScroll(event) {
+ var _a = (0, utils_1.getCurrentPage)().vanPageScroller, vanPageScroller = _a === void 0 ? [] : _a;
+ vanPageScroller.forEach(function (scroller) {
+ if (typeof scroller === 'function') {
+ // @ts-ignore
+ scroller(event);
+ }
+ });
+}
+function pageScrollMixin(scroller) {
+ return Behavior({
+ attached: function () {
+ var page = (0, utils_1.getCurrentPage)();
+ if (!(0, utils_1.isDef)(page)) {
+ return;
+ }
+ var _scroller = scroller.bind(this);
+ var _a = page.vanPageScroller, vanPageScroller = _a === void 0 ? [] : _a;
+ if ((0, validator_1.isFunction)(page.onPageScroll) && page.onPageScroll !== onPageScroll) {
+ vanPageScroller.push(page.onPageScroll.bind(page));
+ }
+ vanPageScroller.push(_scroller);
+ page.vanPageScroller = vanPageScroller;
+ page.onPageScroll = onPageScroll;
+ this._scroller = _scroller;
+ },
+ detached: function () {
+ var _this = this;
+ var page = (0, utils_1.getCurrentPage)();
+ if (!(0, utils_1.isDef)(page) || !(0, utils_1.isDef)(page.vanPageScroller)) {
+ return;
+ }
+ var vanPageScroller = page.vanPageScroller;
+ var index = vanPageScroller.findIndex(function (v) { return v === _this._scroller; });
+ if (index > -1) {
+ page.vanPageScroller.splice(index, 1);
+ }
+ this._scroller = undefined;
+ },
+ });
+}
+exports.pageScrollMixin = pageScrollMixin;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.d.ts
new file mode 100644
index 0000000..35ee831
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.d.ts
@@ -0,0 +1 @@
+export declare const touch: string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.js
new file mode 100644
index 0000000..d762c2c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/touch.js
@@ -0,0 +1,40 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.touch = void 0;
+// @ts-nocheck
+var MIN_DISTANCE = 10;
+function getDirection(x, y) {
+ if (x > y && x > MIN_DISTANCE) {
+ return 'horizontal';
+ }
+ if (y > x && y > MIN_DISTANCE) {
+ return 'vertical';
+ }
+ return '';
+}
+exports.touch = Behavior({
+ methods: {
+ resetTouchStatus: function () {
+ this.direction = '';
+ this.deltaX = 0;
+ this.deltaY = 0;
+ this.offsetX = 0;
+ this.offsetY = 0;
+ },
+ touchStart: function (event) {
+ this.resetTouchStatus();
+ var touch = event.touches[0];
+ this.startX = touch.clientX;
+ this.startY = touch.clientY;
+ },
+ touchMove: function (event) {
+ var touch = event.touches[0];
+ this.deltaX = touch.clientX - this.startX;
+ this.deltaY = touch.clientY - this.startY;
+ this.offsetX = Math.abs(this.deltaX);
+ this.offsetY = Math.abs(this.deltaY);
+ this.direction =
+ this.direction || getDirection(this.offsetX, this.offsetY);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.d.ts
new file mode 100644
index 0000000..dd829e5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.d.ts
@@ -0,0 +1 @@
+export declare function transition(showDefaultValue: boolean): string;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.js
new file mode 100644
index 0000000..922900f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/mixins/transition.js
@@ -0,0 +1,131 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.transition = void 0;
+// @ts-nocheck
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var getClassNames = function (name) { return ({
+ enter: "van-".concat(name, "-enter van-").concat(name, "-enter-active enter-class enter-active-class"),
+ 'enter-to': "van-".concat(name, "-enter-to van-").concat(name, "-enter-active enter-to-class enter-active-class"),
+ leave: "van-".concat(name, "-leave van-").concat(name, "-leave-active leave-class leave-active-class"),
+ 'leave-to': "van-".concat(name, "-leave-to van-").concat(name, "-leave-active leave-to-class leave-active-class"),
+}); };
+function transition(showDefaultValue) {
+ return Behavior({
+ properties: {
+ customStyle: String,
+ // @ts-ignore
+ show: {
+ type: Boolean,
+ value: showDefaultValue,
+ observer: 'observeShow',
+ },
+ // @ts-ignore
+ duration: {
+ type: null,
+ value: 300,
+ observer: 'observeDuration',
+ },
+ name: {
+ type: String,
+ value: 'fade',
+ },
+ },
+ data: {
+ type: '',
+ inited: false,
+ display: false,
+ },
+ ready: function () {
+ if (this.data.show === true) {
+ this.observeShow(true, false);
+ }
+ },
+ methods: {
+ observeShow: function (value, old) {
+ if (value === old) {
+ return;
+ }
+ value ? this.enter() : this.leave();
+ },
+ enter: function () {
+ var _this = this;
+ this.waitEnterEndPromise = new Promise(function (resolve) {
+ var _a = _this.data, duration = _a.duration, name = _a.name;
+ var classNames = getClassNames(name);
+ var currentDuration = (0, validator_1.isObj)(duration) ? duration.enter : duration;
+ if (_this.status === 'enter') {
+ return;
+ }
+ _this.status = 'enter';
+ _this.$emit('before-enter');
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'enter') {
+ return;
+ }
+ _this.$emit('enter');
+ _this.setData({
+ inited: true,
+ display: true,
+ classes: classNames.enter,
+ currentDuration: currentDuration,
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'enter') {
+ return;
+ }
+ _this.transitionEnded = false;
+ _this.setData({ classes: classNames['enter-to'] });
+ resolve();
+ });
+ });
+ });
+ },
+ leave: function () {
+ var _this = this;
+ if (!this.waitEnterEndPromise)
+ return;
+ this.waitEnterEndPromise.then(function () {
+ if (!_this.data.display) {
+ return;
+ }
+ var _a = _this.data, duration = _a.duration, name = _a.name;
+ var classNames = getClassNames(name);
+ var currentDuration = (0, validator_1.isObj)(duration) ? duration.leave : duration;
+ _this.status = 'leave';
+ _this.$emit('before-leave');
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'leave') {
+ return;
+ }
+ _this.$emit('leave');
+ _this.setData({
+ classes: classNames.leave,
+ currentDuration: currentDuration,
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ if (_this.status !== 'leave') {
+ return;
+ }
+ _this.transitionEnded = false;
+ setTimeout(function () { return _this.onTransitionEnd(); }, currentDuration);
+ _this.setData({ classes: classNames['leave-to'] });
+ });
+ });
+ });
+ },
+ onTransitionEnd: function () {
+ if (this.transitionEnded) {
+ return;
+ }
+ this.transitionEnded = true;
+ this.$emit("after-".concat(this.status));
+ var _a = this.data, show = _a.show, display = _a.display;
+ if (!show && display) {
+ this.setData({ display: false });
+ }
+ },
+ },
+ });
+}
+exports.transition = transition;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.js
new file mode 100644
index 0000000..376b561
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ classes: ['title-class'],
+ props: {
+ title: String,
+ fixed: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ leftText: String,
+ rightText: String,
+ customStyle: String,
+ leftArrow: Boolean,
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 46,
+ },
+ created: function () {
+ var statusBarHeight = (0, utils_1.getSystemInfoSync)().statusBarHeight;
+ this.setData({
+ statusBarHeight: statusBarHeight,
+ height: 46 + statusBarHeight,
+ });
+ },
+ mounted: function () {
+ this.setHeight();
+ },
+ methods: {
+ onClickLeft: function () {
+ this.$emit('click-left');
+ },
+ onClickRight: function () {
+ this.$emit('click-right');
+ },
+ setHeight: function () {
+ var _this = this;
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(function () {
+ (0, utils_1.getRect)(_this, '.van-nav-bar').then(function (res) {
+ if (res && 'height' in res) {
+ _this.setData({ height: res.height });
+ }
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxml
new file mode 100644
index 0000000..b6405fd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxml
@@ -0,0 +1,42 @@
+
+
+
+
+
+
+
+
+
+
+ {{ leftText }}
+
+
+
+
+ {{ title }}
+
+
+
+ {{ rightText }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxs
new file mode 100644
index 0000000..55b4158
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function barStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ 'padding-top': data.safeAreaInsetTop ? data.statusBarHeight + 'px' : 0,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxss
new file mode 100644
index 0000000..d11c31e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/nav-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-nav-bar{background-color:var(--nav-bar-background-color,#fff);box-sizing:initial;height:var(--nav-bar-height,46px);line-height:var(--nav-bar-height,46px);position:relative;text-align:center;-webkit-user-select:none;user-select:none}.van-nav-bar__content{height:100%;position:relative}.van-nav-bar__text{color:var(--nav-bar-text-color,#1989fa);display:inline-block;margin:0 calc(var(--padding-md, 16px)*-1);padding:0 var(--padding-md,16px);vertical-align:middle}.van-nav-bar__text--hover{background-color:#f2f3f5}.van-nav-bar__arrow{color:var(--nav-bar-icon-color,#1989fa)!important;font-size:var(--nav-bar-arrow-size,16px)!important;vertical-align:middle}.van-nav-bar__arrow+.van-nav-bar__text{margin-left:-20px;padding-left:25px}.van-nav-bar--fixed{left:0;position:fixed;top:0;width:100%}.van-nav-bar__title{color:var(--nav-bar-title-text-color,#323233);font-size:var(--nav-bar-title-font-size,16px);font-weight:var(--font-weight-bold,500);margin:0 auto;max-width:60%}.van-nav-bar__left,.van-nav-bar__right{align-items:center;bottom:0;display:flex;font-size:var(--font-size-md,14px);position:absolute;top:0}.van-nav-bar__left{left:var(--padding-md,16px)}.van-nav-bar__right{right:var(--padding-md,16px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.js
new file mode 100644
index 0000000..db2a755
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.js
@@ -0,0 +1,125 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ text: {
+ type: String,
+ value: '',
+ observer: 'init',
+ },
+ mode: {
+ type: String,
+ value: '',
+ },
+ url: {
+ type: String,
+ value: '',
+ },
+ openType: {
+ type: String,
+ value: 'navigate',
+ },
+ delay: {
+ type: Number,
+ value: 1,
+ },
+ speed: {
+ type: Number,
+ value: 60,
+ observer: 'init',
+ },
+ scrollable: null,
+ leftIcon: {
+ type: String,
+ value: '',
+ },
+ color: String,
+ backgroundColor: String,
+ background: String,
+ wrapable: Boolean,
+ },
+ data: {
+ show: true,
+ },
+ created: function () {
+ this.resetAnimation = wx.createAnimation({
+ duration: 0,
+ timingFunction: 'linear',
+ });
+ },
+ destroyed: function () {
+ this.timer && clearTimeout(this.timer);
+ },
+ mounted: function () {
+ this.init();
+ },
+ methods: {
+ init: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ Promise.all([
+ (0, utils_1.getRect)(_this, '.van-notice-bar__content'),
+ (0, utils_1.getRect)(_this, '.van-notice-bar__wrap'),
+ ]).then(function (rects) {
+ var contentRect = rects[0], wrapRect = rects[1];
+ var _a = _this.data, speed = _a.speed, scrollable = _a.scrollable, delay = _a.delay;
+ if (contentRect == null ||
+ wrapRect == null ||
+ !contentRect.width ||
+ !wrapRect.width ||
+ scrollable === false) {
+ return;
+ }
+ if (scrollable || wrapRect.width < contentRect.width) {
+ var duration = ((wrapRect.width + contentRect.width) / speed) * 1000;
+ _this.wrapWidth = wrapRect.width;
+ _this.contentWidth = contentRect.width;
+ _this.duration = duration;
+ _this.animation = wx.createAnimation({
+ duration: duration,
+ timingFunction: 'linear',
+ delay: delay,
+ });
+ _this.scroll(true);
+ }
+ });
+ });
+ },
+ scroll: function (isInit) {
+ var _this = this;
+ if (isInit === void 0) { isInit = false; }
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({
+ animationData: this.resetAnimation
+ .translateX(isInit ? 0 : this.wrapWidth)
+ .step()
+ .export(),
+ });
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.setData({
+ animationData: _this.animation
+ .translateX(-_this.contentWidth)
+ .step()
+ .export(),
+ });
+ });
+ this.timer = setTimeout(function () {
+ _this.scroll();
+ }, this.duration + this.data.delay);
+ },
+ onClickIcon: function (event) {
+ if (this.data.mode === 'closeable') {
+ this.timer && clearTimeout(this.timer);
+ this.timer = null;
+ this.setData({ show: false });
+ this.$emit('close', event.detail);
+ }
+ },
+ onClick: function (event) {
+ this.$emit('click', event);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxml
new file mode 100644
index 0000000..21b0973
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxml
@@ -0,0 +1,38 @@
+
+
+
+
+
+
+
+
+
+ {{ text }}
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxs
new file mode 100644
index 0000000..11e6456
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxs
@@ -0,0 +1,15 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ color: data.color,
+ 'background-color': data.backgroundColor,
+ background: data.background,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxss
new file mode 100644
index 0000000..497636c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notice-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notice-bar{align-items:center;background-color:var(--notice-bar-background-color,#fffbe8);color:var(--notice-bar-text-color,#ed6a0c);display:flex;font-size:var(--notice-bar-font-size,14px);height:var(--notice-bar-height,40px);line-height:var(--notice-bar-line-height,24px);padding:var(--notice-bar-padding,0 16px)}.van-notice-bar--withicon{padding-right:40px;position:relative}.van-notice-bar--wrapable{height:auto;padding:var(--notice-bar-wrapable-padding,8px 16px)}.van-notice-bar--wrapable .van-notice-bar__wrap{height:auto}.van-notice-bar--wrapable .van-notice-bar__content{position:relative;white-space:normal}.van-notice-bar__left-icon{align-items:center;display:flex;margin-right:4px;vertical-align:middle}.van-notice-bar__left-icon,.van-notice-bar__right-icon{font-size:var(--notice-bar-icon-size,16px);min-width:var(--notice-bar-icon-min-width,22px)}.van-notice-bar__right-icon{position:absolute;right:15px;top:10px}.van-notice-bar__wrap{flex:1;height:var(--notice-bar-line-height,24px);overflow:hidden;position:relative}.van-notice-bar__content{position:absolute;white-space:nowrap}.van-notice-bar__content.van-ellipsis{max-width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.js
new file mode 100644
index 0000000..a9526aa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ message: String,
+ background: String,
+ type: {
+ type: String,
+ value: 'danger',
+ },
+ color: {
+ type: String,
+ value: color_1.WHITE,
+ },
+ duration: {
+ type: Number,
+ value: 3000,
+ },
+ zIndex: {
+ type: Number,
+ value: 110,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ top: null,
+ },
+ data: {
+ show: false,
+ onOpened: null,
+ onClose: null,
+ onClick: null,
+ },
+ created: function () {
+ var statusBarHeight = (0, utils_1.getSystemInfoSync)().statusBarHeight;
+ this.setData({ statusBarHeight: statusBarHeight });
+ },
+ methods: {
+ show: function () {
+ var _this = this;
+ var _a = this.data, duration = _a.duration, onOpened = _a.onOpened;
+ clearTimeout(this.timer);
+ this.setData({ show: true });
+ wx.nextTick(onOpened);
+ if (duration > 0 && duration !== Infinity) {
+ this.timer = setTimeout(function () {
+ _this.hide();
+ }, duration);
+ }
+ },
+ hide: function () {
+ var onClose = this.data.onClose;
+ clearTimeout(this.timer);
+ this.setData({ show: false });
+ wx.nextTick(onClose);
+ },
+ onTap: function (event) {
+ var onClick = this.data.onClick;
+ if (onClick) {
+ onClick(event.detail);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxml
new file mode 100644
index 0000000..42d913e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+ {{ message }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxs
new file mode 100644
index 0000000..bbb94c2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'z-index': data.zIndex,
+ top: addUnit(data.top),
+ });
+}
+
+function notifyStyle(data) {
+ return style({
+ background: data.background,
+ color: data.color,
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ notifyStyle: notifyStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxss
new file mode 100644
index 0000000..c030e9b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-notify{word-wrap:break-word;font-size:var(--notify-font-size,14px);line-height:var(--notify-line-height,20px);padding:var(--notify-padding,6px 15px);text-align:center}.van-notify__container{box-sizing:border-box;left:0;position:fixed;top:0;width:100%}.van-notify--primary{background-color:var(--notify-primary-background-color,#1989fa)}.van-notify--success{background-color:var(--notify-success-background-color,#07c160)}.van-notify--danger{background-color:var(--notify-danger-background-color,#ee0a24)}.van-notify--warning{background-color:var(--notify-warning-background-color,#ff976a)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.d.ts
new file mode 100644
index 0000000..d5213cb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.d.ts
@@ -0,0 +1,22 @@
+interface NotifyOptions {
+ type?: 'primary' | 'success' | 'danger' | 'warning';
+ color?: string;
+ zIndex?: number;
+ top?: number;
+ message: string;
+ context?: any;
+ duration?: number;
+ selector?: string;
+ background?: string;
+ safeAreaInsetTop?: boolean;
+ onClick?: () => void;
+ onOpened?: () => void;
+ onClose?: () => void;
+}
+declare function Notify(options: NotifyOptions | string): any;
+declare namespace Notify {
+ var clear: (options?: NotifyOptions | undefined) => void;
+ var setDefaultOptions: (options: NotifyOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Notify;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.js
new file mode 100644
index 0000000..d470431
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/notify/notify.js
@@ -0,0 +1,67 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var color_1 = require("../common/color");
+var defaultOptions = {
+ selector: '#van-notify',
+ type: 'danger',
+ message: '',
+ background: '',
+ duration: 3000,
+ zIndex: 110,
+ top: 0,
+ color: color_1.WHITE,
+ safeAreaInsetTop: false,
+ onClick: function () { },
+ onOpened: function () { },
+ onClose: function () { },
+};
+var currentOptions = __assign({}, defaultOptions);
+function parseOptions(message) {
+ if (message == null) {
+ return {};
+ }
+ return typeof message === 'string' ? { message: message } : message;
+}
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+function Notify(options) {
+ options = __assign(__assign({}, currentOptions), parseOptions(options));
+ var context = options.context || getContext();
+ var notify = context.selectComponent(options.selector);
+ delete options.context;
+ delete options.selector;
+ if (notify) {
+ notify.setData(options);
+ notify.show();
+ return notify;
+ }
+ console.warn('未找到 van-notify 节点,请确认 selector 及 context 是否正确');
+}
+exports.default = Notify;
+Notify.clear = function (options) {
+ options = __assign(__assign({}, defaultOptions), parseOptions(options));
+ var context = options.context || getContext();
+ var notify = context.selectComponent(options.selector);
+ if (notify) {
+ notify.hide();
+ }
+};
+Notify.setDefaultOptions = function (options) {
+ Object.assign(currentOptions, options);
+};
+Notify.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.js
new file mode 100644
index 0000000..9b58b5f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ show: Boolean,
+ customStyle: String,
+ duration: {
+ type: null,
+ value: 300,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClick: function () {
+ this.$emit('click');
+ },
+ // for prevent touchmove
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.json
new file mode 100644
index 0000000..c14a65f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxml
new file mode 100644
index 0000000..17fc56f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxml
@@ -0,0 +1,7 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxss
new file mode 100644
index 0000000..d1ad81a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-overlay{background-color:var(--overlay-background-color,rgba(0,0,0,.7));height:100%;left:0;position:fixed;top:0;width:100%}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/overlay.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/overlay.wxml
new file mode 100644
index 0000000..017e801
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/overlay/overlay.wxml
@@ -0,0 +1,10 @@
+
+
+
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.js
new file mode 100644
index 0000000..818b8c5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.js
@@ -0,0 +1,11 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['header-class', 'footer-class'],
+ props: {
+ desc: String,
+ title: String,
+ status: String,
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.json
new file mode 100644
index 0000000..0e5425c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-cell": "../cell/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxml
new file mode 100644
index 0000000..1843703
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxss
new file mode 100644
index 0000000..485edcd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/panel/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-panel{background:var(--panel-background-color,#fff)}.van-panel__header-value{color:var(--panel-header-value-color,#ee0a24)}.van-panel__footer{padding:var(--panel-footer-padding,8px 16px)}.van-panel__footer:empty{display:none}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.js
new file mode 100644
index 0000000..9dbf17c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.js
@@ -0,0 +1,122 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var DEFAULT_DURATION = 200;
+(0, component_1.VantComponent)({
+ classes: ['active-class'],
+ props: {
+ valueKey: String,
+ className: String,
+ itemHeight: Number,
+ visibleItemCount: Number,
+ initialOptions: {
+ type: Array,
+ value: [],
+ },
+ defaultIndex: {
+ type: Number,
+ value: 0,
+ observer: function (value) {
+ this.setIndex(value);
+ },
+ },
+ },
+ data: {
+ startY: 0,
+ offset: 0,
+ duration: 0,
+ startOffset: 0,
+ options: [],
+ currentIndex: 0,
+ },
+ created: function () {
+ var _this = this;
+ var _a = this.data, defaultIndex = _a.defaultIndex, initialOptions = _a.initialOptions;
+ this.set({
+ currentIndex: defaultIndex,
+ options: initialOptions,
+ }).then(function () {
+ _this.setIndex(defaultIndex);
+ });
+ },
+ methods: {
+ getCount: function () {
+ return this.data.options.length;
+ },
+ onTouchStart: function (event) {
+ this.setData({
+ startY: event.touches[0].clientY,
+ startOffset: this.data.offset,
+ duration: 0,
+ });
+ },
+ onTouchMove: function (event) {
+ var data = this.data;
+ var deltaY = event.touches[0].clientY - data.startY;
+ this.setData({
+ offset: (0, utils_1.range)(data.startOffset + deltaY, -(this.getCount() * data.itemHeight), data.itemHeight),
+ });
+ },
+ onTouchEnd: function () {
+ var data = this.data;
+ if (data.offset !== data.startOffset) {
+ this.setData({ duration: DEFAULT_DURATION });
+ var index = (0, utils_1.range)(Math.round(-data.offset / data.itemHeight), 0, this.getCount() - 1);
+ this.setIndex(index, true);
+ }
+ },
+ onClickItem: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.setIndex(index, true);
+ },
+ adjustIndex: function (index) {
+ var data = this.data;
+ var count = this.getCount();
+ index = (0, utils_1.range)(index, 0, count);
+ for (var i = index; i < count; i++) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ for (var i = index - 1; i >= 0; i--) {
+ if (!this.isDisabled(data.options[i]))
+ return i;
+ }
+ },
+ isDisabled: function (option) {
+ return (0, validator_1.isObj)(option) && option.disabled;
+ },
+ getOptionText: function (option) {
+ var data = this.data;
+ return (0, validator_1.isObj)(option) && data.valueKey in option
+ ? option[data.valueKey]
+ : option;
+ },
+ setIndex: function (index, userAction) {
+ var _this = this;
+ var data = this.data;
+ index = this.adjustIndex(index) || 0;
+ var offset = -index * data.itemHeight;
+ if (index !== data.currentIndex) {
+ return this.set({ offset: offset, currentIndex: index }).then(function () {
+ userAction && _this.$emit('change', index);
+ });
+ }
+ return this.set({ offset: offset });
+ },
+ setValue: function (value) {
+ var options = this.data.options;
+ for (var i = 0; i < options.length; i++) {
+ if (this.getOptionText(options[i]) === value) {
+ return this.setIndex(i);
+ }
+ }
+ return Promise.resolve();
+ },
+ getValue: function () {
+ var data = this.data;
+ return data.options[data.currentIndex];
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxml
new file mode 100644
index 0000000..f2c8da2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxml
@@ -0,0 +1,23 @@
+
+
+
+
+
+ {{ computed.optionText(option, valueKey) }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxs
new file mode 100644
index 0000000..2d5a611
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function isObj(x) {
+ var type = typeof x;
+ return x !== null && (type === 'object' || type === 'function');
+}
+
+function optionText(option, valueKey) {
+ return isObj(option) && option[valueKey] != null ? option[valueKey] : option;
+}
+
+function rootStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function wrapperStyle(data) {
+ var offset = addUnit(
+ data.offset + (data.itemHeight * (data.visibleItemCount - 1)) / 2
+ );
+
+ return style({
+ transition: 'transform ' + data.duration + 'ms',
+ 'line-height': addUnit(data.itemHeight),
+ transform: 'translate3d(0, ' + offset + ', 0)',
+ });
+}
+
+module.exports = {
+ optionText: optionText,
+ rootStyle: rootStyle,
+ wrapperStyle: wrapperStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxss
new file mode 100644
index 0000000..519a438
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker-column/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker-column{color:var(--picker-option-text-color,#000);font-size:var(--picker-option-font-size,16px);overflow:hidden;text-align:center}.van-picker-column__item{padding:0 5px}.van-picker-column__item--selected{color:var(--picker-option-selected-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-picker-column__item--disabled{opacity:var(--picker-option-disabled-opacity,.3)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.js
new file mode 100644
index 0000000..06d1826
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.js
@@ -0,0 +1,161 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var shared_1 = require("./shared");
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'toolbar-class', 'column-class'],
+ props: __assign(__assign({}, shared_1.pickerProps), { valueKey: {
+ type: String,
+ value: 'text',
+ }, toolbarPosition: {
+ type: String,
+ value: 'top',
+ }, defaultIndex: {
+ type: Number,
+ value: 0,
+ }, columns: {
+ type: Array,
+ value: [],
+ observer: function (columns) {
+ if (columns === void 0) { columns = []; }
+ this.simple = columns.length && !columns[0].values;
+ if (Array.isArray(this.children) && this.children.length) {
+ this.setColumns().catch(function () { });
+ }
+ },
+ } }),
+ beforeCreate: function () {
+ var _this = this;
+ Object.defineProperty(this, 'children', {
+ get: function () { return _this.selectAllComponents('.van-picker__column') || []; },
+ });
+ },
+ methods: {
+ noop: function () { },
+ setColumns: function () {
+ var _this = this;
+ var data = this.data;
+ var columns = this.simple ? [{ values: data.columns }] : data.columns;
+ var stack = columns.map(function (column, index) {
+ return _this.setColumnValues(index, column.values);
+ });
+ return Promise.all(stack);
+ },
+ emit: function (event) {
+ var type = event.currentTarget.dataset.type;
+ if (this.simple) {
+ this.$emit(type, {
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit(type, {
+ value: this.getValues(),
+ index: this.getIndexes(),
+ });
+ }
+ },
+ onChange: function (event) {
+ if (this.simple) {
+ this.$emit('change', {
+ picker: this,
+ value: this.getColumnValue(0),
+ index: this.getColumnIndex(0),
+ });
+ }
+ else {
+ this.$emit('change', {
+ picker: this,
+ value: this.getValues(),
+ index: event.currentTarget.dataset.index,
+ });
+ }
+ },
+ // get column instance by index
+ getColumn: function (index) {
+ return this.children[index];
+ },
+ // get column value by index
+ getColumnValue: function (index) {
+ var column = this.getColumn(index);
+ return column && column.getValue();
+ },
+ // set column value by index
+ setColumnValue: function (index, value) {
+ var column = this.getColumn(index);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValue: 对应列不存在'));
+ }
+ return column.setValue(value);
+ },
+ // get column option index by column index
+ getColumnIndex: function (columnIndex) {
+ return (this.getColumn(columnIndex) || {}).data.currentIndex;
+ },
+ // set column option index by column index
+ setColumnIndex: function (columnIndex, optionIndex) {
+ var column = this.getColumn(columnIndex);
+ if (column == null) {
+ return Promise.reject(new Error('setColumnIndex: 对应列不存在'));
+ }
+ return column.setIndex(optionIndex);
+ },
+ // get options of column by index
+ getColumnValues: function (index) {
+ return (this.children[index] || {}).data.options;
+ },
+ // set options of column by index
+ setColumnValues: function (index, options, needReset) {
+ if (needReset === void 0) { needReset = true; }
+ var column = this.children[index];
+ if (column == null) {
+ return Promise.reject(new Error('setColumnValues: 对应列不存在'));
+ }
+ var isSame = JSON.stringify(column.data.options) === JSON.stringify(options);
+ if (isSame) {
+ return Promise.resolve();
+ }
+ return column.set({ options: options }).then(function () {
+ if (needReset) {
+ column.setIndex(0);
+ }
+ });
+ },
+ // get values of all columns
+ getValues: function () {
+ return this.children.map(function (child) { return child.getValue(); });
+ },
+ // set values of all columns
+ setValues: function (values) {
+ var _this = this;
+ var stack = values.map(function (value, index) {
+ return _this.setColumnValue(index, value);
+ });
+ return Promise.all(stack);
+ },
+ // get indexes of all columns
+ getIndexes: function () {
+ return this.children.map(function (child) { return child.data.currentIndex; });
+ },
+ // set indexes of all columns
+ setIndexes: function (indexes) {
+ var _this = this;
+ var stack = indexes.map(function (optionIndex, columnIndex) {
+ return _this.setColumnIndex(columnIndex, optionIndex);
+ });
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.json
new file mode 100644
index 0000000..2fcec89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "picker-column": "../picker-column/index",
+ "loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxml
new file mode 100644
index 0000000..8564ccc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxml
@@ -0,0 +1,37 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxs
new file mode 100644
index 0000000..0abbd10
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+var array = require('../wxs/array.wxs');
+
+function columnsStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight * data.visibleItemCount),
+ });
+}
+
+function maskStyle(data) {
+ return style({
+ 'background-size':
+ '100% ' + addUnit((data.itemHeight * (data.visibleItemCount - 1)) / 2),
+ });
+}
+
+function frameStyle(data) {
+ return style({
+ height: addUnit(data.itemHeight),
+ });
+}
+
+function columns(columns) {
+ if (!array.isArray(columns)) {
+ return [];
+ }
+
+ if (columns.length && !columns[0].values) {
+ return [{ values: columns }];
+ }
+
+ return columns;
+}
+
+module.exports = {
+ columnsStyle: columnsStyle,
+ frameStyle: frameStyle,
+ maskStyle: maskStyle,
+ columns: columns,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxss
new file mode 100644
index 0000000..d924abb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-picker{-webkit-text-size-adjust:100%;background-color:var(--picker-background-color,#fff);overflow:hidden;position:relative;-webkit-user-select:none;user-select:none}.van-picker__toolbar{display:flex;height:var(--picker-toolbar-height,44px);justify-content:space-between;line-height:var(--picker-toolbar-height,44px)}.van-picker__cancel,.van-picker__confirm{font-size:var(--picker-action-font-size,14px);padding:var(--picker-action-padding,0 16px)}.van-picker__cancel--hover,.van-picker__confirm--hover{opacity:.7}.van-picker__confirm{color:var(--picker-confirm-action-color,#576b95)}.van-picker__cancel{color:var(--picker-cancel-action-color,#969799)}.van-picker__title{font-size:var(--picker-option-font-size,16px);font-weight:var(--font-weight-bold,500);max-width:50%;text-align:center}.van-picker__columns{display:flex;position:relative}.van-picker__column{flex:1 1;width:0}.van-picker__loading{align-items:center;background-color:var(--picker-loading-mask-color,hsla(0,0%,100%,.9));bottom:0;display:flex;justify-content:center;left:0;position:absolute;right:0;top:0;z-index:4}.van-picker__mask{-webkit-backface-visibility:hidden;backface-visibility:hidden;background-image:linear-gradient(180deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4)),linear-gradient(0deg,hsla(0,0%,100%,.9),hsla(0,0%,100%,.4));background-position:top,bottom;background-repeat:no-repeat;height:100%;left:0;top:0;width:100%;z-index:2}.van-picker__frame,.van-picker__mask{pointer-events:none;position:absolute}.van-picker__frame{left:16px;right:16px;top:50%;transform:translateY(-50%);z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.d.ts
new file mode 100644
index 0000000..c548045
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.d.ts
@@ -0,0 +1,21 @@
+export declare const pickerProps: {
+ title: StringConstructor;
+ loading: BooleanConstructor;
+ showToolbar: BooleanConstructor;
+ cancelButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ confirmButtonText: {
+ type: StringConstructor;
+ value: string;
+ };
+ visibleItemCount: {
+ type: NumberConstructor;
+ value: number;
+ };
+ itemHeight: {
+ type: NumberConstructor;
+ value: number;
+ };
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.js
new file mode 100644
index 0000000..3d40a8c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/shared.js
@@ -0,0 +1,24 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.pickerProps = void 0;
+exports.pickerProps = {
+ title: String,
+ loading: Boolean,
+ showToolbar: Boolean,
+ cancelButtonText: {
+ type: String,
+ value: '取消',
+ },
+ confirmButtonText: {
+ type: String,
+ value: '确认',
+ },
+ visibleItemCount: {
+ type: Number,
+ value: 6,
+ },
+ itemHeight: {
+ type: Number,
+ value: 44,
+ },
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/toolbar.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/toolbar.wxml
new file mode 100644
index 0000000..414f612
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/picker/toolbar.wxml
@@ -0,0 +1,23 @@
+
+
+ {{ cancelButtonText }}
+
+ {{
+ title
+ }}
+
+ {{ confirmButtonText }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.js
new file mode 100644
index 0000000..18b08e6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.js
@@ -0,0 +1,99 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var transition_1 = require("../mixins/transition");
+(0, component_1.VantComponent)({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ 'close-icon-class',
+ ],
+ mixins: [(0, transition_1.transition)(false)],
+ props: {
+ round: Boolean,
+ closeable: Boolean,
+ customStyle: String,
+ overlayStyle: String,
+ transition: {
+ type: String,
+ observer: 'observeClass',
+ },
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ closeIcon: {
+ type: String,
+ value: 'cross',
+ },
+ closeIconPosition: {
+ type: String,
+ value: 'top-right',
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ position: {
+ type: String,
+ value: 'center',
+ observer: 'observeClass',
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetTop: {
+ type: Boolean,
+ value: false,
+ },
+ safeAreaTabBar: {
+ type: Boolean,
+ value: false,
+ },
+ lockScroll: {
+ type: Boolean,
+ value: true,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ created: function () {
+ this.observeClass();
+ },
+ methods: {
+ onClickCloseIcon: function () {
+ this.$emit('close');
+ },
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ if (this.data.closeOnClickOverlay) {
+ this.$emit('close');
+ }
+ },
+ observeClass: function () {
+ var _a = this.data, transition = _a.transition, position = _a.position, duration = _a.duration;
+ var updateData = {
+ name: transition || position,
+ };
+ if (transition === 'none') {
+ updateData.duration = 0;
+ this.originDuration = duration;
+ }
+ else if (this.originDuration != null) {
+ updateData.duration = this.originDuration;
+ }
+ this.setData(updateData);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.json
new file mode 100644
index 0000000..88a6eab
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-overlay": "../overlay/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxml
new file mode 100644
index 0000000..ab824b1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxml
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxs
new file mode 100644
index 0000000..8d59f24
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function popupStyle(data) {
+ return style([
+ {
+ 'z-index': data.zIndex,
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ popupStyle: popupStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxss
new file mode 100644
index 0000000..91983b4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-popup{-webkit-overflow-scrolling:touch;animation:ease both;background-color:var(--popup-background-color,#fff);box-sizing:border-box;max-height:100%;overflow-y:auto;position:fixed;transition-timing-function:ease}.van-popup--center{left:50%;top:50%;transform:translate3d(-50%,-50%,0)}.van-popup--center.van-popup--round{border-radius:var(--popup-round-border-radius,16px)}.van-popup--top{left:0;top:0;width:100%}.van-popup--top.van-popup--round{border-radius:0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--right{right:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--right.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px))}.van-popup--bottom{bottom:0;left:0;width:100%}.van-popup--bottom.van-popup--round{border-radius:var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0 0}.van-popup--left{left:0;top:50%;transform:translate3d(0,-50%,0)}.van-popup--left.van-popup--round{border-radius:0 var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) var(--popup-round-border-radius,var(--popup-round-border-radius,16px)) 0}.van-popup--bottom.van-popup--safe{padding-bottom:env(safe-area-inset-bottom)}.van-popup--bottom.van-popup--safeTabBar,.van-popup--top.van-popup--safeTabBar{bottom:var(--tabbar-height,50px)}.van-popup--safeTop{padding-top:env(safe-area-inset-top)}.van-popup__close-icon{color:var(--popup-close-icon-color,#969799);font-size:var(--popup-close-icon-size,18px);position:absolute;z-index:var(--popup-close-icon-z-index,1)}.van-popup__close-icon--top-left{left:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--top-right{right:var(--popup-close-icon-margin,16px);top:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-left{bottom:var(--popup-close-icon-margin,16px);left:var(--popup-close-icon-margin,16px)}.van-popup__close-icon--bottom-right{bottom:var(--popup-close-icon-margin,16px);right:var(--popup-close-icon-margin,16px)}.van-popup__close-icon:active{opacity:.6}.van-scale-enter-active,.van-scale-leave-active{transition-property:opacity,transform}.van-scale-enter,.van-scale-leave-to{opacity:0;transform:translate3d(-50%,-50%,0) scale(.7)}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-center-enter-active,.van-center-leave-active{transition-property:opacity}.van-center-enter,.van-center-leave-to{opacity:0}.van-bottom-enter-active,.van-bottom-leave-active,.van-left-enter-active,.van-left-leave-active,.van-right-enter-active,.van-right-leave-active,.van-top-enter-active,.van-top-leave-active{transition-property:transform}.van-bottom-enter,.van-bottom-leave-to{transform:translate3d(0,100%,0)}.van-top-enter,.van-top-leave-to{transform:translate3d(0,-100%,0)}.van-left-enter,.van-left-leave-to{transform:translate3d(-100%,-50%,0)}.van-right-enter,.van-right-leave-to{transform:translate3d(100%,-50%,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/popup.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/popup.wxml
new file mode 100644
index 0000000..bd8f508
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/popup/popup.wxml
@@ -0,0 +1,16 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.js
new file mode 100644
index 0000000..3bca928
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.js
@@ -0,0 +1,55 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ props: {
+ inactive: Boolean,
+ percentage: {
+ type: Number,
+ observer: 'setLeft',
+ },
+ pivotText: String,
+ pivotColor: String,
+ trackColor: String,
+ showPivot: {
+ type: Boolean,
+ value: true,
+ },
+ color: {
+ type: String,
+ value: color_1.BLUE,
+ },
+ textColor: {
+ type: String,
+ value: '#fff',
+ },
+ strokeWidth: {
+ type: null,
+ value: 4,
+ },
+ },
+ data: {
+ right: 0,
+ },
+ mounted: function () {
+ this.setLeft();
+ },
+ methods: {
+ setLeft: function () {
+ var _this = this;
+ Promise.all([
+ (0, utils_1.getRect)(this, '.van-progress'),
+ (0, utils_1.getRect)(this, '.van-progress__pivot'),
+ ]).then(function (_a) {
+ var portion = _a[0], pivot = _a[1];
+ if (portion && pivot) {
+ _this.setData({
+ right: (pivot.width * (_this.data.percentage - 100)) / 100,
+ });
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxml
new file mode 100644
index 0000000..e81514d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+ {{ computed.pivotText(pivotText, percentage) }}
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxs
new file mode 100644
index 0000000..5b1e8e6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxs
@@ -0,0 +1,36 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function pivotText(pivotText, percentage) {
+ return pivotText || percentage + '%';
+}
+
+function rootStyle(data) {
+ return style({
+ 'height': data.strokeWidth ? utils.addUnit(data.strokeWidth) : '',
+ 'background': data.trackColor,
+ });
+}
+
+function portionStyle(data) {
+ return style({
+ background: data.inactive ? '#cacaca' : data.color,
+ width: data.percentage ? data.percentage + '%' : '',
+ });
+}
+
+function pivotStyle(data) {
+ return style({
+ color: data.textColor,
+ right: data.right + 'px',
+ background: data.pivotColor ? data.pivotColor : data.inactive ? '#cacaca' : data.color,
+ });
+}
+
+module.exports = {
+ pivotText: pivotText,
+ rootStyle: rootStyle,
+ portionStyle: portionStyle,
+ pivotStyle: pivotStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxss
new file mode 100644
index 0000000..a08972a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/progress/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-progress{background:var(--progress-background-color,#ebedf0);border-radius:var(--progress-height,4px);height:var(--progress-height,4px);position:relative}.van-progress__portion{background:var(--progress-color,#1989fa);border-radius:inherit;height:100%;left:0;position:absolute}.van-progress__pivot{background-color:var(--progress-pivot-background-color,#1989fa);border-radius:1em;box-sizing:border-box;color:var(--progress-pivot-text-color,#fff);font-size:var(--progress-pivot-font-size,10px);line-height:var(--progress-pivot-line-height,1.6);min-width:3.6em;padding:var(--progress-pivot-padding,0 5px);position:absolute;text-align:center;top:50%;transform:translateY(-50%);word-break:keep-all}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.js
new file mode 100644
index 0000000..ddb2a60
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.js
@@ -0,0 +1,24 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useChildren)('radio'),
+ props: {
+ value: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ direction: String,
+ disabled: {
+ type: Boolean,
+ observer: 'updateChildren',
+ },
+ },
+ methods: {
+ updateChildren: function () {
+ this.children.forEach(function (child) { return child.updateFromParent(); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxml
new file mode 100644
index 0000000..0ab17af
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxss
new file mode 100644
index 0000000..4e3b5d4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio-group/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio-group--horizontal{display:flex;flex-wrap:wrap}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.js
new file mode 100644
index 0000000..61a86d5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var version_1 = require("../common/version");
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ field: true,
+ relation: (0, relation_1.useParent)('radio-group', function () {
+ this.updateFromParent();
+ }),
+ classes: ['icon-class', 'label-class'],
+ props: {
+ name: null,
+ value: null,
+ disabled: Boolean,
+ useIconSlot: Boolean,
+ checkedColor: String,
+ labelPosition: {
+ type: String,
+ value: 'right',
+ },
+ labelDisabled: Boolean,
+ shape: {
+ type: String,
+ value: 'round',
+ },
+ iconSize: {
+ type: null,
+ value: 20,
+ },
+ },
+ data: {
+ direction: '',
+ parentDisabled: false,
+ },
+ methods: {
+ updateFromParent: function () {
+ if (!this.parent) {
+ return;
+ }
+ var _a = this.parent.data, value = _a.value, parentDisabled = _a.disabled, direction = _a.direction;
+ this.setData({
+ value: value,
+ direction: direction,
+ parentDisabled: parentDisabled,
+ });
+ },
+ emitChange: function (value) {
+ var instance = this.parent || this;
+ instance.$emit('input', value);
+ instance.$emit('change', value);
+ if ((0, version_1.canIUseModel)()) {
+ instance.setData({ value: value });
+ }
+ },
+ onChange: function () {
+ if (!this.data.disabled && !this.data.parentDisabled) {
+ this.emitChange(this.data.name);
+ }
+ },
+ onClickLabel: function () {
+ var _a = this.data, disabled = _a.disabled, parentDisabled = _a.parentDisabled, labelDisabled = _a.labelDisabled, name = _a.name;
+ if (!(disabled || parentDisabled) && !labelDisabled) {
+ this.emitChange(name);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxml
new file mode 100644
index 0000000..5f898c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxml
@@ -0,0 +1,30 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxs
new file mode 100644
index 0000000..a428aad
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxs
@@ -0,0 +1,33 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function iconStyle(data) {
+ var styles = {
+ 'font-size': addUnit(data.iconSize),
+ };
+
+ if (
+ data.checkedColor &&
+ !(data.disabled || data.parentDisabled) &&
+ data.value === data.name
+ ) {
+ styles['border-color'] = data.checkedColor;
+ styles['background-color'] = data.checkedColor;
+ }
+
+ return style(styles);
+}
+
+function iconCustomStyle(data) {
+ return style({
+ 'line-height': addUnit(data.iconSize),
+ 'font-size': '.8em',
+ display: 'block',
+ });
+}
+
+module.exports = {
+ iconStyle: iconStyle,
+ iconCustomStyle: iconCustomStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxss
new file mode 100644
index 0000000..257b0c7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/radio/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-radio{align-items:center;display:flex;overflow:hidden;-webkit-user-select:none;user-select:none}.van-radio__icon-wrap{flex:none}.van-radio--horizontal{margin-right:var(--padding-sm,12px)}.van-radio__icon{align-items:center;border:1px solid var(--radio-border-color,#c8c9cc);box-sizing:border-box;color:transparent;display:flex;font-size:var(--radio-size,20px);height:1em;justify-content:center;text-align:center;transition-duration:var(--radio-transition-duration,.2s);transition-property:color,border-color,background-color;width:1em}.van-radio__icon--round{border-radius:100%}.van-radio__icon--checked{background-color:var(--radio-checked-icon-color,#1989fa);border-color:var(--radio-checked-icon-color,#1989fa);color:#fff}.van-radio__icon--disabled{background-color:var(--radio-disabled-background-color,#ebedf0);border-color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__icon--disabled.van-radio__icon--checked{color:var(--radio-disabled-icon-color,#c8c9cc)}.van-radio__label{word-wrap:break-word;color:var(--radio-label-color,#323233);line-height:var(--radio-size,20px);padding-left:var(--radio-label-margin,10px)}.van-radio__label--left{float:left;margin:0 var(--radio-label-margin,10px) 0 0}.van-radio__label--disabled{color:var(--radio-disabled-label-color,#c8c9cc)}.van-radio__label:empty{margin:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.js
new file mode 100644
index 0000000..30a96de
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.js
@@ -0,0 +1,93 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var version_1 = require("../common/version");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['icon-class'],
+ props: {
+ value: {
+ type: Number,
+ observer: function (value) {
+ if (value !== this.data.innerValue) {
+ this.setData({ innerValue: value });
+ }
+ },
+ },
+ readonly: Boolean,
+ disabled: Boolean,
+ allowHalf: Boolean,
+ size: null,
+ icon: {
+ type: String,
+ value: 'star',
+ },
+ voidIcon: {
+ type: String,
+ value: 'star-o',
+ },
+ color: String,
+ voidColor: String,
+ disabledColor: String,
+ count: {
+ type: Number,
+ value: 5,
+ observer: function (value) {
+ this.setData({ innerCountArray: Array.from({ length: value }) });
+ },
+ },
+ gutter: null,
+ touchable: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ innerValue: 0,
+ innerCountArray: Array.from({ length: 5 }),
+ },
+ methods: {
+ onSelect: function (event) {
+ var _this = this;
+ var data = this.data;
+ var score = event.currentTarget.dataset.score;
+ if (!data.disabled && !data.readonly) {
+ this.setData({ innerValue: score + 1 });
+ if ((0, version_1.canIUseModel)()) {
+ this.setData({ value: score + 1 });
+ }
+ wx.nextTick(function () {
+ _this.$emit('input', score + 1);
+ _this.$emit('change', score + 1);
+ });
+ }
+ },
+ onTouchMove: function (event) {
+ var _this = this;
+ var touchable = this.data.touchable;
+ if (!touchable)
+ return;
+ var clientX = event.touches[0].clientX;
+ (0, utils_1.getAllRect)(this, '.van-rate__icon').then(function (list) {
+ var target = list
+ .sort(function (cur, next) { return cur.dataset.score - next.dataset.score; })
+ .find(function (item) { return clientX >= item.left && clientX <= item.right; });
+ if (target != null) {
+ _this.onSelect(__assign(__assign({}, event), { currentTarget: target }));
+ }
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxml
new file mode 100644
index 0000000..049714c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxml
@@ -0,0 +1,35 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxss
new file mode 100644
index 0000000..470e4f4
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/rate/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-rate{display:inline-flex;-webkit-user-select:none;user-select:none}.van-rate__item{padding:0 var(--rate-horizontal-padding,2px);position:relative}.van-rate__item:not(:last-child){padding-right:var(--rate-icon-gutter,4px)}.van-rate__icon{color:var(--rate-icon-void-color,#c8c9cc);display:block;font-size:var(--rate-icon-size,20px);height:100%}.van-rate__icon--half{left:var(--rate-horizontal-padding,2px);overflow:hidden;position:absolute;top:0;width:.5em}.van-rate__icon--full,.van-rate__icon--half{color:var(--rate-icon-full-color,#ee0a24)}.van-rate__icon--disabled{color:var(--rate-icon-disabled-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.js
new file mode 100644
index 0000000..c27acd6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.js
@@ -0,0 +1,26 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('col', function (target) {
+ var gutter = this.data.gutter;
+ if (gutter) {
+ target.setData({ gutter: gutter });
+ }
+ }),
+ props: {
+ gutter: {
+ type: Number,
+ observer: 'setGutter',
+ },
+ },
+ methods: {
+ setGutter: function () {
+ var _this = this;
+ this.children.forEach(function (col) {
+ col.setData(_this.data);
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxml
new file mode 100644
index 0000000..69a4359
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxml
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxs
new file mode 100644
index 0000000..f5c5958
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxs
@@ -0,0 +1,18 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ if (!data.gutter) {
+ return '';
+ }
+
+ return style({
+ 'margin-right': addUnit(-data.gutter / 2),
+ 'margin-left': addUnit(-data.gutter / 2),
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxss
new file mode 100644
index 0000000..bb8946b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/row/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-row:after{clear:both;content:"";display:table}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.js
new file mode 100644
index 0000000..b5348b0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.js
@@ -0,0 +1,100 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var version_1 = require("../common/version");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['field-class', 'input-class', 'cancel-class'],
+ props: {
+ value: {
+ type: String,
+ value: '',
+ },
+ label: String,
+ focus: Boolean,
+ error: Boolean,
+ disabled: Boolean,
+ readonly: Boolean,
+ inputAlign: String,
+ showAction: Boolean,
+ useActionSlot: Boolean,
+ useLeftIconSlot: Boolean,
+ useRightIconSlot: Boolean,
+ leftIcon: {
+ type: String,
+ value: 'search',
+ },
+ rightIcon: String,
+ placeholder: String,
+ placeholderStyle: String,
+ actionText: {
+ type: String,
+ value: '取消',
+ },
+ background: {
+ type: String,
+ value: '#ffffff',
+ },
+ maxlength: {
+ type: Number,
+ value: -1,
+ },
+ shape: {
+ type: String,
+ value: 'square',
+ },
+ clearable: {
+ type: Boolean,
+ value: true,
+ },
+ clearTrigger: {
+ type: String,
+ value: 'focus',
+ },
+ clearIcon: {
+ type: String,
+ value: 'clear',
+ },
+ cursorSpacing: {
+ type: Number,
+ value: 0,
+ },
+ },
+ methods: {
+ onChange: function (event) {
+ if ((0, version_1.canIUseModel)()) {
+ this.setData({ value: event.detail });
+ }
+ this.$emit('change', event.detail);
+ },
+ onCancel: function () {
+ var _this = this;
+ /**
+ * 修复修改输入框值时,输入框失焦和赋值同时触发,赋值失效
+ * https://github.com/youzan/vant-weapp/issues/1768
+ */
+ setTimeout(function () {
+ if ((0, version_1.canIUseModel)()) {
+ _this.setData({ value: '' });
+ }
+ _this.$emit('cancel');
+ _this.$emit('change', '');
+ }, 200);
+ },
+ onSearch: function (event) {
+ this.$emit('search', event.detail);
+ },
+ onFocus: function (event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ this.$emit('blur', event.detail);
+ },
+ onClear: function (event) {
+ this.$emit('clear', event.detail);
+ },
+ onClickInput: function (event) {
+ this.$emit('click-input', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.json
new file mode 100644
index 0000000..b4cfe91
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-field": "../field/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxml
new file mode 100644
index 0000000..cbcb47a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+ {{ label }}
+
+
+
+
+
+
+
+
+
+
+ {{ actionText }}
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxss
new file mode 100644
index 0000000..4f306b0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/search/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-search{align-items:center;box-sizing:border-box;display:flex;padding:var(--search-padding,10px 12px)}.van-search__content{background-color:var(--search-background-color,#f7f8fa);border-radius:2px;display:flex;flex:1;padding-left:var(--padding-sm,12px)}.van-search__content--round{border-radius:999px}.van-search__label{color:var(--search-label-color,#323233);font-size:var(--search-label-font-size,14px);line-height:var(--search-input-height,34px);padding:var(--search-label-padding,0 5px)}.van-search__field{flex:1}.van-search__field__left-icon{color:var(--search-left-icon-color,#969799)}.van-search--withaction{padding-right:0}.van-search__action{color:var(--search-action-text-color,#323233);font-size:var(--search-action-font-size,14px);line-height:var(--search-input-height,34px)}.van-search__action--hover{background-color:#f2f3f5}.van-search__action-button{padding:var(--search-action-padding,0 8px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.js
new file mode 100644
index 0000000..11604a7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.js
@@ -0,0 +1,61 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ // whether to show popup
+ show: Boolean,
+ // overlay custom style
+ overlayStyle: String,
+ // z-index
+ zIndex: {
+ type: Number,
+ value: 100,
+ },
+ title: String,
+ cancelText: {
+ type: String,
+ value: '取消',
+ },
+ description: String,
+ options: {
+ type: Array,
+ value: [],
+ },
+ overlay: {
+ type: Boolean,
+ value: true,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ closeOnClickOverlay: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: null,
+ value: 300,
+ },
+ rootPortal: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ methods: {
+ onClickOverlay: function () {
+ this.$emit('click-overlay');
+ },
+ onCancel: function () {
+ this.onClose();
+ this.$emit('cancel');
+ },
+ onSelect: function (event) {
+ this.$emit('select', event.detail);
+ },
+ onClose: function () {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.json
new file mode 100644
index 0000000..15a7c22
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-popup": "../popup/index",
+ "options": "./options"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxml
new file mode 100644
index 0000000..72a5b25
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxml
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxs
new file mode 100644
index 0000000..2149ee9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+function isMulti(options) {
+ if (options == null || options[0] == null) {
+ return false;
+ }
+
+ return "Array" === options.constructor && "Array" === options[0].constructor;
+}
+
+module.exports = {
+ isMulti: isMulti
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxss
new file mode 100644
index 0000000..e8d8dae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__header{padding:12px 16px 4px;text-align:center}.van-share-sheet__title{color:#323233;font-size:14px;font-weight:400;line-height:20px;margin-top:8px}.van-share-sheet__title:empty,.van-share-sheet__title:not(:empty)+.van-share-sheet__title{display:none}.van-share-sheet__description{color:#969799;display:block;font-size:12px;line-height:16px;margin-top:8px}.van-share-sheet__description:empty,.van-share-sheet__description:not(:empty)+.van-share-sheet__description{display:none}.van-share-sheet__cancel{background:#fff;border:none;box-sizing:initial;display:block;font-size:16px;height:auto;line-height:48px;padding:0;text-align:center;width:100%}.van-share-sheet__cancel:before{background-color:#f7f8fa;content:" ";display:block;height:8px}.van-share-sheet__cancel:after{display:none}.van-share-sheet__cancel:active{background-color:#f2f3f5}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.js
new file mode 100644
index 0000000..0432d4f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.js
@@ -0,0 +1,27 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ options: Array,
+ showBorder: Boolean,
+ },
+ methods: {
+ onSelect: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var option = this.data.options[index];
+ this.$emit('select', __assign(__assign({}, option), { index: index }));
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxml
new file mode 100644
index 0000000..2983ebb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxs
new file mode 100644
index 0000000..a116d32
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var PRESET_ICONS = ['qq', 'link', 'weibo', 'wechat', 'poster', 'qrcode', 'weapp-qrcode', 'wechat-moments'];
+
+function getIconURL(icon) {
+ if (PRESET_ICONS.indexOf(icon) !== -1) {
+ return 'https://img.yzcdn.cn/vant/share-sheet-' + icon + '.png';
+ }
+
+ return icon;
+}
+
+module.exports = {
+ getIconURL: getIconURL,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxss
new file mode 100644
index 0000000..b7f5455
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/share-sheet/options.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-share-sheet__options{-webkit-overflow-scrolling:touch;display:flex;overflow-x:auto;overflow-y:visible;padding:16px 0 16px 8px;position:relative}.van-share-sheet__options--border:before{border-top:1px solid #ebedf0;box-sizing:border-box;content:" ";left:16px;pointer-events:none;position:absolute;right:0;top:0;transform:scaleY(.5);transform-origin:center}.van-share-sheet__options::-webkit-scrollbar{height:0}.van-share-sheet__option{align-items:center;display:flex;flex-direction:column;-webkit-user-select:none;user-select:none}.van-share-sheet__option:active{opacity:.7}.van-share-sheet__button{background-color:initial;border:0;height:auto;line-height:inherit;padding:0}.van-share-sheet__button:after{border:0}.van-share-sheet__icon{height:48px;margin:0 16px;width:48px}.van-share-sheet__name{color:#646566;font-size:12px;margin-top:8px;padding:0 4px}.van-share-sheet__option-description{color:#c8c9cc;font-size:12px;padding:0 4px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.js
new file mode 100644
index 0000000..eac568f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.js
@@ -0,0 +1,32 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ classes: ['active-class', 'disabled-class'],
+ relation: (0, relation_1.useParent)('sidebar'),
+ props: {
+ dot: Boolean,
+ badge: null,
+ info: null,
+ title: String,
+ disabled: Boolean,
+ },
+ methods: {
+ onClick: function () {
+ var _this = this;
+ var parent = this.parent;
+ if (!parent || this.data.disabled) {
+ return;
+ }
+ var index = parent.children.indexOf(this);
+ parent.setActive(index).then(function () {
+ _this.$emit('click', index);
+ parent.$emit('change', index);
+ });
+ },
+ setActive: function (selected) {
+ return this.setData({ selected: selected });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.json
new file mode 100644
index 0000000..bf0ebe0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxml
new file mode 100644
index 0000000..c5c08a6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxml
@@ -0,0 +1,18 @@
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxss
new file mode 100644
index 0000000..f1ce421
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar-item{background-color:var(--sidebar-background-color,#f7f8fa);border-left:3px solid transparent;box-sizing:border-box;color:var(--sidebar-text-color,#323233);display:block;font-size:var(--sidebar-font-size,14px);line-height:var(--sidebar-line-height,20px);overflow:hidden;padding:var(--sidebar-padding,20px 12px 20px 8px);-webkit-user-select:none;user-select:none}.van-sidebar-item__text{display:inline-block;position:relative;word-break:break-all}.van-sidebar-item--hover:not(.van-sidebar-item--disabled){background-color:var(--sidebar-active-color,#f2f3f5)}.van-sidebar-item:after{border-bottom-width:1px}.van-sidebar-item--selected{border-color:var(--sidebar-selected-border-color,#ee0a24);color:var(--sidebar-selected-text-color,#323233);font-weight:var(--sidebar-selected-font-weight,500)}.van-sidebar-item--selected:after{border-right-width:1px}.van-sidebar-item--selected,.van-sidebar-item--selected.van-sidebar-item--hover{background-color:var(--sidebar-selected-background-color,#fff)}.van-sidebar-item--disabled{color:var(--sidebar-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.js
new file mode 100644
index 0000000..f3e0a58
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.js
@@ -0,0 +1,36 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('sidebar-item', function () {
+ this.setActive(this.data.activeKey);
+ }),
+ props: {
+ activeKey: {
+ type: Number,
+ value: 0,
+ observer: 'setActive',
+ },
+ },
+ beforeCreate: function () {
+ this.currentActive = -1;
+ },
+ methods: {
+ setActive: function (activeKey) {
+ var _a = this, children = _a.children, currentActive = _a.currentActive;
+ if (!children.length) {
+ return Promise.resolve();
+ }
+ this.currentActive = activeKey;
+ var stack = [];
+ if (currentActive !== activeKey && children[currentActive]) {
+ stack.push(children[currentActive].setActive(false));
+ }
+ if (children[activeKey]) {
+ stack.push(children[activeKey].setActive(true));
+ }
+ return Promise.all(stack);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxml
new file mode 100644
index 0000000..96b11c7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxml
@@ -0,0 +1,3 @@
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxss
new file mode 100644
index 0000000..5a2d44f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sidebar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sidebar{width:var(--sidebar-width,80px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.js
new file mode 100644
index 0000000..2ab3175
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.js
@@ -0,0 +1,48 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['avatar-class', 'title-class', 'row-class'],
+ props: {
+ row: {
+ type: Number,
+ value: 0,
+ observer: function (value) {
+ this.setData({ rowArray: Array.from({ length: value }) });
+ },
+ },
+ title: Boolean,
+ avatar: Boolean,
+ loading: {
+ type: Boolean,
+ value: true,
+ },
+ animate: {
+ type: Boolean,
+ value: true,
+ },
+ avatarSize: {
+ type: String,
+ value: '32px',
+ },
+ avatarShape: {
+ type: String,
+ value: 'round',
+ },
+ titleWidth: {
+ type: String,
+ value: '40%',
+ },
+ rowWidth: {
+ type: null,
+ value: '100%',
+ observer: function (val) {
+ this.setData({ isArray: val instanceof Array });
+ },
+ },
+ },
+ data: {
+ isArray: false,
+ rowArray: [],
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.json
new file mode 100644
index 0000000..a89ef4d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.json
@@ -0,0 +1,4 @@
+{
+ "component": true,
+ "usingComponents": {}
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxml
new file mode 100644
index 0000000..058e2ef
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxml
@@ -0,0 +1,29 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxss
new file mode 100644
index 0000000..d59a5ed
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/skeleton/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-skeleton{box-sizing:border-box;display:flex;padding:var(--skeleton-padding,0 16px);width:100%}.van-skeleton__avatar{background-color:var(--skeleton-avatar-background-color,#f2f3f5);flex-shrink:0;margin-right:var(--padding-md,16px)}.van-skeleton__avatar--round{border-radius:100%}.van-skeleton__content{flex:1}.van-skeleton__avatar+.van-skeleton__content{padding-top:var(--padding-xs,8px)}.van-skeleton__row,.van-skeleton__title{background-color:var(--skeleton-row-background-color,#f2f3f5);height:var(--skeleton-row-height,16px)}.van-skeleton__title{margin:0}.van-skeleton__row:not(:first-child){margin-top:var(--skeleton-row-margin-top,12px)}.van-skeleton__title+.van-skeleton__row{margin-top:20px}.van-skeleton--animate{animation:van-skeleton-blink 1.2s ease-in-out infinite}@keyframes van-skeleton-blink{50%{opacity:.6}}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.js
new file mode 100644
index 0000000..92adca8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.js
@@ -0,0 +1,206 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var version_1 = require("../common/version");
+var utils_1 = require("../common/utils");
+var DRAG_STATUS = {
+ START: 'start',
+ MOVING: 'moving',
+ END: 'end',
+};
+(0, component_1.VantComponent)({
+ mixins: [touch_1.touch],
+ props: {
+ range: Boolean,
+ disabled: Boolean,
+ useButtonSlot: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ max: {
+ type: Number,
+ value: 100,
+ },
+ min: {
+ type: Number,
+ value: 0,
+ },
+ step: {
+ type: Number,
+ value: 1,
+ },
+ value: {
+ type: null,
+ value: 0,
+ observer: function (val) {
+ if (val !== this.value) {
+ this.updateValue(val);
+ }
+ },
+ },
+ vertical: Boolean,
+ barHeight: null,
+ },
+ created: function () {
+ this.updateValue(this.data.value);
+ },
+ methods: {
+ onTouchStart: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ var index = event.currentTarget.dataset.index;
+ if (typeof index === 'number') {
+ this.buttonIndex = index;
+ }
+ this.touchStart(event);
+ this.startValue = this.format(this.value);
+ this.newValue = this.value;
+ if (this.isRange(this.newValue)) {
+ this.startValue = this.newValue.map(function (val) { return _this.format(val); });
+ }
+ else {
+ this.startValue = this.format(this.newValue);
+ }
+ this.dragStatus = DRAG_STATUS.START;
+ },
+ onTouchMove: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.START) {
+ this.$emit('drag-start');
+ }
+ this.touchMove(event);
+ this.dragStatus = DRAG_STATUS.MOVING;
+ (0, utils_1.getRect)(this, '.van-slider').then(function (rect) {
+ var vertical = _this.data.vertical;
+ var delta = vertical ? _this.deltaY : _this.deltaX;
+ var total = vertical ? rect.height : rect.width;
+ var diff = (delta / total) * _this.getRange();
+ if (_this.isRange(_this.startValue)) {
+ _this.newValue[_this.buttonIndex] =
+ _this.startValue[_this.buttonIndex] + diff;
+ }
+ else {
+ _this.newValue = _this.startValue + diff;
+ }
+ _this.updateValue(_this.newValue, false, true);
+ });
+ },
+ onTouchEnd: function () {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ if (this.dragStatus === DRAG_STATUS.MOVING) {
+ this.dragStatus = DRAG_STATUS.END;
+ (0, utils_1.nextTick)(function () {
+ _this.updateValue(_this.newValue, true);
+ _this.$emit('drag-end');
+ });
+ }
+ },
+ onClick: function (event) {
+ var _this = this;
+ if (this.data.disabled)
+ return;
+ var min = this.data.min;
+ (0, utils_1.getRect)(this, '.van-slider').then(function (rect) {
+ var vertical = _this.data.vertical;
+ var touch = event.touches[0];
+ var delta = vertical
+ ? touch.clientY - rect.top
+ : touch.clientX - rect.left;
+ var total = vertical ? rect.height : rect.width;
+ var value = Number(min) + (delta / total) * _this.getRange();
+ if (_this.isRange(_this.value)) {
+ var _a = _this.value, left = _a[0], right = _a[1];
+ var middle = (left + right) / 2;
+ if (value <= middle) {
+ _this.updateValue([value, right], true);
+ }
+ else {
+ _this.updateValue([left, value], true);
+ }
+ }
+ else {
+ _this.updateValue(value, true);
+ }
+ });
+ },
+ isRange: function (val) {
+ var range = this.data.range;
+ return range && Array.isArray(val);
+ },
+ handleOverlap: function (value) {
+ if (value[0] > value[1]) {
+ return value.slice(0).reverse();
+ }
+ return value;
+ },
+ updateValue: function (value, end, drag) {
+ var _this = this;
+ if (this.isRange(value)) {
+ value = this.handleOverlap(value).map(function (val) { return _this.format(val); });
+ }
+ else {
+ value = this.format(value);
+ }
+ this.value = value;
+ var vertical = this.data.vertical;
+ var mainAxis = vertical ? 'height' : 'width';
+ this.setData({
+ wrapperStyle: "\n background: ".concat(this.data.inactiveColor || '', ";\n ").concat(vertical ? 'width' : 'height', ": ").concat((0, utils_1.addUnit)(this.data.barHeight) || '', ";\n "),
+ barStyle: "\n ".concat(mainAxis, ": ").concat(this.calcMainAxis(), ";\n left: ").concat(vertical ? 0 : this.calcOffset(), ";\n top: ").concat(vertical ? this.calcOffset() : 0, ";\n ").concat(drag ? 'transition: none;' : '', "\n "),
+ });
+ if (drag) {
+ this.$emit('drag', { value: value });
+ }
+ if (end) {
+ this.$emit('change', value);
+ }
+ if ((drag || end) && (0, version_1.canIUseModel)()) {
+ this.setData({ value: value });
+ }
+ },
+ getScope: function () {
+ return Number(this.data.max) - Number(this.data.min);
+ },
+ getRange: function () {
+ var _a = this.data, max = _a.max, min = _a.min;
+ return max - min;
+ },
+ getOffsetWidth: function (current, min) {
+ var scope = this.getScope();
+ // 避免最小值小于最小step时出现负数情况
+ return "".concat(Math.max(((current - min) * 100) / scope, 0), "%");
+ },
+ // 计算选中条的长度百分比
+ calcMainAxis: function () {
+ var value = this.value;
+ var min = this.data.min;
+ if (this.isRange(value)) {
+ return this.getOffsetWidth(value[1], value[0]);
+ }
+ return this.getOffsetWidth(value, Number(min));
+ },
+ // 计算选中条的开始位置的偏移量
+ calcOffset: function () {
+ var value = this.value;
+ var min = this.data.min;
+ var scope = this.getScope();
+ if (this.isRange(value)) {
+ return "".concat(((value[0] - Number(min)) * 100) / scope, "%");
+ }
+ return '0%';
+ },
+ format: function (value) {
+ var min = +this.data.min;
+ var max = +this.data.max;
+ var step = +this.data.step;
+ value = (0, utils_1.clamp)(value, min, max);
+ var diff = Math.round((value - min) / step) * step;
+ return (0, utils_1.addNumber)(min, diff);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxml
new file mode 100644
index 0000000..7c0184f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxml
@@ -0,0 +1,68 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxs
new file mode 100644
index 0000000..7c43e6e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxs
@@ -0,0 +1,14 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function barStyle(barHeight, activeColor) {
+ return style({
+ height: addUnit(barHeight),
+ background: activeColor,
+ });
+}
+
+module.exports = {
+ barStyle: barStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxss
new file mode 100644
index 0000000..d1587de
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/slider/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-slider{background-color:var(--slider-inactive-background-color,#ebedf0);border-radius:999px;height:var(--slider-bar-height,2px);position:relative}.van-slider:before{bottom:calc(var(--padding-xs, 8px)*-1);content:"";left:0;position:absolute;right:0;top:calc(var(--padding-xs, 8px)*-1)}.van-slider__bar{background-color:var(--slider-active-background-color,#1989fa);border-radius:inherit;height:100%;position:relative;transition:all .2s;width:100%}.van-slider__button{background-color:var(--slider-button-background-color,#fff);border-radius:var(--slider-button-border-radius,50%);box-shadow:var(--slider-button-box-shadow,0 1px 2px rgba(0,0,0,.5));height:var(--slider-button-height,24px);width:var(--slider-button-width,24px)}.van-slider__button-wrapper,.van-slider__button-wrapper-right{position:absolute;right:0;top:50%;transform:translate3d(50%,-50%,0)}.van-slider__button-wrapper-left{left:0;position:absolute;top:50%;transform:translate3d(-50%,-50%,0)}.van-slider--disabled{opacity:var(--slider-disabled-opacity,.5)}.van-slider--vertical{display:inline-block;height:100%;width:var(--slider-bar-height,2px)}.van-slider--vertical .van-slider__button-wrapper,.van-slider--vertical .van-slider__button-wrapper-right{bottom:0;right:50%;top:auto;transform:translate3d(50%,50%,0)}.van-slider--vertical .van-slider__button-wrapper-left{left:auto;right:50%;top:0;transform:translate3d(50%,-50%,0)}.van-slider--vertical:before{bottom:0;left:-8px;right:-8px;top:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.js
new file mode 100644
index 0000000..1aa2c27
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.js
@@ -0,0 +1,203 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var LONG_PRESS_START_TIME = 600;
+var LONG_PRESS_INTERVAL = 200;
+// add num and avoid float number
+function add(num1, num2) {
+ var cardinal = Math.pow(10, 10);
+ return Math.round((num1 + num2) * cardinal) / cardinal;
+}
+function equal(value1, value2) {
+ return String(value1) === String(value2);
+}
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['input-class', 'plus-class', 'minus-class'],
+ props: {
+ value: {
+ type: null,
+ },
+ integer: {
+ type: Boolean,
+ observer: 'check',
+ },
+ disabled: Boolean,
+ inputWidth: String,
+ buttonSize: String,
+ asyncChange: Boolean,
+ disableInput: Boolean,
+ decimalLength: {
+ type: Number,
+ value: null,
+ observer: 'check',
+ },
+ min: {
+ type: null,
+ value: 1,
+ observer: 'check',
+ },
+ max: {
+ type: null,
+ value: Number.MAX_SAFE_INTEGER,
+ observer: 'check',
+ },
+ step: {
+ type: null,
+ value: 1,
+ },
+ showPlus: {
+ type: Boolean,
+ value: true,
+ },
+ showMinus: {
+ type: Boolean,
+ value: true,
+ },
+ disablePlus: Boolean,
+ disableMinus: Boolean,
+ longPress: {
+ type: Boolean,
+ value: true,
+ },
+ theme: String,
+ alwaysEmbed: Boolean,
+ },
+ data: {
+ currentValue: '',
+ },
+ watch: {
+ value: function () {
+ this.observeValue();
+ },
+ },
+ created: function () {
+ this.setData({
+ currentValue: this.format(this.data.value),
+ });
+ },
+ methods: {
+ observeValue: function () {
+ var value = this.data.value;
+ this.setData({ currentValue: this.format(value) });
+ },
+ check: function () {
+ var val = this.format(this.data.currentValue);
+ if (!equal(val, this.data.currentValue)) {
+ this.setData({ currentValue: val });
+ }
+ },
+ isDisabled: function (type) {
+ var _a = this.data, disabled = _a.disabled, disablePlus = _a.disablePlus, disableMinus = _a.disableMinus, currentValue = _a.currentValue, max = _a.max, min = _a.min;
+ if (type === 'plus') {
+ return disabled || disablePlus || +currentValue >= +max;
+ }
+ return disabled || disableMinus || +currentValue <= +min;
+ },
+ onFocus: function (event) {
+ this.$emit('focus', event.detail);
+ },
+ onBlur: function (event) {
+ var value = this.format(event.detail.value);
+ this.setData({ currentValue: value });
+ this.emitChange(value);
+ this.$emit('blur', __assign(__assign({}, event.detail), { value: value }));
+ },
+ // filter illegal characters
+ filter: function (value) {
+ value = String(value).replace(/[^0-9.-]/g, '');
+ if (this.data.integer && value.indexOf('.') !== -1) {
+ value = value.split('.')[0];
+ }
+ return value;
+ },
+ // limit value range
+ format: function (value) {
+ value = this.filter(value);
+ // format range
+ value = value === '' ? 0 : +value;
+ value = Math.max(Math.min(this.data.max, value), this.data.min);
+ // format decimal
+ if ((0, validator_1.isDef)(this.data.decimalLength)) {
+ value = value.toFixed(this.data.decimalLength);
+ }
+ return value;
+ },
+ onInput: function (event) {
+ var _a = (event.detail || {}).value, value = _a === void 0 ? '' : _a;
+ // allow input to be empty
+ if (value === '') {
+ return;
+ }
+ var formatted = this.filter(value);
+ // limit max decimal length
+ if ((0, validator_1.isDef)(this.data.decimalLength) && formatted.indexOf('.') !== -1) {
+ var pair = formatted.split('.');
+ formatted = "".concat(pair[0], ".").concat(pair[1].slice(0, this.data.decimalLength));
+ }
+ this.emitChange(formatted);
+ },
+ emitChange: function (value) {
+ if (!this.data.asyncChange) {
+ this.setData({ currentValue: value });
+ }
+ this.$emit('change', value);
+ },
+ onChange: function () {
+ var type = this.type;
+ if (this.isDisabled(type)) {
+ this.$emit('overlimit', type);
+ return;
+ }
+ var diff = type === 'minus' ? -this.data.step : +this.data.step;
+ var value = this.format(add(+this.data.currentValue, diff));
+ this.emitChange(value);
+ this.$emit(type);
+ },
+ longPressStep: function () {
+ var _this = this;
+ this.longPressTimer = setTimeout(function () {
+ _this.onChange();
+ _this.longPressStep();
+ }, LONG_PRESS_INTERVAL);
+ },
+ onTap: function (event) {
+ var type = event.currentTarget.dataset.type;
+ this.type = type;
+ this.onChange();
+ },
+ onTouchStart: function (event) {
+ var _this = this;
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ var type = event.currentTarget.dataset.type;
+ this.type = type;
+ this.isLongPress = false;
+ this.longPressTimer = setTimeout(function () {
+ _this.isLongPress = true;
+ _this.onChange();
+ _this.longPressStep();
+ }, LONG_PRESS_START_TIME);
+ },
+ onTouchEnd: function () {
+ if (!this.data.longPress) {
+ return;
+ }
+ clearTimeout(this.longPressTimer);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxml
new file mode 100644
index 0000000..6dd44bc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxml
@@ -0,0 +1,43 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxs
new file mode 100644
index 0000000..a13e818
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxs
@@ -0,0 +1,22 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function buttonStyle(data) {
+ return style({
+ width: addUnit(data.buttonSize),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+function inputStyle(data) {
+ return style({
+ width: addUnit(data.inputWidth),
+ height: addUnit(data.buttonSize),
+ });
+}
+
+module.exports = {
+ buttonStyle: buttonStyle,
+ inputStyle: inputStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxss
new file mode 100644
index 0000000..2561a7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/stepper/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-stepper{font-size:0}.van-stepper__minus,.van-stepper__plus{background-color:var(--stepper-background-color,#f2f3f5);border:0;box-sizing:border-box;color:var(--stepper-button-icon-color,#323233);display:inline-block;height:var(--stepper-input-height,28px);margin:1px;padding:var(--padding-base,4px);position:relative;vertical-align:middle;width:var(--stepper-input-height,28px)}.van-stepper__minus:before,.van-stepper__plus:before{height:1px;width:9px}.van-stepper__minus:after,.van-stepper__plus:after{height:9px;width:1px}.van-stepper__minus:empty.van-stepper__minus:after,.van-stepper__minus:empty.van-stepper__minus:before,.van-stepper__minus:empty.van-stepper__plus:after,.van-stepper__minus:empty.van-stepper__plus:before,.van-stepper__plus:empty.van-stepper__minus:after,.van-stepper__plus:empty.van-stepper__minus:before,.van-stepper__plus:empty.van-stepper__plus:after,.van-stepper__plus:empty.van-stepper__plus:before{background-color:currentColor;bottom:0;content:"";left:0;margin:auto;position:absolute;right:0;top:0}.van-stepper__minus--hover,.van-stepper__plus--hover{background-color:var(--stepper-active-color,#e8e8e8)}.van-stepper__minus--disabled,.van-stepper__plus--disabled{color:var(--stepper-button-disabled-icon-color,#c8c9cc)}.van-stepper__minus--disabled,.van-stepper__minus--disabled.van-stepper__minus--hover,.van-stepper__minus--disabled.van-stepper__plus--hover,.van-stepper__plus--disabled,.van-stepper__plus--disabled.van-stepper__minus--hover,.van-stepper__plus--disabled.van-stepper__plus--hover{background-color:var(--stepper-button-disabled-color,#f7f8fa)}.van-stepper__minus{border-radius:var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0 0 var(--stepper-border-radius,var(--stepper-border-radius,4px))}.van-stepper__minus:after{display:none}.van-stepper__plus{border-radius:0 var(--stepper-border-radius,var(--stepper-border-radius,4px)) var(--stepper-border-radius,var(--stepper-border-radius,4px)) 0}.van-stepper--round .van-stepper__input{background-color:initial!important}.van-stepper--round .van-stepper__minus,.van-stepper--round .van-stepper__plus{border-radius:100%}.van-stepper--round .van-stepper__minus:active,.van-stepper--round .van-stepper__plus:active{opacity:.7}.van-stepper--round .van-stepper__minus--disabled,.van-stepper--round .van-stepper__minus--disabled:active,.van-stepper--round .van-stepper__plus--disabled,.van-stepper--round .van-stepper__plus--disabled:active{opacity:.3}.van-stepper--round .van-stepper__plus{background-color:#ee0a24;color:#fff}.van-stepper--round .van-stepper__minus{background-color:#fff;border:1px solid #ee0a24;color:#ee0a24}.van-stepper__input{-webkit-appearance:none;background-color:var(--stepper-background-color,#f2f3f5);border:0;border-radius:0;border-width:1px 0;box-sizing:border-box;color:var(--stepper-input-text-color,#323233);display:inline-block;font-size:var(--stepper-input-font-size,14px);height:var(--stepper-input-height,28px);margin:1px;min-height:0;padding:1px;text-align:center;vertical-align:middle;width:var(--stepper-input-width,32px)}.van-stepper__input--disabled{background-color:var(--stepper-input-disabled-background-color,#f2f3f5);color:var(--stepper-input-disabled-text-color,#c8c9cc)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.js
new file mode 100644
index 0000000..1a9986a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.js
@@ -0,0 +1,35 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var color_1 = require("../common/color");
+(0, component_1.VantComponent)({
+ classes: ['desc-class'],
+ props: {
+ icon: String,
+ steps: Array,
+ active: Number,
+ direction: {
+ type: String,
+ value: 'horizontal',
+ },
+ activeColor: {
+ type: String,
+ value: color_1.GREEN,
+ },
+ inactiveColor: {
+ type: String,
+ value: color_1.GRAY_DARK,
+ },
+ activeIcon: {
+ type: String,
+ value: 'checked',
+ },
+ inactiveIcon: String,
+ },
+ methods: {
+ onClick: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.$emit('click-step', index);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxml
new file mode 100644
index 0000000..00c8e10
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxml
@@ -0,0 +1,54 @@
+
+
+
+
+
+
+ {{ item.text }}
+ {{ item.desc }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+function get(index, active) {
+ if (index < active) {
+ return 'finish';
+ } else if (index === active) {
+ return 'process';
+ }
+
+ return 'inactive';
+}
+
+module.exports = get;
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxss
new file mode 100644
index 0000000..c653884
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/steps/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-steps{background-color:var(--steps-background-color,#fff);overflow:hidden}.van-steps--horizontal{padding:10px}.van-steps--horizontal .van-step__wrapper{display:flex;overflow:hidden;position:relative}.van-steps--vertical{padding-left:10px}.van-steps--vertical .van-step__wrapper{padding:0 0 0 20px}.van-step{color:var(--step-text-color,#969799);flex:1;font-size:var(--step-font-size,14px);position:relative}.van-step--finish{color:var(--step-finish-text-color,#323233)}.van-step__circle{background-color:var(--step-circle-color,#969799);border-radius:50%;height:var(--step-circle-size,5px);width:var(--step-circle-size,5px)}.van-step--horizontal{padding-bottom:14px}.van-step--horizontal:first-child .van-step__title{transform:none}.van-step--horizontal:first-child .van-step__circle-container{padding:0 8px 0 0;transform:translate3d(0,50%,0)}.van-step--horizontal:last-child{position:absolute;right:0;width:auto}.van-step--horizontal:last-child .van-step__title{text-align:right;transform:none}.van-step--horizontal:last-child .van-step__circle-container{padding:0 0 0 8px;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal .van-step__circle-container{background-color:#fff;bottom:6px;padding:0 var(--padding-xs,8px);position:absolute;transform:translate3d(-50%,50%,0);z-index:1}.van-step--horizontal .van-step__title{display:inline-block;font-size:var(--step-horizontal-title-font-size,12px);transform:translate3d(-50%,0,0)}.van-step--horizontal .van-step__line{background-color:var(--step-line-color,#ebedf0);bottom:6px;height:1px;left:0;position:absolute;right:0;transform:translate3d(0,50%,0)}.van-step--horizontal.van-step--process{color:var(--step-process-text-color,#323233)}.van-step--horizontal.van-step--process .van-step__icon{display:block;font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical{line-height:18px;padding:10px 10px 10px 0}.van-step--vertical:after{border-bottom-width:1px}.van-step--vertical:last-child:after{border-bottom-width:none}.van-step--vertical:first-child:before{background-color:#fff;content:"";height:20px;left:-15px;position:absolute;top:0;width:1px;z-index:1}.van-step--vertical .van-step__circle,.van-step--vertical .van-step__icon,.van-step--vertical .van-step__line{left:-14px;position:absolute;top:19px;transform:translate3d(-50%,-50%,0);z-index:2}.van-step--vertical .van-step__icon{background-color:var(--steps-background-color,#fff);font-size:var(--step-icon-size,12px);line-height:1}.van-step--vertical .van-step__line{background-color:var(--step-line-color,#ebedf0);height:100%;transform:translate3d(-50%,0,0);width:1px;z-index:1}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.js
new file mode 100644
index 0000000..ffeb125
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.js
@@ -0,0 +1,126 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var utils_1 = require("../common/utils");
+var component_1 = require("../common/component");
+var validator_1 = require("../common/validator");
+var page_scroll_1 = require("../mixins/page-scroll");
+var ROOT_ELEMENT = '.van-sticky';
+(0, component_1.VantComponent)({
+ props: {
+ zIndex: {
+ type: Number,
+ value: 99,
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ observer: 'onScroll',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'onScroll',
+ },
+ container: {
+ type: null,
+ observer: 'onScroll',
+ },
+ scrollTop: {
+ type: null,
+ observer: function (val) {
+ this.onScroll({ scrollTop: val });
+ },
+ },
+ },
+ mixins: [
+ (0, page_scroll_1.pageScrollMixin)(function (event) {
+ if (this.data.scrollTop != null) {
+ return;
+ }
+ this.onScroll(event);
+ }),
+ ],
+ data: {
+ height: 0,
+ fixed: false,
+ transform: 0,
+ },
+ mounted: function () {
+ this.onScroll();
+ },
+ methods: {
+ onScroll: function (_a) {
+ var _this = this;
+ var _b = _a === void 0 ? {} : _a, scrollTop = _b.scrollTop;
+ var _c = this.data, container = _c.container, offsetTop = _c.offsetTop, disabled = _c.disabled;
+ if (disabled) {
+ this.setDataAfterDiff({
+ fixed: false,
+ transform: 0,
+ });
+ return;
+ }
+ this.scrollTop = scrollTop || this.scrollTop;
+ if (typeof container === 'function') {
+ Promise.all([(0, utils_1.getRect)(this, ROOT_ELEMENT), this.getContainerRect()])
+ .then(function (_a) {
+ var root = _a[0], container = _a[1];
+ if (offsetTop + root.height > container.height + container.top) {
+ _this.setDataAfterDiff({
+ fixed: false,
+ transform: container.height - root.height,
+ });
+ }
+ else if (offsetTop >= root.top) {
+ _this.setDataAfterDiff({
+ fixed: true,
+ height: root.height,
+ transform: 0,
+ });
+ }
+ else {
+ _this.setDataAfterDiff({ fixed: false, transform: 0 });
+ }
+ })
+ .catch(function () { });
+ return;
+ }
+ (0, utils_1.getRect)(this, ROOT_ELEMENT).then(function (root) {
+ if (!(0, validator_1.isDef)(root) || (!root.width && !root.height)) {
+ return;
+ }
+ if (offsetTop >= root.top) {
+ _this.setDataAfterDiff({ fixed: true, height: root.height });
+ _this.transform = 0;
+ }
+ else {
+ _this.setDataAfterDiff({ fixed: false });
+ }
+ });
+ },
+ setDataAfterDiff: function (data) {
+ var _this = this;
+ wx.nextTick(function () {
+ var diff = Object.keys(data).reduce(function (prev, key) {
+ if (data[key] !== _this.data[key]) {
+ prev[key] = data[key];
+ }
+ return prev;
+ }, {});
+ if (Object.keys(diff).length > 0) {
+ _this.setData(diff);
+ }
+ _this.$emit('scroll', {
+ scrollTop: _this.scrollTop,
+ isFixed: data.fixed || _this.data.fixed,
+ });
+ });
+ },
+ getContainerRect: function () {
+ var nodesRef = this.data.container();
+ if (!nodesRef) {
+ return Promise.reject(new Error('not found container'));
+ }
+ return new Promise(function (resolve) { return nodesRef.boundingClientRect(resolve).exec(); });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxml
new file mode 100644
index 0000000..15e9f4a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxs
new file mode 100644
index 0000000..be99d89
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxs
@@ -0,0 +1,25 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function wrapStyle(data) {
+ return style({
+ transform: data.transform
+ ? 'translate3d(0, ' + data.transform + 'px, 0)'
+ : '',
+ top: data.fixed ? addUnit(data.offsetTop) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+function containerStyle(data) {
+ return style({
+ height: data.fixed ? addUnit(data.height) : '',
+ 'z-index': data.zIndex,
+ });
+}
+
+module.exports = {
+ wrapStyle: wrapStyle,
+ containerStyle: containerStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxss
new file mode 100644
index 0000000..34d76aa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/sticky/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-sticky{position:relative}.van-sticky-wrap--fixed{left:0;position:fixed;right:0}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.js
new file mode 100644
index 0000000..d3bfc25
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.js
@@ -0,0 +1,58 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: ['bar-class', 'price-class', 'button-class'],
+ props: {
+ tip: {
+ type: null,
+ observer: 'updateTip',
+ },
+ tipIcon: String,
+ type: Number,
+ price: {
+ type: null,
+ observer: 'updatePrice',
+ },
+ label: String,
+ loading: Boolean,
+ disabled: Boolean,
+ buttonText: String,
+ currency: {
+ type: String,
+ value: '¥',
+ },
+ buttonType: {
+ type: String,
+ value: 'danger',
+ },
+ decimalLength: {
+ type: Number,
+ value: 2,
+ observer: 'updatePrice',
+ },
+ suffixLabel: String,
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ methods: {
+ updatePrice: function () {
+ var _a = this.data, price = _a.price, decimalLength = _a.decimalLength;
+ var priceStrArr = typeof price === 'number' &&
+ (price / 100).toFixed(decimalLength).split('.');
+ this.setData({
+ hasPrice: typeof price === 'number',
+ integerStr: priceStrArr && priceStrArr[0],
+ decimalStr: decimalLength && priceStrArr ? ".".concat(priceStrArr[1]) : '',
+ });
+ },
+ updateTip: function () {
+ this.setData({ hasTip: typeof this.data.tip === 'string' });
+ },
+ onSubmit: function (event) {
+ this.$emit('submit', event.detail);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.json
new file mode 100644
index 0000000..bda9b8d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-button": "../button/index",
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxml
new file mode 100644
index 0000000..a56dd46
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxml
@@ -0,0 +1,44 @@
+
+
+
+
+
+
+
+
+ {{ tip }}
+
+
+
+
+
+
+
+ {{ label || '合计:' }}
+
+ {{ currency }}
+ {{ integerStr }}{{decimalStr}}
+
+ {{ suffixLabel }}
+
+
+ {{ loading ? '' : buttonText }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxss
new file mode 100644
index 0000000..8379a30
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/submit-bar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-submit-bar{background-color:var(--submit-bar-background-color,#fff);bottom:0;left:0;position:fixed;-webkit-user-select:none;user-select:none;width:100%;z-index:var(--submit-bar-z-index,100)}.van-submit-bar__tip{background-color:var(--submit-bar-tip-background-color,#fff7cc);color:var(--submit-bar-tip-color,#f56723);font-size:var(--submit-bar-tip-font-size,12px);line-height:var(--submit-bar-tip-line-height,1.5);padding:var(--submit-bar-tip-padding,10px)}.van-submit-bar__tip:empty{display:none}.van-submit-bar__tip-icon{margin-right:4px;vertical-align:middle}.van-submit-bar__tip-text{display:inline;vertical-align:middle}.van-submit-bar__bar{align-items:center;background-color:var(--submit-bar-background-color,#fff);display:flex;font-size:var(--submit-bar-text-font-size,14px);height:var(--submit-bar-height,50px);justify-content:flex-end;padding:var(--submit-bar-padding,0 16px)}.van-submit-bar__safe{height:constant(safe-area-inset-bottom);height:env(safe-area-inset-bottom)}.van-submit-bar__text{color:var(--submit-bar-text-color,#323233);flex:1;font-weight:var(--font-weight-bold,500);padding-right:var(--padding-sm,12px);text-align:right}.van-submit-bar__price{color:var(--submit-bar-price-color,#ee0a24);font-size:var(--submit-bar-price-font-size,12px);font-weight:var(--font-weight-bold,500)}.van-submit-bar__price-integer{font-family:Avenir-Heavy,PingFang SC,Helvetica Neue,Arial,sans-serif;font-size:20px}.van-submit-bar__currency{font-size:var(--submit-bar-currency-font-size,12px)}.van-submit-bar__suffix-label{margin-left:5px}.van-submit-bar__button{--button-default-height:var(--submit-bar-button-height,40px)!important;--button-line-height:var(--submit-bar-button-height,40px)!important;font-weight:var(--font-weight-bold,500);width:var(--submit-bar-button-width,110px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.js
new file mode 100644
index 0000000..1582b6c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.js
@@ -0,0 +1,135 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var utils_1 = require("../common/utils");
+var THRESHOLD = 0.3;
+var ARRAY = [];
+(0, component_1.VantComponent)({
+ props: {
+ disabled: Boolean,
+ leftWidth: {
+ type: Number,
+ value: 0,
+ observer: function (leftWidth) {
+ if (leftWidth === void 0) { leftWidth = 0; }
+ if (this.offset > 0) {
+ this.swipeMove(leftWidth);
+ }
+ },
+ },
+ rightWidth: {
+ type: Number,
+ value: 0,
+ observer: function (rightWidth) {
+ if (rightWidth === void 0) { rightWidth = 0; }
+ if (this.offset < 0) {
+ this.swipeMove(-rightWidth);
+ }
+ },
+ },
+ asyncClose: Boolean,
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ mixins: [touch_1.touch],
+ data: {
+ catchMove: false,
+ wrapperStyle: '',
+ },
+ created: function () {
+ this.offset = 0;
+ ARRAY.push(this);
+ },
+ destroyed: function () {
+ var _this = this;
+ ARRAY = ARRAY.filter(function (item) { return item !== _this; });
+ },
+ methods: {
+ open: function (position) {
+ var _a = this.data, leftWidth = _a.leftWidth, rightWidth = _a.rightWidth;
+ var offset = position === 'left' ? leftWidth : -rightWidth;
+ this.swipeMove(offset);
+ this.$emit('open', {
+ position: position,
+ name: this.data.name,
+ });
+ },
+ close: function () {
+ this.swipeMove(0);
+ },
+ swipeMove: function (offset) {
+ if (offset === void 0) { offset = 0; }
+ this.offset = (0, utils_1.range)(offset, -this.data.rightWidth, this.data.leftWidth);
+ var transform = "translate3d(".concat(this.offset, "px, 0, 0)");
+ var transition = this.dragging
+ ? 'none'
+ : 'transform .6s cubic-bezier(0.18, 0.89, 0.32, 1)';
+ this.setData({
+ wrapperStyle: "\n -webkit-transform: ".concat(transform, ";\n -webkit-transition: ").concat(transition, ";\n transform: ").concat(transform, ";\n transition: ").concat(transition, ";\n "),
+ });
+ },
+ swipeLeaveTransition: function () {
+ var _a = this.data, leftWidth = _a.leftWidth, rightWidth = _a.rightWidth;
+ var offset = this.offset;
+ if (rightWidth > 0 && -offset > rightWidth * THRESHOLD) {
+ this.open('right');
+ }
+ else if (leftWidth > 0 && offset > leftWidth * THRESHOLD) {
+ this.open('left');
+ }
+ else {
+ this.swipeMove(0);
+ }
+ this.setData({ catchMove: false });
+ },
+ startDrag: function (event) {
+ if (this.data.disabled) {
+ return;
+ }
+ this.startOffset = this.offset;
+ this.touchStart(event);
+ },
+ noop: function () { },
+ onDrag: function (event) {
+ var _this = this;
+ if (this.data.disabled) {
+ return;
+ }
+ this.touchMove(event);
+ if (this.direction !== 'horizontal') {
+ return;
+ }
+ this.dragging = true;
+ ARRAY.filter(function (item) { return item !== _this && item.offset !== 0; }).forEach(function (item) { return item.close(); });
+ this.setData({ catchMove: true });
+ this.swipeMove(this.startOffset + this.deltaX);
+ },
+ endDrag: function () {
+ if (this.data.disabled) {
+ return;
+ }
+ this.dragging = false;
+ this.swipeLeaveTransition();
+ },
+ onClick: function (event) {
+ var _a = event.currentTarget.dataset.key, position = _a === void 0 ? 'outside' : _a;
+ this.$emit('click', position);
+ if (!this.offset) {
+ return;
+ }
+ if (this.data.asyncClose) {
+ this.$emit('close', {
+ position: position,
+ instance: this,
+ name: this.data.name,
+ });
+ }
+ else {
+ this.swipeMove(0);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxml
new file mode 100644
index 0000000..3f7f726
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxml
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxss
new file mode 100644
index 0000000..3a265bf
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/swipe-cell/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-swipe-cell{overflow:hidden;position:relative}.van-swipe-cell__left,.van-swipe-cell__right{height:100%;position:absolute;top:0}.van-swipe-cell__left{left:0;transform:translate3d(-100%,0,0)}.van-swipe-cell__right{right:0;transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.js
new file mode 100644
index 0000000..1d2317f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.js
@@ -0,0 +1,38 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ field: true,
+ classes: ['node-class'],
+ props: {
+ checked: null,
+ loading: Boolean,
+ disabled: Boolean,
+ activeColor: String,
+ inactiveColor: String,
+ size: {
+ type: String,
+ value: '30',
+ },
+ activeValue: {
+ type: null,
+ value: true,
+ },
+ inactiveValue: {
+ type: null,
+ value: false,
+ },
+ },
+ methods: {
+ onClick: function () {
+ var _a = this.data, activeValue = _a.activeValue, inactiveValue = _a.inactiveValue, disabled = _a.disabled, loading = _a.loading;
+ if (disabled || loading) {
+ return;
+ }
+ var checked = this.data.checked === activeValue;
+ var value = checked ? inactiveValue : activeValue;
+ this.$emit('input', value);
+ this.$emit('change', value);
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.json
new file mode 100644
index 0000000..01077f5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxml
new file mode 100644
index 0000000..4e9789b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxml
@@ -0,0 +1,16 @@
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxs
new file mode 100644
index 0000000..3ae387a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxs
@@ -0,0 +1,26 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function rootStyle(data) {
+ var currentColor = data.checked === data.activeValue ? data.activeColor : data.inactiveColor;
+
+ return style({
+ 'font-size': addUnit(data.size),
+ 'background-color': currentColor,
+ });
+}
+
+var BLUE = '#1989fa';
+var GRAY_DARK = '#969799';
+
+function loadingColor(data) {
+ return data.checked === data.activeValue
+ ? data.activeColor || BLUE
+ : data.inactiveColor || GRAY_DARK;
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+ loadingColor: loadingColor,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxss
new file mode 100644
index 0000000..35929de
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/switch/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-switch{background-color:var(--switch-background-color,#fff);border:var(--switch-border,1px solid rgba(0,0,0,.1));border-radius:var(--switch-node-size,1em);box-sizing:initial;display:inline-block;height:var(--switch-height,1em);position:relative;transition:background-color var(--switch-transition-duration,.3s);width:var(--switch-width,2em)}.van-switch__node{background-color:var(--switch-node-background-color,#fff);border-radius:100%;box-shadow:var(--switch-node-box-shadow,0 3px 1px 0 rgba(0,0,0,.05),0 2px 2px 0 rgba(0,0,0,.1),0 3px 3px 0 rgba(0,0,0,.05));height:var(--switch-node-size,1em);left:0;position:absolute;top:0;transition:var(--switch-transition-duration,.3s) cubic-bezier(.3,1.05,.4,1.05);width:var(--switch-node-size,1em);z-index:var(--switch-node-z-index,1)}.van-switch__loading{height:50%;left:25%;position:absolute!important;top:25%;width:50%}.van-switch--on{background-color:var(--switch-on-background-color,#1989fa)}.van-switch--on .van-switch__node{transform:translateX(calc(var(--switch-width, 2em) - var(--switch-node-size, 1em)))}.van-switch--disabled{opacity:var(--switch-disabled-opacity,.4)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.js
new file mode 100644
index 0000000..ae4d06b
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.js
@@ -0,0 +1,58 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var relation_1 = require("../common/relation");
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useParent)('tabs'),
+ props: {
+ dot: {
+ type: Boolean,
+ observer: 'update',
+ },
+ info: {
+ type: null,
+ observer: 'update',
+ },
+ title: {
+ type: String,
+ observer: 'update',
+ },
+ disabled: {
+ type: Boolean,
+ observer: 'update',
+ },
+ titleStyle: {
+ type: String,
+ observer: 'update',
+ },
+ name: {
+ type: null,
+ value: '',
+ },
+ },
+ data: {
+ active: false,
+ },
+ methods: {
+ getComputedName: function () {
+ if (this.data.name !== '') {
+ return this.data.name;
+ }
+ return this.index;
+ },
+ updateRender: function (active, parent) {
+ var parentData = parent.data;
+ this.inited = this.inited || active;
+ this.setData({
+ active: active,
+ shouldRender: this.inited || !parentData.lazyRender,
+ shouldShow: active || parentData.animated,
+ });
+ },
+ update: function () {
+ if (this.parent) {
+ this.parent.updateTabs();
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxml
new file mode 100644
index 0000000..f5e99f2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxml
@@ -0,0 +1,8 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxss
new file mode 100644
index 0000000..1c90c88
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tab/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{box-sizing:border-box;flex-shrink:0;width:100%}.van-tab__pane{-webkit-overflow-scrolling:touch;box-sizing:border-box;overflow-y:auto}.van-tab__pane--active{height:auto}.van-tab__pane--inactive{height:0;overflow:visible}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.js
new file mode 100644
index 0000000..58a5065
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ props: {
+ info: null,
+ name: null,
+ icon: String,
+ dot: Boolean,
+ url: {
+ type: String,
+ value: '',
+ },
+ linkType: {
+ type: String,
+ value: 'redirectTo',
+ },
+ iconPrefix: {
+ type: String,
+ value: 'van-icon',
+ },
+ },
+ relation: (0, relation_1.useParent)('tabbar'),
+ data: {
+ active: false,
+ activeColor: '',
+ inactiveColor: '',
+ },
+ methods: {
+ onClick: function () {
+ var parent = this.parent;
+ if (parent) {
+ var index = parent.children.indexOf(this);
+ var active = this.data.name || index;
+ if (active !== this.data.active) {
+ parent.$emit('change', active);
+ }
+ }
+ var _a = this.data, url = _a.url, linkType = _a.linkType;
+ if (url && wx[linkType]) {
+ return wx[linkType]({ url: url });
+ }
+ this.$emit('click');
+ },
+ updateFromParent: function () {
+ var parent = this.parent;
+ if (!parent) {
+ return;
+ }
+ var index = parent.children.indexOf(this);
+ var parentData = parent.data;
+ var data = this.data;
+ var active = (data.name || index) === parentData.active;
+ var patch = {};
+ if (active !== data.active) {
+ patch.active = active;
+ }
+ if (parentData.activeColor !== data.activeColor) {
+ patch.activeColor = parentData.activeColor;
+ }
+ if (parentData.inactiveColor !== data.inactiveColor) {
+ patch.inactiveColor = parentData.inactiveColor;
+ }
+ if (Object.keys(patch).length > 0) {
+ this.setData(patch);
+ }
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.json
new file mode 100644
index 0000000..16f174c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-info": "../info/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxml
new file mode 100644
index 0000000..524728f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxml
@@ -0,0 +1,28 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxss
new file mode 100644
index 0000000..21ee224
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar-item/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';:host{flex:1}.van-tabbar-item{align-items:center;color:var(--tabbar-item-text-color,#646566);display:flex;flex-direction:column;font-size:var(--tabbar-item-font-size,12px);height:100%;justify-content:center;line-height:var(--tabbar-item-line-height,1)}.van-tabbar-item__icon{font-size:var(--tabbar-item-icon-size,22px);margin-bottom:var(--tabbar-item-margin-bottom,4px);position:relative}.van-tabbar-item__icon__inner{display:block;min-width:1em}.van-tabbar-item--active{color:var(--tabbar-item-active-color,#1989fa)}.van-tabbar-item__info{margin-top:2px}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.js
new file mode 100644
index 0000000..3db793d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.js
@@ -0,0 +1,68 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var relation_1 = require("../common/relation");
+var utils_1 = require("../common/utils");
+(0, component_1.VantComponent)({
+ relation: (0, relation_1.useChildren)('tabbar-item', function () {
+ this.updateChildren();
+ }),
+ props: {
+ active: {
+ type: null,
+ observer: 'updateChildren',
+ },
+ activeColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ inactiveColor: {
+ type: String,
+ observer: 'updateChildren',
+ },
+ fixed: {
+ type: Boolean,
+ value: true,
+ observer: 'setHeight',
+ },
+ placeholder: {
+ type: Boolean,
+ observer: 'setHeight',
+ },
+ border: {
+ type: Boolean,
+ value: true,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ safeAreaInsetBottom: {
+ type: Boolean,
+ value: true,
+ },
+ },
+ data: {
+ height: 50,
+ },
+ methods: {
+ updateChildren: function () {
+ var children = this.children;
+ if (!Array.isArray(children) || !children.length) {
+ return;
+ }
+ children.forEach(function (child) { return child.updateFromParent(); });
+ },
+ setHeight: function () {
+ var _this = this;
+ if (!this.data.fixed || !this.data.placeholder) {
+ return;
+ }
+ wx.nextTick(function () {
+ (0, utils_1.getRect)(_this, '.van-tabbar').then(function (res) {
+ _this.setData({ height: res.height });
+ });
+ });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxml
new file mode 100644
index 0000000..43bb111
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxss
new file mode 100644
index 0000000..42b6c1e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabbar/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabbar{background-color:var(--tabbar-background-color,#fff);box-sizing:initial;display:flex;height:var(--tabbar-height,50px);width:100%}.van-tabbar--fixed{bottom:0;left:0;position:fixed}.van-tabbar--safe{padding-bottom:env(safe-area-inset-bottom)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.js
new file mode 100644
index 0000000..3121957
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.js
@@ -0,0 +1,327 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var touch_1 = require("../mixins/touch");
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+var relation_1 = require("../common/relation");
+(0, component_1.VantComponent)({
+ mixins: [touch_1.touch],
+ classes: [
+ 'nav-class',
+ 'tab-class',
+ 'tab-active-class',
+ 'line-class',
+ 'wrap-class',
+ ],
+ relation: (0, relation_1.useChildren)('tab', function () {
+ this.updateTabs();
+ }),
+ props: {
+ sticky: Boolean,
+ border: Boolean,
+ swipeable: Boolean,
+ titleActiveColor: String,
+ titleInactiveColor: String,
+ color: String,
+ animated: {
+ type: Boolean,
+ observer: function () {
+ var _this = this;
+ this.children.forEach(function (child, index) {
+ return child.updateRender(index === _this.data.currentIndex, _this);
+ });
+ },
+ },
+ lineWidth: {
+ type: null,
+ value: 40,
+ observer: 'resize',
+ },
+ lineHeight: {
+ type: null,
+ value: -1,
+ },
+ active: {
+ type: null,
+ value: 0,
+ observer: function (name) {
+ if (name !== this.getCurrentName()) {
+ this.setCurrentIndexByName(name);
+ }
+ },
+ },
+ type: {
+ type: String,
+ value: 'line',
+ },
+ ellipsis: {
+ type: Boolean,
+ value: true,
+ },
+ duration: {
+ type: Number,
+ value: 0.3,
+ },
+ zIndex: {
+ type: Number,
+ value: 1,
+ },
+ swipeThreshold: {
+ type: Number,
+ value: 5,
+ observer: function (value) {
+ this.setData({
+ scrollable: this.children.length > value || !this.data.ellipsis,
+ });
+ },
+ },
+ offsetTop: {
+ type: Number,
+ value: 0,
+ },
+ lazyRender: {
+ type: Boolean,
+ value: true,
+ },
+ useBeforeChange: {
+ type: Boolean,
+ value: false,
+ },
+ },
+ data: {
+ tabs: [],
+ scrollLeft: 0,
+ scrollable: false,
+ currentIndex: 0,
+ container: null,
+ skipTransition: true,
+ scrollWithAnimation: false,
+ lineOffsetLeft: 0,
+ inited: false,
+ },
+ mounted: function () {
+ var _this = this;
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.swiping = true;
+ _this.setData({
+ container: function () { return _this.createSelectorQuery().select('.van-tabs'); },
+ });
+ _this.resize();
+ _this.scrollIntoView();
+ });
+ },
+ methods: {
+ updateTabs: function () {
+ var _a = this, _b = _a.children, children = _b === void 0 ? [] : _b, data = _a.data;
+ this.setData({
+ tabs: children.map(function (child) { return child.data; }),
+ scrollable: this.children.length > data.swipeThreshold || !data.ellipsis,
+ });
+ this.setCurrentIndexByName(data.active || this.getCurrentName());
+ },
+ trigger: function (eventName, child) {
+ var currentIndex = this.data.currentIndex;
+ var data = this.getChildData(currentIndex, child);
+ if (!(0, validator_1.isDef)(data)) {
+ return;
+ }
+ this.$emit(eventName, data);
+ },
+ onTap: function (event) {
+ var _this = this;
+ var index = event.currentTarget.dataset.index;
+ var child = this.children[index];
+ if (child.data.disabled) {
+ this.trigger('disabled', child);
+ return;
+ }
+ this.onBeforeChange(index).then(function () {
+ _this.setCurrentIndex(index);
+ (0, utils_1.nextTick)(function () {
+ _this.trigger('click');
+ });
+ });
+ },
+ // correct the index of active tab
+ setCurrentIndexByName: function (name) {
+ var _a = this.children, children = _a === void 0 ? [] : _a;
+ var matched = children.filter(function (child) { return child.getComputedName() === name; });
+ if (matched.length) {
+ this.setCurrentIndex(matched[0].index);
+ }
+ },
+ setCurrentIndex: function (currentIndex) {
+ var _this = this;
+ var _a = this, data = _a.data, _b = _a.children, children = _b === void 0 ? [] : _b;
+ if (!(0, validator_1.isDef)(currentIndex) ||
+ currentIndex >= children.length ||
+ currentIndex < 0) {
+ return;
+ }
+ (0, utils_1.groupSetData)(this, function () {
+ children.forEach(function (item, index) {
+ var active = index === currentIndex;
+ if (active !== item.data.active || !item.inited) {
+ item.updateRender(active, _this);
+ }
+ });
+ });
+ if (currentIndex === data.currentIndex) {
+ if (!data.inited) {
+ this.resize();
+ }
+ return;
+ }
+ var shouldEmitChange = data.currentIndex !== null;
+ this.setData({ currentIndex: currentIndex });
+ (0, utils_1.requestAnimationFrame)(function () {
+ _this.resize();
+ _this.scrollIntoView();
+ });
+ (0, utils_1.nextTick)(function () {
+ _this.trigger('input');
+ if (shouldEmitChange) {
+ _this.trigger('change');
+ }
+ });
+ },
+ getCurrentName: function () {
+ var activeTab = this.children[this.data.currentIndex];
+ if (activeTab) {
+ return activeTab.getComputedName();
+ }
+ },
+ resize: function () {
+ var _this = this;
+ if (this.data.type !== 'line') {
+ return;
+ }
+ var _a = this.data, currentIndex = _a.currentIndex, ellipsis = _a.ellipsis, skipTransition = _a.skipTransition;
+ Promise.all([
+ (0, utils_1.getAllRect)(this, '.van-tab'),
+ (0, utils_1.getRect)(this, '.van-tabs__line'),
+ ]).then(function (_a) {
+ var _b = _a[0], rects = _b === void 0 ? [] : _b, lineRect = _a[1];
+ var rect = rects[currentIndex];
+ if (rect == null) {
+ return;
+ }
+ var lineOffsetLeft = rects
+ .slice(0, currentIndex)
+ .reduce(function (prev, curr) { return prev + curr.width; }, 0);
+ lineOffsetLeft +=
+ (rect.width - lineRect.width) / 2 + (ellipsis ? 0 : 8);
+ _this.setData({ lineOffsetLeft: lineOffsetLeft, inited: true });
+ _this.swiping = true;
+ if (skipTransition) {
+ // waiting transition end
+ setTimeout(function () {
+ _this.setData({ skipTransition: false });
+ }, _this.data.duration);
+ }
+ });
+ },
+ // scroll active tab into view
+ scrollIntoView: function () {
+ var _this = this;
+ var _a = this.data, currentIndex = _a.currentIndex, scrollable = _a.scrollable, scrollWithAnimation = _a.scrollWithAnimation;
+ if (!scrollable) {
+ return;
+ }
+ Promise.all([
+ (0, utils_1.getAllRect)(this, '.van-tab'),
+ (0, utils_1.getRect)(this, '.van-tabs__nav'),
+ ]).then(function (_a) {
+ var tabRects = _a[0], navRect = _a[1];
+ var tabRect = tabRects[currentIndex];
+ var offsetLeft = tabRects
+ .slice(0, currentIndex)
+ .reduce(function (prev, curr) { return prev + curr.width; }, 0);
+ _this.setData({
+ scrollLeft: offsetLeft - (navRect.width - tabRect.width) / 2,
+ });
+ if (!scrollWithAnimation) {
+ (0, utils_1.nextTick)(function () {
+ _this.setData({ scrollWithAnimation: true });
+ });
+ }
+ });
+ },
+ onTouchScroll: function (event) {
+ this.$emit('scroll', event.detail);
+ },
+ onTouchStart: function (event) {
+ if (!this.data.swipeable)
+ return;
+ this.swiping = true;
+ this.touchStart(event);
+ },
+ onTouchMove: function (event) {
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ this.touchMove(event);
+ },
+ // watch swipe touch end
+ onTouchEnd: function () {
+ var _this = this;
+ if (!this.data.swipeable || !this.swiping)
+ return;
+ var _a = this, direction = _a.direction, deltaX = _a.deltaX, offsetX = _a.offsetX;
+ var minSwipeDistance = 50;
+ if (direction === 'horizontal' && offsetX >= minSwipeDistance) {
+ var index_1 = this.getAvaiableTab(deltaX);
+ if (index_1 !== -1) {
+ this.onBeforeChange(index_1).then(function () { return _this.setCurrentIndex(index_1); });
+ }
+ }
+ this.swiping = false;
+ },
+ getAvaiableTab: function (direction) {
+ var _a = this.data, tabs = _a.tabs, currentIndex = _a.currentIndex;
+ var step = direction > 0 ? -1 : 1;
+ for (var i = step; currentIndex + i < tabs.length && currentIndex + i >= 0; i += step) {
+ var index = currentIndex + i;
+ if (index >= 0 &&
+ index < tabs.length &&
+ tabs[index] &&
+ !tabs[index].disabled) {
+ return index;
+ }
+ }
+ return -1;
+ },
+ onBeforeChange: function (index) {
+ var _this = this;
+ var useBeforeChange = this.data.useBeforeChange;
+ if (!useBeforeChange) {
+ return Promise.resolve();
+ }
+ return new Promise(function (resolve, reject) {
+ _this.$emit('before-change', __assign(__assign({}, _this.getChildData(index)), { callback: function (status) { return (status ? resolve() : reject()); } }));
+ });
+ },
+ getChildData: function (index, child) {
+ var currentChild = child || this.children[index];
+ if (!(0, validator_1.isDef)(currentChild)) {
+ return;
+ }
+ return {
+ index: currentChild.index,
+ name: currentChild.getComputedName(),
+ title: currentChild.data.title,
+ };
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.json
new file mode 100644
index 0000000..19c0bc3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-info": "../info/index",
+ "van-sticky": "../sticky/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxml
new file mode 100644
index 0000000..05bb1e1
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxml
@@ -0,0 +1,63 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.title }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxs
new file mode 100644
index 0000000..4059c7f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxs
@@ -0,0 +1,83 @@
+/* eslint-disable */
+var utils = require('../wxs/utils.wxs');
+var style = require('../wxs/style.wxs');
+
+function tabClass(active, ellipsis) {
+ var classes = ['tab-class'];
+
+ if (active) {
+ classes.push('tab-active-class');
+ }
+
+ if (ellipsis) {
+ classes.push('van-ellipsis');
+ }
+
+ return classes.join(' ');
+}
+
+function tabStyle(data) {
+ var titleColor = data.active
+ ? data.titleActiveColor
+ : data.titleInactiveColor;
+
+ var ellipsis = data.scrollable && data.ellipsis;
+
+ // card theme color
+ if (data.type === 'card') {
+ return style({
+ 'border-color': data.color,
+ 'background-color': !data.disabled && data.active ? data.color : null,
+ color: titleColor || (!data.disabled && !data.active ? data.color : null),
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+ }
+
+ return style({
+ color: titleColor,
+ 'flex-basis': ellipsis ? 88 / data.swipeThreshold + '%' : null,
+ });
+}
+
+function navStyle(color, type) {
+ return style({
+ 'border-color': type === 'card' && color ? color : null,
+ });
+}
+
+function trackStyle(data) {
+ if (!data.animated) {
+ return '';
+ }
+
+ return style({
+ left: -100 * data.currentIndex + '%',
+ 'transition-duration': data.duration + 's',
+ '-webkit-transition-duration': data.duration + 's',
+ });
+}
+
+function lineStyle(data) {
+ return style({
+ width: utils.addUnit(data.lineWidth),
+ opacity: data.inited ? 1 : 0,
+ transform: 'translateX(' + data.lineOffsetLeft + 'px)',
+ '-webkit-transform': 'translateX(' + data.lineOffsetLeft + 'px)',
+ 'background-color': data.color,
+ height: data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'border-radius':
+ data.lineHeight !== -1 ? utils.addUnit(data.lineHeight) : null,
+ 'transition-duration': !data.skipTransition ? data.duration + 's' : null,
+ '-webkit-transition-duration': !data.skipTransition
+ ? data.duration + 's'
+ : null,
+ });
+}
+
+module.exports = {
+ tabClass: tabClass,
+ tabStyle: tabStyle,
+ trackStyle: trackStyle,
+ lineStyle: lineStyle,
+ navStyle: navStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxss
new file mode 100644
index 0000000..09a93af
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tabs/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tabs{-webkit-tap-highlight-color:transparent;position:relative}.van-tabs__wrap{display:flex;overflow:hidden}.van-tabs__wrap--scrollable .van-tab{flex:0 0 22%}.van-tabs__wrap--scrollable .van-tab--complete{flex:1 0 auto!important;padding:0 12px}.van-tabs__wrap--scrollable .van-tabs__nav--complete{padding-left:8px;padding-right:8px}.van-tabs__scroll{background-color:var(--tabs-nav-background-color,#fff);overflow:auto}.van-tabs__scroll--line{box-sizing:initial;height:calc(100% + 15px)}.van-tabs__scroll--card{border:1px solid var(--tabs-default-color,#ee0a24);border-radius:2px;box-sizing:border-box;margin:0 var(--padding-md,16px);width:calc(100% - var(--padding-md, 16px)*2)}.van-tabs__scroll::-webkit-scrollbar{display:none}.van-tabs__nav{display:flex;position:relative;-webkit-user-select:none;user-select:none}.van-tabs__nav--card{box-sizing:border-box;height:var(--tabs-card-height,30px)}.van-tabs__nav--card .van-tab{border-right:1px solid var(--tabs-default-color,#ee0a24);color:var(--tabs-default-color,#ee0a24);line-height:calc(var(--tabs-card-height, 30px) - 2px)}.van-tabs__nav--card .van-tab:last-child{border-right:none}.van-tabs__nav--card .van-tab.van-tab--active{background-color:var(--tabs-default-color,#ee0a24);color:#fff}.van-tabs__nav--card .van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tabs__line{background-color:var(--tabs-bottom-bar-color,#ee0a24);border-radius:var(--tabs-bottom-bar-height,3px);bottom:0;height:var(--tabs-bottom-bar-height,3px);left:0;opacity:0;position:absolute;z-index:1}.van-tabs__track{height:100%;position:relative;width:100%}.van-tabs__track--animated{display:flex;transition-property:left}.van-tabs__content{overflow:hidden}.van-tabs--line{height:var(--tabs-line-height,44px)}.van-tabs--card{height:var(--tabs-card-height,30px)}.van-tab{box-sizing:border-box;color:var(--tab-text-color,#646566);cursor:pointer;flex:1;font-size:var(--tab-font-size,14px);line-height:var(--tabs-line-height,44px);min-width:0;padding:0 5px;position:relative;text-align:center}.van-tab--active{color:var(--tab-active-text-color,#323233);font-weight:var(--font-weight-bold,500)}.van-tab--disabled{color:var(--tab-disabled-text-color,#c8c9cc)}.van-tab__title__info{position:relative!important;top:-1px!important;transform:translateX(0)!important}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.js
new file mode 100644
index 0000000..ec4069a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.js
@@ -0,0 +1,23 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ size: String,
+ mark: Boolean,
+ color: String,
+ plain: Boolean,
+ round: Boolean,
+ textColor: String,
+ type: {
+ type: String,
+ value: 'default',
+ },
+ closeable: Boolean,
+ },
+ methods: {
+ onClose: function () {
+ this.$emit('close');
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.json
new file mode 100644
index 0000000..0a336c0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.json
@@ -0,0 +1,6 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxml
new file mode 100644
index 0000000..59352dd
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxml
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxs
new file mode 100644
index 0000000..12d1668
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style({
+ 'background-color': data.plain ? '' : data.color,
+ color: data.textColor || data.plain ? data.textColor || data.color : '',
+ });
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxss
new file mode 100644
index 0000000..0f0cbae
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tag/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tag{align-items:center;border-radius:var(--tag-border-radius,2px);color:var(--tag-text-color,#fff);display:inline-flex;font-size:var(--tag-font-size,12px);line-height:var(--tag-line-height,16px);padding:var(--tag-padding,0 4px);position:relative}.van-tag--default{background-color:var(--tag-default-color,#969799)}.van-tag--default.van-tag--plain{color:var(--tag-default-color,#969799)}.van-tag--danger{background-color:var(--tag-danger-color,#ee0a24)}.van-tag--danger.van-tag--plain{color:var(--tag-danger-color,#ee0a24)}.van-tag--primary{background-color:var(--tag-primary-color,#1989fa)}.van-tag--primary.van-tag--plain{color:var(--tag-primary-color,#1989fa)}.van-tag--success{background-color:var(--tag-success-color,#07c160)}.van-tag--success.van-tag--plain{color:var(--tag-success-color,#07c160)}.van-tag--warning{background-color:var(--tag-warning-color,#ff976a)}.van-tag--warning.van-tag--plain{color:var(--tag-warning-color,#ff976a)}.van-tag--plain{background-color:var(--tag-plain-background-color,#fff)}.van-tag--plain:before{border:1px solid;border-radius:inherit;bottom:0;content:"";left:0;pointer-events:none;position:absolute;right:0;top:0}.van-tag--medium{padding:var(--tag-medium-padding,2px 6px)}.van-tag--large{border-radius:var(--tag-large-border-radius,4px);font-size:var(--tag-large-font-size,14px);padding:var(--tag-large-padding,4px 8px)}.van-tag--mark{border-radius:0 var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) var(--tag-round-border-radius,var(--tag-round-border-radius,999px)) 0}.van-tag--mark:after{content:"";display:block;width:2px}.van-tag--round{border-radius:var(--tag-round-border-radius,999px)}.van-tag__close{margin-left:2px;min-width:1em}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.js
new file mode 100644
index 0000000..0c01366
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.js
@@ -0,0 +1,31 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ props: {
+ show: Boolean,
+ mask: Boolean,
+ message: String,
+ forbidClick: Boolean,
+ zIndex: {
+ type: Number,
+ value: 1000,
+ },
+ type: {
+ type: String,
+ value: 'text',
+ },
+ loadingType: {
+ type: String,
+ value: 'circular',
+ },
+ position: {
+ type: String,
+ value: 'middle',
+ },
+ },
+ methods: {
+ // for prevent touchmove
+ noop: function () { },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.json
new file mode 100644
index 0000000..9b1b78c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.json
@@ -0,0 +1,9 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index",
+ "van-overlay": "../overlay/index",
+ "van-transition": "../transition/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxml
new file mode 100644
index 0000000..69f143e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxml
@@ -0,0 +1,36 @@
+
+
+
+
+ {{ message }}
+
+
+
+
+
+
+
+
+ {{ message }}
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxss
new file mode 100644
index 0000000..3b7a34e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-toast{word-wrap:break-word;align-items:center;background-color:var(--toast-background-color,rgba(0,0,0,.7));border-radius:var(--toast-border-radius,8px);box-sizing:initial;color:var(--toast-text-color,#fff);display:flex;flex-direction:column;font-size:var(--toast-font-size,14px);justify-content:center;line-height:var(--toast-line-height,20px);white-space:pre-wrap}.van-toast__container{left:50%;max-width:var(--toast-max-width,70%);position:fixed;top:50%;transform:translate(-50%,-50%);width:-webkit-fit-content;width:fit-content}.van-toast--text{min-width:var(--toast-text-min-width,96px);padding:var(--toast-text-padding,8px 12px)}.van-toast--icon{min-height:var(--toast-default-min-height,88px);padding:var(--toast-default-padding,16px);width:var(--toast-default-width,88px)}.van-toast--icon .van-toast__icon{font-size:var(--toast-icon-size,36px)}.van-toast--icon .van-toast__text{padding-top:8px}.van-toast__loading{margin:10px 0}.van-toast--top{transform:translateY(-30vh)}.van-toast--bottom{transform:translateY(30vh)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.d.ts
new file mode 100644
index 0000000..de2a4a2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.d.ts
@@ -0,0 +1,28 @@
+///
+///
+type ToastMessage = string | number;
+type ToastContext = WechatMiniprogram.Component.TrivialInstance | WechatMiniprogram.Page.TrivialInstance;
+interface ToastOptions {
+ show?: boolean;
+ type?: string;
+ mask?: boolean;
+ zIndex?: number;
+ context?: (() => ToastContext) | ToastContext;
+ position?: string;
+ duration?: number;
+ selector?: string;
+ forbidClick?: boolean;
+ loadingType?: string;
+ message?: ToastMessage;
+ onClose?: () => void;
+}
+declare function Toast(toastOptions: ToastOptions | ToastMessage): WechatMiniprogram.Component.TrivialInstance | undefined;
+declare namespace Toast {
+ var loading: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var success: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var fail: (options: ToastMessage | ToastOptions) => WechatMiniprogram.Component.TrivialInstance | undefined;
+ var clear: () => void;
+ var setDefaultOptions: (options: ToastOptions) => void;
+ var resetDefaultOptions: () => void;
+}
+export default Toast;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.js
new file mode 100644
index 0000000..f51a89a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/toast/toast.js
@@ -0,0 +1,83 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var validator_1 = require("../common/validator");
+var defaultOptions = {
+ type: 'text',
+ mask: false,
+ message: '',
+ show: true,
+ zIndex: 1000,
+ duration: 2000,
+ position: 'middle',
+ forbidClick: false,
+ loadingType: 'circular',
+ selector: '#van-toast',
+};
+var queue = [];
+var currentOptions = __assign({}, defaultOptions);
+function parseOptions(message) {
+ return (0, validator_1.isObj)(message) ? message : { message: message };
+}
+function getContext() {
+ var pages = getCurrentPages();
+ return pages[pages.length - 1];
+}
+function Toast(toastOptions) {
+ var options = __assign(__assign({}, currentOptions), parseOptions(toastOptions));
+ var context = (typeof options.context === 'function'
+ ? options.context()
+ : options.context) || getContext();
+ var toast = context.selectComponent(options.selector);
+ if (!toast) {
+ console.warn('未找到 van-toast 节点,请确认 selector 及 context 是否正确');
+ return;
+ }
+ delete options.context;
+ delete options.selector;
+ toast.clear = function () {
+ toast.setData({ show: false });
+ if (options.onClose) {
+ options.onClose();
+ }
+ };
+ queue.push(toast);
+ toast.setData(options);
+ clearTimeout(toast.timer);
+ if (options.duration != null && options.duration > 0) {
+ toast.timer = setTimeout(function () {
+ toast.clear();
+ queue = queue.filter(function (item) { return item !== toast; });
+ }, options.duration);
+ }
+ return toast;
+}
+var createMethod = function (type) { return function (options) {
+ return Toast(__assign({ type: type }, parseOptions(options)));
+}; };
+Toast.loading = createMethod('loading');
+Toast.success = createMethod('success');
+Toast.fail = createMethod('fail');
+Toast.clear = function () {
+ queue.forEach(function (toast) {
+ toast.clear();
+ });
+ queue = [];
+};
+Toast.setDefaultOptions = function (options) {
+ Object.assign(currentOptions, options);
+};
+Toast.resetDefaultOptions = function () {
+ currentOptions = __assign({}, defaultOptions);
+};
+exports.default = Toast;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.js
new file mode 100644
index 0000000..55fc8b8
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.js
@@ -0,0 +1,15 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var transition_1 = require("../mixins/transition");
+(0, component_1.VantComponent)({
+ classes: [
+ 'enter-class',
+ 'enter-active-class',
+ 'enter-to-class',
+ 'leave-class',
+ 'leave-active-class',
+ 'leave-to-class',
+ ],
+ mixins: [(0, transition_1.transition)(true)],
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.json
new file mode 100644
index 0000000..467ce29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.json
@@ -0,0 +1,3 @@
+{
+ "component": true
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxml
new file mode 100644
index 0000000..2743785
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxml
@@ -0,0 +1,10 @@
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxs
new file mode 100644
index 0000000..e0babf6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+
+function rootStyle(data) {
+ return style([
+ {
+ '-webkit-transition-duration': data.currentDuration + 'ms',
+ 'transition-duration': data.currentDuration + 'ms',
+ },
+ data.display ? null : 'display: none',
+ data.customStyle,
+ ]);
+}
+
+module.exports = {
+ rootStyle: rootStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxss
new file mode 100644
index 0000000..3a3d37f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/transition/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-transition{transition-timing-function:ease}.van-fade-enter-active,.van-fade-leave-active{transition-property:opacity}.van-fade-enter,.van-fade-leave-to{opacity:0}.van-fade-down-enter-active,.van-fade-down-leave-active,.van-fade-left-enter-active,.van-fade-left-leave-active,.van-fade-right-enter-active,.van-fade-right-leave-active,.van-fade-up-enter-active,.van-fade-up-leave-active{transition-property:opacity,transform}.van-fade-up-enter,.van-fade-up-leave-to{opacity:0;transform:translate3d(0,100%,0)}.van-fade-down-enter,.van-fade-down-leave-to{opacity:0;transform:translate3d(0,-100%,0)}.van-fade-left-enter,.van-fade-left-leave-to{opacity:0;transform:translate3d(-100%,0,0)}.van-fade-right-enter,.van-fade-right-leave-to{opacity:0;transform:translate3d(100%,0,0)}.van-slide-down-enter-active,.van-slide-down-leave-active,.van-slide-left-enter-active,.van-slide-left-leave-active,.van-slide-right-enter-active,.van-slide-right-leave-active,.van-slide-up-enter-active,.van-slide-up-leave-active{transition-property:transform}.van-slide-up-enter,.van-slide-up-leave-to{transform:translate3d(0,100%,0)}.van-slide-down-enter,.van-slide-down-leave-to{transform:translate3d(0,-100%,0)}.van-slide-left-enter,.van-slide-left-leave-to{transform:translate3d(-100%,0,0)}.van-slide-right-enter,.van-slide-right-leave-to{transform:translate3d(100%,0,0)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.js
new file mode 100644
index 0000000..b6f69b2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.js
@@ -0,0 +1,70 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+(0, component_1.VantComponent)({
+ classes: [
+ 'main-item-class',
+ 'content-item-class',
+ 'main-active-class',
+ 'content-active-class',
+ 'main-disabled-class',
+ 'content-disabled-class',
+ ],
+ props: {
+ items: {
+ type: Array,
+ observer: 'updateSubItems',
+ },
+ activeId: null,
+ mainActiveIndex: {
+ type: Number,
+ value: 0,
+ observer: 'updateSubItems',
+ },
+ height: {
+ type: null,
+ value: 300,
+ },
+ max: {
+ type: Number,
+ value: Infinity,
+ },
+ selectedIcon: {
+ type: String,
+ value: 'success',
+ },
+ },
+ data: {
+ subItems: [],
+ },
+ methods: {
+ // 当一个子项被选择时
+ onSelectItem: function (event) {
+ var item = event.currentTarget.dataset.item;
+ var isArray = Array.isArray(this.data.activeId);
+ // 判断有没有超出右侧选择的最大数
+ var isOverMax = isArray && this.data.activeId.length >= this.data.max;
+ // 判断该项有没有被选中, 如果有被选中,则忽视是否超出的条件
+ var isSelected = isArray
+ ? this.data.activeId.indexOf(item.id) > -1
+ : this.data.activeId === item.id;
+ if (!item.disabled && (!isOverMax || isSelected)) {
+ this.$emit('click-item', item);
+ }
+ },
+ // 当一个导航被点击时
+ onClickNav: function (event) {
+ var index = event.detail;
+ var item = this.data.items[index];
+ if (!item.disabled) {
+ this.$emit('click-nav', { index: index });
+ }
+ },
+ // 更新子项列表
+ updateSubItems: function () {
+ var _a = this.data, items = _a.items, mainActiveIndex = _a.mainActiveIndex;
+ var _b = (items[mainActiveIndex] || {}).children, children = _b === void 0 ? [] : _b;
+ this.setData({ subItems: children });
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.json
new file mode 100644
index 0000000..42991a2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.json
@@ -0,0 +1,8 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-sidebar": "../sidebar/index",
+ "van-sidebar-item": "../sidebar-item/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxml
new file mode 100644
index 0000000..2663e52
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxml
@@ -0,0 +1,41 @@
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.text }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxs
new file mode 100644
index 0000000..b1cbb39
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var array = require('../wxs/array.wxs');
+
+function isActive (activeList, itemId) {
+ if (array.isArray(activeList)) {
+ return activeList.indexOf(itemId) > -1;
+ }
+
+ return activeList === itemId;
+}
+
+module.exports.isActive = isActive;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxss
new file mode 100644
index 0000000..5bef0ac
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/tree-select/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-tree-select{display:flex;font-size:var(--tree-select-font-size,14px);position:relative;-webkit-user-select:none;user-select:none}.van-tree-select__nav{--sidebar-padding:12px 8px 12px 12px;background-color:var(--tree-select-nav-background-color,#f7f8fa);flex:1}.van-tree-select__nav__inner{height:100%;width:100%!important}.van-tree-select__content{background-color:var(--tree-select-content-background-color,#fff);flex:2}.van-tree-select__item{font-weight:700;line-height:var(--tree-select-item-height,44px);padding:0 32px 0 var(--padding-md,16px);position:relative}.van-tree-select__item--active{color:var(--tree-select-item-active-color,#ee0a24)}.van-tree-select__item--disabled{color:var(--tree-select-item-disabled-color,#c8c9cc)}.van-tree-select__selected{position:absolute;right:var(--padding-md,16px);top:50%;transform:translateY(-50%)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.d.ts
new file mode 100644
index 0000000..cb0ff5c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.d.ts
@@ -0,0 +1 @@
+export {};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.js
new file mode 100644
index 0000000..5492d40
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.js
@@ -0,0 +1,183 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+var component_1 = require("../common/component");
+var utils_1 = require("./utils");
+var shared_1 = require("./shared");
+var validator_1 = require("../common/validator");
+(0, component_1.VantComponent)({
+ props: __assign(__assign(__assign(__assign({ disabled: Boolean, multiple: Boolean, uploadText: String, useBeforeRead: Boolean, afterRead: null, beforeRead: null, previewSize: {
+ type: null,
+ value: 80,
+ }, name: {
+ type: null,
+ value: '',
+ }, accept: {
+ type: String,
+ value: 'image',
+ }, fileList: {
+ type: Array,
+ value: [],
+ observer: 'formatFileList',
+ }, maxSize: {
+ type: Number,
+ value: Number.MAX_VALUE,
+ }, maxCount: {
+ type: Number,
+ value: 100,
+ }, deletable: {
+ type: Boolean,
+ value: true,
+ }, showUpload: {
+ type: Boolean,
+ value: true,
+ }, previewImage: {
+ type: Boolean,
+ value: true,
+ }, previewFullImage: {
+ type: Boolean,
+ value: true,
+ }, videoFit: {
+ type: String,
+ value: 'contain',
+ }, imageFit: {
+ type: String,
+ value: 'scaleToFill',
+ }, uploadIcon: {
+ type: String,
+ value: 'photograph',
+ } }, shared_1.imageProps), shared_1.videoProps), shared_1.mediaProps), shared_1.messageFileProps),
+ data: {
+ lists: [],
+ isInCount: true,
+ },
+ methods: {
+ formatFileList: function () {
+ var _a = this.data, _b = _a.fileList, fileList = _b === void 0 ? [] : _b, maxCount = _a.maxCount;
+ var lists = fileList.map(function (item) { return (__assign(__assign({}, item), { isImage: (0, utils_1.isImageFile)(item), isVideo: (0, utils_1.isVideoFile)(item), deletable: (0, validator_1.isBoolean)(item.deletable) ? item.deletable : true })); });
+ this.setData({ lists: lists, isInCount: lists.length < maxCount });
+ },
+ getDetail: function (index) {
+ return {
+ name: this.data.name,
+ index: index == null ? this.data.fileList.length : index,
+ };
+ },
+ startUpload: function () {
+ var _this = this;
+ var _a = this.data, maxCount = _a.maxCount, multiple = _a.multiple, lists = _a.lists, disabled = _a.disabled;
+ if (disabled)
+ return;
+ (0, utils_1.chooseFile)(__assign(__assign({}, this.data), { maxCount: maxCount - lists.length }))
+ .then(function (res) {
+ _this.onBeforeRead(multiple ? res : res[0]);
+ })
+ .catch(function (error) {
+ _this.$emit('error', error);
+ });
+ },
+ onBeforeRead: function (file) {
+ var _this = this;
+ var _a = this.data, beforeRead = _a.beforeRead, useBeforeRead = _a.useBeforeRead;
+ var res = true;
+ if (typeof beforeRead === 'function') {
+ res = beforeRead(file, this.getDetail());
+ }
+ if (useBeforeRead) {
+ res = new Promise(function (resolve, reject) {
+ _this.$emit('before-read', __assign(__assign({ file: file }, _this.getDetail()), { callback: function (ok) {
+ ok ? resolve() : reject();
+ } }));
+ });
+ }
+ if (!res) {
+ return;
+ }
+ if ((0, validator_1.isPromise)(res)) {
+ res.then(function (data) { return _this.onAfterRead(data || file); });
+ }
+ else {
+ this.onAfterRead(file);
+ }
+ },
+ onAfterRead: function (file) {
+ var _a = this.data, maxSize = _a.maxSize, afterRead = _a.afterRead;
+ var oversize = Array.isArray(file)
+ ? file.some(function (item) { return item.size > maxSize; })
+ : file.size > maxSize;
+ if (oversize) {
+ this.$emit('oversize', __assign({ file: file }, this.getDetail()));
+ return;
+ }
+ if (typeof afterRead === 'function') {
+ afterRead(file, this.getDetail());
+ }
+ this.$emit('after-read', __assign({ file: file }, this.getDetail()));
+ },
+ deleteItem: function (event) {
+ var index = event.currentTarget.dataset.index;
+ this.$emit('delete', __assign(__assign({}, this.getDetail(index)), { file: this.data.fileList[index] }));
+ },
+ onPreviewImage: function (event) {
+ if (!this.data.previewFullImage)
+ return;
+ var index = event.currentTarget.dataset.index;
+ var _a = this.data, lists = _a.lists, showmenu = _a.showmenu;
+ var item = lists[index];
+ wx.previewImage({
+ urls: lists.filter(function (item) { return (0, utils_1.isImageFile)(item); }).map(function (item) { return item.url; }),
+ current: item.url,
+ showmenu: showmenu,
+ fail: function () {
+ wx.showToast({ title: '预览图片失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewVideo: function (event) {
+ if (!this.data.previewFullImage)
+ return;
+ var index = event.currentTarget.dataset.index;
+ var lists = this.data.lists;
+ var sources = [];
+ var current = lists.reduce(function (sum, cur, curIndex) {
+ if (!(0, utils_1.isVideoFile)(cur)) {
+ return sum;
+ }
+ sources.push(__assign(__assign({}, cur), { type: 'video' }));
+ if (curIndex < index) {
+ sum++;
+ }
+ return sum;
+ }, 0);
+ wx.previewMedia({
+ sources: sources,
+ current: current,
+ fail: function () {
+ wx.showToast({ title: '预览视频失败', icon: 'none' });
+ },
+ });
+ },
+ onPreviewFile: function (event) {
+ var index = event.currentTarget.dataset.index;
+ wx.openDocument({
+ filePath: this.data.lists[index].url,
+ showMenu: true,
+ });
+ },
+ onClickPreview: function (event) {
+ var index = event.currentTarget.dataset.index;
+ var item = this.data.lists[index];
+ this.$emit('click-preview', __assign(__assign({}, item), this.getDetail(index)));
+ },
+ },
+});
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.json b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.json
new file mode 100644
index 0000000..e00a588
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.json
@@ -0,0 +1,7 @@
+{
+ "component": true,
+ "usingComponents": {
+ "van-icon": "../icon/index",
+ "van-loading": "../loading/index"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxml b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxml
new file mode 100644
index 0000000..3e61fd9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxml
@@ -0,0 +1,84 @@
+
+
+
+
+
+
+
+
+
+
+
+ {{ item.name || item.url }}
+
+
+
+
+ {{ item.message }}
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {{ uploadText }}
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxs
new file mode 100644
index 0000000..c567ec2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxs
@@ -0,0 +1,17 @@
+/* eslint-disable */
+var style = require('../wxs/style.wxs');
+var addUnit = require('../wxs/add-unit.wxs');
+
+function sizeStyle(data) {
+ return "Array" === data.previewSize.constructor ? style({
+ width: addUnit(data.previewSize[0]),
+ height: addUnit(data.previewSize[1]),
+ }) : style({
+ width: addUnit(data.previewSize),
+ height: addUnit(data.previewSize),
+ });
+}
+
+module.exports = {
+ sizeStyle: sizeStyle,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxss b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxss
new file mode 100644
index 0000000..11f8696
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/index.wxss
@@ -0,0 +1 @@
+@import '../common/index.wxss';.van-uploader{display:inline-block;position:relative}.van-uploader__wrapper{display:flex;flex-wrap:wrap}.van-uploader__slot:empty{display:none}.van-uploader__slot:not(:empty)+.van-uploader__upload{display:none!important}.van-uploader__upload{align-items:center;background-color:var(--uploader-upload-background-color,#f7f8fa);box-sizing:border-box;display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;margin:0 8px 8px 0;position:relative;width:var(--uploader-size,80px)}.van-uploader__upload:active{background-color:var(--uploader-upload-active-color,#f2f3f5)}.van-uploader__upload-icon{color:var(--uploader-icon-color,#dcdee0);font-size:var(--uploader-icon-size,24px)}.van-uploader__upload-text{color:var(--uploader-text-color,#969799);font-size:var(--uploader-text-font-size,12px);margin-top:var(--padding-xs,8px)}.van-uploader__upload--disabled{opacity:var(--uploader-disabled-opacity,.5)}.van-uploader__preview{cursor:pointer;margin:0 8px 8px 0;position:relative}.van-uploader__preview-image{display:block;height:var(--uploader-size,80px);overflow:hidden;width:var(--uploader-size,80px)}.van-uploader__preview-delete,.van-uploader__preview-delete:after{height:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;width:var(--uploader-delete-icon-size,14px)}.van-uploader__preview-delete:after{background-color:var(--uploader-delete-background-color,rgba(0,0,0,.7));border-radius:0 0 0 12px;content:""}.van-uploader__preview-delete-icon{color:var(--uploader-delete-color,#fff);font-size:var(--uploader-delete-icon-size,14px);position:absolute;right:0;top:0;transform:scale(.7) translate(10%,-10%);z-index:1}.van-uploader__file{align-items:center;background-color:var(--uploader-file-background-color,#f7f8fa);display:flex;flex-direction:column;height:var(--uploader-size,80px);justify-content:center;width:var(--uploader-size,80px)}.van-uploader__file-icon{color:var(--uploader-file-icon-color,#646566);font-size:var(--uploader-file-icon-size,20px)}.van-uploader__file-name{box-sizing:border-box;color:var(--uploader-file-name-text-color,#646566);font-size:var(--uploader-file-name-font-size,12px);margin-top:var(--uploader-file-name-margin-top,8px);padding:var(--uploader-file-name-padding,0 4px);text-align:center;width:100%}.van-uploader__mask{align-items:center;background-color:var(--uploader-mask-background-color,rgba(50,50,51,.88));bottom:0;color:#fff;display:flex;flex-direction:column;justify-content:center;left:0;position:absolute;right:0;top:0}.van-uploader__mask-icon{font-size:var(--uploader-mask-icon-size,22px)}.van-uploader__mask-message{font-size:var(--uploader-mask-message-font-size,12px);line-height:var(--uploader-mask-message-line-height,14px);margin-top:6px;padding:0 var(--padding-base,4px)}.van-uploader__loading{color:var(--uploader-loading-icon-color,#fff)!important;height:var(--uploader-loading-icon-size,22px);width:var(--uploader-loading-icon-size,22px)}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.d.ts
new file mode 100644
index 0000000..e0a0d7e
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.d.ts
@@ -0,0 +1,53 @@
+export declare const imageProps: {
+ sizeType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ showmenu: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+};
+export declare const videoProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ compressed: {
+ type: BooleanConstructor;
+ value: boolean;
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const mediaProps: {
+ capture: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ mediaType: {
+ type: ArrayConstructor;
+ value: string[];
+ };
+ maxDuration: {
+ type: NumberConstructor;
+ value: number;
+ };
+ camera: {
+ type: StringConstructor;
+ value: string;
+ };
+};
+export declare const messageFileProps: {
+ extension: null;
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.js
new file mode 100644
index 0000000..b88fea7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/shared.js
@@ -0,0 +1,60 @@
+"use strict";
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.messageFileProps = exports.mediaProps = exports.videoProps = exports.imageProps = void 0;
+// props for image
+exports.imageProps = {
+ sizeType: {
+ type: Array,
+ value: ['original', 'compressed'],
+ },
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ showmenu: {
+ type: Boolean,
+ value: true,
+ },
+};
+// props for video
+exports.videoProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ compressed: {
+ type: Boolean,
+ value: true,
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for media
+exports.mediaProps = {
+ capture: {
+ type: Array,
+ value: ['album', 'camera'],
+ },
+ mediaType: {
+ type: Array,
+ value: ['image', 'video', 'mix'],
+ },
+ maxDuration: {
+ type: Number,
+ value: 60,
+ },
+ camera: {
+ type: String,
+ value: 'back',
+ },
+};
+// props for file
+exports.messageFileProps = {
+ extension: null,
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.d.ts b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.d.ts
new file mode 100644
index 0000000..1e76ee6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.d.ts
@@ -0,0 +1,24 @@
+export interface File {
+ url: string;
+ size?: number;
+ name?: string;
+ type: string;
+ duration?: number;
+ time?: number;
+ isImage?: boolean;
+ isVideo?: boolean;
+}
+export declare function isImageFile(item: File): boolean;
+export declare function isVideoFile(item: File): boolean;
+export declare function chooseFile({ accept, multiple, capture, compressed, maxDuration, sizeType, camera, maxCount, mediaType, extension, }: {
+ accept: any;
+ multiple: any;
+ capture: any;
+ compressed: any;
+ maxDuration: any;
+ sizeType: any;
+ camera: any;
+ maxCount: any;
+ mediaType: any;
+ extension: any;
+}): Promise;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.js b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.js
new file mode 100644
index 0000000..a5d49c6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/uploader/utils.js
@@ -0,0 +1,112 @@
+"use strict";
+var __assign = (this && this.__assign) || function () {
+ __assign = Object.assign || function(t) {
+ for (var s, i = 1, n = arguments.length; i < n; i++) {
+ s = arguments[i];
+ for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p))
+ t[p] = s[p];
+ }
+ return t;
+ };
+ return __assign.apply(this, arguments);
+};
+Object.defineProperty(exports, "__esModule", { value: true });
+exports.chooseFile = exports.isVideoFile = exports.isImageFile = void 0;
+var utils_1 = require("../common/utils");
+var validator_1 = require("../common/validator");
+function isImageFile(item) {
+ if (item.isImage != null) {
+ return item.isImage;
+ }
+ if (item.type) {
+ return item.type === 'image';
+ }
+ if (item.url) {
+ return (0, validator_1.isImageUrl)(item.url);
+ }
+ return false;
+}
+exports.isImageFile = isImageFile;
+function isVideoFile(item) {
+ if (item.isVideo != null) {
+ return item.isVideo;
+ }
+ if (item.type) {
+ return item.type === 'video';
+ }
+ if (item.url) {
+ return (0, validator_1.isVideoUrl)(item.url);
+ }
+ return false;
+}
+exports.isVideoFile = isVideoFile;
+function formatImage(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['path'])), { type: 'image', url: item.tempFilePath || item.path, thumb: item.tempFilePath || item.path })); });
+}
+function formatVideo(res) {
+ return [
+ __assign(__assign({}, (0, utils_1.pickExclude)(res, ['tempFilePath', 'thumbTempFilePath', 'errMsg'])), { type: 'video', url: res.tempFilePath, thumb: res.thumbTempFilePath }),
+ ];
+}
+function formatMedia(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['fileType', 'thumbTempFilePath', 'tempFilePath'])), { type: item.fileType, url: item.tempFilePath, thumb: item.fileType === 'video' ? item.thumbTempFilePath : item.tempFilePath })); });
+}
+function formatFile(res) {
+ return res.tempFiles.map(function (item) { return (__assign(__assign({}, (0, utils_1.pickExclude)(item, ['path'])), { url: item.path })); });
+}
+function chooseFile(_a) {
+ var accept = _a.accept, multiple = _a.multiple, capture = _a.capture, compressed = _a.compressed, maxDuration = _a.maxDuration, sizeType = _a.sizeType, camera = _a.camera, maxCount = _a.maxCount, mediaType = _a.mediaType, extension = _a.extension;
+ return new Promise(function (resolve, reject) {
+ switch (accept) {
+ case 'image':
+ if (utils_1.isPC || utils_1.isWxWork) {
+ wx.chooseImage({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ sourceType: capture,
+ sizeType: sizeType,
+ success: function (res) { return resolve(formatImage(res)); },
+ fail: reject,
+ });
+ }
+ else {
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType: ['image'],
+ sourceType: capture,
+ maxDuration: maxDuration,
+ sizeType: sizeType,
+ camera: camera,
+ success: function (res) { return resolve(formatImage(res)); },
+ fail: reject,
+ });
+ }
+ break;
+ case 'media':
+ wx.chooseMedia({
+ count: multiple ? Math.min(maxCount, 9) : 1,
+ mediaType: mediaType,
+ sourceType: capture,
+ maxDuration: maxDuration,
+ sizeType: sizeType,
+ camera: camera,
+ success: function (res) { return resolve(formatMedia(res)); },
+ fail: reject,
+ });
+ break;
+ case 'video':
+ wx.chooseVideo({
+ sourceType: capture,
+ compressed: compressed,
+ maxDuration: maxDuration,
+ camera: camera,
+ success: function (res) { return resolve(formatVideo(res)); },
+ fail: reject,
+ });
+ break;
+ default:
+ wx.chooseMessageFile(__assign(__assign({ count: multiple ? maxCount : 1, type: accept }, (extension ? { extension: extension } : {})), { success: function (res) { return resolve(formatFile(res)); }, fail: reject }));
+ break;
+ }
+ });
+}
+exports.chooseFile = chooseFile;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/add-unit.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/add-unit.wxs
new file mode 100644
index 0000000..4f33462
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/add-unit.wxs
@@ -0,0 +1,12 @@
+/* eslint-disable */
+var REGEXP = getRegExp('^-?\d+(\.\d+)?$');
+
+function addUnit(value) {
+ if (value == null) {
+ return undefined;
+ }
+
+ return REGEXP.test('' + value) ? value + 'px' : value;
+}
+
+module.exports = addUnit;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/array.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/array.wxs
new file mode 100644
index 0000000..610089c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/array.wxs
@@ -0,0 +1,5 @@
+function isArray(array) {
+ return array && array.constructor === 'Array';
+}
+
+module.exports.isArray = isArray;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/bem.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/bem.wxs
new file mode 100644
index 0000000..1efa129
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/bem.wxs
@@ -0,0 +1,39 @@
+/* eslint-disable */
+var array = require('./array.wxs');
+var object = require('./object.wxs');
+var PREFIX = 'van-';
+
+function join(name, mods) {
+ name = PREFIX + name;
+ mods = mods.map(function(mod) {
+ return name + '--' + mod;
+ });
+ mods.unshift(name);
+ return mods.join(' ');
+}
+
+function traversing(mods, conf) {
+ if (!conf) {
+ return;
+ }
+
+ if (typeof conf === 'string' || typeof conf === 'number') {
+ mods.push(conf);
+ } else if (array.isArray(conf)) {
+ conf.forEach(function(item) {
+ traversing(mods, item);
+ });
+ } else if (typeof conf === 'object') {
+ object.keys(conf).forEach(function(key) {
+ conf[key] && mods.push(key);
+ });
+ }
+}
+
+function bem(name, conf) {
+ var mods = [];
+ traversing(mods, conf);
+ return join(name, mods);
+}
+
+module.exports = bem;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/memoize.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/memoize.wxs
new file mode 100644
index 0000000..8f7f46d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/memoize.wxs
@@ -0,0 +1,55 @@
+/**
+ * Simple memoize
+ * wxs doesn't support fn.apply, so this memoize only support up to 2 args
+ */
+/* eslint-disable */
+
+function isPrimitive(value) {
+ var type = typeof value;
+ return (
+ type === 'boolean' ||
+ type === 'number' ||
+ type === 'string' ||
+ type === 'undefined' ||
+ value === null
+ );
+}
+
+// mock simple fn.call in wxs
+function call(fn, args) {
+ if (args.length === 2) {
+ return fn(args[0], args[1]);
+ }
+
+ if (args.length === 1) {
+ return fn(args[0]);
+ }
+
+ return fn();
+}
+
+function serializer(args) {
+ if (args.length === 1 && isPrimitive(args[0])) {
+ return args[0];
+ }
+ var obj = {};
+ for (var i = 0; i < args.length; i++) {
+ obj['key' + i] = args[i];
+ }
+ return JSON.stringify(obj);
+}
+
+function memoize(fn) {
+ var cache = {};
+
+ return function() {
+ var key = serializer(arguments);
+ if (cache[key] === undefined) {
+ cache[key] = call(fn, arguments);
+ }
+
+ return cache[key];
+ };
+}
+
+module.exports = memoize;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/object.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/object.wxs
new file mode 100644
index 0000000..e077107
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/object.wxs
@@ -0,0 +1,13 @@
+/* eslint-disable */
+var REGEXP = getRegExp('{|}|"', 'g');
+
+function keys(obj) {
+ return JSON.stringify(obj)
+ .replace(REGEXP, '')
+ .split(',')
+ .map(function(item) {
+ return item.split(':')[0];
+ });
+}
+
+module.exports.keys = keys;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/style.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/style.wxs
new file mode 100644
index 0000000..d88ca7c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/style.wxs
@@ -0,0 +1,42 @@
+/* eslint-disable */
+var object = require('./object.wxs');
+var array = require('./array.wxs');
+
+function kebabCase(word) {
+ var newWord = word
+ .replace(getRegExp("[A-Z]", 'g'), function (i) {
+ return '-' + i;
+ })
+ .toLowerCase()
+
+ return newWord;
+}
+
+function style(styles) {
+ if (array.isArray(styles)) {
+ return styles
+ .filter(function (item) {
+ return item != null && item !== '';
+ })
+ .map(function (item) {
+ return style(item);
+ })
+ .join(';');
+ }
+
+ if ('Object' === styles.constructor) {
+ return object
+ .keys(styles)
+ .filter(function (key) {
+ return styles[key] != null && styles[key] !== '';
+ })
+ .map(function (key) {
+ return [kebabCase(key), [styles[key]]].join(':');
+ })
+ .join(';');
+ }
+
+ return styles;
+}
+
+module.exports = style;
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/utils.wxs b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/utils.wxs
new file mode 100644
index 0000000..f66d33a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/lib/wxs/utils.wxs
@@ -0,0 +1,10 @@
+/* eslint-disable */
+var bem = require('./bem.wxs');
+var memoize = require('./memoize.wxs');
+var addUnit = require('./add-unit.wxs');
+
+module.exports = {
+ bem: memoize(bem),
+ memoize: memoize,
+ addUnit: addUnit
+};
diff --git a/src/miniprogram-4/node_modules/@vant/weapp/package.json b/src/miniprogram-4/node_modules/@vant/weapp/package.json
new file mode 100644
index 0000000..d37d7e7
--- /dev/null
+++ b/src/miniprogram-4/node_modules/@vant/weapp/package.json
@@ -0,0 +1,71 @@
+{
+ "name": "@vant/weapp",
+ "version": "1.11.3",
+ "author": "vant-ui",
+ "license": "MIT",
+ "miniprogram": "lib",
+ "description": "轻量、可靠的小程序 UI 组件库",
+ "publishConfig": {
+ "access": "public",
+ "registry": "https://registry.npmjs.org/"
+ },
+ "scripts": {
+ "dev": "node build/dev.mjs",
+ "lint": "eslint ./packages --ext .js,.ts --fix && stylelint \"packages/**/*.less\" --fix",
+ "prepare": "husky install",
+ "release": "sh build/release.sh",
+ "release:site": "vant-cli build-site && gh-pages -d site-dist --add",
+ "build:lib": "yarn && npx gulp -f build/compiler.js --series buildEs buildLib",
+ "build:changelog": "vant-cli changelog",
+ "upload:weapp": "node build/upload.js",
+ "test": "jest",
+ "test:watch": "jest --watch"
+ },
+ "files": [
+ "dist",
+ "lib"
+ ],
+ "repository": {
+ "type": "git",
+ "url": "git+ssh://git@github.com/youzan/vant-weapp.git"
+ },
+ "lint-staged": {
+ "*.{ts,js}": [
+ "eslint --fix",
+ "prettier --write"
+ ],
+ "*.{css,less}": [
+ "stylelint --fix",
+ "prettier --write"
+ ]
+ },
+ "homepage": "https://github.com/youzan/vant-weapp#readme",
+ "devDependencies": {
+ "@babel/plugin-transform-modules-commonjs": "^7.16.0",
+ "@babel/preset-typescript": "^7.16.0",
+ "@types/jest": "^27.0.2",
+ "@vant/cli": "^4.0.0",
+ "@vant/icons": "^3.0.1",
+ "gulp": "^4.0.2",
+ "gulp-insert": "^0.5.0",
+ "gulp-less": "^5.0.0",
+ "gulp-postcss": "^9.0.1",
+ "gulp-rename": "^2.0.0",
+ "gulp-typescript": "^6.0.0-alpha.1",
+ "jest": "^27.3.1",
+ "lint-staged": "^13.0.3",
+ "merge2": "^1.4.1",
+ "miniprogram-api-typings": "^3.1.6",
+ "miniprogram-ci": "^1.6.1",
+ "miniprogram-simulate": "^1.4.2",
+ "ts-jest": "^27.0.7",
+ "tscpaths": "^0.0.9",
+ "typescript": "^4.4.4",
+ "vue": "^3.2.30"
+ },
+ "browserslist": [
+ "Chrome >= 53",
+ "ChromeAndroid >= 53",
+ "iOS >= 9"
+ ]
+}
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.babelrc b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.babelrc
new file mode 100644
index 0000000..0b40319
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.babelrc
@@ -0,0 +1,11 @@
+{
+ "plugins": [
+ ["module-resolver", {
+ "root": ["./src"],
+ "alias": {}
+ }]
+ ],
+ "presets": [
+ ["env", {"loose": true, "modules": "commonjs"}]
+ ]
+}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.eslintrc.js b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.eslintrc.js
new file mode 100644
index 0000000..1f42ef5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/.eslintrc.js
@@ -0,0 +1,96 @@
+module.exports = {
+ 'extends': [
+ 'airbnb-base',
+ 'plugin:promise/recommended'
+ ],
+ 'parserOptions': {
+ 'ecmaVersion': 9,
+ 'ecmaFeatures': {
+ 'jsx': false
+ },
+ 'sourceType': 'module'
+ },
+ 'env': {
+ 'es6': true,
+ 'node': true,
+ 'jest': true
+ },
+ 'plugins': [
+ 'import',
+ 'node',
+ 'promise'
+ ],
+ 'rules': {
+ 'arrow-parens': 'off',
+ 'comma-dangle': [
+ 'error',
+ 'only-multiline'
+ ],
+ 'complexity': ['error', 10],
+ 'func-names': 'off',
+ 'global-require': 'off',
+ 'handle-callback-err': [
+ 'error',
+ '^(err|error)$'
+ ],
+ 'import/no-unresolved': [
+ 'error',
+ {
+ 'caseSensitive': true,
+ 'commonjs': true,
+ 'ignore': ['^[^.]']
+ }
+ ],
+ 'import/prefer-default-export': 'off',
+ 'linebreak-style': 'off',
+ 'no-catch-shadow': 'error',
+ 'no-continue': 'off',
+ 'no-div-regex': 'warn',
+ 'no-else-return': 'off',
+ 'no-param-reassign': 'off',
+ 'no-plusplus': 'off',
+ 'no-shadow': 'off',
+ 'no-multi-assign': 'off',
+ 'no-underscore-dangle': 'off',
+ 'node/no-deprecated-api': 'error',
+ 'node/process-exit-as-throw': 'error',
+ 'object-curly-spacing': [
+ 'error',
+ 'never'
+ ],
+ 'operator-linebreak': [
+ 'error',
+ 'after',
+ {
+ 'overrides': {
+ ':': 'before',
+ '?': 'before'
+ }
+ }
+ ],
+ 'prefer-arrow-callback': 'off',
+ 'prefer-destructuring': 'off',
+ 'prefer-template': 'off',
+ 'quote-props': [
+ 1,
+ 'as-needed',
+ {
+ 'unnecessary': true
+ }
+ ],
+ 'semi': [
+ 'error',
+ 'never'
+ ]
+ },
+ 'globals': {
+ 'window': true,
+ 'document': true,
+ 'App': true,
+ 'Page': true,
+ 'Component': true,
+ 'Behavior': true,
+ 'wx': true,
+ 'getCurrentPages': true,
+ }
+}
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/LICENSE b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/LICENSE
new file mode 100644
index 0000000..3617210
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2019 wechat-miniprogram
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/README.md b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/README.md
new file mode 100644
index 0000000..8983a29
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/README.md
@@ -0,0 +1,191 @@
+# 小程序的 MobX 绑定辅助库
+
+小程序的 MobX 绑定辅助库。
+
+> 此 behavior 依赖开发者工具的 npm 构建。具体详情可查阅 [官方 npm 文档](https://developers.weixin.qq.com/miniprogram/dev/devtools/npm.html) 。
+
+> 可配合 MobX 的小程序构建版 npm 模块 [`mobx-miniprogram`](https://github.com/wechat-miniprogram/mobx) 使用。
+
+## 使用方法
+
+需要小程序基础库版本 >= 2.2.3 的环境。
+
+也可以直接参考这个代码片段(在微信开发者工具中打开): [https://developers.weixin.qq.com/s/36j8NZmC74ac](https://developers.weixin.qq.com/s/36j8NZmC74ac) 。
+
+1. 安装 `mobx-miniprogram` 和 `mobx-miniprogram-bindings` :
+
+```
+npm install --save mobx-miniprogram mobx-miniprogram-bindings
+```
+
+2. 创建 MobX Store。
+
+```js
+// store.js
+import { observable, action } from 'mobx-miniprogram'
+
+export const store = observable({
+
+ // 数据字段
+ numA: 1,
+ numB: 2,
+
+ // 计算属性
+ get sum() {
+ return this.numA + this.numB
+ },
+
+ // actions
+ update: action(function () {
+ const sum = this.sum
+ this.numA = this.numB
+ this.numB = sum
+ })
+
+})
+```
+
+3. 在 Component 构造器中使用:
+
+```js
+import { storeBindingsBehavior } from 'mobx-miniprogram-bindings'
+import { store } from './store'
+
+Component({
+ behaviors: [storeBindingsBehavior],
+ data: {
+ someData: '...'
+ },
+ /**
+ * 也可配置数组,支持多store场景
+ * @exmaple storeBindings: [{store: storeA, fields:{sumA: 'sum'}}, {store: storeB, fields:{sumB: 'sum'}}]
+ */
+ storeBindings: {
+ store,
+ fields: {
+ numA: () => store.numA,
+ numB: (store) => store.numB,
+ sum: 'sum'
+ },
+ actions: {
+ buttonTap: 'update'
+ },
+ },
+ methods: {
+ myMethod() {
+ this.data.sum // 来自于 MobX store 的字段
+ }
+ }
+})
+```
+
+4. 在 Page 构造器中使用:
+
+如果小程序基础库版本在 2.9.2 以上,可以直接像上面 Component 构造器那样引入 behaviors 。
+
+如果需要比较好的兼容性,可以使用下面这种方式(或者直接改用 Component 构造器来创建页面)。
+
+```js
+import { createStoreBindings } from 'mobx-miniprogram-bindings'
+import { store } from './store'
+
+Page({
+ data: {
+ someData: '...'
+ },
+ onLoad() {
+ this.storeBindings = createStoreBindings(this, {
+ store,
+ fields: ['numA', 'numB', 'sum'],
+ actions: ['update'],
+ })
+ },
+ onUnload() {
+ this.storeBindings.destroyStoreBindings()
+ },
+ myMethod() {
+ this.data.sum // 来自于 MobX store 的字段
+ }
+})
+```
+
+## 接口
+
+将页面、自定义组件和 store 绑定有两种方式: **behavior 绑定** 和 **手工绑定** 。
+
+### behavior 绑定
+
+**behavior 绑定** 适用于 `Component` 构造器。做法:使用 `storeBindingsBehavior` 这个 behavior 和 `storeBindings` 定义段。
+
+注意:你可以用 `Component` 构造器构造页面, [参考文档](https://developers.weixin.qq.com/miniprogram/dev/framework/app-service/page.html#%E4%BD%BF%E7%94%A8-Component-%E6%9E%84%E9%80%A0%E5%99%A8%E6%9E%84%E9%80%A0%E9%A1%B5%E9%9D%A2) 。
+
+```js
+import { storeBindingsBehavior } from 'mobx-miniprogram-bindings'
+Component({
+ behaviors: [storeBindingsBehavior],
+ storeBindings: {
+ /* 绑定配置(见下文) */
+ }
+})
+```
+
+### 手工绑定
+
+**手工绑定** 适用于全部场景。做法:使用 `createStoreBindings` 创建绑定,它会返回一个包含清理函数的对象用于取消绑定。
+
+注意:在页面 onUnload (自定义组件 detached )时一定要调用清理函数,否则将导致内存泄漏!
+
+```js
+import { createStoreBindings } from 'mobx-miniprogram-bindings'
+
+Page({
+ onLoad() {
+ this.storeBindings = createStoreBindings(this, {
+ /* 绑定配置(见下文) */
+ })
+ },
+ onUnload() {
+ this.storeBindings.destroyStoreBindings()
+ }
+})
+```
+
+### 绑定配置
+
+无论使用哪种绑定方式,都必须提供一个绑定配置对象。这个对象包含的字段如下:
+
+| 字段名 | 类型 | 含义 |
+| ------ | ---- | ---- |
+| store | 一个 MobX observable | 默认的 MobX store |
+| fields | 数组或者对象 | 用于指定需要绑定的 data 字段 |
+| actions | 数组或者对象 | 用于指定需要映射的 actions |
+
+#### `fields`
+
+`fields` 有三种形式:
+
+* 数组形式:指定 data 中哪些字段来源于 `store` 。例如 `['numA', 'numB', 'sum']` 。
+* 映射形式:指定 data 中哪些字段来源于 `store` 以及它们在 `store` 中对应的名字。例如 `{ a: 'numA', b: 'numB' }` ,此时 `this.data.a === store.numA` `this.data.b === store.numB` 。
+* 函数形式:指定 data 中每个字段的计算方法。例如 `{ a: () => store.numA, b: () => anotherStore.numB }` ,此时 `this.data.a === store.numA` `this.data.b === anotherStore.numB` 。
+
+上述三种形式中,映射形式和函数形式可以在一个配置中同时使用。
+
+如果仅使用了函数形式,那么 `store` 字段可以为空,否则 `store` 字段必填。
+
+#### `actions`
+
+`actions` 可以用于将 store 中的一些 actions 放入页面或自定义组件的 this 下,来方便触发一些 actions 。有两种形式:
+
+* 数组形式:例如 `['update']` ,此时 `this.update === store.update` 。
+* 映射形式:例如 `{ buttonTap: 'update' }` ,此时 `this.buttonTap === store.update` 。
+
+只要 `actions` 不为空,则 `store` 字段必填。
+
+### 延迟更新与立刻更新
+
+为了提升性能,在 store 中的字段被更新后,并不会立刻同步更新到 `this.data` 上,而是等到下个 `wx.nextTick` 调用时才更新。(这样可以显著减少 setData 的调用次数。)
+
+如果需要立刻更新,可以调用:
+
+* `this.updateStoreBindings()` (在 **behavior 绑定** 中)
+* `this.storeBindings.updateStoreBindings()` (在 **手工绑定** 中)
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/gulpfile.js b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/gulpfile.js
new file mode 100644
index 0000000..e7a8722
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/gulpfile.js
@@ -0,0 +1,60 @@
+const path = require('path')
+const gulp = require('gulp')
+const clean = require('gulp-clean')
+const webpack = require('webpack')
+const nodeExternals = require('webpack-node-externals')
+
+const src = path.resolve(__dirname, 'src')
+const dist = path.resolve(__dirname, 'miniprogram_dist')
+
+function getWebpackConfig(production) {
+ return {
+ mode: production ? 'production' : 'development',
+ entry: src,
+ output: {
+ path: dist,
+ filename: 'index.js',
+ libraryTarget: 'commonjs2',
+ },
+ target: 'node',
+ externals: [nodeExternals()],
+ module: {
+ rules: [{
+ test: /\.js$/i,
+ use: [
+ 'babel-loader',
+ 'eslint-loader'
+ ],
+ exclude: /node_modules/
+ }],
+ },
+ resolve: {
+ modules: [src, 'node_modules'],
+ extensions: ['.js', '.json'],
+ },
+ plugins: [
+ new webpack.DefinePlugin({}),
+ new webpack.optimize.LimitChunkCountPlugin({maxChunks: 1}),
+ ],
+ optimization: {
+ minimize: false,
+ },
+ devtool: 'nosources-source-map',
+ performance: {
+ hints: 'warning',
+ assetFilter: assetFilename => assetFilename.endsWith('.js')
+ }
+ }
+}
+
+gulp.task('clean', () => gulp.src(dist, {read: false, allowEmpty: true}).pipe(clean()))
+
+gulp.task('dev', (cb) => {
+ webpack(getWebpackConfig(false), cb)
+})
+
+gulp.task('build', (cb) => {
+ webpack(getWebpackConfig(true), cb)
+})
+
+gulp.task('default', gulp.series('clean', 'build'))
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js
new file mode 100644
index 0000000..109e62d
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js
@@ -0,0 +1,292 @@
+module.exports =
+/******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+/******/
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+/******/
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId]) {
+/******/ return installedModules[moduleId].exports;
+/******/ }
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ i: moduleId,
+/******/ l: false,
+/******/ exports: {}
+/******/ };
+/******/
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+/******/
+/******/ // Flag the module as loaded
+/******/ module.l = true;
+/******/
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+/******/
+/******/
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+/******/
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+/******/
+/******/ // define getter function for harmony exports
+/******/ __webpack_require__.d = function(exports, name, getter) {
+/******/ if(!__webpack_require__.o(exports, name)) {
+/******/ Object.defineProperty(exports, name, { enumerable: true, get: getter });
+/******/ }
+/******/ };
+/******/
+/******/ // define __esModule on exports
+/******/ __webpack_require__.r = function(exports) {
+/******/ if(typeof Symbol !== 'undefined' && Symbol.toStringTag) {
+/******/ Object.defineProperty(exports, Symbol.toStringTag, { value: 'Module' });
+/******/ }
+/******/ Object.defineProperty(exports, '__esModule', { value: true });
+/******/ };
+/******/
+/******/ // create a fake namespace object
+/******/ // mode & 1: value is a module id, require it
+/******/ // mode & 2: merge all properties of value into the ns
+/******/ // mode & 4: return value when already ns object
+/******/ // mode & 8|1: behave like require
+/******/ __webpack_require__.t = function(value, mode) {
+/******/ if(mode & 1) value = __webpack_require__(value);
+/******/ if(mode & 8) return value;
+/******/ if((mode & 4) && typeof value === 'object' && value && value.__esModule) return value;
+/******/ var ns = Object.create(null);
+/******/ __webpack_require__.r(ns);
+/******/ Object.defineProperty(ns, 'default', { enumerable: true, value: value });
+/******/ if(mode & 2 && typeof value != 'string') for(var key in value) __webpack_require__.d(ns, key, function(key) { return value[key]; }.bind(null, key));
+/******/ return ns;
+/******/ };
+/******/
+/******/ // getDefaultExport function for compatibility with non-harmony modules
+/******/ __webpack_require__.n = function(module) {
+/******/ var getter = module && module.__esModule ?
+/******/ function getDefault() { return module['default']; } :
+/******/ function getModuleExports() { return module; };
+/******/ __webpack_require__.d(getter, 'a', getter);
+/******/ return getter;
+/******/ };
+/******/
+/******/ // Object.prototype.hasOwnProperty.call
+/******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };
+/******/
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+/******/
+/******/
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(__webpack_require__.s = 0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ (function(module, exports, __webpack_require__) {
+
+"use strict";
+
+
+exports.__esModule = true;
+exports.storeBindingsBehavior = undefined;
+
+var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
+
+exports.createStoreBindings = createStoreBindings;
+
+var _mobxMiniprogram = __webpack_require__(1);
+
+function _createActions(methods, options) {
+ var store = options.store,
+ actions = options.actions;
+
+
+ if (!actions) return;
+
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+
+ if (actions instanceof Array) {
+ // eslint-disable-next-line arrow-body-style
+ actions.forEach(function (field) {
+ methods[field] = function () {
+ return store[field].apply(store, arguments);
+ };
+ });
+ } else if ((typeof actions === 'undefined' ? 'undefined' : _typeof(actions)) === 'object') {
+ // for object-typed fields definition
+ Object.keys(actions).forEach(function (field) {
+ var def = actions[field];
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition');
+ }
+ methods[field] = function () {
+ return store[def].apply(store, arguments);
+ };
+ });
+ }
+}
+
+function _createDataFieldsReactions(target, options) {
+ var store = options.store,
+ fields = options.fields;
+
+ // setData combination
+
+ var pendingSetData = null;
+ function applySetData() {
+ if (pendingSetData === null) return;
+ var data = pendingSetData;
+ pendingSetData = null;
+ target.setData(data);
+ }
+ function scheduleSetData(field, value) {
+ if (!pendingSetData) {
+ pendingSetData = {};
+ wx.nextTick(applySetData);
+ }
+ pendingSetData[field] = value;
+ }
+
+ // handling fields
+ var reactions = [];
+ if (fields instanceof Array) {
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+ // eslint-disable-next-line arrow-body-style
+ reactions = fields.map(function (field) {
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return store[field];
+ }, function (value) {
+ scheduleSetData(field, value);
+ }, {
+ fireImmediately: true
+ });
+ });
+ } else if ((typeof fields === 'undefined' ? 'undefined' : _typeof(fields)) === 'object' && fields) {
+ // for object-typed fields definition
+ reactions = Object.keys(fields).map(function (field) {
+ var def = fields[field];
+ if (typeof def === 'function') {
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return def.call(target, store);
+ }, function (value) {
+ scheduleSetData(field, value);
+ }, {
+ fireImmediately: true
+ });
+ }
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition');
+ }
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified');
+ }
+ return (0, _mobxMiniprogram.reaction)(function () {
+ return store[def];
+ }, function (value) {
+ scheduleSetData(String(field), value);
+ }, {
+ fireImmediately: true
+ });
+ });
+ }
+
+ var destroyStoreBindings = function destroyStoreBindings() {
+ reactions.forEach(function (reaction) {
+ return reaction();
+ });
+ };
+
+ return {
+ updateStoreBindings: applySetData,
+ destroyStoreBindings: destroyStoreBindings
+ };
+}
+
+function createStoreBindings(target, options) {
+ _createActions(target, options);
+ return _createDataFieldsReactions(target, options);
+}
+
+var storeBindingsBehavior = exports.storeBindingsBehavior = Behavior({
+ definitionFilter: function definitionFilter(defFields) {
+ if (!defFields.methods) {
+ defFields.methods = {};
+ }
+ var storeBindings = defFields.storeBindings;
+
+ defFields.methods._mobxMiniprogramBindings = function () {
+ return storeBindings;
+ };
+ if (storeBindings) {
+ if (Array.isArray(storeBindings)) {
+ storeBindings.forEach(function (binding) {
+ _createActions(defFields.methods, binding);
+ });
+ } else {
+ _createActions(defFields.methods, storeBindings);
+ }
+ }
+ },
+ attached: function attached() {
+ if (typeof this._mobxMiniprogramBindings !== 'function') return;
+ var storeBindings = this._mobxMiniprogramBindings();
+ if (!storeBindings) {
+ this._mobxMiniprogramBindings = null;
+ return;
+ }
+ if (Array.isArray(storeBindings)) {
+ var that = this;
+ this._mobxMiniprogramBindings = storeBindings.map(function (item) {
+ return _createDataFieldsReactions(that, item);
+ });
+ } else {
+ this._mobxMiniprogramBindings = _createDataFieldsReactions(this, storeBindings);
+ }
+ },
+ detached: function detached() {
+ if (this._mobxMiniprogramBindings) {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.destroyStoreBindings();
+ });
+ } else {
+ this._mobxMiniprogramBindings.destroyStoreBindings();
+ }
+ }
+ },
+
+ methods: {
+ updateStoreBindings: function updateStoreBindings() {
+ if (this._mobxMiniprogramBindings && typeof this._mobxMiniprogramBindings !== 'function') {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.updateStoreBindings();
+ });
+ } else {
+ this._mobxMiniprogramBindings.updateStoreBindings();
+ }
+ }
+ }
+ }
+});
+
+/***/ }),
+/* 1 */
+/***/ (function(module, exports) {
+
+module.exports = require("mobx-miniprogram");
+
+/***/ })
+/******/ ]);
+//# sourceMappingURL=index.js.map
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js.map b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js.map
new file mode 100644
index 0000000..eeb46a3
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/miniprogram_dist/index.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["webpack:///webpack/bootstrap","webpack:///./src/index.js","webpack:///external \"mobx-miniprogram\""],"names":["createStoreBindings","_createActions","methods","options","store","actions","Error","Array","forEach","field","Object","keys","def","_createDataFieldsReactions","target","fields","pendingSetData","applySetData","data","setData","scheduleSetData","value","wx","nextTick","reactions","map","fireImmediately","call","String","destroyStoreBindings","reaction","updateStoreBindings","storeBindingsBehavior","Behavior","definitionFilter","defFields","storeBindings","_mobxMiniprogramBindings","isArray","binding","attached","that","item","detached","bd"],"mappings":";;QAAA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;;QAEA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;;;QAGA;QACA;;QAEA;QACA;;QAEA;QACA;QACA;QACA,0CAA0C,gCAAgC;QAC1E;QACA;;QAEA;QACA;QACA;QACA,wDAAwD,kBAAkB;QAC1E;QACA,iDAAiD,cAAc;QAC/D;;QAEA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA;QACA,yCAAyC,iCAAiC;QAC1E,gHAAgH,mBAAmB,EAAE;QACrI;QACA;;QAEA;QACA;QACA;QACA,2BAA2B,0BAA0B,EAAE;QACvD,iCAAiC,eAAe;QAChD;QACA;QACA;;QAEA;QACA,sDAAsD,+DAA+D;;QAErH;QACA;;;QAGA;QACA;;;;;;;;;;;;;;;QCoBgBA,mB,GAAAA,mB;;AAtGhB;;AAEA,SAASC,cAAT,CAAwBC,OAAxB,EAAiCC,OAAjC,EAA0C;AAAA,MACjCC,KADiC,GACfD,OADe,CACjCC,KADiC;AAAA,MAC1BC,OAD0B,GACfF,OADe,CAC1BE,OAD0B;;;AAGxC,MAAI,CAACA,OAAL,EAAc;;AAEd;AACA,MAAI,OAAOD,KAAP,KAAiB,WAArB,EAAkC;AAChC,UAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;;AAED,MAAID,mBAAmBE,KAAvB,EAA8B;AAC5B;AACAF,YAAQG,OAAR,CAAgB,UAACC,KAAD,EAAW;AACzBP,cAAQO,KAAR,IAAiB,YAAmB;AAClC,eAAOL,MAAMK,KAAN,yBAAP;AACD,OAFD;AAGD,KAJD;AAKD,GAPD,MAOO,IAAI,QAAOJ,OAAP,yCAAOA,OAAP,OAAmB,QAAvB,EAAiC;AACtC;AACAK,WAAOC,IAAP,CAAYN,OAAZ,EAAqBG,OAArB,CAA6B,UAACC,KAAD,EAAW;AACtC,UAAMG,MAAMP,QAAQI,KAAR,CAAZ;AACA,UAAI,OAAOA,KAAP,KAAiB,QAAjB,IAA6B,OAAOA,KAAP,KAAiB,QAAlD,EAA4D;AAC1D,cAAM,IAAIH,KAAJ,CAAU,kDAAV,CAAN;AACD;AACDJ,cAAQO,KAAR,IAAiB,YAAmB;AAClC,eAAOL,MAAMQ,GAAN,yBAAP;AACD,OAFD;AAGD,KARD;AASD;AACF;;AAED,SAASC,0BAAT,CAAoCC,MAApC,EAA4CX,OAA5C,EAAqD;AAAA,MAC5CC,KAD4C,GAC3BD,OAD2B,CAC5CC,KAD4C;AAAA,MACrCW,MADqC,GAC3BZ,OAD2B,CACrCY,MADqC;;AAGnD;;AACA,MAAIC,iBAAiB,IAArB;AACA,WAASC,YAAT,GAAwB;AACtB,QAAID,mBAAmB,IAAvB,EAA6B;AAC7B,QAAME,OAAOF,cAAb;AACAA,qBAAiB,IAAjB;AACAF,WAAOK,OAAP,CAAeD,IAAf;AACD;AACD,WAASE,eAAT,CAAyBX,KAAzB,EAAgCY,KAAhC,EAAuC;AACrC,QAAI,CAACL,cAAL,EAAqB;AACnBA,uBAAiB,EAAjB;AACAM,SAAGC,QAAH,CAAYN,YAAZ;AACD;AACDD,mBAAeP,KAAf,IAAwBY,KAAxB;AACD;;AAED;AACA,MAAIG,YAAY,EAAhB;AACA,MAAIT,kBAAkBR,KAAtB,EAA6B;AAC3B;AACA,QAAI,OAAOH,KAAP,KAAiB,WAArB,EAAkC;AAChC,YAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;AACD;AACAkB,gBAAYT,OAAOU,GAAP,CAAW,UAAChB,KAAD,EAAW;AAChC,aAAO,+BAAS;AAAA,eAAML,MAAMK,KAAN,CAAN;AAAA,OAAT,EAA6B,UAACY,KAAD,EAAW;AAC7CD,wBAAgBX,KAAhB,EAAuBY,KAAvB;AACD,OAFM,EAEJ;AACDK,yBAAiB;AADhB,OAFI,CAAP;AAKD,KANW,CAAZ;AAOD,GAbD,MAaO,IAAI,QAAOX,MAAP,yCAAOA,MAAP,OAAkB,QAAlB,IAA8BA,MAAlC,EAA0C;AAC/C;AACAS,gBAAYd,OAAOC,IAAP,CAAYI,MAAZ,EAAoBU,GAApB,CAAwB,UAAChB,KAAD,EAAW;AAC7C,UAAMG,MAAMG,OAAON,KAAP,CAAZ;AACA,UAAI,OAAOG,GAAP,KAAe,UAAnB,EAA+B;AAC7B,eAAO,+BAAS;AAAA,iBAAMA,IAAIe,IAAJ,CAASb,MAAT,EAAiBV,KAAjB,CAAN;AAAA,SAAT,EAAwC,UAACiB,KAAD,EAAW;AACxDD,0BAAgBX,KAAhB,EAAuBY,KAAvB;AACD,SAFM,EAEJ;AACDK,2BAAiB;AADhB,SAFI,CAAP;AAKD;AACD,UAAI,OAAOjB,KAAP,KAAiB,QAAjB,IAA6B,OAAOA,KAAP,KAAiB,QAAlD,EAA4D;AAC1D,cAAM,IAAIH,KAAJ,CAAU,kDAAV,CAAN;AACD;AACD,UAAI,OAAOF,KAAP,KAAiB,WAArB,EAAkC;AAChC,cAAM,IAAIE,KAAJ,CAAU,uCAAV,CAAN;AACD;AACD,aAAO,+BAAS;AAAA,eAAMF,MAAMQ,GAAN,CAAN;AAAA,OAAT,EAA2B,UAACS,KAAD,EAAW;AAC3CD,wBAAgBQ,OAAOnB,KAAP,CAAhB,EAA+BY,KAA/B;AACD,OAFM,EAEJ;AACDK,yBAAiB;AADhB,OAFI,CAAP;AAKD,KApBW,CAAZ;AAqBD;;AAED,MAAMG,uBAAuB,SAAvBA,oBAAuB,GAAM;AACjCL,cAAUhB,OAAV,CAAkB,UAACsB,QAAD;AAAA,aAAcA,UAAd;AAAA,KAAlB;AACD,GAFD;;AAIA,SAAO;AACLC,yBAAqBd,YADhB;AAELY;AAFK,GAAP;AAID;;AAEM,SAAS7B,mBAAT,CAA6Bc,MAA7B,EAAqCX,OAArC,EAA8C;AACnDF,iBAAea,MAAf,EAAuBX,OAAvB;AACA,SAAOU,2BAA2BC,MAA3B,EAAmCX,OAAnC,CAAP;AACD;;AAEM,IAAM6B,wDAAwBC,SAAS;AAC5CC,oBAAkB,0BAACC,SAAD,EAAe;AAC/B,QAAI,CAACA,UAAUjC,OAAf,EAAwB;AACtBiC,gBAAUjC,OAAV,GAAoB,EAApB;AACD;AAH8B,QAIxBkC,aAJwB,GAIPD,SAJO,CAIxBC,aAJwB;;AAK/BD,cAAUjC,OAAV,CAAkBmC,wBAAlB,GAA6C,YAAY;AACvD,aAAOD,aAAP;AACD,KAFD;AAGA,QAAIA,aAAJ,EAAmB;AACjB,UAAI7B,MAAM+B,OAAN,CAAcF,aAAd,CAAJ,EAAkC;AAChCA,sBAAc5B,OAAd,CAAsB,UAAU+B,OAAV,EAAmB;AACvCtC,yBAAekC,UAAUjC,OAAzB,EAAkCqC,OAAlC;AACD,SAFD;AAGD,OAJD,MAIO;AACLtC,uBAAekC,UAAUjC,OAAzB,EAAkCkC,aAAlC;AACD;AACF;AACF,GAlB2C;AAmB5CI,UAnB4C,sBAmBjC;AACT,QAAI,OAAO,KAAKH,wBAAZ,KAAyC,UAA7C,EAAyD;AACzD,QAAMD,gBAAgB,KAAKC,wBAAL,EAAtB;AACA,QAAI,CAACD,aAAL,EAAoB;AAClB,WAAKC,wBAAL,GAAgC,IAAhC;AACA;AACD;AACD,QAAI9B,MAAM+B,OAAN,CAAcF,aAAd,CAAJ,EAAkC;AAChC,UAAMK,OAAO,IAAb;AACA,WAAKJ,wBAAL,GAAgCD,cAAcX,GAAd,CAAkB,UAAUiB,IAAV,EAAgB;AAChE,eAAO7B,2BAA2B4B,IAA3B,EAAiCC,IAAjC,CAAP;AACD,OAF+B,CAAhC;AAGD,KALD,MAKO;AACL,WAAKL,wBAAL,GAAgCxB,2BAA2B,IAA3B,EAAiCuB,aAAjC,CAAhC;AACD;AACF,GAlC2C;AAmC5CO,UAnC4C,sBAmCjC;AACT,QAAI,KAAKN,wBAAT,EAAmC;AACjC,UAAI9B,MAAM+B,OAAN,CAAc,KAAKD,wBAAnB,CAAJ,EAAkD;AAChD,aAAKA,wBAAL,CAA8B7B,OAA9B,CAAsC,UAAUoC,EAAV,EAAc;AAClDA,aAAGf,oBAAH;AACD,SAFD;AAGD,OAJD,MAIO;AACL,aAAKQ,wBAAL,CAA8BR,oBAA9B;AACD;AACF;AACF,GA7C2C;;AA8C5C3B,WAAS;AACP6B,uBADO,iCACe;AACpB,UAAI,KAAKM,wBAAL,IAAiC,OAAO,KAAKA,wBAAZ,KAAyC,UAA9E,EAA0F;AACxF,YAAI9B,MAAM+B,OAAN,CAAc,KAAKD,wBAAnB,CAAJ,EAAkD;AAChD,eAAKA,wBAAL,CAA8B7B,OAA9B,CAAsC,UAAUoC,EAAV,EAAc;AAClDA,eAAGb,mBAAH;AACD,WAFD;AAGD,SAJD,MAIO;AACL,eAAKM,wBAAL,CAA8BN,mBAA9B;AACD;AACF;AACF;AAXM;AA9CmC,CAAT,CAA9B,C;;;;;;AC3GP,6C","file":"index.js","sourceRoot":""}
\ No newline at end of file
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/package.json b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/package.json
new file mode 100644
index 0000000..63975d2
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/package.json
@@ -0,0 +1,74 @@
+{
+ "name": "mobx-miniprogram-bindings",
+ "version": "1.2.1",
+ "description": "Mobx binding utils for WeChat miniprogram",
+ "main": "miniprogram_dist/index.js",
+ "scripts": {
+ "dev": "gulp dev",
+ "build": "gulp",
+ "dist": "npm run build",
+ "clean-dev": "gulp clean --develop",
+ "clean": "gulp clean",
+ "test": "jest ./test/* --bail",
+ "coverage": "jest ./test/* --coverage --bail",
+ "lint": "eslint \"src/**/*.js\"",
+ "lint-tools": "eslint \"tools/**/*.js\""
+ },
+ "miniprogram": "miniprogram_dist",
+ "jest": {
+ "testEnvironment": "jsdom",
+ "testURL": "https://jest.test",
+ "collectCoverageFrom": [
+ "src/**/*.js"
+ ],
+ "moduleDirectories": [
+ "node_modules",
+ "src"
+ ]
+ },
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/wechat-miniprogram/mobx-miniprogram-bindings.git"
+ },
+ "keywords": [
+ "mobx",
+ "wechat",
+ "miniprogram"
+ ],
+ "author": "wechat-miniprogram",
+ "license": "MIT",
+ "bugs": {
+ "url": "https://github.com/wechat-miniprogram/mobx-miniprogram-bindings/issues"
+ },
+ "homepage": "https://github.com/wechat-miniprogram/mobx-miniprogram-bindings#readme",
+ "devDependencies": {
+ "babel-core": "^6.26.3",
+ "babel-loader": "^7.1.5",
+ "babel-plugin-module-resolver": "^3.2.0",
+ "babel-preset-env": "^1.7.0",
+ "colors": "^1.3.1",
+ "eslint": "^5.14.1",
+ "eslint-config-airbnb-base": "13.1.0",
+ "eslint-loader": "^2.1.2",
+ "eslint-plugin-import": "^2.16.0",
+ "eslint-plugin-node": "^7.0.1",
+ "eslint-plugin-promise": "^3.8.0",
+ "gulp": "^4.0.0",
+ "gulp-clean": "^0.4.0",
+ "gulp-if": "^2.0.2",
+ "gulp-install": "^1.1.0",
+ "gulp-less": "^4.0.1",
+ "gulp-rename": "^1.4.0",
+ "gulp-sourcemaps": "^2.6.5",
+ "jest": "^23.5.0",
+ "miniprogram-simulate": "^1.0.7",
+ "mobx-miniprogram": "^4.0.0",
+ "through2": "^2.0.3",
+ "vinyl": "^2.2.0",
+ "webpack": "^4.29.5",
+ "webpack-node-externals": "^1.7.2"
+ },
+ "peerDependencies": {
+ "mobx-miniprogram": "^4.0.0"
+ }
+}
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/src/index.js b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/src/index.js
new file mode 100644
index 0000000..2da55eb
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram-bindings/src/index.js
@@ -0,0 +1,167 @@
+import {reaction} from 'mobx-miniprogram'
+
+function _createActions(methods, options) {
+ const {store, actions} = options
+
+ if (!actions) return
+
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified')
+ }
+
+ if (actions instanceof Array) {
+ // eslint-disable-next-line arrow-body-style
+ actions.forEach((field) => {
+ methods[field] = function (...args) {
+ return store[field](...args)
+ }
+ })
+ } else if (typeof actions === 'object') {
+ // for object-typed fields definition
+ Object.keys(actions).forEach((field) => {
+ const def = actions[field]
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition')
+ }
+ methods[field] = function (...args) {
+ return store[def](...args)
+ }
+ })
+ }
+}
+
+function _createDataFieldsReactions(target, options) {
+ const {store, fields} = options
+
+ // setData combination
+ let pendingSetData = null
+ function applySetData() {
+ if (pendingSetData === null) return
+ const data = pendingSetData
+ pendingSetData = null
+ target.setData(data)
+ }
+ function scheduleSetData(field, value) {
+ if (!pendingSetData) {
+ pendingSetData = {}
+ wx.nextTick(applySetData)
+ }
+ pendingSetData[field] = value
+ }
+
+ // handling fields
+ let reactions = []
+ if (fields instanceof Array) {
+ // for array-typed fields definition
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified')
+ }
+ // eslint-disable-next-line arrow-body-style
+ reactions = fields.map((field) => {
+ return reaction(() => store[field], (value) => {
+ scheduleSetData(field, value)
+ }, {
+ fireImmediately: true
+ })
+ })
+ } else if (typeof fields === 'object' && fields) {
+ // for object-typed fields definition
+ reactions = Object.keys(fields).map((field) => {
+ const def = fields[field]
+ if (typeof def === 'function') {
+ return reaction(() => def.call(target, store), (value) => {
+ scheduleSetData(field, value)
+ }, {
+ fireImmediately: true
+ })
+ }
+ if (typeof field !== 'string' && typeof field !== 'number') {
+ throw new Error('[mobx-miniprogram] unrecognized field definition')
+ }
+ if (typeof store === 'undefined') {
+ throw new Error('[mobx-miniprogram] no store specified')
+ }
+ return reaction(() => store[def], (value) => {
+ scheduleSetData(String(field), value)
+ }, {
+ fireImmediately: true
+ })
+ })
+ }
+
+ const destroyStoreBindings = () => {
+ reactions.forEach((reaction) => reaction())
+ }
+
+ return {
+ updateStoreBindings: applySetData,
+ destroyStoreBindings
+ }
+}
+
+export function createStoreBindings(target, options) {
+ _createActions(target, options)
+ return _createDataFieldsReactions(target, options)
+}
+
+export const storeBindingsBehavior = Behavior({
+ definitionFilter: (defFields) => {
+ if (!defFields.methods) {
+ defFields.methods = {}
+ }
+ const {storeBindings} = defFields
+ defFields.methods._mobxMiniprogramBindings = function () {
+ return storeBindings
+ }
+ if (storeBindings) {
+ if (Array.isArray(storeBindings)) {
+ storeBindings.forEach(function (binding) {
+ _createActions(defFields.methods, binding)
+ })
+ } else {
+ _createActions(defFields.methods, storeBindings)
+ }
+ }
+ },
+ attached() {
+ if (typeof this._mobxMiniprogramBindings !== 'function') return
+ const storeBindings = this._mobxMiniprogramBindings()
+ if (!storeBindings) {
+ this._mobxMiniprogramBindings = null
+ return
+ }
+ if (Array.isArray(storeBindings)) {
+ const that = this
+ this._mobxMiniprogramBindings = storeBindings.map(function (item) {
+ return _createDataFieldsReactions(that, item)
+ })
+ } else {
+ this._mobxMiniprogramBindings = _createDataFieldsReactions(this, storeBindings)
+ }
+ },
+ detached() {
+ if (this._mobxMiniprogramBindings) {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.destroyStoreBindings()
+ })
+ } else {
+ this._mobxMiniprogramBindings.destroyStoreBindings()
+ }
+ }
+ },
+ methods: {
+ updateStoreBindings() {
+ if (this._mobxMiniprogramBindings && typeof this._mobxMiniprogramBindings !== 'function') {
+ if (Array.isArray(this._mobxMiniprogramBindings)) {
+ this._mobxMiniprogramBindings.forEach(function (bd) {
+ bd.updateStoreBindings()
+ })
+ } else {
+ this._mobxMiniprogramBindings.updateStoreBindings()
+ }
+ }
+ }
+ }
+})
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/CHANGELOG.md b/src/miniprogram-4/node_modules/mobx-miniprogram/CHANGELOG.md
new file mode 100644
index 0000000..b331ad9
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/CHANGELOG.md
@@ -0,0 +1,1277 @@
+Changelog continues [here](https://github.com/mobxjs/mobx/blob/master/CHANGELOG.md)
+
+---
+
+# 4.3.1
+
+* Fixed [#1534](Fixes https://github.com/mobxjs/mobx/issues/1534): @computed({keepAlive: true}) no long calculates before being accessed.
+* Added the `$mobx` export symbol for MobX 5 forward compatibity
+
+# 4.3.0
+
+* Introduced the `entries(observable)` API, by @samjacobclift through [#1536](https://github.com/mobxjs/mobx/pull/1536)
+* Fixed [#1535](https://github.com/mobxjs/mobx/issues/1535): Change in nested computed value was not propagated if read outside action context when there is a pending reaction. For more details see the exact test case.
+* Illegal property access through prototypes is now a warning instead of an error. Fixes [#1506](https://github.com/mobxjs/mobx/issues/1506). By @AmazingTurtle through [#1529](https://github.com/mobxjs/mobx/pull/1529)
+* Fixed issue where providing a custom setter to `@computed({ set: ... })` wasn't picked up
+* Fixed #1545: Actions properties where not re-assignable when using TypeScript
+* Illegal Access checks are now a warning instead of an error. Fix
+
+# 4.2.1
+
+* Fixed flow typings for `mobx.configure` [#1521](https://github.com/mobxjs/mobx/pull/1521) by @andrew--r
+* Improved typings for `mobx.flow`, fixes [#1527](https://github.com/mobxjs/mobx/issues/1527)
+* Throw error when using `@observable` in combination with a getter. [#1511](https://github.com/mobxjs/mobx/pull/1511) by @quanganhtran
+* `toJS` now uses Map internally, for faster detection of cycles. [#1517](https://github.com/mobxjs/mobx/pull/1517) by @loatheb
+* Fixed [#1512](https://github.com/mobxjs/mobx/issues/1512): `observe` hooks not being triggered when using `mobx.set`, Fixed in [#1514](https://github.com/mobxjs/mobx/pull/1514) by @quanganhtran
+* Several minor improvements, additional tests and doc improvements.
+
+# 4.2.0
+
+* Introduced `configure({ enforceActions: "strict" })`, which is more strict then `enforceActions: true`, as it will also throw on non-observed changes to observables. See also [#1473](https://github.com/mobxjs/mobx/issues/1473)
+* Fixed [#1480](https://github.com/mobxjs/mobx/issues/1480): Exceptions in the effect handler of `reaction` where not properly picked up by the global reaction system
+* Fixed a bug where computed values updated their cached value, even when the comparer considered the new value equal to the previous one. Thanks @kuitos for finding this and fixing it! [#1499](https://github.com/mobxjs/mobx/pull/1499)
+* Undeprecated `ObservableMap`, fixes [#1496](https://github.com/mobxjs/mobx/issues/1496)
+* Observable arrays now support `Symbol.toStringTag` (if available / polyfilled). This allows libraries like Ramda to detect automatically that observable arrays are arrays. Fixes [#1490](https://github.com/mobxjs/mobx/issues/1490). Note that `Array.isArray` will keep returning false for the entire MobX 4 range.
+* Actions are now always `configurable` and `writable`, like in MobX 3. Fixes [#1477](https://github.com/mobxjs/mobx/issues/1477)
+* Merged several improvements to the flow typings. [#1501](https://github.com/mobxjs/mobx/pull/1501) by @quanganhtran
+* Fixed several accidental usages of the global `fail`, by @mtaran-google through [#1483](https://github.com/mobxjs/mobx/pull/1483) and [#1482](https://github.com/mobxjs/mobx/pull/1482)
+
+# 4.1.1
+
+* Import `default` from MobX will no longer throw, but only warn instead. This fixes some issues with tools that reflect on the `default` export of a module.
+* Disposing a spy listener inside a spy handler no longer causes an exception. Fixes [#1459](https://github.com/mobxjs/mobx/issues/1459) through [#1460](https://github.com/mobxjs/mobx/pull/1460) by [farwayer](https://github.com/farwayer)
+* Added a missing `runInAction` overload in the flow typings. [#1451](https://github.com/mobxjs/mobx/pull/1451) by [AMilassin](https://github.com/mobxjs/mobx/issues?q=is%3Apr+author%3AAMilassin)
+* Improved the typings of `decorate`. See [#1450](https://github.com/mobxjs/mobx/pull/1450) by [makepost](https://github.com/mobxjs/mobx/issues?q=is%3Apr+author%3Amakepost)
+
+# 4.1.0
+
+* Introduced `keepAlive` as option to `computed`
+* All observable api's now default to `any` for their generic arguments
+* Improved `flow` cancellation
+* The effect of `when` is now automatically an action.
+* `@computed` properties are now declared on their owner rather then the protoptype. Fixes an issue where `@computed` fields didn't work in React Native on proxied objects. See [#1396](https://github.com/mobxjs/mobx/issues/1396)
+* `action` and `action.bound` decorated fields are now reassignable, so that they can be stubbed
+
+# 4.0.2
+
+* Fixed issue where exceptions like `TypeError: Cannot define property:__mobxDidRunLazyInitializers, object is not extensible.` were thrown. Fixes [#1404](https://github.com/mobxjs/mobx/issues/1404)
+* Improved flow typings for `flow`, [#1399](https://github.com/mobxjs/mobx/pull/1399) by @ismailhabib
+
+# 4.0.1
+
+* Updated flow typings, see [#1393](https://github.com/mobxjs/mobx/pull/1393) by [andrew--r](https://github.com/andrew--r)
+
+# 4.0.0
+
+* For the highlights of this release, read the [blog](https://medium.com/p/c1fbc08008da/):
+* For migration notes: see the [wiki page](https://github.com/mobxjs/mobx/wiki/Migrating-from-mobx-3-to-mobx-4)
+* Note; many things that were removed to make the api surface smaller. If you think some feature shouldn't have been removed, feel free to open an issue!
+
+This is the extensive list of all changes.
+
+### New features
+
+The changes mentioned here are discussed in detail in the [release highlights](https://medium.com/p/c1fbc08008da/), or were simply updated in the docs.
+
+* MobX 4 introduces separation between the production and non production build. The production build strips most typechecks, resulting in a faster and smaller build. Make sure to substitute process.env.NODE_ENV = "production" in your build process! If you are using MobX in a react project, you most probably already have set this up. Otherwise, the idea is explained [here](https://reactjs.org/docs/add-react-to-an-existing-app.html).
+* Introduced `flow` to create a chain of async actions. This is the same function as [`asyncActions`](https://github.com/mobxjs/mobx-utils#asyncaction) of the mobx-utils package
+* These `flow`'s are now cancellable, by calling `.cancel()` on the returned promise, which will throw a cancellation exception into the generator function.
+* `flow` also has experimental support for async iterators (`async * function`)
+* Introduced `decorate(thing, decorators)` to decorate classes or object without needing decorator syntax.
+* Introduced `onBecomeObserved` and `onBecomeUnobserved`. These API's enable hooking into the observability system and get notified about when an observable starts / stops becoming used. This is great to automaticaly fetch data from external resources, or stop doing so.
+* `computed` / `@computed` now accepts a `requiresReaction` option. If it set, the computed value will throw an exception if it is being read while not being tracked by some reaction.
+* To make `requiresReaction` the default, use `mobx.configure({ computedRequiresReaction: true })`
+* Introduced `mobx.configure({ disableErrorBoundaries })`, for easier debugging of exceptoins. By [NaridaL](https://github.com/NaridaL) through [#1262](https://github.com/mobxjs/mobx/pull/1262)
+* `toJS` now accepts the options: `{ detectCycles?: boolean, exportMapsAsObjects?: boolean }`, both `true` by default
+* Observable maps are now backed by real ES6 Maps. This means that any value can be used as key now, not just strings and numbers.
+* The flow typings have been updated. Since this is a manual effort, there can be mistakes, so feel free to PR!
+
+* `computed(fn, options?)` / `@computed(options) get fn()` now accept the following options:
+ * `set: (value) => void` to set a custom setter on the computed property
+ * `name: "debug name"`
+ * `equals: fn` the equality value to use for the computed to determine whether its output has changed. The default is `comparer.default`. Alternatives are `comparer.structural`, `comparer.identity` or just your own comparison function.
+ * `requiresReaction: boolean` see above.
+
+* `autorun(fn, options?)` now accepts the following options:
+ * `delay: number` debounce the autorun with the given amount of milliseconds. This replaces the MobX 3 api `autorunAsync`
+ * `name: "debug name"`
+ * `scheduler: function` a custom scheduler to run the autorun. For example to connect running the autorun to `requestAnimationFrame`. See the docs for more details
+ * `onError`. A custom error handler to be notified when an autorun throws an exception.
+
+* `reaction(expr, effect, options?)` now accepts the following options:
+ * `delay: number` debounce the autorun with the given amount of milliseconds. This replaces the MobX 3 api `autorunAsync`
+ * `fireImmediately`. Immediately fire the effect function after the first evaluation of `expr`
+ * `equals`. Custom equality function to determine whether the `expr` function differed from its previous result, and hence should fire `effect`. Accepts the same options as the `equals` option of computed.
+ * All the options `autorun` accepts
+
+* `when(predicate, effect?, options?)` now accepts the following options:
+ * `name: "debug name"`
+ * `onError`. A custom error handler to be notified when an autorun throws an exception.
+ * `timeout: number` a timeout in milliseconds, after which the `onError` handler will be triggered to signal the condition not being met within a certain time
+* The `effect` parameter of `when` has become optional. If it is omitted, `when` will return a promise. This makes it easy to `await` a condition, for example: `await when(() => user.profile.loaded)`. The returned promise can be cancelled using `promise.cancel()`
+
+* There is now an utility API that enables manipulating observable maps, objects and arrays with the same api. These api's are fully reactive, which means that even new property declarations can be detected by mobx if `set` is used to add them, and `values` or `keys` to iterate them.
+ * `values(thing)` returns all values in the collection as array
+ * `keys(thing)` returns all keys in the collection as array
+ * `set(thing, key, value)` or `set(thing, { key: value })` Updates the given collection with the provided key / value pair(s).
+ * `remove(thing, key)` removes the specified child from the collection. For arrays splicing is used.
+ * `has(thing, key)` returns true if the collection has the specified _observable_ property.
+ * `get(thing, key)` returns the chlid under the specified key.
+
+* `observable`, `observable.array`, `observable.object`, `observable.map` and `extendObservable` now accept an additional options object, which can specify the following attributes:
+ * `name: "debug name"`
+ * `deep: boolean`. `true` by default, indicates whether the children of this collection are automatically converted into observables as well.
+ * `defaultDecorator: ` specifies the default decorator used for new children / properties, by default: `observable.deep`, but could be changed to `observable.ref`, `observable.struct` etc. (The `deep` property is just a short-hand for switching between `observable.deep` or `observable.ref` as default decorator for new properties)
+
+
+### Breaking changes
+
+The changes mentioned here are discussed in detail in the [migration notes](https://github.com/mobxjs/mobx/wiki/Migrating-from-mobx-3-to-mobx-4)
+
+* MobX 4 requires `Map` to be globally available. Polyfill it if targeting IE < 11 or other older browsers.
+* For typescript users, MobX now requires `Map` and several `Symbol`s to exist for its typings. So make sure that the `lib` configuration of your project is set to `"es6"`. (The compilation target can still be `"es5"`)
+* `observable.shallowArray(values)` has been removed, instead use `observable.array(values, { deep: false })`
+* `observable.shallowMap(values)` has been removed, instead use `observable.map(values, { deep: false })`
+* `observable.shallowObject(values)` has been removed, instead use `observable.object(values, {}, { deep: false })`
+* `extendShallowObservable(target, props)`, instead use `extendObservable(target, props, {}, { deep: false })`
+* The decorators `observable.ref`, `observable.shallow`, `observable.deep`, `observable.struct` can no longer be used as functions. Instead, they should be passed as part of the `decorators` param to resp. `observable.object` and `extendObservable`
+* The new signature of `extendObservable` is `extendObservable(target, props, decorators?, options?)`. This also means it is no longer possible to pass multiple bags of properties to `extendObservable`. `extendObservable` can no longer be used to re-declare properties. Use `set` instead to update existing properties (or introduce new ones).
+* Iterating maps now follows the spec, that is, `map.values()`, `map.entries()`, `map.keys()`, `map[@@iterator]()` and `array[@@iterator]()` no longer return an array, but an iterator. Use `mobx.values(map)` or `Array.from(map)` to convert the iterators to arrays.
+* dropped `@computed.equals`, instead, you can now use `@computed({ equals: ... })`
+* `useStrict(boolean)` was dropped, use `configure({ enforceActions: boolean })` instead
+* `isolateGlobalState` was dropped, use `configure({ isolateGlobalState: true})` instead
+* If there are multiple mobx instances active in a single project, an exception will be thrown. Previously only a warning was printed. Fixes #1098. For details, see [#1082](https://github.com/mobxjs/mobx/issues/1082).
+* Dropped the `shareGlobalState` feature. Instead, projects should be setup properly and it is up to the hosting package to make sure that there is only one MobX instance
+* `expr` has been moved to mobx-utils. Remember, `expr(fn)` is just `computed(fn).get()`
+* `createTransformer` has been moved to mobx-utils
+* Passing `context` explicitly to `autorun`, `reaction` etc is no longer supported. Use arrow functions or function.bind instead.
+* Removed `autorunAsync`. Use the `delay` option of `autorun` instead.
+* `autorun`, `when`, `reaction` don't support name as first argument anymore, instead pass the `name` option.
+* The `extras.` namespace has been dropped to enable tree-shaking non-used MobX features. All methods that where there originally are now exported at top level. If they are part of the official public API (you are encouraged to use them) they are exported as is. If they are experimental or somehow internal (you are discouraged to use them), they are prefixed with `_`.
+* Dropped bower support. Fixes #1263
+* The `spyReportStart`, `spyReportEnd`, `spyReport` and `isSpyEnabled` are no longer public. It is no longer possible to emit custom spy events as to avoid confusing in listeners what the possible set of events is.
+* Dropped `isStrictModeEnabled`
+* `observable(value)` will only succeed if it can turn the value into an observable data structure (a Map, Array or observable object). But it will no longer create an observable box for other values to avoid confusion. Call `observable.box(value)` explictly in such cases.
+* `isComputed` and `isObservable` no longer accept a property as second argument. Instead use `isComputedProp` and `isObservableProp`.
+* Removed `whyRun`, use `trace` instead
+* The spy event signature has slightly changed
+* The `Atom` class is no longer exposed. Use `createAtom` instead (same signature).
+* Calling reportObserved() on a self made atom will no longer trigger the hooks if reportObserved is triggered outside a reactive context.
+* The options `struct` and `compareStructural` for computed values are deprecated, use `@computed.struct` or `computed({ equals: comparer.structural})` instead.
+* `isModifierDescriptor` is no longer exposed.
+* `deepEqual` is no longer exposed, use `comparer.structural` instead.
+* `setReactionScheduler` -> `configure({ reactionScheduler: fn })`
+* `reserveArrayBuffer` -> `configure({ reactionErrorHandler: fn })`
+* `ObservableMap` is no longer exposed as constructor, use `observable.map` or `isObservableMap` instead
+* `map` -> `observable.map`
+* `runInAction` no longer accepts a custom scope
+* Dropped the already deprecated and broken `default` export that made it harder to tree-shake mobx. Make sure to always use `import { x } from "mobx"` and not `import mobx from "mobx"`.
+* Killed the already deprecated modifiers `asFlat` etc. If you war still using this, see the MobX 2 -> 3 migration notes.
+* Observable maps now fully implement the map interface. See [#1361](https://github.com/mobxjs/mobx/pull/1361) by [Marc Fallows](https://github.com/marcfallows)
+* Observable arrays will no longer expose the `.move` method
+* Dropped the `observable.deep.struct` modifier
+* Dropped the `observable.ref.struct` modifier
+* `observable.struct` now behaves like `observable.ref.struct` (this used to be `observable.deep.struct`). That is; values in an `observable.struct` field will be stored as is, but structural comparison will be used when assigning a new value
+* IReactionDisposer.onError has been removed, use the `onError` option of reactions instead
+
+### Issues fixed in this release:
+
+The issues are incoprorated in the above notes.
+
+* [#1316](https://github.com/mobxjs/mobx/issues/1316) - Improve `observable` api
+* [#992](https://github.com/mobxjs/mobx/issues/992) - `onBecomeObserved` & `onBecomeUnobserved`
+* [#1301](https://github.com/mobxjs/mobx/issues/1301) - Set `onError` handler when creating reactions
+* [#817](https://github.com/mobxjs/mobx/issues/817) - Improve typings of `observe`
+* [#800](https://github.com/mobxjs/mobx/issues/800) - Use `Map` as backend implementation of observable maps
+* [#1361](https://github.com/mobxjs/mobx/issues/1361) - Make observableMaps structurally correct maps
+* [#813](https://github.com/mobxjs/mobx/issues/813) - Create separate dev and production builds
+* [#961](https://github.com/mobxjs/mobx/issues/961), [#1197](https://github.com/mobxjs/mobx/issues/1197) - Make it possible to forbid reading an untracked computed value
+* [#1098](https://github.com/mobxjs/mobx/issues/1098) - Throw instead of warn if multiple MobX instances are active
+* [#1122](https://github.com/mobxjs/mobx/issues/1122) - Atom hooks fired to often for observable maps
+* [#1148](https://github.com/mobxjs/mobx/issues/1148) - Disposer of reactions should also cancel all scheduled effects
+* [#1241](https://github.com/mobxjs/mobx/issues/1241) - Make it possible to disable error boundaries, to make it easier to find exceptions
+* [#1263](https://github.com/mobxjs/mobx/issues/1263) - Remove bower.json
+
+# 3.6.2
+
+* Fixed accidental dependency on the `node` typings. Fixes [#1387](https://github.com/mobxjs/mobx/issues/1387) / [#1362](https://github.com/mobxjs/mobx/issues/1387)
+
+# 3.6.1
+
+* Fixed [#1358](https://github.com/mobxjs/mobx/pull/1359): Deep comparison failing on IE11. By [le0nik](https://github.com/le0nik) through [#1359](https://github.com/mobxjs/mobx/pull/1359)
+
+# 3.6.0
+
+* Fixed [#1118](https://github.com/mobxjs/mobx/issues/1118), the deepEquals operator build into mobx gave wrong results for non-primitive objects. This affected for example `computed.struct`, or the `compareStructural` of `reaction`
+
+# 3.5.0/1
+
+* Introduced `trace` for easier debugging of reactions / computed values. See the [docs](https://mobx.js.org/best/trace.html) for details.
+* Improved typings of `observableArray.find`, see [#1324](https://github.com/mobxjs/mobx/pull/1324) for details.
+
+# 3.4.1
+
+* Republished 3.4.0, because the package update doesn't seem to distibute consistently through yarn / npm
+
+# 3.4.0
+
+* Improve Flow support by exposing typings regularly. Flow will automatically include them now. In your `.flowconfig` will have to remove the import in the `[libs]` section (as it's done [here](https://github.com/mobxjs/mobx/pull/1254#issuecomment-348926416)). Fixes [#1232](https://github.com/mobxjs/mobx/issues/1232).
+
+# 3.3.3
+
+* Fixed regression bug where observable map contents could not be replaced using another observable map [#1258](https://github.com/mobxjs/mobx/issues/1258)
+* Fixed weird exception abot not being able to read `length` property of a function, see[#1238](https://github.com/mobxjs/mobx/issues/1238) through [#1257](https://github.com/mobxjs/mobx/issues/1238) by @dannsam
+
+# 3.3.2
+
+* Fix bug where custom comparers could be invoked with `undefined` values. Fixes [#1208](https://github.com/mobxjs/mobx/issues/1208)
+* Make typings for observable stricter when using flow [#1194](https://github.com/mobxjs/mobx/issues/1194), [#1231](https://github.com/mobxjs/mobx/issues/1231)
+* Fix a bug where `map.replace` would trigger reactions for unchanged values, fixes [#1243](https://github.com/mobxjs/mobx/issues/1243)
+* Fixed issue where `NaN` was considered unequal to `NaN` when a deep compare was made [#1249](https://github.com/mobxjs/mobx/issues/1249)
+
+# 3.3.1
+
+* Fix bug allowing maps to be modified outside actions when using strict mode, fixes [#940](https://github.com/mobxjs/mobx/issues/940)
+* Fixed [#1139](https://github.com/mobxjs/mobx/issues/1139) properly: `transaction` is no longer deprecated and doesn't disable tracking properties anymore
+* Fixed [#1120](https://github.com/mobxjs/mobx/issues/1139): `isComputed` should return false for non-existing properties
+
+# 3.3.0
+
+* Undeprecated `transaction`, see [#1139](https://github.com/mobxjs/mobx/issues/1139)
+* Fixed typings of reaction [#1136](https://github.com/mobxjs/mobx/issues/1136)
+* It is now possible to re-define a computed property [#1121](https://github.com/mobxjs/mobx/issues/1121)
+* Print an helpful error message when using `@action` on a getter [#971](https://github.com/mobxjs/mobx/issues/971)
+* Improved typings of intercept [#1119](https://github.com/mobxjs/mobx/issues/1119)
+* Made code base Prettier [#1103](https://github.com/mobxjs/mobx/issues/1103)
+* react-native will now by default use the es module build as well.
+* Added support for Weex, see [#1163](https://github.com/mobxjs/mobx/pull/1163/)
+* Added workaround for Firefox issue causing MobX to crash, see [#614](https://github.com/mobxjs/mobx/issues/614)
+
+# 3.2.2
+
+* Fixes a bug (or a known limitation) described in [#1092](https://github.com/mobxjs/mobx/issue/1092/). It is now possible to have different observable administration on different levels of the prototype chain. By @guillaumeleclerc
+* Fixed a build issue when using mobx in a project that was using rollup, fixes [#1099](https://github.com/mobxjs/mobx/issue/1099/) by @rossipedia
+* Fixed typings of `useStrict`, by @rickbeerendonk
+
+# 3.2.1
+
+* Introduced customizable value comperators to reactions and computed values. `reaction` and `computed` now support an additional option, `equals`, which takes a comparision function. See [#951](https://github.com/mobxjs/mobx/pull/951/) by @jamiewinder. Fixes #802 and #943. See the updated [`computed` docs](https://mobx.js.org/refguide/computed-decorator.html) for more details.
+
+# 3.2.0
+
+* MobX will warn again when there are multiple instances of MobX loaded, as this lead to often to confusing bugs if the project setup was not properly. The signal mobx that multiple instances are loaded on purpose, use `mobx.extras.runInSandbox`. See [#1082](https://github.com/mobxjs/mobx/issues/1082) for details.
+
+# 3.1.17
+
+* Improved typings of `IObservableArray.intercept`: use more restrictive types for `change` parameter of `handler`, by @bvanreeven
+* Fixed [#1072](https://github.com/mobxjs/mobx/issues/1072), fields without a default value could not be observed yet when using TypeScript
+
+# 3.1.16
+
+* Restored `default` export (and added warning), which broke code that was importing mobx like `import mobx from "mobx"`. Use `import * as mobx from "mobx"` or use named importes instead. By @andykog, see #1043, #1050
+* Fixed several typos in exceptions and documentation
+
+# 3.1.15
+
+* Fixed issue where `array.remove` did not work correctly in combination with `extras.interceptReads`
+
+# 3.1.14
+
+* Fixed 3.1.12 / 3.1.13 module packing. See #1039; `module` target is now transpiled to ES5 as well
+
+# 3.1.13 (Unpublished: Uglify chokes on it in CRA)
+
+* Fixed build issue with webpack 2, see #1040
+
+# 3.1.12 (Unpublished: wasn't being bundled correctly by all bundlers)
+
+* Added support for ES modules. See #1027 by @rossipedia
+* Improved flow typings. See #1019 by @fb55
+* Introduced experimental feature `extras.interceptReads(observable: ObservableMap | ObservableArray | ObservableObject | ObservableBox, property?: string, handler: value => value): Disposer` that can be used to intercept _reads_ from observable objects, to transform values on the fly when a value is read. One can achieve similar things with this as with proxying reads. See #1036
+
+# 3.1.11
+
+* Using rollup as bundler, instead of custom hacked build scripts, by @rossipedia, see #1023
+
+# 3.1.10
+
+* Fixed flow typings for `when`, by @jamsea
+* Add flow typings for `map.replace`, by @leader22
+* Added `observableArray.findIndex`, by @leader22
+* Improved typings of `autorun` / `autorunAsync` to better support async / await, by @capaj
+* Fixed typings of `action.bound`, see #803
+
+# 3.1.9
+
+* Introduced explicit `.get(index)` and `.set(index, value)` methods on observable arrays, for issues that have trouble handling many property descriptors on objects. See also #734
+* Made sure it is safe to call `onBecomeObserved` twice in row, fixes #874, #898
+* Fixed typings of `IReactionDisposer`
+
+# 3.1.8
+
+* Fixed edge case where `autorun` was not triggered again if a computed value was invalidated by the reaction itself, see [#916](https://github.com/mobxjs/mobx/issues/916), by @andykog
+* Added support for primtive keys in `createTransformer`, See #920 by @dnakov
+* Improved typings of `isArrayLike`, see #904, by @mohsen1
+
+# 3.1.7
+
+* Reverted ES2015 module changes, as they broke with webpack 2 (will be re-released later)
+
+# 3.1.6 (Unpublished)
+
+* Expose ES2015 modules to be used with advanced bundlers, by @mohsen1, fixes #868
+* Improved typings of `IObservableArray.intercept`: remove superflous type parameter, by @bvanreeven
+* Improved typings of map changes, by @hediet
+
+# 3.1.5
+
+* Improved typings of map changes, see #847, by @hediet
+* Fixed issue with `reaction` if `fireImmediately` was combined with `delay` option, see #837, by @SaboteurSpk
+
+# 3.1.4
+
+* Observable maps initialized from ES6 didn't deeply convert their values to observables. (fixes #869,by @ggarek)
+
+# 3.1.3
+
+* Make sure that `ObservableArray.replace` can handle large arrays by not using splats internally. (See e.g. #859)
+* Exposed `ObservableArray.spliceWithArray`, that unlike a normal splice, doesn't use a variadic argument list so that it is possible to splice in new arrays that are larger then allowed by the callstack.
+
+# 3.1.2
+
+* Fixed incompatiblity issue with `mobx-react@4.1.0`
+
+# 3.1.1 (unpublished)
+
+* Introduced `isBoxedObservable(value)`, fixes #804
+
+# 3.1.0
+
+### Improved strict mode
+
+Strict mode has been relaxed a bit in this release. Also computed values can now better handle creating new observables (in an action if needed). The semantics are now as follows:
+
+* In strict mode, it is not allowed to modify state that is already being _observed_ by some reaction.
+* It is allowed to create and modify observable values in computed blocks, as long as they are not _observed_ yet.
+
+In order words: Observables that are not in use anywhere yet, are not protected by MobX strict mode.
+This is fine as the main goal of strict mode is to avoid kicking of reactions at undesired places.
+Also strict mode enforces batched mutations of observables (through action).
+However, for unobserved observables this is not relevant; they won't kick of reactions at all.
+
+This fixes some uses cases where one now have to jump through hoops like:
+* Creating observables in computed properties was fine already, but threw if this was done with the aid of an action. See issue [#798](https://github.com/mobxjs/mobx/issues/798).
+* In strict mode, it was not possible to _update_ observable values without wrapping the code in `runInAction` or `action`. See issue [#563](https://github.com/mobxjs/mobx/issues/563)
+
+Note that the following constructions are still anti patterns, although MobX won't throw anymore on them:
+* Changing unobserved, but not just created observables in a computed value
+* Invoke actions in computed values. Use reactions like `autorun` or `reaction` instead.
+
+Note that observables that are not in use by a reaction, but that have `.observe` listeners attached, do *not* count towards being observed.
+Observe and intercept callbacks are concepts that do not relate to strict mode, actions or transactions.
+
+### Other changes
+
+* Reactions and observable values now consider `NaN === NaN`, See #805 by @andykog
+* Merged #783: extract error messages to seperate file, so that they can be optimized in production builds (not yet done), by @reisel, #GoodnessSquad
+* Improved typings of actions, see #796 by @mattiamanzati
+
+# 3.0.2
+
+* Fixed issue where MobX failed on environments where `Map` is not defined, #779 by @dirtyrolf
+* MobX can now be compiled on windows as well! #772 by @madarauchiha #GoodnessSquad
+* Added documentation on how Flow typings can be used, #766 by @wietsevenema
+* Added support for `Symbol.toPrimitive()` and `valueOf()`, see #773 by @eladnava #GoodnessSquad
+* Supressed an exception that was thrown when using the Chrome Developer tools to inspect arrays, see #752
+
+Re-introduced _structural comparison_. Seems we couldn't part from it yet :). So the following things have been added:
+
+* `struct` option to `reaction` (alias for `compareStructural`, to get more consistency in naming)
+* `observable.struct`, as alias for `observable.deep.struct`
+* `observable.deep.struct`: Only stores a new value and notify observers if the new value is not structurally the same as the previous value. Beware of cycles! Converts new values automatically to observables (like `observable.deep`)
+* `observable.ref.struct`: Only stores a new value and notify observers if the new value is not structurally the same as the previous value. Beware of cycles! Doesn't convert the new value into observables.
+* `extras.deepEquals`: Check if two data structures are deeply equal. supports observable and non observable data structures.
+
+# 3.0.1
+
+* `toString()` of observable arrays now behaves like normal arrays (by @capaj, see #759)
+* Improved flow types of `toJS`by @jamsea (#758)
+
+# 3.0.0
+
+The changelog of MobX 3 might look quite overwhelming, but migrating to MobX 3 should be pretty straight forward nonetheless.
+The api has now become more layered, and the api is more uniform and modifiers are cleaned up.
+In practice, you should check your usage of modifiers (`asFlat`, `asMap` etc.). Besides that the migration should be pretty painless.
+Please report if this isn't the case!
+Note that no changes to the runtime algorithm where made, almost all changes evolve in making the creation of observables more uniform, and removing deprecated stuff.
+
+## `observable` api has been redesigned
+
+The api to create observables has been redesigned.
+By default, it keeps the automatic conversion behavior from MobX 2.
+However, one can now have more fine grained control on how / which observables are constructed.
+Modifiers still exists, but they are more regular, and there should be less need for them.
+
+### `observable(plainObject)` will no longer enhance objects, but clone instead
+
+When passing a plain object to `observable`, MobX used to modify that object inplace and give it observable capabilities.
+This also happened when assigning a plain object to an observable array etc.
+However, this behavior has changed for a few reasons
+
+1. Both arrays and maps create new data structure, however, `observable(object)` didn't
+2. It resulted in unnecessary and confusing side effects. If you passed an object you received from some api to a function that added it, for example, to an observable collection. Suddenly your object would be modified as side effect of passing it down to that function. This was often confusing for beginners and could lead to subtle bugs.
+3. If MobX in the future uses Proxies behind the scenes, this would need to change as well
+
+If you want, you can still enhance existing plainObjects, but simply using `extendObservable(data, data)`. This was actually the old implementation, which has now changed to `extendObservable({}, data)`.
+
+As always, it is best practice not to have transportation objects etc lingering around; there should be only one source of truth, and that is the data that is in your observable state.
+If you already adhered to this rule, this change won't impact you.
+
+See [#649](https://github.com/mobxjs/mobx/issues/649)
+
+### Factories per observable type
+
+There are now explicit methods to create an observable of a specific type.
+
+* `observable.object(props, name?)` creates a new observable object, by cloning the give props and making them observable
+* `observable.array(initialValues, name?)`. Take a guess..
+* `observable.map(initialValues, name?)`
+* `observable.box(initialValue, name?)`. Creates a [boxed](http://mobxjs.github.io/mobx/refguide/boxed.html) value, which can be read from / written to using `.get()` and `.set(newValue)`
+* `observable(value)`, as-is, based on the type of `value`, uses any of the above four functions to create a new observable.
+
+### Shallow factories per type
+
+The standard observable factories create observable structures that will try to turn any plain javascript value (arrays, objects or Maps) into observables.
+Allthough this is fine in most cases, in some cases you might want to disable this autoconversion.
+For example when storing objects from external libraries.
+In MobX 2 you needed to use `asFlat` or `asReference` modifiers for this.
+In MobX 3, there are factories to directly create non-converting data structures:
+
+* `observable.shallowObject(props, name?)`
+* `observable.shallowArray(initialValues, name?)`
+* `observable.shallowMap(initialValues, name?)`
+* `observable.shallowBox(initialValue, name?)`
+
+So for example, `observable.shallowArray([todo1, todo2])` will create an observable array, but it won't try to convert the todos inside the array into observables as well.
+
+### Shallow properties
+
+The `@observable` decorator can still be used to introduce observable properties. And like in MobX 2, it will automatically convert its values.
+
+However, sometimes you want to create an observable property that does not convert its _value_ into an observable automatically.
+Previously that could be written as `@observable x = asReference(value)`.
+
+### Structurally comparison of observables have been removed
+
+This was not for a technical reason, but they just seemed hardly used.
+Structural comparision for computed properties and reactions is still possible.
+Feel free to file an issue, including use case, to re-introduce this feature if you think you really need it.
+However, we noticed that in practice people rarely use it. And in cases where it is used `reference` / `shallow` is often a better fit (when using immutable data for example).
+
+### Modifiers
+
+Modifiers can be used in combination `@observable`, `extendObservable` and `observable.object` to change the autoconversion rules of specific properties.
+
+The following modifiers are available:
+
+* `observable.deep`: This is the default modifier, used by any observable. It converts any assigned, non-primitive value into an observable value if it isn't one yet.
+* `observable.ref`: Disables automatic observable conversion, just creates an observable reference instead.
+* `observable.shallow`: Can only used in combination with collections. Turns any assigned collection into an collection, which is shallowly observable (instead of deep)
+
+Modifiers can be used as decorator:
+
+```javascript
+class TaskStore {
+ @observable.shallow tasks = []
+}
+```
+
+Or as property modifier in combination with `observable.object` / `observable.extendObservable`.
+Note that modifiers always 'stick' to the property. So they will remain in effect even if a new value is assigned.
+
+```javascript
+const taskStore = observable({
+ tasks: observable.shallow([])
+})
+```
+
+See [modifiers](http://mobxjs.github.io/mobx/refguide/modifiers.html)
+
+### `computed` api has been simplified
+
+Using `computed` to create boxed observables has been simplified, and `computed` can now be invoked as follows:
+* `computed(expr)`
+* `computed(expr, setter)`
+* `computed(expr, options)`, where options is an object that can specify one or more of the following fields: `name`, `setter`, `compareStructural` or `context` (the "this").
+
+Computed can also be used as a decorator:
+
+* `@computed`
+* `@computed.struct` when you want to compareStructural (previously was `@computed({asStructure: true})`)
+
+### `reaction` api has been simplified
+
+The signature of `reaction` is now `reaction(dataFunc, effectFunc, options?)`, where the following options are accepted:
+
+* `context`: The `this` to be used in the functions
+* `fireImmediately`
+* `delay`: Number in milliseconds that can be used to debounce the effect function.
+* `compareStructural`: `false` by default. If `true`, the return value of the *data* function is structurally compared to its previous return value, and the *effect* function will only be invoked if there is a structural change in the output.
+* `name`: String
+
+### Bound actions
+
+It is now possible to create actions and bind them in one go using `action.bound`. See [#699](https://github.com/mobxjs/mobx/issues/699).
+This means that now the following is possible:
+
+```javascript
+class Ticker {
+ @observable tick = 0
+
+ @action.bound
+ increment() {
+ this.tick++ // 'this' will always be correct
+ }
+}
+
+const ticker = new Ticker()
+setInterval(ticker.increment, 1000)
+```
+
+### Improve error handling
+
+Error handling in MobX has been made more consistent. In MobX 2 there was a best-effort recovery attempt if a derivation throws, but MobX 3 introduced
+more consistent behavior:
+
+* Computed values that throw, store the exception and throw it to the next consumer(s). They keep tracking their data, so they are able to recover from exceptions in next re-runs.
+* Reactions (like `autorun`, `when`, `reaction`, `render()` of `observer` components) will always catch their exceptions, and just log the error. They will keep tracking their data, so they are able to recover in next re-runs.
+* The disposer of a reaction exposes an `onError(handler)` method, which makes it possible to attach custom error handling logic to an reaction (that overrides the default logging behavior).
+* `extras.onReactionError(handler)` can be used to register a global onError handler for reactions (will fire after spy "error" event). This can be useful in tests etc.
+
+See [#731](https://github.com/mobxjs/mobx/issues/731)
+
+### Removed error handling, improved error recovery
+
+MobX always printed a warning when an exception was thrown from a computed value, reaction or react component: `[mobx] An uncaught exception occurred while calculating....`.
+This warning was often confusing for people because they either had the impression that this was a mobx exception, while it actually is just informing about an exception that happened in userland code.
+And sometimes, the actual exception was silently caught somewhere else.
+MobX now does not print any warnings anymore, and just makes sure its internal state is still stable.
+Not throwing or handling an exception is now entirely the responsibility of the user.
+
+Throwing an exception doesn't revert the causing mutation, but it does reset tracking information, which makes it possible to recover from exceptions by changing the state in such a way that a next run of the derivation doesn't throw.
+
+### Flow-Types Support 🎉🎉🎉
+
+Flow typings have been added by [A-gambit](https://github.com/A-gambit).
+Add flow types for methods and interfaces of observable variables:
+
+```js
+const observableValue: IObservableValue = observable(1)
+const observableArray: IObservableArray = observable([1,2,3])
+
+const sum: IComputedValue = computed(() => {
+ return observableArray.reduce((a: number, b: number): number => a + b, 0)
+})
+```
+
+See [#640](https://github.com/mobxjs/mobx/issues/640)
+
+### MobX will no longer share global state by default
+
+For historical reasons (at Mendix), MobX had a feature that it would warn if different versions of the MobX package are being loaded into the same javascript runtime multiple times.
+This is because multiple instances by default try to share their state.
+This allows reactions from one package to react to observables created by another package,
+even when both packages where shipped with their own (embedded) version of MobX (!).
+
+Obviously this is a nasty default as it breaks package isolation and might actually start to throw errors unintentionally when MobX is loaded multiple times in the same runtime by completely unrelated packages.
+So this sharing behavior is now by default turned off.
+Sharing MobX should be achieved by means of proper bundling, de-duplication of packages or using peer dependencies / externals if needed.
+This is similar to packages like React, which will also bail out if you try to load it multiple times.
+
+If you still want to use the old behavior, this can be achieved by running `mobx.extras.shareGlobalState()` on _all_ packages that want to share state with each other.
+Since this behavior is probably not used outside Mendix, it has been deprecated immediately, so if you rely on this feature, please report in #621, so that it can be undeprecated if there is no more elegant solution.
+
+See [#621](https://github.com/mobxjs/mobx/issues/621)
+
+### Other changes
+
+* **Breaking change:** The arguments to `observe` listeners for computed and boxed observables have changed and are now consistent with the other apis. Instead of invoking the callback with `(newValue: T, oldValue: T)` they are now invoked with a single change object: `(change: {newValue: T, oldValue: T, object, type: "update"})`
+* Using transaction is now deprecated, use `action` or `runInAction` instead. Transactions now will enter an `untracked` block as well, just as actions, which removes the conceptual difference.
+* Upgraded to typescript 2
+* It is now possible to pass ES6 Maps to `observable` / observable maps. The map will be converted to an observable map (if keys are string like)
+* Made `action` more debug friendly, it should now be easier to step through
+* ObservableMap now has an additional method, `.replace(data)`, which is a combination of `clear()` and `merge(data)`.
+* Passing a function to `observable` will now create a boxed observable refering to that function
+* Fixed #603: exceptions in transaction breaks future reactions
+* Fixed #698: createTransformer should support default arguments
+* Transactions are no longer reported grouped in spy events. If you want to group events, use actions instead.
+* Normalized `spy` events further. Computed values and actions now report `object` instead of `target` for the scope they have been applied to.
+* The following deprecated methods have been removed:
+ * `transaction`
+ * `autorunUntil`
+ * `trackTransitions`
+ * `fastArray`
+ * `SimpleEventEmitter`
+ * `ObservableMap.toJs` (use `toJS`)
+ * `toJSlegacy`
+ * `toJSON` (use `toJS`)
+ * invoking `observe` and `inject` with plain javascript objects
+
+---
+
+# 2.7.0
+
+### Automatic inference of computed properties has been deprecated.
+
+A deprecation message will now be printed if creating computed properties while relying on automatical inferrence of argumentless functions as computed values. In other words, when using `observable` or `extendObservable` in the following manner:
+
+```javascript
+const x = observable({
+ computedProp: function() {
+ return someComputation
+ }
+})
+
+// Due to automatic inferrence now available as computed property:
+x.computedProp
+// And not !
+x.computedProp()
+```
+
+Instead, to create a computed property, use:
+
+```javascript
+observable({
+ get computedProp() {
+ return someComputation
+ }
+})
+```
+
+or alternatively:
+
+```javascript
+observable({
+ computedProp: computed(function() {
+ return someComputation
+ })
+})
+```
+
+This change should avoid confusing experiences when trying to create methods that don't take arguments.
+The current behavior will be kept as-is in the MobX 2.* range,
+but from MobX 3 onward the argumentless functions will no longer be turned
+automatically into computed values; they will be treated the same as function with arguments.
+An observable _reference_ to the function will be made and the function itself will be preserved.
+See for more details [#532](https://github.com/mobxjs/mobx/issues/532)
+
+N.B. If you want to introduce actions on an observable that modify its state, using `action` is still the recommended approach:
+
+```javascript
+observable({
+ counter: 0,
+ increment: action(function() {
+ this.counter++
+ })
+})
+```
+
+By the way, if you have code such as:
+
+```
+observable({
+ @computed get someProp() { ... }
+});
+```
+
+That code will no longer work. Rather, reactions will fail silently. Remove `@computed`.
+Note, this only applies when using observable in this way; it doesn't apply when using
+`@observable` on a property within a class declaration.
+
+### Misc
+
+* Fixed #701: `toJS` sometimes failing to convert objects decorated with `@observable` (cause: `isObservable` sometimes returned false on these object)
+* Fixed typings for `when` / `autorun` / `reaction`; they all return a disposer function.
+
+
+# 2.6.5
+
+* Added `move` operation to observable array, see [#697](https://github.com/mobxjs/mobx/pull/697)
+
+# 2.6.4
+
+* Fixed potential clean up issue if an exception was thrown from an intercept handler
+* Improved typings of `asStructure` (by @nidu, see #687)
+* Added support for `computed(asStructure(() => expr))` (by @yotambarzilay, see #685)
+
+# 2.6.3
+
+* Fixed #603: exceptions in transaction breaks future reactions
+* Improved typings of `toJS`
+* Introduced `setReactionScheduler`. Internal api used by mobx-react@4 to be notified when reactions will be run
+
+# 2.6.2
+
+* Changes related to `toJS` as mentioned in version `2.6.0` where not actually shipped. This has been fixed, so see release notes below.
+
+# 2.6.1
+
+* Introduced convenience `isArrayLike`: returns whether the argument is either a JS- or observable array. By @dslmeinte
+* Improved readme. By @DavidLGoldberg
+* Improved assertion message, by @ncammarate (See [#618](https://github.com/mobxjs/mobx/pull/618))
+* Added HashNode badge, by @sandeeppanda92
+
+# 2.6.0
+
+_Marked as minor release as the behavior of `toJS` has been changed, which might be interpreted both as bug-fix or as breaking change, depending of how you interpreted the docs_
+
+* Fixed [#566](https://github.com/mobxjs/mobx/pull/566): Fixed incorrect behavior of `toJS`: `toJS` will now only recurse into observable object, not all objects. The new behavior is now aligned with what is suggested in the docs, but as a result the semantics changed a bit. `toJSlegacy` will be around for a while implementing the old behavior. See [#589](See https://github.com/mobxjs/mobx/pull/589) for more details.
+* Fixed [#571](https://github.com/mobxjs/mobx/pull/571): Don't use `instanceof` operator. Should fix issues if MobX is included multiple times in the same bundle.
+* Fixed [#576](https://github.com/mobxjs/mobx/pull/576): disallow passing actions directly to `autorun`; as they won't be tracked by @jeffijoe
+* Extending observable objects with other observable (objects) is now explicitly forbidden, fixes [#540](https://github.com/mobxjs/mobx/pull/540).
+
+# 2.5.2
+
+* Introduced `isComputed`
+* Observable objects can now have a type: `IObservableObject`, see [#484](https://github.com/mobxjs/mobx/pull/484) by @spiffytech
+* Restored 2.4 behavior of boxed observables inside observable objects, see [#558](https://github.com/mobxjs/mobx/issues/558)
+
+# 2.5.1
+
+* Computed properties can now be created by using getter / setter functions. This is the idiomatic way to introduce computed properties from now on:
+
+```javascript
+const box = observable({
+ length: 2,
+ get squared() {
+ return this.length * this.length
+ },
+ set squared(value) {
+ this.length = Math.sqrt(value)
+ }
+})
+```
+
+# 2.5.0
+
+* Core derivation algorithm has received some majore improvements by @asterius1! See below. Pr #452, 489
+* Introduced setters for computed properties, use `computed(expr, setter)` or `@computed get name() { return expr } set name (value) { action }`. `computed` can now be used as modifier in `observable` / `extendObservable`, #421, #463 (see below for example)
+* Introduced `isStrictModeEnabled()`, deprecated `useStrict()` without arguments, see #464
+* Fixed #505, accessing an observable property throws before it is initialized
+
+MobX is now able track and memoize computed values while an (trans)action is running.
+Before 2.5, accessing a computed value during a transaction always resulted in a recomputation each time the computed value was accessed, because one of the upstream observables (might) have changed.
+In 2.5, MobX actively tracks whether one of the observables has changed and won't recompute computed values unnecessary.
+This means that computed values are now always memoized for the duration of the current action.
+In specific cases, this might signficantly speed up actions that extensively make decisions based on computed values.
+
+Example:
+```javascript
+class Square {
+ @observable length = 2
+ @computed get squared() {
+ return this.length * this.length
+ }
+ // mobx now supports setters for computed values
+ set squared(surfaceSize) {
+ this.length = Math.sqrt(surfaceSize)
+ }
+
+ // core changes make actions more efficient if extensively using computed values:
+ @action stuff() {
+ this.length = 3
+ console.log(this.squared) // recomputes in both 2.5 and before
+ console.log(this.squared) // no longer recomputes
+ this.length = 4
+ console.log(this.squared) // recomputes in both 2.5 and before
+ // after the action, before 2.5 squared would compute another time (if in use by a reaction), that is no longer the case
+ }
+}
+```
+
+ES5 example for setters:
+```javascript
+function Square() {
+ extendObservable(this, {
+ length: 2,
+ squared: computed(
+ function() {
+ return this.squared * this.squared
+ },
+ function(surfaceSize) {
+ this.length = Math.sqrt(surfaceSize)
+ }
+ )
+ })
+}
+```
+
+# 2.4.4
+
+* Fixed #503: map.delete returns boolean
+* Fix return type of `runInAction`, #499 by @Strate
+* Fixed enumerability of observable array methods, see #496.
+* Use TypeScript typeguards, #487 by @Strate
+* Added overloads to `action` for better type inference, #500 by @Strate
+* Fixed #502: `extendObservable` fails on objects created with `Object.create(null)`
+* Implemented #480 / #488: better typings for `asMap`, by @Strate
+
+# 2.4.3
+
+* Objects with a `null` prototype are now considered plain objects as well
+* Improved error message for non-converging cyclic reactions
+* Fixed potential HMR issue
+
+# 2.4.2
+
+* Improved error message when wrongly using `@computed`, by @bb (#450)
+* `observableArray.slice` now automatically converts observable arrays to plain arrays, fixes #460
+* Improved error message when an uncaught exception is thrown by a MobX tracked function
+
+# 2.4.1
+
+* `@action` decorated methods are now configurable. Fixes #441
+* The `onBecomeObserved` event handler is now triggered when an atom is observed, instead of when it is bound as dependency. Fixes #427 and makes atoms easier to extend.
+* if `useStrict()` is invoked without arguments, it now returns the current value of strict mode.
+* the current reaction is now always passed as first argument to the callbacks of `autorun`, `autorunAsync`, `when` and `reaction`. This allows reactions to be immediately disposed during the first run. See #438, by @andykog
+
+# 2.4.0
+
+* _Note: the internal version of MobX has been bumped. This version has no breaking api changes, but if you have MobX loaded multiple times in your project, they all have to be upgraded to `2.4.0`. MobX will report this when starting._
+* Made dependency tracking and binding significant faster. Should result in huge performance improvements when working with large collections.
+* Fixed typescript decorator issue, #423, #425? (by @bb)
+
+# 2.3.7
+
+* Fixed issue where computed values were tracked and accidentally kept alive during actions
+
+# 2.3.6
+* Fixed #406: Observable maps doesn't work with empty initial value in Safari
+* Implemented #357, #348: ObservableMap and ObservableArray now support iterators. Use [`@@iterator()` or iterall](https://github.com/leebyron/iterall) in ES5 environments.
+
+# 2.3.5
+
+* Fixed #364: Observable arrays not reacting properly to index assignments under iOS safari (mobile) 9.1.1 By @andykog
+* Fixed #387: Typings of boxed values
+* Added warning when reading array entries out of bounds. See #381
+
+# 2.3.4
+
+* Fixed #360: Removed expensive cycle detection (cycles are still detected, but a bit later)
+* Fixed #377: `toJS` serialization of Dates and Regexes preserves the original values
+* Fixed #379: `@action` decorated methods can now be inherited / overriden
+
+# 2.3.3
+
+* Fixed #186: Log a warning instead of an error if an exception is thrown in a derivation. Fixes issue where React Native would produce unusable error screens (because it shows the first logged error)
+* Fixed #333: Fixed some interoperability issues in combination with `Reflect` / `InversifyJS` decorators. @andykog
+* Fixed #333: `@observable` class properties are now _owned_ by their instance again, meaning they will show up in `Object.keys()` and `.hasOwnProperty` @andykog
+
+# 2.3.2
+
+* Fixed #328: Fixed exception when inspecting observable in `onBecomeObserved`
+* Fixed #341: `array.find` now returns `undefined` instead of `null` when nothing was found, behavior now matches the docs. (By @hellectronic)
+
+# 2.3.1
+
+* Fixed #327: spy not working with runInAction
+
+# 2.3.0
+
+### Introduced `whyRun`:
+Usage:
+* `whyRun()`
+* `whyRun(Reaction object / ComputedValue object / disposer function)`
+* `whyRun(object, "computed property name")`
+
+`whyRun` is a small utility that can be used inside computed value or reaction (`autorun`, `reaction` or the `render` method of an `observer` React component)
+and prints why the derivation is currently running, and under which circumstances it will run again.
+This should help to get a deeper understanding when and why MobX runs stuff, and prevent some beginner mistakes.
+
+This feature can probably be improved based on your feedback, so feel free to file issues with suggestions!
+
+### Semantic changes:
+* `@observable` is now always defined on the class prototypes and not in the instances. This means that `@observable` properties are enumerable, but won't appear if `Object.keys` or `hasOwnProperty` is used on a class _instance_.
+* Updated semantics of `reaction` as discussed in `#278`. The expression now needs to return a value and the side effect won't be triggered if the result didn't change. `asStructure` is supported in these cases. In contrast to MobX 2.2, effects will no longer be run if the output of the expression didn't change.
+
+### Enhancements
+
+* Introduces `isAction(fn)` #290
+* If an (argumentless) action is passed to `observable` / `extendObservable`, it will not be converted into a computed property.
+* Fixed #285: class instances are now also supported by `toJS`. Also members defined on prototypes which are enumerable are converted.
+* Map keys are now always coerced to strings. Fixes #308
+* `when`, `autorun` and `autorunAsync` now accept custom debug names (see #293, by @jamiewinder)
+* Fixed #286: autoruns no longer stop working if an action throws an exception
+* Implemented `runInAction`, can be used to create on the fly actions (especially useful in combination with `async/await`, see #299
+* Improved performance and reduced mem usage of decorators signficantly (by defining the properties on the prototype if possible), and removed subtle differences between the implementation and behavior in babel and typescript.
+* Updated logo as per #244. Tnx @osenvosem!
+
+# 2.2.2:
+
+* Fixed issue #267: exception when `useStrict(true)` was invoked in combination with `@observable` attributes when using Babel
+* Fixed issue #269: @action in combination with typescript targeting ES6 and reflect.ts
+* Improved compatibility with `JSON.stringify`, removed incorrect deprecation message
+* Improved some error messages
+
+# 2.2.1
+
+* Fixed issue where typescript threw a compile error when using `@action` without params on a field
+* Fixed issue where context was accidentally shared between class instances when using `@action` on a field
+
+# 2.2.0
+
+See the [release announcement](https://medium.com/@mweststrate/45cdc73c7c8d) for the full details of this release:
+
+Introduced:
+* `action` / `@action`
+* `intercept`
+* `spy`
+* `reaction`
+* `useStrict`
+* improved debug names
+* `toJSON` was renamed to `toJS`
+* `observable(asMap())` is the new idiomatic way to create maps
+* the effect of `when` is now untracked, similar to `reaction.
+* `extras.trackTransations` is deprecated, use `spy` instead
+* `untracked` has been undeprecated
+* introduced / documented: `getAtom`, `getDebugName`, `isSpyEnabled`, `spyReport`, `spyReportStart`, `spyReportEnd`
+* deprecated `extras.SimpleEventEmitter`
+* array splice events now also report the `added` collection and `removedCount`
+
+# 2.1.7
+
+* Fixed a false negative in cycle detection, as reported in #236
+
+# 2.1.6
+
+* Fixed #236, #237 call stack issues when working with large arrays
+
+# 2.1.5
+
+* Fix #222 (by @andykog) run `observe` callback of computed properties in untracked mode.
+
+# 2.1.4
+
+* Fixed #201 (see also #160), another iOS enumerability issue... By @luosong
+
+# 2.1.3
+
+* Fixed #191, when using babel, complex field initializers where shared. By @andykog
+* Added `lib/mobx.umd.min.js` for minified cdn builds, see #85
+
+# 2.1.2
+
+* Improved debug names of objects created using a constructor
+* Fixed(?) some issues with iOS7 as reported in #60 by @bstst
+
+# 2.1.1
+
+* Fixed issue where `autorun`s created inside `autorun`s were not always kicked off. (`mobx-react`'s `observer` was not affected). Please upgrade if you often use autorun.
+* Fixed typings of `mobx.map`, a list of entries is also acceptable.
+* (Experimental) Improved error recovery a bit further
+
+# 2.1.0
+
+* MobX is now chatty again when an exception occurs inside a autorun / computed value / React.render. Previously this was considered to be the responsibility of the surrounding code. But if exceptions were eaten this would be really tricky to debug.
+* (Experimental) MobX will now do a poor attempt to recover from exceptions that occured in autorun / computed value / React.render.
+
+# 2.0.6
+
+* `resetGlobalState` is now part of the `mobx.extras` namespace, as it is useful for test setup, to restore inconsistent state after test failures.
+* `resetGlobalState` now also resets the caches of `createTransformer`, see #163.
+
+# 2.0.5
+
+* WIP on bower support
+
+# 2.0.4
+
+* `$transformId` property on transformed objects should be non-enumerable. Fixes #170.
+
+# 2.0.3
+
+* Always peek if inspecting a stale, computed value. Fixes #165.
+
+# 2.0.2
+
+* Fixed issue where changing an object property was tracked, which could lead to unending loops in `autorunAsync`.
+
+# 2.0.1
+
+* Undeprecated `observable(scalar)` (see 143)
+* `expr` no longer prints incorrect deprecated messages (see 143)
+* Requires `mobx` twice no longer fails.
+
+# 2.0.0
+
+## A new name...
+Welcome to ~Mobservable~ MobX 2! First of all, there is the name change.
+The new name is shorter and funnier and it has the right emphasis: MobX is about reactive programming.
+Not about observability of data structures, which is just a technical necessity.
+MobX now has its own [mobxjs](https://github.com/mobxjs) organization on GitHub. Just report an issue if you want to join.
+
+All MobX 2.0 two compatible packages and repos have been renamed. So `mobx-react`, `mobx-react-devtools` etc.
+For the 1.0 versions, use the old `mobservable` based names.
+
+## Migrating from Mobservable 1.x to MobX 2.0
+
+Migrating from Mobservable should be pretty straight-forward as the public api is largely the same.
+However there are some conceptual changes which justifies a Major version bump as it might alter the behavior of MobX in edge cases.
+Besides that, MobX is just a large collection of minor improvements over Mobservable.
+Make sure to remove your old `mobservable` dependencies when installing the new `mobx` dependencies!
+
+## `autorun`s are now allowed to cause cycles!
+`autorun` is now allowed to have cycles. In Mobservable 1 an exception was thrown as soon as an autorun modified a variable which it was reading as well.
+In MobX 2 these situations are now allowed and the autorun will trigger itself to be fired again immediately after the current execution.
+This is fine as long as the autorun terminates within a reasonable amount of iterations (100).
+This should avoid the need for work-arounds involving `setTimeout` etc.
+Note that computed values (created using `observable(func)` are still not allowed to have cycles.
+
+## [Breaking] `observable(scalar)` returns an object instead of a function and has been deprecated.
+
+Creating an observable from a primitive or a reference no longer returns a getter/setter function, but a method with a `.get` and `.set` method.
+This is less confusing, easier to debug and more efficient.
+
+So to read or write from an observable scalar use:
+```javascript
+const temperature = observable(27);
+temperature.set(15); // previously: temperature(15)
+temperature.get(); // previously: temperature()
+```
+
+`observable(scalar)` has been deprecated to make the api smaller and the syntax more uniform. In practice having observable objects, arrays and decorators seems to suffice in 99% of the cases. Deprecating this functionality means that people have simply less concepts to learn. Probably creating observable scalars will continue to work for a long time, as it is important to the internals of MobX and very convenient for testing.
+
+## Introduced `@computed`
+
+MobX introduced the `@computed` decorator for ES6 class properties with getter functions.
+It does technically the same as `@observable` for getter properties. But having a separate decorator makes it easier to communicate about the code.
+`@observable` is for mutable state properties, `@computed` is for derived values.
+
+`@computed` can now also be parameterized. `@computed({asStructure: true})` makes sure that the result of a derivation is compared structurally instead of referentially with its preview value. This makes sure that observers of the computation don't re-evaluate if new structures are returned that are structurally equal to the original ones. This is very useful when working with point, vector or color structures for example. It behaves the same as the `asStructure` modifier for observable values.
+
+`@computed` properties are no longer enumerable.
+
+## MobX is now extensible!
+
+The core algorithm of MobX has been largely rewritten to improve the clarity, extensibility, performance and stability of the source code.
+It is now possible to define your own custom observable data sources by using the `Atom` class.
+It is also possible to create your own reactive functions using the `Reaction` class. `autorun`, `autorunAsync` and `@observer` have now all been implemented using the concept of Reactions.
+So feel free to write your own reactive [constructions](http://mobxjs.github.io/mobx/refguide/extending.html)!
+
+## Mobservable now fails fast
+
+In Mobservable 1 exceptions would be caught and sometimes re-thrown after logging them.
+This was confusing and not all derivations were able to recover from these exceptions.
+In MobX 2 it is no longer allowed for a computed function or `autorun` to throw an exception.
+
+## Improved build
+
+* MobX is roughly 20% faster
+* MobX is smaller: 75KB -> 60KB unminified, and 54KB -> 30KB minified.
+* Distributable builds are no longer available in the git repository, use unpkg instead:
+* Commonjs build: https://unpkg.com/mobx@^2.0.0/lib/mobx.js
+* Minified commonjs build: https://unpkg.com/mobx@^2.0.0/lib/mobx.min.js
+* UMD build: https://unpkg.com/mobx@^2.0.0/lib/mobx.umd.js
+* To use the minified build, require / import the lib from `"mobx/lib/mobx.min.js"` (or set up an alias in your webpack configuration if applicable)
+
+## Other changes
+
+* Improved debug names of all observables. This is especially visible when using `mobx-react-devtools` or `extras.trackTransitions`.
+* Renamed `extras.SimpleEventEmitter` to `SimpleEventEmitter`
+* Removed already deprecated methods: `isReactive`, `makeReactive`, `observeUntil`, `observeAsync`
+* Removed `extras.getDNode`
+* Invoking `ObservableArray.peek` is no longer registered as listener
+* Deprecated `untracked`. It wasn't documented and nobody seems to miss it.
+
+# 1.2.5
+
+* Map no longer throws when `.has`, `.get` or `.delete` is invoked with an invalid key (#116)
+* Files are now compiled without sourcemap to avoid issues when loading mobservable in a debugger when `src/` folder is not available.
+
+# 1.2.4
+
+* Fixed: observable arrays didn't properly apply modifiers if created using `asFlat([])` or `fastArray([])`
+* Don't try to make frozen objects observable (by @andykog)
+* `observableArray.reverse` no longer mutates the arry but just returns a sorted copy
+* Updated tests to use babel6
+
+# 1.2.3
+
+* observableArray.sort no longer mutates the array being sorted but returns a sorted clone instead (#90)
+* removed an incorrect internal state assumption (#97)
+
+# 1.2.2
+
+* Add bower support
+
+# 1.2.1
+
+* Computed value now yields consistent results when being inspected while in transaction
+
+# 1.2.0
+
+* Implemented #67: Reactive graph transformations. See: http://mobxjs.github.io/mobservable/refguide/create-transformer.html
+
+# 1.1.8
+
+* Implemented #59, `isObservable` and `observe` now support a property name as second param to observe individual values on maps and objects.
+
+# 1.1.7
+
+* Fixed #77: package consumers with --noImplicitAny should be able to build
+
+# 1.1.6
+
+* Introduced `mobservable.fastArray(array)`, in addition to `mobservable.observable(array)`. Which is much faster when adding items but doesn't support enumerability (`for (var idx in ar) ..` loops).
+* Introduced `observableArray.peek()`, for fast access to the array values. Should be used read-only.
+
+# 1.1.5
+
+* Fixed 71: transactions should not influence running computations
+
+# 1.1.4
+
+* Fixed #65; illegal state exception when using a transaction inside a reactive function. Credits: @kmalakoff
+
+# 1.1.3
+
+* Fixed #61; if autorun was created during a transaction, postpone execution until the end of the transaction
+
+# 1.1.2
+
+* Fixed exception when autorunUntil finished immediately
+
+# 1.1.1
+
+* `toJSON` now serializes object trees with cycles as well. If you know the object tree is acyclic, pass in `false` as second parameter for a performance gain.
+
+# 1.1.0
+
+* Exposed `ObservableMap` type
+* Introduced `mobservable.untracked(block)`
+* Introduced `mobservable.autorunAsync(block, delay)`
+
+# 1.0.9
+
+Removed accidental log message
+
+# 1.0.7 / 1.0.8
+
+Fixed inconsistency when using `transaction` and `@observer`, which sometimes caused stale values to be displayed.
+
+# 1.0.6
+
+Fix incompatibility issue with systemjs bundler (see PR 52)
+
+# 1.0.4/5
+
+* `map.size` is now a property instead of a function
+* `map()` now accepts an array as entries to construct the new map
+* introduced `isObservableObject`, `isObservableArray` and `isObservableMap`
+* introduced `observe`, to observe observable arrays, objects and maps, similarly to Object.observe and Array.observe
+
+# 1.0.3
+
+* `extendObservable` now supports passing in multiple object properties
+
+# 1.0.2
+
+* added `mobservable.map()`, which creates a new map similarly to ES6 maps, yet observable. Until properly documentation, see the [MDN docs](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map).
+
+# 1.0.1
+
+* Stricter argument checking for several apis.
+
+# 1.0
+
+## Renames
+
+* `isReactive` -> `isObservable`
+* `makeReactive` -> `observable`
+* `extendReactive` -> `extendObservable`
+* `observe` -> `autorun`
+* `observeUntil` -> `autorunUntil`
+* `observeAsync` -> `autorunAsync`
+* `reactiveComponent` -> `observer` (in `mobservable-react` package)
+
+## Breaking changes
+
+* dropped the `strict` and `logLevel` settings of mobservable. View functions are by default run in `strict` mode, `autorun` (formerly: `observe`) functions in `non-strict` mode (strict indicates that it is allowed to change other observable values during the computation of a view funtion).
+Use `extras.withStrict(boolean, block)` if you want to deviate from the default behavior.
+* `observable` (formerly `makeReactive`) no longer accepts an options object. The modifiers `asReference`, `asStructure` and `asFlat` can be used instead.
+* dropped the `default` export of observable
+* Removed all earlier deprecated functions
+
+## Bugfixes / improvements
+
+* `mobservable` now ships with TypeScript 1.6 compliant module typings, no external typings file is required anymore.
+* `mobservable-react` supports React Native as well through the import `"mobservable-react/native"`.
+* Improved debugger support
+* `for (var key in observablearray)` now lists the correct keys
+* `@observable` now works correct on classes that are transpiled by either TypeScript or Babel (Not all constructions where supported in Babel earlier)
+* Simplified error handling, mobservable will no longer catch errors in views, which makes the stack traces easier to debug.
+* Removed the initial 'welcom to mobservable' logline that was printed during start-up.
+
+# 0.7.1
+
+* Backported Babel support for the @observable decorator from the 1.0 branch. The decorator should now behave the same when compiled with either Typescript or Babeljs.
+
+# 0.7.0
+
+* Introduced `strict` mode (see issues [#30](), [#31]())
+* Renamed `sideEffect` to `observe`
+* Renamed `when` to `observeUntil`
+* Introduced `observeAsync`.
+* Fixed issue where changing the `logLevel` was not picked up.
+* Improved typings.
+* Introduces `asStructure` (see [#8]()) and `asFlat`.
+* Assigning a plain object to a reactive structure no longer clones the object, instead, the original object is decorated. (Arrays are still cloned due to Javascript limitations to extend arrays).
+* Reintroduced `expr(func)` as shorthand for `makeReactive(func)()`, which is useful to create temporarily views inside views
+* Deprecated the options object that could be passed to `makeReactive`.
+* Deprecated the options object that could be passed to `makeReactive`:
+ * A `thisArg` can be passed as second param.
+ * A name (for debugging) can be passed as second or third param
+ * The `as` modifier is no longer needed, use `asReference` (instead of `as:'reference'`) or `asFlat` (instead of `recurse:false`).
+
+# 0.6.10
+
+* Fixed issue where @observable did not properly create a stand-alone view
+
+# 0.6.9
+
+* Fixed bug where views where sometimes not triggered again if the dependency tree changed to much.
+
+# 0.6.8
+
+* Introduced `when`, which, given a reactive predicate, observes it until it returns true.
+* Renamed `sideEffect -> observe`
+
+# 0.6.7:
+
+* Improved logging
+
+# 0.6.6:
+
+* Deprecated observable array `.values()` and `.clone()`
+* Deprecated observeUntilInvalid; use sideEffect instead
+* Renamed mobservable.toJson to mobservable.toJSON
+
+# 0.6.5:
+
+* It is no longer possible to create impure views; views that alter other reactive values.
+* Update links to the new documentation.
+
+# 0.6.4:
+
+* 2nd argument of sideEffect is now the scope, instead of an options object which hadn't any useful properties
+
+# 0.6.3
+
+* Deprecated: reactiveComponent, reactiveComponent from the separate package mobservable-react should be used instead
+* Store the trackingstack globally, so that multiple instances of mobservable can run together
+
+# 0.6.2
+
+* Deprecated: @observable on functions (use getter functions instead)
+* Introduced: `getDependencyTree`, `getObserverTree` and `trackTransitions`
+* Minor performance improvements
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/LICENSE b/src/miniprogram-4/node_modules/mobx-miniprogram/LICENSE
new file mode 100644
index 0000000..b58beca
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2015 Michel Weststrate
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/README.md b/src/miniprogram-4/node_modules/mobx-miniprogram/README.md
new file mode 100644
index 0000000..9f0ed1a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/README.md
@@ -0,0 +1,453 @@
+# mobx-miniprogram
+
+可用于小程序的 MobX 构建版本。基于 MobX 4 (因为 iOS 9 不支持 MobX 5 )。
+
+`npm install mobx-miniprogram --save`
+
+以下是 MobX 原本的 README 。
+
+
+
+# MobX
+
+_Simple, scalable state management_
+
+[](https://circleci.com/gh/mobxjs/mobx/tree/mobx4-master)
+[](https://coveralls.io/github/mobxjs/mobx?branch=master)
+[](https://gitter.im/mobxjs/mobx?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
+[](https://hashnode.com/n/mobx)
+[](#backers)
+[](#sponsors)
+[](https://github.com/prettier/prettier)
+
+MobX is proudly sponsored by Mendix, Coinbase, Facebook Open Source and many [individual sponsors](#backers)
+
+
+
+# Installation
+
+* Installation: `npm install mobx --save`. React bindings: `npm install mobx-react --save`. To enable ESNext decorators (optional), see below.
+* CDN:
+- https://unpkg.com/mobx/lib/mobx.umd.js
+- https://cdnjs.com/libraries/mobx
+
+## Translations
+
+* [中文](http://cn.mobx.js.org)
+
+## Getting started
+
+* Egghead.io course
+* [Ten minute, interactive MobX + React tutorial](https://mobxjs.github.io/mobx/getting-started.html)
+* [Official MobX 4 documentation and API overview](https://mobxjs.github.io/mobx/refguide/api.html) ([MobX 3](https://github.com/mobxjs/mobx/blob/54557dc319b04e92e31cb87427bef194ec1c549c/docs/refguide/api.md), [MobX 2](https://github.com/mobxjs/mobx/blob/7c9e7c86e0c6ead141bb0539d33143d0e1f576dd/docs/refguide/api.md))
+* Videos:
+ * [ReactNext 2016: Real World MobX](https://www.youtube.com/watch?v=Aws40KOx90U) - 40m [slides](https://docs.google.com/presentation/d/1DrI6Hc2xIPTLBkfNH8YczOcPXQTOaCIcDESdyVfG_bE/edit?usp=sharing)
+ * [Practical React with MobX](https://www.youtube.com/watch?v=XGwuM_u7UeQ). In depth introduction and explanation to MobX and React by Matt Ruby on OpenSourceNorth (ES5 only) - 42m.
+ * LearnCode.academy MobX tutorial [Part I: MobX + React is AWESOME (7m)](https://www.youtube.com/watch?v=_q50BXqkAfI) [Part II: Computed Values and Nested/Referenced Observables (12m.)](https://www.youtube.com/watch?v=nYvNqKrl69s)
+ * [Screencast: intro to MobX](https://www.youtube.com/watch?v=K8dr8BMU7-8) - 8m
+ * [Talk: State Management Is Easy, React Amsterdam 2016 conf](https://www.youtube.com/watch?v=ApmSsu3qnf0&feature=youtu.be) ([slides](https://speakerdeck.com/mweststrate/state-management-is-easy-introduction-to-mobx))
+* [Boilerplates and related projects](http://mobxjs.github.io/mobx/faq/boilerplates.html)
+* More tutorials, blogs, videos, and other helpful resources can be found on the [MobX awesome list](https://github.com/mobxjs/awesome-mobx#awesome-mobx)
+
+
+## Introduction
+
+MobX is a battle tested library that makes state management simple and scalable by transparently applying functional reactive programming (TFRP).
+The philosophy behind MobX is very simple:
+
+_Anything that can be derived from the application state, should be derived. Automatically._
+
+which includes the UI, data serialization, server communication, etc.
+
+
+
+React and MobX together are a powerful combination. React renders the application state by providing mechanisms to translate it into a tree of renderable components. MobX provides the mechanism to store and update the application state that React then uses.
+
+Both React and MobX provide optimal and unique solutions to common problems in application development. React provides mechanisms to optimally render UI by using a virtual DOM that reduces the number of costly DOM mutations. MobX provides mechanisms to optimally synchronize application state with your React components by using a reactive virtual dependency state graph that is only updated when strictly needed and is never stale.
+
+## Core concepts
+
+MobX has only a few core concepts. The following snippets can be tried online using [codesandbox example](https://codesandbox.io/s/v3v0my2370).
+
+### Observable state
+
+Egghead.io lesson 1: observable & observer
+
+MobX adds observable capabilities to existing data structures like objects, arrays and class instances.
+This can simply be done by annotating your class properties with the [@observable](http://mobxjs.github.io/mobx/refguide/observable-decorator.html) decorator (ES.Next).
+
+```javascript
+import { observable } from "mobx"
+
+class Todo {
+ id = Math.random();
+ @observable title = "";
+ @observable finished = false;
+}
+```
+
+Using `observable` is like turning a property of an object into a spreadsheet cell.
+But unlike spreadsheets, these values can be not only primitive values, but also references, objects and arrays.
+
+If your environment doesn't support decorator syntax, don't worry.
+You can read [here](http://mobxjs.github.io/mobx/best/decorators.html) about how to set them up.
+Or you can skip them altoghether, as MobX can be used fine without decorator _syntax_, by leveraging the _decorate_ utility.
+Many MobX users do prefer the decorator syntax though, as it is slightly more concise.
+
+```javascript
+import { decorate, observable } from "mobx"
+
+class Todo {
+ id = Math.random();
+ title = "";
+ finished = false;
+}
+decorate(Todo, {
+ title: observable,
+ finished: observable
+})
+```
+
+### Computed values
+
+Egghead.io lesson 3: computed values
+
+With MobX you can define values that will be derived automatically when relevant data is modified.
+By using the [`@computed`](http://mobxjs.github.io/mobx/refguide/computed-decorator.html) decorator or by using getter / setter functions when using `(extend)Observable` (Of course, you can use `decorate` here again as alternative to the `@` syntax).
+
+```javascript
+class TodoList {
+ @observable todos = [];
+ @computed get unfinishedTodoCount() {
+ return this.todos.filter(todo => !todo.finished).length;
+ }
+}
+```
+
+MobX will ensure that `unfinishedTodoCount` is updated automatically when a todo is added or when one of the `finished` properties is modified.
+Computations like these resemble formulas in spreadsheet programs like MS Excel. They update automatically and only when required.
+
+### Reactions
+
+Egghead.io lesson 9: custom reactions
+
+Reactions are similar to a computed value, but instead of producing a new value, a reaction produces a side effect for things like printing to the console, making network requests, incrementally updating the React component tree to patch the DOM, etc.
+In short, reactions bridge [reactive](https://en.wikipedia.org/wiki/Reactive_programming) and [imperative](https://en.wikipedia.org/wiki/Imperative_programming) programming.
+
+#### React components
+
+Egghead.io lesson 1: observable & observer
+
+If you are using React, you can turn your (stateless function) components into reactive components by simply adding the [`observer`](http://mobxjs.github.io/mobx/refguide/observer-component.html) function / decorator from the `mobx-react` package onto them.
+
+```javascript
+import React, {Component} from 'react';
+import ReactDOM from 'react-dom';
+import {observer} from 'mobx-react';
+
+@observer
+class TodoListView extends Component {
+ render() {
+ return
+
+ {this.props.todoList.todos.map(todo =>
+
+ )}
+
+ Tasks left: {this.props.todoList.unfinishedTodoCount}
+
+ }
+}
+
+const TodoView = observer(({todo}) =>
+
+ todo.finished = !todo.finished}
+ />{todo.title}
+
+)
+
+const store = new TodoList();
+ReactDOM.render(, document.getElementById('mount'));
+```
+
+`observer` turns React (function) components into derivations of the data they render.
+When using MobX there are no smart or dumb components.
+All components render smartly but are defined in a dumb manner. MobX will simply make sure the components are always re-rendered whenever needed, but also no more than that. So the `onClick` handler in the above example will force the proper `TodoView` to render, and it will cause the `TodoListView` to render if the number of unfinished tasks has changed.
+However, if you would remove the `Tasks left` line (or put it into a separate component), the `TodoListView` will no longer re-render when ticking a box. You can verify this yourself by changing the [JSFiddle](https://jsfiddle.net/mweststrate/wv3yopo0/).
+
+#### Custom reactions
+Custom reactions can simply be created using the [`autorun`](http://mobxjs.github.io/mobx/refguide/autorun.html),
+[`reaction`](http://mobxjs.github.io/mobx/refguide/reaction.html) or [`when`](http://mobxjs.github.io/mobx/refguide/when.html) functions to fit your specific situations.
+
+For example the following `autorun` prints a log message each time the amount of `unfinishedTodoCount` changes:
+
+```javascript
+autorun(() => {
+ console.log("Tasks left: " + todos.unfinishedTodoCount)
+})
+```
+
+### What will MobX react to?
+
+Why does a new message get printed each time the `unfinishedTodoCount` is changed? The answer is this rule of thumb:
+
+_MobX reacts to any existing observable property that is read during the execution of a tracked function._
+
+For an in-depth explanation about how MobX determines to which observables needs to be reacted, check [understanding what MobX reacts to](https://github.com/mobxjs/mobx/blob/gh-pages/docs/best/react.md).
+
+### Actions
+
+Egghead.io lesson 5: actions
+
+Unlike many flux frameworks, MobX is unopinionated about how user events should be handled.
+
+* This can be done in a Flux like manner.
+* Or by processing events using RxJS.
+* Or by simply handling events in the most straightforward way possible, as demonstrated in the above `onClick` handler.
+
+In the end it all boils down to: Somehow the state should be updated.
+
+After updating the state `MobX` will take care of the rest in an efficient, glitch-free manner. So simple statements, like below, are enough to automatically update the user interface.
+
+There is no technical need for firing events, calling a dispatcher or what more. A React component in the end is nothing more than a fancy representation of your state. A derivation that will be managed by MobX.
+
+```javascript
+store.todos.push(
+ new Todo("Get Coffee"),
+ new Todo("Write simpler code")
+);
+store.todos[0].finished = true;
+```
+
+Nonetheless, MobX has an optional built-in concept of [`actions`](https://mobxjs.github.io/mobx/refguide/action.html).
+Read this section as well if you want to know more about writing asynchronous actions. It's easy!
+Use them to your advantage; they will help you to structure your code better and make wise decisions about when and where state should be modified.
+
+## MobX: Simple and scalable
+
+MobX is one of the least obtrusive libraries you can use for state management. That makes the `MobX` approach not just simple, but very scalable as well:
+
+### Using classes and real references
+
+With MobX you don't need to normalize your data. This makes the library very suitable for very complex domain models (At Mendix for example ~500 different domain classes in a single application).
+
+### Referential integrity is guaranteed
+
+Since data doesn't need to be normalized, and MobX automatically tracks the relations between state and derivations, you get referential integrity for free. Rendering something that is accessed through three levels of indirection?
+
+No problem, MobX will track them and re-render whenever one of the references changes. As a result staleness bugs are a thing of the past. As a programmer you might forget that changing some data might influence a seemingly unrelated component in a corner case. MobX won't forget.
+
+### Simpler actions are easier to maintain
+
+As demonstrated above, modifying state when using MobX is very straightforward. You simply write down your intentions. MobX will take care of the rest.
+
+### Fine grained observability is efficient
+
+MobX builds a graph of all the derivations in your application to find the least number of re-computations that is needed to prevent staleness. "Derive everything" might sound expensive, MobX builds a virtual derivation graph to minimize the number of recomputations needed to keep derivations in sync with the state.
+
+In fact, when testing MobX at Mendix we found out that using this library to track the relations in our code is often a lot more efficient than pushing changes through our application by using handwritten events or "smart" selector based container components.
+
+The simple reason is that MobX will establish far more fine grained 'listeners' on your data than you would do as a programmer.
+
+Secondly MobX sees the causality between derivations so it can order them in such a way that no derivation has to run twice or introduces a glitch.
+
+How that works? See this [in-depth explanation of MobX](https://medium.com/@mweststrate/becoming-fully-reactive-an-in-depth-explanation-of-mobservable-55995262a254).
+
+### Easy interoperability
+
+MobX works with plain javascript structures. Due to its unobtrusiveness it works with most javascript libraries out of the box, without needing MobX specific library flavors.
+
+So you can simply keep using your existing router, data fetching, and utility libraries like `react-router`, `director`, `superagent`, `lodash` etc.
+
+For the same reason you can use it out of the box both server and client side, in isomorphic applications and with react-native.
+
+The result of this is that you often need to learn less new concepts when using MobX in comparison to other state management solutions.
+
+---
+
+
+## Credits
+
+MobX is inspired by reactive programming principles as found in spreadsheets. It is inspired by MVVM frameworks like in MeteorJS tracker, knockout and Vue.js. But MobX brings Transparent Functional Reactive Programming to the next level and provides a stand alone implementation. It implements TFRP in a glitch-free, synchronous, predictable and efficient manner.
+
+A ton of credits for [Mendix](https://github.com/mendix), for providing the flexibility and support to maintain MobX and the chance to proof the philosophy of MobX in a real, complex, performance critical applications.
+
+And finally kudos for all the people that believed in, tried, validated and even [sponsored](https://github.com/mobxjs/mobx/blob/master/sponsors.md) MobX.
+
+## Further resources and documentation
+
+* [MobX homepage](http://mobxjs.github.io/mobx/faq/blogs.html)
+* [API overview](http://mobxjs.github.io/mobx/refguide/api.html)
+* [Tutorials, Blogs & Videos](http://mobxjs.github.io/mobx/faq/blogs.html)
+* [Boilerplates](http://mobxjs.github.io/mobx/faq/boilerplates.html)
+* [Related projects](http://mobxjs.github.io/mobx/faq/related.html)
+
+
+## What others are saying...
+
+> Guise, #mobx isn't pubsub, or your grandpa's observer pattern. Nay, it is a carefully orchestrated observable dimensional portal fueled by the power cosmic. It doesn't do change detection, it's actually a level 20 psionic with soul knife, slashing your viewmodel into submission.
+
+> After using #mobx for lone projects for a few weeks, it feels awesome to introduce it to the team. Time: 1/2, Fun: 2X
+
+> Working with #mobx is basically a continuous loop of me going “this is way too simple, it definitely won’t work” only to be proven wrong
+
+> Try react-mobx with es6 and you will love it so much that you will hug someone.
+
+> I have built big apps with MobX already and comparing to the one before that which was using Redux, it is simpler to read and much easier to reason about.
+
+> The #mobx is the way I always want things to be! It's really surprising simple and fast! Totally awesome! Don't miss it!
+
+## Contributing
+
+* Feel free to send small pull requests. Please discuss new features or big changes in a GitHub issue first.
+* Use `npm test` to run the basic test suite, `npm run coverage` for the test suite with coverage and `npm run perf` for the performance tests.
+
+## Flow support
+MobX ships with [flow typings](flow-typed/mobx.js). Flow will automatically include them when you import mobx modules. Although you **do not** need to import the types explicitly, you can still do it like this: `import type { ... } from 'mobx'`.
+
+To use the [flow typings](flow-typed/mobx.js) shipped with MobX:
+
+* In `.flowconfig`, you **cannot** ignore `node_modules`.
+* In `.flowconfig`, you **cannot** import it explicitly in the `[libs]` section.
+* You **do not** need to install library definition using [flow-typed](https://github.com/flowtype/flow-typed).
+
+## Donating
+
+Was MobX key in making your project a success?
+Join our [open collective](https://opencollective.com/mobx#) or use the [donate button](https://mobxjs.github.io/mobx/donate.html)!
+
+### Backers
+Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/mobx#backer)]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+One time donations through paypal are welcome as well and are recorded in the [sponsors](sponsors.md) list.
+
+[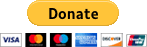](https://mobxjs.github.io/mobx/donate.html)
+
+### Sponsors
+
+Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/mobx#sponsor)]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/action.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/action.d.ts
new file mode 100644
index 0000000..c83b4a0
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/action.d.ts
@@ -0,0 +1,25 @@
+import { IAction } from "../internal";
+export interface IActionFactory {
+ R>(fn: T): T & IAction;
+ R>(fn: T): T & IAction;
+ R>(fn: T): T & IAction;
+ R>(fn: T): T & IAction;
+ R>(fn: T): T & IAction;
+ R>(fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ R>(name: string, fn: T): T & IAction;
+ (fn: T): T & IAction;
+ (name: string, fn: T): T & IAction;
+ (customName: string): (target: Object, key: string | symbol, baseDescriptor?: PropertyDescriptor) => void;
+ (target: Object, propertyKey: string | symbol, descriptor?: PropertyDescriptor): void;
+ bound(target: Object, propertyKey: string | symbol, descriptor?: PropertyDescriptor): void;
+}
+export declare const action: IActionFactory;
+export declare function runInAction(block: () => T): T;
+export declare function runInAction(name: string, block: () => T): T;
+export declare function isAction(thing: any): boolean;
+export declare function defineBoundAction(target: any, propertyName: string, fn: Function): void;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/actiondecorator.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/actiondecorator.d.ts
new file mode 100644
index 0000000..7cd708a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/actiondecorator.d.ts
@@ -0,0 +1,16 @@
+import { BabelDescriptor } from "../internal";
+declare function dontReassignFields(): void;
+export declare function namedActionDecorator(name: string): (target: any, prop: any, descriptor: BabelDescriptor) => any;
+export declare function actionFieldDecorator(name: string): (target: any, prop: any, descriptor: any) => void;
+export declare function boundActionDecorator(target: any, propertyName: any, descriptor: any, applyToInstance?: boolean): {
+ configurable: boolean;
+ enumerable: boolean;
+ get(): any;
+ set: typeof dontReassignFields;
+} | {
+ enumerable: boolean;
+ configurable: boolean;
+ set(v: any): void;
+ get(): undefined;
+} | null;
+export {};
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/autorun.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/autorun.d.ts
new file mode 100644
index 0000000..2cd7182
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/autorun.d.ts
@@ -0,0 +1,19 @@
+import { IReactionPublic, IReactionDisposer, IEqualsComparer } from "../internal";
+export interface IAutorunOptions {
+ delay?: number;
+ name?: string;
+ scheduler?: (callback: () => void) => any;
+ onError?: (error: any) => void;
+}
+/**
+ * Creates a named reactive view and keeps it alive, so that the view is always
+ * updated if one of the dependencies changes, even when the view is not further used by something else.
+ * @param view The reactive view
+ * @returns disposer function, which can be used to stop the view from being updated in the future.
+ */
+export declare function autorun(view: (r: IReactionPublic) => any, opts?: IAutorunOptions): IReactionDisposer;
+export declare type IReactionOptions = IAutorunOptions & {
+ fireImmediately?: boolean;
+ equals?: IEqualsComparer;
+};
+export declare function reaction(expression: (r: IReactionPublic) => T, effect: (arg: T, r: IReactionPublic) => void, opts?: IReactionOptions): IReactionDisposer;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/become-observed.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/become-observed.d.ts
new file mode 100644
index 0000000..a4f1e60
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/become-observed.d.ts
@@ -0,0 +1,5 @@
+import { IObservableArray, IObservable, IComputedValue, ObservableMap, ObservableSet, Lambda } from "../internal";
+export declare function onBecomeObserved(value: IObservable | IComputedValue | IObservableArray | ObservableMap | ObservableSet, listener: Lambda): Lambda;
+export declare function onBecomeObserved(value: ObservableMap | Object, property: K, listener: Lambda): Lambda;
+export declare function onBecomeUnobserved(value: IObservable | IComputedValue | IObservableArray | ObservableMap | ObservableSet, listener: Lambda): Lambda;
+export declare function onBecomeUnobserved(value: ObservableMap | Object, property: K, listener: Lambda): Lambda;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/computed.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/computed.d.ts
new file mode 100644
index 0000000..7666069
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/computed.d.ts
@@ -0,0 +1,14 @@
+import { IComputedValueOptions, IComputedValue } from "../internal";
+export interface IComputed {
+ (options: IComputedValueOptions): any;
+ (func: () => T, setter: (v: T) => void): IComputedValue;
+ (func: () => T, options?: IComputedValueOptions): IComputedValue;
+ (target: Object, key: string | symbol, baseDescriptor?: PropertyDescriptor): void;
+ struct(target: Object, key: string | symbol, baseDescriptor?: PropertyDescriptor): void;
+}
+export declare const computedDecorator: Function;
+/**
+ * Decorator for class properties: @computed get value() { return expr; }.
+ * For legacy purposes also invokable as ES5 observable created: `computed(() => expr)`;
+ */
+export declare const computed: IComputed;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/configure.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/configure.d.ts
new file mode 100644
index 0000000..65fe386
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/configure.d.ts
@@ -0,0 +1,9 @@
+export declare function configure(options: {
+ enforceActions?: boolean | "strict" | "never" | "always" | "observed";
+ computedRequiresReaction?: boolean;
+ computedConfigurable?: boolean;
+ isolateGlobalState?: boolean;
+ disableErrorBoundaries?: boolean;
+ arrayBuffer?: number;
+ reactionScheduler?: (f: () => void) => void;
+}): void;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/decorate.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/decorate.d.ts
new file mode 100644
index 0000000..ebf5551
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/decorate.d.ts
@@ -0,0 +1,6 @@
+export declare function decorate(clazz: new (...args: any[]) => T, decorators: {
+ [P in keyof T]?: MethodDecorator | PropertyDecorator | Array | Array;
+}): void;
+export declare function decorate(object: T, decorators: {
+ [P in keyof T]?: MethodDecorator | PropertyDecorator | Array | Array;
+}): T;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extendobservable.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extendobservable.d.ts
new file mode 100644
index 0000000..bb374fa
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extendobservable.d.ts
@@ -0,0 +1,7 @@
+import { CreateObservableOptions } from "../internal";
+export declare function extendShallowObservable(target: A, properties: B, decorators?: {
+ [K in keyof B]?: Function;
+}): A & B;
+export declare function extendObservable(target: A, properties: B, decorators?: {
+ [K in keyof B]?: Function;
+}, options?: CreateObservableOptions): A & B;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extras.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extras.d.ts
new file mode 100644
index 0000000..929fda6
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/extras.d.ts
@@ -0,0 +1,10 @@
+export interface IDependencyTree {
+ name: string;
+ dependencies?: IDependencyTree[];
+}
+export interface IObserverTree {
+ name: string;
+ observers?: IObserverTree[];
+}
+export declare function getDependencyTree(thing: any, property?: string): IDependencyTree;
+export declare function getObserverTree(thing: any, property?: string): IObserverTree;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/flow.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/flow.d.ts
new file mode 100644
index 0000000..7d0b166
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/flow.d.ts
@@ -0,0 +1,12 @@
+export declare type CancellablePromise = Promise & {
+ cancel(): void;
+};
+export interface FlowYield {
+ "!!flowYield": undefined;
+}
+export interface FlowReturn {
+ "!!flowReturn": T;
+}
+export declare type FlowReturnType = IfAllAreFlowYieldThenVoid ? FR extends Promise ? FRP : FR : R extends Promise ? FlowYield : R>;
+export declare type IfAllAreFlowYieldThenVoid = Exclude extends never ? void : Exclude;
+export declare function flow(generator: (...args: Args) => IterableIterator): (...args: Args) => CancellablePromise>;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept-read.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept-read.d.ts
new file mode 100644
index 0000000..bceda0a
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept-read.d.ts
@@ -0,0 +1,8 @@
+import { Lambda, IObservableValue, IObservableArray, ObservableMap, ObservableSet } from "../internal";
+export declare type ReadInterceptor = (value: any) => T;
+/** Experimental feature right now, tested indirectly via Mobx-State-Tree */
+export declare function interceptReads(value: IObservableValue, handler: ReadInterceptor): Lambda;
+export declare function interceptReads(observableArray: IObservableArray, handler: ReadInterceptor): Lambda;
+export declare function interceptReads(observableMap: ObservableMap, handler: ReadInterceptor): Lambda;
+export declare function interceptReads(observableSet: ObservableSet, handler: ReadInterceptor): Lambda;
+export declare function interceptReads(object: Object, property: string, handler: ReadInterceptor): Lambda;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept.d.ts
new file mode 100644
index 0000000..4828539
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/intercept.d.ts
@@ -0,0 +1,8 @@
+import { IInterceptor, IValueWillChange, IObservableValue, Lambda, IObservableArray, IArrayWillChange, IArrayWillSplice, ObservableMap, IMapWillChange, ObservableSet, ISetWillChange, IObjectWillChange } from "../internal";
+export declare function intercept(value: IObservableValue, handler: IInterceptor>): Lambda;
+export declare function intercept(observableArray: IObservableArray, handler: IInterceptor | IArrayWillSplice>): Lambda;
+export declare function intercept(observableMap: ObservableMap, handler: IInterceptor>): Lambda;
+export declare function intercept(observableMap: ObservableSet, handler: IInterceptor>): Lambda;
+export declare function intercept(observableMap: ObservableMap, property: K, handler: IInterceptor>): Lambda;
+export declare function intercept(object: Object, handler: IInterceptor): Lambda;
+export declare function intercept(object: T, property: K, handler: IInterceptor>): Lambda;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/iscomputed.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/iscomputed.d.ts
new file mode 100644
index 0000000..9d28a33
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/iscomputed.d.ts
@@ -0,0 +1,3 @@
+export declare function _isComputed(value: any, property?: string): boolean;
+export declare function isComputed(value: any): boolean;
+export declare function isComputedProp(value: any, propName: string): boolean;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/isobservable.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/isobservable.d.ts
new file mode 100644
index 0000000..c15deed
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/isobservable.d.ts
@@ -0,0 +1,2 @@
+export declare function isObservable(value: any): boolean;
+export declare function isObservableProp(value: any, propName: string): boolean;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/object-api.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/object-api.d.ts
new file mode 100644
index 0000000..7f9dba5
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/object-api.d.ts
@@ -0,0 +1,34 @@
+import { ObservableMap, IObservableArray, ObservableSet } from "../internal";
+export declare function keys(map: ObservableMap): ReadonlyArray;
+export declare function keys(ar: IObservableArray): ReadonlyArray;
+export declare function keys(set: ObservableSet): ReadonlyArray;
+export declare function keys(obj: T): ReadonlyArray;
+export declare function values(map: ObservableMap): ReadonlyArray;
+export declare function values(set: ObservableSet): ReadonlyArray;
+export declare function values(ar: IObservableArray): ReadonlyArray;
+export declare function values(obj: T): ReadonlyArray;
+export declare function entries(map: ObservableMap): ReadonlyArray<[K, T]>;
+export declare function entries(set: ObservableSet): ReadonlyArray<[T, T]>;
+export declare function entries(ar: IObservableArray): ReadonlyArray<[number, T]>;
+export declare function entries(obj: T): ReadonlyArray<[string, any]>;
+export declare function set(obj: ObservableMap, values: {
+ [key: string]: V;
+}): any;
+export declare function set(obj: ObservableMap, key: K, value: V): any;
+export declare function set(obj: ObservableSet, value: T): any;
+export declare function set(obj: IObservableArray, index: number, value: T): any;
+export declare function set(obj: T, values: {
+ [key: string]: any;
+}): any;
+export declare function set(obj: T, key: string, value: any): any;
+export declare function remove(obj: ObservableMap, key: K): any;
+export declare function remove(obj: ObservableSet, key: T): any;
+export declare function remove(obj: IObservableArray, index: number): any;
+export declare function remove(obj: T, key: string): any;
+export declare function has(obj: ObservableMap, key: K): boolean;
+export declare function has(obj: ObservableSet, key: T): boolean;
+export declare function has(obj: IObservableArray, index: number): boolean;
+export declare function has(obj: T, key: string): boolean;
+export declare function get(obj: ObservableMap, key: K): V | undefined;
+export declare function get(obj: IObservableArray, index: number): T | undefined;
+export declare function get(obj: T, key: string): any;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observable.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observable.d.ts
new file mode 100644
index 0000000..a51d49f
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observable.d.ts
@@ -0,0 +1,54 @@
+import { IEqualsComparer, IObservableDecorator, IEnhancer, IObservableArray, ObservableMap, IObservableObject, IObservableValue, IObservableSetInitialValues, ObservableSet, IObservableMapInitialValues } from "../internal";
+export declare type CreateObservableOptions = {
+ name?: string;
+ equals?: IEqualsComparer;
+ deep?: boolean;
+ defaultDecorator?: IObservableDecorator;
+};
+export declare const defaultCreateObservableOptions: CreateObservableOptions;
+export declare const shallowCreateObservableOptions: {
+ deep: boolean;
+ name: undefined;
+ defaultDecorator: undefined;
+};
+export declare function asCreateObservableOptions(thing: any): CreateObservableOptions;
+export declare const deepDecorator: IObservableDecorator;
+export declare const refDecorator: IObservableDecorator;
+export interface IObservableFactory {
+ (value: number | string | null | undefined | boolean): never;
+ (target: Object, key: string | symbol, baseDescriptor?: PropertyDescriptor): any;
+ (value: T[], options?: CreateObservableOptions): IObservableArray;
+ (value: Set, options?: CreateObservableOptions): ObservableSet;
+ (value: Map, options?: CreateObservableOptions): ObservableMap;
+ (value: T, decorators?: {
+ [K in keyof T]?: Function;
+ }, options?: CreateObservableOptions): T & IObservableObject;
+}
+export interface IObservableFactories {
+ box(value?: T, options?: CreateObservableOptions): IObservableValue;
+ shallowBox(value?: T, options?: CreateObservableOptions): IObservableValue;
+ array(initialValues?: T[], options?: CreateObservableOptions): IObservableArray;
+ shallowArray(initialValues?: T[], options?: CreateObservableOptions): IObservableArray;
+ set(initialValues?: IObservableSetInitialValues, options?: CreateObservableOptions): ObservableSet;
+ map(initialValues?: IObservableMapInitialValues, options?: CreateObservableOptions): ObservableMap;
+ shallowMap(initialValues?: IObservableMapInitialValues, options?: CreateObservableOptions): ObservableMap;
+ object(props: T, decorators?: {
+ [K in keyof T]?: Function;
+ }, options?: CreateObservableOptions): T & IObservableObject;
+ shallowObject(props: T, decorators?: {
+ [K in keyof T]?: Function;
+ }, options?: CreateObservableOptions): T & IObservableObject;
+ /**
+ * Decorator that creates an observable that only observes the references, but doesn't try to turn the assigned value into an observable.ts.
+ */
+ ref: IObservableDecorator;
+ /**
+ * Decorator that creates an observable converts its value (objects, maps or arrays) into a shallow observable structure
+ */
+ shallow: IObservableDecorator;
+ deep: IObservableDecorator;
+ struct: IObservableDecorator;
+}
+export declare const observable: IObservableFactory & IObservableFactories & {
+ enhancer: IEnhancer;
+};
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observabledecorator.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observabledecorator.d.ts
new file mode 100644
index 0000000..c2b8ace
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observabledecorator.d.ts
@@ -0,0 +1,6 @@
+import { IEnhancer } from "../internal";
+export declare type IObservableDecorator = {
+ (target: Object, property: string | symbol, descriptor?: PropertyDescriptor): void;
+ enhancer: IEnhancer;
+};
+export declare function createDecoratorForEnhancer(enhancer: IEnhancer): IObservableDecorator;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observe.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observe.d.ts
new file mode 100644
index 0000000..cdf996c
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/observe.d.ts
@@ -0,0 +1,8 @@
+import { IObservableArray, IArrayChange, IArraySplice, IObservableValue, IComputedValue, IValueDidChange, Lambda, ObservableSet, ISetDidChange, ObservableMap, IMapDidChange, IObjectDidChange } from "../internal";
+export declare function observe(value: IObservableValue | IComputedValue, listener: (change: IValueDidChange) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(observableArray: IObservableArray, listener: (change: IArrayChange | IArraySplice) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(observableMap: ObservableSet, listener: (change: ISetDidChange) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(observableMap: ObservableMap, listener: (change: IMapDidChange) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(observableMap: ObservableMap, property: K, listener: (change: IValueDidChange) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(object: Object, listener: (change: IObjectDidChange) => void, fireImmediately?: boolean): Lambda;
+export declare function observe(object: T, property: K, listener: (change: IValueDidChange) => void, fireImmediately?: boolean): Lambda;
diff --git a/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/tojs.d.ts b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/tojs.d.ts
new file mode 100644
index 0000000..38ba7dc
--- /dev/null
+++ b/src/miniprogram-4/node_modules/mobx-miniprogram/lib/api/tojs.d.ts
@@ -0,0 +1,11 @@
+export declare type ToJSOptions = {
+ detectCycles?: boolean;
+ exportMapsAsObjects?: boolean;
+ recurseEverything?: boolean;
+};
+/**
+ * Basically, a deep clone, so that no reactive property will exist anymore.
+ */
+export declare function toJS